The `imshow` function in MATLAB displays an image in a figure window, allowing users to visualize matrix data as an image.
Here’s a simple example of how to use `imshow`:
% Read an image and display it
img = imread('example_image.png'); % Load an image file
imshow(img); % Display the image
What is `imshow`?
`imshow` is a built-in function in MATLAB that is essential for anyone working in image processing or computer vision. It serves the primary purpose of displaying images in a figure window, making it possible to visualize image data quickly and effectively. By default, `imshow` can handle various image formats, showcasing grayscale images, RGB images, and binary images clearly and intuitively.
The output of the `imshow` function is displayed in a MATLAB figure window, where the user can see the rendered image based on the provided data. Understanding how `imshow` presents different types of images is critical, as it can greatly affect how you interpret and analyze the visual information.
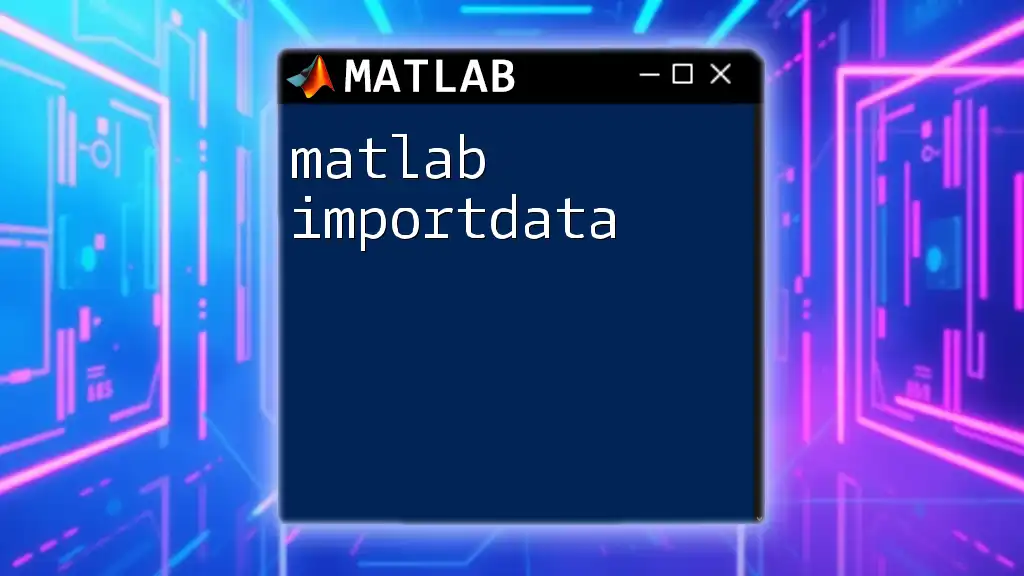
Syntax of `imshow`
Basic Syntax
The basic syntax of the `imshow` function is straightforward:
imshow(I)
Here, `I` represents the image data you want to display. Whether it’s a matrix representing a grayscale image or a 3D array for an RGB image, `I` is the central argument required to show any image using this function.
Advanced Syntax Options
For more control over the display, `imshow` accepts additional parameters. Here’s how you might use them:
imshow(I, 'InitialMagnification', 'fit')
In this example, the additional parameter `'InitialMagnification'` dictates how the image will be scaled when displayed. Using `'fit'` ensures that the entire image fits within the figure window, allowing for an optimal viewing experience.
Other important parameters include:
- `'DisplayRange'`: This allows you to specify the intensity value range to show. This can be very useful when displaying high dynamic range images or correcting contrast.
