To plot a single point in MATLAB, you can use the `plot` function by specifying the x and y coordinates of the point. Here’s a simple example:
plot(2, 3, 'ro'); % Plots a red circle at the point (2, 3)
Understanding Basic Plotting in MATLAB
What is Plotting?
Plotting is the graphical representation of data. It allows you to visualize relationships and trends in the data, making it easier to understand complex information at a glance. In MATLAB, plotting is a fundamental capability extensively used in data analysis, enabling users to create visualizations to present their findings effectively.
Key Functions in MATLAB for Plotting
MATLAB provides a range of functions for plotting, with the most common being `plot`, `scatter`, and `bar`. These functions serve different purposes but are all designed to help you visualize data points in a clear and informative manner. To plot a point in MATLAB, the `plot` function is the primary tool utilized.
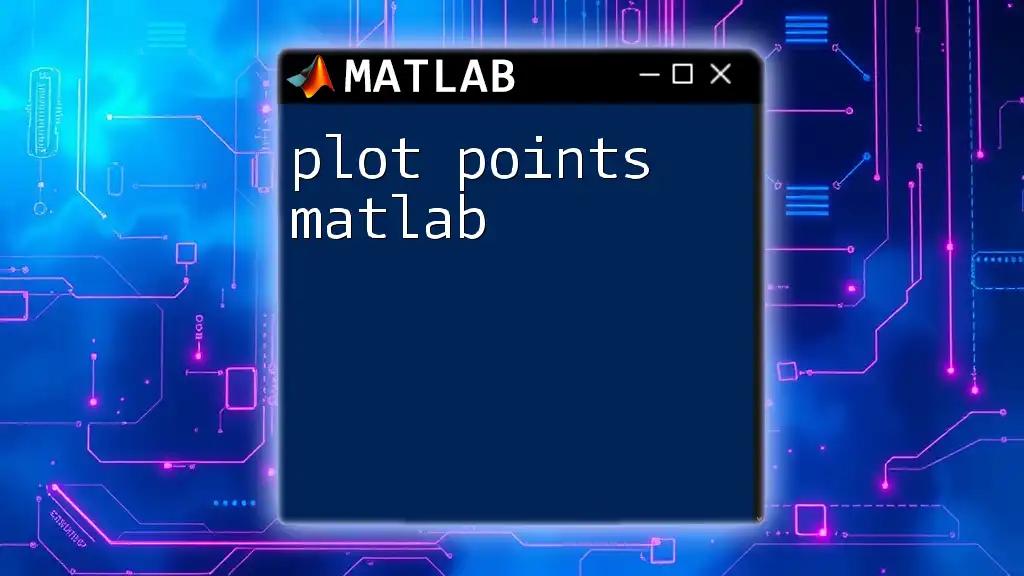
Setting Up MATLAB Environment
Installing MATLAB
To begin using MATLAB, you'll first need to install it. You can find the installation guidelines on the MathWorks website. After installation, your MATLAB environment will be ready for use!
Understanding the MATLAB Workspace
Once MATLAB is up and running, you'll see its interface consisting of various components, such as the Command Window and Editor. The workspace holds all the variables you've defined, and understanding your workspace is critical to executing commands and plotting effectively.
