To transpose a matrix in MATLAB, you can use the apostrophe (`'`) operator, which flips the matrix over its diagonal, switching the row and column indices of each element.
Here’s a code snippet demonstrating how to transpose a matrix:
A = [1, 2, 3; 4, 5, 6]; % Original matrix
B = A'; % Transposed matrix
Understanding Matrix Transposition
Definition of Transpose
The transpose of a matrix involves switching the rows and columns of the original matrix. For a given matrix A, the transpose is denoted by A'. Mathematically, if matrix A has dimensions of m x n (m rows and n columns), then its transpose, A', will have dimensions of n x m.
Visual Representation
For a clearer understanding, consider the following example:
Original Matrix A:
[1, 2, 3;
4, 5, 6]
Transposed Matrix A':
[1, 4;
2, 5;
3, 6]
This example illustrates how the first row of A transforms into the first column of A', and so forth for the other rows and columns.
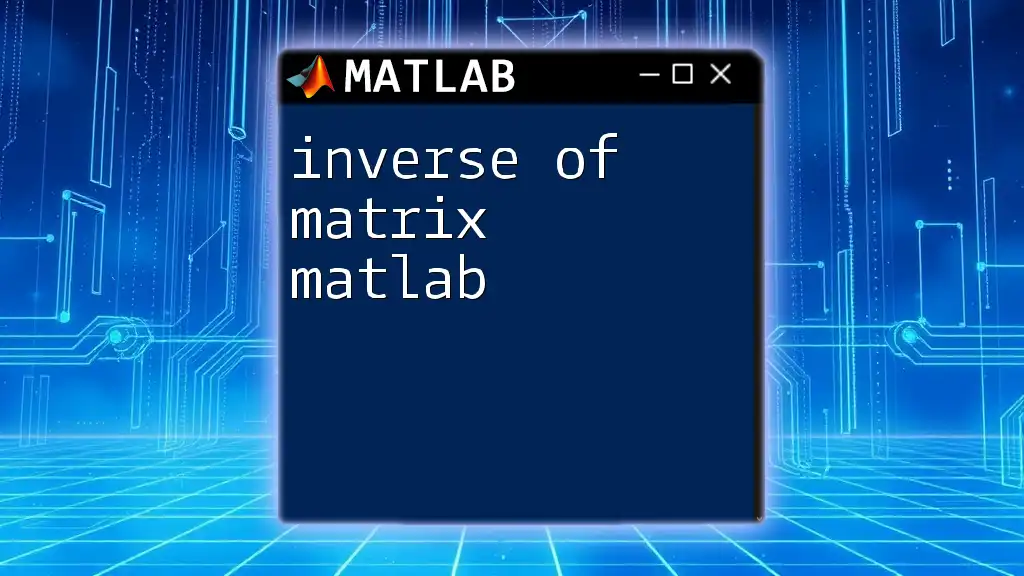
Why Use Transpose in MATLAB?
Applications of Transpose
Understanding how to perform the transpose of a matrix in MATLAB is fundamental to various applications:
- Linear Algebra: Many operations in linear algebra, such as solving systems of equations, require the transpose of matrices.
- Statistics and Data Manipulation: Data analysis often involves reshaping datasets, and transposing can simplify data manipulation processes.
- Machine Learning: Algorithms frequently utilize matrix transposition for operations involving training data, spanning features and samples.
