To add a vertical line at a specific x-value in a MATLAB plot, you can use the `xline` function, which simplifies the process of drawing vertical lines on your figure.
Here's a code snippet demonstrating how to draw a vertical line at x = 3:
xline(3, 'r--', 'Line at x = 3'); % Draws a red dashed vertical line at x = 3
Understanding Vertical Lines
In the realm of data visualization, a vertical line marks a specified x-coordinate on a plot. These lines can effectively indicate thresholds or important events within your data, enhancing the clarity of visual interpretations. Using vertical lines in your MATLAB plots can highlight key data points, making it easier to convey significant information quickly.
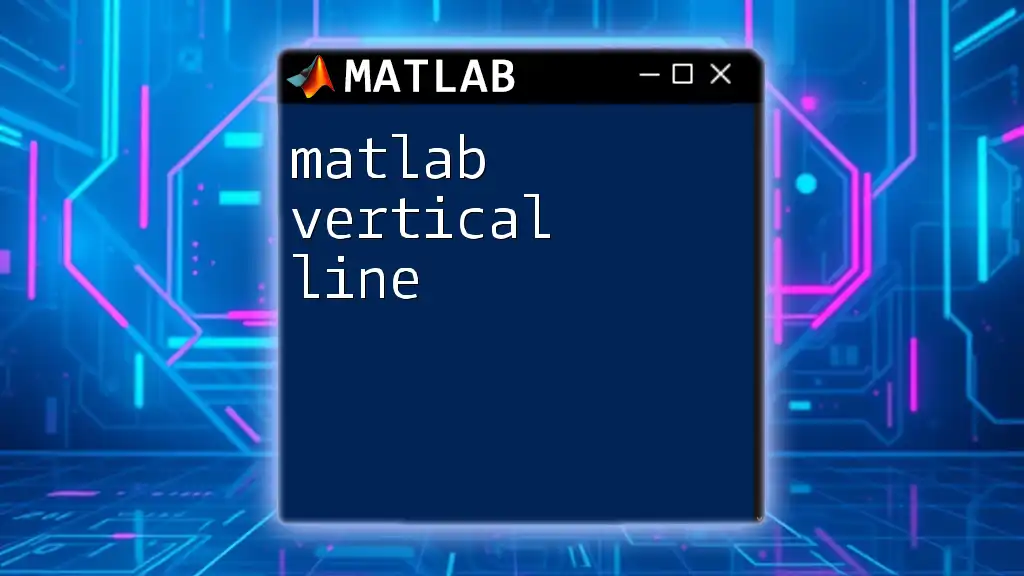
Basics of Plotting in MATLAB
To effectively utilize the `matlab plot vertical line`, you must first familiarize yourself with basic plotting commands. The `plot()` function is fundamental in creating standard 2D line plots. Understanding how the x and y axes interact is crucial, as it sets the foundation for any vertical lines you wish to add.
Overview of MATLAB Syntax
Before diving into vertical line commands, it's essential to grasp the basic syntax. MATLAB employs a straightforward-style scripting language. Variables can be defined easily, operators are intuitive, and the emphasis is on readability. Knowing these will significantly ease your plotting endeavors.
