In MATLAB, you can easily set titles for subplots using the `title` function after creating your subplots, allowing you to label each section of your figure clearly.
Here's a code snippet demonstrating how to do this:
% Create a figure with multiple subplots and titles
subplot(2, 2, 1);
plot(rand(1, 10));
title('Random Data 1');
subplot(2, 2, 2);
plot(rand(1, 10));
title('Random Data 2');
subplot(2, 2, 3);
plot(rand(1, 10));
title('Random Data 3');
subplot(2, 2, 4);
plot(rand(1, 10));
title('Random Data 4');
Understanding Subplots in MATLAB
What is a Subplot?
A subplot is a powerful feature in MATLAB that allows you to display multiple plots in a single figure window. This is particularly useful for comparing different datasets side by side without needing to create multiple figure windows. The primary benefit of subplots is the ability to convey complex information in a clear and organized manner, enhancing the viewer's understanding of the data.
Creating Subplots
To create subplots, you use the `subplot` function, which divides a figure into smaller sections. The basic syntax is:
subplot(rows, columns, index)
Here’s an example that creates a 2x2 layout of subplots:
subplot(2,2,1); % Creates the first subplot in a 2x2 grid
plot(rand(10,1)); % Generates a random plot
In this example, we specify `2` rows, `2` columns, and `1` as the index for the first subplot, allowing you to fill in subsequent positions by changing the index accordingly.
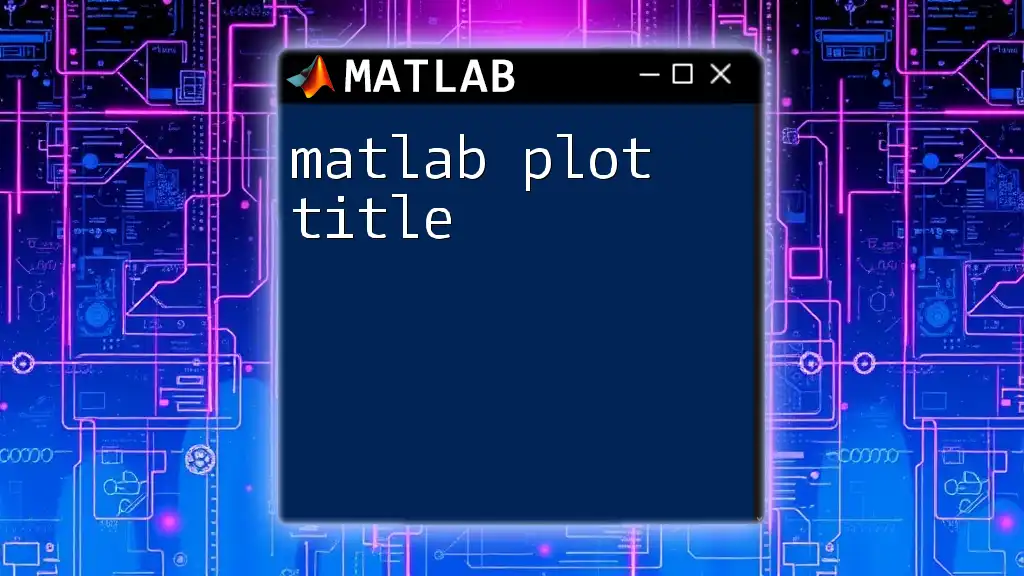
Adding Titles to Subplots
The Importance of Titles in Subplots
Titles in subplots serve more than just a decorative purpose; they are essential for conveying information about what each plot represents. A well-chosen title can guide the audience's understanding and interpretation of the results displayed in each subplot, thereby increasing engagement and clarity.
Using the Title Command
To add titles to each individual subplot, you utilize the `title` command. The syntax is straightforward and can be embedded right after you create the plot. Here’s an example demonstrating how to use it:
subplot(2,2,1);
plot(rand(10,1));
title('Random Data Plot 1'); % Adds a title to the first subplot
In this snippet, the title “Random Data Plot 1” clarifies what the viewer should expect from this particular subplot, thereby aiding in the overall comprehension of the figure.
