The `xcorr` function in MATLAB computes the cross-correlation of two signals, helping to identify the similarity between them over varying time lags.
% Example: Calculate cross-correlation of two signals
signal1 = [1, 2, 3, 4, 5];
signal2 = [5, 4, 3, 2, 1];
[crossCorr, lags] = xcorr(signal1, signal2);
What is Cross-Correlation?
Definition and Concept
Cross-correlation is a statistical measure used to evaluate the similarity between two signals as a function of the time-lag applied to one of them. Mathematically, it is defined as the integral of the product of two signals after one is shifted by some amount. In simple terms, cross-correlation helps to identify the degree to which one signal correlates with another signal, as it shifts in time.
Applications of Cross-Correlation
Cross-correlation has a wide range of applications across various fields:
- Signal Processing: It is commonly used to determine the time delay between signals, which is crucial in various applications, such as audio processing and seismic analysis.
- Communications: In telecommunications, cross-correlation helps achieve synchronization of signals, ensuring that data is accurately received and interpreted.
- Statistics: In statistical analysis, it can be used for feature detection, helping to uncover relationships between different datasets.
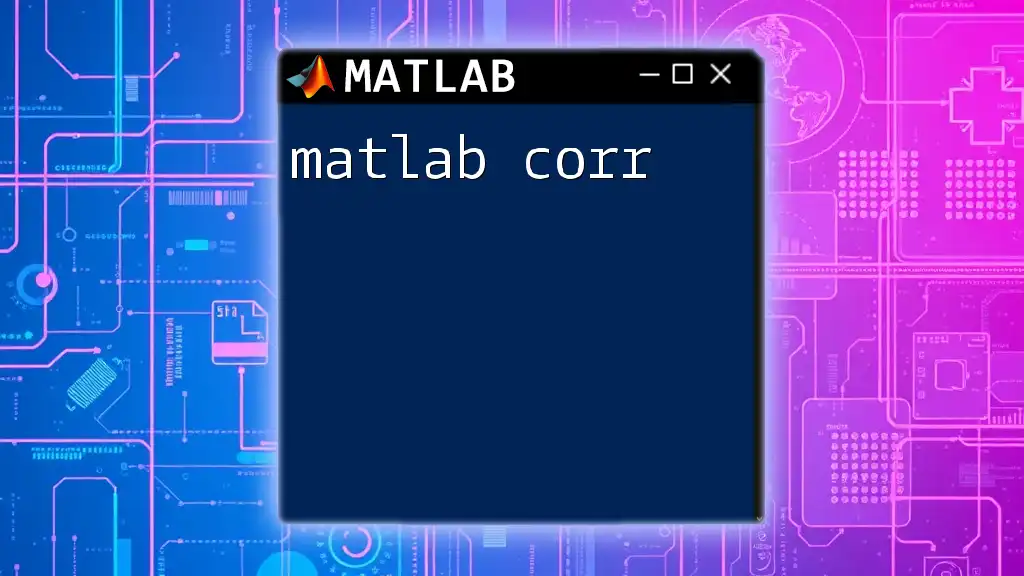
Understanding MATLAB's xcorr Command
Syntax of xcorr
In MATLAB, the `xcorr` function serves as the primary tool for computing cross-correlation. Its general syntax is as follows:
[c, lags] = xcorr(x, y, maxLag, 'option')
Here, `x` and `y` are the input signals (vectors), `maxLag` is the maximum lag you want to consider, and `'option'` can be used for normalization (e.g., `'coeff'` for normalized cross-correlation).
Basic Usage
To grasp the functionality of `matlab xcorr`, let's consider a simple example:
% Simple example with two signals
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 5, 6];
[c, lags] = xcorr(x, y);
In this example, `c` will contain the cross-correlation values between the two signals, while `lags` indicates the corresponding time lags for these values. Analyzing the results will showcase how well the signals correlate with one another across the specified lags.