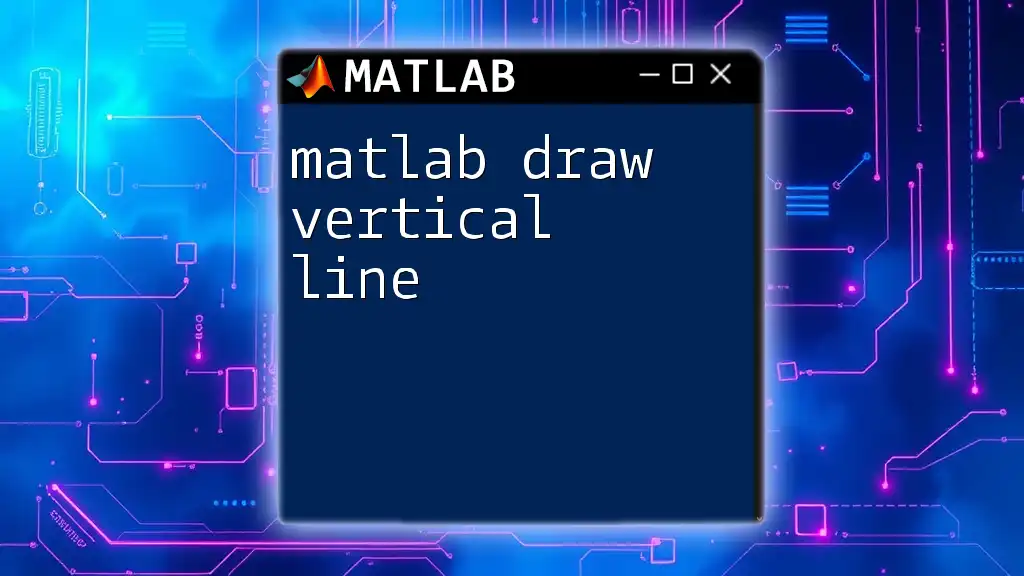
Plotting Vertical Lines in MATLAB
To draw a vertical line in your plot, you can use either the `xline()` or `line()` functions, each serving its purpose for different scenarios.
Using `xline()` Function
The `xline()` function is particularly useful for adding vertical lines with ease and precision.
Usage Explanation
This function allows you to create a vertical line at a specified position on the x-axis and can accommodate multiple customizable options.
Basic Syntax
The syntax for `xline` is straightforward:
xline(x_value, LineSpec, label)
Example Code: Simple Vertical Line
Here’s a quick example illustrating how to create a simple vertical line at x = 5 on a sine wave plot:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
xline(5, 'r--', 'Threshold', 'LabelHorizontalAlignment', 'left');
title('Sine Wave with Vertical Line');
xlabel('X-axis');
ylabel('Y-axis');
In this example, we plot the sine wave and add a vertical dashed red line at x = 5, labeled as "Threshold".
Using `line()` Function
For more control and customization, the `line()` function serves as a robust alternative.
Usage Explanation
The `line()` function gives you the flexibility to specify the coordinates, color, and style of the line.
Basic Syntax
The general syntax for the `line()` function is:
line([x1, x2], [y1, y2], 'PropertyName', PropertyValue)
Example Code: Vertical Line with Custom Properties
Below is an example where we use the `line()` function to draw a vertical line at x = 3, over a cosine wave plot:
x = 0:0.1:10;
y = cos(x);
plot(x, y);
hold on; % Hold the current plot
line([3 3], [-1 1], 'Color', 'blue', 'LineStyle', '--', 'LineWidth', 2);
hold off; % Release the plot
title('Cosine Wave with Custom Vertical Line');
xlabel('X-axis');
ylabel('Y-axis');
In this script, a vertical dashed blue line is created at x = 3, spanning from -1 to 1 on the y-axis, offering a clean representation of the point of interest.
