In MATLAB, the `step` function is used to process a discrete-time system input and output, allowing users to evaluate system behavior at each discrete time step.
Here's an example of using the `step` function with a predefined transfer function:
% Define a transfer function
num = [1]; % Numerator coefficients
den = [1, 5, 6]; % Denominator coefficients
sys = tf(num, den); % Create a transfer function
% Use the step function to plot the step response
step(sys);
title('Step Response of the System');
xlabel('Time (seconds)');
ylabel('Amplitude');
What is MATLAB Step?
The MATLAB step function is a powerful tool used primarily in control systems and signal processing to analyze the behavior of dynamic systems. It provides insights into how systems respond to step inputs, which is crucial for evaluating system stability, performance, and design.
What is the Step Function?
In MATLAB, the step function calculates the step response of a system characterized by either a transfer function or state-space representation. The step response reflects the output of a system when a step input is applied, typically identifying how the system reacts over time.
Syntax of the Step Function
The basic syntax of the step function is straightforward:
step(sys)
Here, `sys` is a representation of the system you want to analyze. This could be a transfer function, state-space model, or any other valid control system representation. Understanding the parameters and their significance is vital for effective application.
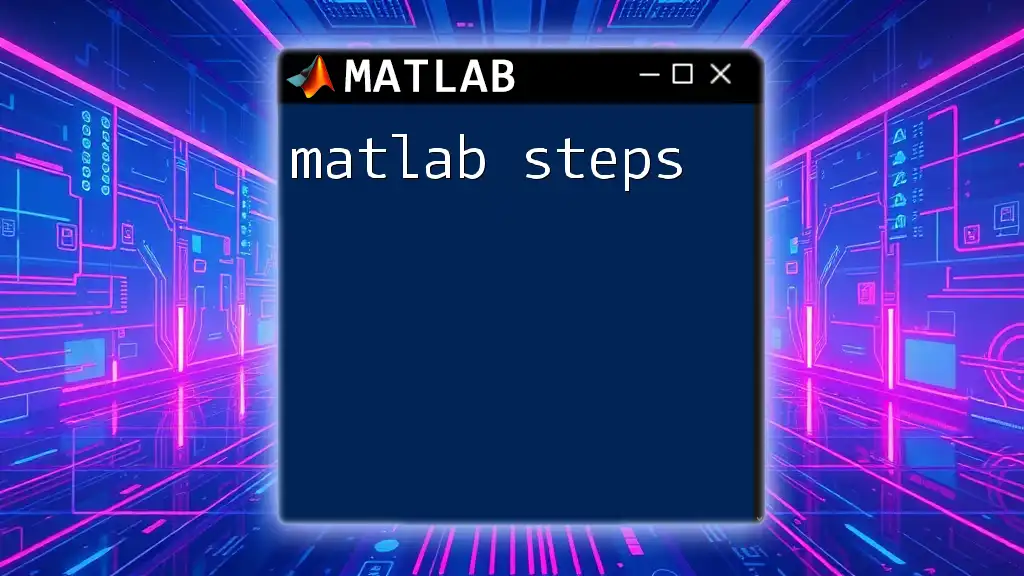
Practical Applications of Step in MATLAB
Controlled Systems Analysis
The step function is essential for understanding the response of dynamic systems, especially in control engineering. It allows engineers to assess how quickly a system can respond to changes and helps in designing controllers accordingly.
Example of a Transfer Function
As an illustration, consider a basic transfer function characterized by a simple numerator and denominator:
num = [1]; % Numerator coefficients
den = [1, 2, 1]; % Denominator coefficients
sys = tf(num, den);
step(sys)
In this example, MATLAB calculates the step response of a second-order system. The output graph generated would provide valuable insights about the system's behavior, such as the rise time, settling time, and overshoot.
Signal Processing
The step function also plays a crucial role in signal processing applications. Understanding the step response can help analyze and predict how systems handle various types of signals, particularly in evaluating the stability and effectiveness of filters and controllers.
For example, simulating a signal's response can be done using the following lines of code:
t = 0:0.01:10; % Time vector
u = ones(size(t)); % Step input
y = lsim(sys, u, t); % System response to step input
plot(t, y)
title('Response of the System to Step Input')
xlabel('Time (s)')
ylabel('Response')
This MATLAB script generates a plot illustrating how the system reacts to a step input signal over time.
