In MATLAB, the marker size of points in plots can be adjusted using the 'MarkerSize' property to enhance visibility and aesthetics of the plotted data. Here's a code snippet demonstrating how to set the marker size in a scatter plot:
x = 1:10;
y = rand(1, 10);
scatter(x, y, 'MarkerSize', 10); % Set the marker size to 10
xlabel('X-axis');
ylabel('Y-axis');
title('Scatter Plot with Specified Marker Size');
Understanding Marker Properties
What are Markers in MATLAB?
In MATLAB, markers represent specific data points on a plot, providing a visual cue to distinguish different data values. These graphical elements come in various shapes, such as circles, squares, and diamonds, allowing users to choose the most effective representation for their datasets. Markers are integral in enhancing the readability of plots by making key data points stand out.
Importance of Marker Size
The size of markers plays a critical role in data visualization. Adjusting the marker size can significantly impact the plot's clarity and interpretation. Larger markers may highlight significant data points or trends, while smaller markers can be useful for displaying dense datasets without cluttering the visualization. The key is finding a balance that enhances understanding without overwhelming the viewer.
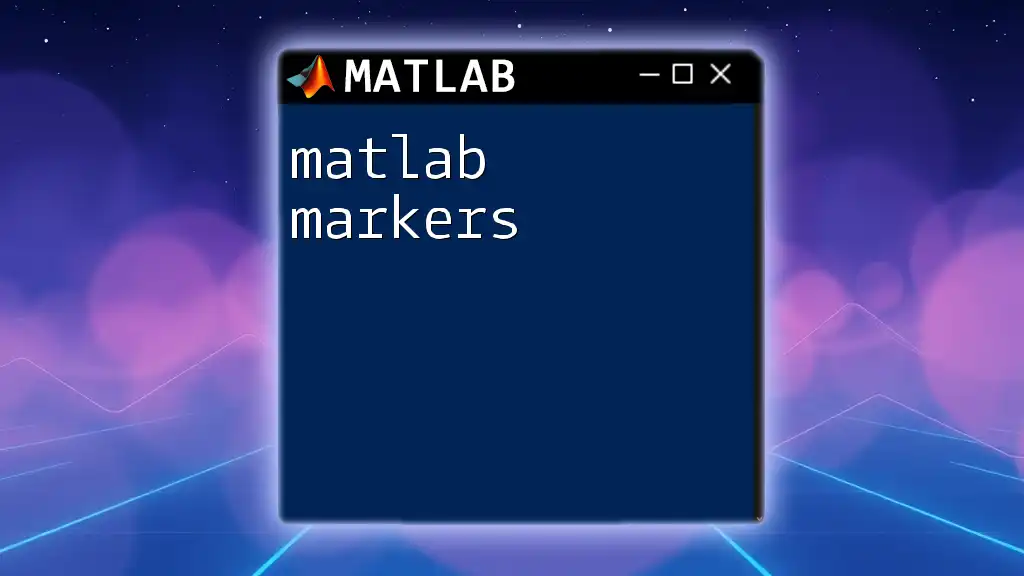
Adjusting Marker Size in MATLAB
Basic Marker Size Adjustment
To alter marker sizes in MATLAB, you can use straightforward syntax in your plotting functions. The basic command for defining marker size is both intuitive and versatile.
For instance, consider the following scatter plot example where we increase the marker size to enhance visibility:
x = rand(1, 10);
y = rand(1, 10);
scatter(x, y, 100, 'filled'); % 100 denotes the marker size
In this code, the parameter `100` sets the marker size. The `'filled'` option provides a solid color fill for the markers, making them stand out even more.
Marker Size in Different Plot Types
Line Plots
Marker size can also be adjusted in line plots, allowing for significant data points to be highlighted within the data series. Here’s how you can set markers for a line plot:
x = 0:0.1:10;
y = sin(x);
plot(x, y, 'o-', 'MarkerSize', 10); % ‘o-’ indicates circle markers with connection
In this example, the `'MarkerSize', 10` command specifies the size of the markers used to depict data points along the sine wave curve.
Scatter Plots
In scatter plots, marker size is particularly powerful for conveying additional information about the data. You can set marker size based on another dataset as shown in the following code snippet:
sizes = rand(1, 10) * 100; % Random sizes for markers
scatter(x, y, sizes, 'filled'); % Use sizes to define individual marker sizes
Each marker now corresponds to a different size based on the values extracted from the `sizes` array. This approach allows users to visually interpret data variance effectively.
