To add a column to a MATLAB table, you can simply assign a new variable to the table using dot notation or by using the `addvars` function.
Here’s an example using dot notation:
% Create an example table
T = table([1; 2; 3], ['A'; 'B'; 'C'], 'VariableNames', {'ID', 'Letter'});
% Add a new column named 'Value'
T.Value = [10; 20; 30];
And here’s an example using the `addvars` function:
% Create an example table
T = table([1; 2; 3], ['A'; 'B'; 'C'], 'VariableNames', {'ID', 'Letter'});
% Add a new column named 'Value' at the end
T = addvars(T, [10; 20; 30], 'NewVariableNames', 'Value');
Understanding Tables in Matlab
What is a Matlab Table?
A Matlab table is a type of data structure that allows you to store data in a way that is easy to manage and manipulate. Each column in a table can contain data of a different type, making tables a versatile choice for organizing datasets. Unlike arrays that only support one data type, tables provide a more flexible way to work with varied types of data such as numeric values, text, or even categorical values.
Benefits of Using Tables
Tables offer several advantages over traditional arrays and cell arrays:
- Ease of Access: You can easily reference table columns by their variable names.
- Versatility: Each column can contain different data types, which is especially useful when working with complex datasets.
- Powerful Data Manipulation: Matlab provides built-in functions specifically designed for tables, enhancing your ability to handle and process data efficiently.
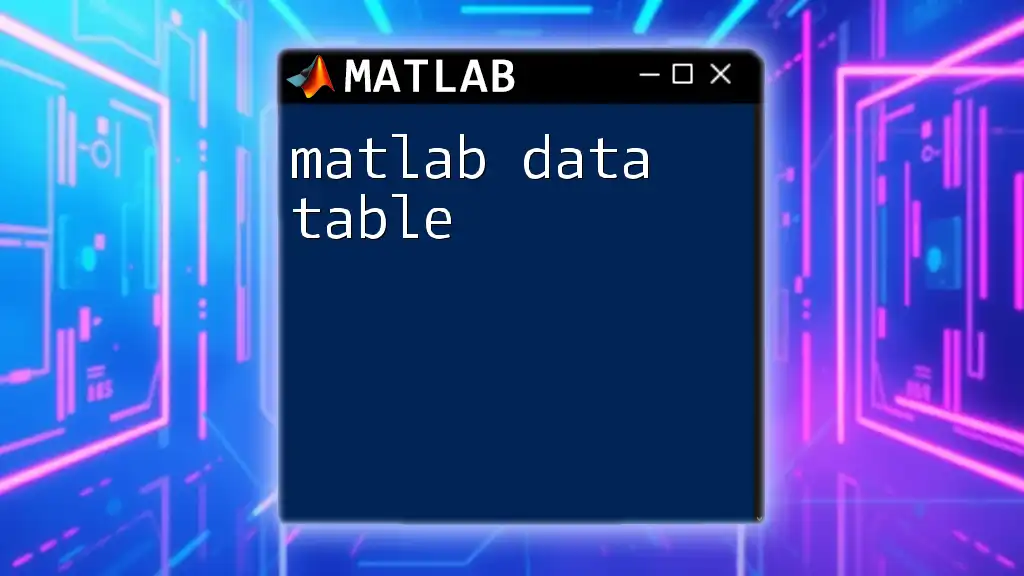
Basic Syntax for Adding a Column to a Table
General Syntax Breakdown
To add a new column to a table, you can simply assign a new value to a column name that does not yet exist. The basic syntax resembles:
T.NewColumnName = NewData;
This simple command will create a new column named "NewColumnName" in the table `T` and assign it the values contained in `NewData`.
Example of Basic Column Addition
Here is an example showing how to create a table and add a new column:
T = table([1; 2; 3], ['A'; 'B'; 'C'], 'VariableNames', {'ID', 'Letter'});
T.NewColumn = [10; 20; 30];
disp(T);
In this example:
- A table `T` is created with two columns: `ID` (numeric) and `Letter` (character).
