In MATLAB, you can round numbers to the nearest integer using the `round` function, which can handle both positive and negative numbers effectively.
% Example of rounding in MATLAB
value = 3.7;
roundedValue = round(value); % roundedValue will be 4
Understanding Rounding Functions in MATLAB
Overview of Rounding Functions
MATLAB provides a suite of powerful functions designed for rounding numbers, which can be essential in various computational tasks. These include the following built-in functions:
- `round`
- `ceil`
- `floor`
- `fix`
Each of these functions serves a specific purpose and is optimized for particular rounding behaviors. In the sections that follow, we will explore each of these functions in detail.
round
The `round` function rounds elements of an array to the nearest integer. It considers the number's fractional part:
- If the fractional part is 0.5 or greater, it rounds up.
- If the fractional part is less than 0.5, it rounds down.
Syntax:
y = round(x)
Example:
x = [1.2, 2.5, 3.7, 4.1];
y = round(x); % Output: [1, 3, 4, 4]
This function is particularly useful in scenarios like financial calculations or when ensuring that the results fit into certain discrete categories. For instance, when dealing with currency, rounding to the nearest cent using `round` ensures accuracy.
ceil
The `ceil` function rounds each element of an array towards positive infinity. This means that any fractional value will be rounded up to the nearest whole number.
Syntax:
y = ceil(x)
Example:
x = [1.2, 2.5, 3.7, 4.1];
y = ceil(x); % Output: [2, 3, 4, 5]
In practice, you might use `ceil` in situations where you need to allocate resources or budget constraints — for instance, ensuring that you have enough items when dealing with whole quantities, such as boxes that cannot be partially filled.
floor
The `floor` function, on the other hand, rounds values towards negative infinity. It always rounds down, regardless of the number's sign.
Syntax:
y = floor(x)
Example:
x = [1.2, 2.5, 3.7, 4.1];
y = floor(x); % Output: [1, 2, 3, 4]
Using `floor` is especially beneficial in algorithms where the need to ensure you do not exceed countable resources is critical, such as in graphics programming when defining pixel values.
fix
The `fix` function rounds towards zero by truncating the decimal part of a number, effectively keeping the whole part intact while discarding the fraction.
Syntax:
y = fix(x)
Example:
x = [-1.2, 2.5, -3.7, 4.1];
y = fix(x); % Output: [-1, 2, -3, 4]
This function can be particularly useful when you want to ensure cohesion in scenarios where you need to truncate data rather than round it, such as in data analytics when filtering out unwanted decimals.
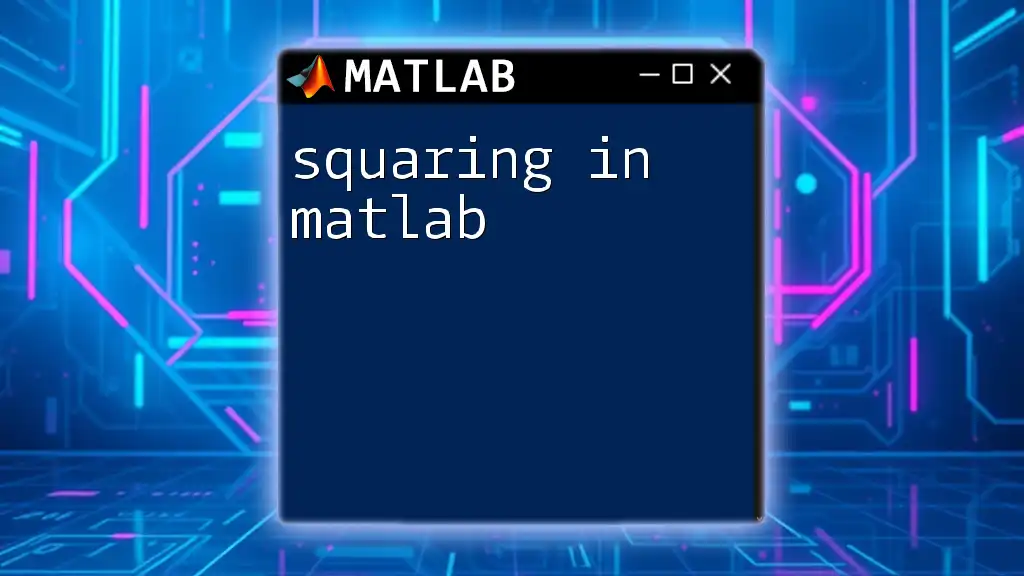
Comparing Rounding Methods
Differences between Rounding Functions
To clarify the distinctions between the rounding methods, it is useful to benchmark their outputs on the same set of values. Let's consider an array of numbers:
x = [1.2, 2.5, 3.7, 4.1];
fprintf('Round: %s\n', mat2str(round(x)));
fprintf('Ceil: %s\n', mat2str(ceil(x)));
fprintf('Floor: %s\n', mat2str(floor(x)));
fprintf('Fix: %s\n', mat2str(fix(x)));
The output will provide a clear illustration of how each function treats the same input.