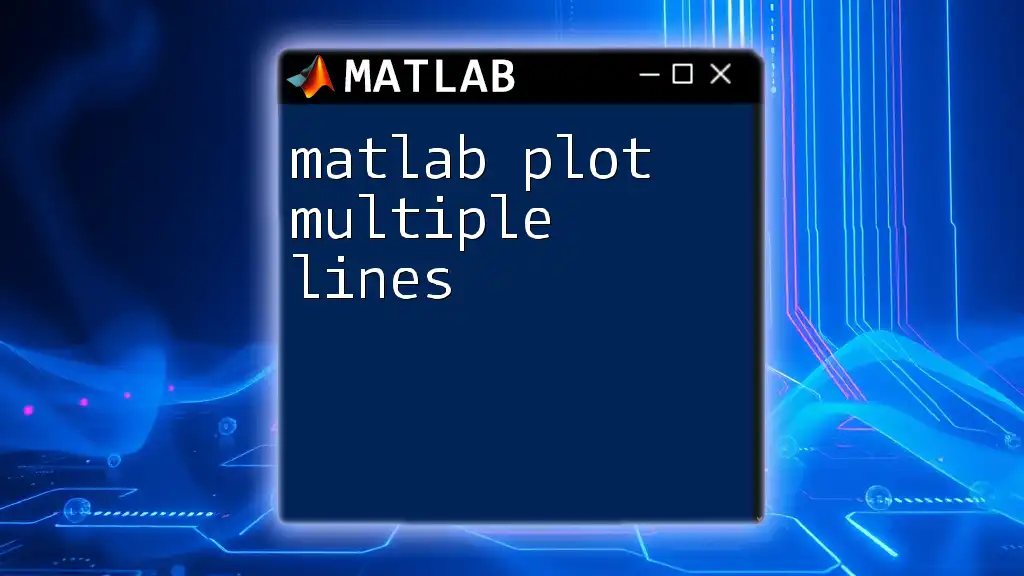
Customizing Vertical Lines
Customization enhances the clarity and efficacy of vertical lines in your plots. You can modify colors, styles, and labels for better visual communication.
Color and Style Customizations
MATLAB offers numerous predefined colors and styles. You can use basic colors, specify RGB values, or choose line styles such as dashed or dotted to make the vertical line stand out. For instance, you can specify the color with built-in options like `'r'` for red, or use RGB values such as `[0 0.5 0]` for a specific shade of green.
Adding Labels to Vertical Lines
Labels are instrumental in providing context to your vertical lines. They help viewers understand what the line represents without requiring additional explanation.
Example Code Snippet
Here's an example using `xline()` to add a descriptive label to a vertical line:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
xline(8, 'g:', 'Event', 'LabelHorizontalAlignment', 'right');
In this instance, a green dotted vertical line at x = 8 is labeled as "Event", enhancing the informational value of the plot.
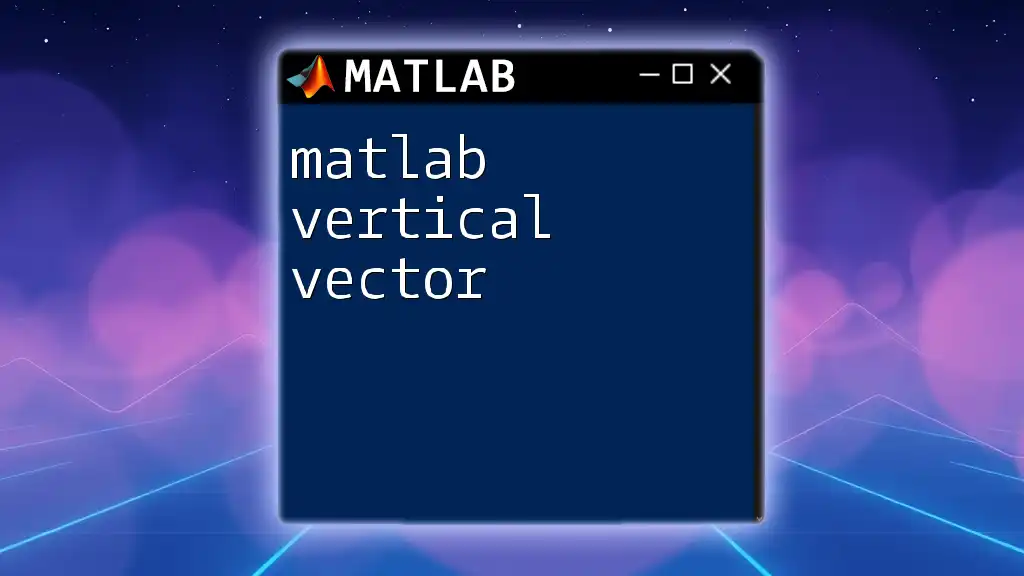
Practical Examples
Example: Annotating a Data Set with Vertical Lines
Consider a scenario where you have a data set representing sales over time. By adding vertical lines at critical sales figures or milestones (like product launches), you can visually annotate the data for better understanding.
