A MATLAB 3D bar plot visually represents multivariate data using vertical bars in a 3D coordinate system, allowing for the comparison of several variables across different categories.
Here’s a simple code snippet to create a 3D bar plot:
% Sample data for a 3D bar plot
data = rand(4,3); % Generate random data
bar3(data); % Create a 3D bar plot
xlabel('Category');
ylabel('Series');
zlabel('Values');
title('3D Bar Plot Example');
Understanding 3D Bar Plots in MATLAB
What is a 3D Bar Plot?
A 3D bar plot is a graphical representation that allows you to visualize data across three dimensions. Unlike traditional 2D bar plots, a MATLAB 3D bar plot adds depth to your data presentation, making it easier to interpret complex datasets.
Importance of 3D Bar Plots
The significance of using a 3D bar plot lies in its ability to convey additional information. These plots are particularly useful in data scenarios where you need to present relationships among three different variables. Accordingly, they are often utilized in business analytics, scientific research, and education to visualize results and distributions clearly.
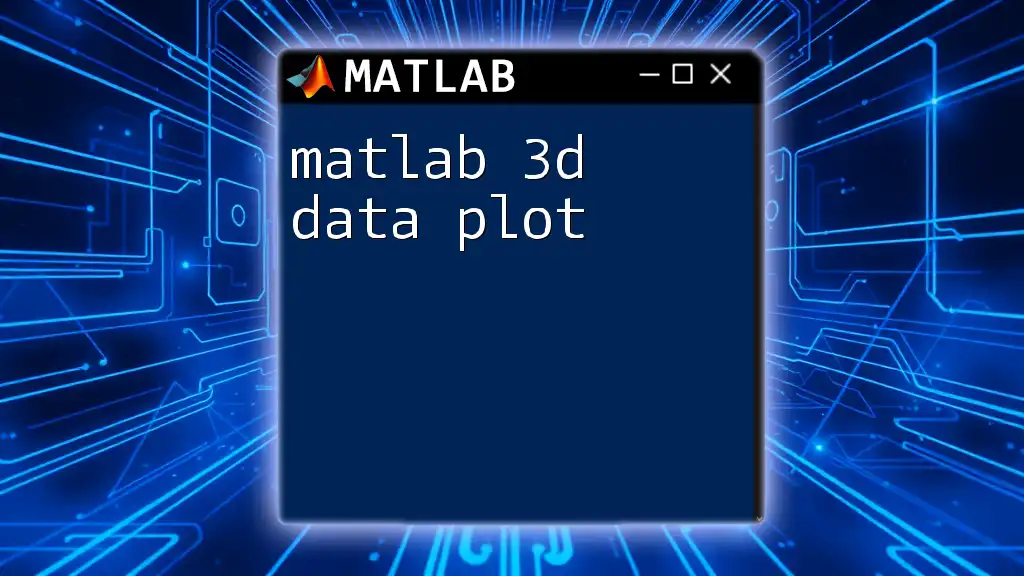
Getting Started with 3D Bar Plots in MATLAB
Prerequisites
Before diving into creating a MATLAB 3D bar plot, it's essential to have a basic understanding of MATLAB syntax and be familiar with matrices and arrays. This knowledge will help you manage data efficiently and utilize MATLAB's myriad functions effectively.
Setting Up MATLAB Environment
Ensure that your MATLAB software is installed correctly, as well as any additional toolboxes that might be required for advanced plotting. You may check for these toolboxes in the Add-Ons section in the MATLAB interface.