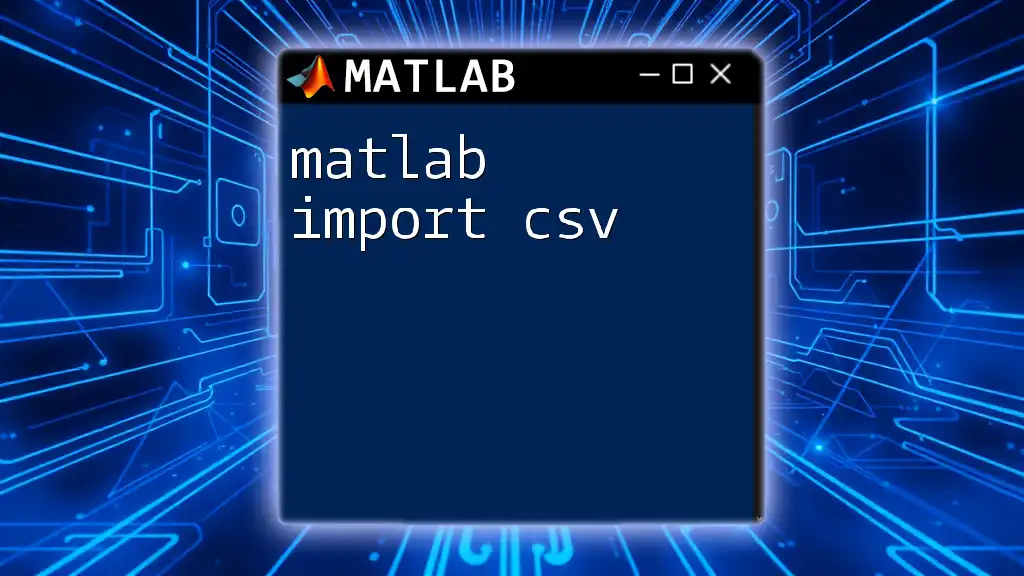
Preparing Image Data for `imshow`
Image Formats Supported by `imshow`
When working with images in MATLAB, it’s important to know what formats are supported by `imshow`. Common formats include:
- JPEG
- PNG
- BMP
- TIFF
Each of these formats can be easily loaded into MATLAB using the `imread` function, making `imshow` highly versatile.
Loading Images into MATLAB
Before you can use `imshow`, you need to load your image data into the environment. The simplest way to do this is by using the `imread` function:
I = imread('image.jpg');
In this code snippet, `imread` reads the specified image file ('image.jpg') and stores the image data in the variable `I`. It's essential to ensure that the file path is correct and accessible.
Image Preprocessing
Once you've loaded your image, it’s vital to ensure that the data type is appropriate for `imshow`. Typically, images are stored in types like `uint8` or `double`. You may often need to convert your image data type to ensure proper visualization:
I = im2uint8(I);
This conversion ensures that the range of pixel values is correctly represented in uint8 format, which is standard for image display.
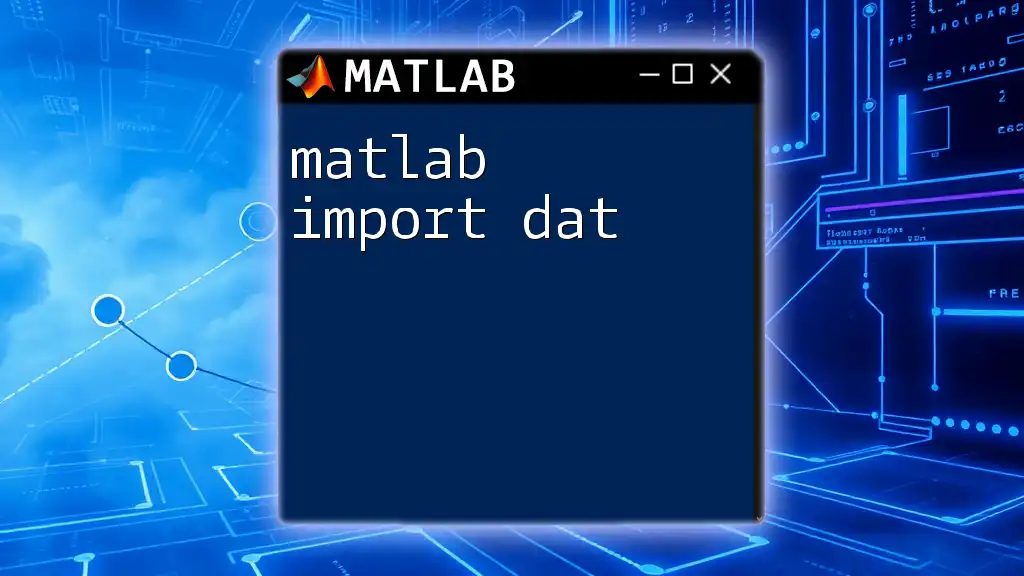
Displaying Images with `imshow`
Basic Display
To display an image using the `imshow` function, you can simply input the image variable:
imshow(I);
Upon executing this command, a new figure window appears, showcasing the image `I`. This is the most basic usage of `imshow`, and it allows you to see the loaded image in its raw form.