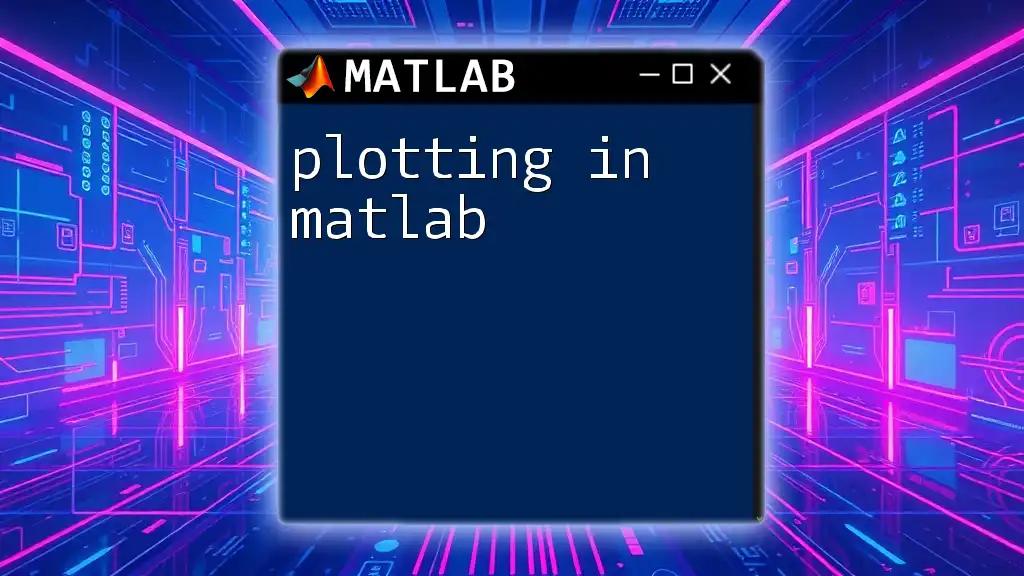
Plotting a Single Point
Preparing the Data
Before you can plot a point, you need to prepare your data. Typically, you'll use coordinates to represent the point in a Cartesian plane. For a 2D plot, you'll have an X value and a Y value—together, they define a specific location on the graph.
Using the `plot` Function
The `plot` function in MATLAB is powerful and easy to use for plotting points.
Basic Syntax of the `plot` function is:
plot(x, y, 'MarkerStyle')
Here, `MarkerStyle` specifies how the point looks.
Example 1: Let's plot a single point at coordinates (5, 10).
x = 5;
y = 10;
plot(x, y, 'ro'); % Red circle
title('Plotting a Single Point');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
In this example, the `plot` command takes `x` and `y` as inputs, and the 'ro' argument specifies that we want to display this point as a red circle. The title, labels, and grid are also added for better visualization.
Customizing the Point
Changing Marker Style
MATLAB offers various marker styles to represent points. Some commonly used markers include:
- `'o'` for circles
- `'x'` for crosses
- `'+'` for plus signs
Example 2: Plotting multiple points with different styles.
x = [1, 2, 5];
y = [3, 4, 10];
plot(x, y, 'g*'); % Green star
hold on; % Retain current graph
plot(2, 4, 'bs'); % Blue square for a single point
title('Multiple Points with Different Styles');
grid on;
legend('Data Points', 'Single Point');
In this example, we plot multiple points using a green star while also using a blue square to highlight a specific point. The `hold on` command allows us to add new plots to the existing graph without overwriting it.
Adding Annotations
Annotations can enhance the clarity of your plots. They provide additional context for the points you plot.
Example 3: Adding text labels to points.
text(5, 10, 'Point (5,10)', 'VerticalAlignment', 'bottom');
In this example, the `text` function places a label at the point (5,10), helping viewers identify its significance.
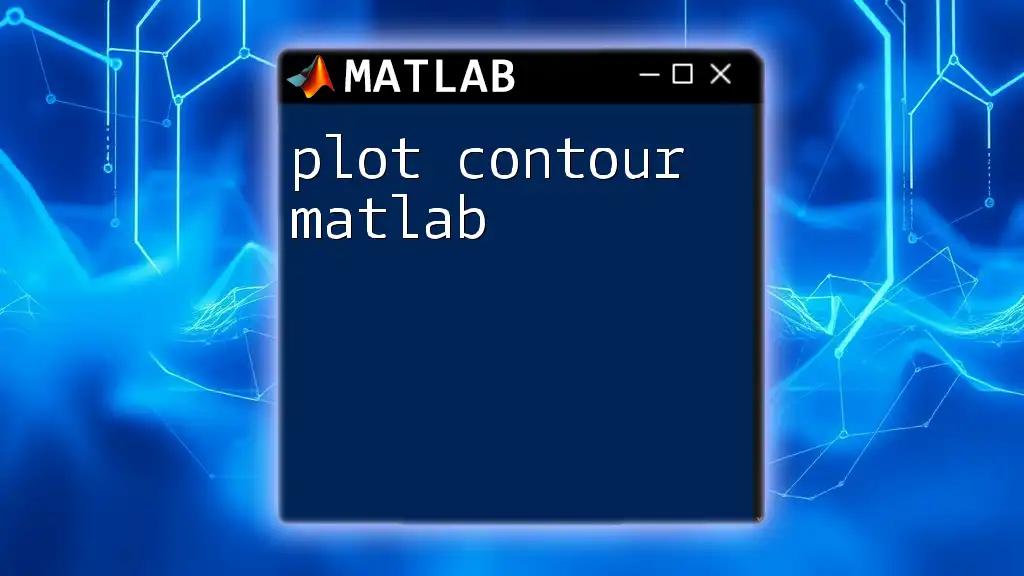
Plotting Points in 3D
Introduction to 3D Plotting
Visualizing data in three dimensions can provide insights that 2D plots cannot. 3D plotting is essential for data involving three variables, allowing for better assessment of relationships among multiple datasets.