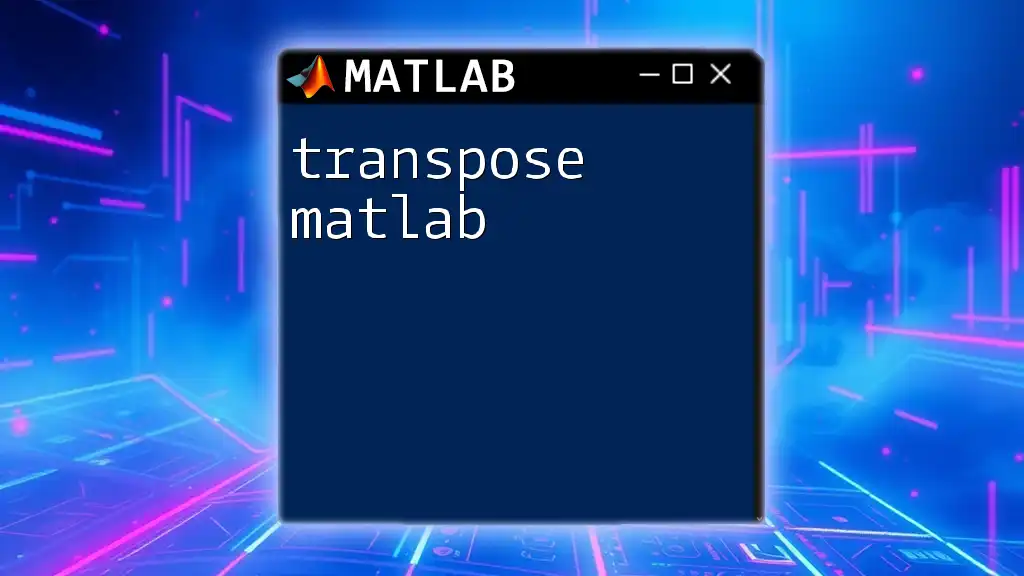
Basic MATLAB Command for Transposing a Matrix
Using the Transpose Operator
In MATLAB, the most straightforward way to transpose a matrix is using the transpose operator '. This operator is intuitive and easy to remember.
Example Code:
A = [1, 2, 3; 4, 5, 6];
B = A'; % Transpose of matrix A
disp(B);
In this code snippet, the original matrix A is transposed and stored in matrix B. The output will show:
1 4
2 5
3 6
This demonstrates that the rows of A have become the columns of B.
Alternative Transpose Command: `transpose()`
Alternatively, you can use the `transpose()` function in MATLAB. This function serves the same purpose but might be preferable in some contexts for clarity.
Example Code:
B = transpose(A);
disp(B);
Here, the function `transpose()` performs the same operation. The resulting output will be identical to the previous example, reinforcing the concept.
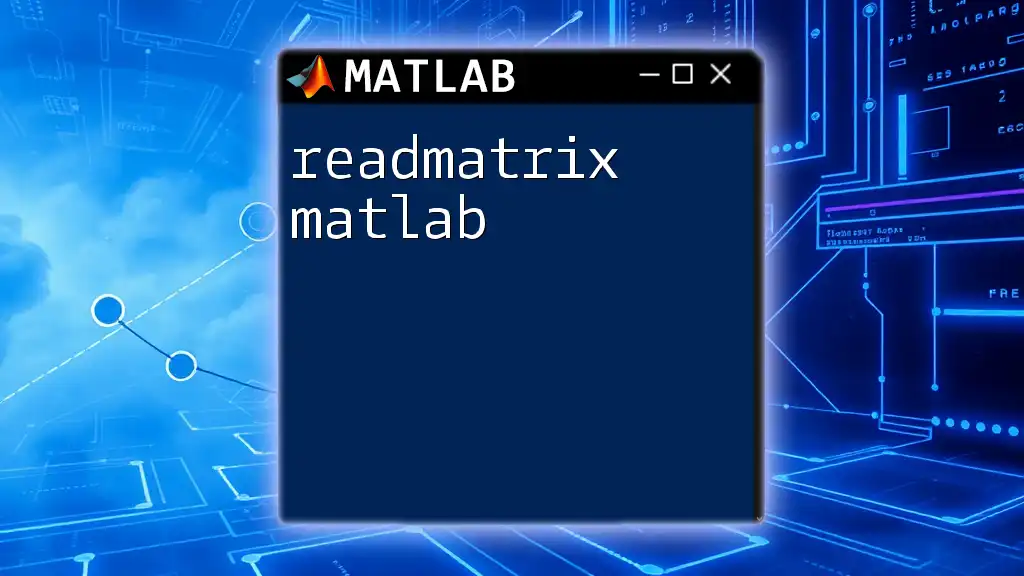
Special Cases of Transposing
Transposing a Row Vector
A row vector is defined as a matrix consisting of a single row. When transposed, a row vector converts into a column vector.
