In MATLAB, the `corr` function computes the Pearson correlation coefficient between two sets of data, allowing users to assess the degree of linear relationship between variables.
% Example: Calculate the correlation between two vectors, x and y
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 5, 6];
correlation_coefficient = corr(x', y');
Understanding the Basics of Correlation in MATLAB
Correlation is a statistical measure that describes the extent to which two variables change together. In MATLAB, understanding correlation is essential for analyzing data patterns and relationships. It is particularly valuable in fields like data science, finance, and engineering.
The MATLAB function `corr` is primarily used to compute the correlation coefficients between two data sets or matrices. This function is highly versatile, enabling users to examine linear and non-linear relationships, as well as dealing with various data structures.
Types of Correlation
There are several types of correlation that users should be aware of when working with the `corr` function:
- Positive Correlation: When one variable increases, the other also tends to increase. For example, height and weight often show a positive correlation.
- Negative Correlation: When one variable increases, the other tends to decrease. An example could be the number of hours studied and the number of errors on a test.
MATLAB offers three popular methods for evaluating correlation:
- Pearson Correlation: A measure of linear correlation between two sets of data. It assumes that both datasets are normally distributed.
- Spearman Correlation: A non-parametric measure based on rank values. It evaluates how well the relationship between two variables can be described using a monotonic function.
- Kendall Correlation: Another non-parametric method that measures ordinal association between two variables.
Knowing which type of correlation to use is crucial and depends on the nature of the data you are analyzing. Typically, Pearson is used for continuous data, while Spearman and Kendall are suitable for ordinal or non-linear data.

Syntax and Parameters of `corr`
The basic syntax for using `corr` in MATLAB is straightforward:
R = corr(X, Y);
Where `X` and `Y` are your data inputs, and `R` is the resultant correlation coefficient.
Parameters
- 'Rows' Parameter:
- This parameter allows you to specify how MATLAB should handle rows containing missing values.
- Options include:
- `'pairwise'`: Computes the correlation using all pairs of data available, ignoring NaNs in either variable.
- `'complete'`: Uses only rows with non-missing values across both variables.
- Method Parameter:
- You can specify the type of correlation to be used:
- 'Pearson'
- 'Spearman'
- 'Kendall'
- You can specify the type of correlation to be used:
Here's an example incorporating these options:
% Using Pearson correlation with complete rows
R = corr(X, Y, 'Rows', 'complete', 'Type', 'Pearson');
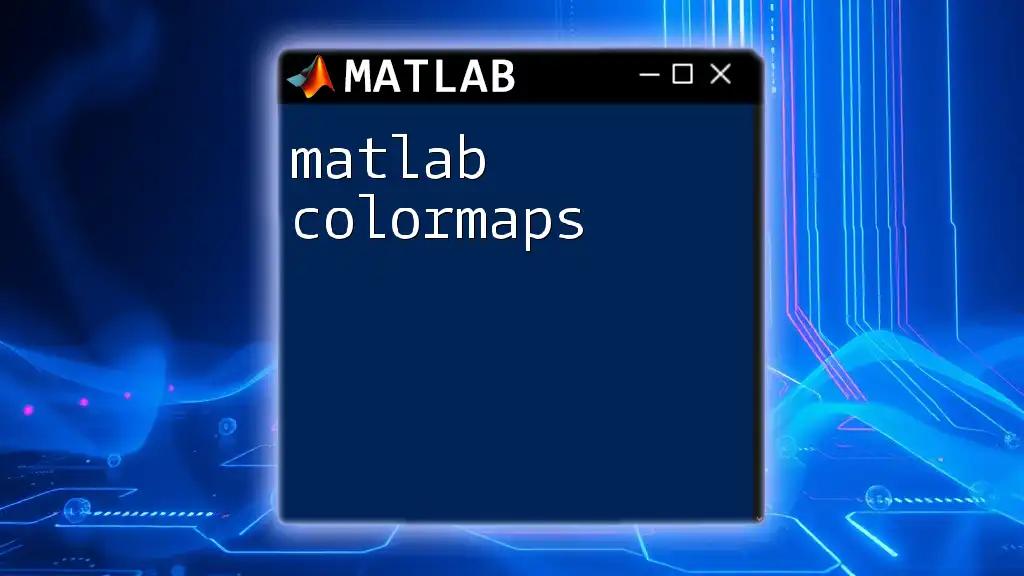
Working with `corr` in MATLAB
Generating Sample Data
To effectively use the `corr` function, it's essential to work with some sample data. You can create random datasets to demonstrate correlation:
X = rand(100, 1); % 100 random numbers between 0 and 1
Y = 2 * X + randn(100, 1) * 0.1; % Y is correlated with X (with some noise)
Calculating Correlation Coefficients
Once you have your data, calculating the correlation coefficients is simple:
R = corr(X, Y); % Computes the Pearson correlation coefficient
fprintf('The correlation coefficient is: %.2f\n', R);
The output value of `R` will range between -1 and 1:
- 1 indicates a perfect positive correlation,
- 0 indicates no correlation,
- -1 indicates a perfect negative correlation.
