In MATLAB, you can specify line colors in plots using either color abbreviations (e.g., 'r' for red) or RGB triplet values to customize the appearance of your data visualization.
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'r', 'LineWidth', 2); % 'r' sets the line color to red
Understanding Line Colors in MATLAB
What Are Line Colors?
MATLAB line colors are essential for distinguishing among multiple datasets and enhancing the overall readability of graphical representations. By effectively utilizing line colors, you provide clarity in your visual analysis. In MATLAB, colors can be represented using the RGB color model, which defines colors through the combination of red, green, and blue light. Each color component ranges from 0 to 1, allowing for a broad spectrum of colors based on varying intensities.
Default Line Colors in MATLAB
By default, MATLAB employs a specific color order for plotting lines. This helps to create a consistent look across plots. For example, if you use the following code:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, x, y2);
You will notice a default color cycling for each line. The first line will appear in blue, the second in orange, and so forth, thus establishing a visual hierarchy through color.
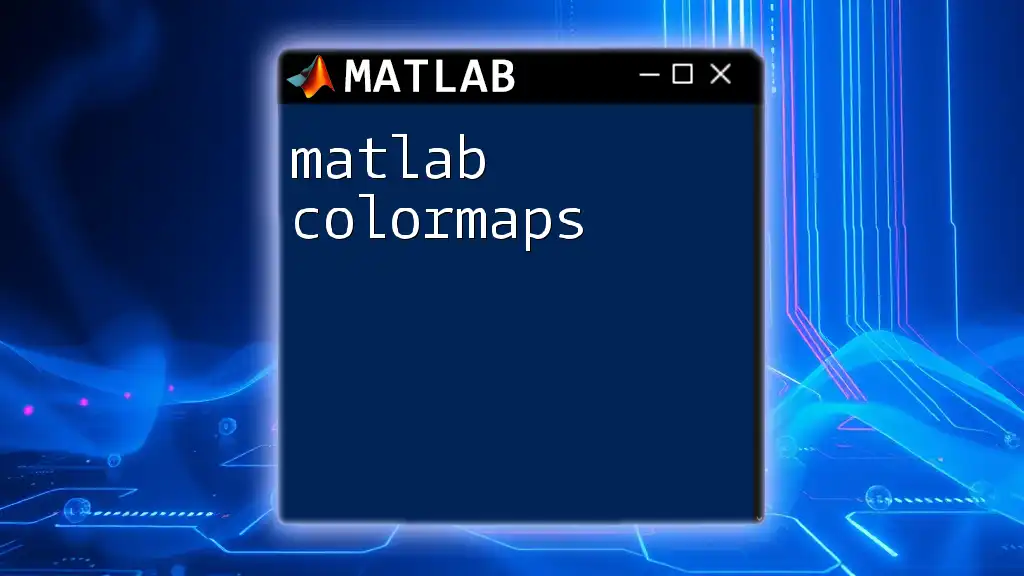
Specifying Line Colors
Using Basic Color Names
MATLAB allows you to specify colors using simple, one-letter identifiers. These include:
- 'r' for red
- 'g' for green
- 'b' for blue
- 'k' for black
- 'm' for magenta
- 'c' for cyan
- 'y' for yellow
You can easily apply these color names to improve the visual presentation of your plots. For instance, consider the following code:
plot(x, y1, 'r', x, y2, 'g');
In this example, the sine curve will be plotted in red, while the cosine curve will be plotted in green, making it instantly clear which line represents which function.
Custom Colors with RGB Values
Beyond basic colors, MATLAB allows for the definition of custom colors using RGB triplets. Each RGB value should range between 0 and 1. For example, an RGB triplet of [1, 0, 0] corresponds to bright red. You can apply RGB values like so:
plot(x, y1, 'Color', [0.1, 0.2, 0.5]); % Custom dark blue color
This flexibility permits a unique customization of the color palette for your plots.
Using Hexadecimal Color Codes
MATLAB also supports hexadecimal color codes, which can be particularly useful for web-based color schemes. For example, the HEX code for a vibrant orange is `#FF5733`. You can utilize this color in your plots with the following code:
plot(x, y1, 'Color', '#FF5733'); % Custom orange color
Using hex codes allows for a more extensive variety of colors, enabling you to match specific design requirements.