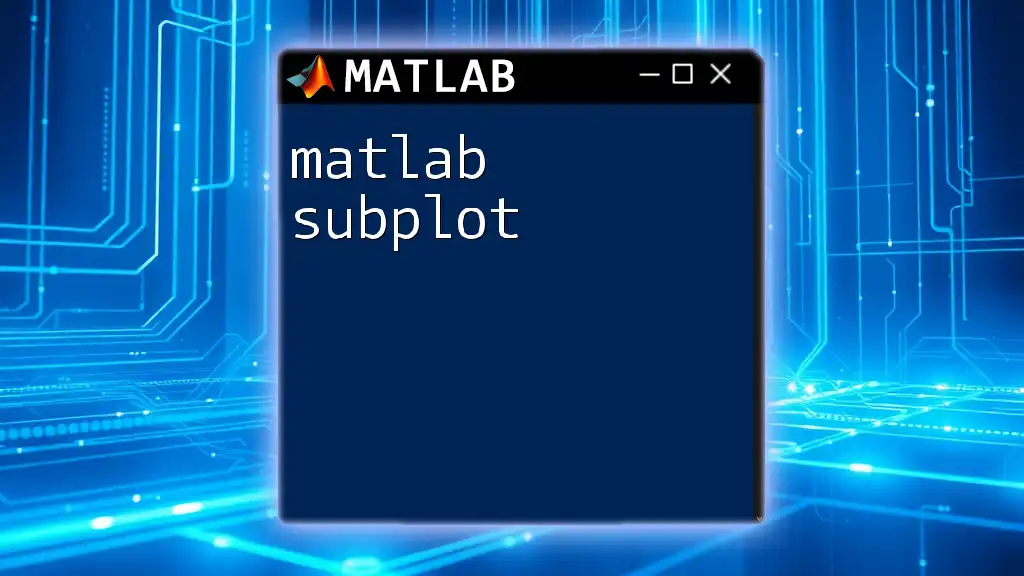
Creating Your First 3D Bar Plot
Basic Structure of a 3D Bar Plot
The core function for creating a 3D bar plot in MATLAB is the `bar3` function. The basic syntax is:
bar3(Z)
Here, `Z` is a matrix, where each element corresponds to the height of the bars at respective coordinates.
Step-by-Step Example
To create your first 3D bar plot, start by preparing some sample data. For instance, you might want to create a matrix like this:
Z = [1 2 3; 4 5 6; 7 8 9];
The subsequent step is to generate a visual representation of this matrix. You can do this using the following command:
figure;
bar3(Z)
When you run this code, MATLAB generates a 3D bar plot where the heights of the bars are determined by the values in matrix `Z`. The resulting plot helps you to visualize how the data varies in three dimensions.
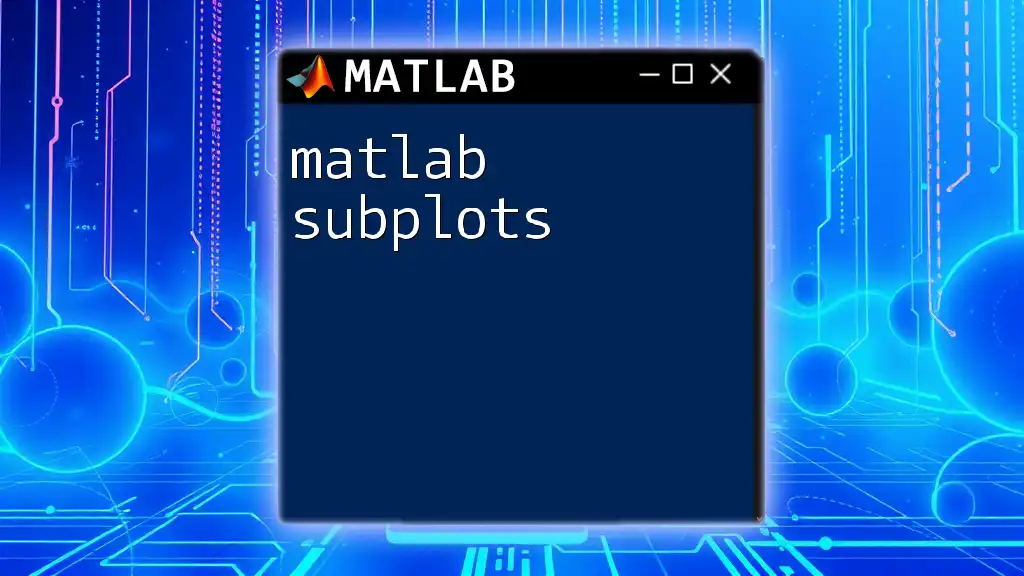
Customizing Your 3D Bar Plots
Adding Titles and Labels
A well-structured plot is incomplete without proper titles and axis labels. These elements enhance the clarity and meaning of your visualization. You can easily add titles and labels with the following commands:
title('My 3D Bar Plot');
xlabel('X-axis label');
ylabel('Y-axis label');
zlabel('Z-axis label');
Including these annotations can significantly enhance your audience's understanding of your data.
