A MATLAB bar graph is a visual representation of categorical data using rectangular bars, where the heights of the bars represent the values of the data points.
Here’s a simple code snippet to create a bar graph in MATLAB:
% Sample data
categories = {'Category A', 'Category B', 'Category C'};
values = [10, 15, 7];
% Create bar graph
bar(values);
set(gca, 'XTickLabel', categories);
title('Sample Bar Graph');
xlabel('Categories');
ylabel('Values');
Introduction to Bar Graphs
What is a Bar Graph?
A bar graph is a visual representation of data in which individual bars stand for different categories or groups. Typically, the lengths or heights of the bars illustrate the magnitude or frequency of the data they represent. Bar graphs are particularly useful when comparing multiple datasets side by side, allowing for quick visual assessments of trends and differences.
Why Use MATLAB for Bar Graphs?
MATLAB stands out as a powerful tool for technical computing and data visualization. Its built-in functions allow users to create sophisticated bar graphs with ease. The advantages of using MATLAB for this purpose include:
- Flexibility: Users can create basic to complex visualizations and customize every element.
- Ease of Use: The straightforward syntax allows even beginners to produce accurate graphs efficiently.
- Integration with Analysis: MATLAB seamlessly integrates data analysis and visualization, making it an ideal choice for researchers and engineers.
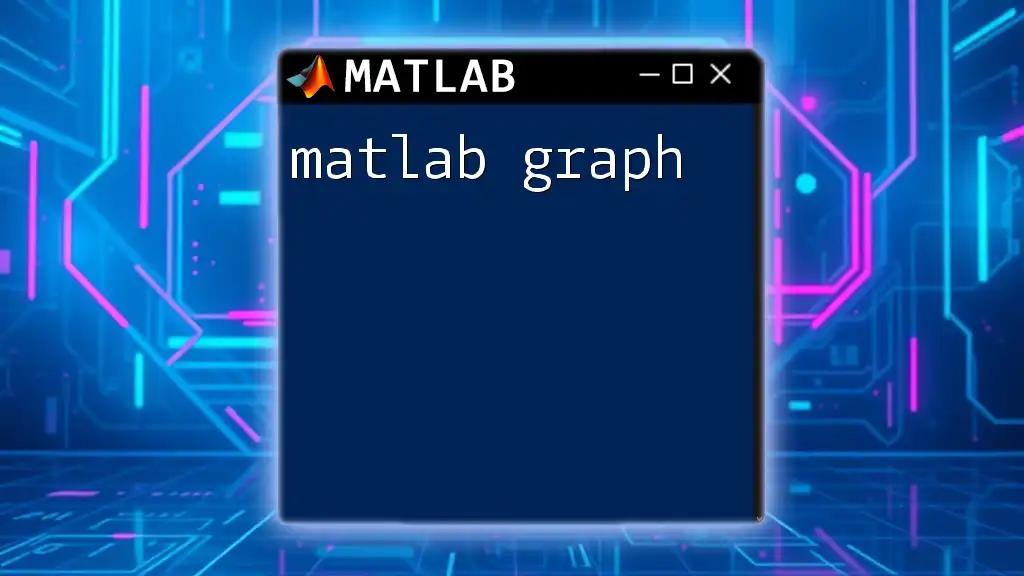
Setting Up the MATLAB Environment
Installing MATLAB
Before diving into creating bar graphs, you need to have MATLAB installed. Installation can be guided by following online resources provided by MathWorks or other tutorials. It's important to ensure that you have a compatible version of MATLAB that supports the commands and functions discussed.
Getting Familiar with the MATLAB Interface
Once MATLAB is installed, familiarizing yourself with its user interface is crucial. The Command Window is where you will enter commands, while the Workspace lets you view variables. Understanding the structure will help you navigate efficiently when plotting your data visually.
