MATLAB and Python are both powerful programming languages for numerical computing, and transitioning from MATLAB to Python can be simplified by understanding the equivalent commands and syntax.
Here's a code snippet demonstrating a basic matrix operation in both languages:
% MATLAB code to create a 2x2 matrix and calculate its transpose
A = [1, 2; 3, 4];
B = A';
And the equivalent Python code using NumPy:
import numpy as np
# Python code to create a 2x2 matrix and calculate its transpose
A = np.array([[1, 2], [3, 4]])
B = A.T
Understanding the Basics
What is MATLAB?
MATLAB (Matrix Laboratory) is a high-level programming language and interactive environment primarily used for numerical computing, data analysis, algorithm development, and modeling. Its syntax and features are designed specifically for matrix operations, making it particularly powerful in fields such as engineering, physics, and financial analysis. MATLAB is equipped with various built-in functions that streamline complex mathematical calculations and visualizations.
What is Python?
Python is a versatile, high-level programming language known for its readability and simplicity. It supports multiple programming paradigms, including object-oriented, functional, and procedural programming. As one of the most popular languages in the world, Python finds applications in web development, data analysis, machine learning, automation, and beyond. Its rich ecosystem of libraries and frameworks enhances its capabilities, making it a favored choice among developers and researchers alike.
MATLAB vs. Python: A Comparative Overview
When transitioning from MATLAB to Python, one of the first aspects users must consider is the syntax. MATLAB uses a matrix-based approach, while Python utilizes standard programming concepts. Performance can vary between the two; Python, particularly with libraries like NumPy and SciPy, can be efficient for large datasets and complex computations. Additionally, the vast community support and contributing libraries in Python enhance its effectiveness for a wide range of applications.
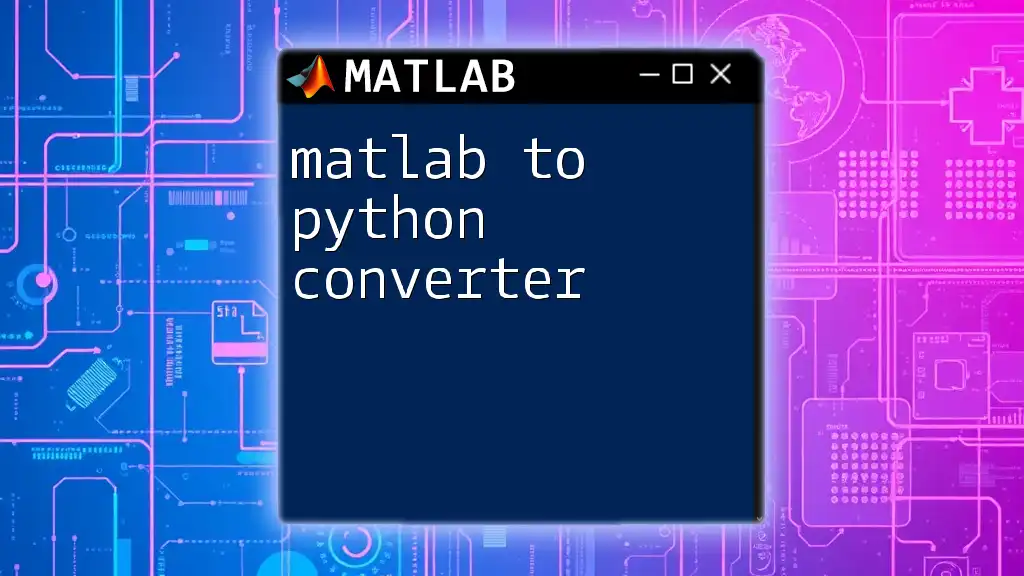
Setting Up Your Python Environment
Installing Python
To get started with Python, you first need to install it on your machine. The preferred method is downloading from the official Python website. After installing, it is advisable to choose an Integrated Development Environment (IDE) where you will write and run your code. Some popular IDEs are Jupyter Notebook, PyCharm, and VS Code.