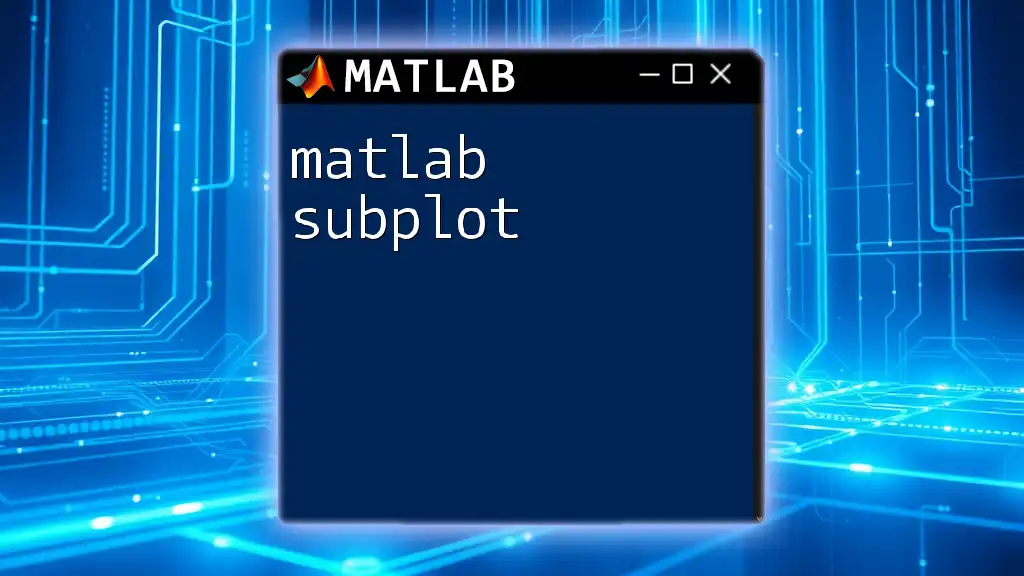
Advanced Title Customization
Using the `suptitle` Function for Overall Titles
When you want to add an overarching title that encompasses all the subplots, the `suptitle` function comes in handy. This function provides a convenient way to label the entire grid of subplots at once. Here’s how to use `suptitle`:
subplot(2,2,1);
plot(rand(10,1));
title('Plot 1');
subplot(2,2,2);
plot(rand(10,1));
title('Plot 2');
suptitle('Overall Title for Subplots'); % Adds a title for all subplots
This method helps set the context for the grouped plots, making it easier for the viewer to understand their relationship.
Customizing Title Appearance
MATLAB allows for extensive customization of title appearance, including font styles, colors, and sizes. For instance, you can make the title stand out by adjusting these parameters:
title('Customized Title', 'FontSize', 14, 'Color', 'red', 'FontWeight', 'bold');
In this example:
- FontSize changes the size of the title text.
- Color sets the title text color to red.
- FontWeight can be set to 'bold' for emphasis.
These features not only enhance visual appeal but also improve legibility.
Advanced Usage: Adding Subplot Titles Programmatically
Creating dynamic titles for subplots can save time, especially when dealing with multiple datasets. By using loops, you can automate the title generation process, making your code cleaner and more efficient. Here’s an example:
data = rand(2, 5);
for i = 1:length(data)
subplot(1, length(data), i); % Create subplots in a single row
plot(data(i,:));
title(['Data Set ' num2str(i)]); % Dynamically generates title
end
In this script, each subplot is labeled with “Data Set 1,” “Data Set 2,” etc., based on the index, enhancing clarity and organization.
