The `pcolor` function in MATLAB creates a pseudocolor plot, which displays matrix data as a grid of colored rectangles, with color representing the value of Z at each point defined by X and Y.
% Example of using pcolor in MATLAB
[X, Y] = meshgrid(-3:0.1:3, -3:0.1:3);
Z = sin(sqrt(X.^2 + Y.^2));
pcolor(X, Y, Z);
colorbar; % Adds a color scale to interpret values
Understanding `pcolor`
What is `pcolor`?
The `pcolor` function in MATLAB is a powerful tool for creating color-coded 2D representations of matrices. It essentially displays the values of a matrix as colored rectangular patches, making it easy to visualize data and identify patterns at a glance. While it offers basic plotting capabilities, its strength lies in quick visualizations that are visually intuitive.
When comparing `pcolor` with other plotting functions like `imagesc` or `surf`, it’s essential to recognize that `pcolor` displays the patch sizes based on the input grid, making it particularly effective for surface plots where you want to show discrete values across a continuous space.
When to Use `pcolor`
`pcolor` is particularly beneficial in scenarios where:
- You are visualizing spatial data, such as temperature maps or geographical data.
- You want to quickly represent large datasets where detail can be shown through color gradients rather than individual data points.
- You need a visual representation of matrix values without the overhead of 3D rendering that comes with functions like `surf`.
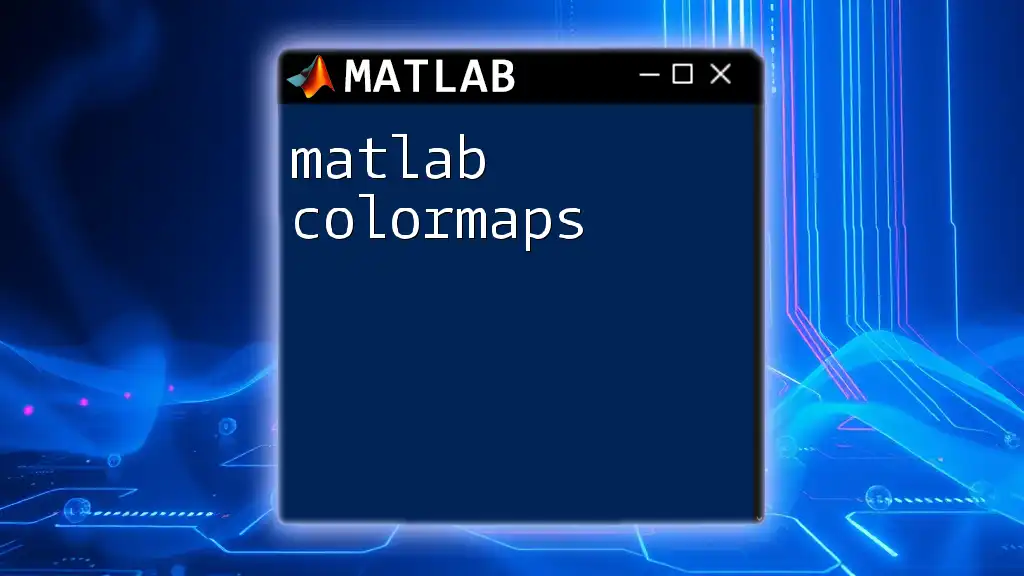
Getting Started with `pcolor`
Basic Syntax of `pcolor`
The basic structure of the `pcolor` command is straightforward. To call it, you typically use:
pcolor(X, Y, Z);
In this syntax:
- `X` corresponds to the grid for the x-axis.
- `Y` corresponds to the grid for the y-axis.
- `Z` is the matrix that contains the values to be plotted, where color representation will correspond to these values.
Required Inputs
To use `pcolor`, you need to understand its required inputs:
- `X` and `Y` can be matrices or vectors that define the grid points.
- `Z` should be a matrix that is one row and one column smaller than `X` and `Y`. Each element of `Z` represents the color at the respective grid defined by `(X, Y)`.
For instance, if you have a meshgrid generated from `X` and `Y`, ensure that `Z` is structured accordingly:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2)); % A sinusoidal function
pcolor(X, Y, Z);
colorbar; % Adds a color scale to the plot
Optional Arguments
`pcolor` also supports various optional parameters that can modify the visual output. You can customize color, shading, and edge properties. Here’s an example that removes the edges from the patches:
pcolor(X, Y, Z, 'EdgeColor', 'none');
This enhancement helps to create a more seamless and softer visual representation by blending colors together, removing the grid effects.