Adjusting Image Display Settings
MATLAB’s `imshow` function allows for customization of how images are displayed. You can modify several display parameters to enhance your image viewing experience. One common adjustment is setting the display range for the image intensity values:
imshow(I, [minValue, maxValue]);
In this example, replacing `minValue` and `maxValue` with appropriate intensity values allows you to control the contrast and brightness of the displayed image.
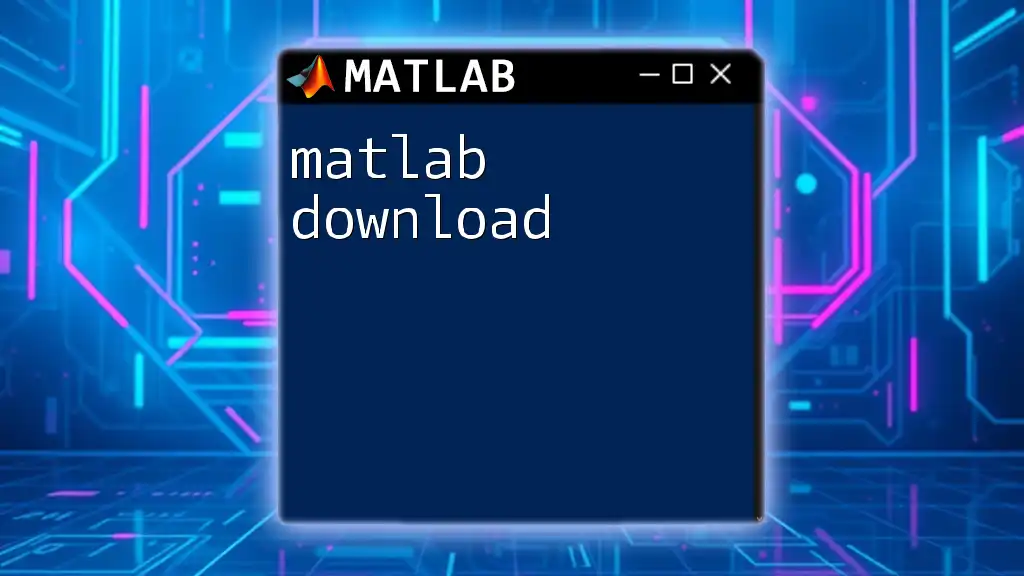
Customizing the Display of Images
Using Additional Parameters
Beyond just displaying the image, `imshow` offers a range of additional parameters to customize your viewing experience. For instance, you can specify a background color or define a specific axes to display the image, enhancing your visual representation in complex projects.
Overlaying Annotations or Graphics
Overlying graphics such as text or shapes can be helpful for providing context or labeling parts of your image. Here’s how you can do this:
imshow(I);
hold on;
text(10, 10, 'Sample Text', 'Color', 'red');
hold off;
In this snippet, the `text` function places a red label at the coordinates (10, 10). This approach enables users to annotate critical features directly on the displayed image, further enriching the data visualization.