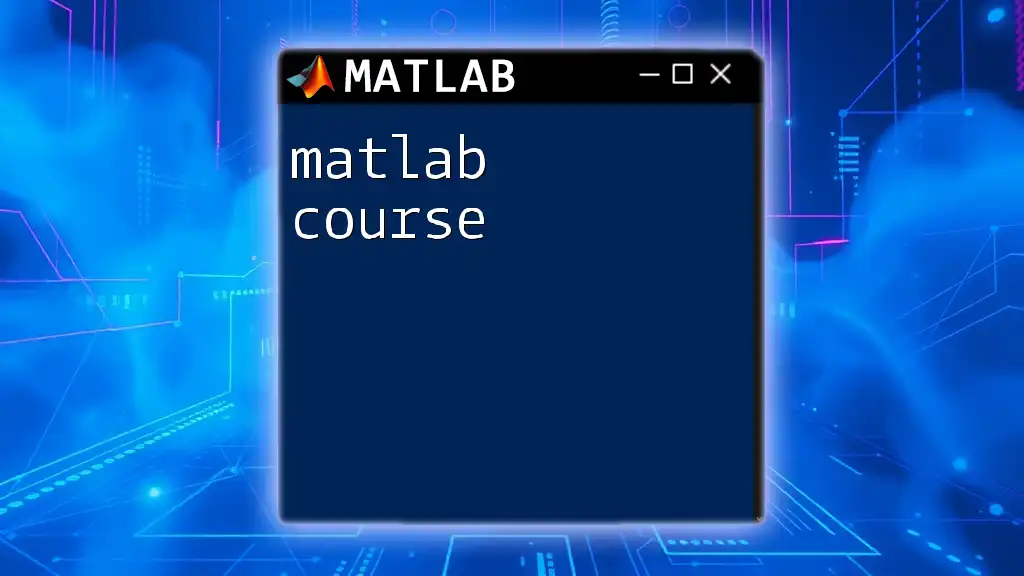
Advanced Usage of xcorr
Cross-Correlation with Noise
In real-world applications, signals are often influenced by noise. Let’s consider how we can analyze the effect of noise on cross-correlation:
% Example with noise
x_noise = x + randn(size(x)); % adding random noise
[c_noise, lags_noise] = xcorr(x_noise, y);
By adding random noise to our signal `x`, we can observe changes in the cross-correlation output. This scenario demonstrates the common challenges faced when dealing with real-world data, emphasizing the need for careful analysis and interpretation.
Zero-Padding and Its Importance
Zero-padding is a technique that helps to avoid issues with signal length and improve the resolution of the cross-correlation calculation. When using `xcorr`, signals are often padded with zeros to facilitate better analysis. This can be utilized as follows:
% Example with zero-padding
[c_zp, lags_zp] = xcorr(x, y, 'coeff'); % ensure zero-padding
Zero-padding can significantly affect the shape and interpretation of the resulting cross-correlation, especially for long signals. Understanding its use is crucial for effective data analysis.
