In MATLAB, line styles are used to customize the appearance of plotted lines in graphs, allowing users to differentiate between multiple datasets through various patterns, such as solid, dashed, or dotted lines.
Here's a code snippet demonstrating how to set different line styles in a plot:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, '-r', 'LineWidth', 2); % Solid red line
hold on;
plot(x, y2, '--b', 'LineWidth', 2); % Dashed blue line
xlabel('X-axis');
ylabel('Y-axis');
title('MATLAB Line Styles');
legend('sin(x)', 'cos(x)');
hold off;
Understanding Line Styles in MATLAB
What are Line Styles?
In MATLAB, line styles determine how lines appear in graphical plots, significantly influencing the visual representation of data. By customizing line styles, you can easily distinguish between multiple data series, thereby enhancing clarity and making your plots more informative.
Default Line Styles in MATLAB
MATLAB provides several default line styles that can be effortlessly used within plotting commands. The primary options include:
- Solid lines (default)
- Dashed lines
- Dotted lines
- Dash-dot lines
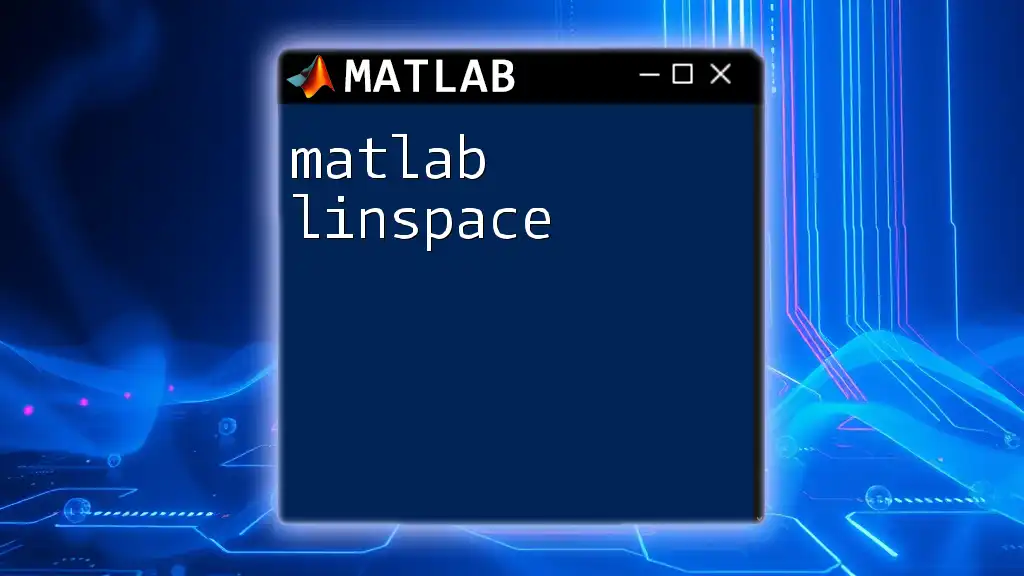
Basic Syntax for Setting Line Styles
Creating Simple Plots
To get started, you can create a simple plot without specifying any line styles. Here's a basic example:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
In this code, the `plot()` function generates a line graph of the sine function without any specific line style applied.
Customizing Line Styles
You can easily customize the appearance of your line plot by specifying a line style parameter in the `plot()` function. For example, if you want to create a dashed line, you would use:
plot(x, y, '--'); % Dashed line
Here, the `--` parameter instructs MATLAB to use a dashed line style.
