In MATLAB, you can compute the peak centroid of an audio signal by identifying the position of the peak amplitude and calculating its weighted average based on the audio sample values.
Here's a code snippet to illustrate this:
% Load audio file
[x, fs] = audioread('audiofile.wav');
% Find the index of the peak
[~, peak_index] = max(x);
% Compute the centroid around the peak
centroid = sum((1:length(x)) .* x) / sum(x);
% Display the results
fprintf('Peak Index: %d\nCentroid: %.2f\n', peak_index, centroid);
Understanding Peak Centroid in Audio Processing
What is Peak Centroid?
Peak centroid refers to a measure in audio processing that identifies the "center of mass" of the peak amplitudes within a given audio signal. In simpler terms, it provides a way to quantify the distribution of loud parts of an audio signal. The concept is crucial for various analyses, such as determining the loudness or clarity of a sound.
The peak centroid is particularly significant in scenarios where the perception of audio is essential, such as in music production, speech processing, and audio engineering. By understanding where the bulk of sound energy lies within the signal, audio engineers can make informed decisions about mixing, mastering, and enhancing sound quality.
Applications of Peak Centroid
The applications of peak centroid in audio processing are vast. Understanding how it functions can aid professionals in several fields:
- Audio Quality Analysis: Peak centroid can help in evaluating the overall quality of recordings by indicating which frequencies dominate at any given moment.
- Music Informatics: In the field of music analysis, peak centroid aids in the classification of musical pieces based on their auditory characteristics.
- Speech Processing: In analyzing speech signals, identifying the peak centroid can improve recognition algorithms, helping voice assistants and transcription services understand speech patterns better.
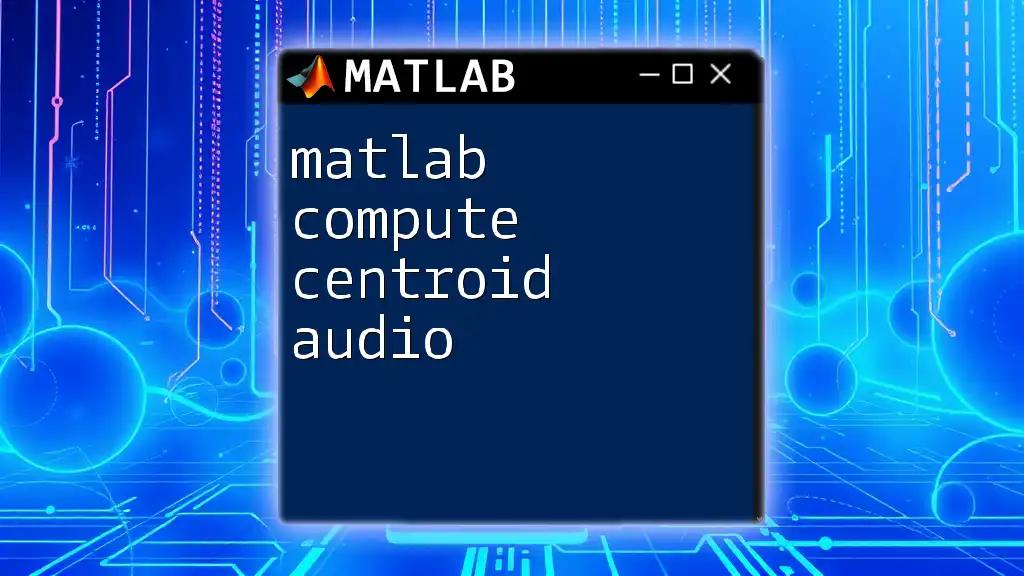
Introduction to MATLAB for Audio Signal Processing
Overview of MATLAB as a Tool for Audio Analysis
MATLAB is a powerful computational tool that is popular among engineers and researchers for audio analysis. With its robust built-in functions and user-friendly syntax, users can effectively manipulate and analyze audio signals. Key functions related to audio processing include `audioread()`, `findpeaks()`, and various mathematical operations to manipulate arrays.
Using MATLAB for audio analysis not only streamlines the process but also allows for sophisticated modeling and simulation, making it an indispensable tool for professionals working with sound.
Setting Up Your MATLAB Environment
Before diving into audio analysis, ensure you have the right setup:
- Required Toolboxes: The Signal Processing Toolbox is essential; it provides many functions that facilitate the handling and processing of audio data.
- Installing Additional Packages: If additional functionalities are needed, such as machine learning support for audio analysis, consider installing relevant add-ons from the MATLAB environment.
