To save an image in MATLAB, you can use the `imwrite` function, which writes an array as an image to a specified file format. Here’s a simple example:
img = imread('example_image.png'); % Read an image
imwrite(img, 'saved_image.jpg'); % Save the image as a JPEG file
Understanding Image Formats
Commonly Used Image Formats
When working with image files in MATLAB, understanding the different formats is crucial. Here are some of the most commonly used formats:
JPEG: This is a widely used standard for lossy compression of digital images, particularly for photographs. It effectively reduces file size, making it ideal for web use. However, be aware that repeated saving can lead to quality degradation.
PNG: Portable Network Graphics is a lossless format that supports transparency, making it perfect for images that require clear edges. If you need to maintain the original quality of the image without loss, PNG is the way to go.
TIFF: Tagged Image File Format is often used in professional environments due to its ability to handle high color depth and multiple layers. It's favored for printing and archival purposes because of its superior quality.
BMP: Bitmap is an uncompressed format, resulting in larger file sizes. It offers high-quality images but is less suitable for everyday use, especially where storage space is a concern.
Choosing the Right Format
Choosing the right image format is essential for maintaining the quality and usability of the files. Consider the following when selecting a format:
- If you're working with photographs or web images, JPEG might be ideal due to its balance of quality and file size.
- For graphics and images requiring transparency, opt for PNG.
- Use TIFF for high-quality prints or when you need to retain varying image layers.
- Choose BMP for scenarios where file quality supersedes file size limitations.
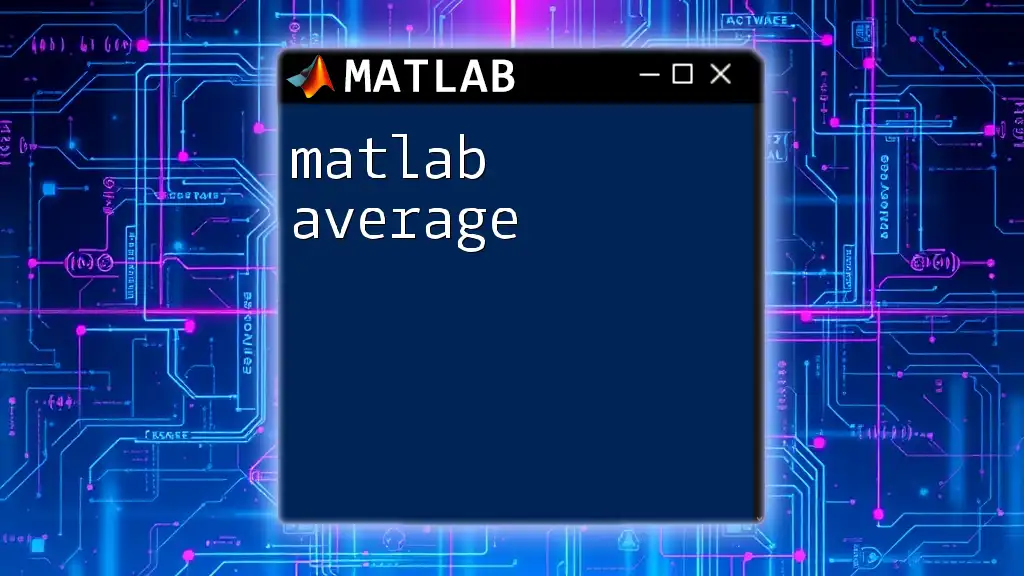
The Basics of Saving Images in MATLAB
Using the `imwrite` Function
The `imwrite` function is your primary tool for saving image data in MATLAB. This function allows you to save images in various formats, providing flexibility based on your needs.
General syntax:
imwrite(A, filename, fmt)
- A: The matrix representing the image.
- filename: The desired name of the output file, including its extension.
- fmt: An optional argument to specify the format.
Example of Saving an Image
Suppose you want to save an example image. Here's a straightforward example of how to do it:
% Creating a simple image and saving it as PNG
A = imread('example.jpg'); % Read an example image
imwrite(A, 'saved_image.png'); % Save the image
In this snippet:
- We load an image using `imread`.
- Then, we save that image as 'saved_image.png' with the desired format automatically inferred from the file extension.