Visualizing Correlation
A great way to understand the relationship between your variables is by using scatter plots:
scatter(X, Y);
title('Scatter plot of X and Y');
xlabel('X values');
ylabel('Y values');
This visualization will provide insights into whether `X` and `Y` are indeed correlated and how strong that correlation is.
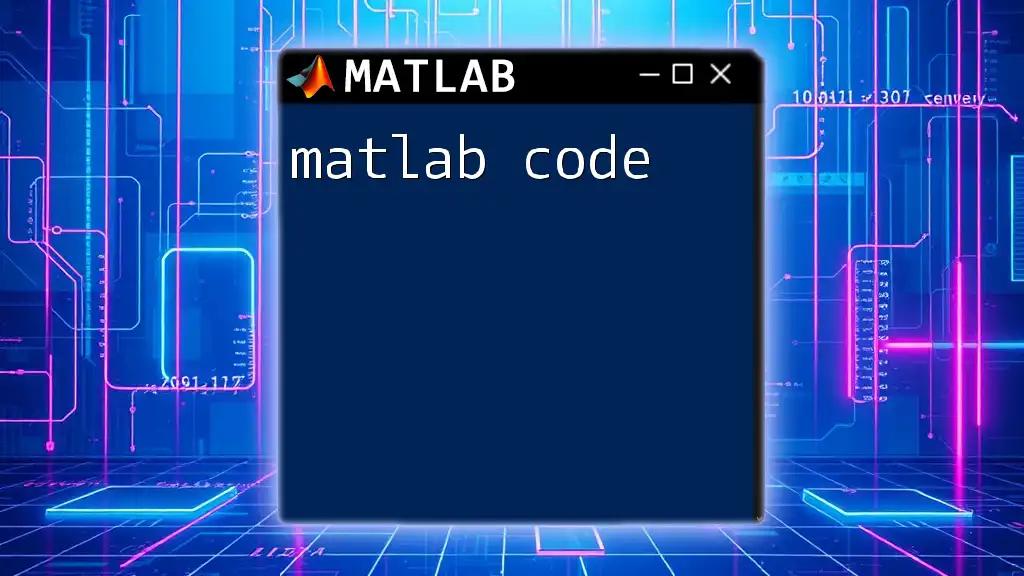
Advanced Usage of `corr`
Handling Multi-dimensional Arrays
The `corr` function isn't limited to just two variables; it can also compute correlation across matrices:
Z = rand(10, 3); % Creates a dataset with 10 observations of 3 variables
R_matrix = corr(Z); % Computes correlation matrix
The result, `R_matrix`, is a square matrix showing the correlation coefficients between each pair of variables.
Using Tables and Timetable Data Types
MATLAB allows users to handle complex data structures. You can apply the `corr` function to tables seamlessly:
T = table(X, Y, 'VariableNames', {'Var1', 'Var2'});
R_table = corr(T.Var1, T.Var2);
This method maintains clarity and organization, especially when working with large datasets.
Correlation Between More than Two Variables
To analyze multiple variables simultaneously, you can create a correlation matrix:
R_matrix = corr(Z); % Correlation matrix for multiple variables
This matrix provides a comprehensive view of the relationships among all variables in your dataset.