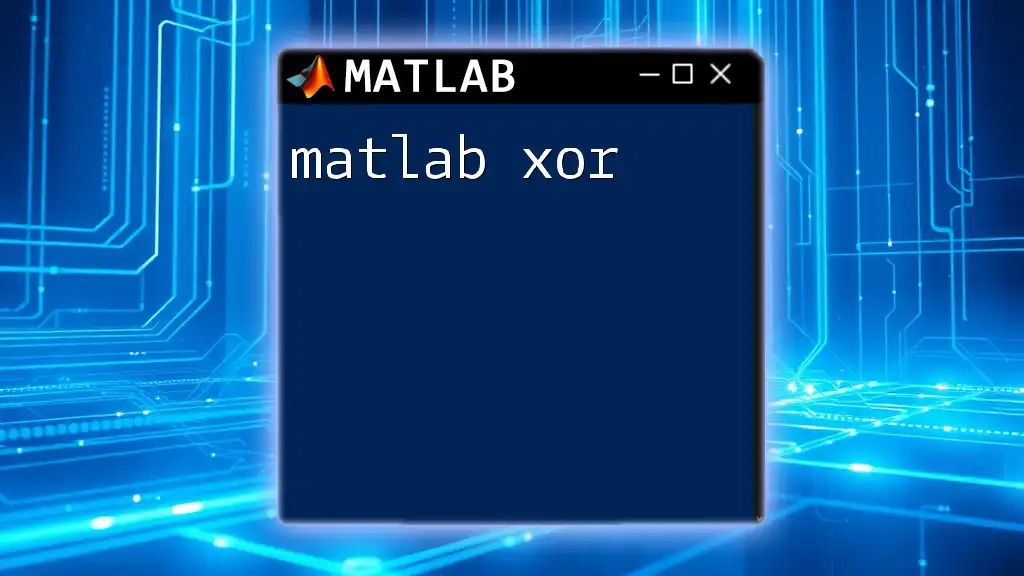
Normalizing Cross-Correlation
Importance of Normalization
Normalization is essential in cross-correlation analysis, as it ensures that the resulting values are on a comparable scale, thereby enhancing interpretability. This can be performed in `matlab xcorr` using different normalization options, including unnormalized and normalized correlations.
Normalization Examples
To compute a normalized cross-correlation, you can use the option `'coeff'`:
% Normalized cross-correlation
[c_norm, lags_norm] = xcorr(x, y, 'coeff');
The resulting values will be constrained between -1 and 1, providing a clearer insight into the relationship between the signals. Interpreting these normalized values can help establish stronger conclusions about the signal similarities.
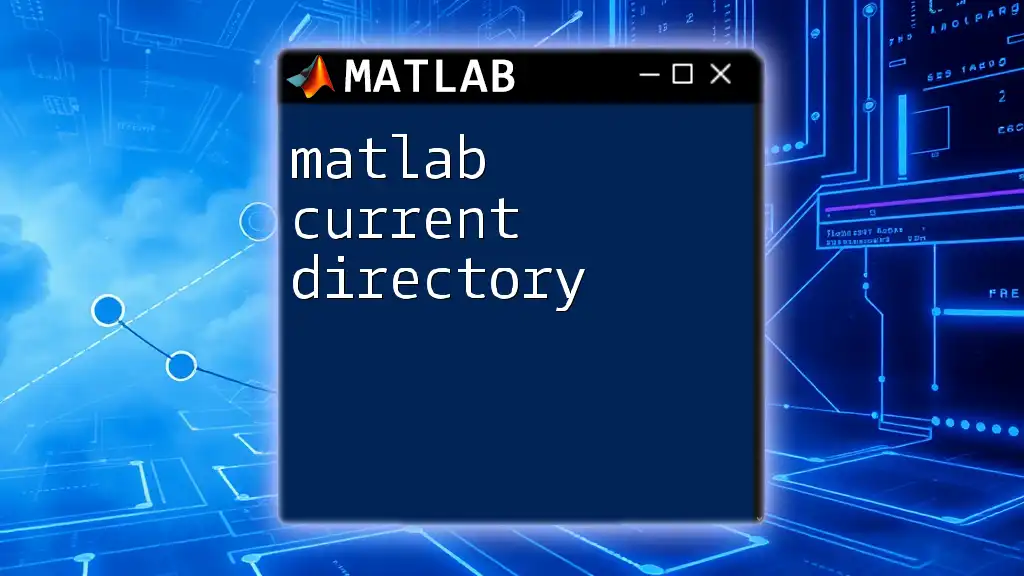
Visualizing Cross-Correlation Results
Plotting Cross-Correlation
Visualizing the results from `xcorr` is crucial for understanding and interpreting the data accurately. You can create a simple plot to illustrate the cross-correlation results:
% Plotting the results
figure;
stem(lags, c); % Using stem plot for discrete values
title('Cross-Correlation');
xlabel('Lags');
ylabel('Correlation Coefficient');
This visualization will help you identify peaks in the correlation, which indicate strong relationships between the signals at specific lags.