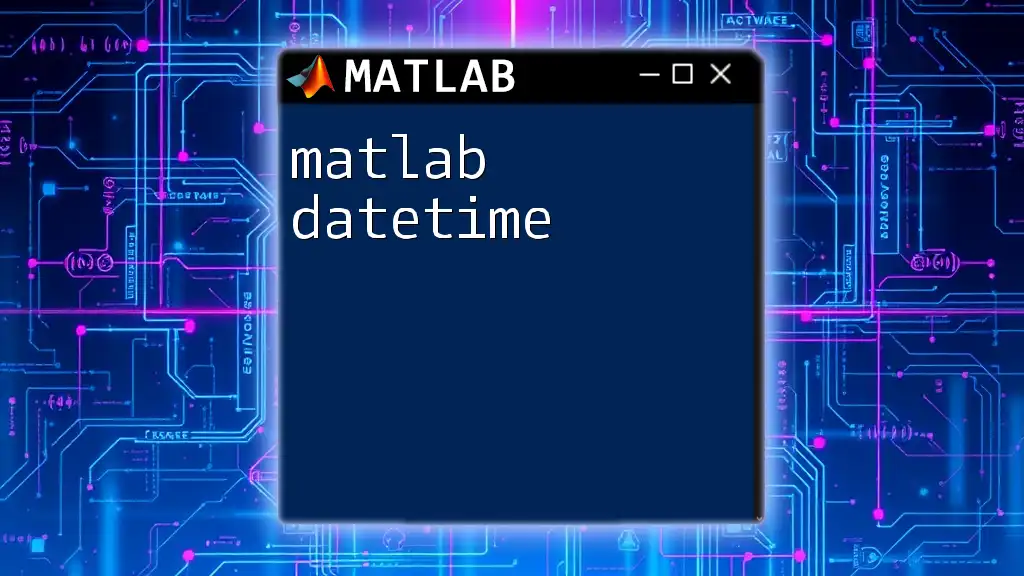
Saving Images with Different Options
Specifying Image Metadata
You can add metadata while saving images, which is useful for providing context. For example:
imwrite(A, 'saved_image_with_metadata.png', 'Comment', 'This is an example image');
In this code snippet, we've included a comment that can be retrieved later. Adding metadata can help enhance the understanding and usability of your images in collaborative environments.
Controlling Image Quality
For formats like JPEG, it’s possible to specify the compression quality. Lower-quality settings reduce the file size but can compromise clarity. For example:
imwrite(A, 'saved_image_quality.jpg', 'Quality', 90); % 90% quality
In this case, a quality value of 90 means that most of the image's original quality is preserved while optimizing file size.
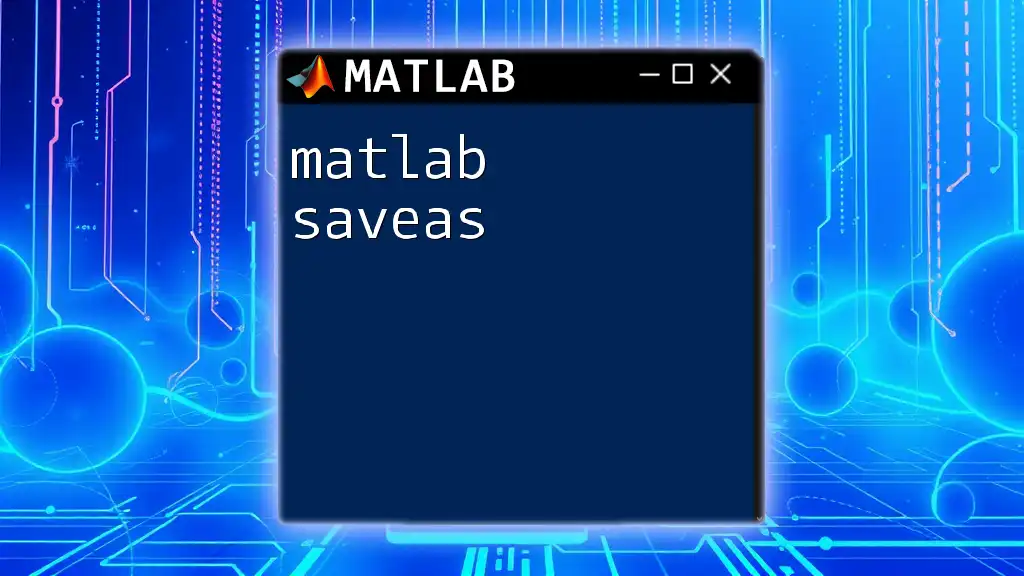
Saving Figures as Images
Exporting Figures Using `saveas`
Sometimes, you're looking to save a figure rather than just an image array. The `saveas` function is perfect for this scenario.
figure;
imshow(A);
saveas(gcf, 'figure_image.png'); % Save current figure
Here, `gcf` refers to the current figure, allowing you to save it directly. You can save figures in various formats, such as PDF or EPS, based on your requirements.
Providing Figure Customization
Customization is key to making your visualizations effective. Use titles, labels, and legends to convey important information before saving:
figure;
imshow(A);
title('Sample Image');
xlabel('X-axis label');
ylabel('Y-axis label');
saveas(gcf, 'customized_figure_image.png');
This technique not only enhances the visual aspect but also delivers pertinent information straight from the image file.
