In MATLAB, you can easily set the title of your graph by using the `title` function, which allows you to provide a string that will appear at the top of your plot. Here's a simple example:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
Understanding Graph Titles in MATLAB
What is a Graph Title?
A graph title is a crucial component of data visualizations, serving as a label that concisely summarizes what the graph represents. It acts as an introductory statement that provides context before delving into the details of the data visualization. In essence, a good graph title enables the audience to quickly grasp the purpose and significance of the graph.
Why are Titles Important?
Effective titles are more than just words; they play a pivotal role in enhancing understanding and engagement. A clear and concise title can significantly impact how the audience interprets the data presented. It sets expectations and guides viewers through the information, making it easier for them to draw conclusions.
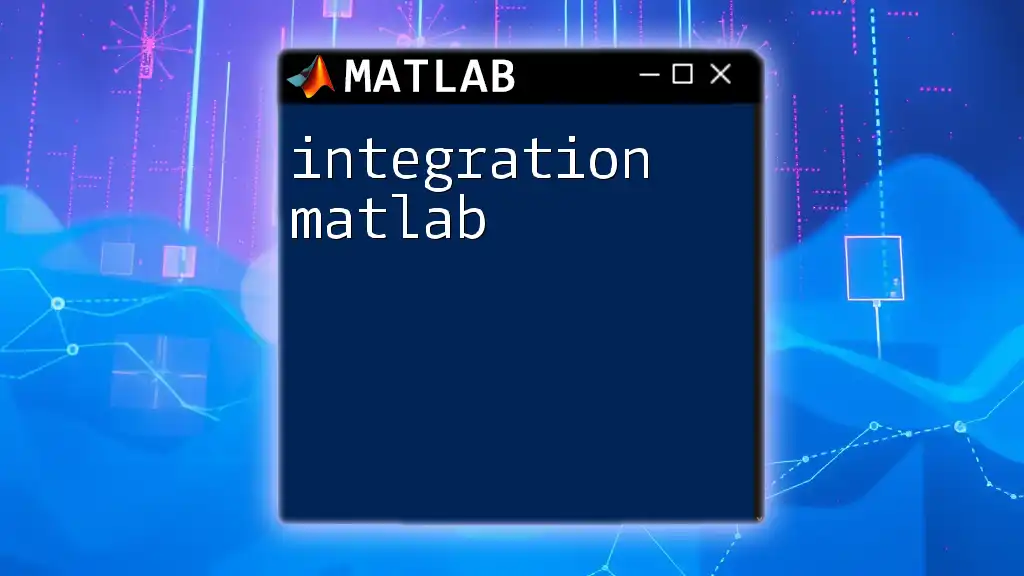
Setting Up Your MATLAB Environment
Installing MATLAB
To get started, first, ensure you have MATLAB installed on your computer. For beginners, a quick search for installation guides can be extremely helpful. Official resources from MathWorks provide comprehensive instructions tailored to different operating systems.
Opening MATLAB and Preparing to Plot
Once you have MATLAB up and running, familiarize yourself with the interface, which typically includes the Command Window, Workspace, and various tools. Before plotting, you can initiate a new figure window using the command:
figure;
This sets the stage for your data plots.
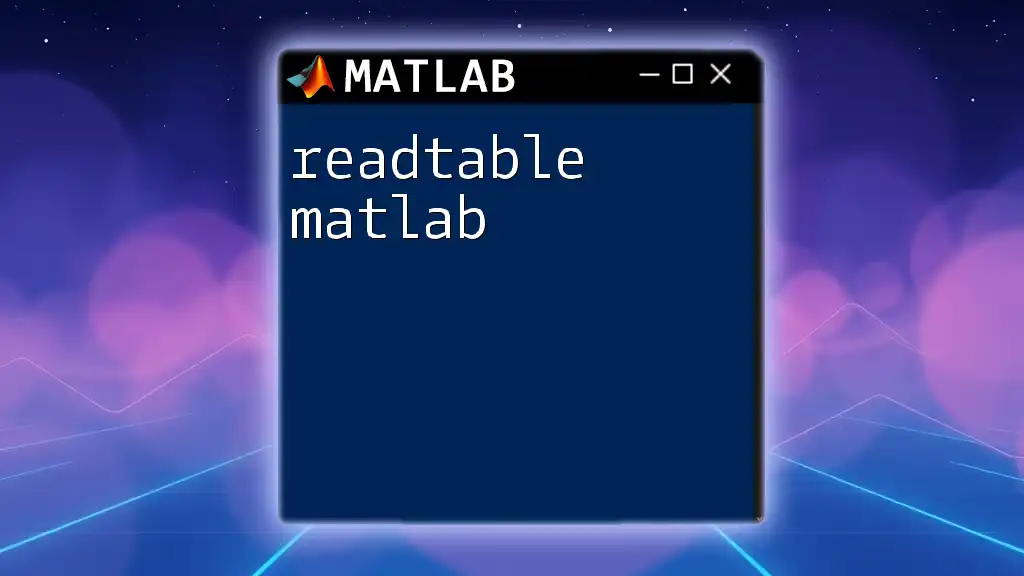
Creating a Basic Graph
Sample Data Preparation
Creating a graph requires data, so let's generate some sample data for this example. Here, we'll generate a simple vector using MATLAB's built-in functionality:
x = 1:10;
y = rand(1, 10); % Generate random data
This snippet creates a vector `x` with values from 1 to 10 and a `y` vector of random values.