Example: Vertical Lines to Represent Time Intervals
In time series analysis, vertical lines can signify important occurrences like changes in trends. For example, you may use vertical lines to mark economic events such as recessions or significant policy changes, allowing for clear correlations to be made with the plotted data.
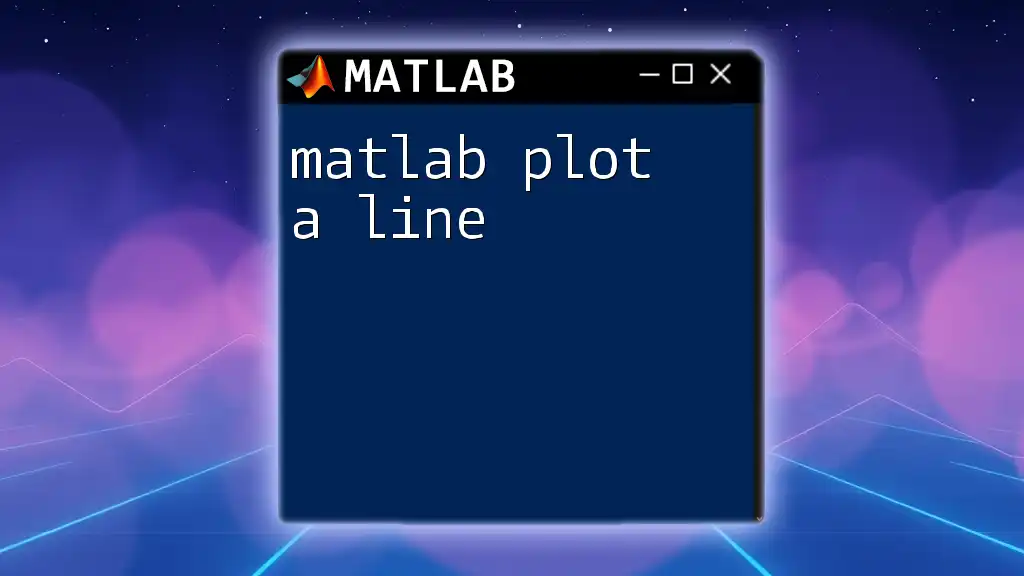
Debugging Common Issues
Why Vertical Lines Might Not Appear
Sometimes, vertical lines may not show up as intended. Common issues include incorrect x-axis limits or overlapping with plot boundaries. Adjusting the axes using `xlim()` or by ensuring that the line's x-coordinate is within the limits can resolve these issues.
Ensuring Line Visibility
To ensure that vertical lines are visible against your plot, double-check the limits of your axes. For instance, using `axis tight` may prevent important lines from being displayed if they fall outside the automatically set limits.
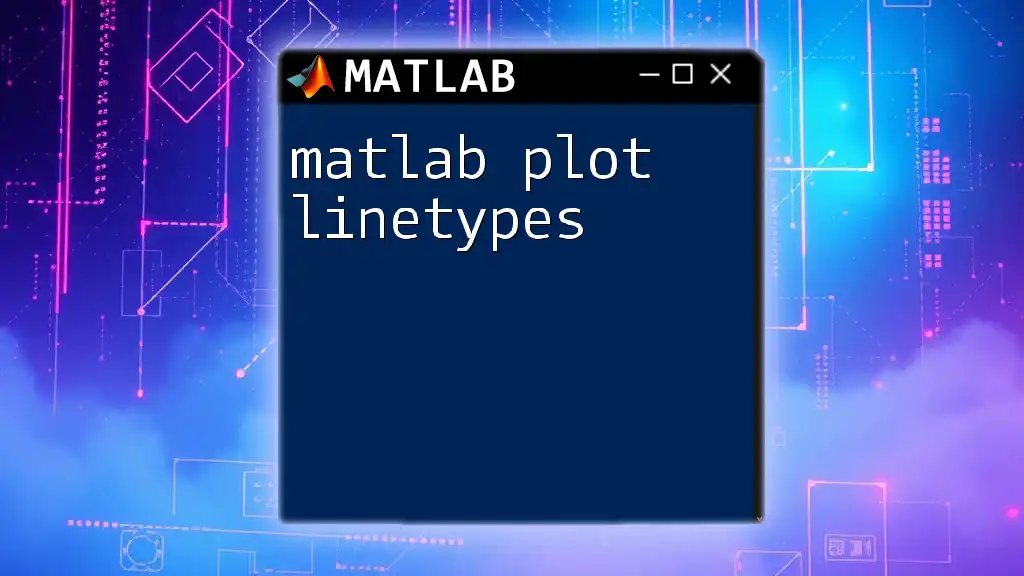
Conclusion
Incorporating vertical lines into your MATLAB plots is a simple yet powerful method to enhance data visualization. With tools like `xline()` and `line()`, you can customize your plots and clearly indicate significant data points. The use of vertical lines not only aids in visual storytelling but also enhances your audience’s comprehension.