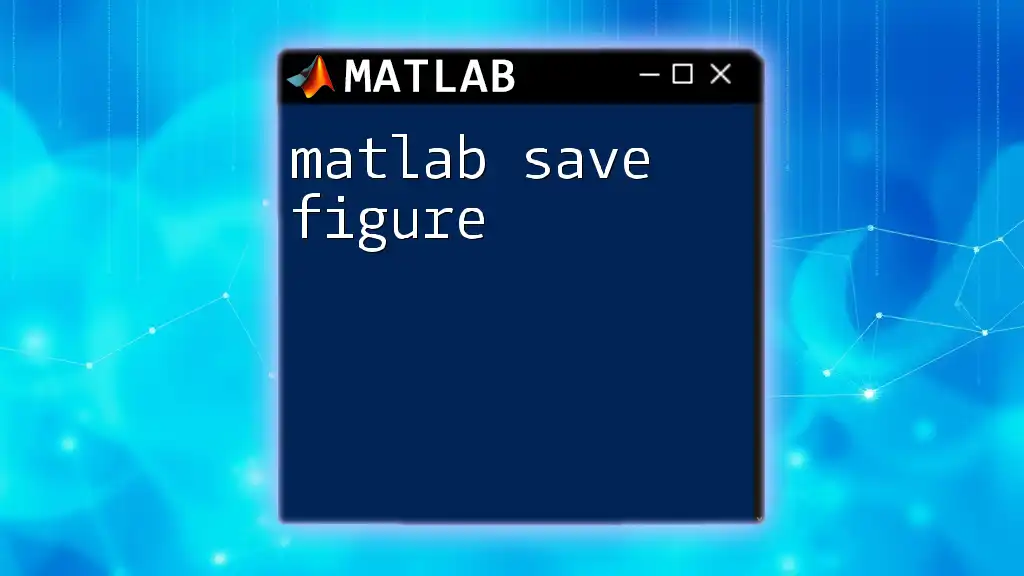
Advanced Image Saving Techniques
Working with Color Maps
Color maps can significantly alter the display of your images, particularly for grayscale or indexed images. Here's how to save an image with a color map:
imshow(A, []);
colormap(gray);
imwrite(A, 'gray_image.png');
In this case, using the `gray` colormap allows you to save the image in grayscale format, capturing nuances not visible in color.
Using `exportgraphics` for High-Quality Figures
For those needing high-resolution outputs, `exportgraphics` is a powerful function introduced in later MATLAB versions.
exportgraphics(gcf, 'high_quality_image.png', 'Resolution', 300);
With the resolution parameter set to 300, this command ensures a high-quality output suitable for printing or detailed analysis.
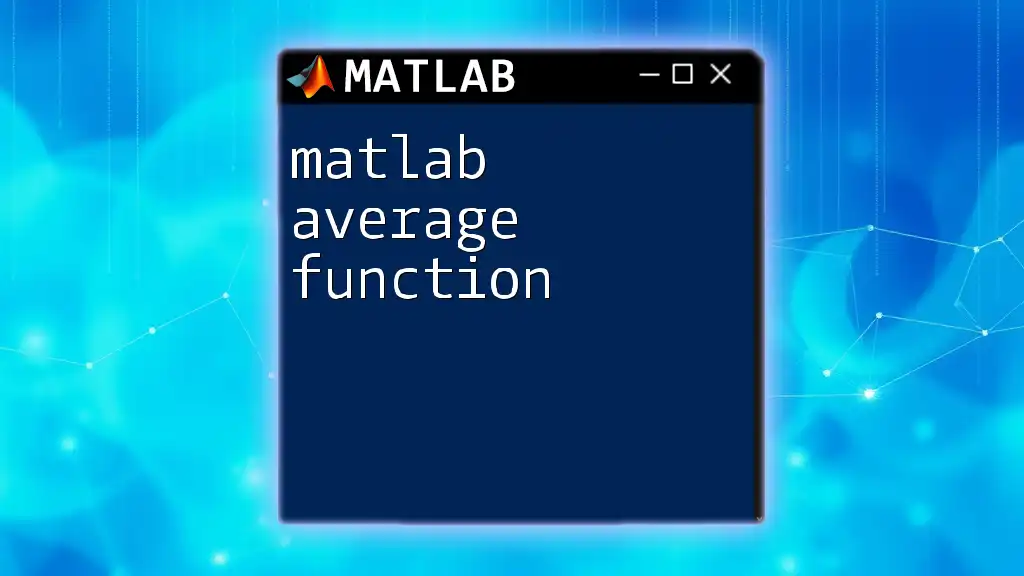
Troubleshooting Common Issues
File Permissions
When saving images, you may encounter file permission issues. Ensure you have write access to the designated folder to avoid "permission denied" errors. Check file paths and permission settings, especially in network environments.