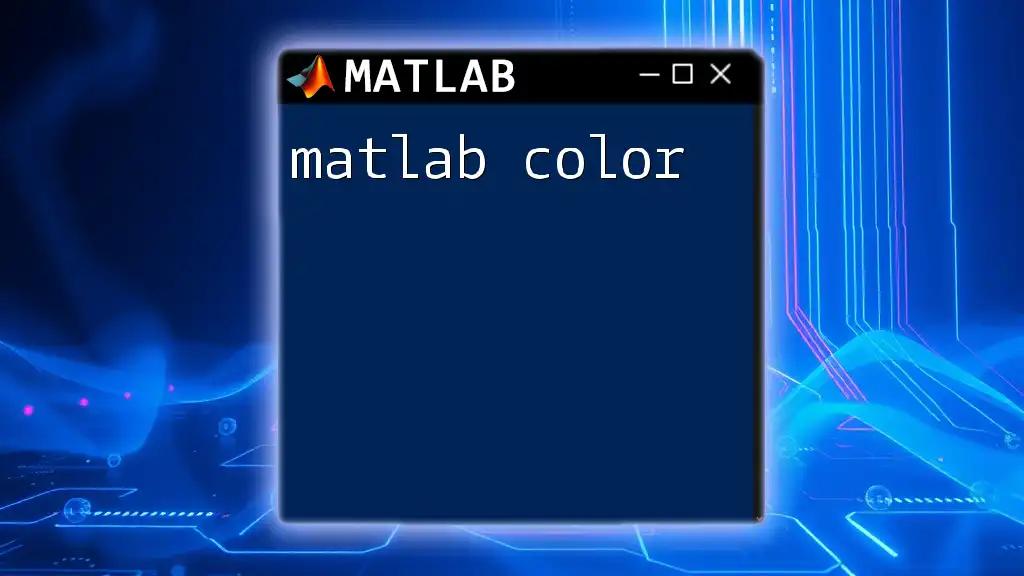
Common Errors and Troubleshooting
Understanding Errors in Applying `corr`
Beginners may encounter common pitfalls while using the `corr` function, such as mismatches in sizes of input vectors. Ensure that both `X` and `Y` possess the same number of observations.
Dealing with Missing Data
Handling missing values is paramount in correlation analysis. Depending on your choice of the `Rows` parameter, you can either ignore NaNs within pairs or use only complete cases. Understanding how these options affect your results is crucial for accurate interpretation.
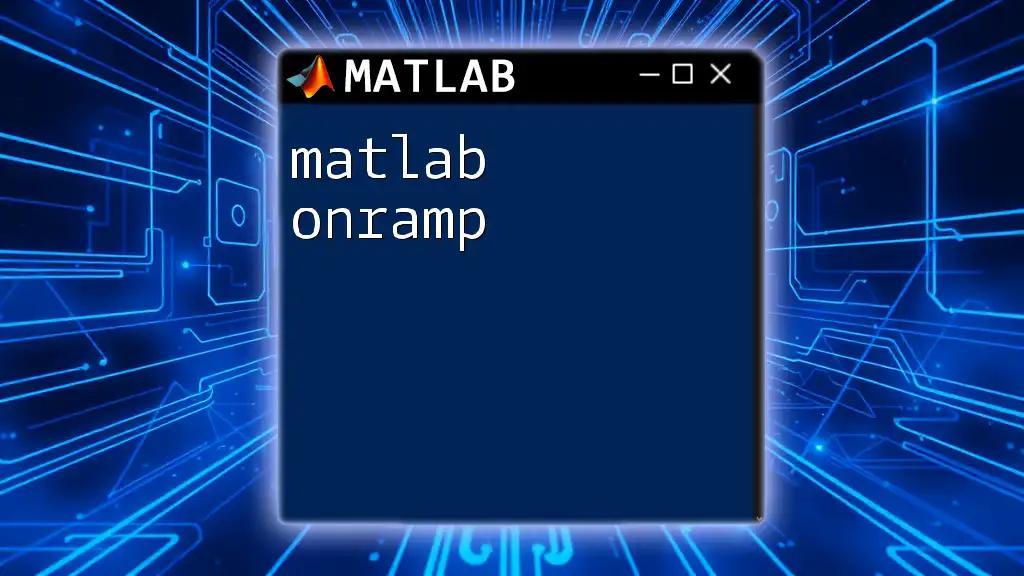
Real-world Applications of `corr`
Case Studies
Correlation analysis is widely used across many domains. For example:
- Finance: Investors often analyze the correlation between the returns of different stocks. Identifying which stocks move together can assist in diversifying a portfolio.
- Healthcare: In clinical studies, researchers might look at correlations between patient metrics, such as blood pressure and cholesterol levels, to identify potential risk factors for diseases.
Significance Testing
When interpreting correlation results, it is also essential to assess the significance of your findings. You may need to conduct hypothesis testing to determine if the observed correlation is statistically significant, which further informs your conclusions.