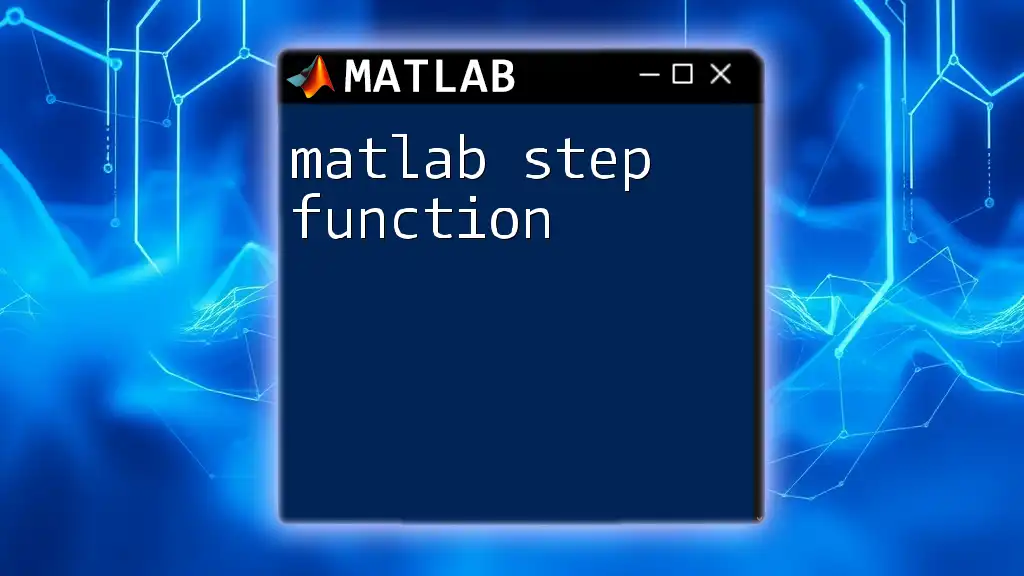
Visualizing the Step Response
Plotting Step Responses
Visualization is an integral component of understanding MATLAB step responses. The following command generates a simple yet informative plot:
step(sys)
grid on
title('Step Response of the System')
xlabel('Time (seconds)')
ylabel('Output')
By effectively using plots, engineers can easily identify key characteristics of the system's response and make informed decisions regarding adjustments and improvements.
Analyzing the Graph
When analyzing the step response graph, several crucial features should be examined:
- Rise Time: The time it takes for the system's response to rise from a specified low to a specified high percentage of the final value.
- Settling Time: The time required for the system's response to stay within a certain percentage of the final value.
- Overshoot: The amount by which the response exceeds its final steady-state value before settling down.
These characteristics are pivotal in determining system performance and stability.
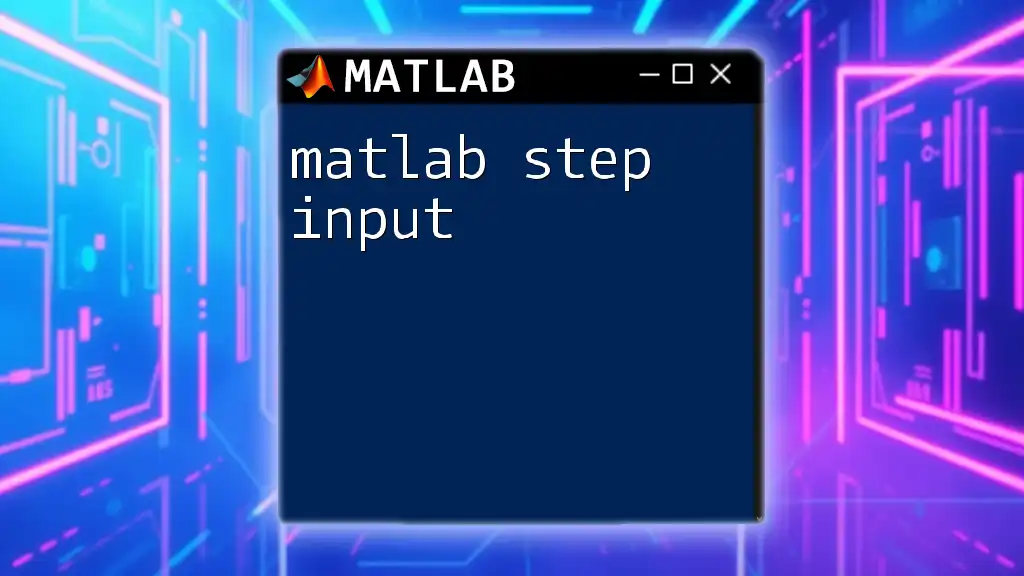
Advanced Features
Multiple Systems and Customization
MATLAB allows the analysis of multiple systems simultaneously. For example, if you wanted to compare the step responses of different systems, you can do so with the following command:
sys1 = tf([1], [1, 2, 1]);
sys2 = tf([1], [1, 1]);
step(sys1, sys2)
legend('System 1', 'System 2')
This approach provides a nuanced view of how different systems compare when subjected to the same step input.