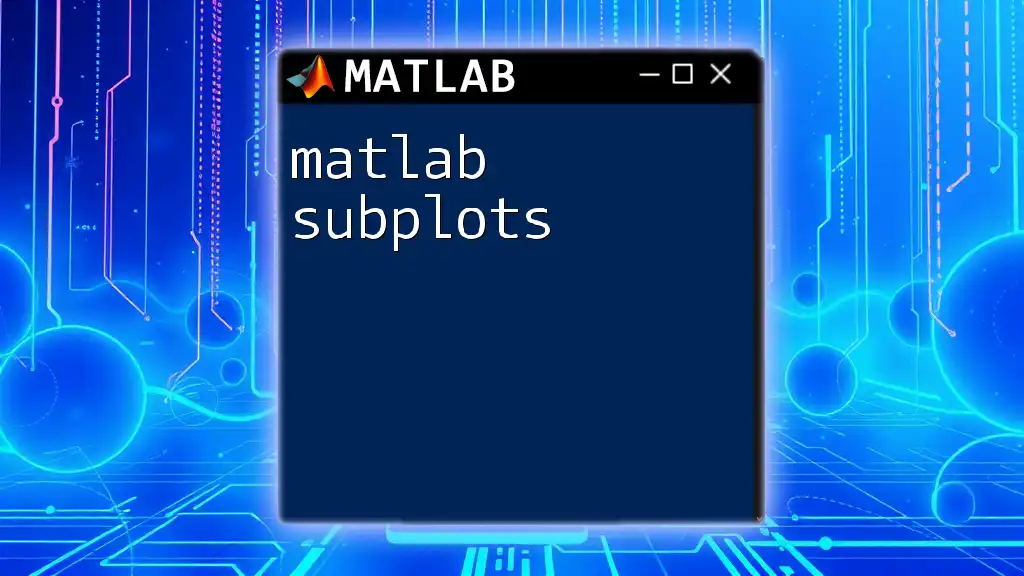
Common Issues and Troubleshooting
Overlapping Titles and Labels
One common issue with subplot titles is that they may overlap with plot labels or even with each other if not carefully positioned. When this happens, it can lead to confusion and misinterpretation. To avoid overlap, ensure that you allocate sufficient space for titles and that the titles are concise yet informative.
Figure Size and Layout Considerations
The size of the figure also plays a critical role in how clearly titles and plots are presented. If the figure is too small, certain elements may become cramped or lost. You can adjust the figure size with the following code:
set(gcf, 'Position', [100, 100, 800, 600]); % Adjust the figure size and position
This example expands the figure window, giving more room for plots and titles, thereby improving the overall presentation.
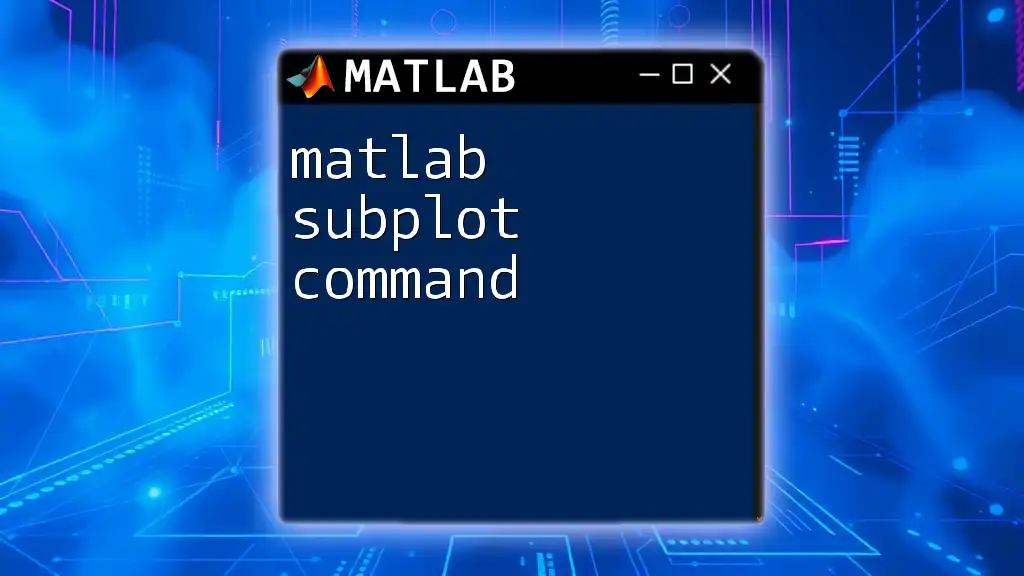
Case Studies: Real-World Applications of Subplots
Example 1: Comparing Data Trends
Subplots are especially effective for comparing trends across different datasets. For instance, if you were to visualize the sales trends of different products in separate subplots, the titles can specify each product, aiding in quick comparisons, making it easy for stakeholders to analyze performance.