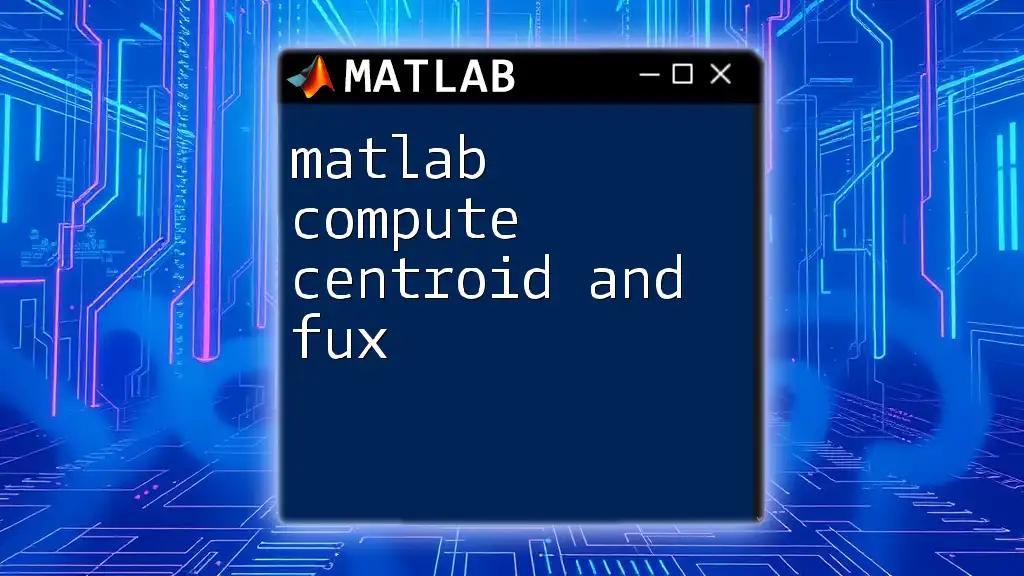
Loading and Visualizing Audio Data in MATLAB
Loading Audio Files
The first step in audio signal analysis is to load your audio file into MATLAB. You can accomplish this using the `audioread()` function, which efficiently imports various audio file formats.
To load an audio file, you would typically use the following command:
[audioData, fs] = audioread('audiofile.wav');
In this snippet:
- `audioData` contains the waveform of the audio signal.
- `fs` represents the sampling frequency in hertz (Hz) of the audio signal, crucial for any time-based analysis.
Visualizing Audio Signals
Once the audio data is loaded, visual representation can provide significant insights. Using the `plot()` function, you can create a time-domain visualization of the audio waveform.
Here’s an example snippet that plots the audio signal:
t = (0:length(audioData)-1)/fs;
plot(t, audioData);
title('Audio Waveform');
xlabel('Time (s)');
ylabel('Amplitude');
This code snippet computes the time vector and generates a plot. By examining the resulting graph, you can get insight into the signal's amplitude variations over time, allowing you to identify peaks, silence, and overall loudness.
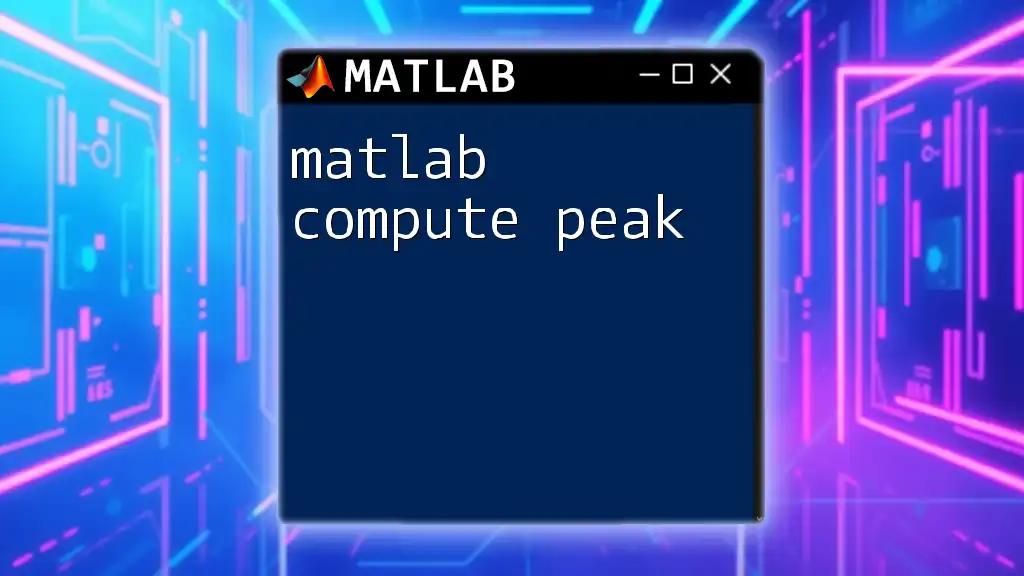
Computing the Peak Centroid
What is the Peak Centroid Calculation?
