The `fftshift` function in MATLAB rearranges the zero frequency component to the center of the spectrum for a more intuitive visualization of the frequency domain representation of signals.
Here's a code snippet demonstrating its use:
% Example: Use fftshift to center the zero-frequency component
x = linspace(-10, 10, 1024); % Create a sample signal
y = sin(x); % Sample signal (sine wave)
Y = fft(y); % Compute the Fast Fourier Transform
Y_shifted = fftshift(Y); % Shift the zero frequency component to the center
freqs = linspace(-512, 511, 1024); % Frequency axis for visualization
% Plot the original and shifted FFT
figure;
subplot(2,1,1);
plot(freqs, abs(Y)); % Plot original FFT
title('Original FFT');
xlabel('Frequency');
ylabel('Magnitude');
subplot(2,1,2);
plot(freqs, abs(Y_shifted)); % Plot shifted FFT
title('Shifted FFT using fftshift');
xlabel('Frequency');
ylabel('Magnitude');
Understanding `fftshift`
What is `fftshift`?
`fftshift` is a built-in MATLAB function that rearranges the values of a Fourier-transform output. This function moves the zero frequency component to the center of the spectrum, which is crucial for visualizing frequency components accurately. In many applications, especially in signal processing and communications, it is often essential to analyze the frequency response centered around \(0\) Hz for clarity and understanding.
The Need for `fftshift`
When performing an FFT, the output is arranged such that the lower frequency components are at the start of the array and the higher frequency components are at the end. However, in many cases—like when visualizing the frequency spectrum—shifting the zero frequency component to the center provides a better understanding of how the signal behaves in the frequency domain. Leaving the spectrum unshifted can lead to misinterpretations of frequency content and complicate further analyses.
Common use cases include:
- Analyzing periodic signals.
- Filtering applications that require a visual inspection of frequency responses.
- Sound signal analysis and manipulation.
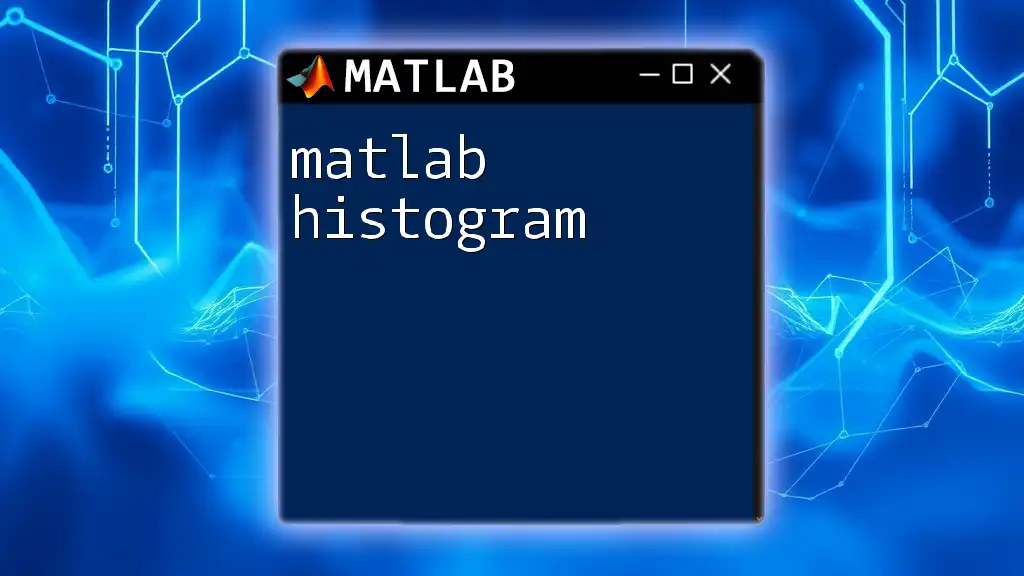
Basic Usage of `fftshift`
Syntax and Parameters
The basic syntax of `fftshift` is straightforward:
Y = fftshift(X);
Where:
- X is the input array representing the result of an FFT computation.