Essential Python Libraries for MATLAB Users
To make the transition smooth and maximize Python's capability, familiarity with essential libraries is critical. Key libraries include:
- NumPy: This library supports large, multi-dimensional arrays and matrices and is fundamental for numerical computations, similar to MATLAB's array operations.
- SciPy: Built on NumPy, SciPy offers additional modules for optimization, integration, and statistics, paralleling MATLAB’s built-in functions.
- Matplotlib: For data visualization, Matplotlib allows you to create static, animated, and interactive visualizations, akin to MATLAB's plotting capabilities.
- Pandas: This library provides data manipulation and analysis tools that allow for easy handling of data structures, such as DataFrames, similar to MATLAB tables.
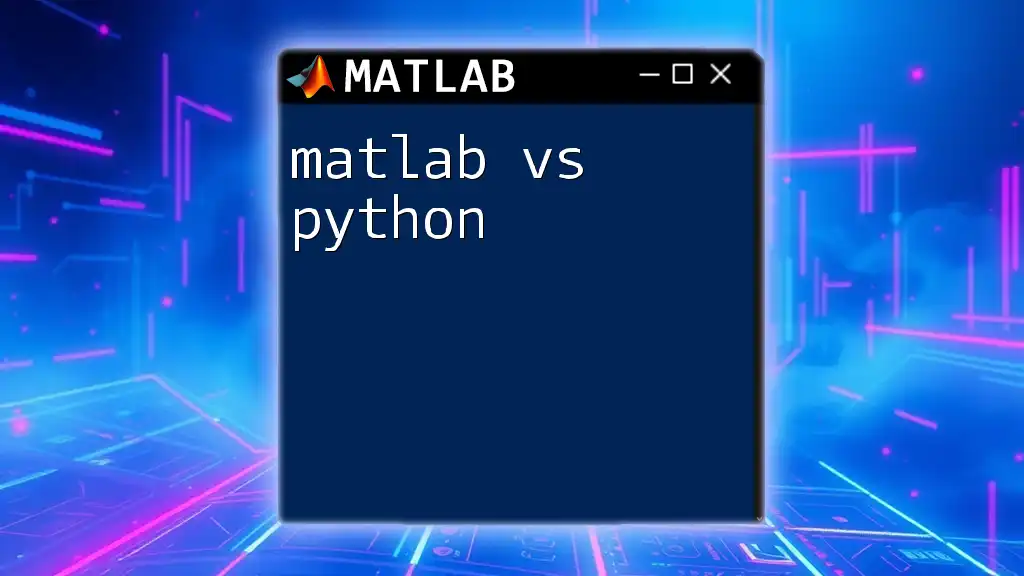
Syntax and Command Comparisons
Basic Syntax Differences
One of the initial hurdles for MATLAB users is adapting to Python's syntax. For example, variable assignment is done in the following ways:
In Python:
a = 5
In MATLAB:
a = 5;
Data types also differ, with Python emphasizing dynamic typing while MATLAB is more rigid due to its matrix focus.
Control Structures
Conditional Statements
Conditional statements are crucial in programming. Here’s how they vary:
Python example:
if a > b:
print("A is greater")
MATLAB example:
if A > B
disp('A is greater')
end
Loops
Loops allow repetition in programming. The syntax for `for` loops exhibits notable differences:
For loops in Python:
for i in range(5):
print(i)
In MATLAB:
for i = 1:5
disp(i)
end
While Loops
Both languages offer while loops, enabling the execution of code as long as a specific condition is met. The syntax is similar but varies in structure.