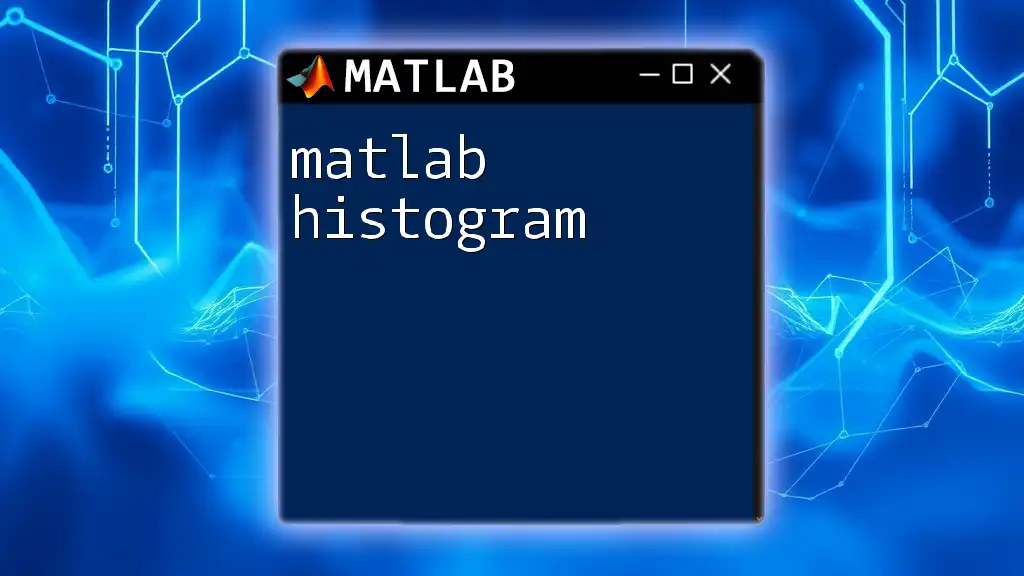
Common Errors and Troubleshooting
Identifying Common Error Messages
When using `imshow`, you may encounter various error messages. One frequent issue occurs when the input data type is not appropriate. For instance, the common error message "Data must be in the range [0, 1]" indicates that the data values need to be normalized before display.
Troubleshooting Tips
To resolve issues effectively, ensure that:
- The image data is in the correct format and range.
- You are using valid parameters and that your image paths are correct.
Additionally, consult the MATLAB documentation for insights and troubleshooting advice.
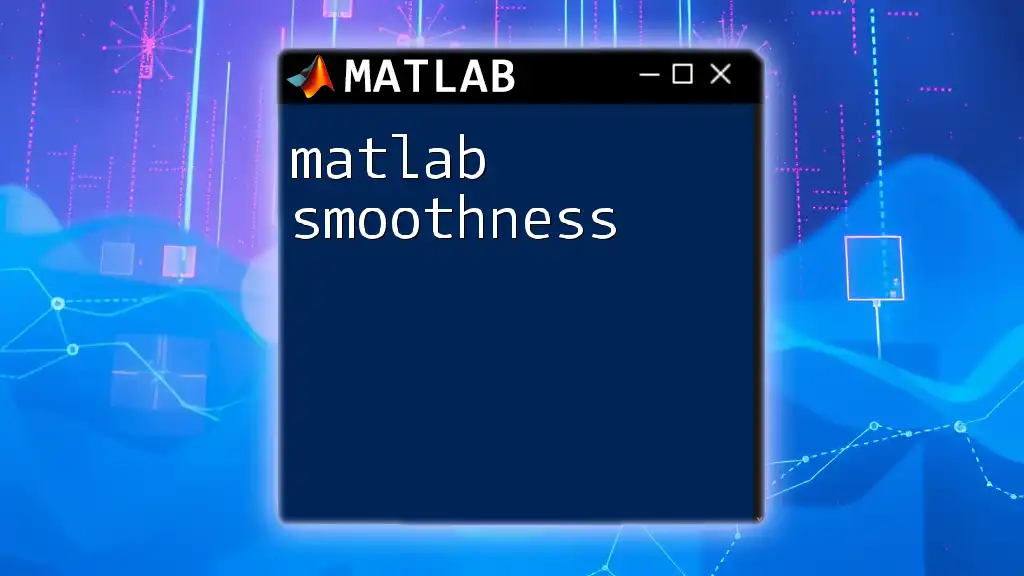
Practical Applications of `imshow`
Image Analysis in Research
In academic and research settings, `imshow` plays a crucial role in analyzing biological images or satellite data. For example, researchers can visualize microscopy images to make critical observations about cell structures. Quickly displaying these images facilitates better understanding and efficient communication of research findings.
