A MATLAB color plot allows you to visualize data using color to represent values, enhancing the interpretation of complex datasets.
Here’s a simple example using a scatter plot:
x = 1:10; % X data
y = rand(1, 10); % Random Y data
colors = linspace(1, 10, 10); % Color values
scatter(x, y, 100, colors, 'filled'); % Create scatter plot with color gradient
colorbar; % Add color bar to indicate the color scale
Understanding Color Mapping in MATLAB
Basics of Color Mapping
Color mapping is a foundational concept in data visualization that helps translate scalar values into colors. In MATLAB, colors are often used in matrices and surface plots to provide a visual interpretation of data, making it easier to understand complex datasets. By mapping ranges of values to specific colors, users can quickly identify patterns, anomalies, and trends.
Commonly Used Colormaps
MATLAB offers a variety of colormaps which can significantly enhance your color plots. Here are some of the commonly used colormaps:
- Jet: A rainbow color map that transitions from blue through cyan, green, yellow, and red. It's visually appealing but can sometimes mislead the viewer due to its uneven color distribution.
- Hot: Ranges from black to red to yellow, making it great for emphasizing areas of high intensity.
- Cool: A gradient from cyan to magenta, offering a good alternative for subtle visualizations.
- Parula: The default colormap in MATLAB, which provides a perceptually uniform mapping perfect for displaying data without a biasing effect.
Choosing the right colormap is essential for effective data communication. To illustrate how to display the default colormap in MATLAB, you can use the following code:
colormap('parula');
imagesc(peaks);
colorbar;
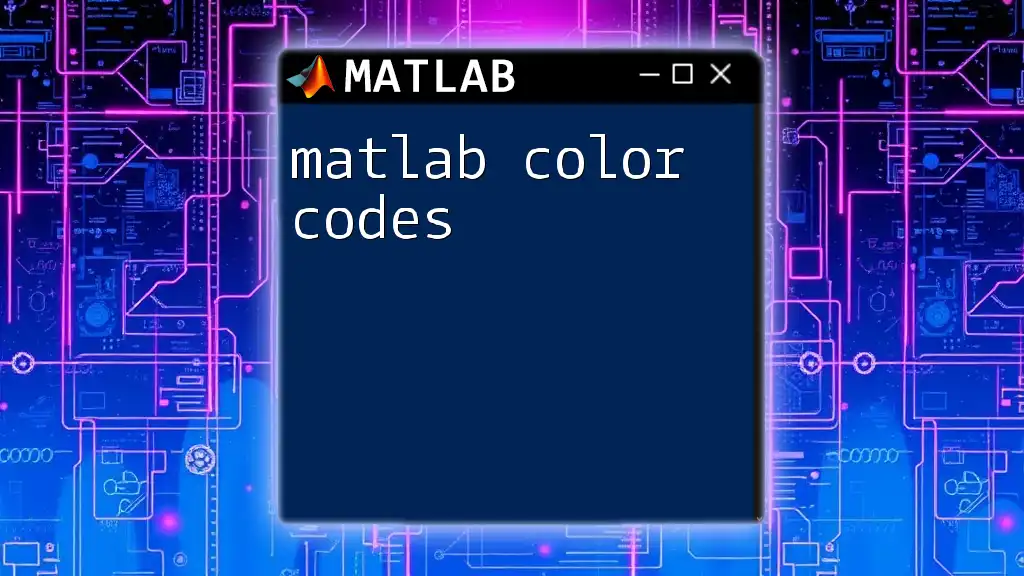
Creating Basic Color Plots
Using `imagesc` to Display Matrices
The `imagesc` function is a powerful tool in MATLAB for displaying matrix data as images where the color represents the magnitude of the data. It automatically scales the display based on the min and max values of your data, which is incredibly useful for quickly analyzing datasets.
To create a simple heatmap using random data, you can implement the following code:
data = rand(10); % Generating random data
imagesc(data);
colorbar; % Show color scale
In this example, each cell's color reflects the corresponding value in the matrix, allowing for an immediate visual representation of data distributions.
Using `surf` for 3D Surface Plots
For a more dynamic visualization, the `surf` function allows you to create 3D surface plots. This provides an impressive view of the relationships between data points in three-dimensional space.