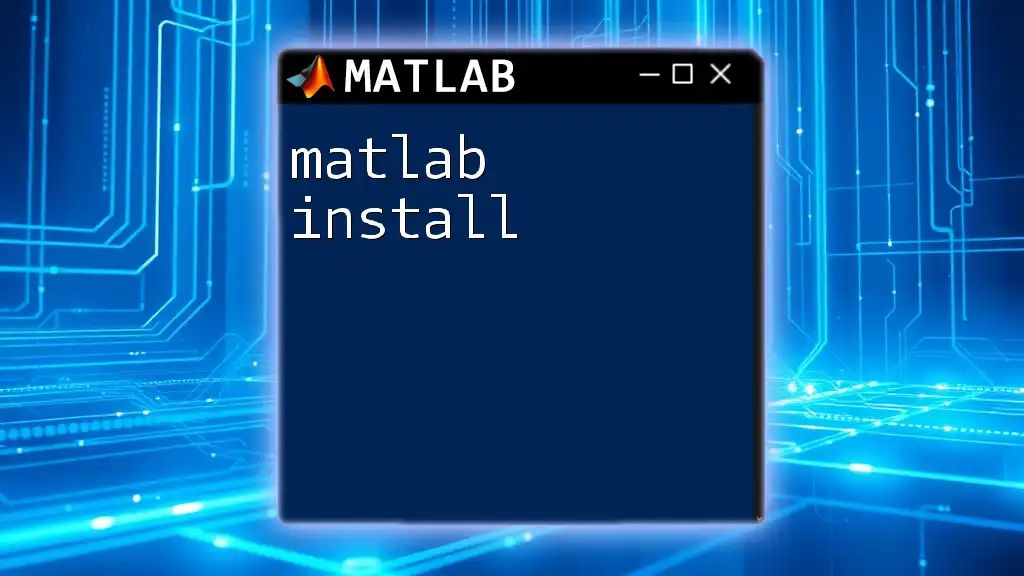
Detailed Overview of Line Style Options
Solid Line
Solid lines are the most commonly used line style in MATLAB. They provide a clear and strong visual representation of data. Utilizing a solid line can be particularly effective when showcasing primary trends in your data.
Example code to plot using a solid line:
plot(x, y, 'k-', 'LineWidth', 2); % Black solid line with width 2
This code displays a black, solid line that's twice as thick as the default, emphasizing it on your graph.
Dashed Line
Dashed lines are excellent for indicating secondary trends or for projections that require distinction from the primary data series. This helps viewers segregate primary information from auxiliary data easily.
Example code for a dashed line:
plot(x, y, 'r--'); % Red dashed line
In this example, a red dashed line represents the sine function, effectively standing out in the plot.
Dotted Line
Dotted lines serve the purpose of indicating data points that should not be connected, often helpful when presenting discrete data. They provide a visual cue that differentiates certain data points from others.
Example code for a dotted line:
plot(x, y, 'b.'); % Blue dotted line
This code snippet utilizes blue dots, providing a clear representation of data points without implying continuity.
Dash-dot Line
Dash-dot lines combine visual elements of both dash and solid lines. They are often used in scenarios where an intermediate distinction is needed between various data series.
Example code for a dash-dot line:
plot(x, y, 'g-.'); % Green dash-dot line
This approach allows you to communicate multiple layers of information effectively.
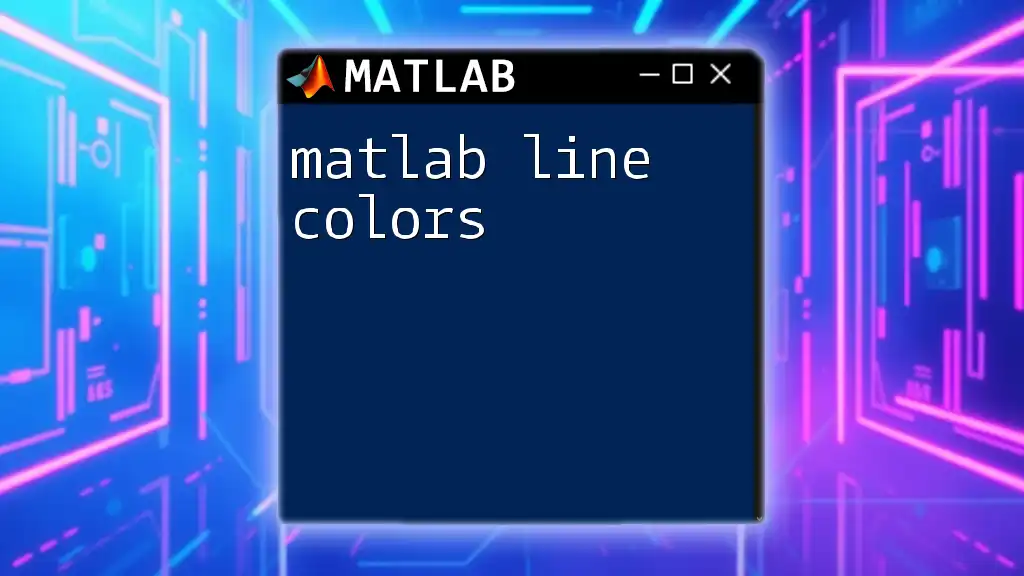
Combining Multiple Line Styles in One Plot
Overlaying Different Lines
MATLAB allows you to overlay multiple lines on a single graph, which enhances the comparability of different data sets. When skillfully employed, this can greatly improve the interpretability of your graph.
