`griddata` in MATLAB is used to interpolate scattered data points onto a grid for the purpose of creating surface plots or visualizing data more effectively. Here's a simple example of how to use `griddata` in MATLAB:
% Example of using griddata for interpolation
x = rand(1, 10); % Random x-coordinates
y = rand(1, 10); % Random y-coordinates
z = sin(x) + cos(y); % Function values at (x,y)
% Create a grid for interpolation
[xq, yq] = meshgrid(linspace(0, 1, 50), linspace(0, 1, 50));
zq = griddata(x, y, z, xq, yq); % Interpolated values on the grid
% Visualize the results
surf(xq, yq, zq);
shading interp;
title('Interpolated Surface using griddata');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
Introduction to griddata
What is griddata?
`griddata` is a powerful MATLAB function designed for interpolating scattered data. It allows users to estimate values at specific points based on known values scattered across a defined grid. This capability is crucial in data analysis, particularly when working with incomplete datasets where values have not been uniformly recorded.
Overview of interpolation in MATLAB
Interpolation is a method used in numerical analysis to derive new data points within the range of a discrete set of known data points. In MATLAB, this can involve several types of interpolation methods, including linear, nearest-neighbor, and cubic methods. Understanding how to utilize `griddata` effectively can significantly enhance your ability to model and analyze complex datasets.
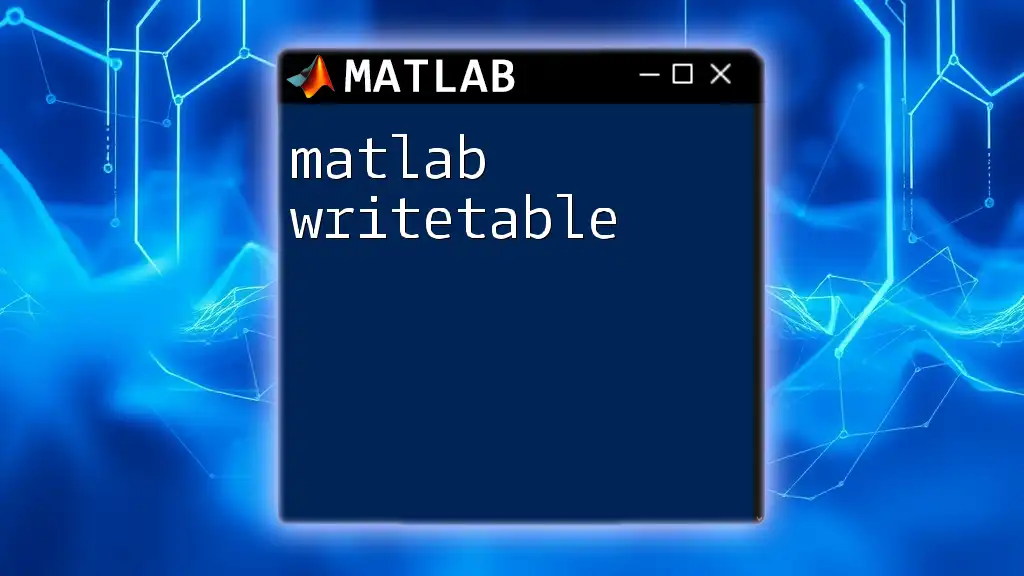
Syntax and Usage
Basic syntax of griddata
When using `griddata`, the basic syntax is straightforward and follows a consistent structure. The format you will encounter most frequently is:
Z = griddata(X, Y, Z, XI, YI)
- X, Y: Vectors containing the coordinates of the data points.
- Z: The values at the (X, Y) locations.
- XI, YI: The coordinates at which you want to interpolate the values.
Understanding this syntax is fundamental for effective use of `griddata`.
