Kohonen's animal data in MATLAB is used to demonstrate self-organizing maps (SOM) for clustering and visualizing data about different animal species based on multiple features.
Here's a simple example of how to load and visualize Kohonen's animal data using a self-organizing map in MATLAB:
% Load Kohonen's animal data
load('animal.mat'); % Assuming 'animal.mat' contains the data
% Create a self-organizing map
net = selforgmap(3); % 3-dimensional output
% Train the network
net = train(net, animalData);
% View the trained network
view(net);
% Visualize the clustering
plotsompos(net, animalData);
Understanding Kohonen's Self-Organizing Maps
What is a Self-Organizing Map?
Kohonen's Self-Organizing Map (SOM) is a type of unsupervised neural network that uses competitive learning to produce a low-dimensional representation of high-dimensional data. The main idea behind SOM is to transform the input space into a grid of neurons, where similar input patterns are represented by topologically adjacent neurons. This process allows you to visualize complex datasets with high dimensionality in a simpler form, making it easier to understand underlying patterns.
Applications of SOM in Animal Data
Analyzing animal data with SOM opens up various avenues in ecological studies, behavioral analysis, and genetics. For instance, researchers can:
- Categorize animal behavior based on various traits, identifying patterns and correlations.
- Examine genetic variations among species, aiding in conservation biology.
- Understand habitat preferences by clustering data points related to environmental factors.