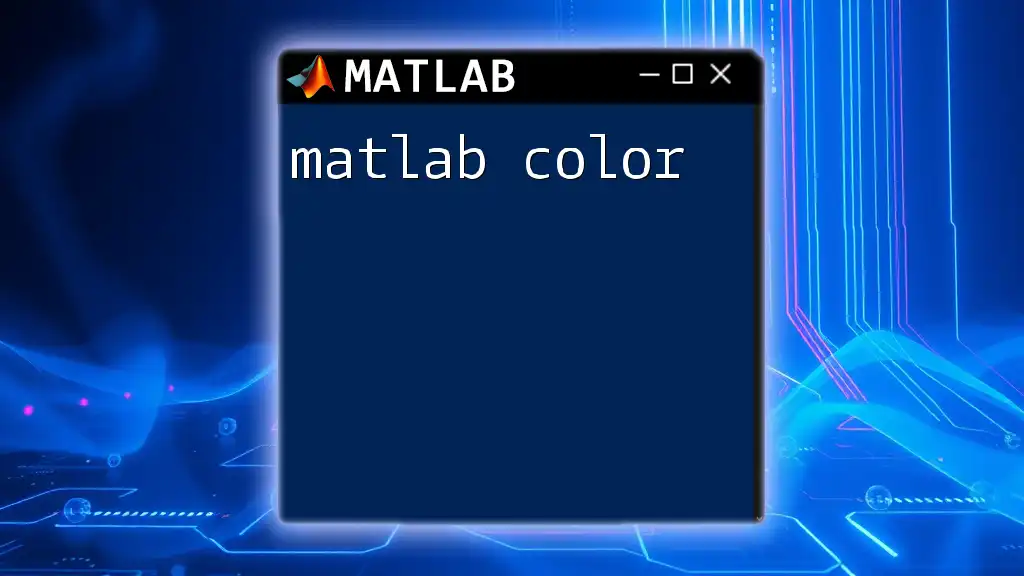
Practical Applications of `pcolor`
Example 1: Basic 2D Color Map
To illustrate how to create a basic 2D color map, start with generative data:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
pcolor(X, Y, Z);
colorbar; % Displays the scale for values
This code generates a sine wave function and displays it where color variations signify differences in value.
Example 2: Customizing the `pcolor` Plot
Changing Color Maps
You can modify the color scheme using the `colormap` function. MATLAB provides various built-in colormaps like `jet`, `hot`, and `parula`. For example, if you want to visualize the same data with a different color scheme:
colormap(jet); % Changes the color scheme
Adding Titles and Labels
For clarity and better understanding, it’s vital to label your plots. This can include titles or labels for axes, which can be easily added using:
title('Sine Wave Function using pcolor');
xlabel('X-axis');
ylabel('Y-axis');
Example 3: Using Grids and Display Options
Customizing the grid display can enhance the overall clarity. The `shading` command is particularly useful:
pcolor(X, Y, Z);
shading interp; % Smoothes color transitions
This command smooths out the colors, creating a gradient effect that visually enhances the plot.
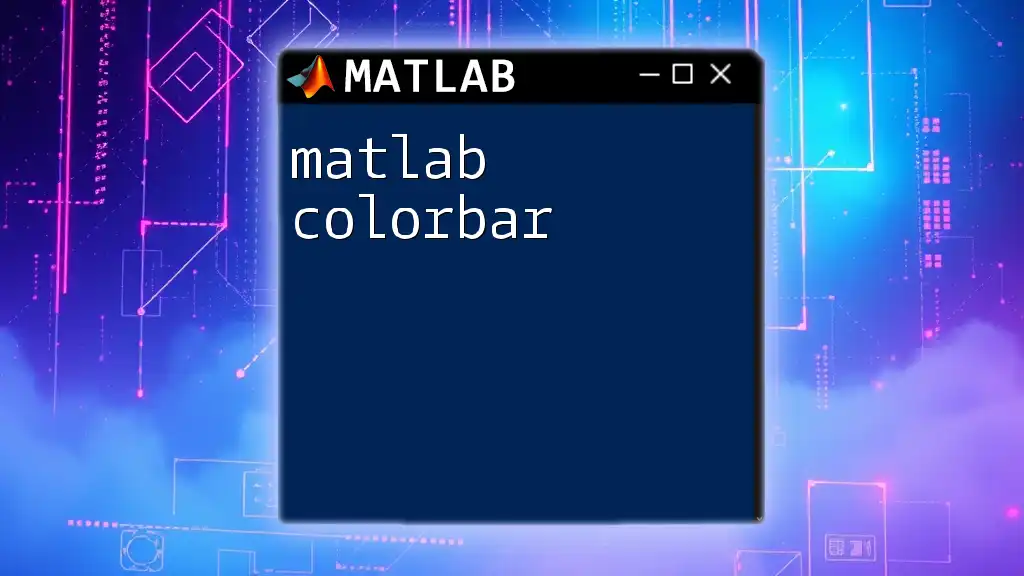
Advanced Techniques with `pcolor`
Combining `pcolor` with Other Plot Types
One of the strengths of MATLAB is its flexibility in combining multiple plots. It’s easy to overlay a `pcolor` plot with contour lines, for enhanced data visualization:
hold on; % Retain current plot
contour(X, Y, Z, 'k'); % Adds contour lines in black
hold off;
This combination allows you to maintain the color representation from `pcolor`, while providing contour lines for specific value levels.