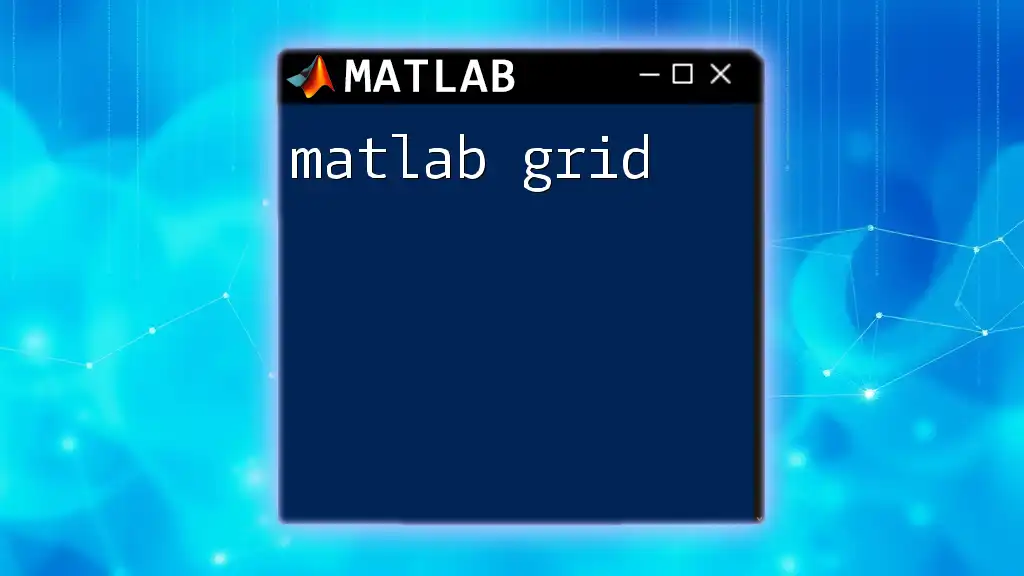
Understanding the Inputs
Input parameters detailed
- Input vectors (X, Y, Z)
The input parameters (X, Y, Z) represent the known data points. It's crucial that these vectors contain the same number of elements, as they define a set of (x, y) coordinates with corresponding z-values. For example:
x = [1, 2, 3, 4, 5];
y = [2, 3, 5, 1, 4];
z = [10, 20, 30, 40, 50];
In this snippet, `x` and `y` represent the coordinates of the scattered data, while `z` consists of the associated values at those coordinates.
- Output grid coordinates (XI, YI)
To generate interpolated values, you need to define the grid over which interpolation will occur. This can be done using MATLAB’s `meshgrid` function to create matrices from the vector inputs X and Y. Here is how you can set this up:
[XI, YI] = meshgrid(1:0.1:5, 1:0.1:5);
In this example, `XI` and `YI` will create a grid that spans from 1 to 5 in both axes, with a step of 0.1.
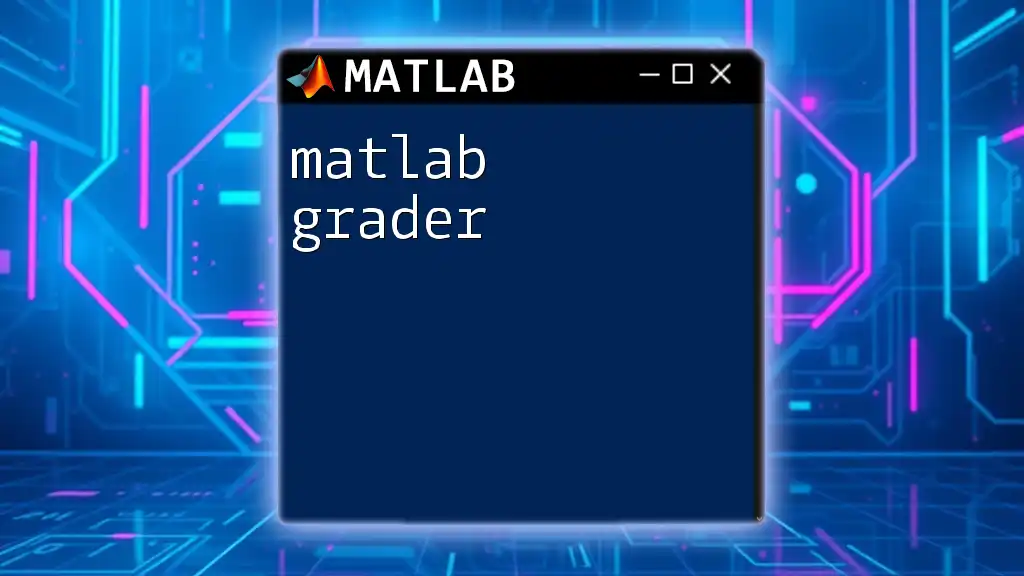
Interpolation Methods
Types of interpolation methods in griddata
- Linear Interpolation
This is the simplest method, which connects adjacent data points with straight lines. It’s often used when the data points are relatively evenly distributed. For example, to perform linear interpolation, you might use:
ZI = griddata(x, y, z, XI, YI, 'linear');
This approach is efficient for many applications, especially when a quick approximation is sufficient.