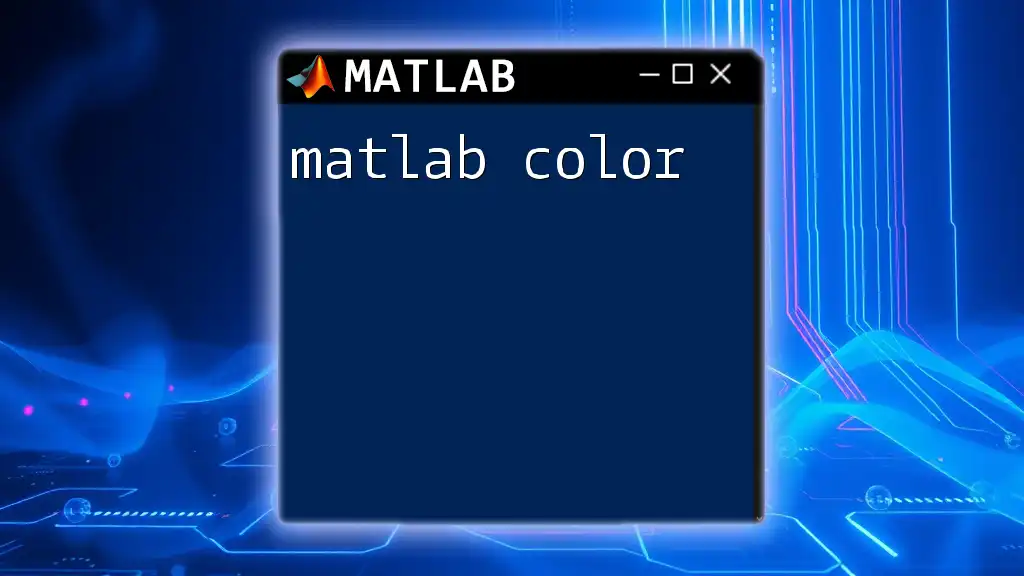
Advanced Color Techniques
Using Color Maps
In MATLAB, colormaps are used to define a range of colors used in a plot, particularly in applications such as scatter plots, heatmaps, or surface plots. You can apply a standard colormap like 'jet' to enhance the visual richness of your plot:
colormap(jet);
scatter(x, y1, 100, y1, 'filled');
This creates a scatter plot where the colors adapt according to the y-values in a gradient fashion, yielding more insightful visual distinctions.
Cycle Colors for Multiple Lines
When plotting multiple datasets, it’s essential to maintain color clarity. MATLAB facilitates this through color cycling. Here’s how you could implement it:
hold on;
for i = 1:5
plot(x, sin(x + i), 'Color', [i/5, 0.5, 1 - i/5]);
end
hold off;
By employing such an approach, you can create a series of lines that smoothly transition in color, enhancing the visual appeal and clarity.
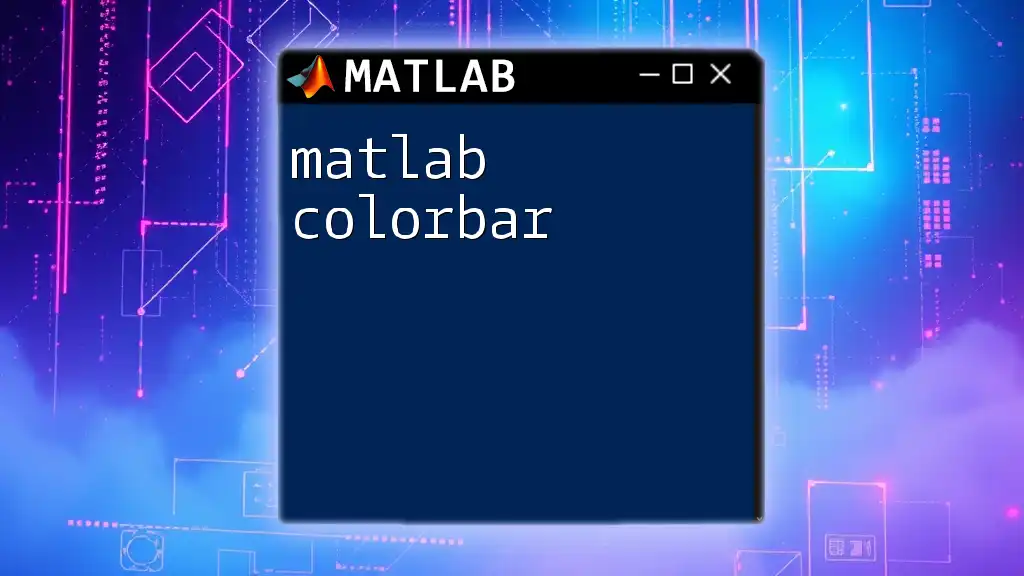
MATLAB Plotting Functions and Line Colors
Common MATLAB Plotting Functions
Different plotting functions in MATLAB inherently support color specifications. For instance, with `scatter`, you can specify the color and size of the markers, as shown below:
scatter(x, y1, 50, 'r', 'filled'); % Scatter plot with red filled circles
bar(x, y1, 'FaceColor', 'b'); % Bar plot with blue bars
These functions exemplify how to tune your plots further to make them visually distinct and engaging.
