To compute the centroid of a set of points in MATLAB, you can use the mean function on the coordinates, while "fux" seems to be a typo; if you meant "flux," the context is unclear, so please clarify that term.
Here’s a simple code snippet to calculate the centroid:
% Example code to compute the centroid of a set of 2D points
points = [x1, y1; x2, y2; x3, y3]; % Replace with your points
centroid = mean(points);
Make sure to define `points` with your specific coordinates to get the centroid.
Understanding Centroids
What is a Centroid?
A centroid is a geometric center of a shape, often referred to as its center of mass or center of gravity. It represents a point where the shape could be perfectly balanced if it were made of a uniform material. The concept of a centroid is crucial in various fields, such as engineering, physics, and computer graphics, as it provides vital information regarding the spatial properties of an object.
Mathematically Computing the Centroid
The mathematical computation of a centroid is straightforward but varies depending on the number of dimensions:
-
1D centroid: For a set of points on a line, the centroid is the average of the x-coordinates.
\[ C_x = \frac{x_1 + x_2 + ... + x_n}{n} \]
-
2D centroid: For a set of points in a plane, the centroid is the average of the x-coordinates and the y-coordinates.
\[ C_x = \frac{x_1 + x_2 + ... + x_n}{n}, \quad C_y = \frac{y_1 + y_2 + ... + y_n}{n} \]
-
3D centroid: For a space-bound object, the centroid accounts for the z-coordinate as well.
\[ C_x = \frac{x_1 + x_2 + ... + x_n}{n}, \quad C_y = \frac{y_1 + y_2 + ... + y_n}{n}, \quad C_z = \frac{z_1 + z_2 + ... + z_n}{n} \]
Using MATLAB to Compute the Centroid
MATLAB Function for Centroid
To compute the centroid using MATLAB, you can define a function that takes in x and y coordinates and returns the centroid's coordinates. Here’s a basic implementation:
function c = computeCentroid(x, y)
c_x = sum(x) / length(x);
c_y = sum(y) / length(y);
c = [c_x, c_y];
end
Example of Centroid Calculation in MATLAB
Let’s see how to use this function in practice. Below is a simple example where we calculate the centroid of a 2D shape defined by sets of x and y coordinates:
x = [1, 2, 3, 4];
y = [5, 6, 7, 8];
c = computeCentroid(x, y);
disp(['Centroid: ', num2str(c)]);
When you run this code, it calculates and displays the centroid, emphasizing the importance of creating concise and reusable MATLAB functions to facilitate multiple computations efficiently.
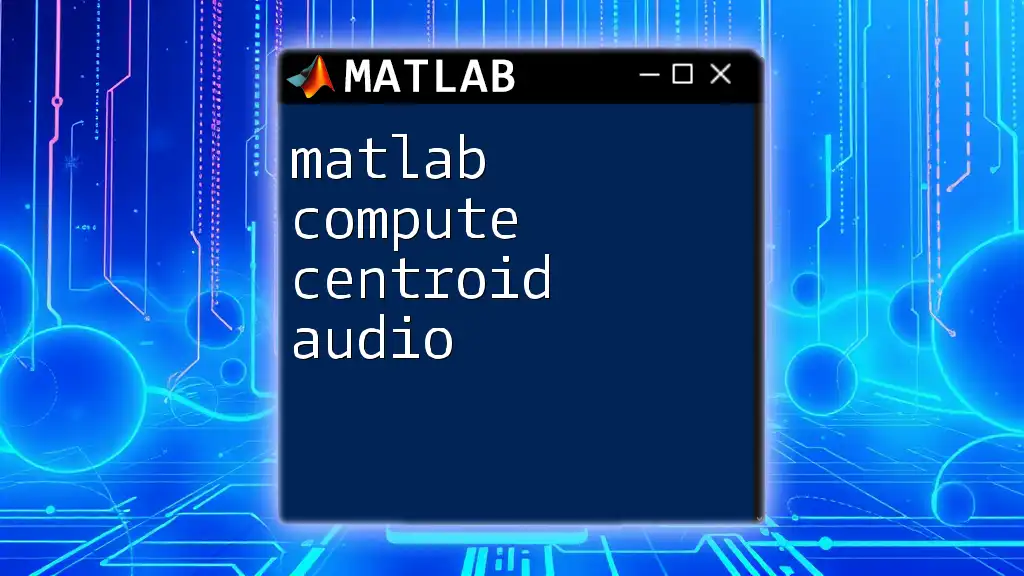
Understanding Flux
What is Flux?