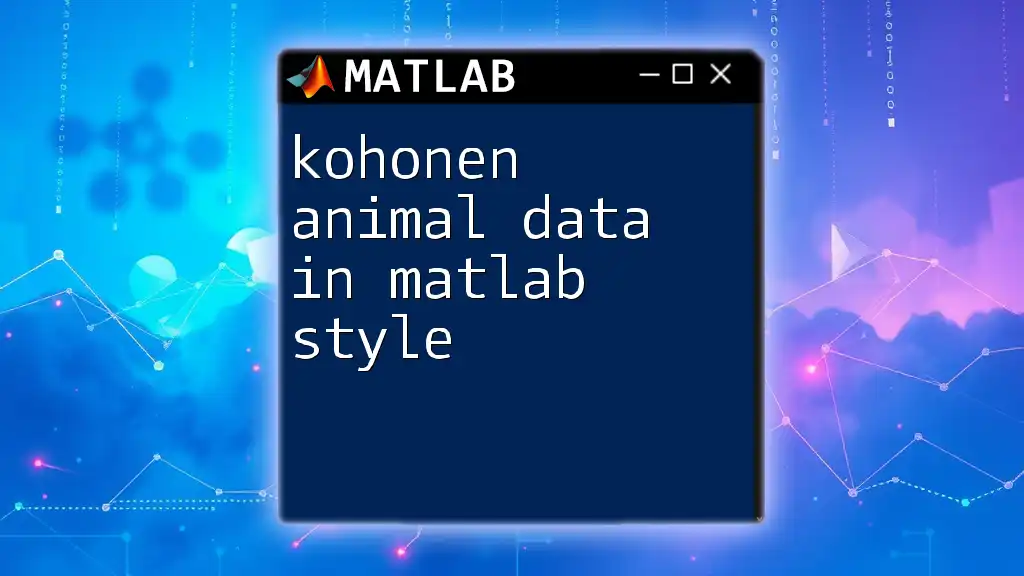
Preparing Your MATLAB Environment
Installing MATLAB and Required Toolboxes
To get started with Kohonen's animal data in MATLAB, ensure that you have installed MATLAB along with the Statistics and Machine Learning Toolbox. This toolbox is crucial for implementing SOM and handling data analysis tasks efficiently. If you need to check whether you have the necessary toolbox, you can use the following command in MATLAB:
ver % Displays the list of installed toolboxes
Loading and Preparing the Animal Data
For this guide, we will use an example dataset that contains various animal attributes. It's essential to understand the structure of the dataset to preprocess it effectively. Make sure your data is formatted correctly, ideally in a MATLAB `.mat` file or a CSV file. To load your dataset, use the following command:
load('animalData.mat') % Load the dataset containing animal data
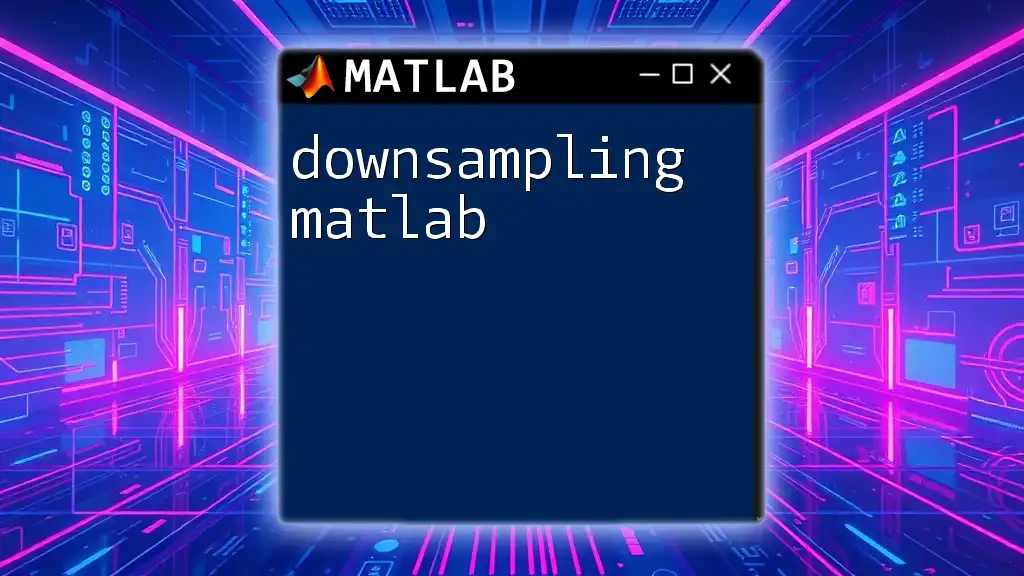
Exploring Kohonen's Animal Data
Analyzing the Dataset
Once the data is loaded, begin by performing some basic statistical analysis to get an overview. This may include mean, median, variance, etc. Visualizing the data can be highly informative. For instance, you may create scatter plots to display relationships between key features. Here’s how you can visualize your dataset:
scatter(animalData(:,1), animalData(:,2)); % Example scatter plot
xlabel('Feature 1');
ylabel('Feature 2');
title('Animal Data Scatter Plot');
This visual representation helps identify any obvious trends or clustering in the data, which is crucial for further analysis.
