"MATLAB SS" refers to the state-space representation used in control systems and signal processing, where state-space models are defined by matrices that describe system dynamics.
% Example of creating a state-space model in MATLAB
A = [0 1; -2 -3]; % System matrix
B = [0; 1]; % Input matrix
C = [1 0]; % Output matrix
D = [0]; % Feedforward matrix
sys = ss(A, B, C, D); % Create state-space system
Understanding State-Space Representation
What is State-Space Representation?
The state-space representation is a powerful mathematical framework used to model dynamic systems. It captures the dynamics of systems in terms of variables known as state variables, which are represented in a structured form using matrices.
The state-space model consists of four main matrices:
- A (State matrix): Defines the system dynamics.
- B (Input matrix): Relates the input to the state.
- C (Output matrix): Connects the state to the output.
- D (Feedforward matrix): Represents the direct transmission from input to output.
Benefits of Using State-Space Models
State-space models offer significant advantages over traditional transfer function representations, particularly in the context of Multiple Input Multiple Output (MIMO) systems. The flexibility provided through state-space representations allows for the seamless modeling of complex systems that may not be easily represented with transfer functions.
Key Benefits:
- Enable a comprehensive understanding of system dynamics.
- Easily handle systems with multiple inputs and outputs.
- Facilitate control strategies and stability analysis.
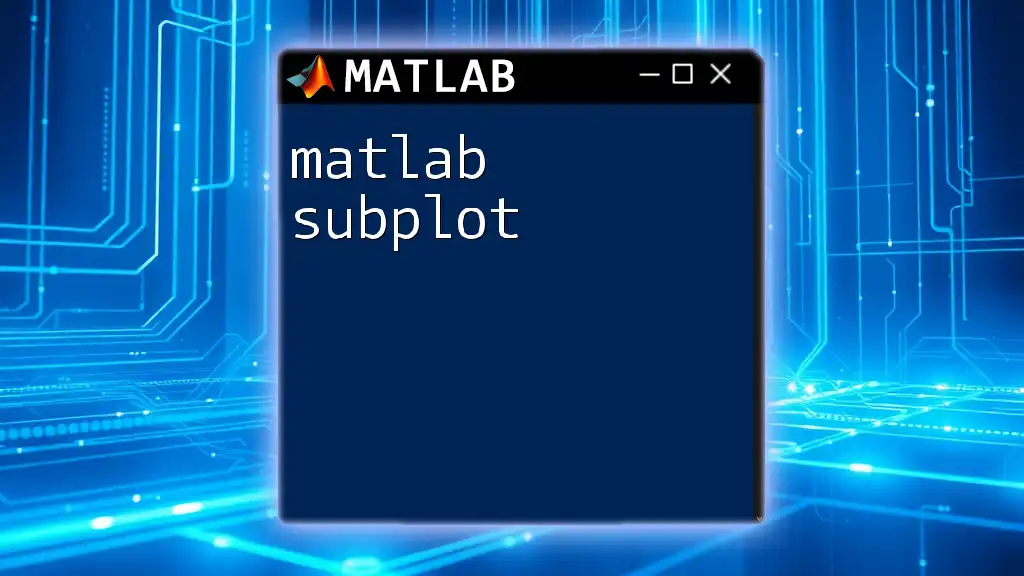
Syntax of the `ss` Command
Basic Syntax
The `ss` command in MATLAB is essential for creating state-space models. The general form of the command is as follows:
sys = ss(A, B, C, D)
In this syntax:
- A: The state matrix, which describes the system dynamics.
- B: The input matrix, indicating how the control inputs affect the states.
- C: The output matrix, revealing how states contribute to the outputs.
- D: The feedforward matrix, showing any direct influence of inputs on outputs.
Creating State-Space Systems
Example 1: Simple State-Space Model
To illustrate the creation of a state-space model using the `ss` command:
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
sys = ss(A, B, C, D);
In this example, the state matrix `A` and the input matrix `B` structure the system dynamics, while the output matrix `C` and feedforward matrix `D` relate the states to the output. This simple model represents a second-order dynamic system where the state variables are directly influenced by the input.