Flux, in a broad context, refers to the flow quantity passing through a surface per unit time. This could be in the form of fluid flow, electricity, heat transfer, or any physical quantity. Understanding flux is essential for computations regarding energy flow, conservation laws, and maximizing efficiencies in engineering designs.
Mathematical Representation of Flux
Flux can often be represented mathematically depending on the scenario. For instance:
- Electric flux (`Φ_E`): Represents the total electric field passing through a given area, calculated as:
\[ Φ_E = \int \vec{E} \cdot d\vec{A} \]
where \(\vec{E}\) is the electric field vector and \(d\vec{A}\) is the differential area vector.
- Magnetic flux (`Φ_B`): Analogously relates to magnetic field lines passing through an area:
\[ Φ_B = \int \vec{B} \cdot d\vec{A} \]
Using MATLAB to Compute Flux
Implementing Flux Calculation in MATLAB
To compute flux using MATLAB, you can define a function like this:
function f = computeFlux(E, area)
f = sum(E .* area);
end
Example of Flux Calculation in MATLAB
Here's how to use this function in a realistic example:
E = [1, 2, 3]; % Electric field vector
area = [4, 5, 6]; % Area vector
f = computeFlux(E, area);
disp(['Flux: ', num2str(f)]);
In this example, we compute the product of the electric field and area vectors, showcasing how easy it is to handle vector mathematics in MATLAB.
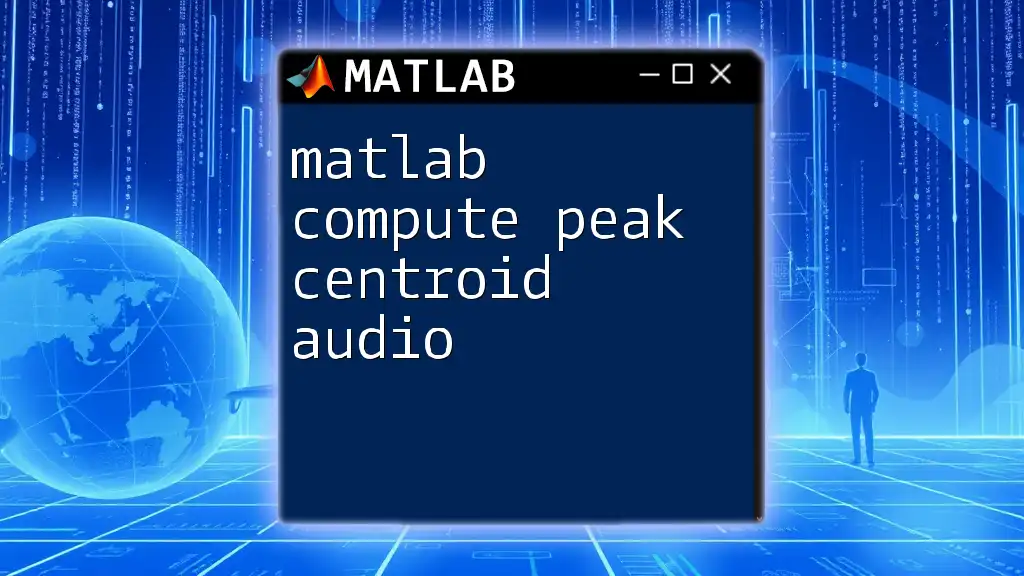
Combined Application: Centroid and Flux
Why Compute Both Centroid and Flux?
In many applications, such as mechanical systems or fluid flow problems, it may be necessary to compute both the centroid and the flux. The centroid provides the location for analysis, while the flux informs how energy or mass flows through that point.
Example Scenario: Center of Mass and Energy Flow Calculation
Imagine a situation where you have a uniform beam subjected to a force distributed across it. By calculating the centroid, you can determine the location of equilibrium, and by evaluating the flux, you can measure the energy or force rates acting at that position.
As an illustrative example, consider a beam with several forces acting on it. First, compute the centroid to find where the combined force can be considered to act. Then, assess the flux to determine energy flow, using the earlier defined functions in conjunction to deliver results.