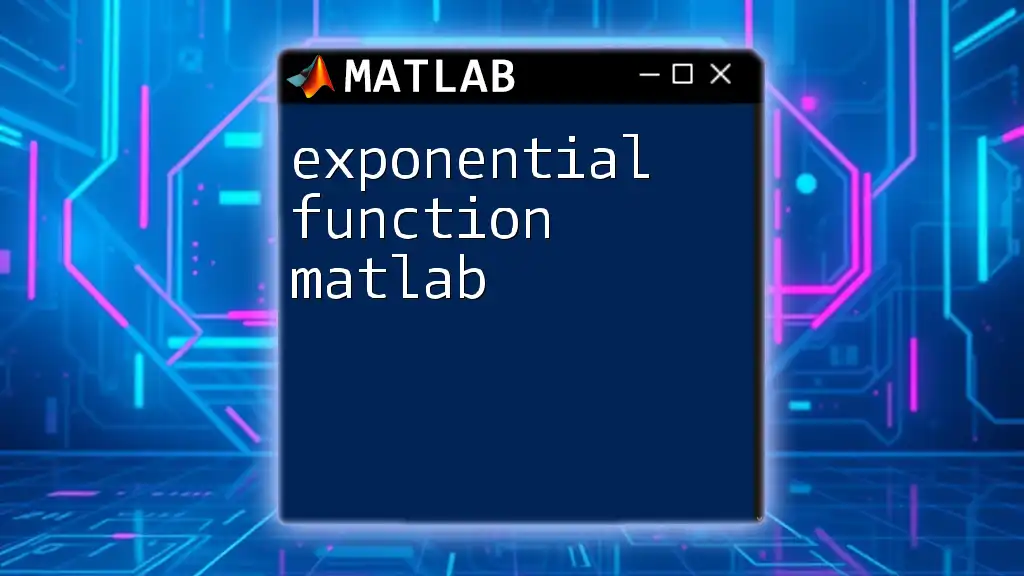
Implementing Kohonen's Self-Organizing Map in MATLAB
Step-by-Step Guide to Creating SOM
To create a self-organizing map in MATLAB, you'll follow a series of steps, including initializing the SOM and training it with your dataset. Define the size of the map based on the complexity of your data. For example, if you have a 10x10 grid, you can initialize your SOM as follows:
numNeurons = [10 10]; % Specify the grid size
som = selforgmap(numNeurons);
[som, tr] = train(som, animalData'); % Training the SOM
Visualizing the Results
U-Matrix Visualization
After the training process, visualizing the U-Matrix is essential as it helps in understanding the distances between neurons. The U-Matrix can reveal clusters and the relationship among them. Use the following command to display the U-Matrix:
view(som,'all');
This output contains valuable insights regarding how the data is clustered on the SOM grid.
Component Planes
The component planes give insights into individual features and how they contribute to cluster formation. You can visualize these planes using:
plotsom(som); % Visualizing component planes
These plots enable you to see how each feature is represented across the SOM and identify correlations between different features.
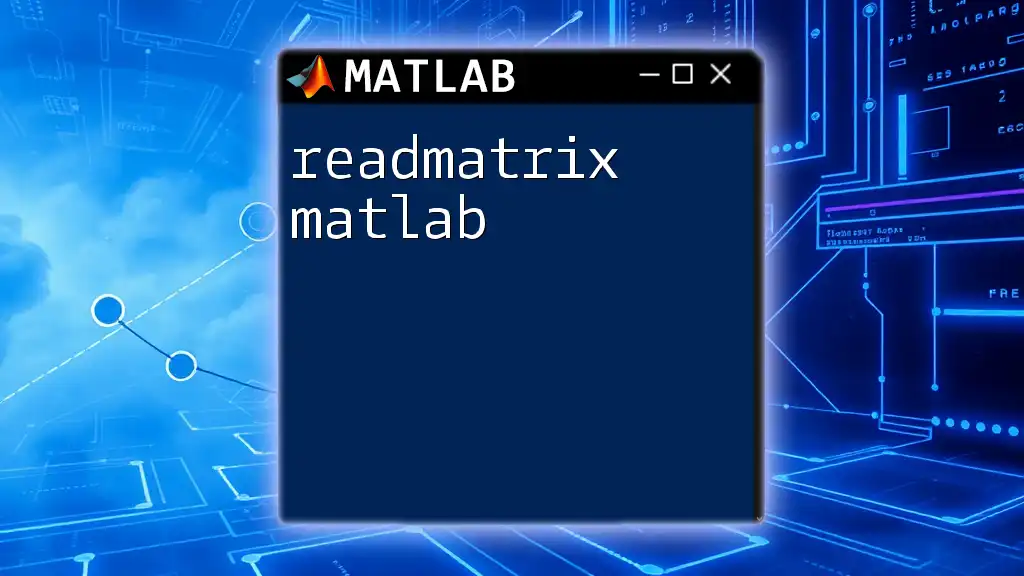
Interpreting the Results
Clustering Analysis
Interpreting the clusters formed by your SOM will provide insights into how different animals or their traits are grouped. Look for patterns such as clustering of specific species based on their behaviors or environmental adaptations.