Plotting the Data
Now that we have our data, we can create a basic plot:
plot(x, y);
This command generates a simple line graph, displaying the relationship between `x` and `y`.
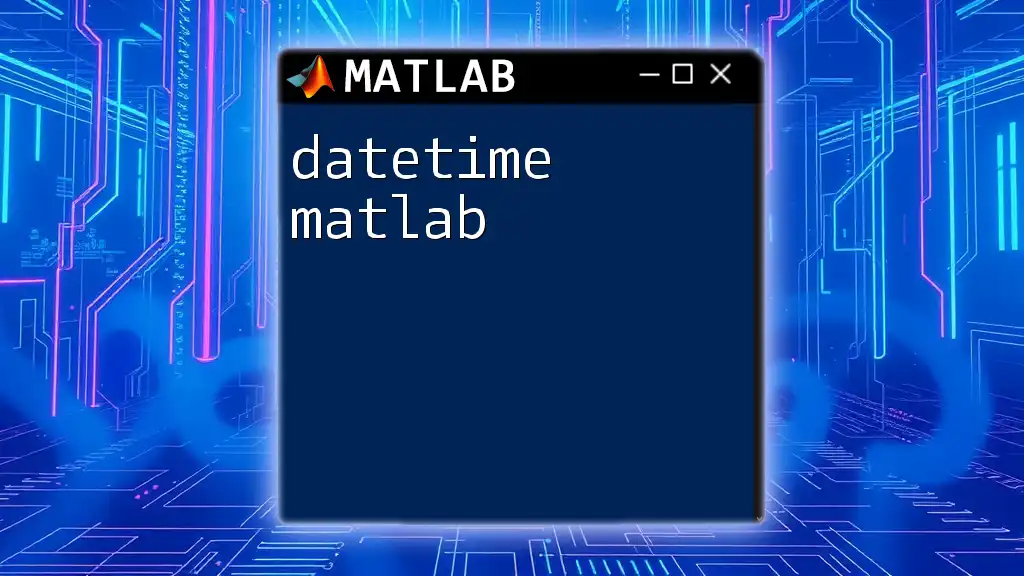
Adding Titles to Your Graph
Syntax for Adding Titles
In MATLAB, adding a title to your graph is straightforward using the `title` function. Here’s a fundamental example of how to do this:
title('Your Graph Title Here');
This command allows you to specify the title, providing viewers with an immediate understanding of the graph's content.
Title Formatting Options
Font Size and Color
Customization can significantly improve the readability of your graph title. For instance, you can adjust the font size and color with the following command:
title('Your Graph Title Here', 'FontSize', 14, 'Color', 'r');
This command sets the title's font size to 14 and changes the text color to red. Such customizations not only enhance visibility but also help emphasize critical information.
Title Positioning
Strategic placement can further improve clarity. You can specify the position of your title using the `Position` parameter:
title('Your Graph Title Here', 'Position', [x, y]);
Adjusting the position can help achieve optimal visual balance and ensure that the title complements the overall graph layout.