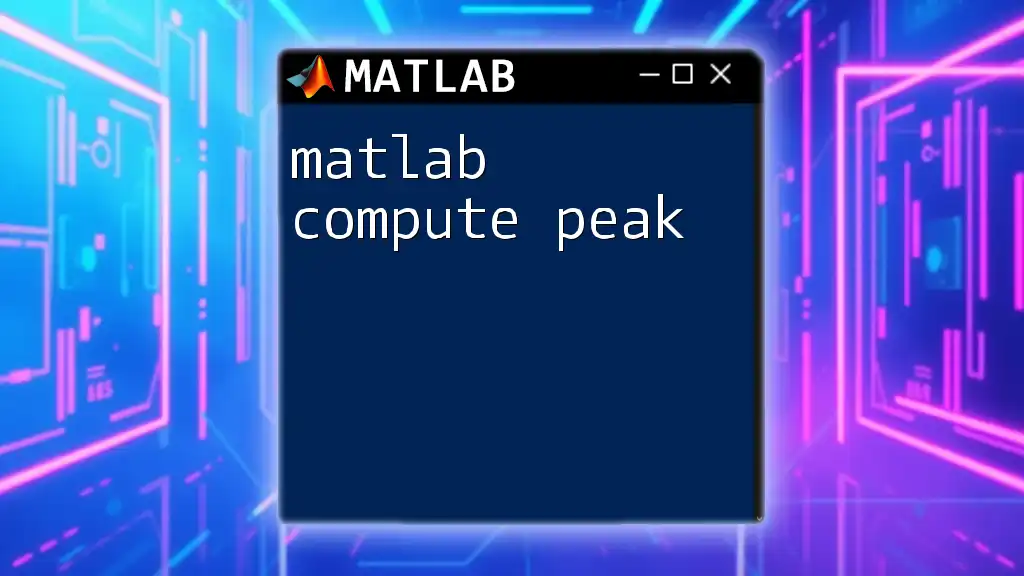
Visualization Techniques in MATLAB
Plotting the Centroid
Visualization is a critical part of interpreting data in MATLAB. You can plot shapes and highlight their centroids using built-in functions:
x = [1, 2, 3, 4];
y = [5, 6, 7, 8];
c = computeCentroid(x, y);
plot(x, y, 'b-o', 'MarkerFaceColor', 'r');
hold on;
plot(c(1), c(2), 'k*', 'MarkerSize', 10); % Plotting the centroid
hold off;
This script visualizes the shape and marks the centroid with a distinct star symbol.
Visualizing Flux through Surfaces
Visual representation of flux can enhance understanding. While complex, here's a simplified example using surfaces:
% Simplified surface plot example
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = exp(-0.1*(X.^2 + Y.^2));
surf(X, Y, Z);
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('Flux Visualization');
In this example, a three-dimensional surface is plotted, providing a visual context to the concept of flux across a surface.
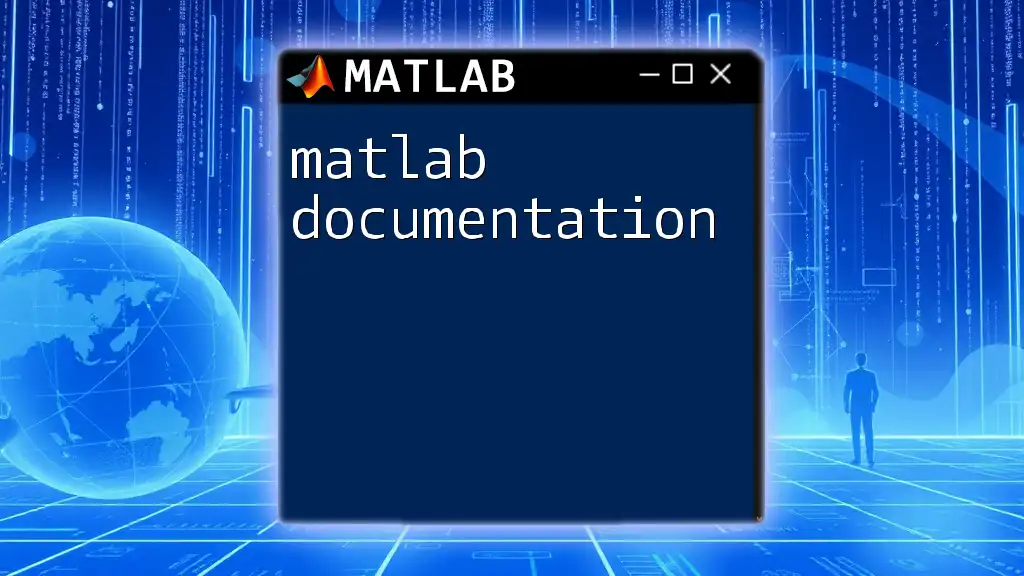
Troubleshooting Common Issues
Common Errors in Centroid Calculation
When working with centroid computations, common errors might arise from incorrect data points or dimensional mismatches. Always ensure that your x and y vectors are of equal length. Miscalculations can often be avoided by restructuring the function to include validations.
Flux Calculation Pitfalls
Errors in flux calculations typically involve misunderstanding vector components or using wrong area representations. To troubleshoot, verify the vectors are correctly defined and follow the vector multiplication rules inherent to MATLAB.