- Cubic Interpolation
Cubic interpolation provides smoother results by fitting a cubic polynomial to each cell of the grid. This method is especially useful when dealing with data that has a curved nature. Here’s how you can implement cubic interpolation:
ZI = griddata(x, y, z, XI, YI, 'cubic');
This method yields a more refined surface but may require more computational power than linear interpolation.
- Nearest Neighbor Interpolation
This method assigns the value of the nearest data point to the unknown locations. It is the simplest technique but may introduce abrupt changes in the resultant grid. Use it like this:
ZI = griddata(x, y, z, XI, YI, 'nearest');
This approach is particularly effective in categorical data applications.
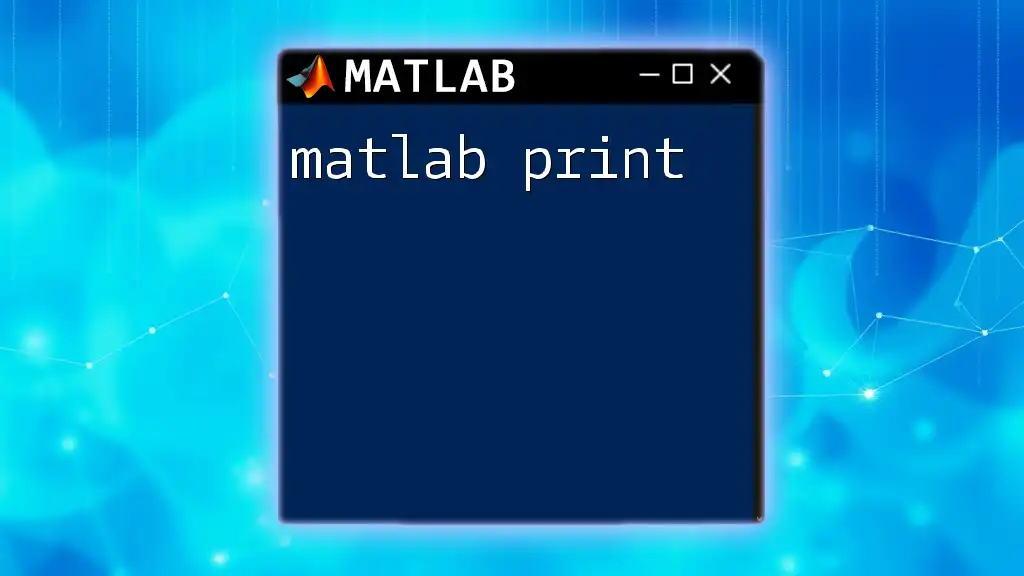
Practical Applications of griddata
Use cases in scientific computing
`griddata` is frequently utilized in fields like meteorology, environmental science, and engineering. For instance, interpolating geographic temperature readings to create a heat map can help in analyzing temperature distributions over an area.
Data visualization
One of the key advantages of `griddata` is its ability to seamlessly integrate with MATLAB’s visualization capabilities. By creating surface plots from the interpolated data, practitioners can gain insights into trends and patterns within their datasets. Here’s an example:
surf(XI, YI, ZI);
title('Surface Plot of Interpolated Data');
xlabel('X Axis');
ylabel('Y Axis');
zlabel('Z Axis');
This code generates a 3D surface plot, allowing for a comprehensive view of how the interpolated values correlate with the input coordinates.
Real-world examples
Consider a situation where air quality data is collected from various sensors around a city. Using `griddata`, analysts can create a smoothed surface map that highlights regions of high or low pollution, facilitating better urban planning decisions.