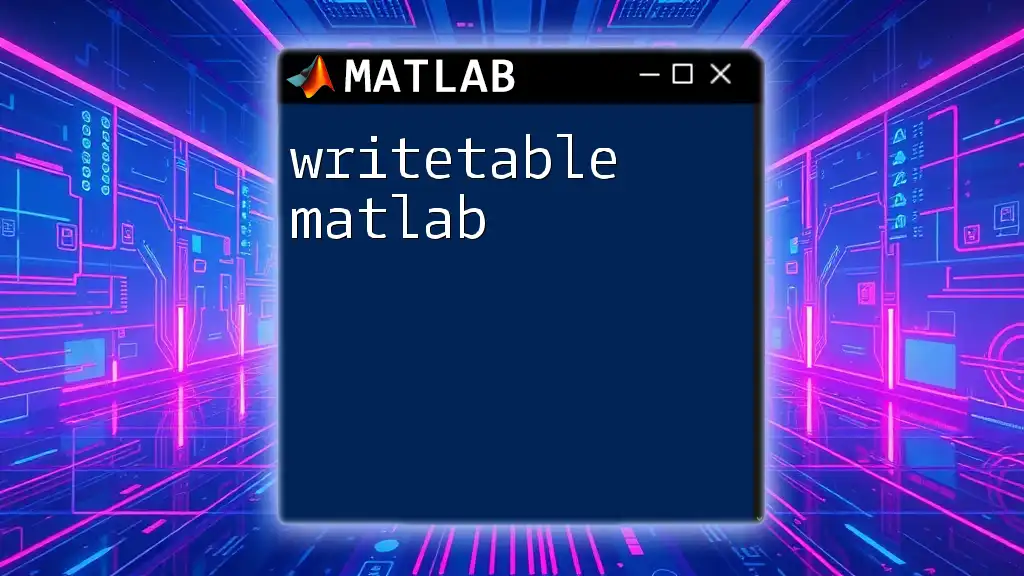
Advanced Title Customization
Using LaTeX for Title Formatting
For users seeking more flexibility in formatting, MATLAB allows the use of LaTeX-like syntax to enhance title presentation. This capability lets you create complex titles easily:
title('This is \textbf{Bold} and \textit{Italic}', 'Interpreter', 'latex');
Using LaTeX commands, you can create titles that incorporate mathematical expressions or styled text, making your graph more engaging and informative.
Dynamic Titles
Adding interactivity and dynamic elements can keep your graphs fresh and informative. You can include calculated values in titles, which can be particularly useful for presenting ongoing analysis:
averageValue = mean(y);
title(['Average Value: ', num2str(averageValue)]);
By incorporating variable data directly into the title, you ensure that your audience always has access to the most relevant information.
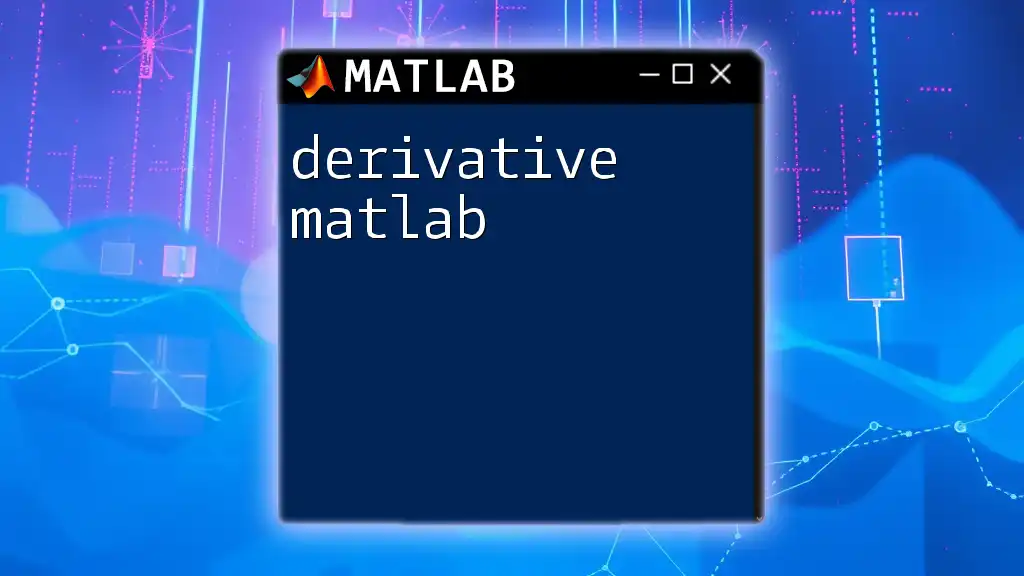
Common Mistakes to Avoid
Overcomplicating Titles
While it's tempting to be creative with titles, it’s crucial to maintain clarity and brevity. Overly complex titles can confuse viewers rather than inform them. Aim for a balance between creativity and practicality.
Ignoring Audience Understanding
Always consider the audience when crafting titles. Avoid jargon unless you are certain your readers are familiar with the terminology. Tailoring your language ensures that your audience can easily grasp the information presented.