Example 2: Displaying Multiple Related Variables
In cases where you need to visualize interrelated variables, subplot titles can significantly increase the understanding of the relations being represented. For instance, if you plot temperature changes over time for different cities, titles such as "City A Temperature" or "City B Temperature" can help viewers quickly grasp the data's significance.
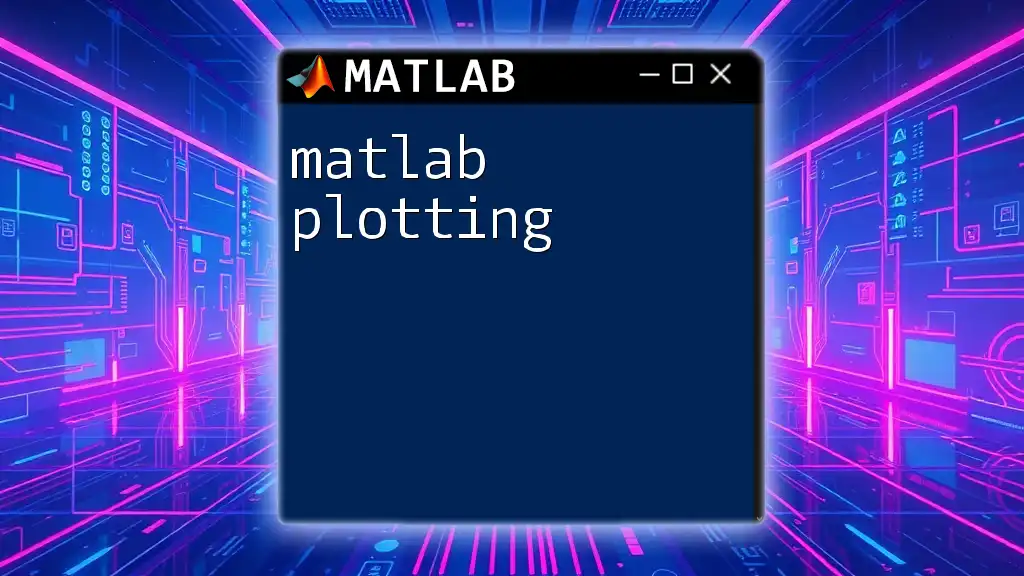
Summary
In summary, understanding how to effectively use MATLAB subplot titles greatly enhances data visualization. Titles not only provide context but also improve the interpretation of complex datasets. By mastering the syntax and customization options available in MATLAB, you can significantly boost the quality of your visual presentations.
