The `eigs` function in MATLAB efficiently computes a specified number of eigenvalues and corresponding eigenvectors of a matrix, particularly useful for large sparse matrices.
Here’s a code snippet to demonstrate its usage:
% Define a sparse matrix
A = sparse([1, 2; 2, 1]);
% Compute the largest eigenvalue and eigenvector
[eigenvectors, eigenvalues] = eigs(A, 1);
Understanding Eigenvalues and Eigenvectors
What are Eigenvalues and Eigenvectors?
Eigenvalues and eigenvectors are fundamental concepts in linear algebra. Given a square matrix \( A \), an eigenvalue \( \lambda \) is a scalar that satisfies the equation:
\[ A \mathbf{v} = \lambda \mathbf{v} \]
where \( \mathbf{v} \) is a non-zero vector known as the eigenvector. In essence, when a matrix \( A \) acts on an eigenvector \( \mathbf{v} \), it transforms it into a vector that is a scaled version of \( \mathbf{v} \). This property is crucial in various applications, such as stability analysis, vibrations analysis, and data reduction techniques such as Principal Component Analysis (PCA).
How Eigenvalues and Eigenvectors are Calculated
Eigenvalues and eigenvectors can typically be found by solving the characteristic equation:
\[ \text{det}(A - \lambda I) = 0 \]
where \( I \) is the identity matrix of the same size as \( A \). The solutions to this polynomial equation give the eigenvalues, and substituting these values back into \( (A - \lambda I)\mathbf{v} = 0 \) allows you to compute the corresponding eigenvectors.
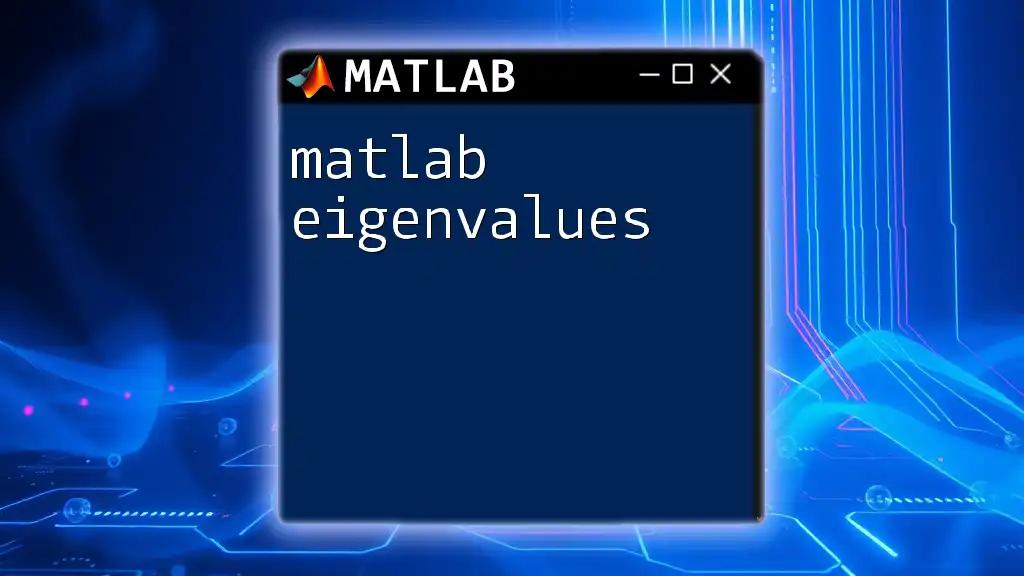
Introduction to `eigs`
What is the `eigs` Function?
The `eigs` function in MATLAB is used for computing a subset of eigenvalues and eigenvectors of a large matrix, particularly when the matrix is sparse or when only a few eigenvalues are of interest. This contrasts with the `eig` function, which computes all eigenvalues and eigenvectors, potentially leading to high computational costs and inefficiencies when dealing with large datasets.
Syntax of `eigs`
The basic syntax for using the `eigs` function is as follows:
[V, D] = eigs(A, k)
In this syntax:
- `A` is the square matrix whose eigenvalues and eigenvectors are to be computed.
- `k` denotes the number of eigenvalues and corresponding eigenvectors to compute.
- `V` will store the eigenvectors, while `D` contains the eigenvalues on the diagonal.

Key Features of `eigs`
Performance Benefits
The `eigs` function is particularly beneficial when dealing with sparse matrices. Sparse matrices, where most of the elements are zero, allow MATLAB to use memory and computational resources more efficiently compared to dense matrices. This means that computations are faster and can handle larger datasets more effectively.
