In MATLAB, you can skip specific entries in the legend using the `legend` function by providing a set of labels while excluding certain data series.
Here’s a code snippet demonstrating how to skip specific legend entries:
x = 1:10;
y1 = rand(1, 10);
y2 = rand(1, 10);
y3 = rand(1, 10);
plot(x, y1, '-r', x, y2, '-g', x, y3, '-b');
legend('Series 1', 'Series 3'); % Skips 'Series 2'
Understanding MATLAB Legends
Legends serve as an essential component in data visualization, providing context and clarity to plots. They identify the different datasets represented in a graph, enabling viewers to understand which color or line corresponds to which data series. In MATLAB, legends not only enhance interpretability but also help convey complex information in an accessible manner.
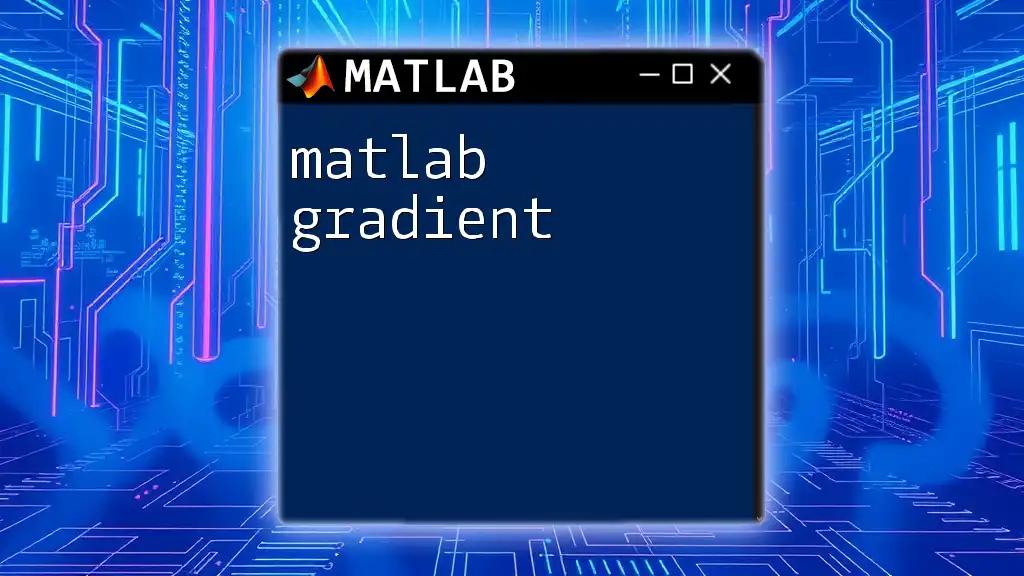
Purpose of Skipping Entries in Legends
Sometimes, you may find it necessary to skip certain entries from your legend. This could arise from a variety of situations, such as when the legend becomes cluttered with unnecessary entries or when specific datasets are irrelevant for the context of the presentation.
Common scenarios where skipping is beneficial include:
- Simplifying the visualization: When a plot contains multiple datasets and you want to focus only on the most relevant ones.
- Highlighting key results: In presentations or reports, you may wish to emphasize specific findings while omitting others.
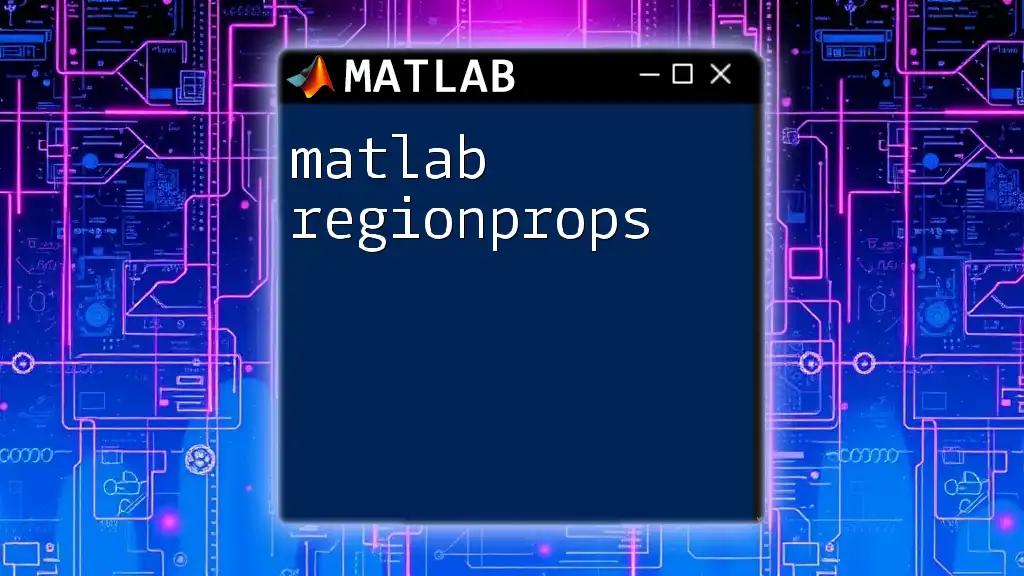
Basics of Creating Legends in MATLAB
Creating legends in MATLAB is straightforward, but it’s crucial to know the basics of plotting before diving into legend adjustments.