Here’s a sample code snippet to demonstrate this:
[X, Y, Z] = peaks(30);
surf(X, Y, Z, 'EdgeColor', 'none');
colormap(jet);
colorbar;
By omitting the edges (`'EdgeColor', 'none'`), the plot focuses purely on the data surface colors, which effectively communicates trends in the data elevations.
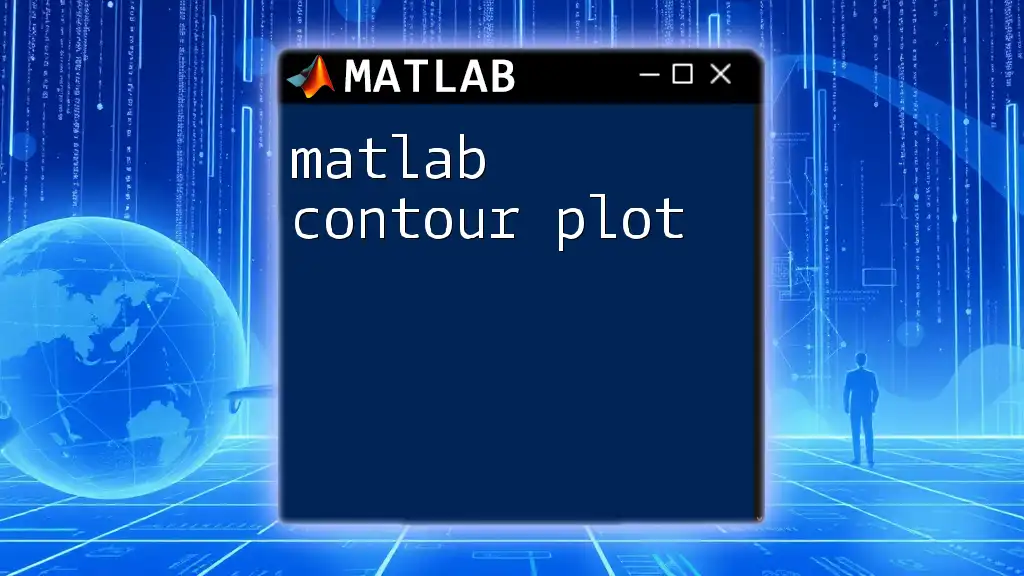
Advanced Color Plot Techniques
Customizing Color Scales
To make your visualizations clearer, customizing the color scales allows for better data representation. By default, MATLAB adjusts color limits automatically, but in some cases, you may want to define these limits explicitly for clarity.
Consider the following example, where we adjust the color limits using `caxis`:
imagesc(data);
caxis([0 0.5]); % Set color limits
colorbar;
Setting the `caxis` limits can highlight certain ranges of your data effectively, leading to better insights during analysis.
Creating Contour Plots with Color
Contour plots provide an alternative to visualize data distributions through contour lines, where different regions of the same value are highlighted.
This method is particularly useful for understanding intricate details in datasets. Here’s how you can create a colored contour plot with a sample dataset:
[X, Y] = meshgrid(-3:0.5:3, -3:0.5:3);
Z = X.^2 + Y.^2;
contourf(X, Y, Z, 20); % 20 contour levels
colorbar;
In this example, the `contourf` function fills the regions between contour lines, creating a vivid representation of the data.
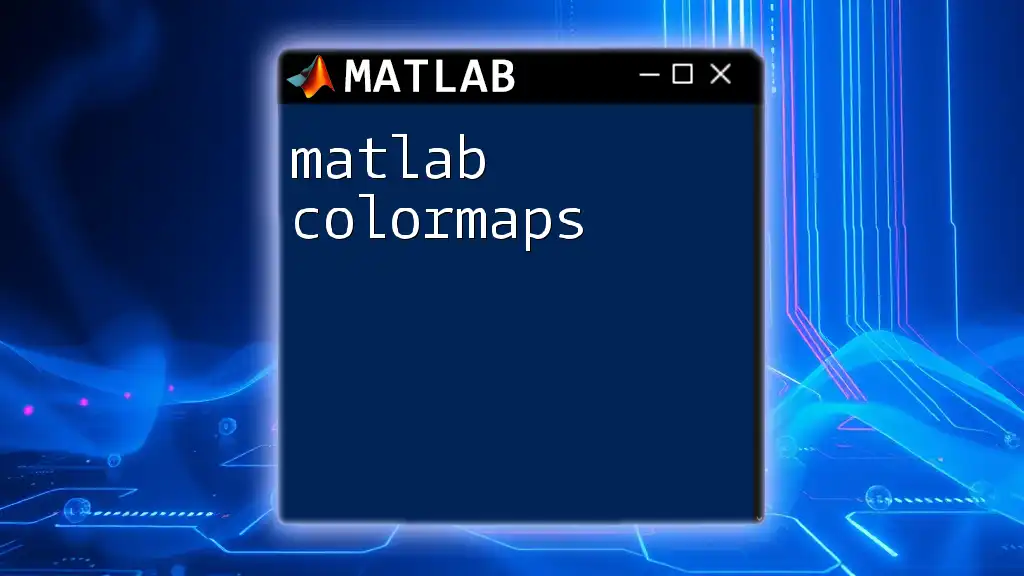
Interactive Color Plots in MATLAB
Using `heatmap` for Enhanced Visualization
The `heatmap` function delivers a modern visualization method and comes with interactive features. It seamlessly allows you to display, explore, and assess your data visually.