Options for Computing Eigenvalues
The `eigs` function also allows for flexibility in determining which eigenvalues you wish to compute:
- You can specify the largest or smallest eigenvalues based on their absolute values.
- The function can be directed to compute a specified number of eigenvalues, making it suitable for applications where only specific values are relevant.
- Unlike the `eig` function, `eigs` can also compute eigenvalues for non-symmetric matrices, which broadens its applicability.
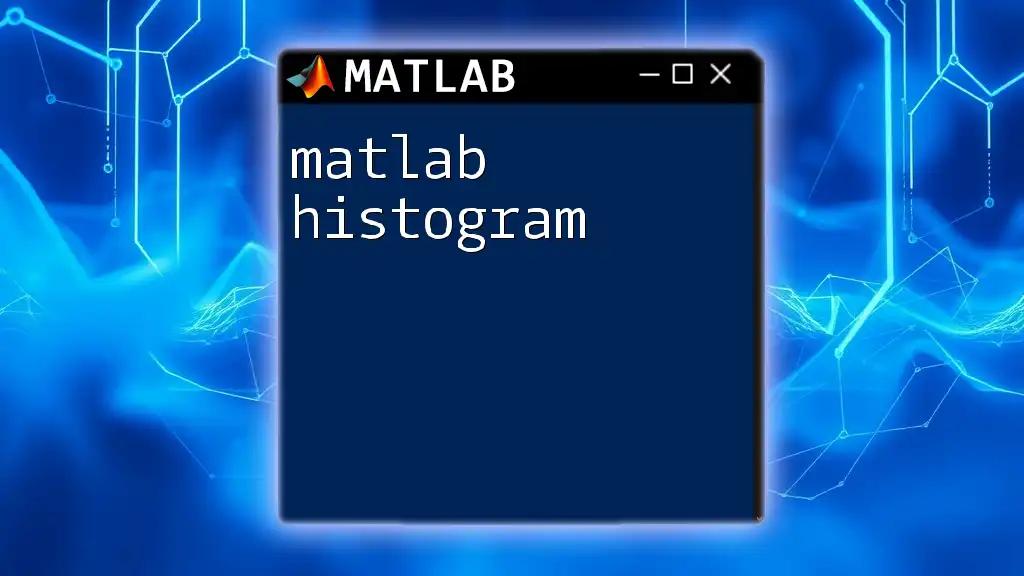
Detailed Example
Example Problem
Let’s consider a matrix \( A \) that represents a simple system and we want to find its eigenvalues and eigenvectors.
Step-by-Step Solution
-
Setting up the matrix: To define the matrix in MATLAB, you can use:
A = [4 1; 2 3];
-
Using `eigs` to calculate eigenvalues: Now, you can use the following command to calculate the eigenvalues and eigenvectors:
[V, D] = eigs(A);
-
Explanation of the output matrices:
- `V` is the matrix of eigenvectors. Each column of `V` corresponds to an eigenvector of the matrix \( A \).
- `D` is a diagonal matrix containing the eigenvalues on its diagonal. The value of each eigenvalue corresponds to the scale factor of the corresponding eigenvector.
Analyzing the Results
After executing the above commands, you can interpret the eigenvalues and eigenvectors computed. Each eigenvalue describes the factor by which its corresponding eigenvector is stretched or squished during the linear transformation defined by matrix \( A \).