Modifying Colors and Styles
Another advantage of utilizing a MATLAB 3D bar plot is the ability to customize colors and styles. You can change the color of the bars using the `set` function. Below is an example:
h = bar3(Z);
for k = 1:length(h)
set(h(k), 'FaceColor', rand(1,3)); % Random color
end
In this instance, each bar is assigned a random color, adding a visually appealing element to your plot. You can also refer to predefined color palettes to maintain a professional aesthetic.
Adjusting Bar Width and Edge Color
Customizing the width and edge color of the bars can help emphasize different aspects of your data. For instance, to modify the width and set the edge color, you may use:
set(h(k), 'BarWidth', 0.5);
set(h(k), 'EdgeColor', 'k'); % Black edges
By adjusting these attributes, you can tailor your plot according to your preferences or specific requirements.
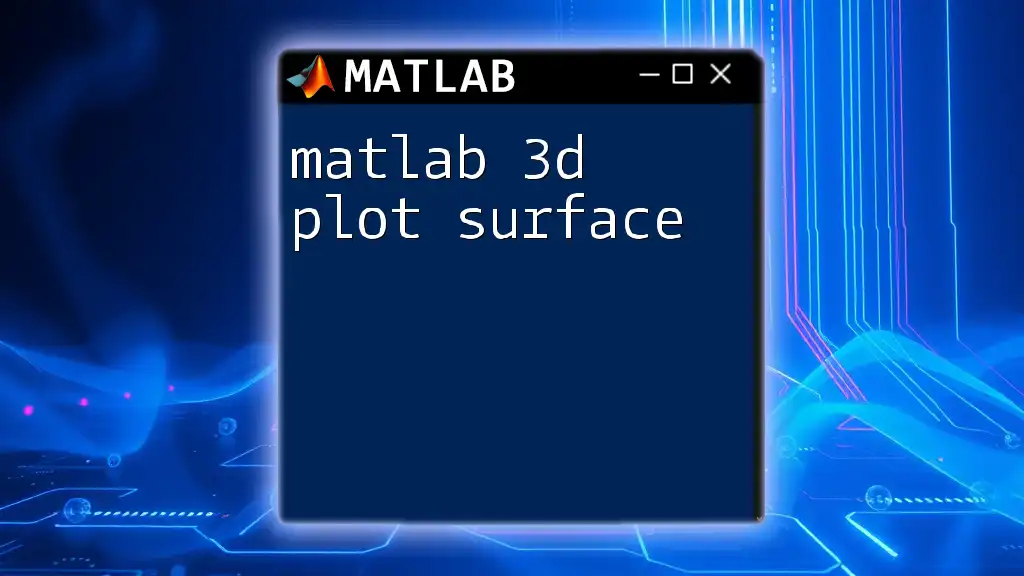
Advanced Features of 3D Bar Plots
Adding Annotations and Legends
Adding annotations can significantly improve the comprehensibility of your plot by providing context to specific data points. To include text annotations, use:
text(X,Y,Z,'label');
Moreover, creating a legend is especially beneficial when displaying multiple datasets in a single plot, allowing viewers to easily distinguish between them.