Example code demonstrating multiple line styles:
hold on; % Save current plot to add overlay
plot(x, sin(x), 'k-'); % Solid line for sine
plot(x, cos(x), 'r--'); % Dashed line for cosine
hold off; % Release the plot
By using `hold on`, you ensure that both the sine and cosine functions are plotted on the same graph, making it easy to compare their behaviors visually.
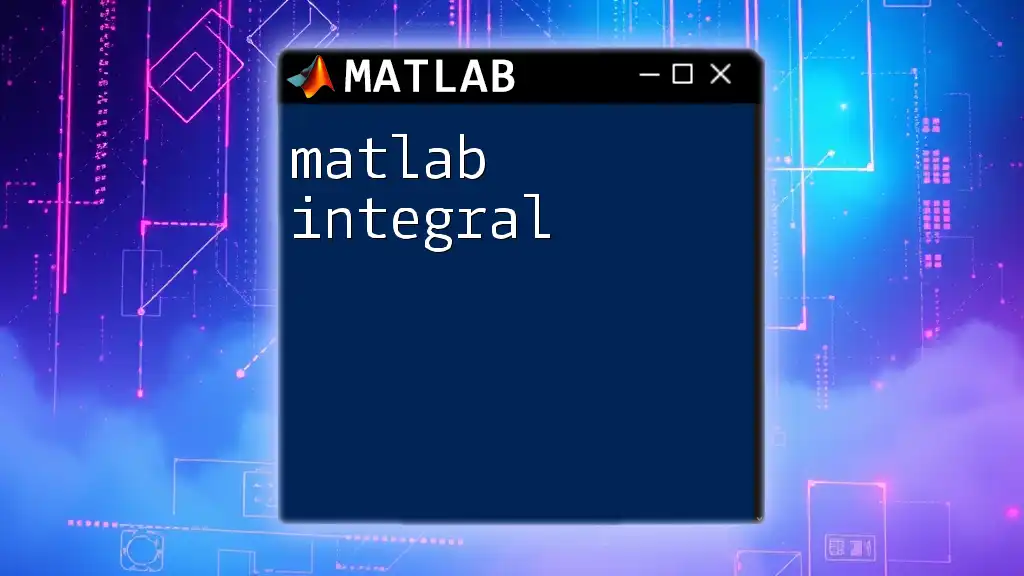
Customizing Line Properties
Changing Line Width
Line width is a crucial aspect of visual representation. Thicker lines can be more eye-catching and assertive, while thinner lines offer a more subtle appearance. Customizing line width can enhance the clarity of your plots.
Example code to set line width:
plot(x, y, 'b-', 'LineWidth', 3); % Thicker blue line
In this snippet, the blue line is made noticeably thicker, helping it stand out in the overall graph.
Changing Line Color
MATLAB allows you to customize line colors using both built-in names and RGB values. Choosing the right color enhances the visual aspect of the data presentation and helps convey meaning.