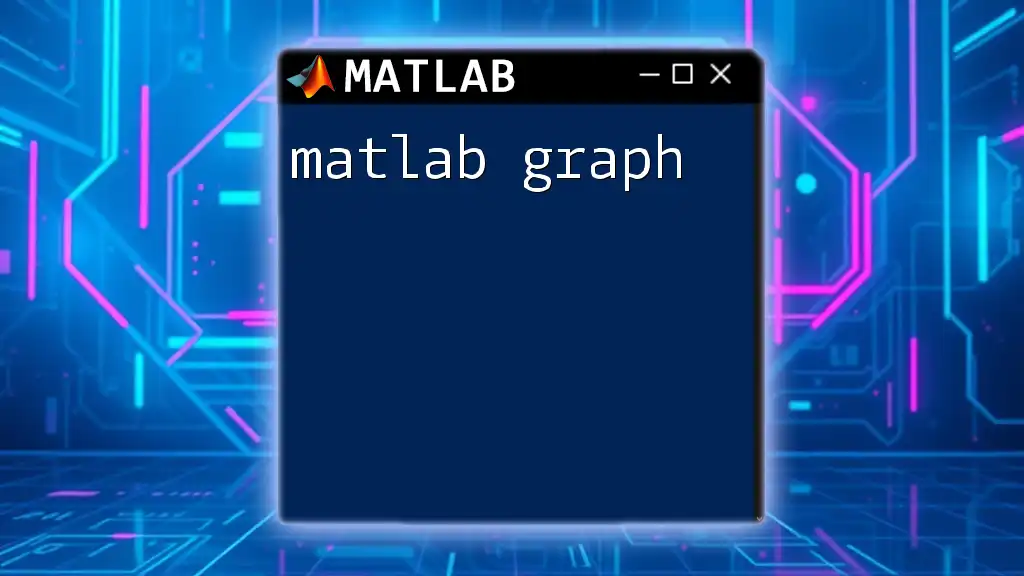
Best Practices
Choosing the right interpolation method
The choice of interpolation technique should be guided by the nature of the data. Linear interpolation is suitable for uniformly scattered data, while cubic interpolation works better for smoother and more continuous data series.
Performance Optimization
When handling large datasets, performance can become an issue. Optimizing the data by using smaller subsets or employing faster interpolation techniques can help speed up the process.
Common pitfalls and how to avoid them
Users should be cautious when interpreting the output of `griddata`. Misunderstandings can arise from irregular data that can lead to misleading results. Always visualize your griddata results to ensure they make sense in the context of your analysis.
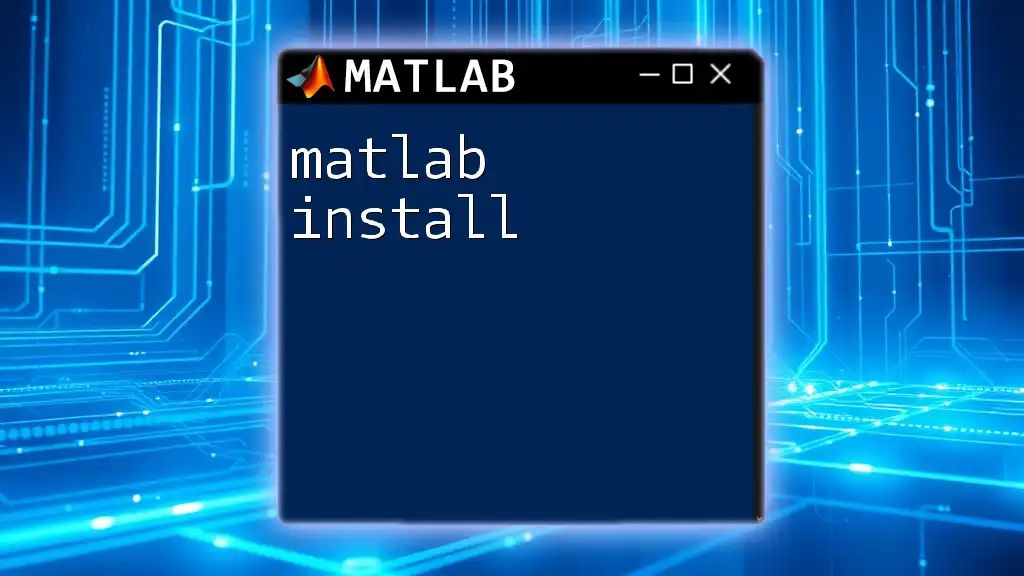
Advanced Techniques
Handling large datasets
To manage extensive datasets efficiently, consider down-sampling or using sparse matrices. These strategies reduce memory load and increase processing speed.
Integration with other MATLAB functions
You can combine `griddata` with functions like `surf`, `contour`, and `mesh` to enhance your visual analysis and better convey your findings.
Custom interpolation methods
For specialized needs, MATLAB allows users to implement custom interpolation methods. This requires a deeper understanding of numerical analysis but can be invaluable for unique datasets.