Combining Multiple Data Sets
You may find it useful to overlay multiple datasets in a single MATLAB 3D bar plot. This offers a comparative view of different sets of data. For example, you may use:
bar3(Z1);
hold on;
bar3(Z2);
hold off;
The `hold on` command allows you to add another set of bars to the existing plot, showcasing the relationship between these data points.
Exporting 3D Bar Plots
Once you’ve created your plot, you might want to share or include it in reports. MATLAB allows you to save your figures in various formats:
saveas(gcf, 'my3DBarPlot.png');
Choosing the appropriate format (such as PNG, JPEG, or even EPS) is crucial depending on your end-use, whether for online publication or traditional printing.
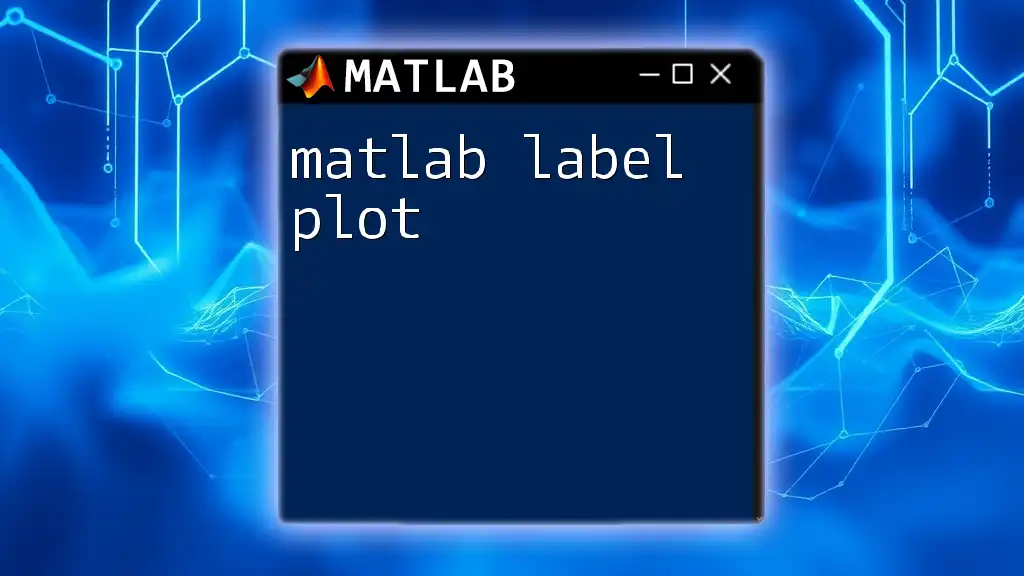
Troubleshooting Common Issues
Incorrect Display of Bar Plots
If your 3D bar plot does not display correctly, it could be due to a variety of reasons, such as issues related to data dimensions or MATLAB settings. Ensuring the proper dimensions of matrix `Z` is key to avoiding graphical problems.
Performance Optimization for Large Data Sets
Working with large datasets can slow down MATLAB’s plotting features. To enhance performance, one technique is to simplify your dataset or reduce the resolution when necessary. This can be particularly helpful for quick evaluations or presentations.