Function | Rounds To |
---|---|
`round` | Nearest Integer |
`ceil` | Positive Infinity |
`floor` | Negative Infinity |
`fix` | Zero |
By examining these differences, users can make informed decisions about which rounding method best suits their needs based on specific data scenarios.
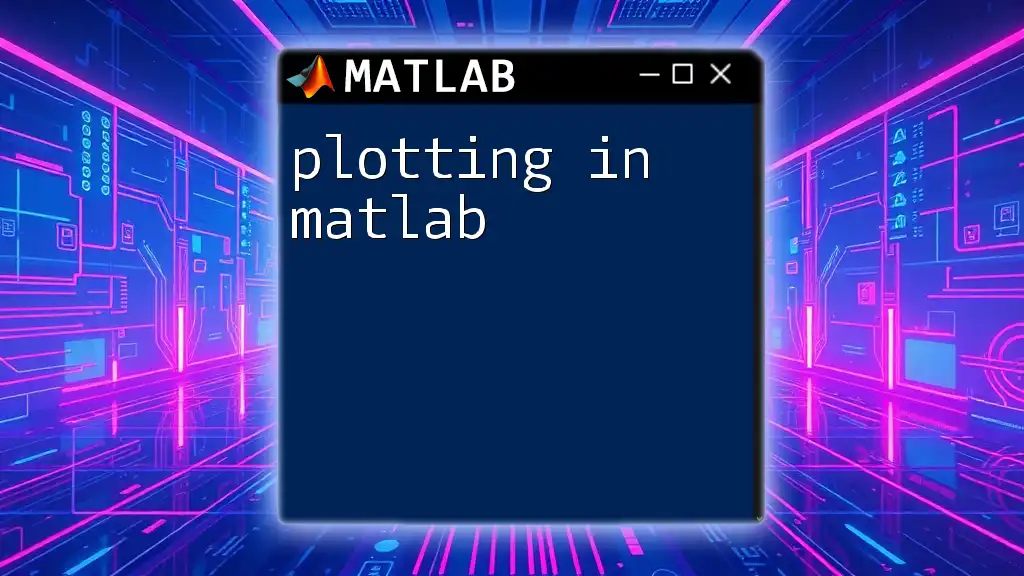
Special Cases in Rounding
Rounding Negative Numbers
An important aspect to consider is how each rounding function behaves with negative numbers. For example, when rounding -1.5:
- `round` will yield -2 (rounds away from zero).
- `ceil` will yield -1 (rounds towards positive infinity).
- `floor` will yield -2 (rounds towards negative infinity).
- `fix` will yield -1 (truncates towards zero).
This behavior can significantly impact your data, especially in statistical computations and algorithm designs, emphasizing the importance of selecting the proper function based on the context.
Rounding for Complex Numbers
MATLAB also allows for the rounding of complex numbers, which can sometimes be overlooked. When rounding complex numbers, MATLAB applies the rounding functions to the real and imaginary parts independently.
For example:
x = [1.5 + 2i, 3.4 - 1.2i];
rounded_real = round(real(x));
rounded_imag = round(imag(x));
In this example, only the real and imaginary components are adjusted according to the chosen rounding function, leaving a structured output that maintains the integrity of the complex data type.