Customizing Line Properties
In addition to colors, you can further customize line properties, such as line style and thickness. Here's an example:
plot(x, y1, 'Color', 'g', 'LineWidth', 2, 'LineStyle', '--');
In this case, the sine wave appears in a green dashed line with increased thickness, thereby enhancing its visibility in crowded plots.
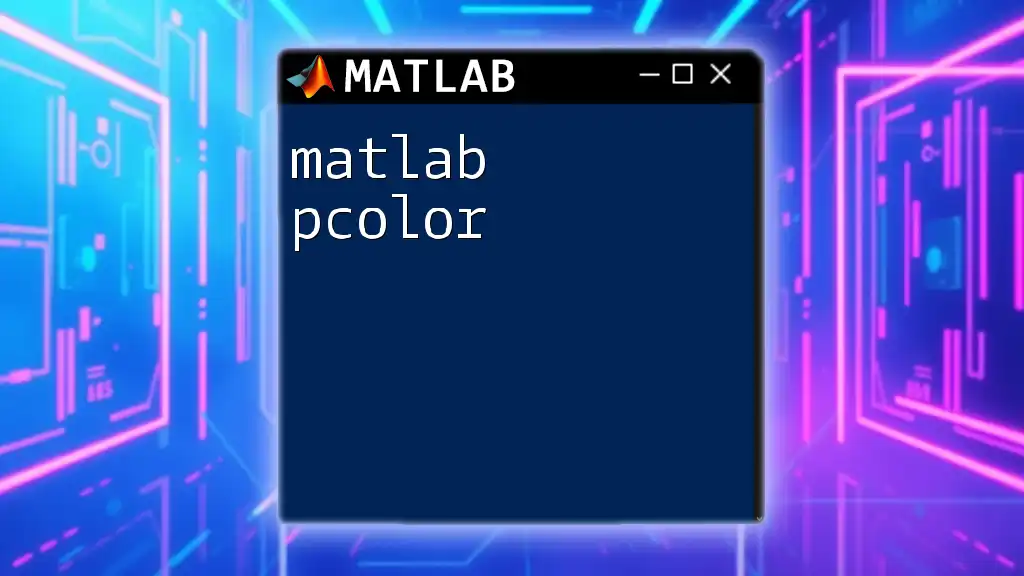
Best Practices for Choosing Line Colors
Consider Color Blindness
When selecting colors for your plots, it’s crucial to be mindful of color visibility, particularly for those with color blindness. Consider using color combinations that are proven to be friendly for color-blind individuals. For instance, using shades of blue and orange often works well.
Creating Effective Visualizations
To establish clarity in your data visualization:
- Limit the number of colors to avoid overwhelming the viewer.
- Utilize contrasting colors for lines that are near each other.
- Choose colors that convey meaning (e.g., red for negative trends, green for positive trends).
Effective use of color not only makes your data more understandable but also maintains viewer engagement throughout your analysis.
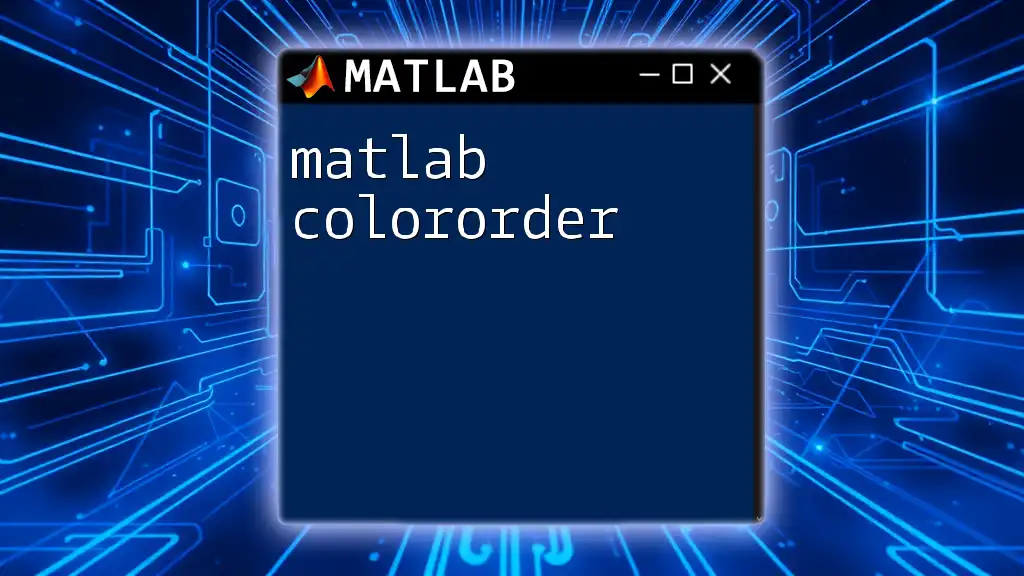
Conclusion
Understanding and effectively using MATLAB line colors is paramount for creating impactful visualizations. By exploring basic color identifiers, RGB values, and hexadecimal codes, you can craft visuals that convey clarity and insight in your data presentations.