Example code using RGB values:
plot(x, y, 'Color', [1, 0.5, 0]); % RGB Custom Color
This code sets a custom orange color for the line, giving your plot a personalized touch.
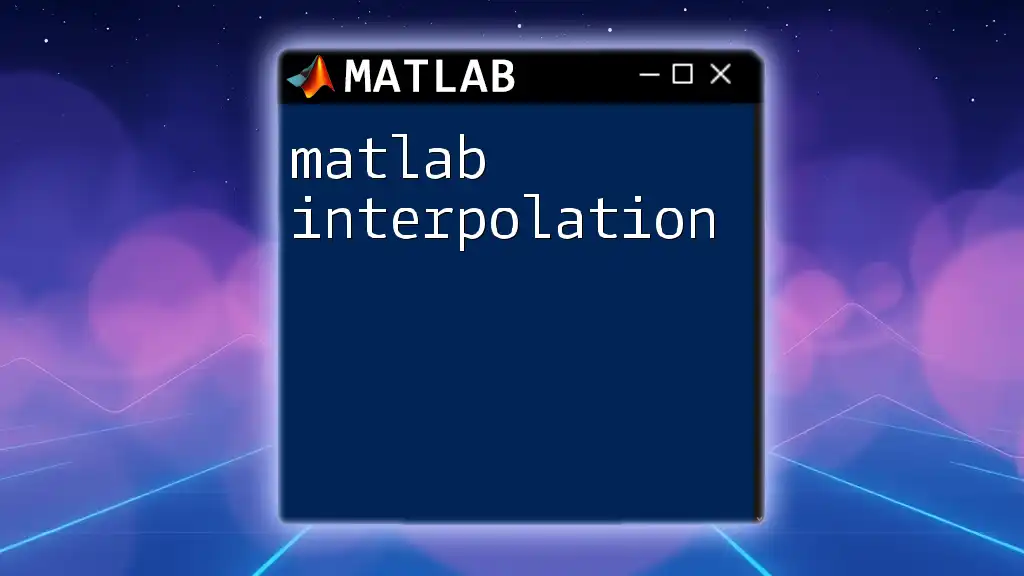
Adding Markers to Lines
Importance of Markers
Markers can be beneficial for indicating specific data points directly on the graph, adding another layer of information and clarity. They are especially useful when working with discrete data or want to emphasize particular values.
Overview of Marker Options
Choosing a variety of marker styles (such as circles, squares, and triangles) can impact how data is interpreted in your visualizations.
Example code demonstrating the use of markers:
plot(x, y, 'o--'); % Dashed line with circular markers
In this case, the circular markers on a dashed line help identify each data point along the sine curve clearly.
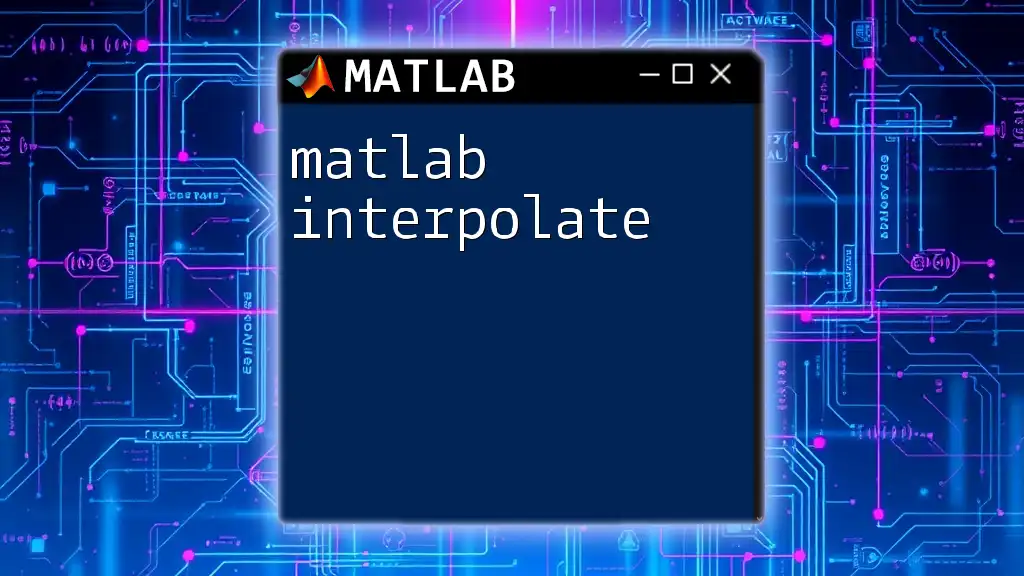
Practical Applications of Line Styles
Use Cases in Different Fields
Different fields utilize specific line styles based on their visualization needs:
- Engineering: Solid lines often represent primary systems, whereas dashed lines might denote secondary options.