Bar Plots
Markers can also make their way into bar plots. By overlaying markers on bars, you can provide further information, as illustrated in this example:
bar(x, y);
hold on;
scatter(x, y, 50, 'r', 'filled'); % Overlay red markers for emphasis
Incorporating markers helps to highlight specific values in the bar plot, enhancing overall data interpretation.
Using Marker Size in Different Contexts
Data Highlighting
Using larger markers to emphasize specific data points can be invaluable in visual data storytelling. Specific datasets can be effectively showcased by adjusting the size for emphasis:
highlightIdx = [2, 5];
scatter(x(highlightIdx), y(highlightIdx), 200, 'g', 'filled'); % Highlight specific points
In this case, indices `2` and `5` are highlighted with larger green markers, drawing attention to those specific data points.
Customizing According to Data Variance
By adjusting marker sizes based on data values, you can create a visually compelling average representation. For example:
sizes = abs(y) * 100; % Larger values in y produce larger markers
scatter(x, y, sizes, 'b', 'filled');
This snippet adjusts marker size according to the absolute values of the `y` dataset, visually representing the variation in data.
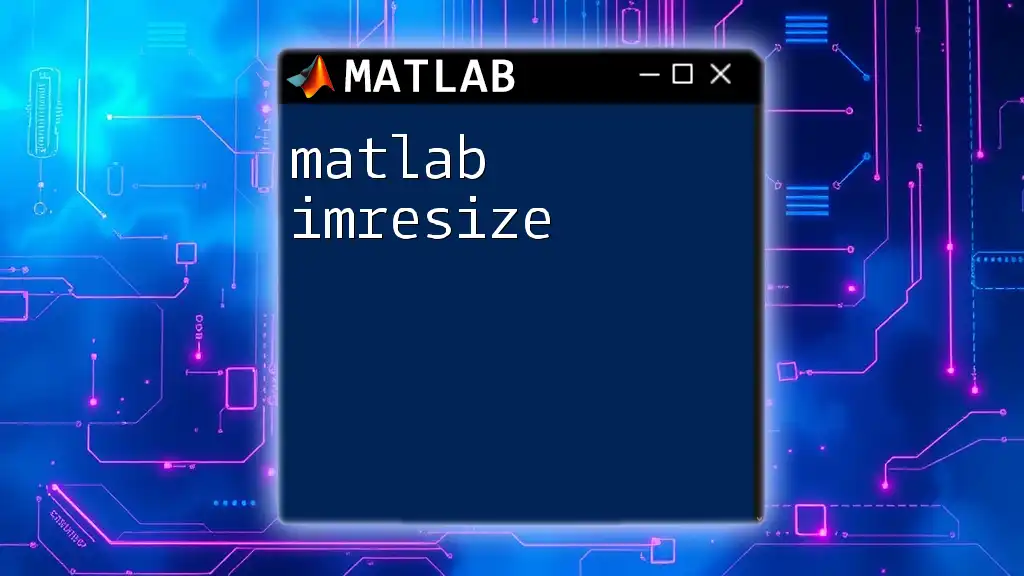
Fine-Tuning Marker Appearance
Color and Edge Properties
Beyond just size, it is also essential to consider color and edge properties for markers. Customizing these features can create a more visually appealing plot:
scatter(x, y, sizes, 'MarkerEdgeColor','k', 'MarkerFaceColor', 'flat');
In this example, `MarkerEdgeColor` sets the outline of markers while `MarkerFaceColor` allows for a gradient effect, resulting in more dynamic visuals.
Combining with Legends
Combining markers with legends enhances the plot's utility, ensuring viewers can understand what each marker represents. A good practice is to create clear legend entries that denote the sizes, like so:
legend('Data Set A', 'Data Set B', 'Location', 'Best');
Legends help in providing context to the varying marker sizes utilized within the visual dataset.
