In MATLAB, you can calculate the exponent of a number using the `.^` operator for element-wise exponentiation, or `^` for matrix exponentiation.
% Element-wise exponentiation
result1 = 2 .^ [1, 2, 3]; % result1 will be [2, 4, 8]
% Matrix exponentiation (raising a matrix to a power)
A = [1, 2; 3, 4];
result2 = A ^ 2; % result2 will be [7, 10; 15, 22]
Understanding Exponentiation in MATLAB
What is Exponentiation?
Exponentiation is a fundamental mathematical operation in which a number, called the base, is raised to the power of an exponent. In mathematical terms, it is represented as \(x^y\), where \(x\) is the base and \(y\) is the exponent. This operation is essential in various mathematical computations, from basic arithmetic to complex algorithms used in fields like data science, physics, and engineering.
Basic Syntax in MATLAB
In MATLAB, the syntax for performing exponentiation is straightforward. The operator used for exponentiation is `^`. The basic syntax looks like this:
result = base ^ exponent;
Here, `base` represents the number you want to raise to a power, and `exponent` is the power you want to raise it to. For instance, if you want to calculate \(2^3\), you would write:
result = 2 ^ 3; % result will be 8
This simple expression computes 2 multiplied by itself three times, resulting in 8.
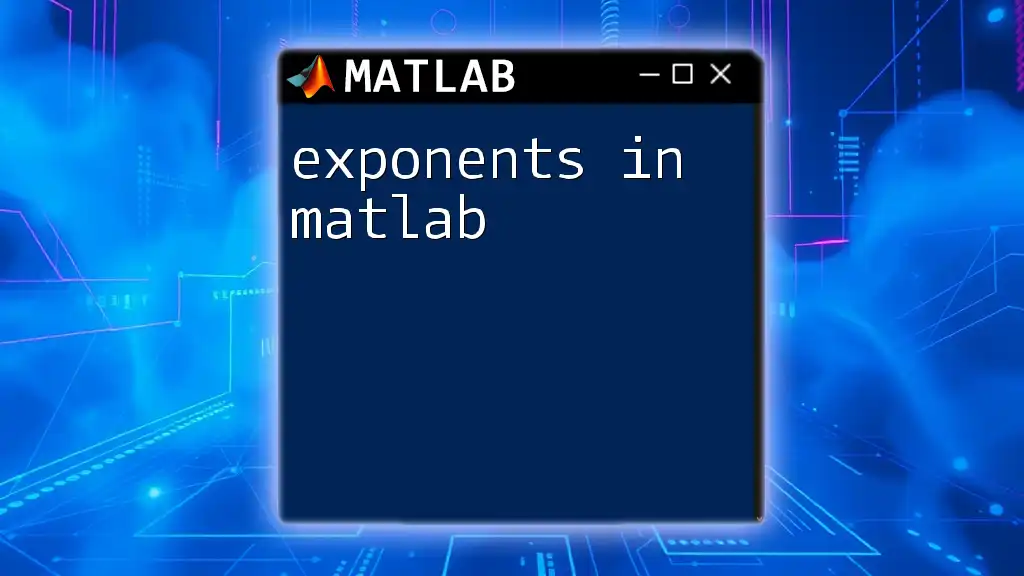
MATLAB Exponentiation Operators
The `^` Operator
The `^` operator is the primary method for performing exponentiation in MATLAB. It takes a base and raises it to a specified power. This operator operates seamlessly with scalar numbers.
For example:
result = 5 ^ 4; % result will be 625
In this example, 5 raised to the power of 4 equals 625, as it evaluates to \(5 \times 5 \times 5 \times 5\).
The `.^` Operator for Element-wise Exponentiation
While the `^` operator is great for scalar computations, MATLAB also allows for element-wise operations using the `.^` operator. This is particularly useful for working with matrices and arrays.