Creating a Simple Plot
To begin, you need to master basic plotting commands and their syntax. Here is a simple example of plotting and adding a legend:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
figure;
plot(x, y1, '-r', 'DisplayName', 'Sine');
hold on;
plot(x, y2, '-b', 'DisplayName', 'Cosine');
legend show;
In this example, two datasets—sine and cosine functions—are plotted with corresponding display names.
Understanding `DisplayName` Property
The `DisplayName` property is crucial for legends, as it specifies the names that will appear in the legend for each plotted dataset. Here is how you can set a display name:
plot(x, y1, '-r', 'DisplayName', 'Sine Function');
This sets the legend entry to "Sine Function." Properly naming your datasets not only helps in creating a clearer legend but also allows you the flexibility to control how your data is represented.
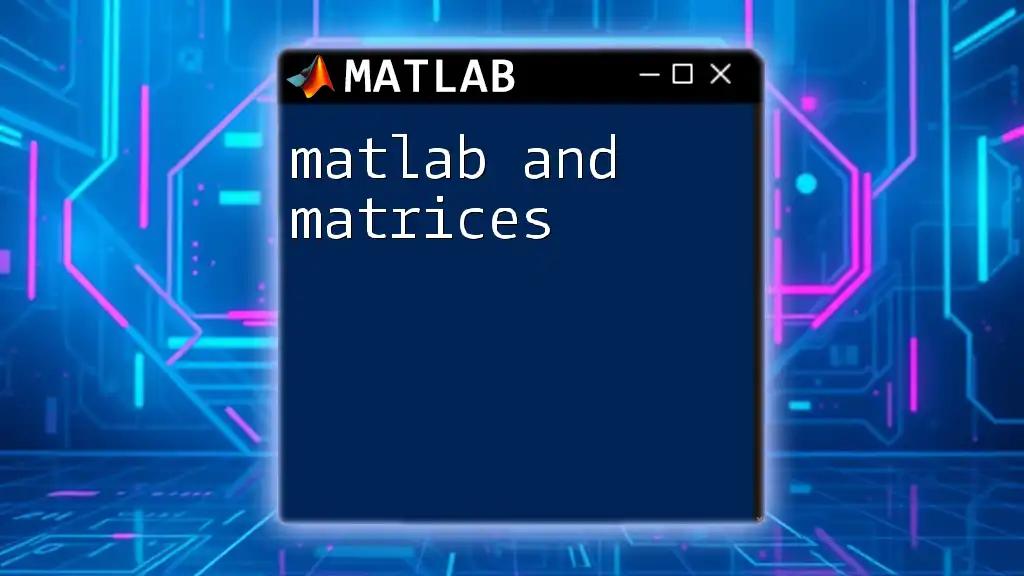
Skipping Entries in Legends
Introduction to Skipping Entries
Skipping entries means excluding certain datasets from appearing in the legend. This feature enhances the clarity of visualizations when presenting multiple data series.
Methods to Skip Legend Entries
Using the `legend` Function
One straightforward way to skip entries in the legend is to directly specify which entries you want to appear. For example:
plot(x, y1, '-r', 'DisplayName', 'Sine');
plot(x, y2, '-b', 'DisplayName', 'Cosine');
legend({'Sine'}, 'Location', 'best');
In this snippet, only the "Sine" entry is included in the legend while the "Cosine" entry is omitted.
Using `NaN` Value for Skipping
Another approach is to use `NaN` values in the data you wish to skip. By plotting `NaN` values, those datasets will not show up in the legend. Here's how:
y3 = NaN(size(x));
plot(x, y1, '-r', 'DisplayName', 'Sine');
plot(x, y3, '-b', 'DisplayName', 'Skipped Entry');
plot(x, y2, '-g', 'DisplayName', 'Cosine');
legend show;
In this case, since `y3` consists entirely of `NaN` values, it does not generate a visible line or legend entry, effectively skipping it.