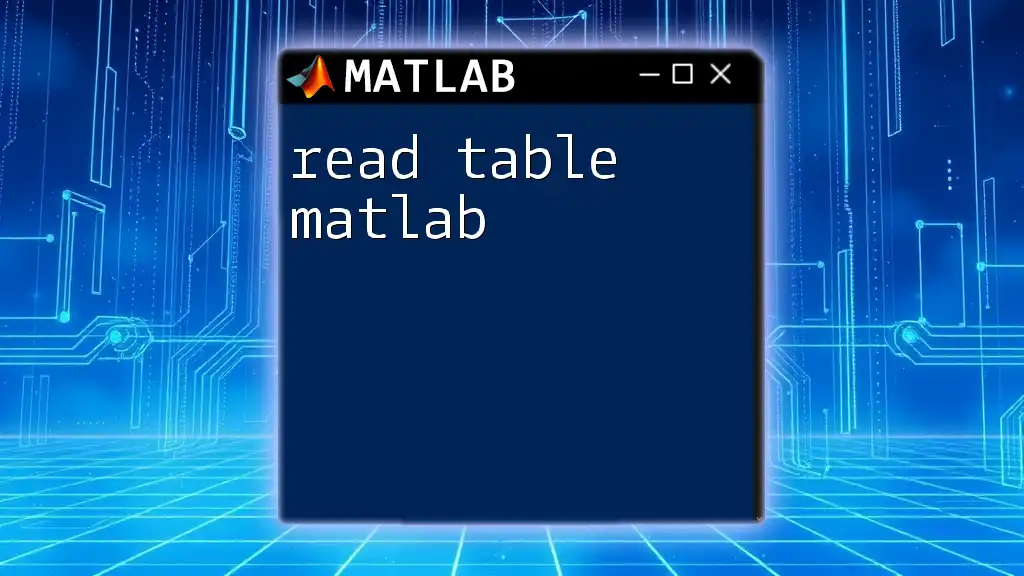
Case Studies and Examples
Example 1: Simple Line Graph with Title
Here’s a simple step-by-step creation of a line plot with an appropriate title. Start by defining your data:
x = 1:10;
y = sin(x);
Then plot the data and add a title:
plot(x, y);
title('Sine Wave');
This yields a clear, straightforward graph that accurately describes the content it represents.
Example 2: Bar Graph with Customized Title
Consider the following example of creating a bar graph with enhanced title customization:
data = [3, 5, 2, 7];
bar(data);
title('Performance Metrics for Q1', 'FontSize', 16, 'Color', 'b');
Here, the title is larger and blue, making it stand out. This attention to detail enhances the viewer's experience and aids in data interpretation.
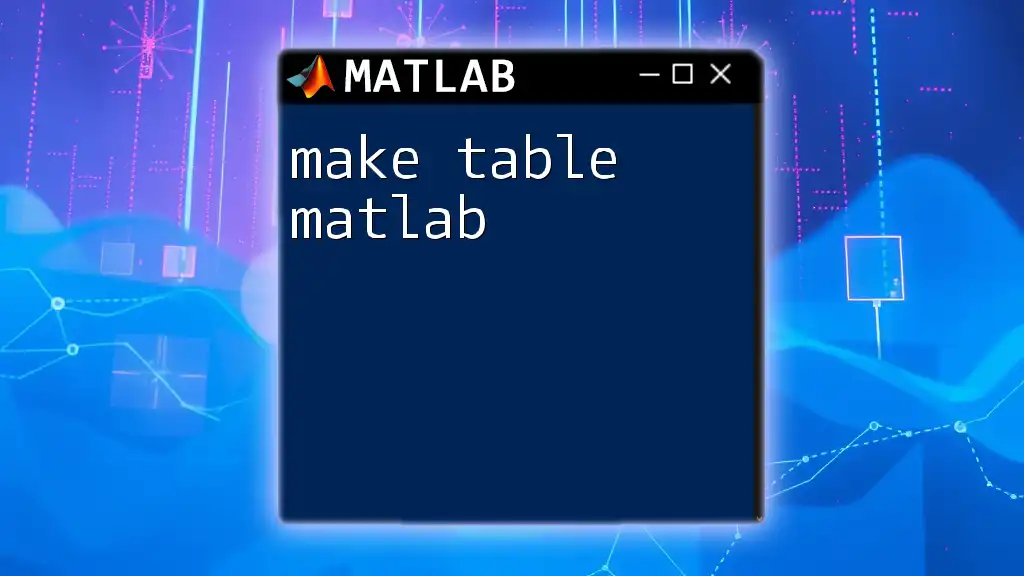
Conclusion
In conclusion, understanding how to effectively use graph titles in MATLAB is essential for clear and engaging data visualization. Crafting informative and visually appealing titles not only enhances viewer engagement but also improves data retention and interpretation. As you continue to experiment, consider how titles can elevate your work—making complex information easily accessible.