Functions
Defining functions in Python also showcases the differences between the two languages. A simple function in Python looks like this:
def my_function(x):
return x + 1
In MATLAB, the same function would be defined as:
function y = my_function(x)
y = x + 1;
end
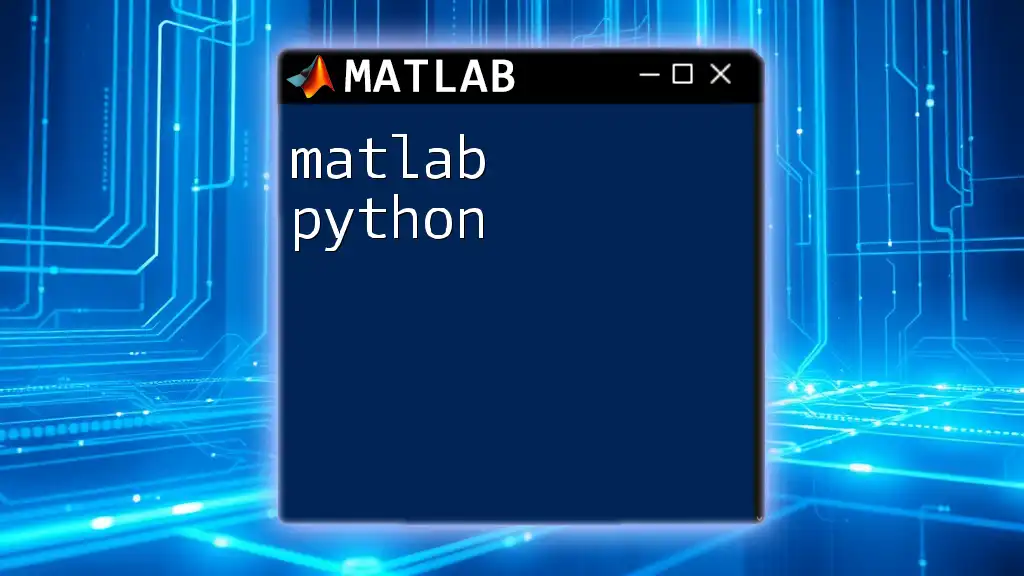
Data Structures
Arrays and Matrices
MATLAB efficiently handles arrays and matrices, but Python's NumPy library offers similar functionality. To create a multi-dimensional array in Python, an example is as follows:
import numpy as np
a = np.array([[1, 2], [3, 4]])
In MATLAB, you would define it like this:
A = [1, 2; 3, 4];
Lists, Tuples, and Dictionaries
Python offers versatile data structures such as lists, tuples, and dictionaries. Lists can be defined as follows:
my_list = [1, 2, 3]
Dictionaries in Python resemble MATLAB's structures:
my_dict = {'key': 'value'}
In MATLAB, you can create cell arrays or structures to achieve a similar effect:
myCell = {1, 2, 3}; % for lists
myStruct.key = 'value'; % for dictionaries
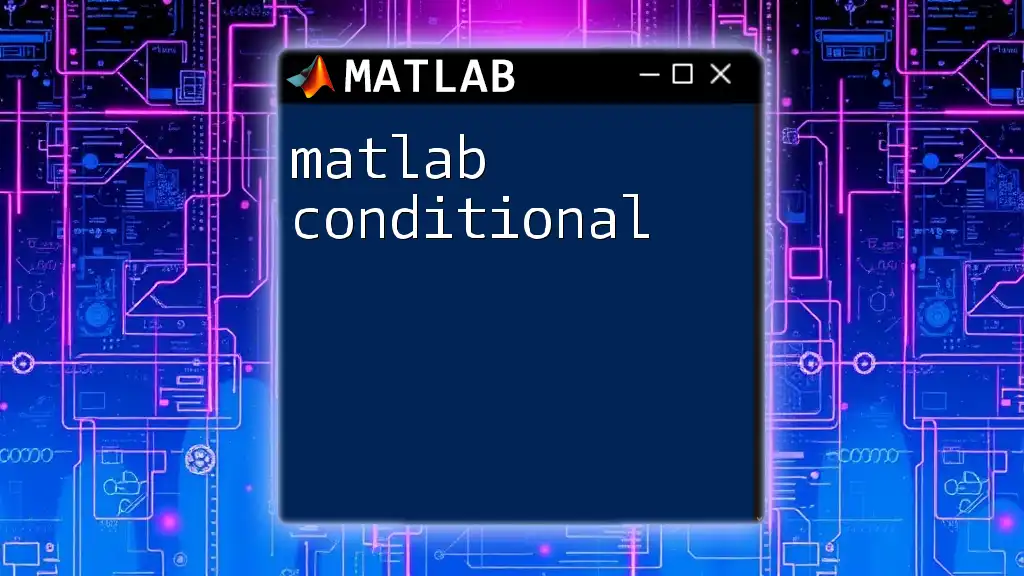
Plotting and Data Visualization
Basics of Plotting in MATLAB
MATLAB excels in its built-in functions for plotting. A simple 2D plot in MATLAB can be created with the following command:
plot(x, y);
Transitioning to Python Visualization
In Python, Matplotlib serves as the primary plotting library. The syntax to generate a basic plot would look like this:
import matplotlib.pyplot as plt
plt.plot(x, y)
plt.show()
This transition to Python maintains the ease of visualizing data while expanding upon the options available for customization and interactivity.