Encouraging experimentation will help you fully leverage MATLAB’s plotting capabilities to convey information more effectively.
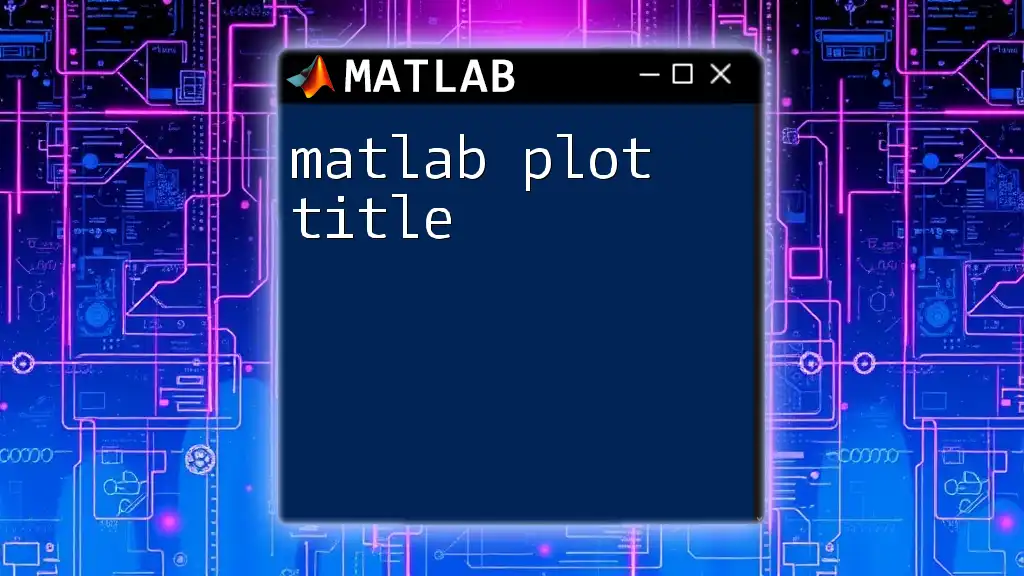
Frequently Asked Questions (FAQ)
What is the best way to add multiple vertical lines?
You can use a loop or simply call `xline()` or `line()` multiple times for different x-coordinates to add several vertical lines to your plot.
How can I save a plot with a vertical line to a file?
Use the `saveas()` or `print()` commands to save your plot in various formats such as PNG, JPEG, or PDF.
Can I use vertical lines in 3D plots?
Yes, you can create vertical lines in 3D plots using similar methods, ensuring you adjust the z-coordinate accordingly to visualize the line properly in three-dimensional space.