Handling Non-Rectangular Data
For data that isn't laid out on a rectangular grid, such as triangulated data, `pcolor` can still be used effectively with additional functions like `trisurf`:
trisurf(triangulation, X, Y, Z);
This approach allows for complex data structures while utilizing `pcolor`'s visualization strengths.
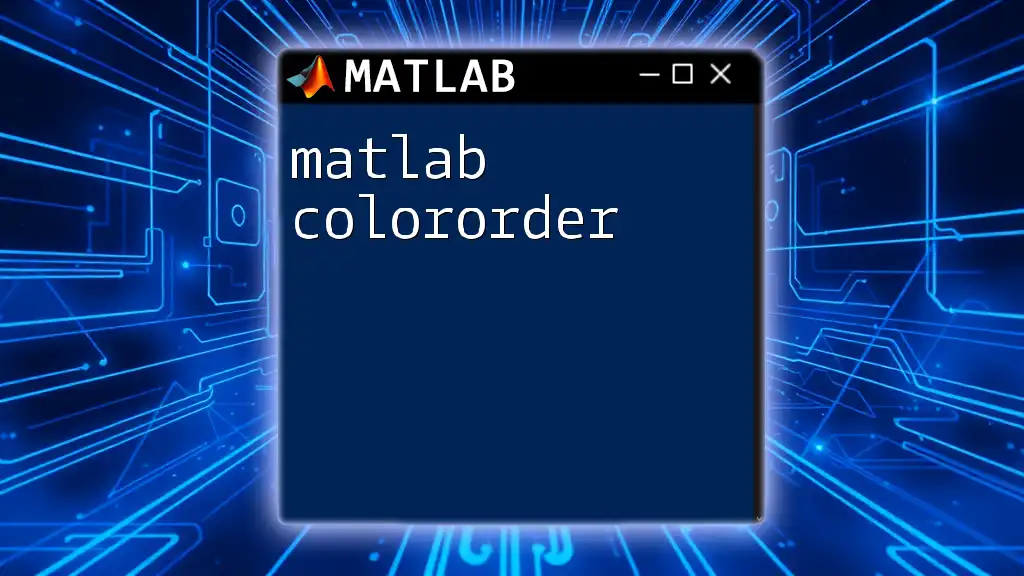
Tips and Best Practices
Common Mistakes to Avoid
When using `pcolor`, beginners might make common mistakes such as mismatching dimensions between matrices, which results in errors or misleading plots. Always ensure that your `Z` matrix size corresponds correctly to the `X` and `Y` matrices.
Performance Optimization
When working with large datasets, plot performance may lag. Techniques such as data downsampling or using sparse matrices can significantly enhance rendering performance while maintaining an adequate level of detail.
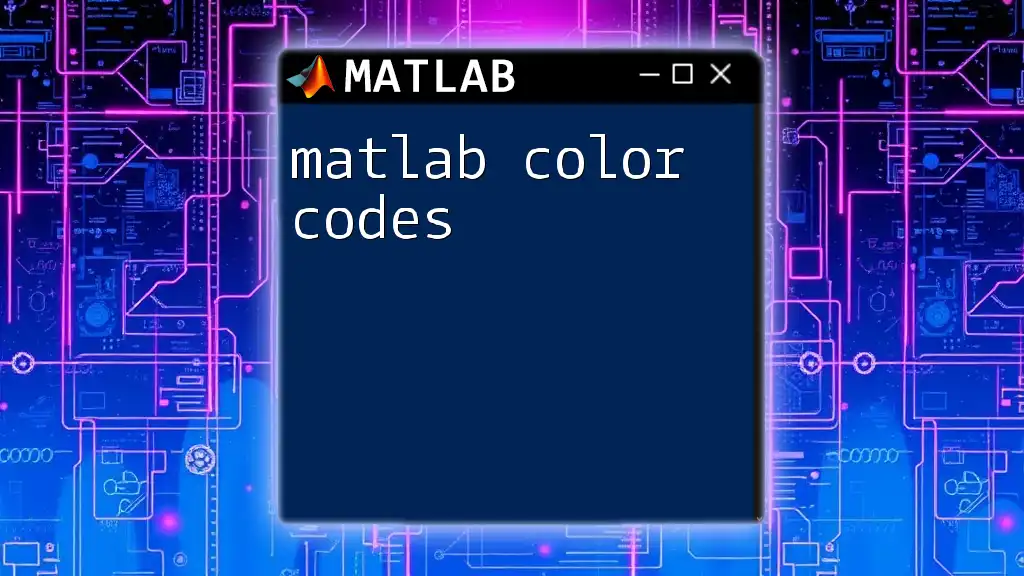
Conclusion
By understanding the powerful capabilities of the `matlab pcolor` function, you can create informative and visually appealing 2D representations of your data. With a blend of basic and advanced techniques, you'll be able to showcase data insights more effectively. Practice using `pcolor` in various applications to develop your skills further.