Format-Specific Errors
You might also face errors related to unsupported formats or missing toolboxes. Always confirm that you have the required toolboxes installed and that you're using an appropriate format supported by MATLAB.
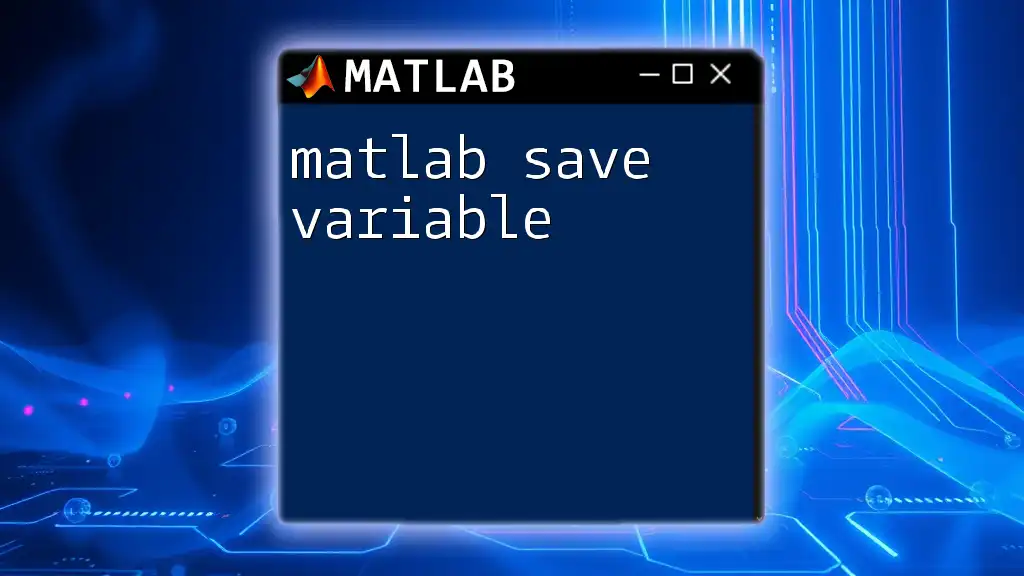
Conclusion
In this comprehensive guide on how to matlab save image, you've learned about selecting suitable image formats, using functions like `imwrite` and `saveas`, and optimizing image quality and metadata. Experimenting with different commands and options can significantly enhance your image processing skills in MATLAB. Remember to practice these techniques, as hands-on experience solidifies your understanding and makes the learning process effective.
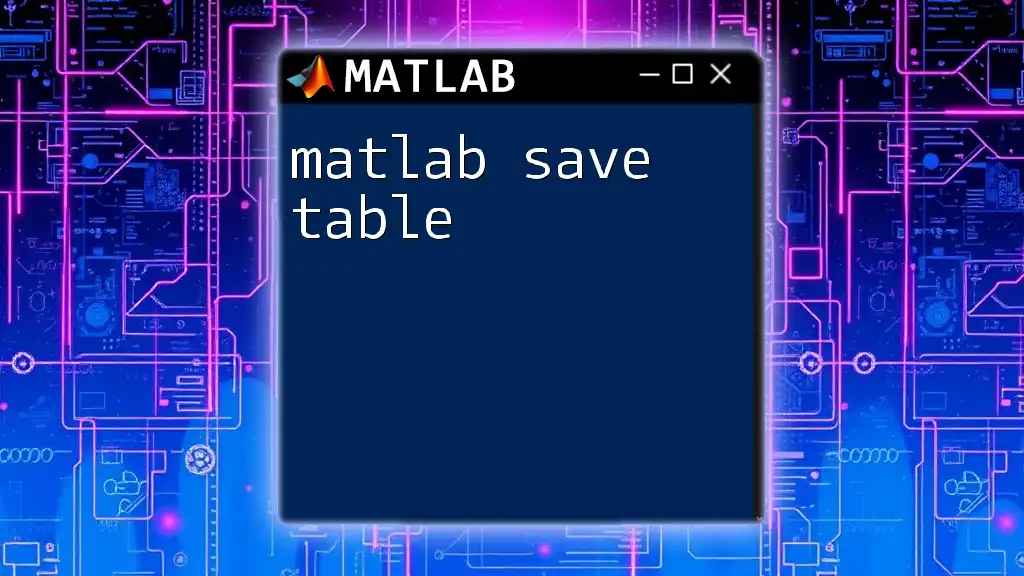
Additional Resources
For further exploration around image processing in MATLAB, consider reading the official MATLAB documentation focused on Image Processing. Also, community forums can be a great place to ask questions and share your experiences with others.
Join our community to stay up-to-date with the latest tips and tricks in MATLAB, and don't hesitate to share your own journey with saving images!