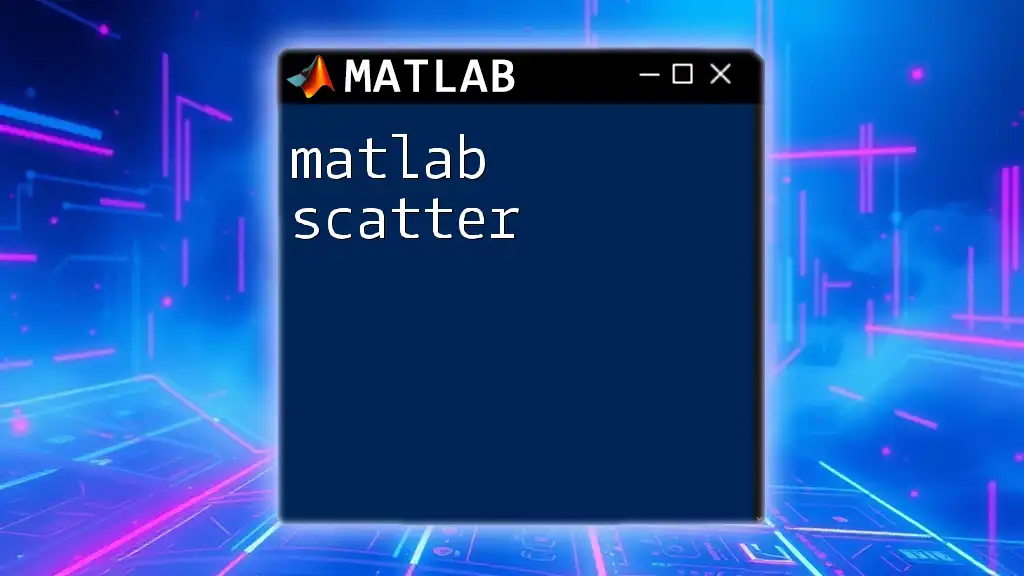
Converting Multiple Systems into State-Space
Using `ss` with Different Matrices
The `ss` command is also incredibly versatile and can be employed to convert different types of models into state-space forms.
Example 2: Converting Transfer Functions to State-Space
When a transfer function is available, MATLAB provides a straightforward mechanism to convert it into a state-space representation:
num = [1];
den = [1, 3, 2];
sys_tf = tf(num, den);
sys_ss = ss(sys_tf);
In this example, the transfer function defined by `num` and `den` is transformed into a state-space model using the `ss` command. Understanding this conversion is essential as it allows for the application of advanced control techniques that may be easier to express in state-space form.
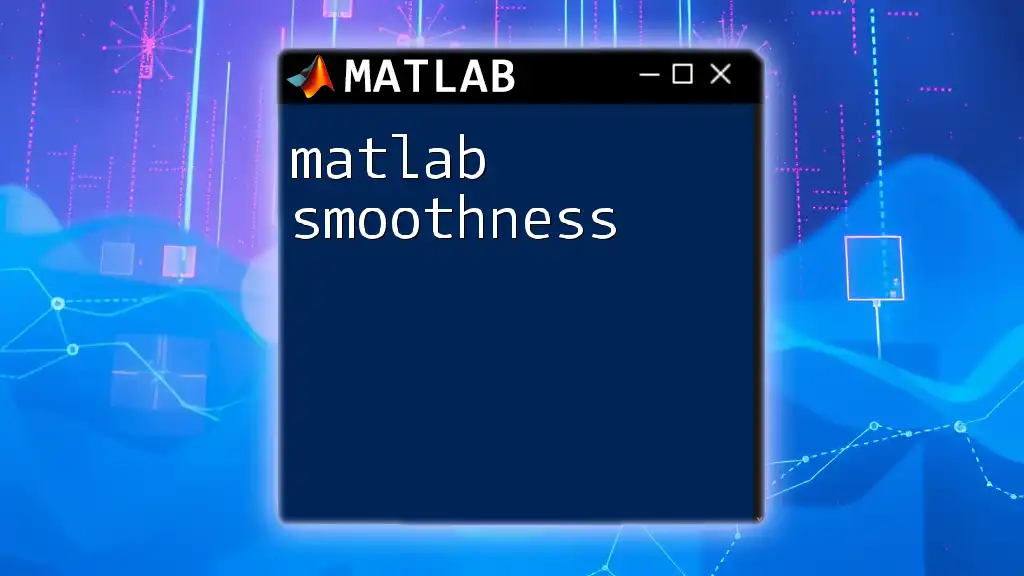
Analyzing the State-Space Model
Exploring Properties of the State-Space Object
Once you've created a state-space model, it's crucial to analyze its properties to gain insights into the stability and behavior of the system.