Using Spectrogram for Enhanced Analysis
A spectrogram offers a time-frequency representation of signals and can provide deeper insights when analyzing cross-correlation. By using MATLAB's `spectrogram` function, we can visualize signal characteristics over time:
% Spectrogram example
spectrogram(x, hamming(256), 128, 512, 'yaxis');
This additional analysis will contextualize your cross-correlation results by revealing how frequency components change over time.
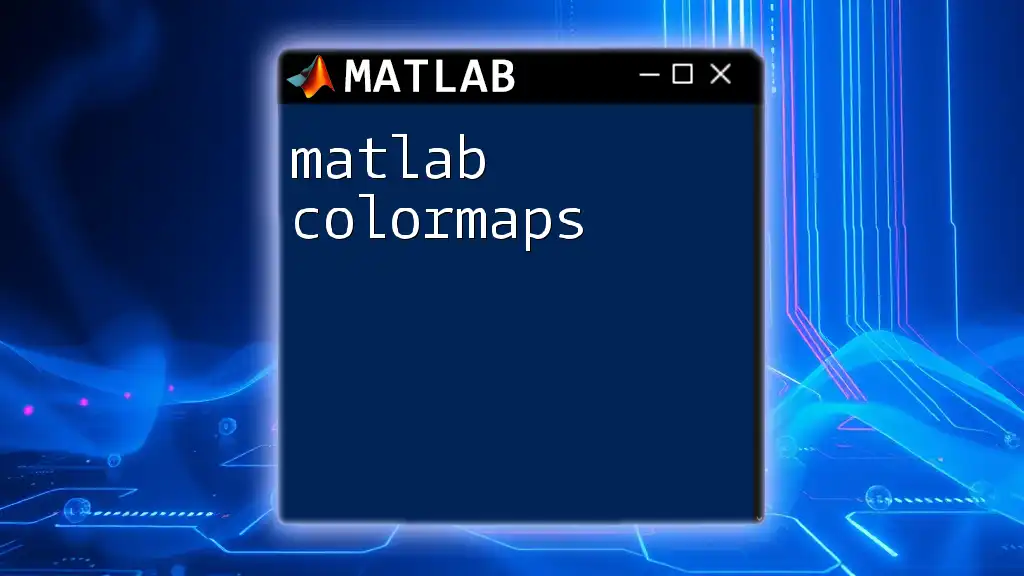
Common Errors and Troubleshooting
Common Issues with xcorr
When working with `matlab xcorr`, common pitfalls include:
- Mismatched vector lengths: Ensure that both input signals have the same number of elements when performing cross-correlation.
- Null results: Review your data and the specified parameters if results are unexpectedly low.
Debugging Tips
In any troubleshooting process, interpreting the results is essential. Check the dimensions of your input signals with the command `size(x)` and `size(y)` before performing cross-correlation. If you encounter warnings or errors, consider revisiting your input conditions and normalization options to diagnose the issues effectively.