Using `plot3` Function
To plot points in a 3D space, MATLAB uses the `plot3` function.
Basic Syntax for 3D Plotting is:
plot3(x, y, z, 'MarkerStyle')
Example 4: Let's plot a point in 3D at coordinates (3, 4, 5).
x = 3;
y = 4;
z = 5;
plot3(x, y, z, 'bo');
title('3D Point Plotting');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
grid on;
In this example, `plot3` allows us to visualize a point in 3D space, using a blue circle to indicate its location. We also label the axes for clarity.
Customizing 3D Points
Customizing 3D plots can make them more informative. You can change the view angles and manipulate point markers for better visualization.
Example 5: Changing the view angle to improve aesthetics.
view(45, 30); % Set view angle
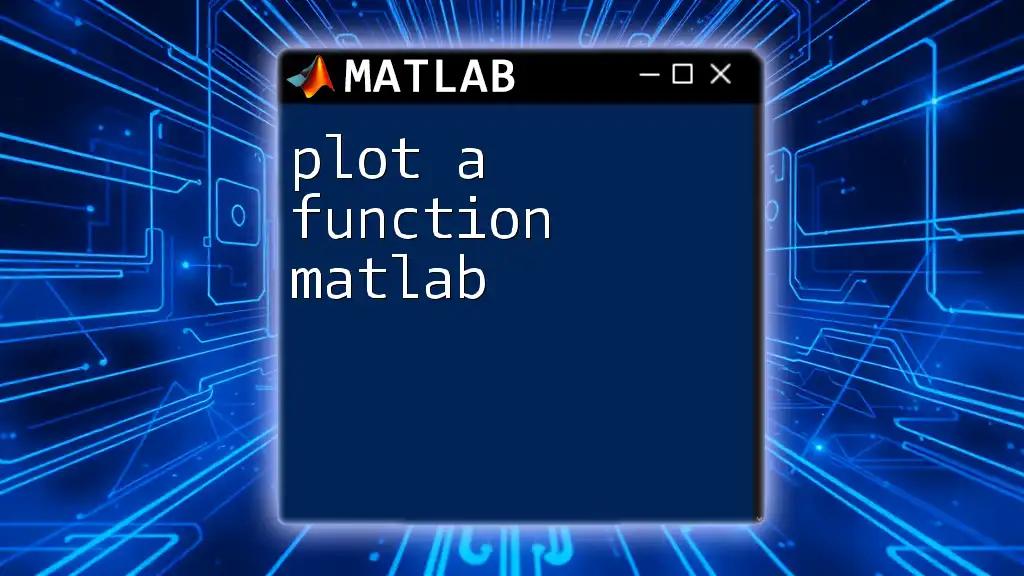
Troubleshooting Common Issues
Common Errors and How to Fix Them
It’s not uncommon to run into issues when plotting in MATLAB. Common errors include:
- Undefined function errors, usually due to typos or missing data points.
- Incorrect axes when markers are plotted outside of the defined range.
Verifying Your Plot
Always inspect your plot to ensure points are plotted correctly. You can access properties of the current axes using `gca`, which helps verify settings like limits and visibility.