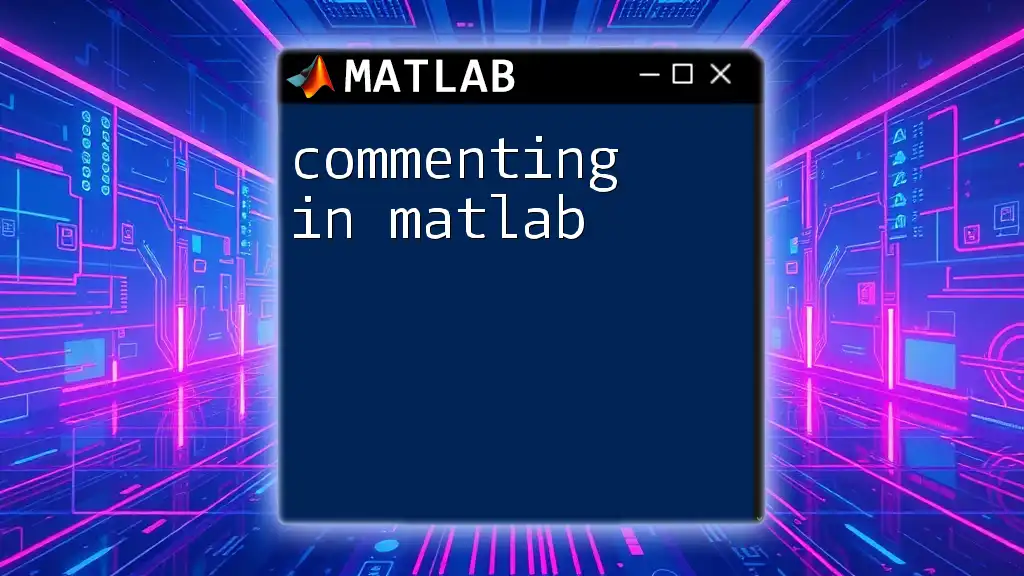
Best Practices for Rounding
Choosing the Right Function
When deciding which rounding function to use, consider your application’s requirements. If you need to round financial data, `round` is typically the best choice. For resource allocation, consider `ceil` or `floor`, depending on whether you need to round up or down.
Common Mistakes to Avoid
Avoid overlooking rounding errors that can arise from repeatedly rounding numbers in iterative calculations. For example, rounding intermediate results can lead to accumulating errors, affecting the accuracy of your final result. In financial scenarios, it's vital to round only when necessary to maintain precision.
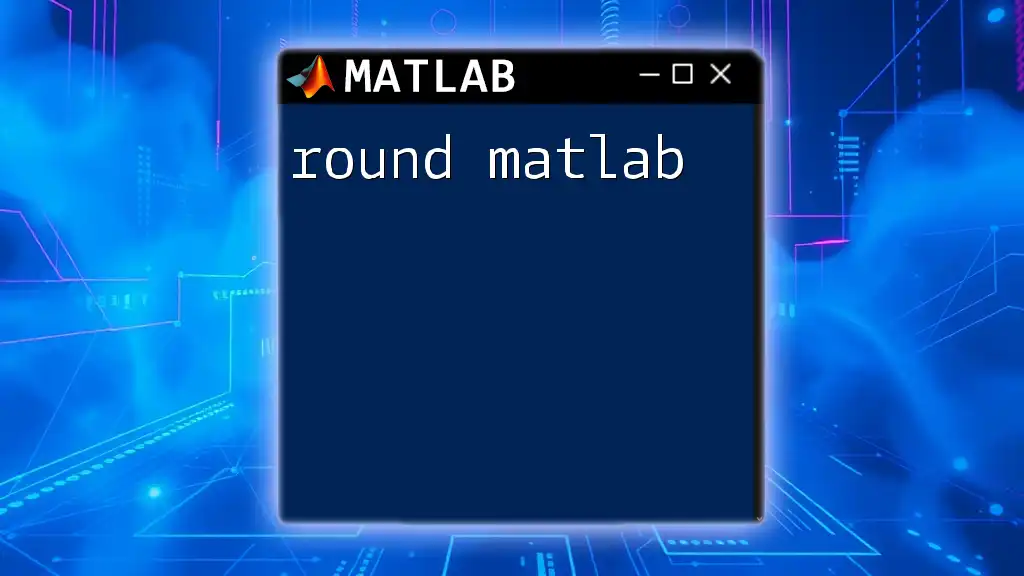
Conclusion
Rounding in MATLAB provides crucial functionality for numerical computations across diverse applications. By thoroughly understanding how each rounding function works — `round`, `ceil`, `floor`, and `fix` — you can optimize your calculations and ensure the robustness of your output. Practice implementing these functions with sample data to solidify your understanding and enhance your ability to apply them effectively in your projects.