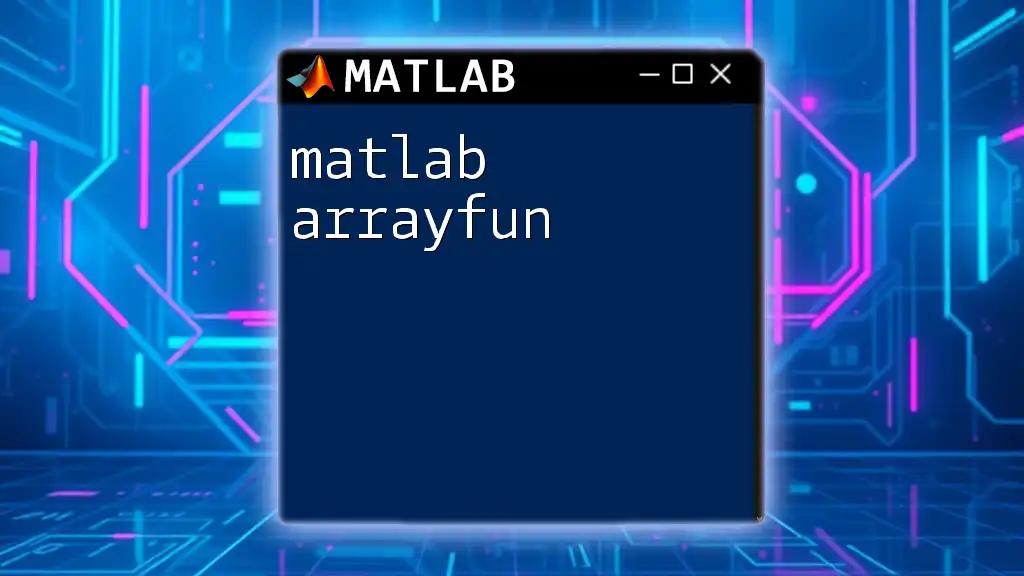
Creating Basic Bar Graphs
Understanding the `bar` Function
At the heart of creating bar graphs in MATLAB is the `bar` function. The simplest form of this function requires just one argument, the data array `Y`.
- Syntax: `bar(Y)`
- Y can be a vector (for a single data series) or a matrix (for grouped data).
Example: Creating a Simple Bar Graph
To illustrate the use of a basic bar graph, consider the following example:
% Example: Basic Bar Graph
data = [3, 5, 7, 2, 4];
bar(data);
title('Simple Bar Graph Example');
xlabel('Categories');
ylabel('Values');
In this example, MATLAB generates a bar graph where the heights of the bars are determined by the values in `data`. The graph is annotated with a title and labeled axes for clarity.
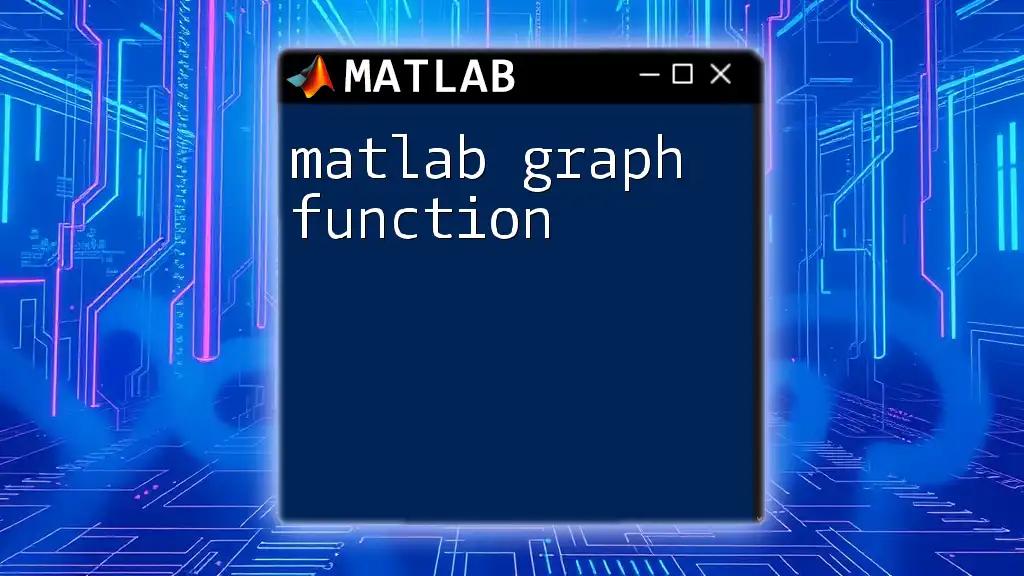
Customizing Bar Graphs
Changing Bar Colors
Customization can greatly enhance the effectiveness of a bar graph. For instance, changing the bar colors can help highlight important data. This can be done using the `FaceColor` property. Here’s how:
% Example: Custom Bar Colors
data = [3, 5, 7, 2, 4];
bar(data, 'FaceColor', 'cyan');
This example demonstrates how the bars can be colored cyan, enhancing their visual appeal and drawing attention to the data presented.
Adding Titles and Labels
The significance of titling and labeling in graphs cannot be overstated. They provide context and make your data comprehensible to the audience. Use the following snippet to add appropriate textual annotations to your graph:
title('Customized Bar Graph');
xlabel('Categories');
ylabel('Values');
Utilizing these functions ensures that anyone reading the graph understands what data it's representing.