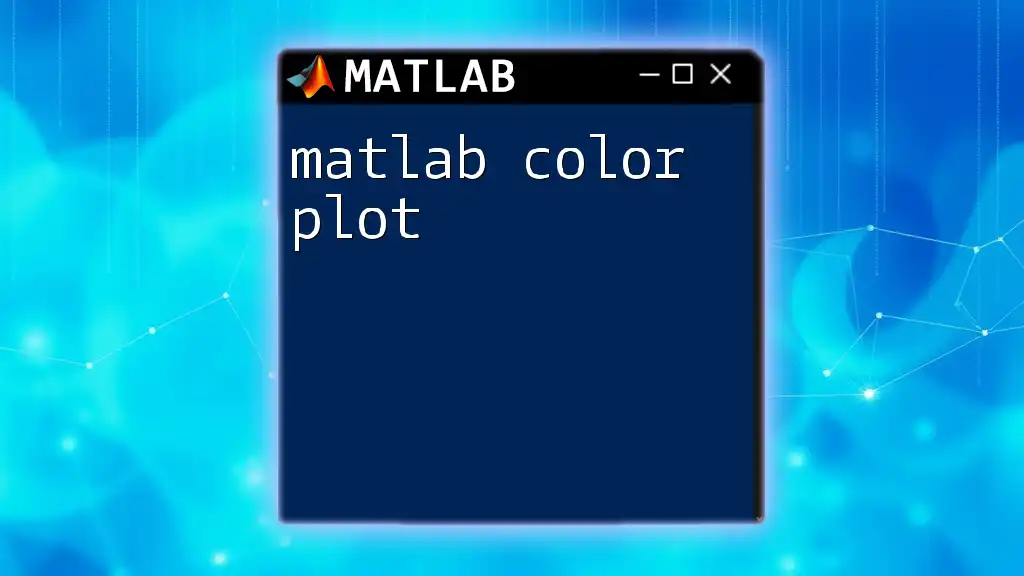
Conclusion
Recap of Important Points
In summary, MATLAB 3D bar plots are indispensable tools for visualizing data across three dimensions. They enhance understanding, provide clarity, and can be customized to meet specific needs.
Next Steps
Encourage your readers to explore further by trying out their own 3D bar plots and experimenting with different datasets and customizations. With practice, they will become adept at creating compelling visual narratives from their data.
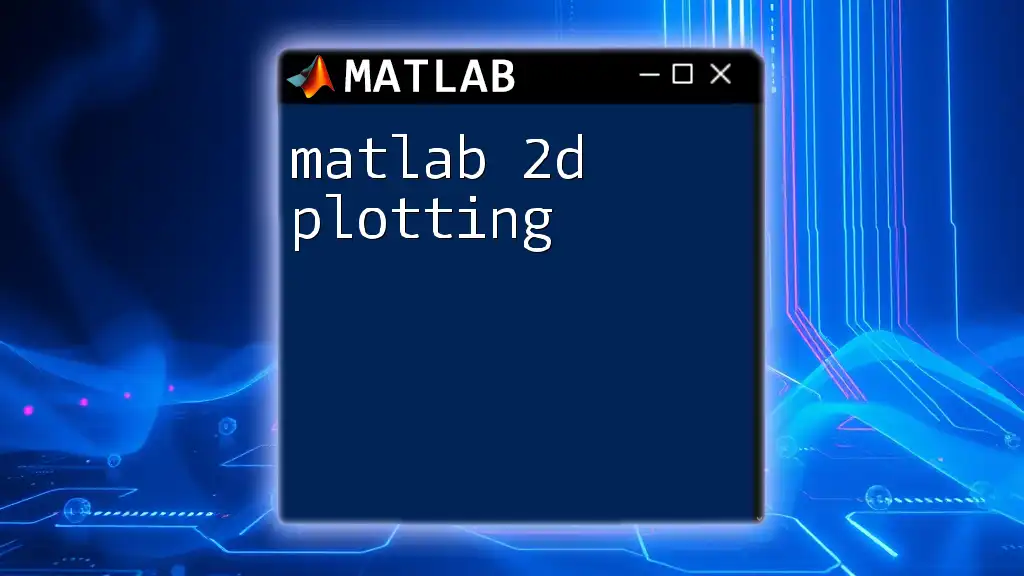
Additional Resources
Recommended Reading
To deepen your understanding, consider exploring textbooks and online courses focused on data visualization and MATLAB programming.
MATLAB Community and Forums
Leveraging community resources, such as MATLAB forums and user groups, can greatly enhance your knowledge and provide practical insights into advanced plotting techniques.