Experimenting with colormaps and adhering to best practices can greatly enhance your plotting skills, making your work more accessible and visually appealing. Don’t hesitate to explore colors that suit your data's narrative, and always strive to improve your visual communication!
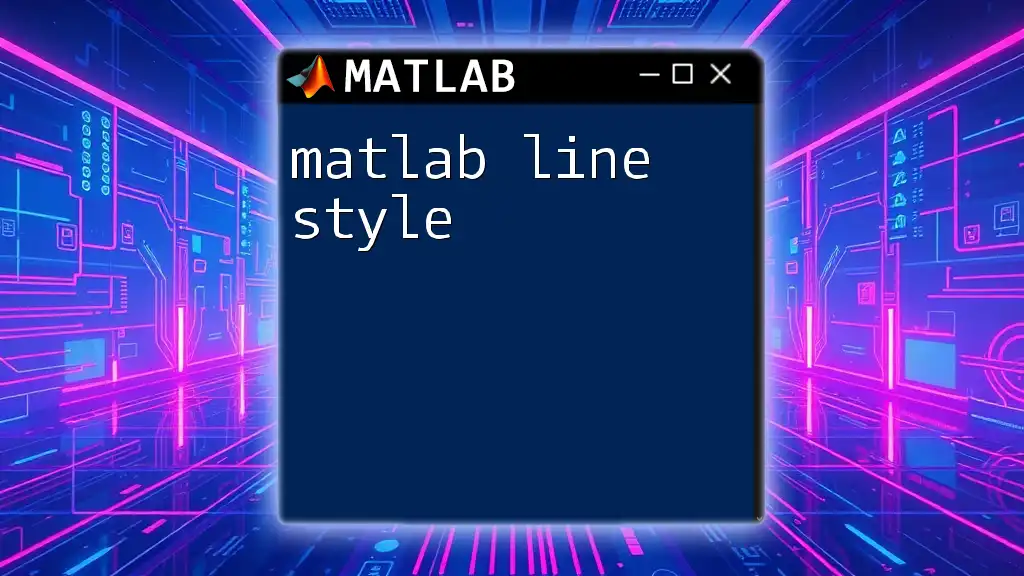
Additional Resources
For further exploration of MATLAB line colors and specifications, refer to the official [MATLAB documentation on color specification](https://www.mathworks.com/help/matlab/ref/colorspec.html). Additionally, consider reading about color theory to understand more about how colors influence perception in visual contexts.
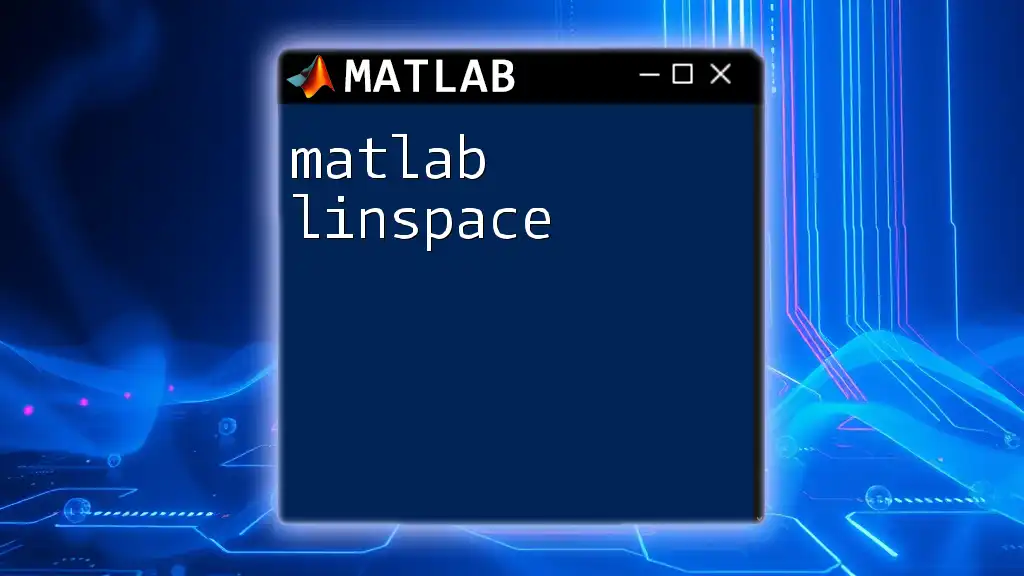
Call to Action
If you found this guide helpful, subscribe for more insights and tutorials on MATLAB commands and techniques. You will also gain access to a free downloadable guide on MATLAB line colors that can serve as a handy reference in your projects!