Example Code:
row_vector = [1, 2, 3];
col_vector = row_vector'; % Transposing row to column
disp(col_vector);
The output will be:
1
2
3
Transposing a Column Vector
Conversely, a column vector consists of a single column. When transposed, it becomes a row vector.
Example Code:
col_vector = [1; 2; 3];
row_vector = col_vector'; % Transposing column to row
disp(row_vector);
The output will display:
1 2 3
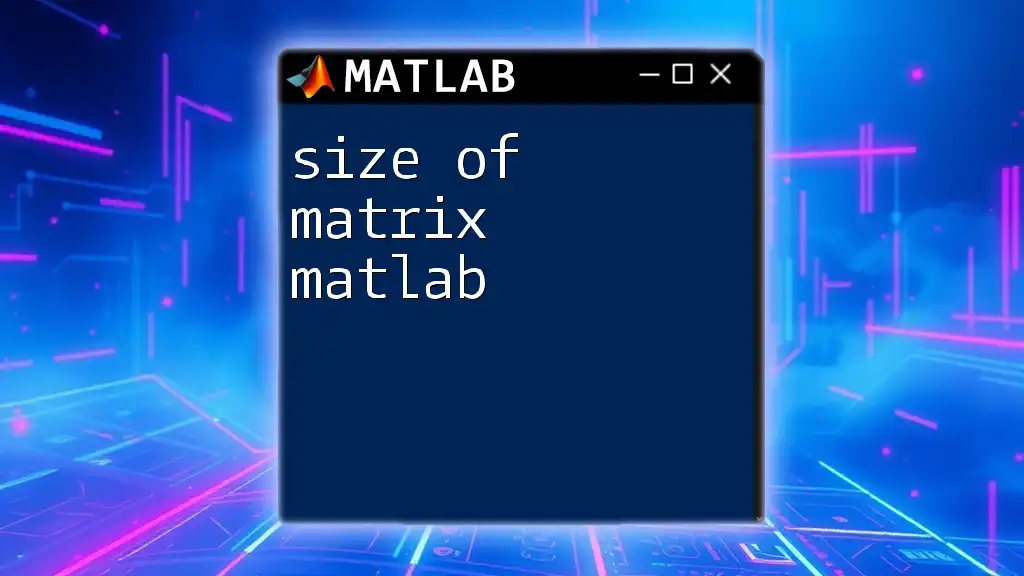
Important Considerations
Size Compatibility
Transposing operations maintain dimensional integrity. However, it's crucial to note the implications when dealing with non-square matrices. For example, if you transpose a matrix of size 3 x 2, the resulting matrix will be 2 x 3.
Complex Numbers
When dealing with complex numbers, the concept of transposition becomes more nuanced. The standard transpose of a complex matrix does not take the complex conjugate. To obtain the conjugate transpose, you can use the command `C.'`.
Example Code:
C = [1+2i, 3+4i; 5+6i, 7+8i];
C_transpose = C'; % Standard transpose
disp(C_transpose);
The above command gives a regular transpose without conjugation. To perform a conjugate transpose, you would opt for:
C_conjugate_transpose = C.'; % Conjugate transpose
disp(C_conjugate_transpose);
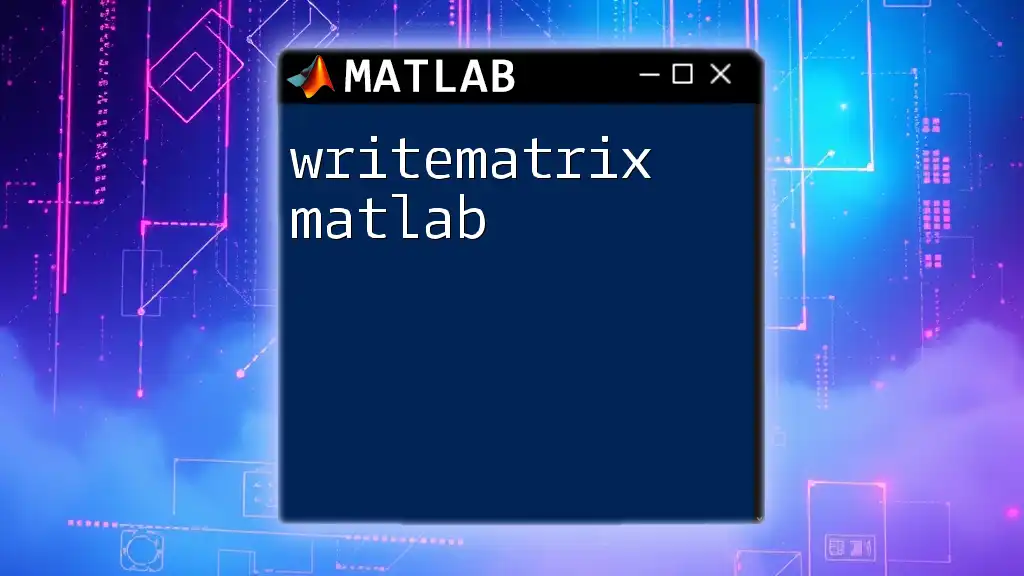
Practical Applications of Matrix Transposition in MATLAB
Data Manipulation
One of the core uses of matrix transposition is to reshape datasets efficiently. For instance, when you have a dataset structured in a way that features are arranged in columns, and you require the data in rows for processing, transposing can help quickly adapt the data format for analysis.
Applications in Solving Systems of Equations
Transpose operations can also be utilized in solving systems of linear equations. Consider the equation represented in the form Ax = b, where A is a matrix. Transposing can help align our variables more conveniently for approaches like least squares.
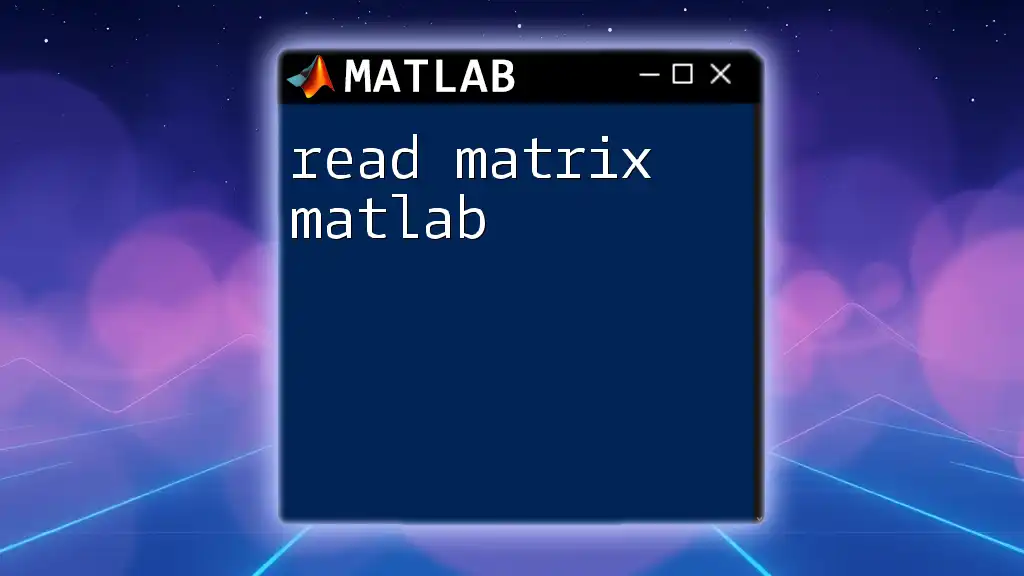
Conclusion
Understanding the transpose of a matrix in MATLAB is a fundamental skill for anyone working with matrices in programming. It opens up a breadth of avenues for data manipulation and mathematical operations essential in various fields. By mastering the transpose operation, you empower yourself to tackle more complex challenges effectively.