Customizing Step Response Graphics
To enhance clarity and presentation, MATLAB enables customization of step response graphics. Consider the following snippet:
h = stepplot(sys);
h.LineStyle = '--'; % Changing line style
h.Color = 'r'; % Changing line color
title('Customized Step Response Plot')
Customizing the appearance of your plots can make your results more comprehensible and visually appealing, especially when presenting to others.
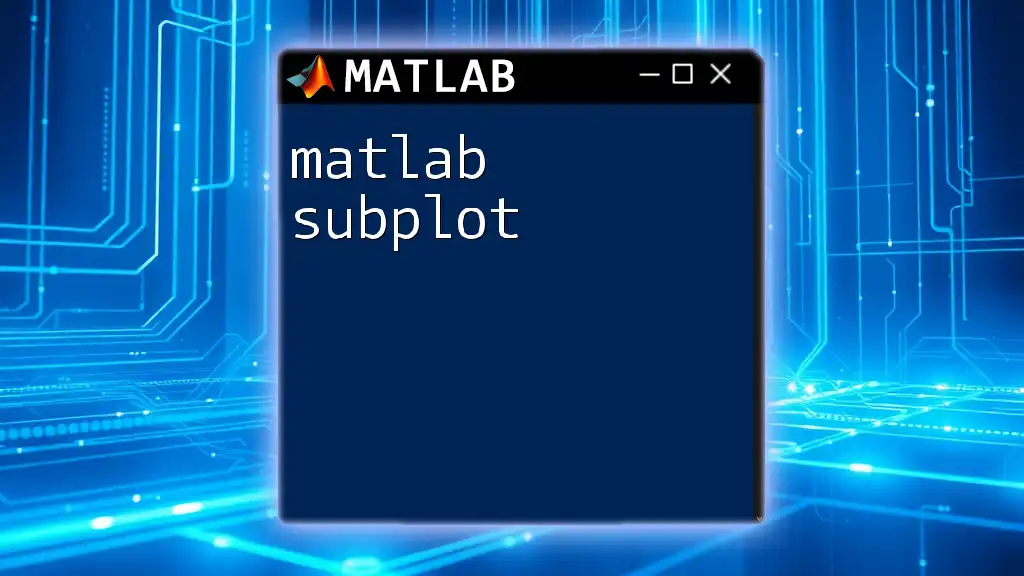
Troubleshooting Common Issues
While using the MATLAB step function, you may encounter several issues. It is crucial to understand common errors and how to resolve them.
-
Error Messages: MATLAB will often return error messages that point to issues with the system's representation. Ensure that the system provided is valid and correctly defined.
-
Pitfalls to Avoid: A frequent mistake is attempting to analyze a non-dynamic system or incorrect function parameters. Double-check your system definition and ensure it is appropriate for the analysis intended.
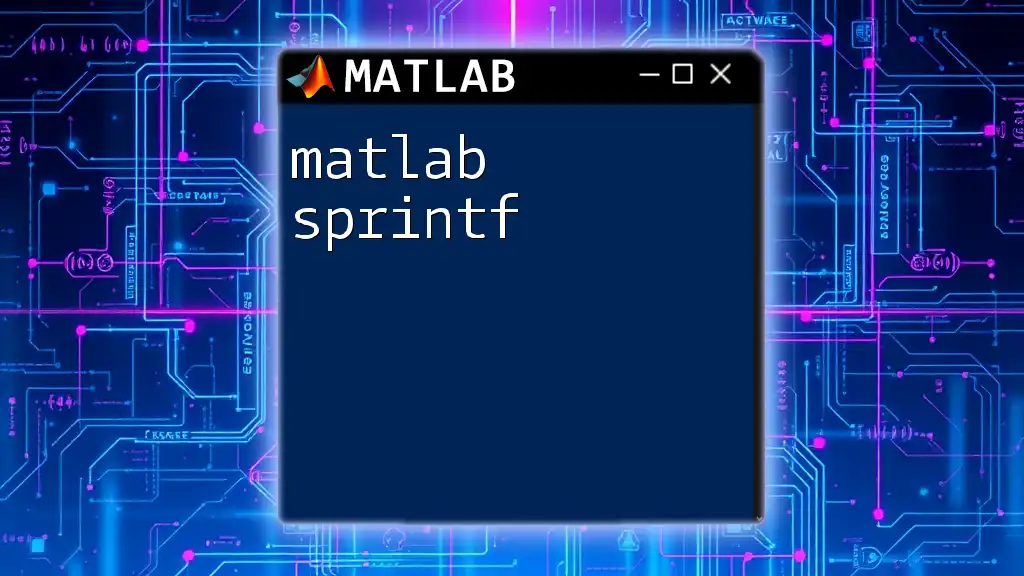
Recap of Key Takeaways
The MATLAB step function is an invaluable tool for analyzing and visualizing the behavior of dynamic systems. By mastering the step function, you can gain critical insights into system performance, stability, and responsiveness, greatly enhancing your skills in control systems and signal processing.