Here’s how you can use the `heatmap` function for displaying matrix data:
data = rand(10);
h = heatmap(data);
h.Colormap = parula; % Set custom colormap
This function offers customizable features such as tooltips that provide additional context about the data at any point on the plot.
Creating Dynamic Color Plots with GUI
MATLAB's graphical user interface (GUI) capabilities allow even greater interactivity in your color plots. Using MATLAB apps such as `plotedit`, users can easily modify plots, adjust colors, and explore their datasets dynamically.
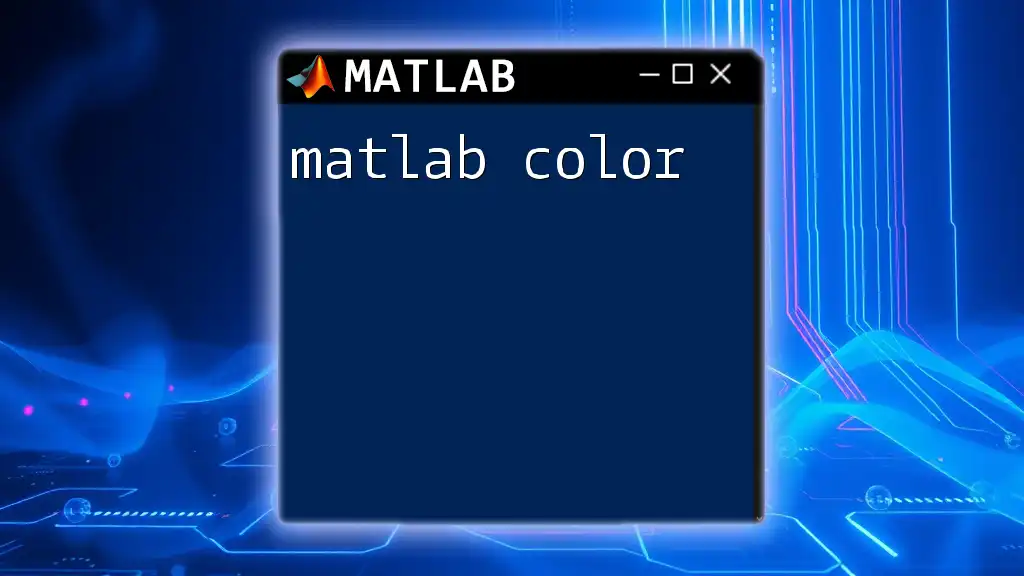
Exporting Color Plots for Reports
Once your color plots are ready, exporting them properly is essential, especially for reports and presentations. MATLAB allows you to save figures in various formats, optimizing for both quality and resolution.
An example of saving a figure as a PNG file looks like this:
print('MyColorPlot', '-dpng'); % Save as PNG file
Choosing the right resolution and format ensures that your visualizations remain clear and communicative when integrated into your work.