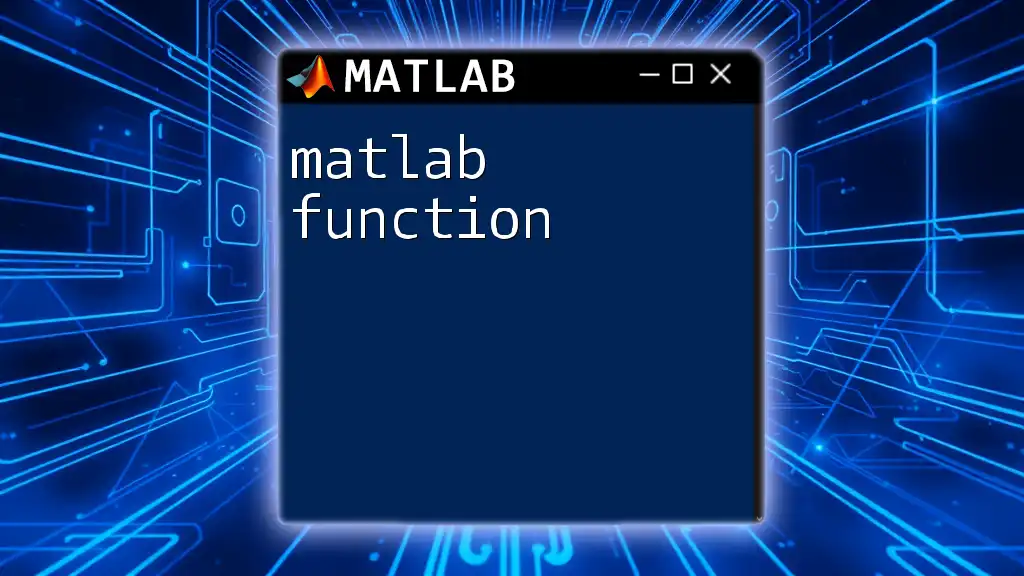
Best Practices for Code Transition
Organizing Your Code
As you begin writing in Python, the organization of code becomes vital. Using clear function definitions and separating code into modules will help maintain clarity and reusability.
Commenting and Documentation
Proper documentation is essential in programming. Python encourages extensive commenting using the `#` symbol, which aids in code maintainability. Adhering to best practices for documentation will result in better comprehension by yourself and others.