- Finance: Dashed and dotted lines frequently signify forecasts and projections, allowing for visual distinctions between historical and future data.
- Data Science: In exploratory data analysis, innovative use of line styles can enhance the interpretability of complex data.
Visualization Best Practices
It’s essential to choose line styles that not only align with the data’s nature but also enhance clarity. When in doubt, stick with solid lines for primary data and consider dashed or dotted lines for secondary insights.
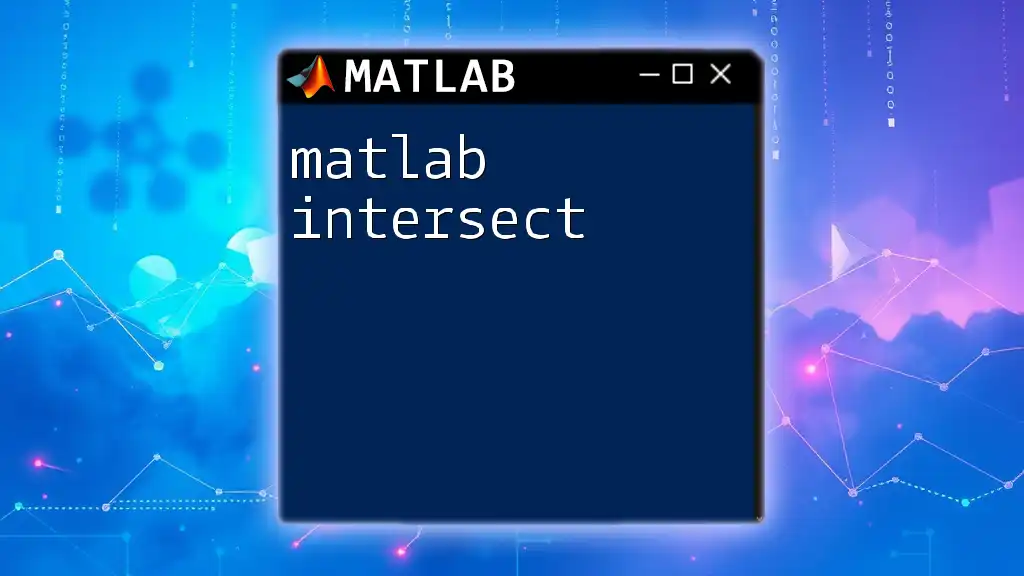
Conclusion
In conclusion, understanding and using various MATLAB line styles is vital for creating clear, informative, and aesthetically pleasing graphical representations of data. Whether you are plotting simple data or combining multiple lines for complex analysis, knowing how to customize line styles ensures that your visuals communicate effectively.