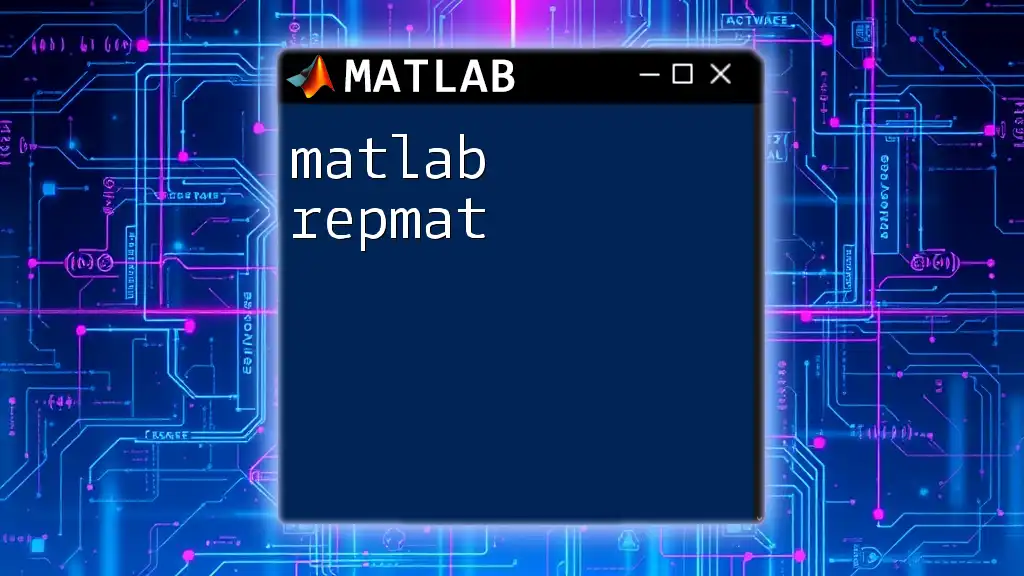
Conclusion
Mastering the `griddata` function in MATLAB is essential for anyone working with spatial data and interpolation. By understanding the syntax, input parameters, and suitable interpolation techniques, users can significantly improve their data analysis tasks. Experimenting with different methods and visualizing results will deepen your grasp and mastery of this critical function in MATLAB.
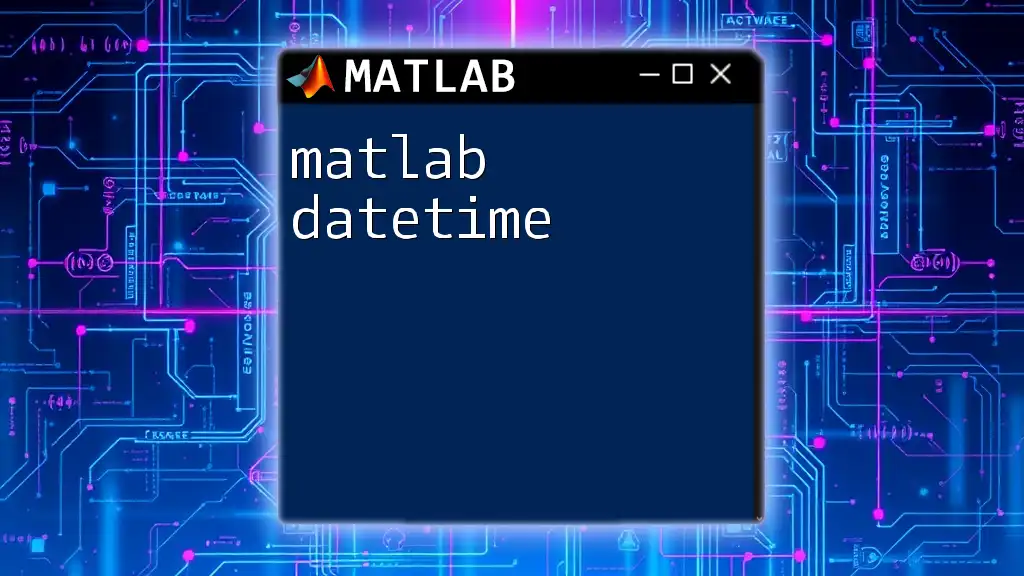
Further Resources
For those looking to deepen their understanding further, consider exploring MATLAB's official documentation on `griddata`, numerous online forums, and communities devoted to MATLAB programming. Engaging with these resources can provide additional support and broaden your MATLAB skills. Practicing on sample datasets will solidify your learning and help you apply these concepts effectively in real-world situations.