- A new column `NewColumn` is added with numeric values.
- The `disp` function outputs the modified table to display the new structure.
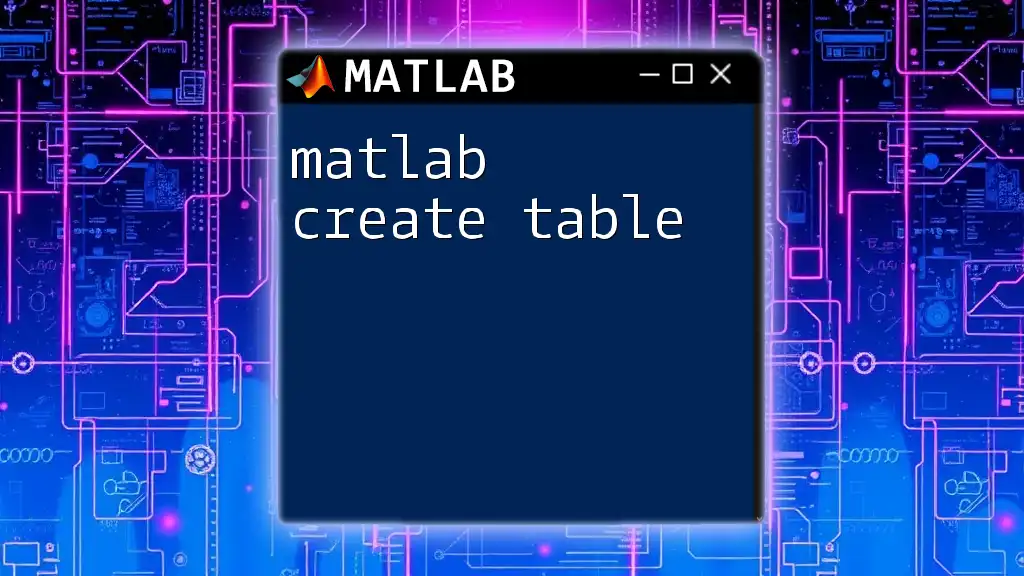
Methods for Adding a Column
Adding a Column with a Constant Value
If you want to add a column that contains the same constant value across all rows, you can do it as follows:
T.ConstantColumn = 5;
This command creates a new column `ConstantColumn`, which will have the value `5` for every entry in that column. This approach can be particularly useful when initializing columns for default values or when creating flags.
Adding a Column from an Array or Vector
Syntax and Example
You can also populate a new column using data from a separate array or vector:
NewData = [100; 200; 300];
T.NewDataColumn = NewData;
disp(T);
It’s crucial that the size of `NewData` matches the number of rows in `T`; otherwise, you will encounter an error. For example, if `T` has three rows, `NewData` must also have three elements.
Adding a Column Based on Calculations
Creating a New Column from Existing Columns
You can derive a new column from existing data within the same table. Here is an example:
T.Total = T.NewDataColumn + T.ConstantColumn;
In this scenario, a new column `Total` is created by adding values from `NewDataColumn` and `ConstantColumn`. This method is handy for performing operations on existing columns to derive new insights or calculations.
Adding Multiple Columns at Once
Syntax and Example
If you need to add multiple columns simultaneously, you can use the `addvars` function:
T = addvars(T, NewData1, NewData2, 'NewVariableNames', {'Var1', 'Var2'});
This syntax aids in adding `NewData1` and `NewData2` as new columns `Var1` and `Var2` respectively, which can significantly simplify your code when performing bulk operations.