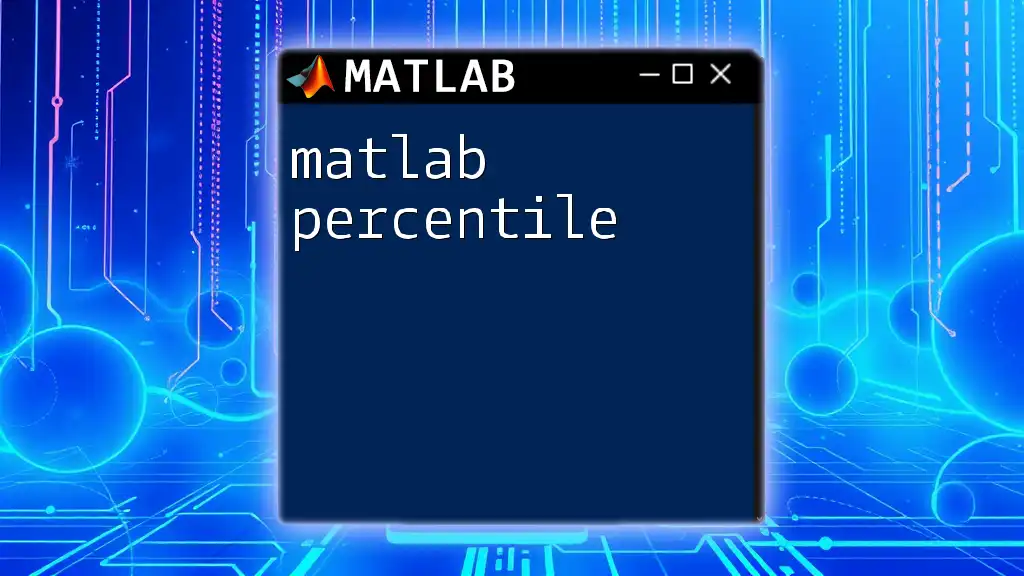
Conclusion
In summary, understanding how to matlab compute centroid and flux is essential for various applications across disciplines. By leveraging the concise functions created, you can streamline your computations and analysis effectively. Practicing these techniques through MATLAB will enable deeper insights into spatial and flow phenomena. Explore more tutorials, engage with resources, and expand your MATLAB skills further!
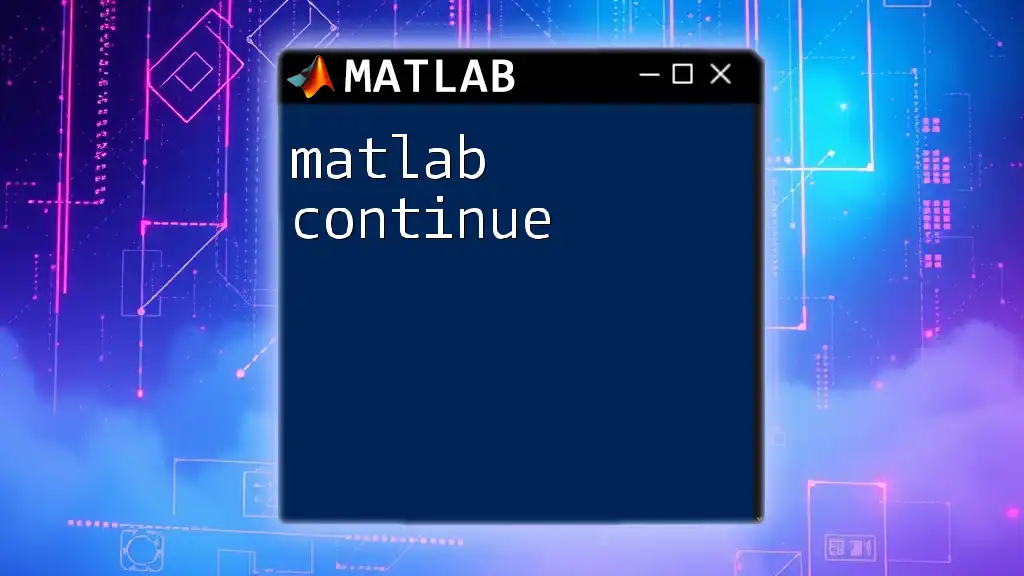
Additional Resources
Recommended MATLAB Documentation
To develop your understanding, refer to MATLAB's [official documentation](https://www.mathworks.com/help/matlab/) on the topics discussed.
Suggested Reading Material
Check out articles, textbooks, and online courses for a deeper exploration of centroids and flux in MATLAB beyond this basic introduction.