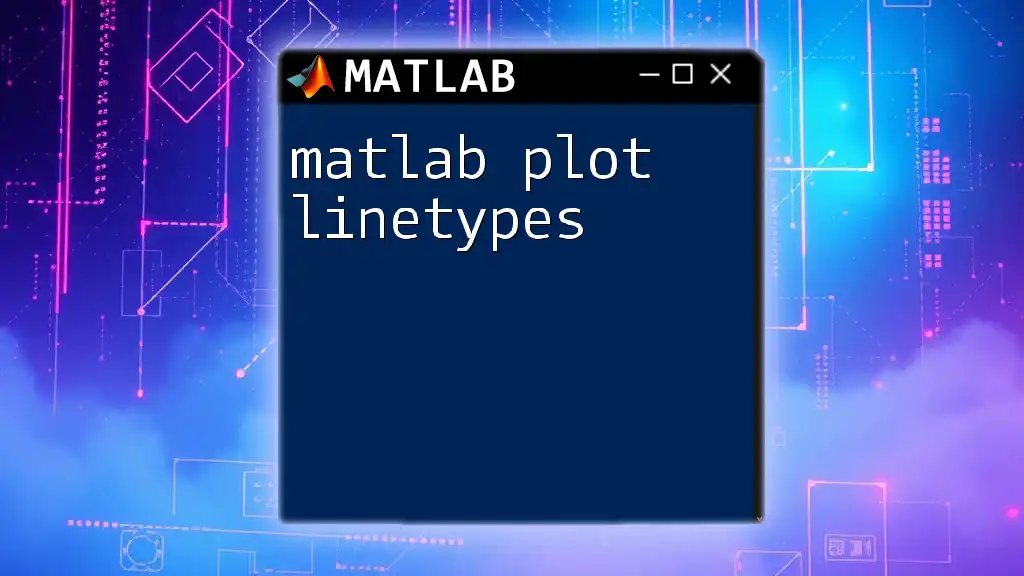
Additional Resources
Helpful Functions and Tools
Familiarize yourself with other MATLAB functions that can enhance subplot functionality, such as `xlabel`, `ylabel`, and advanced plotting tools like `subplot2grid` for more complex arrangements.
Recommended Reading
For further exploration, consider diving into MATLAB’s official documentation and literature, which offer extensive examples and best practices for data visualization techniques.
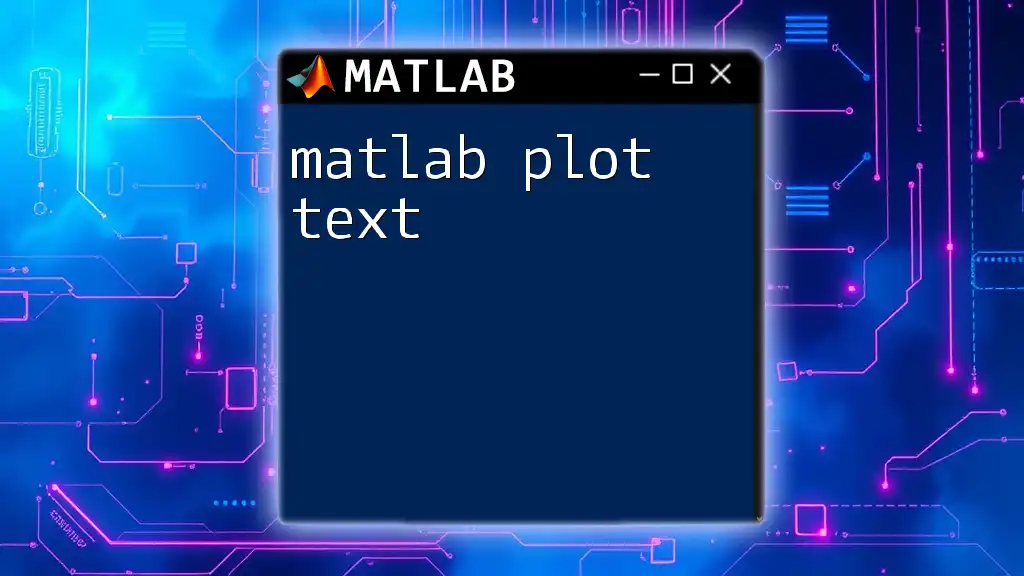
Conclusion
Mastering subplot titles in MATLAB can transform how you present your data. A well-labeled figure communicates information more effectively and provides clarity to your audience. Embrace these strategies in your work, developing clean, informative, and engaging visualizations.