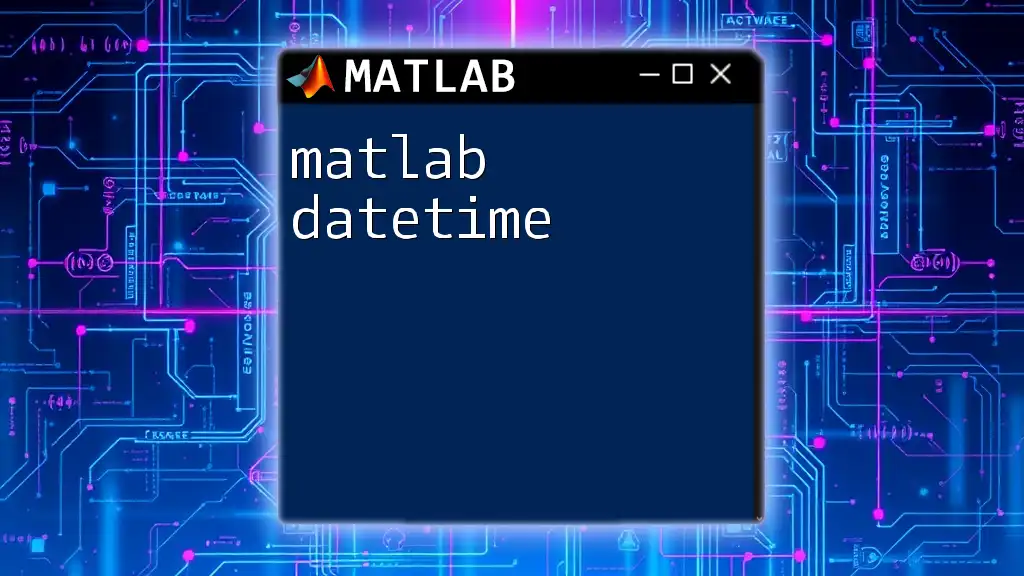
Practical Tips for Effective Data Visualization
Choosing the Right Marker Size
When selecting marker sizes, consider the viewer’s perspective. Guidelines suggest that too large markers can obscure data points, while too small markers may make them hard to identify. Thus, the best approach is often to experiment, observing how changes affect readability and understanding.
Common Mistakes to Avoid
While it's tempting to maximize visibility with larger markers, common pitfalls include overlapping markers that create visual clutter and interpretations that can mislead the audience. Here’s an example revealing an issue:
scatter(x, y, 100, 'filled');
scatter(x + 0.1, y + 0.1, 100, 'filled'); % Overlapping markers
Here, two sets of markers overlap due to insufficient spacing, leading to confusion. To avoid this, strategically spread your data or utilize transparency to mitigate clutter.
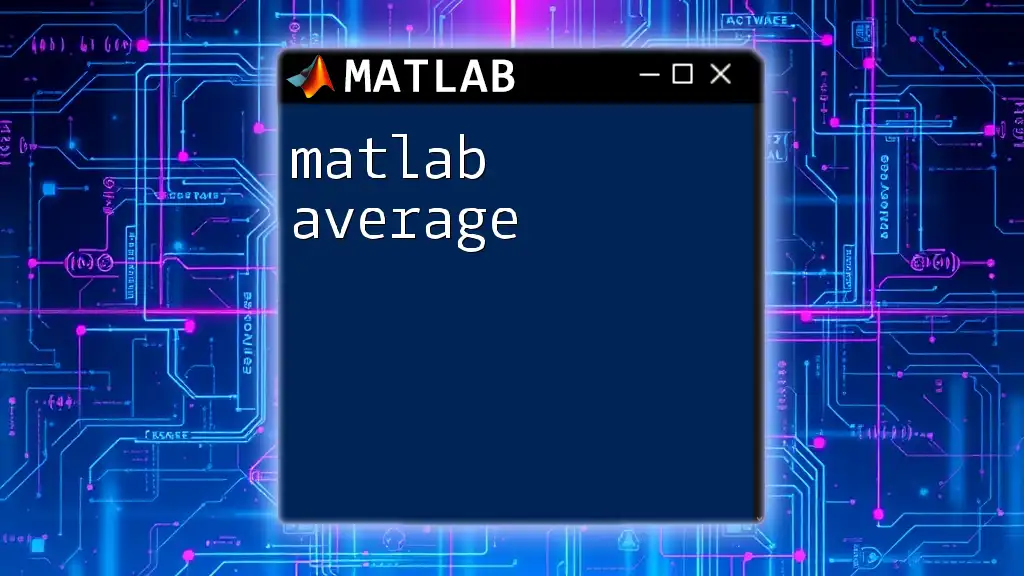
Conclusion
In summary, mastering MATLAB marker size enhances your ability to visualize data meaningfully. By adjusting marker sizes appropriately, employing color and edge properties, and avoiding common mistakes, you can significantly improve the readability and interpretability of your plots. Experimentation is key; each dataset may require different strategies, and MATLAB provides the perfect playground to find what works best for you.