Case Studies and Practical Examples
Let's consider a practical example in clustering animal behaviors. When applied to a dataset of animal activity patterns, the SOM can effectively segregate differing behaviors, allowing researchers to pinpoint trends. This technique finds its application in various fields—ecology, conservation efforts, and even in domestic animal training.
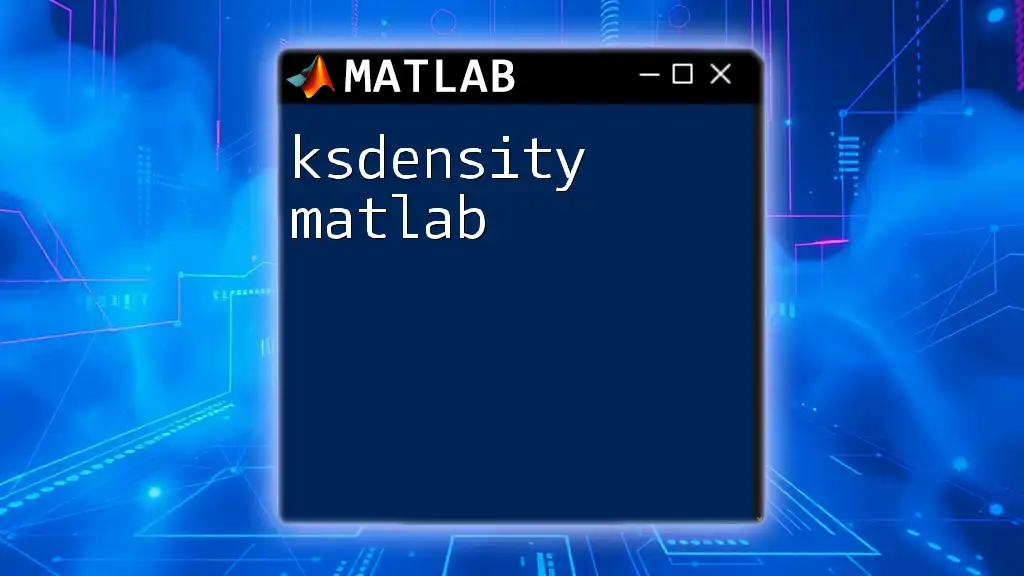
Enhancing Your SOM Analysis
Parameter Tuning
The adaptability of the SOM can significantly improve the quality of your results. Tuning parameters like learning rate and neighborhood size creates a more refined model. Here’s an example of how you can adjust the learning rate:
som = selforgmap(numNeurons, 'topologyFcn', 'gridtop', 'distanceFcn', 'linkdist');
som.trainParam.epochs = 100; % Set the number of training epochs
som.trainParam.lr = 0.05; % Set learning rate
[som, tr] = train(som, animalData');
Using Custom Datasets
If you wish to work with your own animal data, ensure it's structured similarly to the provided dataset. Import your data appropriately, using:
customData = readtable('customAnimalData.csv'); % Importing custom .csv file
After loading your custom dataset, apply the same preprocessing and training steps discussed previously.