For instance, if you have a matrix and want to square each element, you can use the `.^` operator like this:
A = [1, 2, 3; 4, 5, 6];
result = A .^ 2; % squaring each element
In this case, the result would be a new matrix where each element from matrix `A` has been squared:
result = [1, 4, 9;
16, 25, 36]
This operator is essential for performing operations over entire datasets without needing to employ loops, making your code more efficient and elegant.
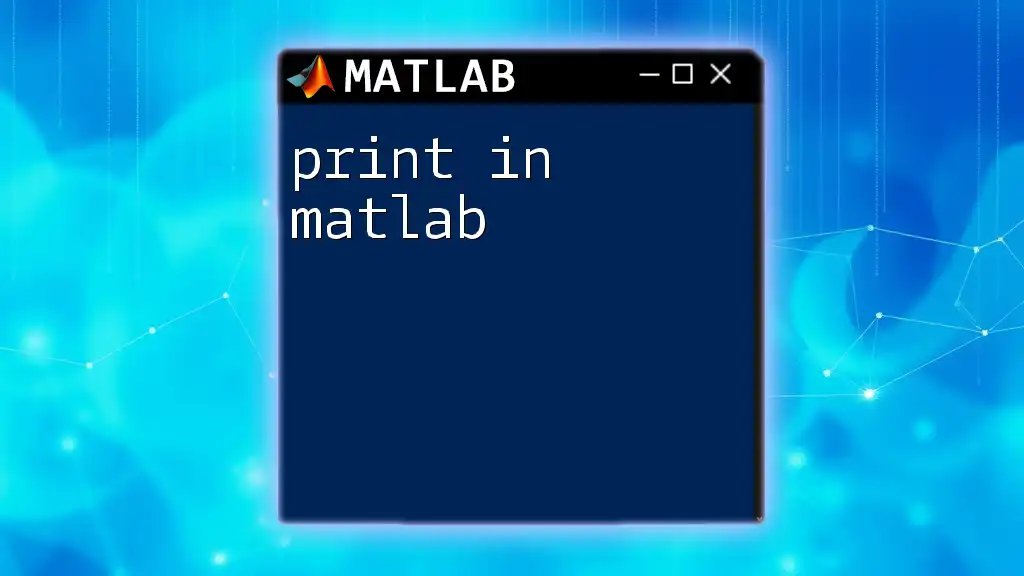
Functions Related to Exponentiation
Using the `exp` Function
The `exp` function in MATLAB calculates the value of e raised to the power of a specified number. Here, \(e\) is the base of the natural logarithm, approximately equal to 2.71828. To use it, simply call the function with your desired exponent:
a = 1;
result = exp(a); % result will be e
In this example, the output for `result` will be approximately 2.71828, which is \(e^1\). This function is particularly useful in mathematical models like growth and decay.
Using the `power` Function
Another way to perform exponentiation in MATLAB is by using the `power` function, which serves as an alternative to the `^` operator and achieves the same results with a slightly different syntax.
The syntax is as follows:
result = power(base, exponent);
For example:
result = power(2, 3); % result will be 8
This computes \(2^3\) and again gives us 8. The `power` function can also be applied to vectors and matrices by automatically handling element-wise operations similar to the `.^` operator.