- Y is the output array with the zero frequency component shifted to the center.
Example of Using `fftshift`
To illustrate how `fftshift` can be applied, consider a simple example where a noisy cosine signal is analyzed:
% Sample MATLAB code for demonstration
Fs = 1000; % Sampling frequency
t = 0:1/Fs:1-1/Fs; % Time vector
x = cos(2*pi*100*t) + randn(size(t)); % Sample signal
X = fft(x); % Compute FFT
Y = fftshift(X); % Shift the zero frequency component
In this code:
- We define a sampling frequency and a time vector.
- A sample signal composed of a cosine wave and random noise is generated.
- The Fourier transform of this signal is computed using `fft`.
- Finally, `fftshift` is called to center the zero frequency component in the output.
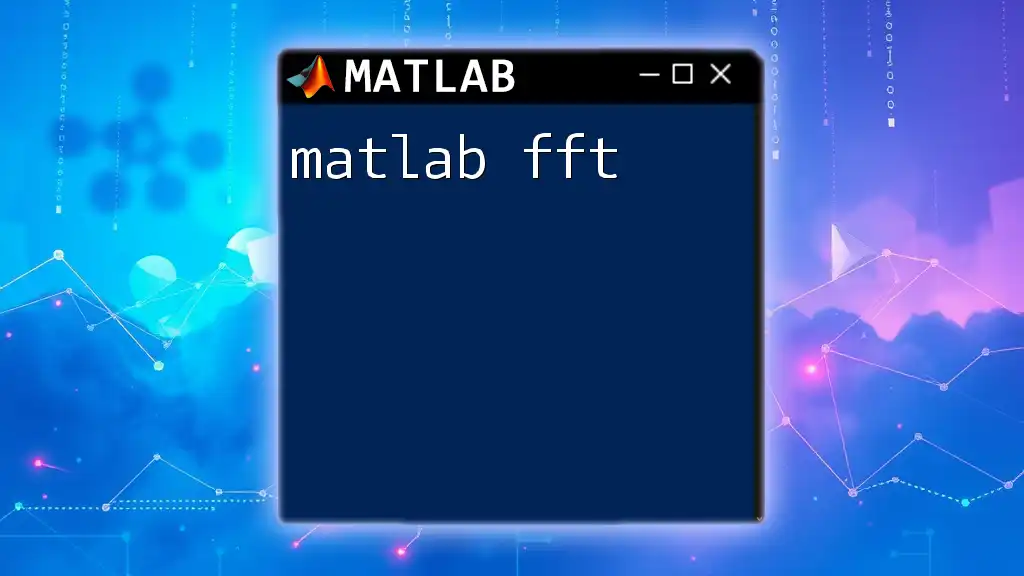
Visualizing `fftshift`
Plotting Before and After `fftshift`
Visualization is key to understanding the effects of `fftshift`. Here’s how to plot the results both before and after applying `fftshift`:
% Visualization code
figure;
subplot(2,1,1);
plot(abs(X));
title('Magnitude Spectrum (Before fftshift)');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
subplot(2,1,2);
plot(abs(Y));
title('Magnitude Spectrum (After fftshift)');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
In this example:
- The first subplot shows the magnitude spectrum of the FFT result before the shift, which may lead to an unclear interpretation of frequency components.
- The second subplot displays the magnitude spectrum after applying `fftshift`, providing a clear view of how the components are centered around zero frequency. This visualization helps identify signal characteristics more intuitively.