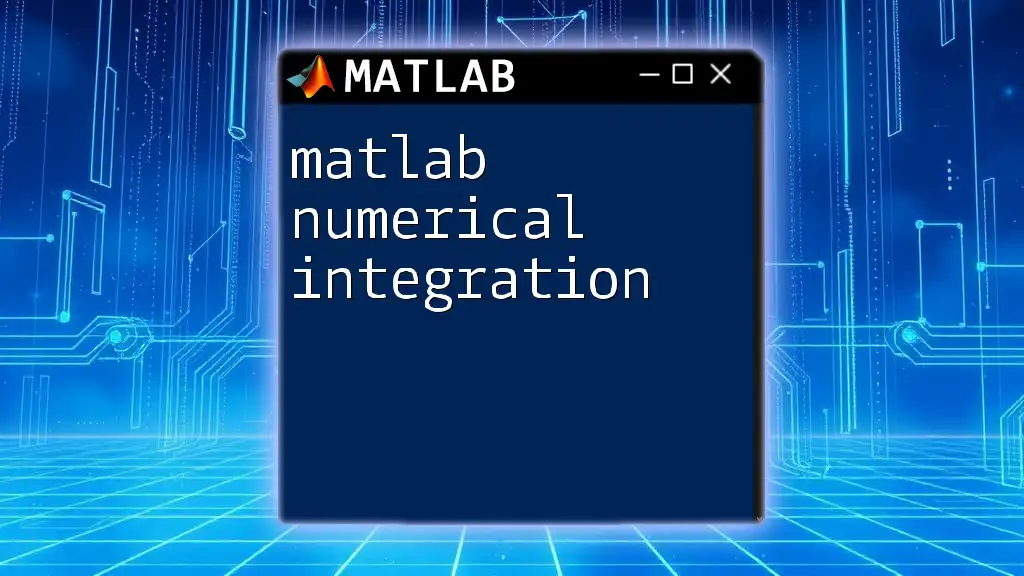
Additional Resources
For further exploration, refer to the official MATLAB documentation, engage with comprehensive tutorials online, and consider joining relevant forums and newsgroups for community support.
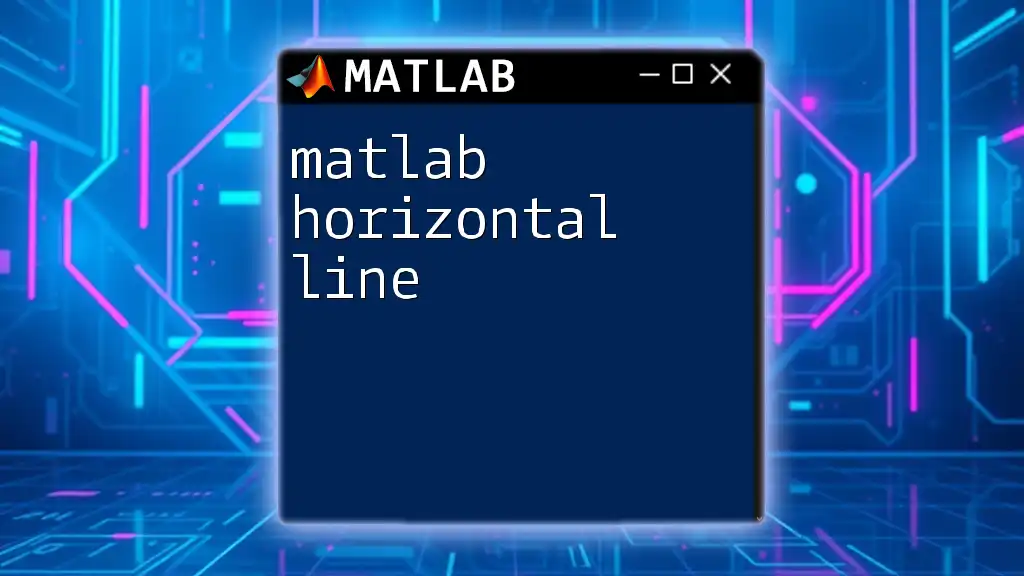
Call to Action
We encourage you to practice adding vertical lines to your own MATLAB projects and share your visualizations with others. For more tips and tricks on effectively using MATLAB, don’t forget to subscribe or follow us! Happy plotting!