Stability Analysis
Stability is a key aspect in control systems design. To analyze stability, one can examine the eigenvalues of the state matrix `A`:
eigenvalues = eig(A);
Interpretation: The locations of the eigenvalues in the complex plane determine the stability of the system. If all eigenvalues have negative real parts, the system is stable. Conversely, positive real parts indicate instability.
Frequency Response of the State-Space Model
Another important analysis involves examining the frequency response of the system using Bode plots, which provide insight into how the system reacts to different frequencies.
Bode Plots
To generate a Bode plot for the state-space model, simply use:
bode(sys);
Bode plots detail the magnitude and phase of the system's output relative to its input across a spectrum of frequencies, helping engineers to understand the system behavior comprehensively.
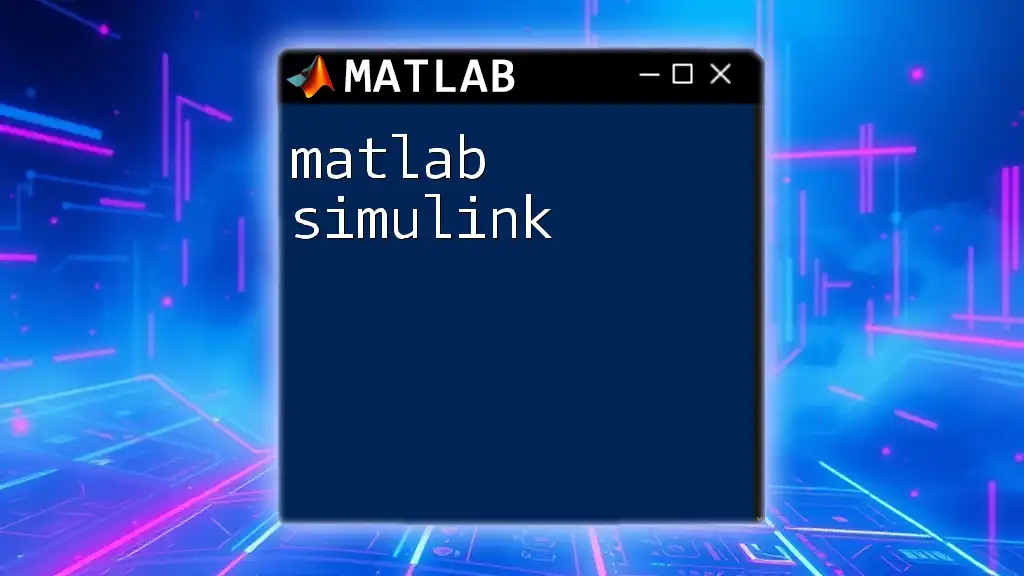
Practical Applications of the `ss` Command
Control System Design
Control system design often makes extensive use of the state-space representation due to its flexibility and effectiveness in managing system responses.
Feedback Control Systems
Designing a closed-loop feedback control system is a significant application of the `ss` command. The placement of poles can be achieved with commands to ensure necessary stability and performance criteria.
K = place(A, B, [-1 -2]);
A_cl = A - B*K;
sys_cl = ss(A_cl, B, C, D);
In this scenario, the state feedback matrix `K` is derived using pole placement, and the modified state matrix `A_cl` defines the closed-loop dynamics.
Simulation of State-Space Models
Simulation of the state-space model is vital for visualizing system performance over time.
Time Response Analysis
Two fundamental functions, `step` and `impulse`, allow for the simulation of system responses to inputs.
step(sys);
impulse(sys);
Significance: The step response indicates how the system reacts to a sudden change, while the impulse response provides insights into how the system responds to a brief input pulse. Both are crucial for designing control strategies.