The peak centroid calculation is essential for understanding the distribution of intensity in an audio signal. The mathematical definition of the peak centroid is expressed as a ratio comparing the weighted sum of peak positions to their respective amplitudes.
By analyzing the peak centroid, one can gain insights into how audio content can be perceived by listeners, especially when they are trying to understand which parts of the audio are more prominent.
Steps to Compute Peak Centroid in MATLAB
To compute the peak centroid in MATLAB, follow these steps:
- Extract Peak Values: Utilize the `findpeaks()` function to identify the peaks and their locations within the audio signal.
Here is an illustrative example:
[pks, locs] = findpeaks(audioData);
In this example, `pks` will contain the amplitude values of the detected peaks, while `locs` indicates their positions in the audio signal.
- Analyzing the Peak Locations: After extracting the peak values, you can compute the centroid using a simple formula that incorporates these peaks:
centroid = sum(locs .* pks) / sum(pks);
The `centroid` variable will now hold the calculated peak centroid position in samples, which can give a sense of where the loudest sections of an audio recording are located over its duration.
Full Example Implementation
For clarity, here’s the complete process encapsulated in a single MATLAB script:
% Load the audio file
[audioData, fs] = audioread('audiofile.wav');
% Find peaks
[pks, locs] = findpeaks(audioData);
% Calculate centroid
centroid = sum(locs .* pks) / sum(pks);
% Display the result
fprintf('Peak Centroid: %f samples\n', centroid);
Running this script will load your specified audio file, identify peak values and locations, calculate the peak centroid, and finally print the result to the command window for review.
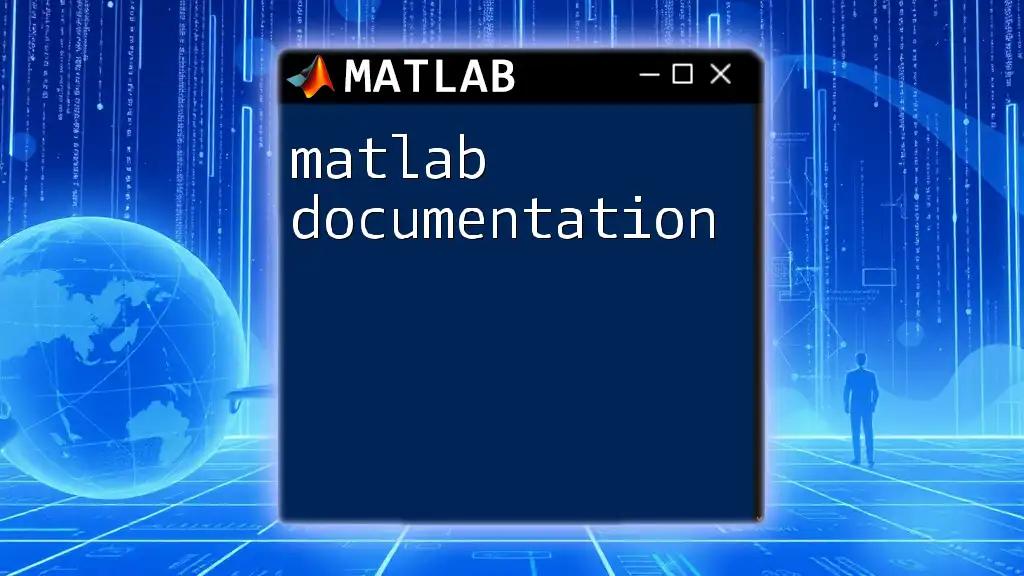
Visualizing the Peak Centroid
Creating Visuals
An effective way to confirm and visualize the peak centroid against the audio waveform involves plotting the peaks and the centroid on the corresponding graph. Below is an example of how to augment your previous waveform plot with peak and centroid markers:
figure;
hold on;
plot(t, audioData);
plot(locs/fs, pks, 'ro'); % Marking peaks
plot(centroid/fs, audioData(centroid), 'g*', 'MarkerSize', 10); % Centroid
hold off;
title('Peak Centroid Visualization');
xlabel('Time (s)');
ylabel('Amplitude');
legend('Audio Signal', 'Peaks', 'Centroid');
In this visualization:
- Red circles indicate the prominent peaks in the audio signal.
- Green star denotes the calculated centroid position, allowing for a clear interpretation of how the peak distribution centers around the overall sound.
Interpreting the Visualization
By analyzing the graph, one can easily observe the relationship between peak locations and the centroid. If the centroid lies closer to high-amplitude peaks, it suggests that these areas significantly contribute to the overall loudness of the audio.