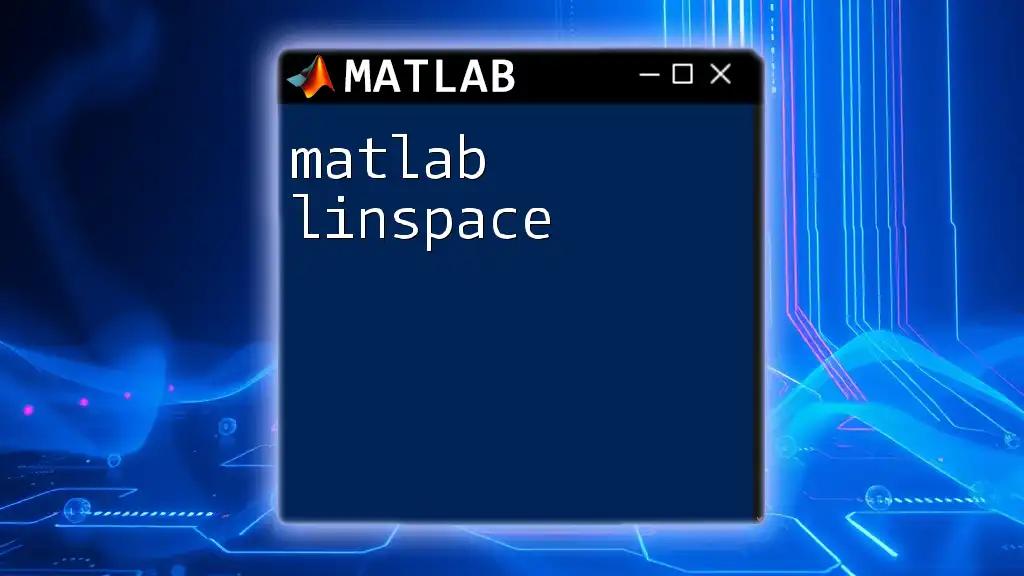
Advanced Uses of `eigs`
Using `eigs` with Sparse Matrices
An important feature of `eigs` is its ability to handle large, sparse matrices very efficiently. Consider a sparse matrix \( S \):
S = sparse(1000, 1000);
S(1, 1) = 1; S(2, 2) = 2; S(3, 3) = 3;
[V, D] = eigs(S, 5);
In this example, the `sparse` function creates a 1000x1000 sparse matrix with only three non-zero elements. The `eigs` function computes the 5 largest eigenvalues and their corresponding eigenvectors, effectively managing memory and computation time.
Customizing the `eigs` Function
Specifying the Largest or Smallest Magnitude
You can control whether `eigs` calculates the largest or smallest eigenvalues by passing additional options. For instance, to compute the smallest eigenvalues, use:
opts.issym = 1;
[V, D] = eigs(A, 5, 'smallestabs', opts);
Here, including `opts.issym = 1` indicates that \( A \) is symmetric, which can improve the efficiency of the computation.
Setting Options with Structs
You can also customize the behavior of the `eigs` function by creating an options structure. Common options include setting the matrix to be symmetric or specifying the maximum number of iterations. For example:
opts.maxit = 1000; % Set the maximum number of iterations
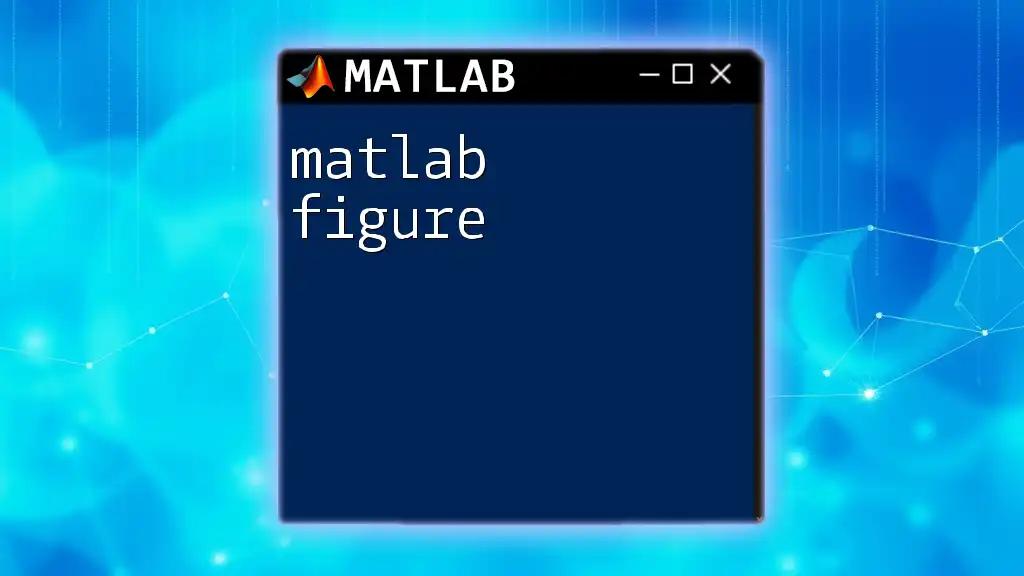
Common Issues and Troubleshooting
Errors in Using `eigs`
When using the `eigs` function, users may encounter errors related to matrix dimensions or types. Common mistakes include trying to compute eigenvalues from non-square matrices, or not ensuring that the matrix is symmetric when specified.
Performance Issues
To optimize performance, especially with large matrices, consider the following:
- Ensure the sparsity of your matrices by utilizing the `sparse` function in MATLAB.
- Preallocate matrices where possible to minimize memory reallocation during execution.