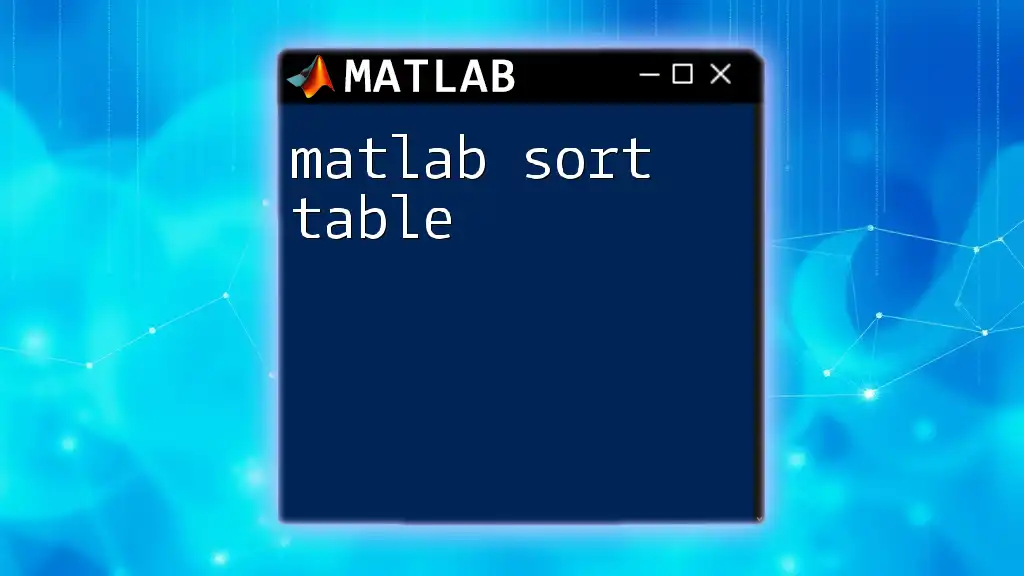
Verifying and Modifying Columns After Addition
How to Check the Structure of a Table
Once you have made modifications to your table, it’s beneficial to verify the changes. The `summary` and `head` functions can be used to evaluate your table structure:
summary(T);
head(T);
Using these functions provides a succinct overview of the contents in your table, confirming the success of any modifications.
Renaming Columns
If necessary, you can rename columns for clarity:
T.Properties.VariableNames{T.Properties.VariableNames == 'OldName'} = 'NewName';
Renaming a column ensures that your table is semantically clear, enhancing readability and making it easier to understand the data contained within.
Removing Columns
To remove a column that is no longer needed:
T.NewDataColumn = [];
This approach sets the column to an empty array, effectively deleting it from the table.
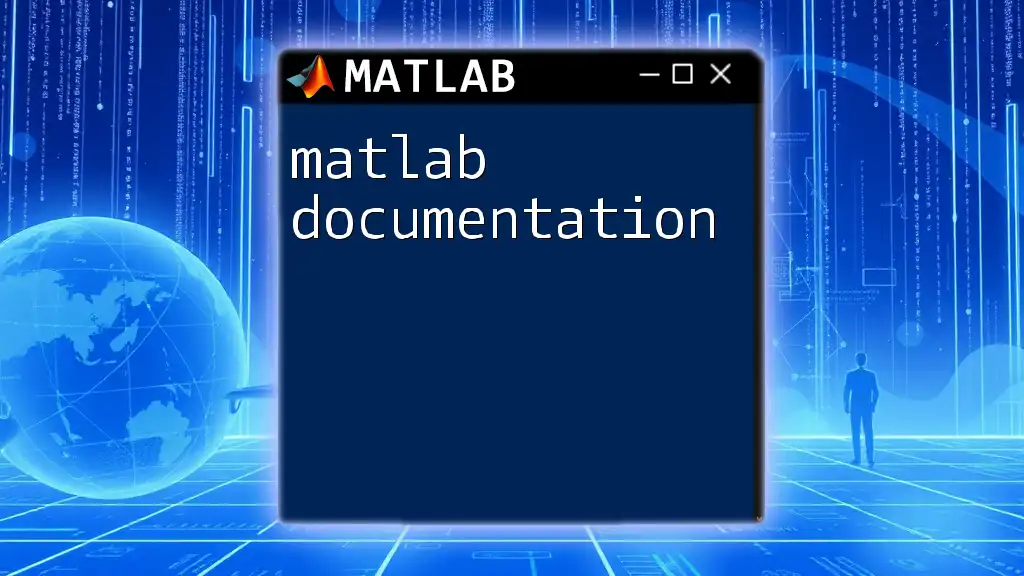
Practical Applications
Use Cases in Data Analysis
Adding columns to tables is an essential skill in data analysis. For example, if you are analyzing sales data, you may want to add a column for profit margins calculated from sales and cost data. By mastering how to add columns, you empower yourself to manipulate datasets dynamically as requirements change.