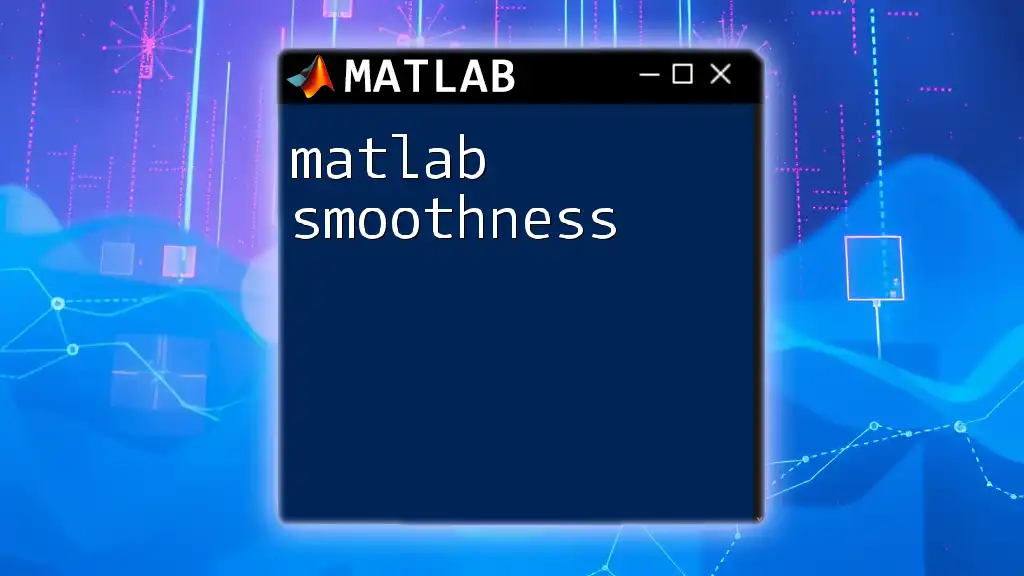
Resources for Continued Learning
Online Courses and Tutorials
Several online learning platforms offer courses specifically designed for transitioning from MATLAB to Python. These courses can provide structured, in-depth knowledge tailored to your learning pace.
Books and eBooks
Finding comprehensive research or instructional books dedicated to MATLAB and Python will support your continued growth. Titles focusing on data science, machine learning, or scientific computing may bridge the gap between the two languages.
Community and Support Forums
Engaging with Python communities is invaluable as you learn. Platforms like Stack Overflow and GitHub host discussions and documentation that can clarify doubts and inspire new ideas, aiding you as you transition from MATLAB to Python.
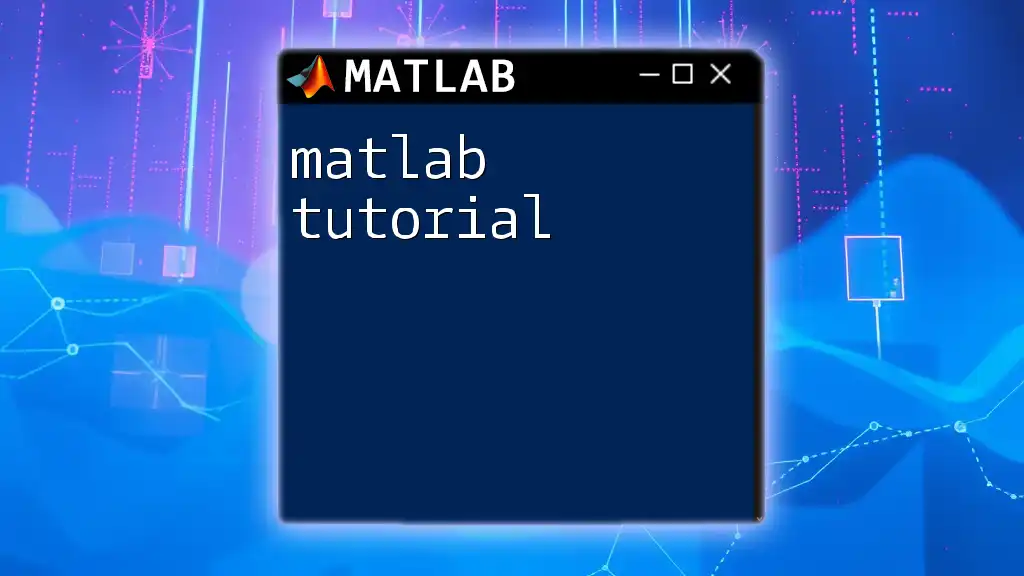
Conclusion
Transitioning from MATLAB to Python represents a significant step that can enhance your programming capabilities and versatility. By understanding the fundamental differences, learning how to use essential libraries, and improving your coding skills, you become well-equipped for a seamless transition and can leverage Python's broader application in technology and data science. Embrace the journey and dive into the world of Python, enriched with new opportunities for innovation and growth.