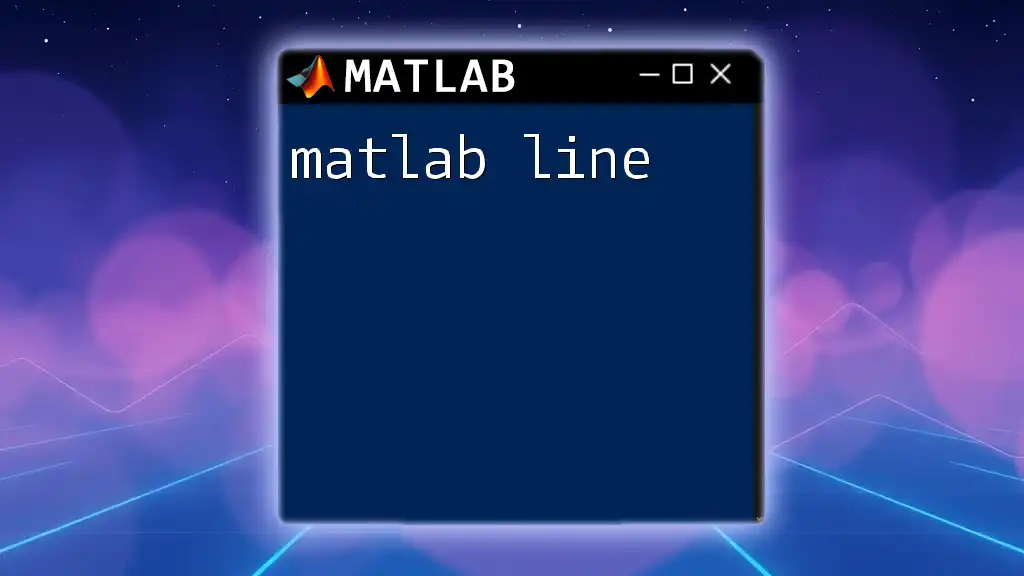
Additional Resources
Recommended Reading and Tutorials
To further develop your skills with MATLAB line styles, consider checking out online tutorials, instructional videos, or relevant books on data visualization practices in MATLAB.
MATLAB Documentation Links
Utilize the official MATLAB documentation for in-depth explanations and updates on line styles and other plotting options.
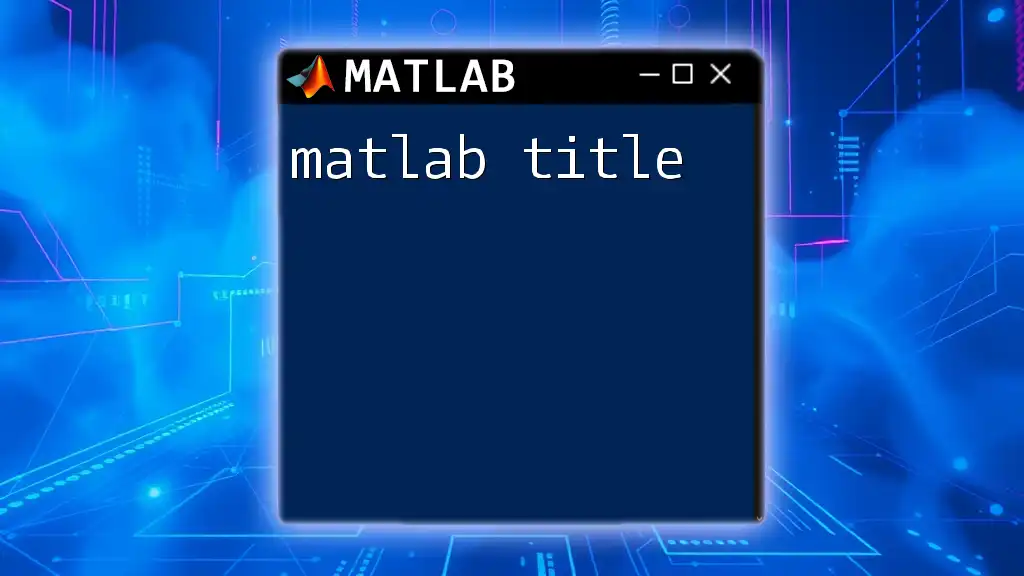
Call to Action
Start incorporating various MATLAB line styles into your plots today! Experiment with different configurations and discover how effective visual representations can enhance your data analysis. Join our community for more tutorials and tips on MATLAB commands.