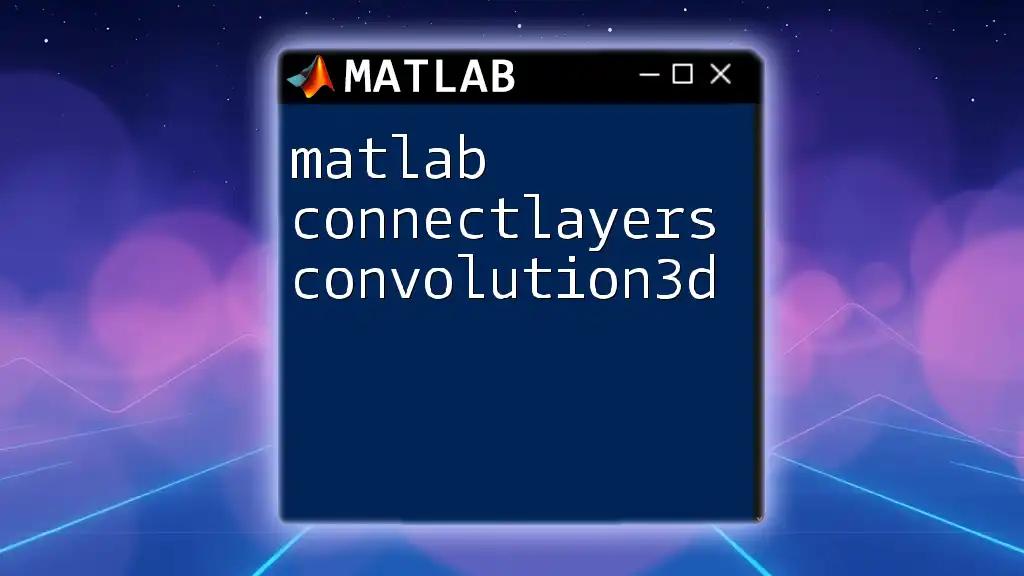
Conclusion
Recap of Key Concepts
In this guide, we delved into the fundamental ideas surrounding MATLAB compute peak centroid audio, explored its significance in audio signal processing, and demonstrated how to effectively load, analyze, and visualize audio data in MATLAB.
Encouragement for Further Exploration
With peak centroid analysis, audio professionals can gain invaluable insights into their recordings, but this is just the beginning. From here, users are encouraged to explore advanced techniques and algorithms that enhance audio quality further, aiding in processes such as noise reduction, frequency analysis, and pitch detection.
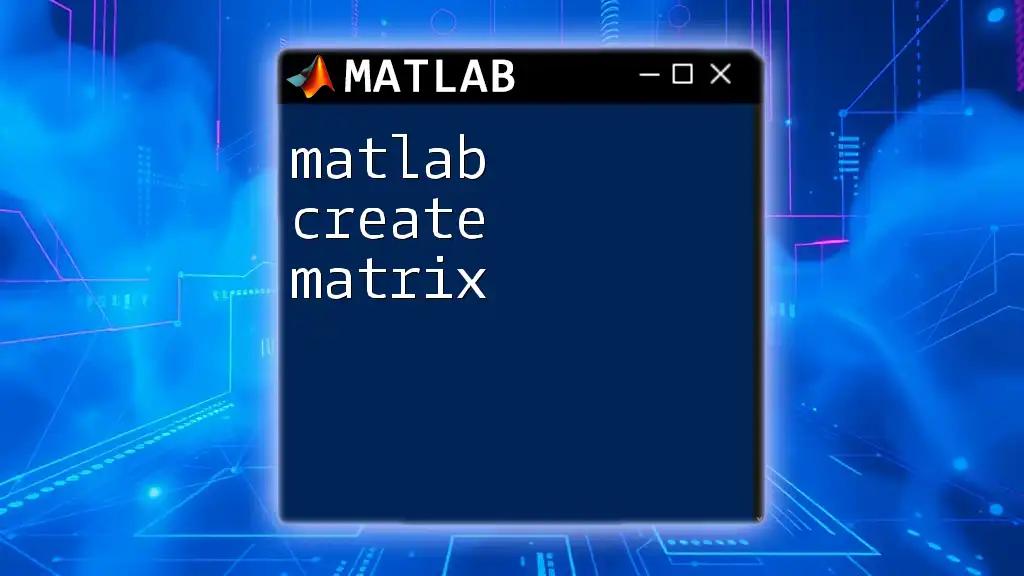
Additional Resources
Recommended MATLAB Documentation
Explore the MATLAB documentation for more in-depth information on functions like `audioread()` and `findpeaks()`, ensuring you maximize MATLAB's full potential in your audio analysis journey.
Online Tutorials and Courses
Consider engaging in online courses or tutorials that focus on audio processing using MATLAB, which can help deepen your understanding and skillset in this domain.