Modifying Bar Width and Orientation
Creatively adjusting the bar's width can also affect readability. To change the width, simply add a second argument to the `bar` function call (value between 0 and 1). Alternatively, for horizontal bar graphs, you can use `barh`:
% Example: Horizontal Bar Graph
data = [3, 5, 7, 2, 4];
barh(data);
title('Horizontal Bar Graph Example');
This generates a horizontal bar graph, which can sometimes provide a clearer view of certain datasets, particularly when there are lengthy category names.
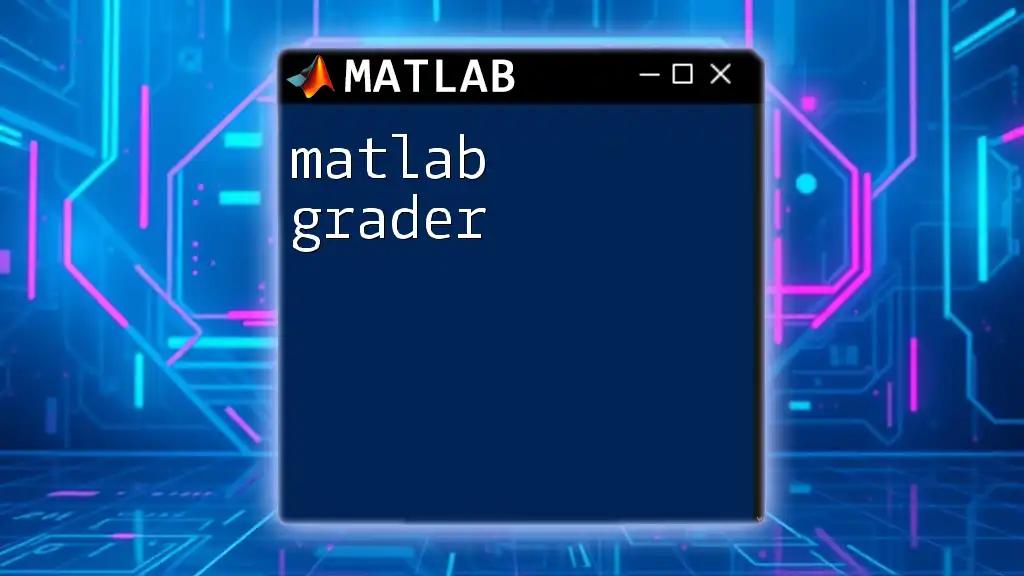
Advanced Customizations
Grouped Bar Graphs
When you want to compare multiple datasets, a grouped bar graph is invaluable. It visually distinguishes between different data series. Here’s an example of how to create a grouped bar graph:
% Example: Grouped Bar Graph
data = [3, 5, 7; 2, 4, 6];
bar(data);
title('Grouped Bar Graph');
xlabel('Categories');
legend('Group 1', 'Group 2');
In this example, two groups of data are plotted side by side, allowing for an immediate visual comparison.
Stacked Bar Graphs
Another effective way to display relationships between datasets is through stacked bar graphs. This representation shows various groups as segments of the same bar, enabling viewers to see the total directly, alongside individual contributions:
% Example: Stacked Bar Graph
data = [3, 5, 7; 2, 4, 6];
bar(data, 'stacked');
title('Stacked Bar Graph');
In this case, the `stacked` option provides immediate insights into how different segments contribute to the total values.
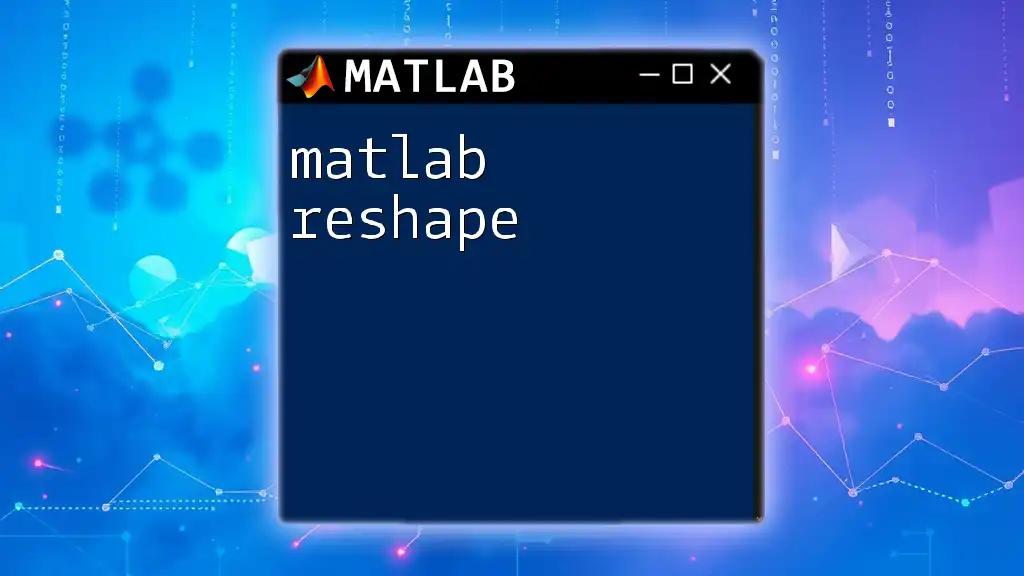
Customizing Axes and Ticks
Modifying Axis Limits
Setting clear limits on your axes can help users focus on the relevant data range. Using `xlim` and `ylim` functions, you can define specific axis boundaries:
% Example: Setting Axis Limits
xlim([0 10]);
ylim([0 8]);
These commands adjust the x-axis and y-axis limits, preventing clutter and highlighting the most important data.