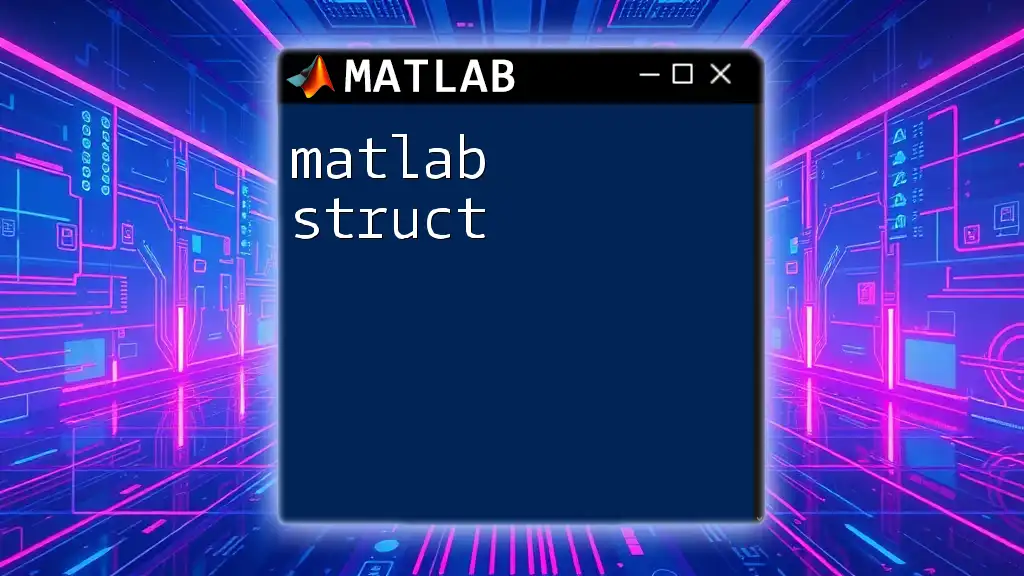
Encouragement for Further Learning
To deepen your understanding of MATLAB functionalities, explore additional resources. The official MATLAB documentation is a treasure trove of information, while community forums and learning platforms offer opportunities for hands-on practice and engagement with fellow learners. Embrace the journey of learning MATLAB step and enhance your technical expertise in system analysis!
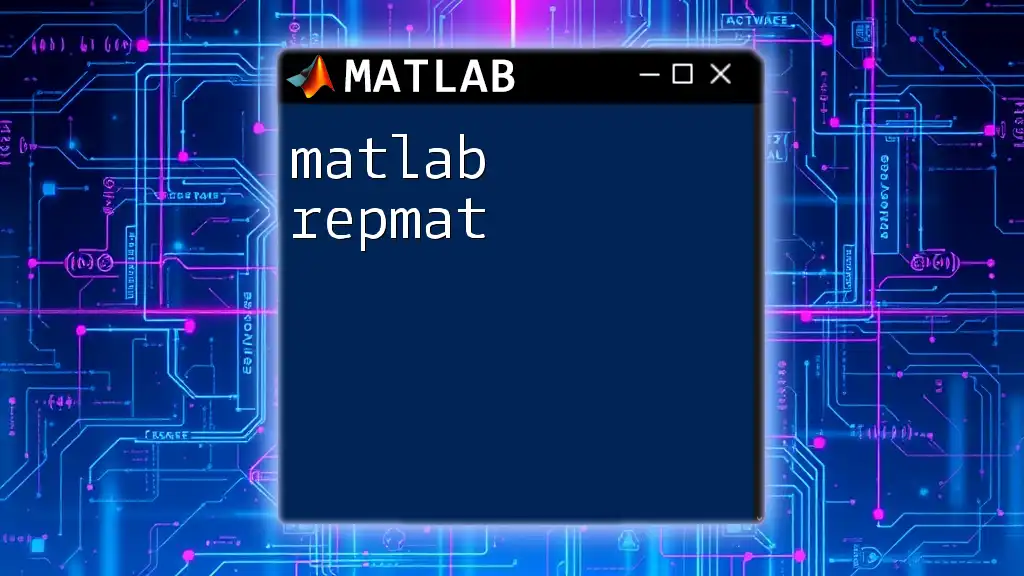
Additional Resources
Make use of useful MATLAB documentation and community forums to expand your knowledge further. Engaging in these resources can provide additional examples and support as you continue your MATLAB learning journey.