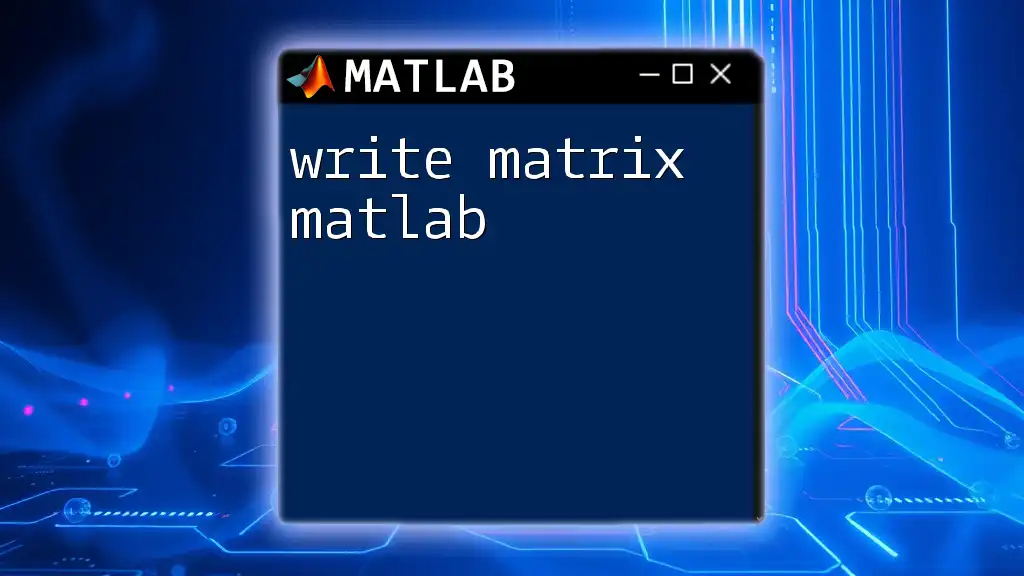
Additional Resources
Further Reading
For further insights on MATLAB and matrix operations, consider exploring textbooks on linear algebra, MATLAB documentation, or online courses on computational mathematics.
Community and Support
Engage with MATLAB forums and communities to share knowledge or seek assistance for any queries you may have regarding matrix operations or programming challenges.
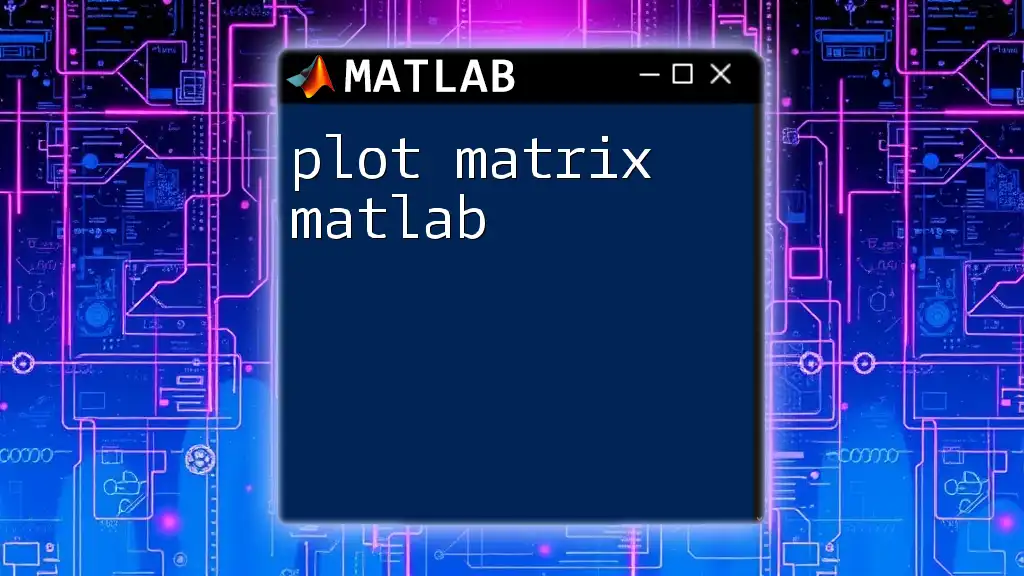
FAQs
Are you still uncertain about certain aspects of transposing a matrix? Common questions may include issues regarding errors when transposing mismatched dimension matrices or how to efficiently transpose large datasets. Engaging in community discussions can foster a deeper understanding and clarify these misconceptions.