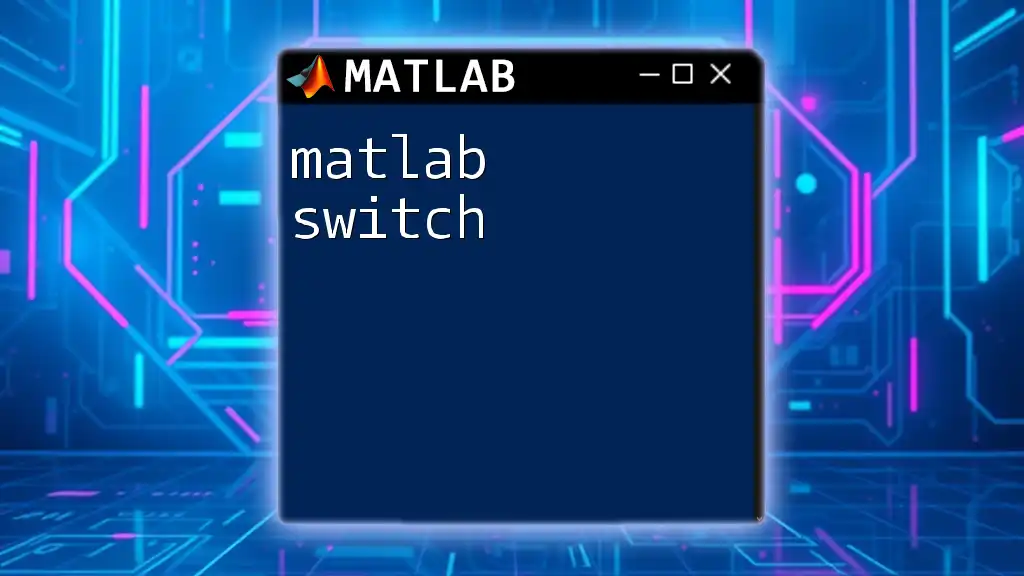
Visualization Techniques
Plotting the Response of the State-Space Model
Visual representation of system responses can greatly aid in understanding and communicating system behavior.
figure;
subplot(2,1,1);
step(sys);
title('Step Response');
subplot(2,1,2);
impulse(sys);
title('Impulse Response');
Using MATLAB’s built-in plotting functions allows you to generate detailed visual outputs that illustrate both step and impulse responses, enabling a clearer understanding of the system's dynamic characteristics.
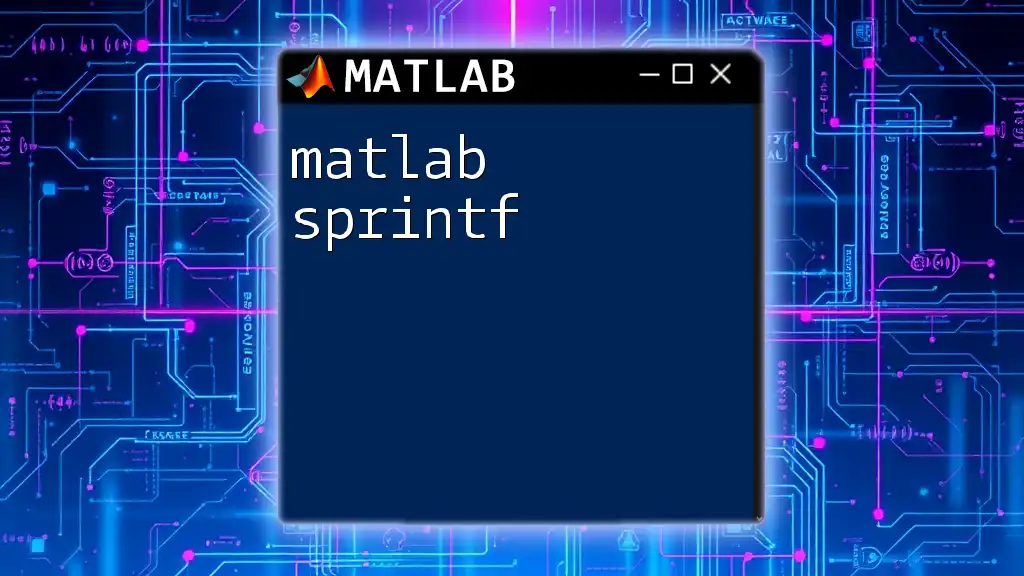
Conclusion
The `ss` command in MATLAB stands as a cornerstone for creating and analyzing state-space models. By mastering this command, engineers and researchers can effectively model dynamic systems, perform stability analyses, and design sophisticated control systems.
This guide has provided you with a comprehensive understanding of the `ss` command, its syntax, practical applications, and methods for analysis and visualization. To fully leverage the capabilities of MATLAB, we encourage you to practice exploring the `ss` command in various contexts and gain hands-on experience.
For additional resources and community discussions, consider joining forums and exploring tutorials that can help further broaden your knowledge in MATLAB.