Conditional Plotting
Conditional plotting allows for dynamic skipping of entries based on specified criteria. For instance, you can plot data only if a condition is met:
condition = rand(size(x)) > 0.5;
plot(x(condition), y1(condition), '-r', 'DisplayName', 'Sine (Condition Met)');
plot(x(~condition), y2(~condition), '-b', 'DisplayName', 'Cosine (Condition Not Met)');
legend show;
In this example, only the sine function that meets the condition is plotted, while the cosine function is conditionally included or excluded, allowing for a cleaner legend.
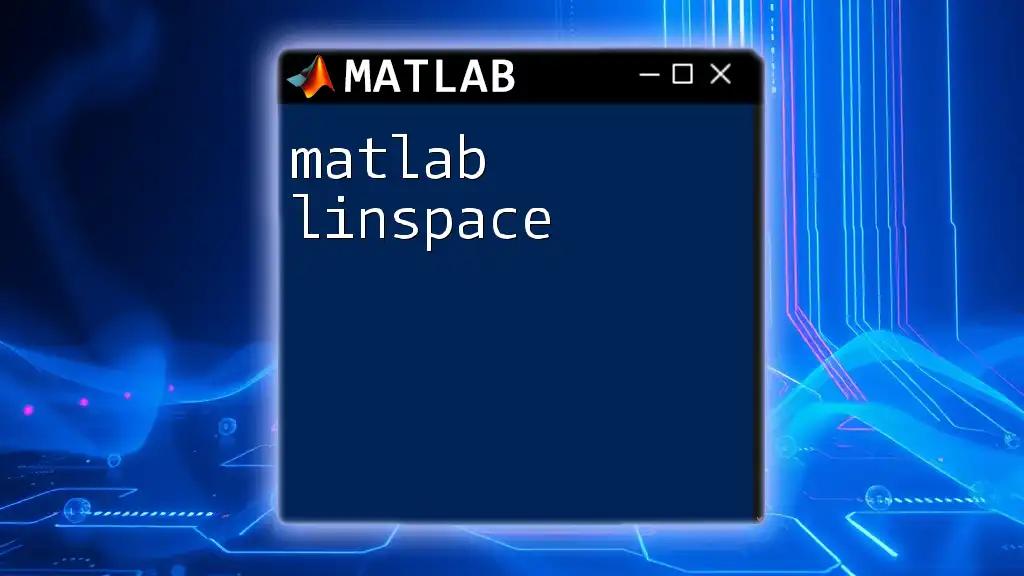
Best Practices for Legends
Keeping Legends Informative
To maximize the effectiveness of your legends, ensure that they provide concise and relevant information. A good practice is to only include entries that matter for the specific audience or context, ensuring that the plot remains readable.
Positioning of Legends
The positioning of your legends can significantly impact the clarity of your visual representation. Optimal placement can prevent overlap with data points. For example, you might change the location of your legend with:
legend({'Sine', 'Cosine'}, 'Location', 'northeast');
Choosing the right location helps maintain focus on the data without obscuring any vital elements of the plot.
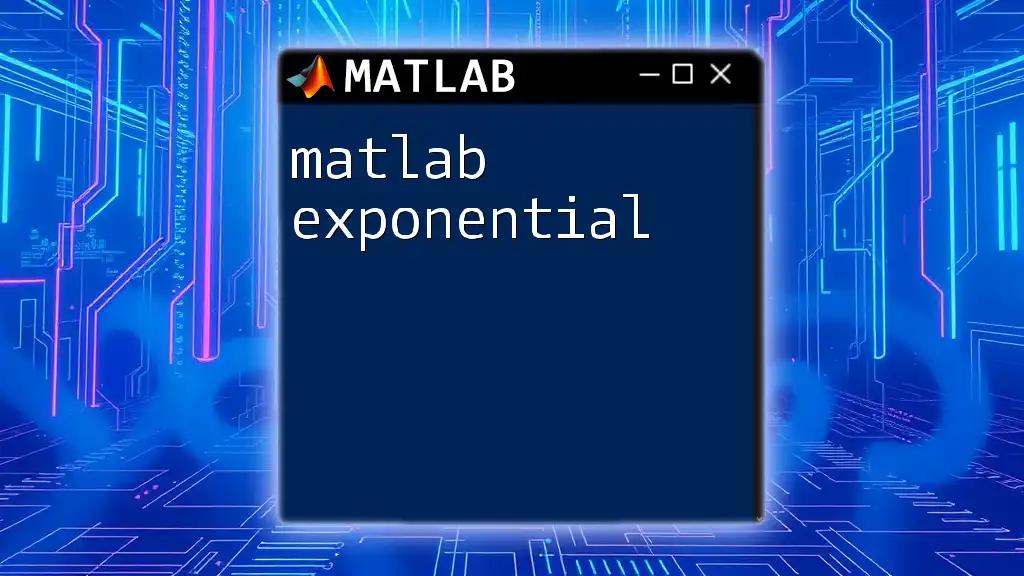
Conclusion
In summary, mastering how to effectively manage your legends, particularly through methods to skip entries, is an essential skill in MATLAB. Utilizing the strategies outlined above will improve the clarity of your visualizations, allowing your audience to grasp the information you aim to convey swiftly.