Computer Vision Projects
In computer vision applications, `imshow` helps showcase algorithm outputs, enabling developers to visualize changes after processing steps. Projects that involve image transformations, object detection, or image filtering heavily rely on this visualization function.
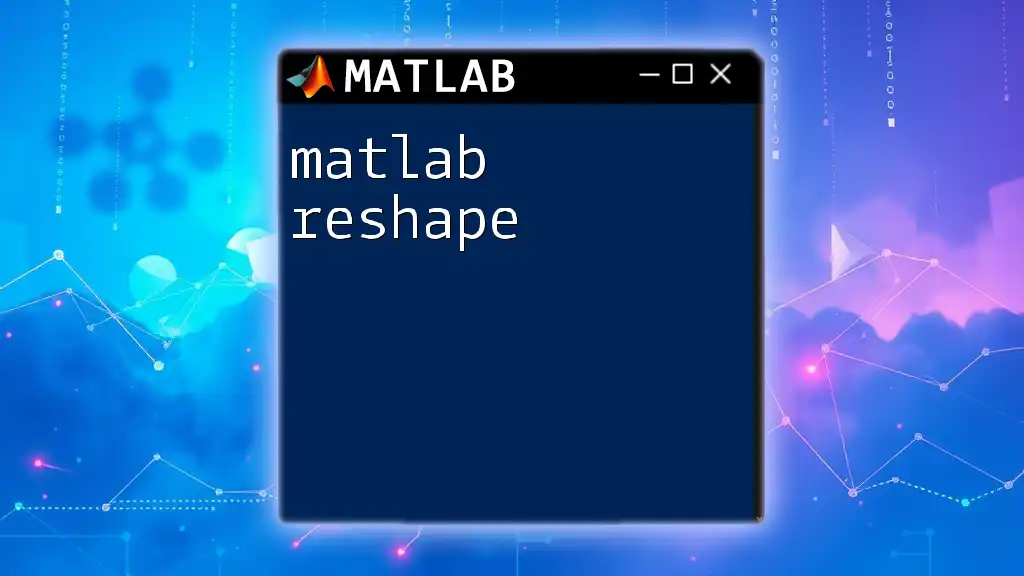
Conclusion
The `imshow` function is a fundamental tool for anyone delving into image processing with MATLAB. Its simplicity, coupled with its powerful customization options, allows users to explore and analyze images effectively. By practicing with `imshow` and diving deeper into MATLAB's capabilities, individuals can enhance their image processing tasks and insights.