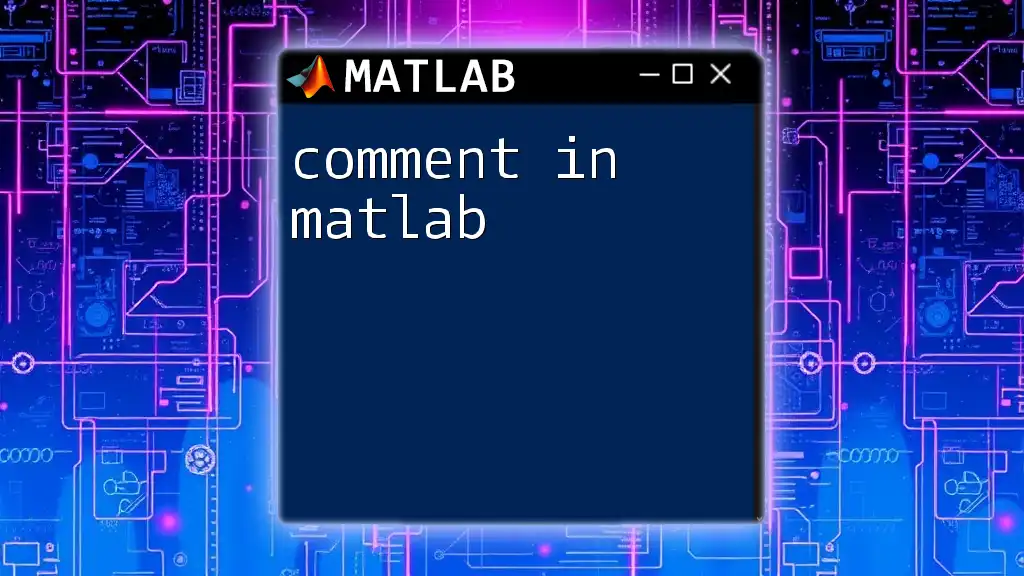
Practical Applications of Exponentiation
Mathematical Calculations
Exponentiation is crucial for performing complex mathematical calculations. For example, if you are working with polynomial equations or functions involving logarithms, exponentiation becomes a key component.
You can also create plots to visualize mathematical functions that involve exponentiation to understand their behavior better. MATLAB allows you to plot these functions with ease.
Data Analysis and Engineering
In the fields of data analysis and engineering, exponentiation is often used in algorithms for modeling phenomena that exhibit exponential growth or decay, such as population growth or half-life decay in radioactive materials.
Consider the following example of modeling exponential growth:
t = 0:0.01:5;
growth = 100 * exp(0.1 * t);
plot(t, growth);
title('Exponential Growth');
In this script, the variable `growth` simulates exponential growth over time and is plotted against time `t`, visualizing how quickly the value increases.
Simulations
Exponentiation also plays a critical role in simulations. For example, in physics, the behavior of oscillating systems can often be modeled with exponential functions.
Here's a simple MATLAB code snippet demonstrating a damped oscillation using exponentiation:
x = 0:0.1:10;
y = sin(x) .* exp(-0.1 * x);
plot(x, y);
title('Damped Oscillation');
In this case, the `y` values represent a combination of a sine function multiplied by an exponential decay factor, illustrating how the amplitude of the oscillation decreases over time.
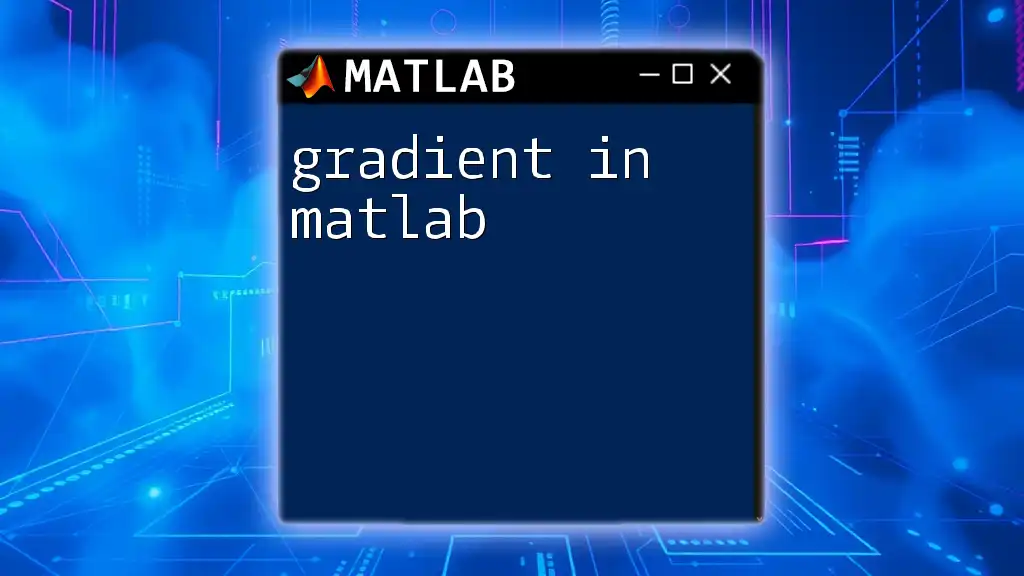
Common Errors and Troubleshooting
Mismatched Dimensions
When performing element-wise operations using the `.^` operator, it's crucial to ensure that the dimensions of the matrices or vectors involved are compatible. A mismatch can result in runtime errors.