Customizing Tick Marks and Labels
Custom tick marks create a more straightforward understanding of your data. You can personalize tick marks and their labels to make them more descriptive:
% Example: Custom Tick Marks
xticks([1 2 3]);
xticklabels({'A', 'B', 'C'});
In this example, the x-ticks have been customized to represent specific categories instead of numerical values, making the graph more intuitive.
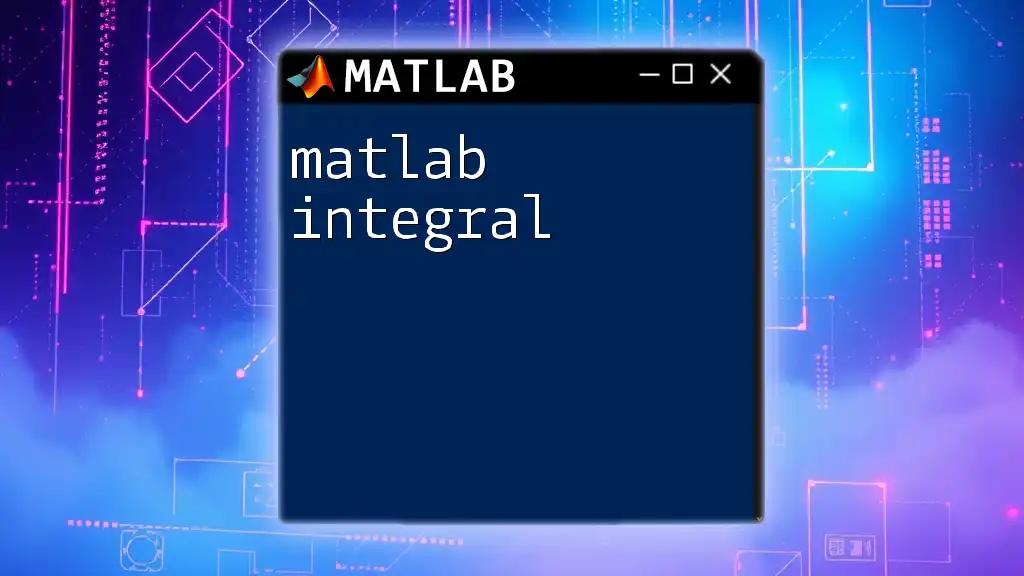
Adding Data Labels
Importance of Data Labels
Data labels on a bar graph enhance accessibility, providing exact values that contribute to a better understanding of the displayed data. This feature is particularly important when precise values matter most.
Example: Adding Data Labels to Bars
Incorporating data labels into your bar graph can be achieved with a simple loop:
% Example: Adding Data Labels
data = [3, 5, 7];
b = bar(data);
for i = 1:length(b.YData)
text(b.XData(i), b.YData(i), num2str(b.YData(i)), 'HorizontalAlignment', 'center', 'VerticalAlignment', 'bottom');
end
This example places numeric values above each bar, ensuring that specific data can be interpreted instantly alongside the visual representation.