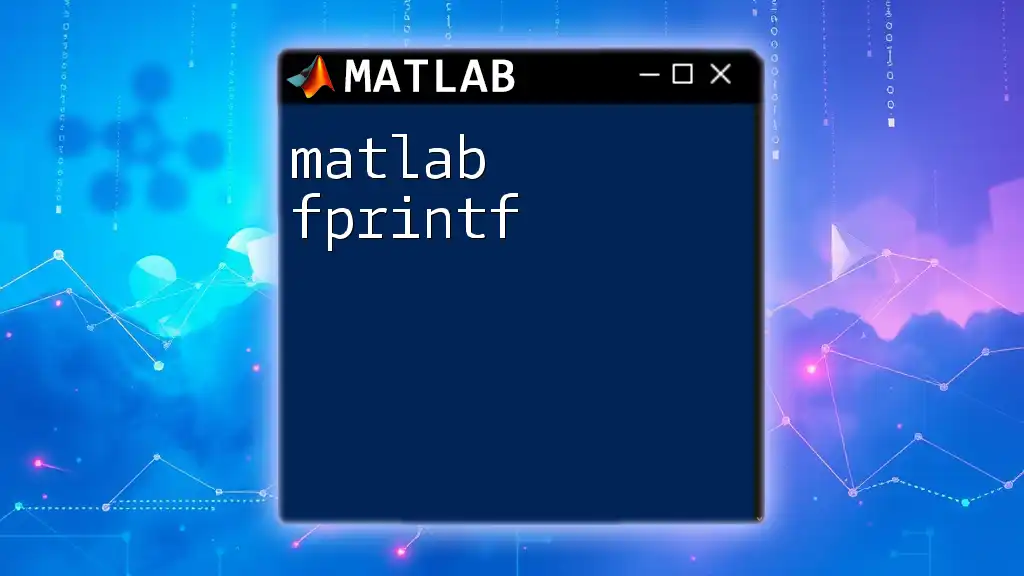
Practical Applications of `fftshift`
Use in Signal Processing
In signal processing, `fftshift` is often used for filtering, modulation, and demodulation. When analyzing a signal’s frequency response, shifting the zero frequency component to the center allows engineers to conveniently inspect how various frequencies contribute to the signal. This understanding can guide decisions on whether to apply filters or adjust signal parameters.
Application in Image Processing
`fftshift` also finds a prominent place in image processing applications, particularly when transforming images into the frequency domain. For instance, consider a grayscale image analyzed using its 2D FFT:
% Image processing example
img = imread('example.png');
img_gray = rgb2gray(img);
F = fft2(img_gray);
F_shifted = fftshift(F);
In this code:
- An image is read and converted to grayscale.
- The 2D FFT of the grayscale image is computed using `fft2`.
- Finally, `fftshift` is applied to center the frequency components. This step is essential for analysis, especially when observing patterns, noise, or compression in images.
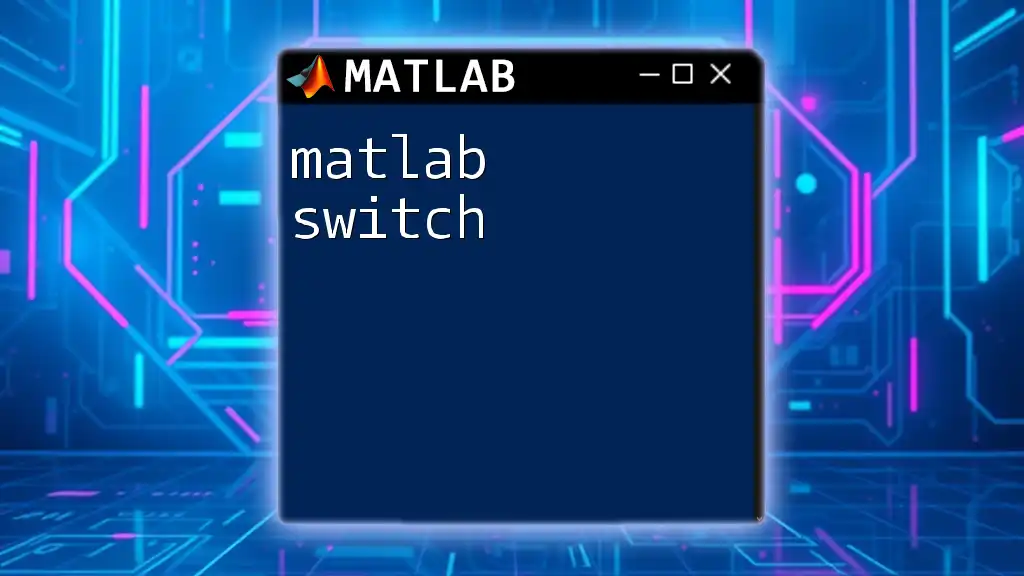
Key Considerations
Common Pitfalls
While `fftshift` is a powerful tool, it is important to be aware of common pitfalls. A misunderstanding of the shifting process can lead users to misinterpret frequency responses or apply the function inappropriately. Ensure that you apply `fftshift` only after computing the FFT to benefit fully from its capabilities.