For example, if you try to perform an operation like this:
A = [1, 2; 3, 4];
B = [5; 6];
result = A .^ B; % This may produce an error
To avoid dimension mismatch errors, ensure that both `A` and `B` are the same size or that one is a scalar.
Complex Numbers
Exponentiation behaves differently with complex numbers. When using the `^` operator on complex numbers, the result is influenced by the properties of complex arithmetic.
For instance:
z = 1 + 2i;
result1 = z ^ 2; % Square of the complex number
result2 = z .^ 2; % Element-wise squaring (not applicable here)
The first command computes the square of the complex number while the second one would typically not apply itself to an array of complex numbers directly since `z` is a single scalar.
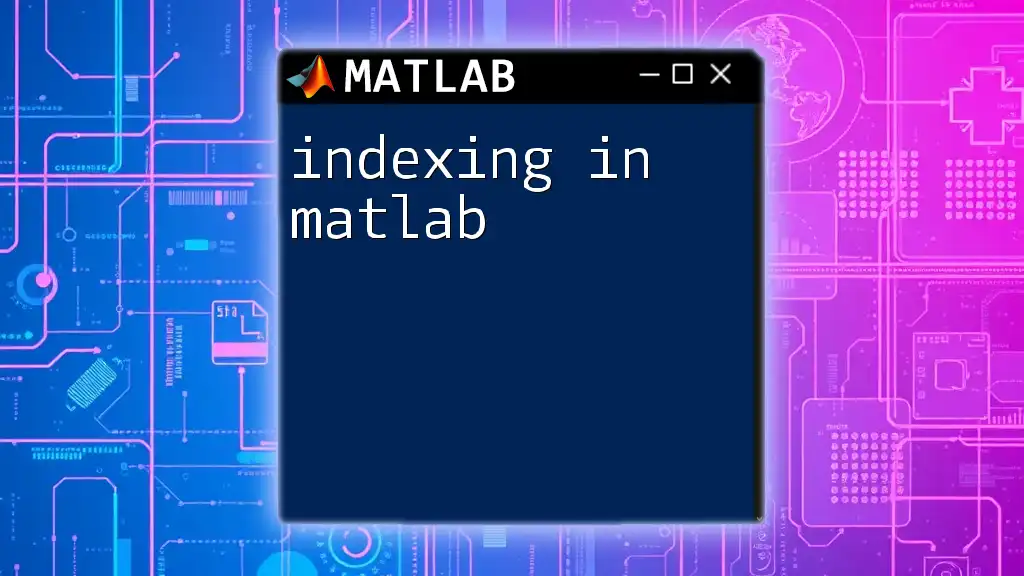
Conclusion
Exponentiation is a powerful mathematical operation and is integral to utilizing MATLAB effectively. By understanding the different operators—`^` for scalars and `.^` for element-wise operations—you can easily implement exponential calculations in your programming.
Additionally, the `exp` and `power` functions provide flexible alternatives for specific use cases. Whether you're performing mathematical computations, modeling real-world scenarios, or simulating systems, mastery of exponentiation in MATLAB will significantly enhance your analytical capabilities.
Encouragingly, by practicing these concepts and stunning examples, you can develop a deeper understanding of how to effectively use exponentiation in MATLAB for various applications.