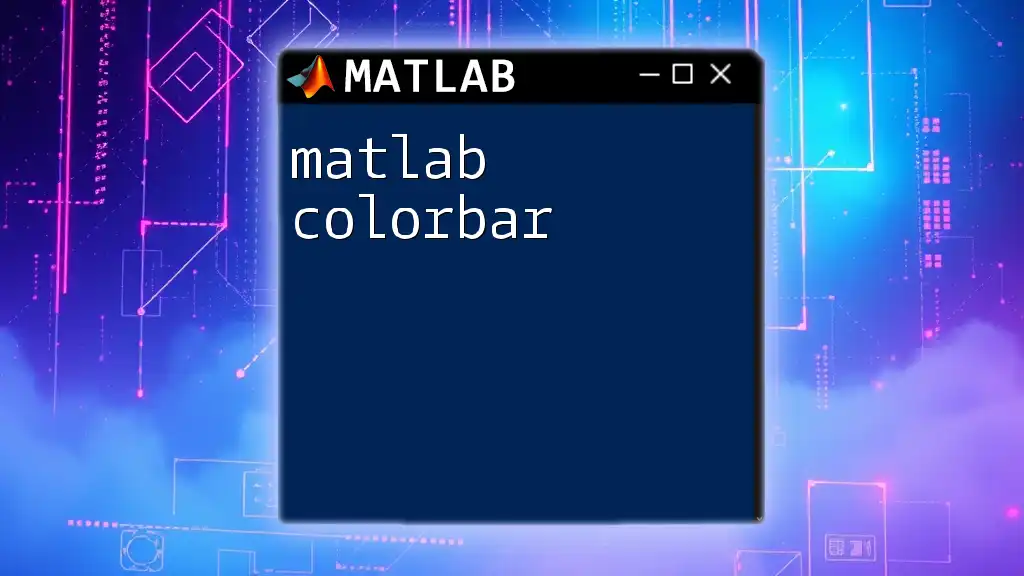
Common Pitfalls and Troubleshooting
Misinterpretation of Color Ranges
One of the most significant challenges in visualizing data through color plots is the misinterpretation of color ranges. Some colormaps can distort data perceptions, leading to incorrect assumptions. Hence, being mindful of colormap selection is crucial for clarity.
Performance Issues with Large Datasets
While MATLAB handles a wide array of datasets, performance can slow down significantly with very large matrices. To combat this, consider using downscaling techniques or sampling your data to provide insight without overwhelming the computational resources.
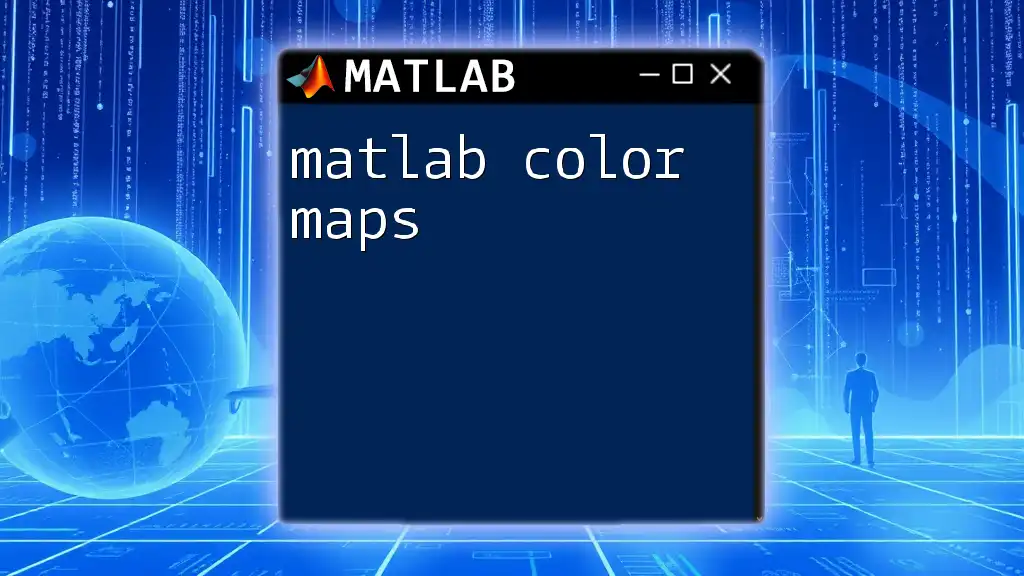
Conclusion
In conclusion, mastering MATLAB color plots can profoundly enhance your ability to visualize and interpret data effectively. With an array of functions, such as `imagesc`, `surf`, and `heatmap`, you have numerous tools at your disposal to create visually compelling narratives from your data. Experiment with different colormaps and techniques, and engage with the community to further your understanding and skills in MATLAB.