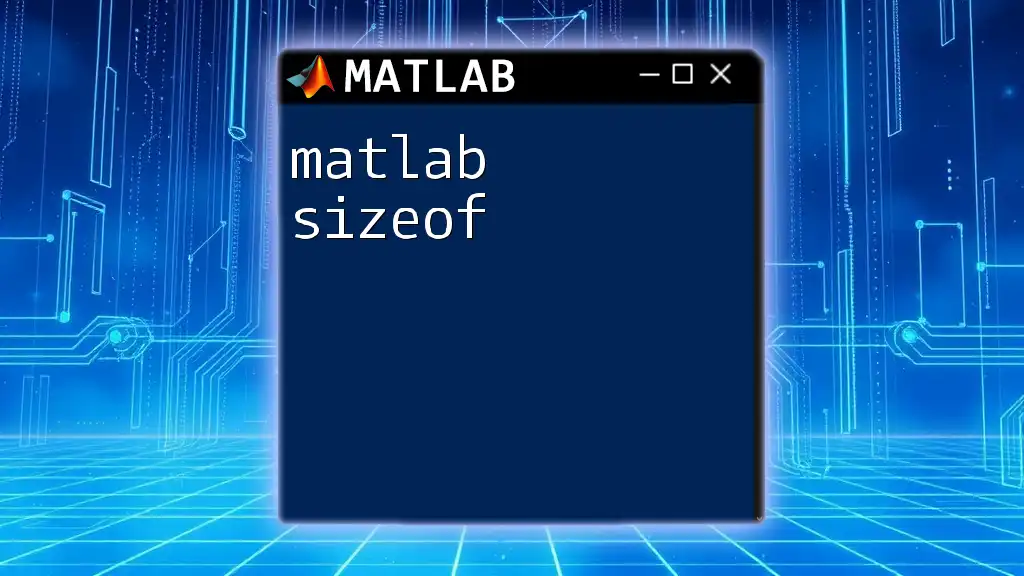
Call to Action
Don’t forget to subscribe for more insightful posts on MATLAB tips and commands! Dive deeper into MATLAB's plotting capabilities, and feel free to share your unique MATLAB plots and how you're utilizing marker sizes to tell your data's story better.
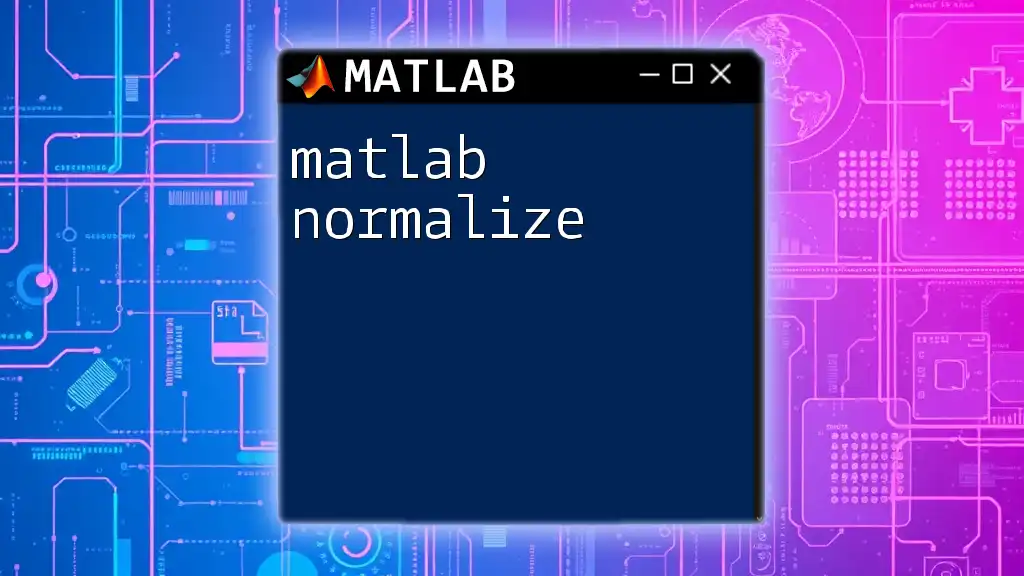
References
For further reading and deeper learning, explore additional MATLAB documentation and resources that can expand your knowledge on this topic.