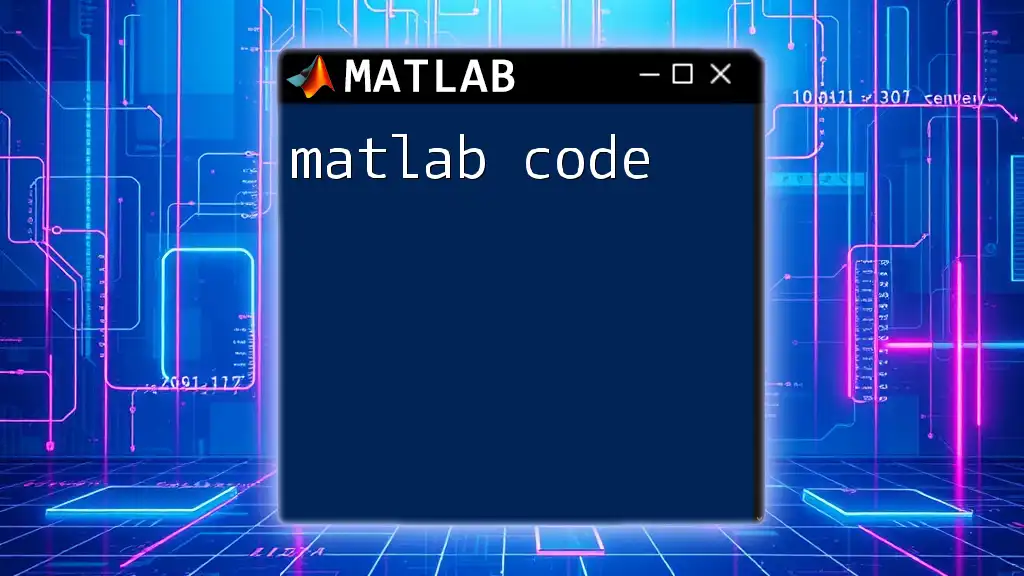
Conclusion
The matlab xcorr function is a powerful tool in signal analysis that provides extensive functionalities for exploring relationships between signals. By mastering this command and its options, you can uncover valuable insights from your data, leading to enhanced understanding and applications in fields ranging from engineering to data science. As you continue your journey with MATLAB, experimenting with different scenarios and setups will only enhance your skills further.
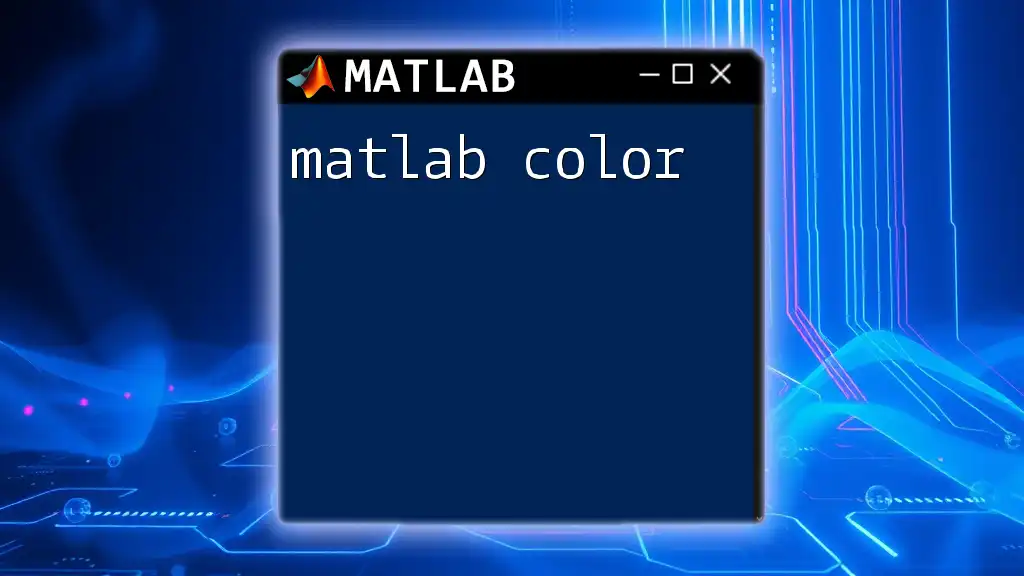
Additional Resources
Recommended Reading and References
To deepen your knowledge, consider exploring MATLAB's official documentation on the `xcorr` function and other relevant signal processing tools. Books and online tutorials can greatly aid in the journey of mastering MATLAB functionalities.
Community and Support
Engaging with the MATLAB community through forums and discussion groups can provide continued support, practical advice, and opportunities to learn from others who share similar interests.