Best Practices
To maximize the effectiveness of `fftshift`, consider the following best practices:
- Always visualize your data before and after using `fftshift` to confirm its effects.
- Use `fftshift` in combination with other signal processing functions for enhanced analysis.
- When working with multidimensional signals or images, remember that `fftshift` operates independently on each dimension, so incorporate it strategically to understand the overall behavior.
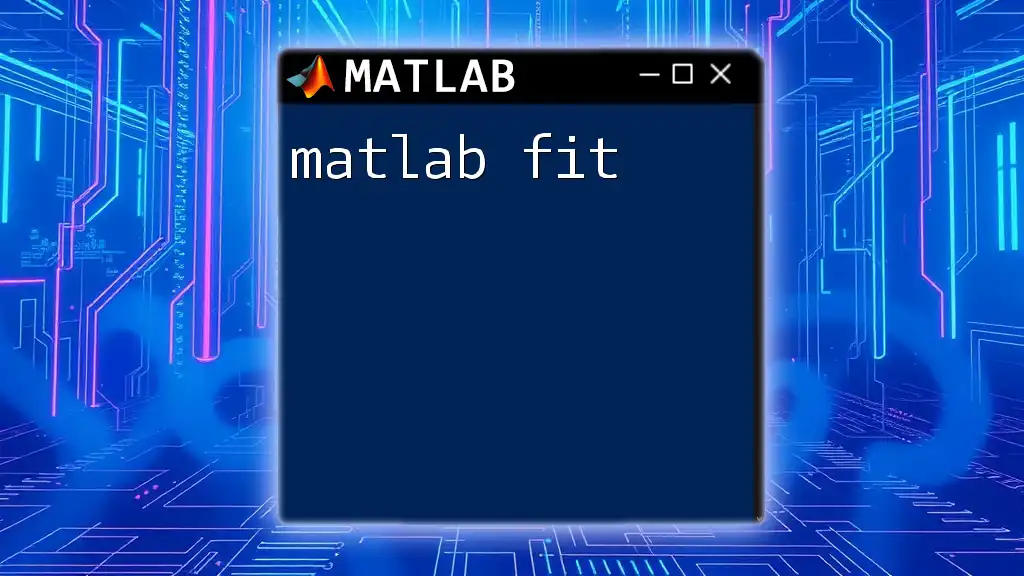
Conclusion
The `fftshift` function in MATLAB is essential for proper analysis and interpretation of frequency domain data. By centering the zero frequency component, users gain valuable clarity and insights into their signals and images. Practice using this function across various applications to enhance your proficiency in MATLAB.