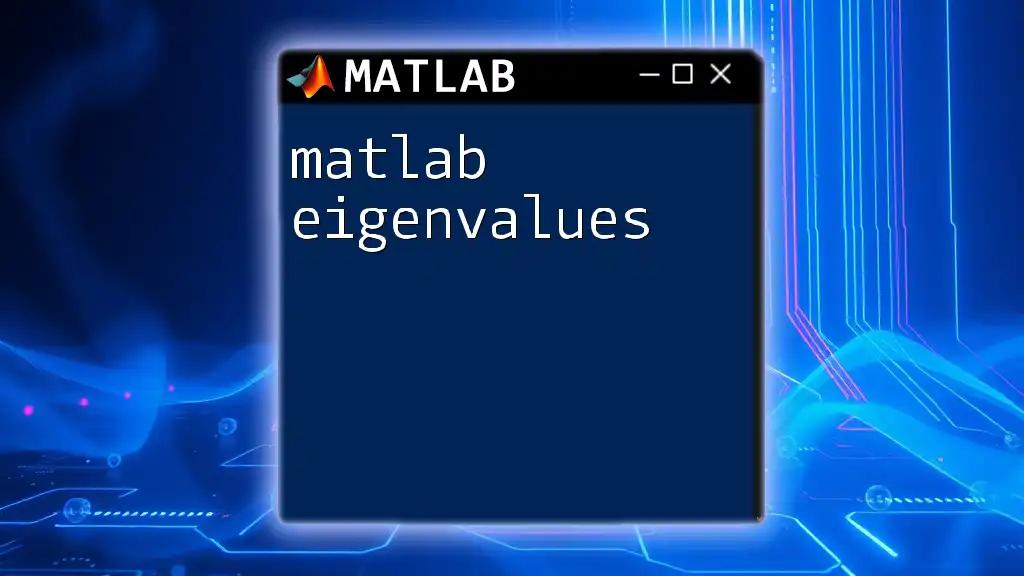
Additional Resources
For those looking to deepen their understanding, consider exploring MATLAB documentation and online tutorials. Engaging with community forums and courses can also provide valuable insights and practical experiences.
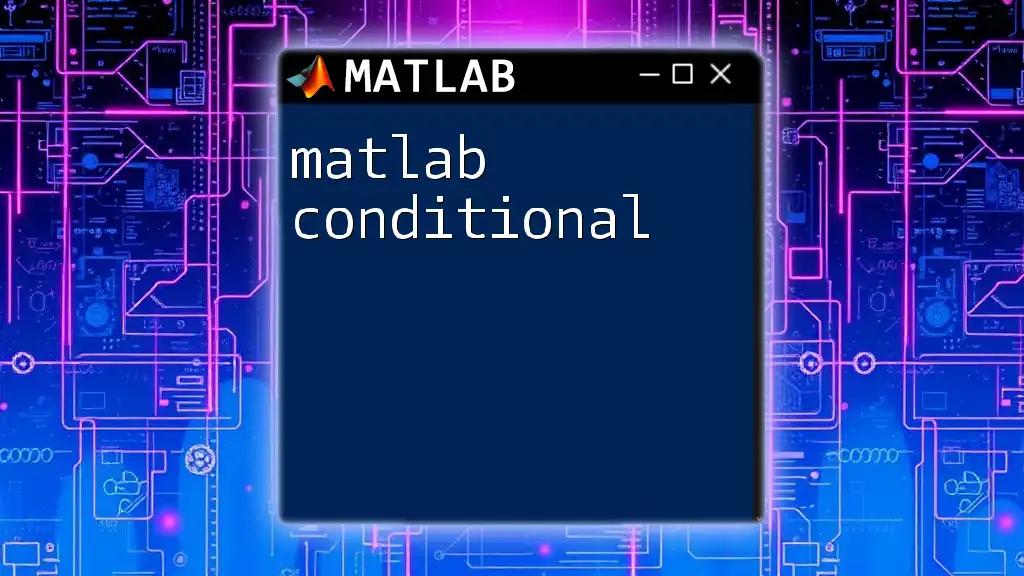
Call to Action
Start practicing today! Challenge yourself to find datasets and apply the techniques discussed above to skip legend entries. By doing so, you’ll enhance your skills in MATLAB and create clear, impactful visualizations.