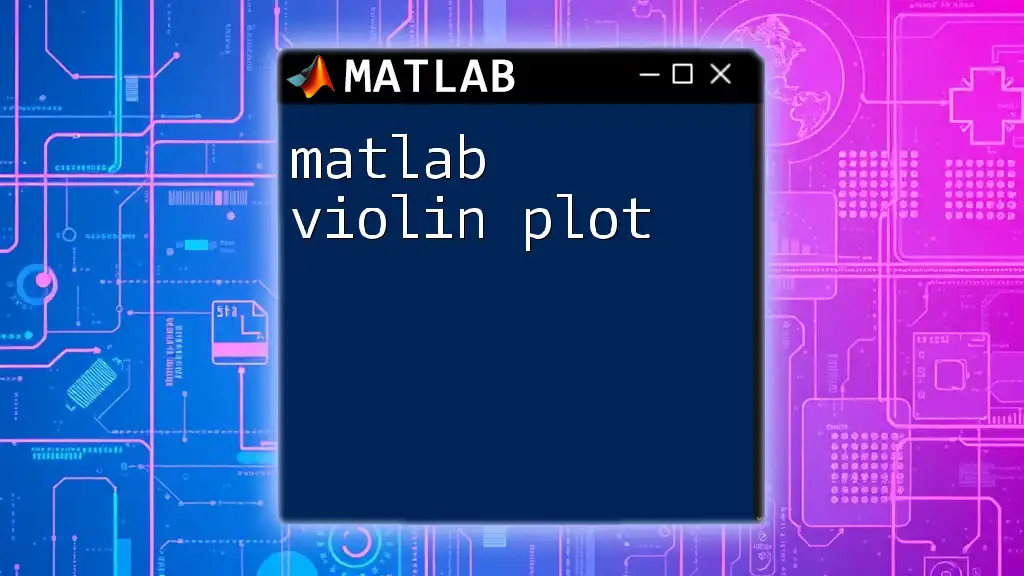
Further Resources
For additional learning, consider diving into MATLAB's official documentation and available tutorials that cover color plotting in depth. Additionally, engaging with forums and online communities can open up opportunities for collaborative learning and support as you explore the world of MATLAB visualizations.
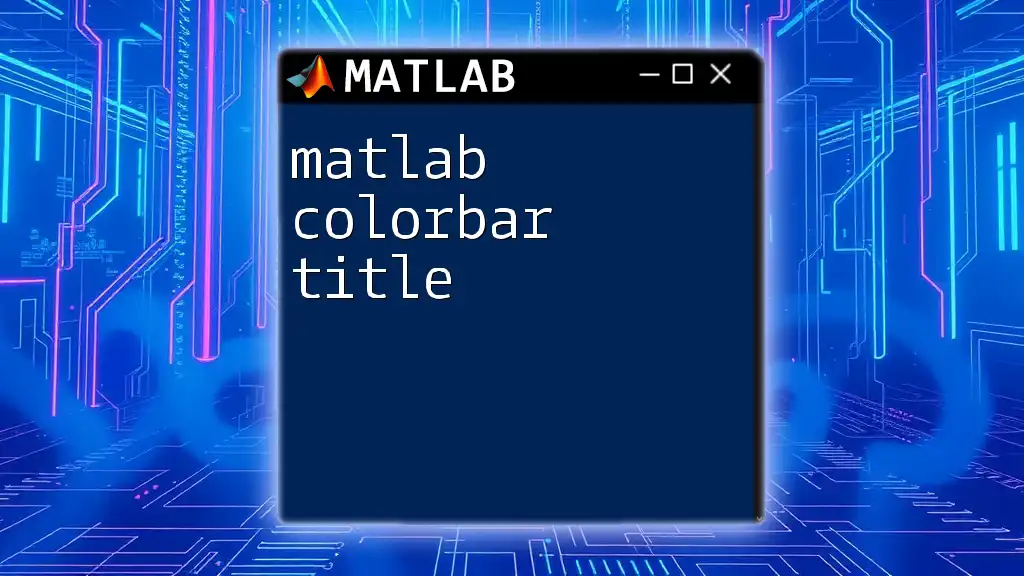
Call to Action
Stay updated by subscribing to our blog for more concise MATLAB tips and tricks. Share your creations using color plots, and let's foster a community of learning and innovation together!