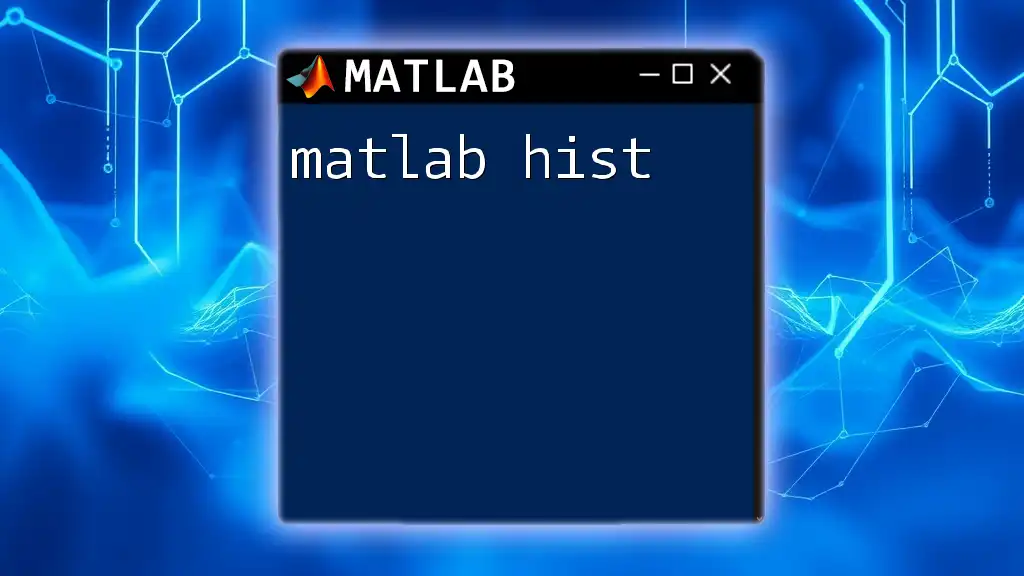
Additional Resources
For further learning, you can refer to the [MATLAB Documentation on `fftshift`](https://www.mathworks.com/help/matlab/ref/fftshift.html) and explore suggested readings on FFT and its applications in different domains.
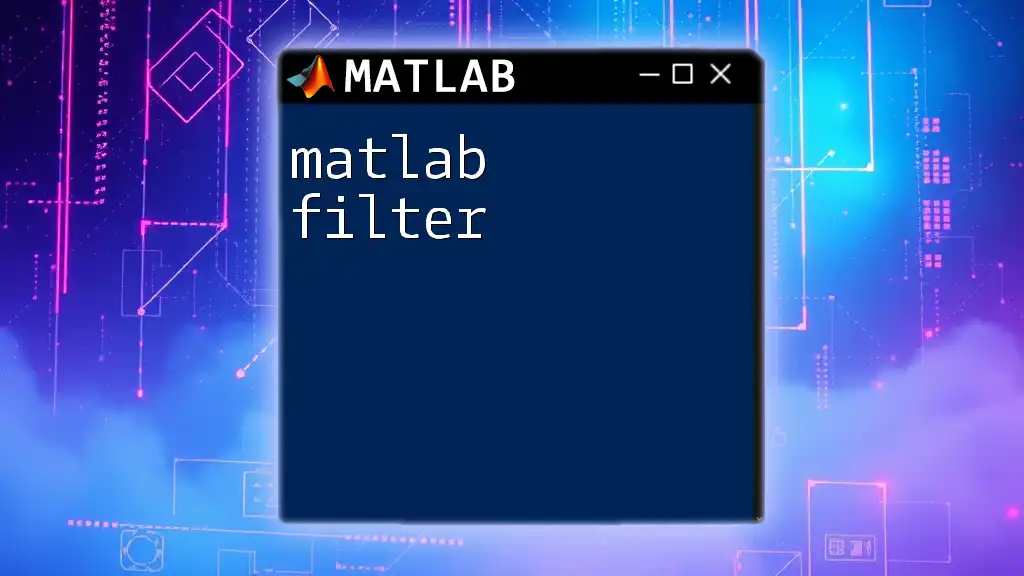
Call to Action
If you have any questions or experiences with `fftshift` that you'd like to share, please leave a comment below! Your insights are valuable and can help others in the MATLAB community.