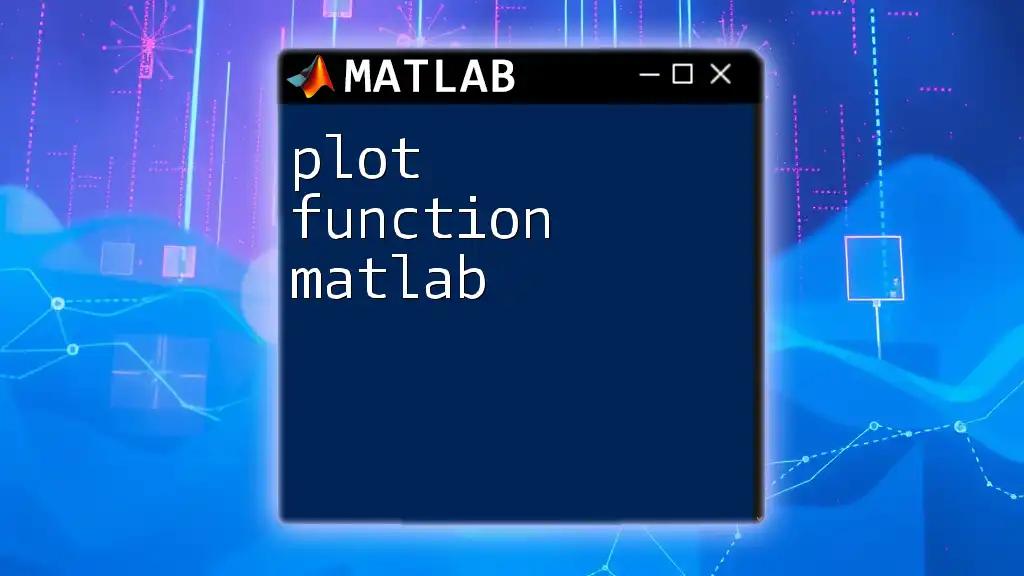
Advanced Topics
Combining Multiple Points into One Plot
You can plot multiple points in a single command by utilizing vectors or matrices.
Example 6: Plotting a series of points.
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 7, 11];
plot(x, y, 'r*'); % Multiple red star markers
title('Multiple Points in a Single Plot');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Saving Your Plot
After you’ve created a visually appealing plot, you might want to save it. MATLAB provides several options to save plots as files.
Example of saving figures:
saveas(gcf, 'myPlot.png');
This command will save the current figure (denoted by `gcf`—Get Current Figure) as a PNG image.
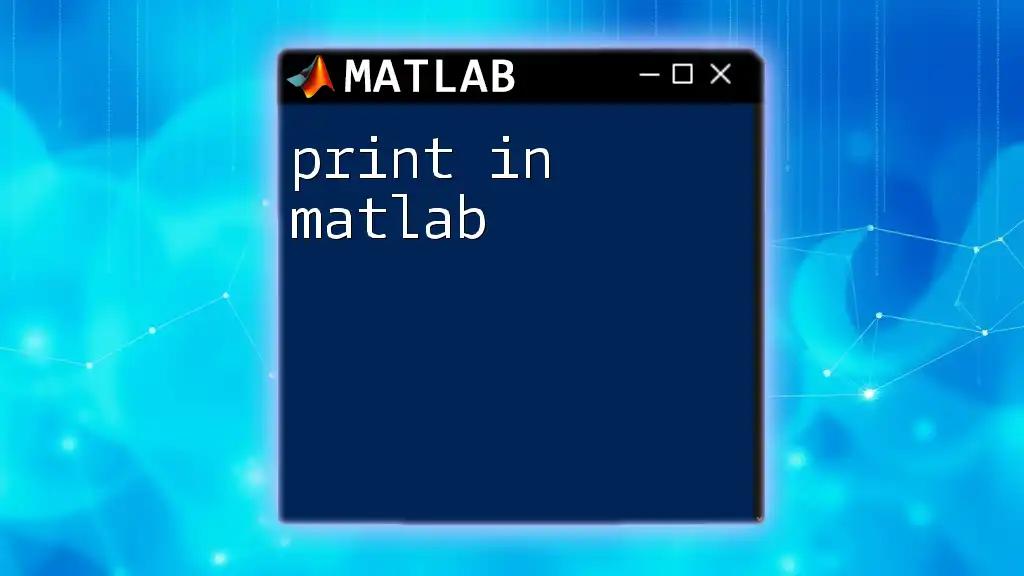
Conclusion
Having explored how to plot a point in MATLAB, from the basics of data preparation to advanced topics like 3D visualization and saving plots, you should now feel equipped to create your own informative and visually appealing plots. The world of MATLAB is vast, and this technique is just one of the many powerful tools at your disposal. Continue practicing and exploring to deepen your understanding and mastery of MATLAB plotting techniques.