Tips for Efficient Table Management
- Avoiding Duplicates: Ensure that variable names are unique to prevent data overwrites unintentionally.
- Clear Variable Names: Use descriptive names for your columns to make it easier for yourself and others to understand the dataset later.
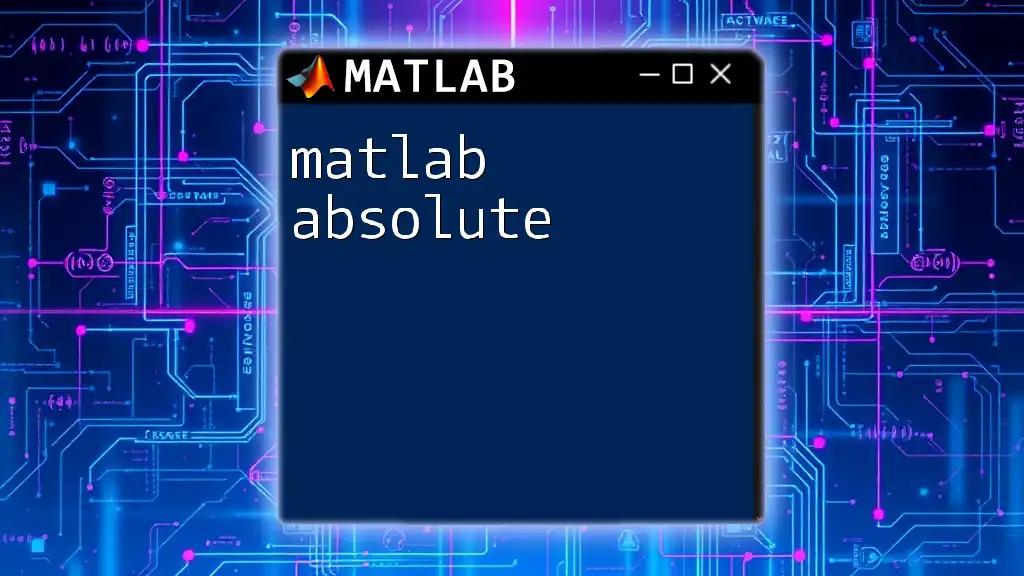
Troubleshooting Common Issues
Size Mismatch Errors
One common issue when adding columns is size mismatch errors. Always ensure that the new data you're trying to add has the same number of rows as the existing table. If not, Matlab will throw an error, preventing you from executing your commands successfully.
Debugging Incorrect Data Behavior
If you find that your data behaves unpredictably after adding new columns, consider reviewing your data types and sizes with the `class` and `size` functions. These can help identify mismatches before they become problematic.