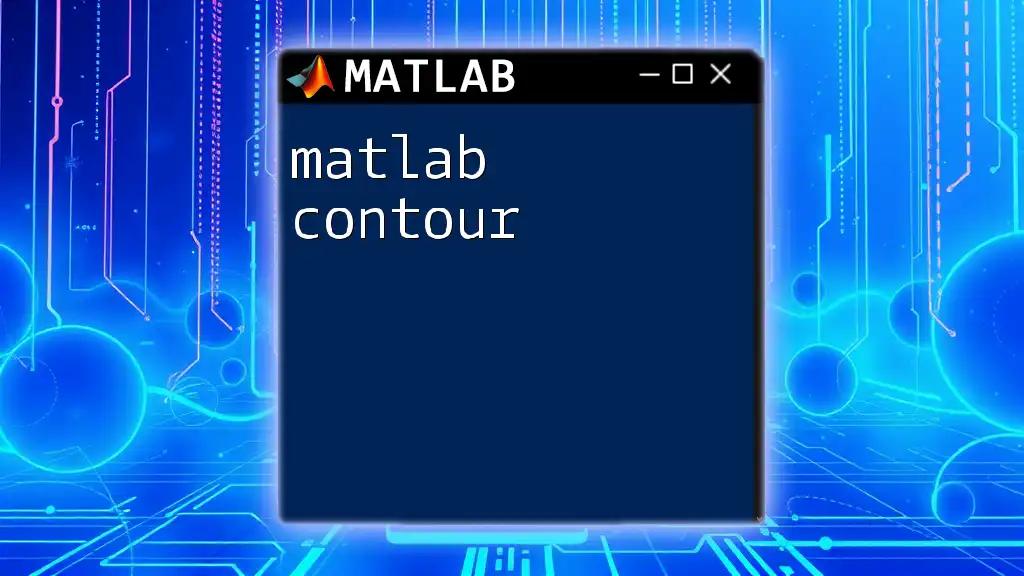
Conclusion
The `corr` function in MATLAB serves as a powerful tool for understanding relationships between variables, allowing researchers and analysts to draw meaningful insights from their data. By utilizing different correlation methods and effectively handling data structures, you can robustly analyze trends and dependencies across a wide array of disciplines.

FAQs about `corr`
-
What is a good correlation coefficient? A good correlation coefficient often depends on your specific field and the context of your analysis. Generally, values closer to 1 or -1 signify a strong correlation.
-
How to interpret a correlation coefficient of 0? A correlation coefficient of 0 implies no linear relationship between the two variables being analyzed. However, the relationship could still be non-linear.
-
Can `corr` handle categorical variables? The `corr` function is not designed for categorical variables directly; however, you can encode categorical data into numerical values before performing correlation analysis.
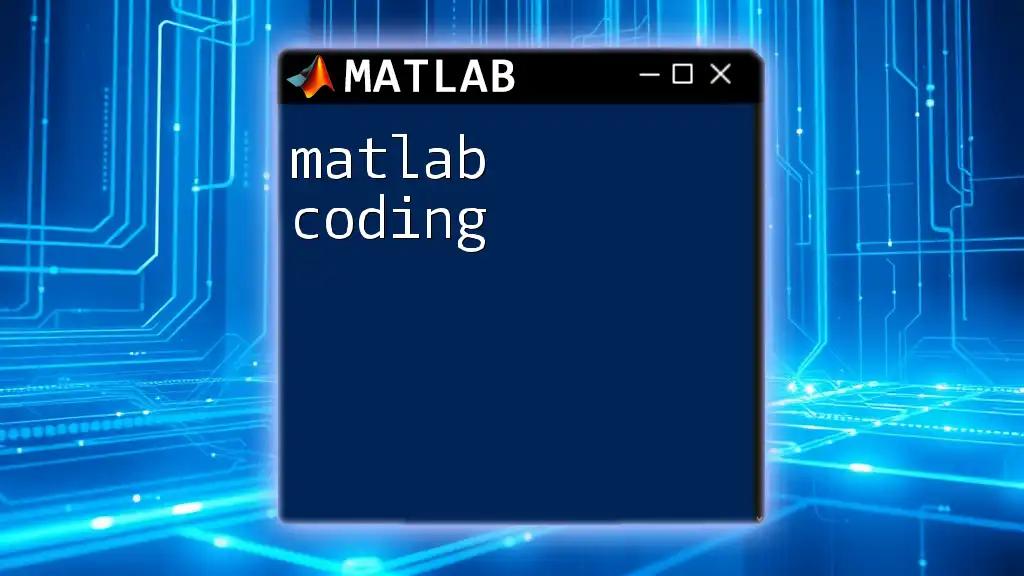
References
For deeper insights, refer back to the MATLAB official documentation and explore additional resources on correlation and statistical methods. These will further enhance your understanding of data correlations and their applications in real-world scenarios.