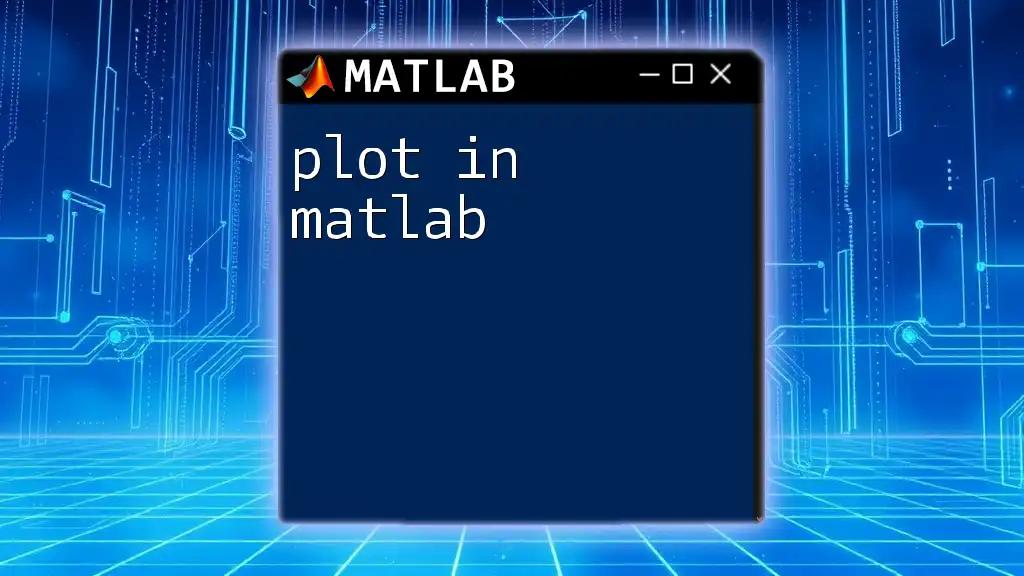
Additional Resources
For further learning, you can check official MATLAB documentation and other resources. Engaging with these materials will allow you to expand your skills and confidence in data visualization.
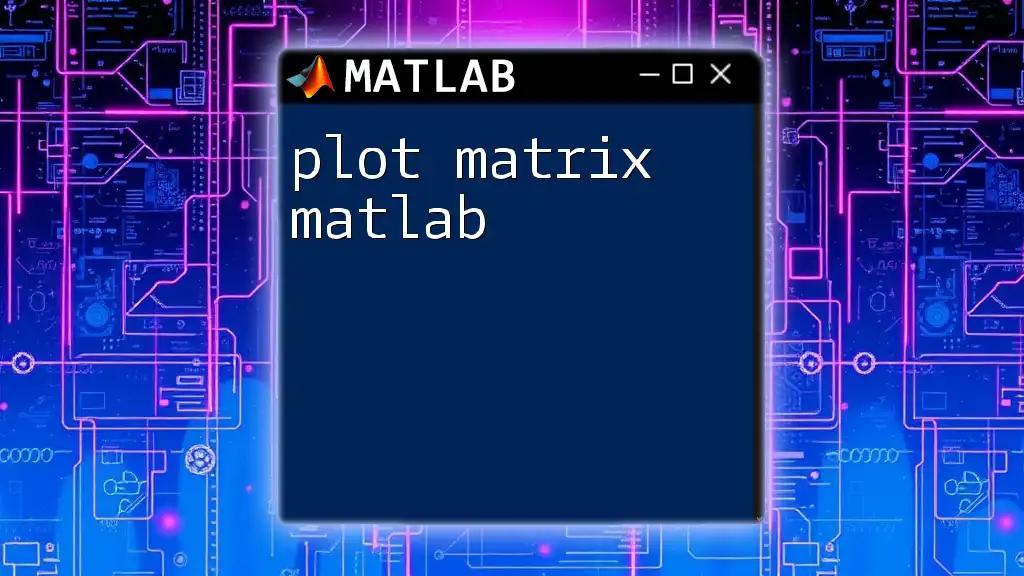
Call to Action
Consider signing up for our MATLAB tutorial services to enhance your understanding of data visualization and exploration!