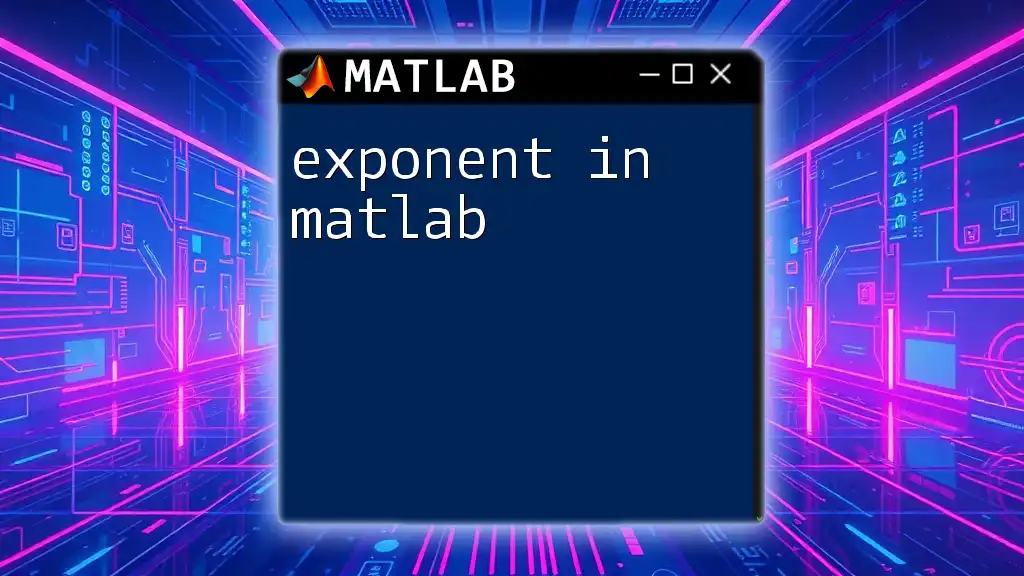
Conclusion
Kohonen's animal data analysis in MATLAB provides a robust framework for uncovering complex relationships within animal datasets. The Self-Organizing Maps serve as an invaluable tool for visualizing and interpreting data patterns that might otherwise be hidden. Experiment with your datasets to discover new insights, and remember that effective data analysis requires continuous learning and adaptation.
Matthews to explore the intricacies of MATLAB further with our courses, where we empower you to leverage these techniques effectively in your projects!
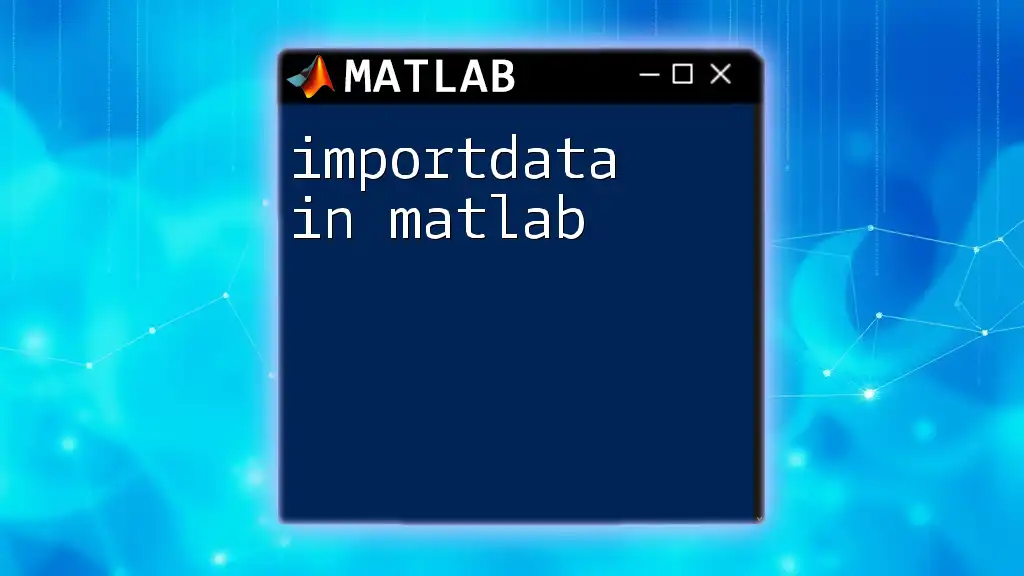
Additional Resources
Recommended Reading
For those interested in diving deeper, consider reading foundational texts on Kohonen's Self-Organizing Maps and exploring online courses that focus on advanced data visualization techniques using MATLAB.
Community and Support
Engage with MATLAB forums and communities for additional insights. Joining discussion groups can also provide valuable peer support and additional learning opportunities as you hone your skills in MATLAB and data analysis.