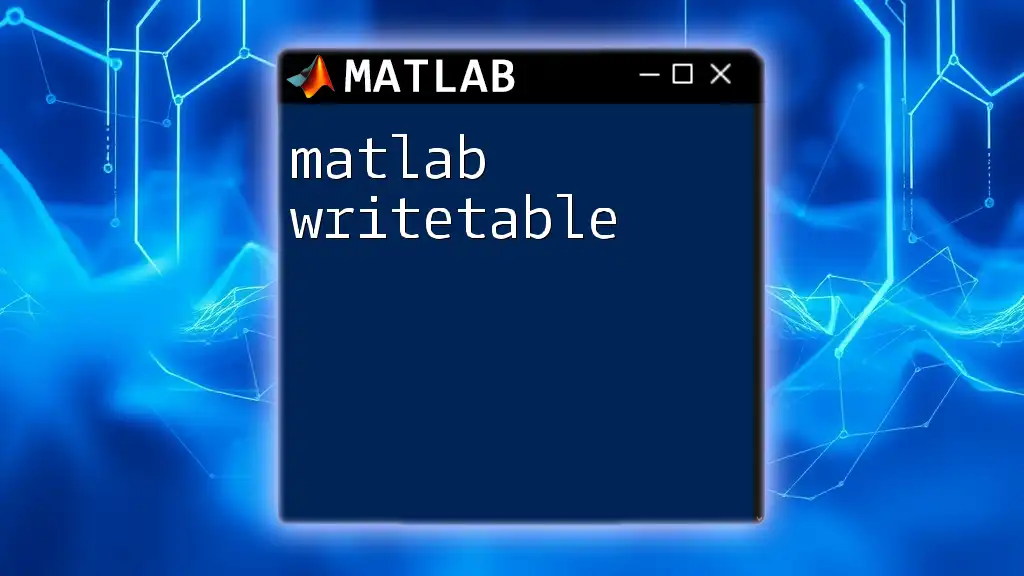
Conclusion
Mastering how to matlab add column to table is essential for efficient data manipulation and analysis in Matlab. The techniques and examples provided will aid in understanding how to modify tables effectively, providing you with the skills needed to handle complex datasets with ease. Practice with these examples to solidify your grasp on Matlab's table functionalities, and explore more advanced techniques as you become comfortable with these basics.
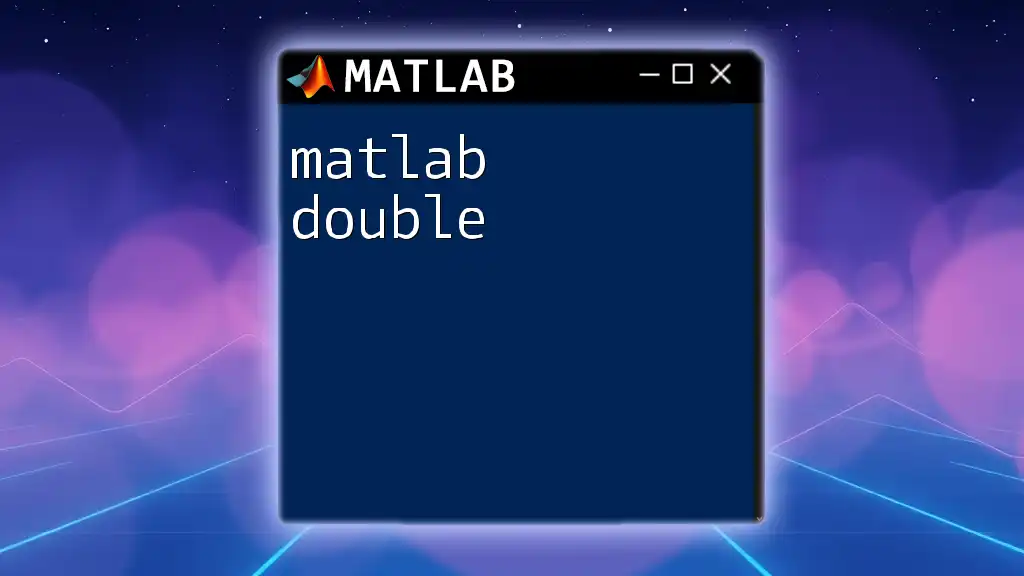
Additional Resources
For further exploration, consult the Matlab documentation on tables and consider completing exercises that challenge your new skills. Happy coding!