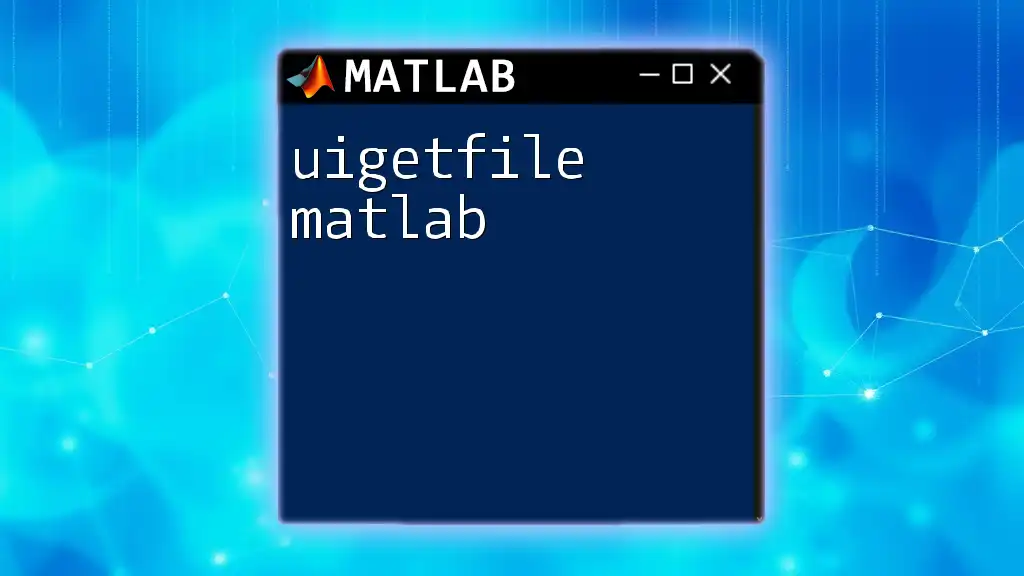
Further Resources
To deepen your understanding of graph titles in MATLAB, you can consult the official MATLAB documentation on the `title` function. Various online tutorials and platforms also offer additional insights, helping you practice and refine your skills in MATLAB graphing.
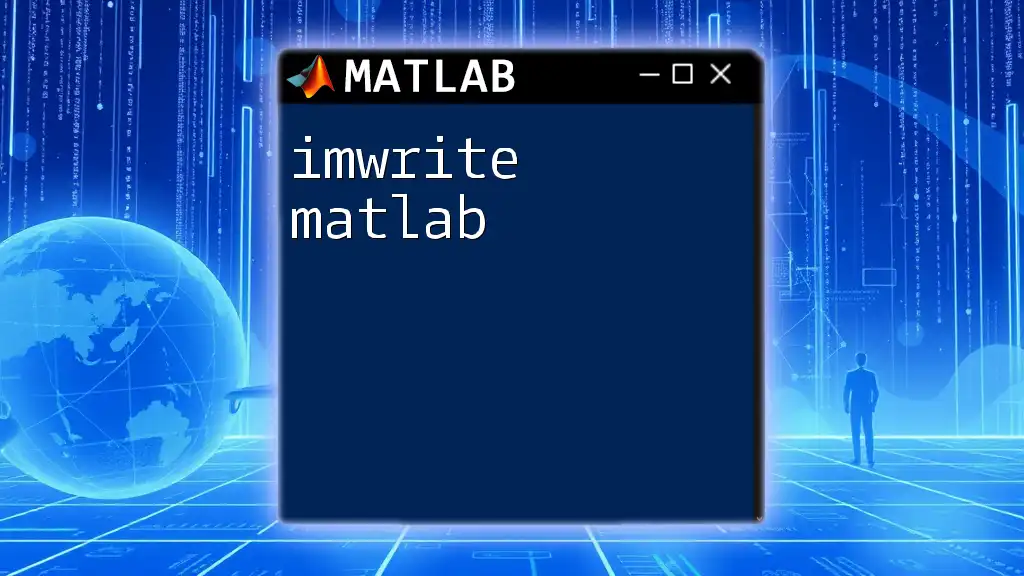
FAQs
How do I change the title after plotting?
You can easily update a graph's title at any time with the following command:
title('Updated Title');
Can I add line breaks in a title?
Indeed! You can create line breaks within a title using the `newline` function:
title('Line 1\nLine 2', 'Interpreter', 'none');
This feature allows for greater flexibility in how information is presented, enhancing clarity and impact.