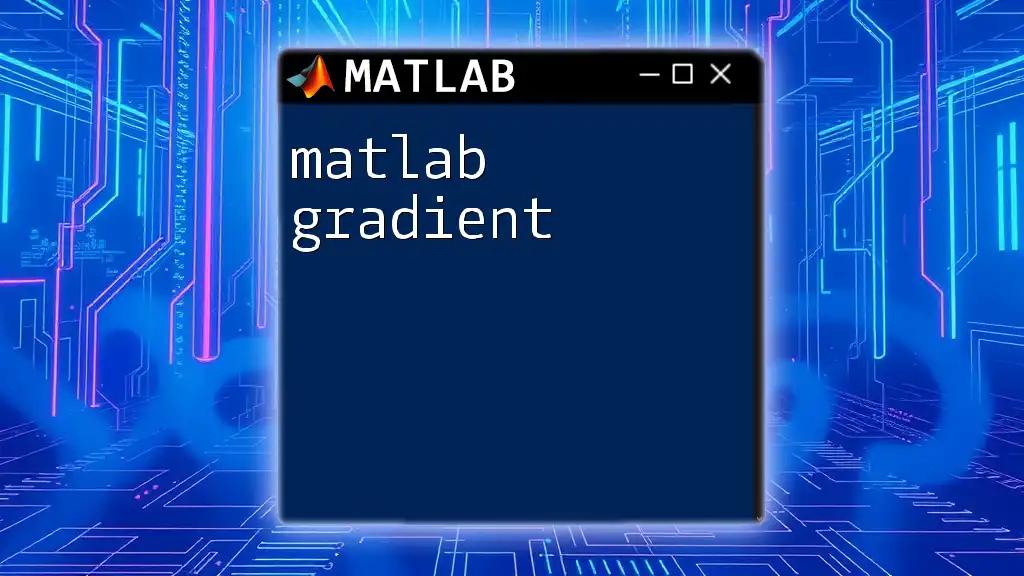
Exporting and Saving Bar Graphs
Saving as Image Files
After creating an informative bar graph, you may want to share or include it in a report. MATLAB provides multiple formats for exporting figures efficiently:
% Example: Saving a Graph
saveas(gcf, 'my_bar_graph.png');
This code saves the current figure in PNG format, ideal for online and print use.
Exporting to MATLAB Figures
For sharing with colleagues who also use MATLAB, saving your figure as a .fig file is practical. This allows recipients to open and edit the figure in their MATLAB environment:
savefig('my_bar_graph.fig');
This maintains the editable properties of your graph, making collaborative work seamless.
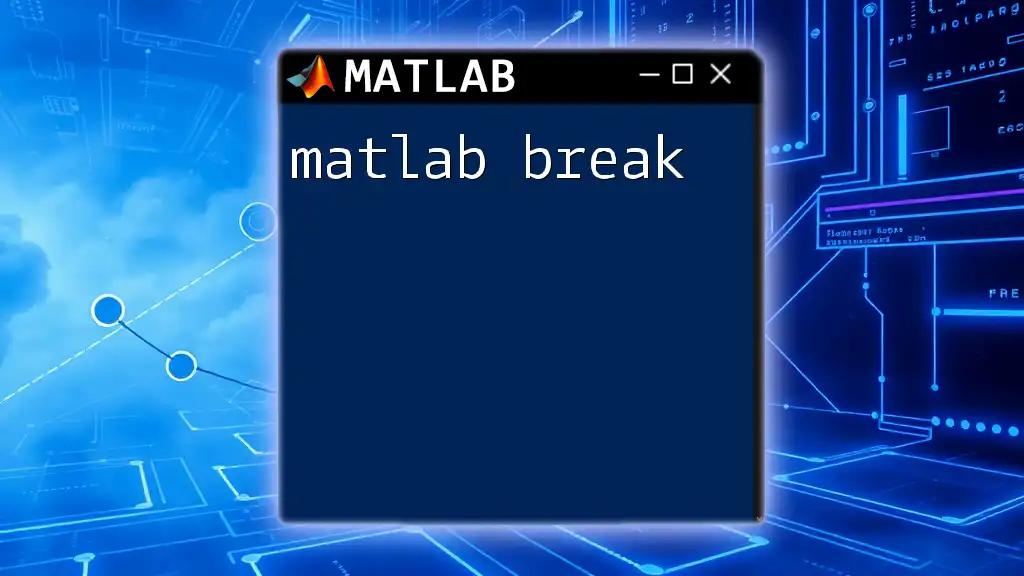
Conclusion
Recap of Key Points
In this guide, we explored the functionalities of creating and customizing MATLAB bar graphs. This included simple bar graphs, advanced grouped and stacked techniques, as well as best practices for labeling and exporting.
Additional Resources
For further learning, MATLAB’s official documentation is an excellent resource, providing in-depth insights and examples. Additionally, engaging with online forums and communities can enhance understanding and application.

Call to Action
Now that you have a comprehensive understanding of creating and customizing bar graphs in MATLAB, it's time to get hands-on. Challenge yourself by creating your own visualizations and exploring the various customization options available. For any questions or personalized guidance, feel free to reach out!