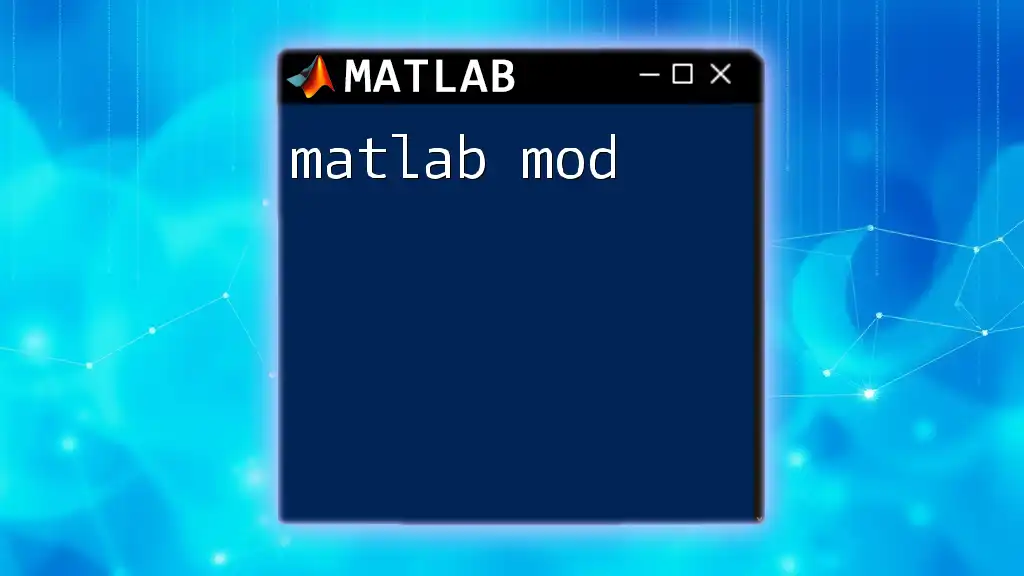
Additional Resources
For further enrichment, users are encouraged to explore MATLAB's official documentation, where they can delve deeper into the nuances of `imshow` and related functions. Additionally, various online courses and tutorials are available to help users master image processing basics and advanced techniques.
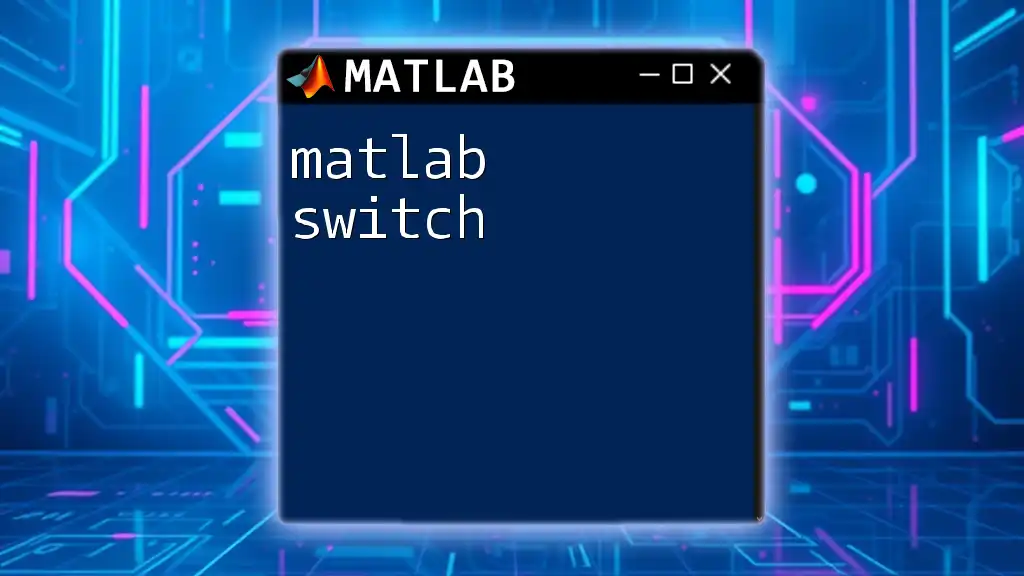
Frequently Asked Questions
What types of images can I use with `imshow`?
You can use various image types such as JPEG, PNG, BMP, and TIFF with `imshow`.
Why is my image displaying incorrectly in MATLAB?
The most common reasons include incorrect data type or out-of-range pixel values. Ensure your image data is appropriately formatted before calling `imshow`.
How do I adjust the size of the displayed image?
You can use the `InitialMagnification` parameter in `imshow` to control how the image is displayed within the figure window. Use options like `'fit'` to fit the image to the window size.