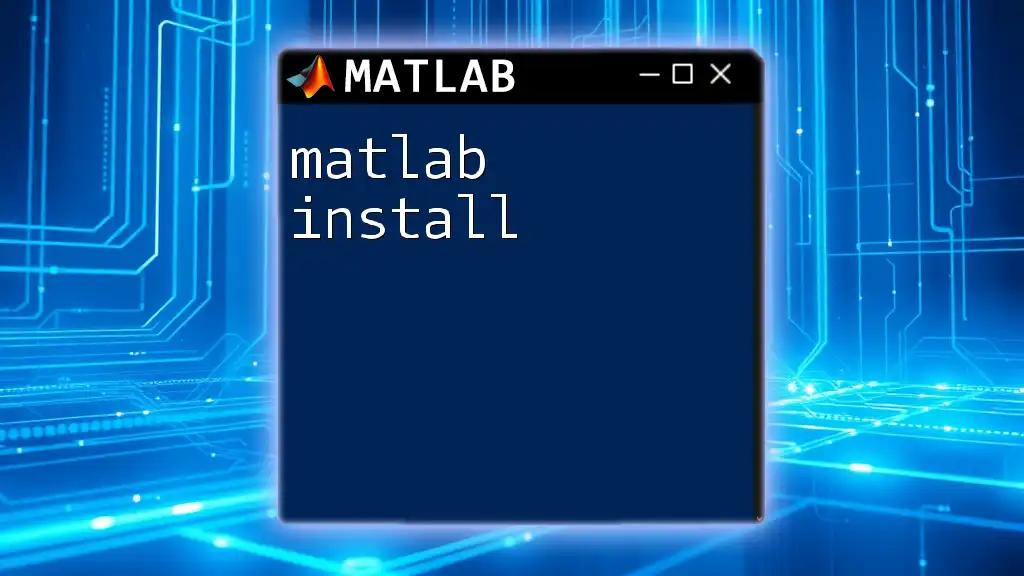
Conclusion
The MATLAB `eigs` function plays a crucial role in efficiently computing eigenvalues and eigenvectors, especially for large and sparse matrices. Understanding its functionalities empowers users to leverage its full potential in various mathematical and engineering contexts. By practicing the techniques and examples outlined, readers can deepen their understanding of this powerful tool and apply it effectively in their projects.
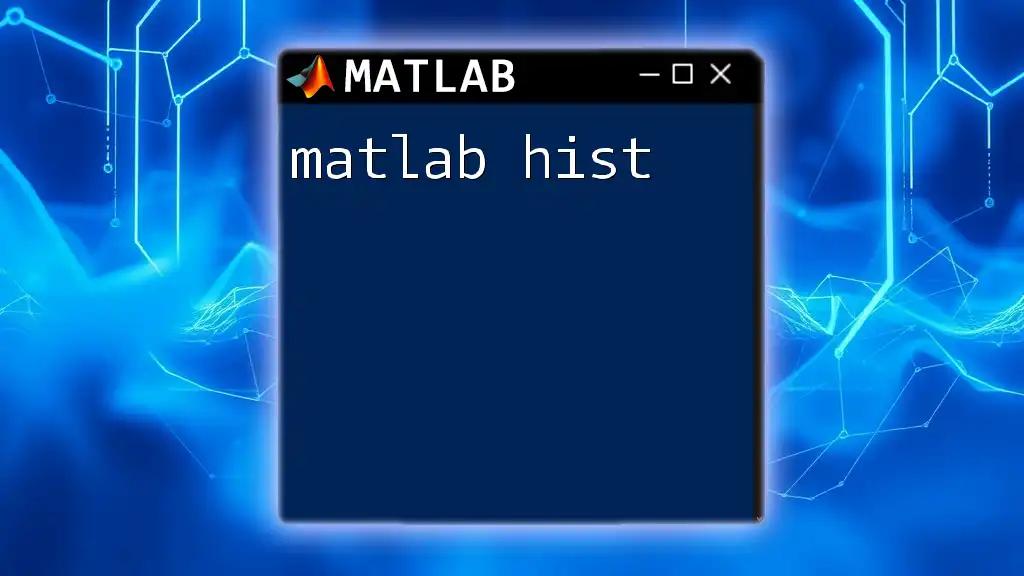
Additional Resources
To further explore the capabilities of `eigs`, refer to the official MATLAB documentation. For more in-depth knowledge, consider MATLAB textbooks or online courses. Engaging with community forums can also provide valuable insights and troubleshooting tips for mastering MATLAB commands efficiently.