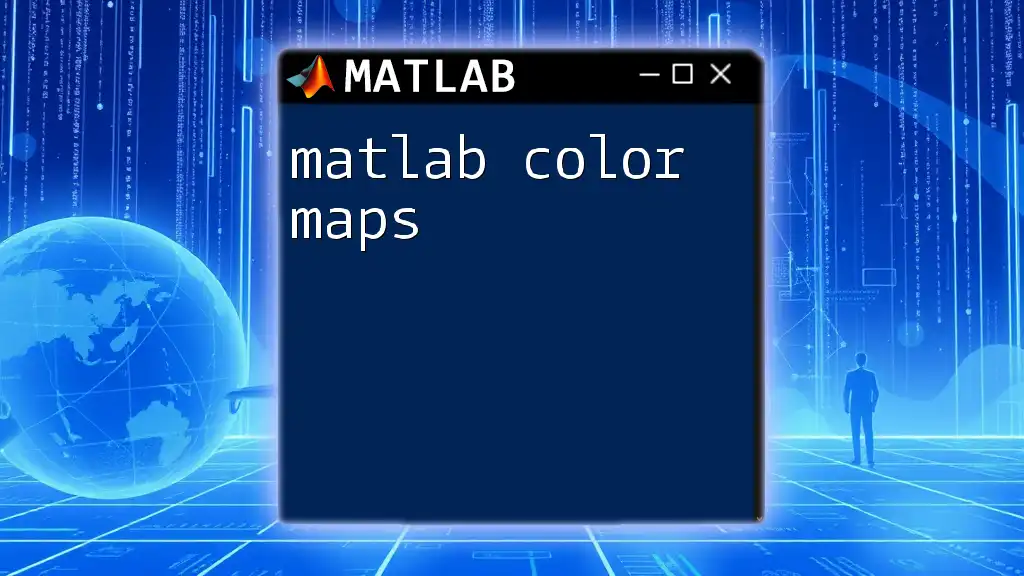
Additional Resources
For deeper insights, refer to the official MATLAB documentation for `pcolor`. Video tutorials, forums, and online classes are excellent supplemental resources for enhancing your learning experience and mastery of MATLAB's visualization tools.
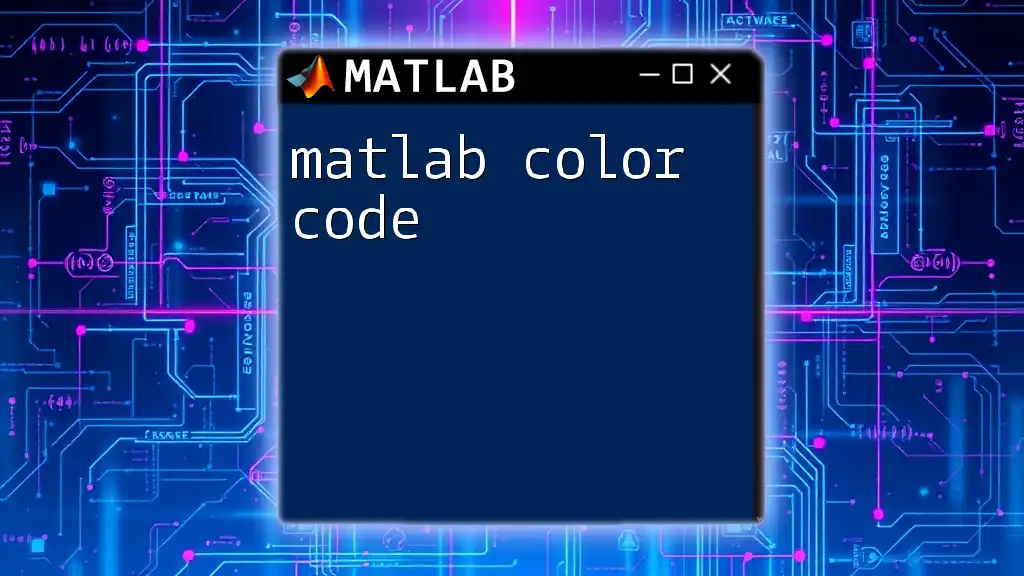
Call to Action
Share your `pcolor` experiences and the projects you have created using this function! Let us know what topics you'd like to see covered next based on your interests and needs.