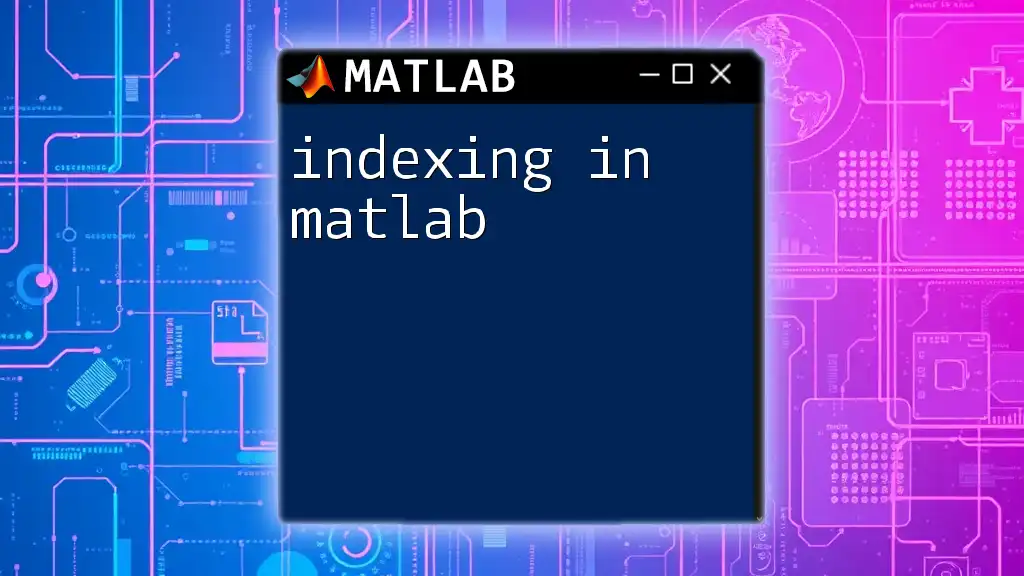
Additional Resources
For further reading, refer to the official [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/round.html) for in-depth explanations and additional examples on rounding functions. Engaging with MATLAB communities like MATLAB Central can also provide you with insights and alternative methods shared by fellow users.
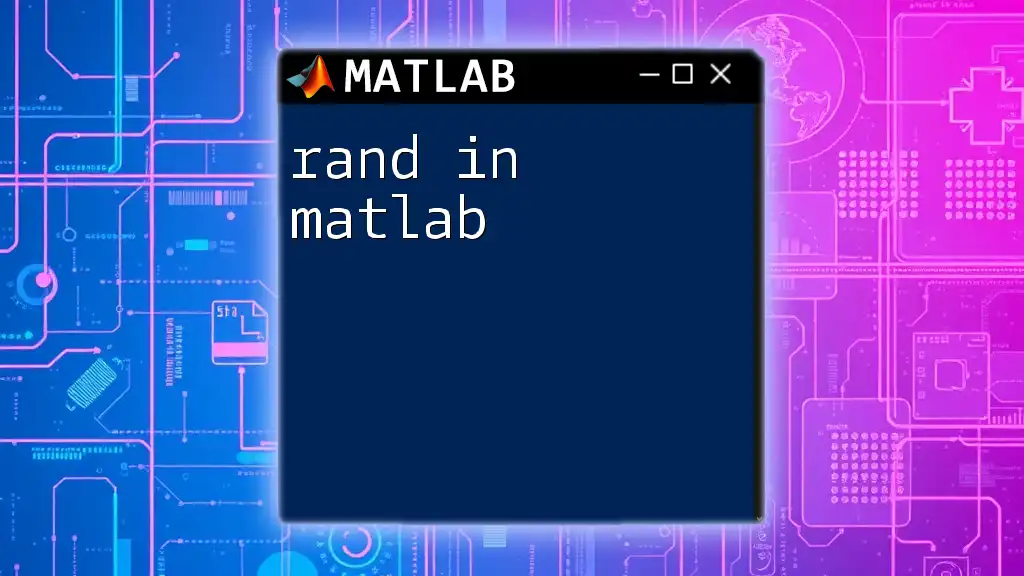
Call to Action
Stay tuned for more tutorials on MATLAB commands and tips to enhance your coding skills. Don’t forget to subscribe for updates to unlock a wealth of knowledge on MATLAB functionality!