In MATLAB, you can use the `pollabledataqueue` to efficiently send and receive data between parallel workers by allowing workers to poll for available data asynchronously.
Here’s a simple example:
% Create a pollable data queue
pdq = parallel.pool.PollableDataQueue;
% Create a parfeval task that sends data to the queue
f = parfeval(@myFunction, 0, pdq);
% Function to send data to the queue
function myFunction(queue)
for k = 1:5
pause(1); % Simulate some work
send(queue, k); % Send data to the queue
end
end
% Retrieve available data from the queue
while true
data = poll(pdq); % Poll for available data
if isempty(data), break; end % Exit if no data
fprintf('Received: %d\n', data); % Process received data
end
Understanding Pollable Data Queues
What is a `pollabledataqueue`?
A `pollabledataqueue` is a specialized object in MATLAB that facilitates the efficient exchange of data between workers in a parallel computing environment. This allows for real-time communication where data can be sent and retrieved as needed, making it particularly useful for tasks requiring collaboration between multiple processing threads.
Why Use `pollabledataqueue`?
Using `pollabledataqueue` is crucial for enhancing the performance of parallel computations. Here are several key benefits of incorporating this function:
- Efficient communication: It minimizes the overhead commonly associated with inter-worker communication, ensuring smooth data transfer.
- Reduced latency: Polling data in real-time helps decrease wait time, which can significantly speed up computations.
- Dynamic data handling: Unlike static queuing systems, `pollabledataqueue` allows for a more flexible approach in data retrieval, where workers can actively listen and process incoming data.
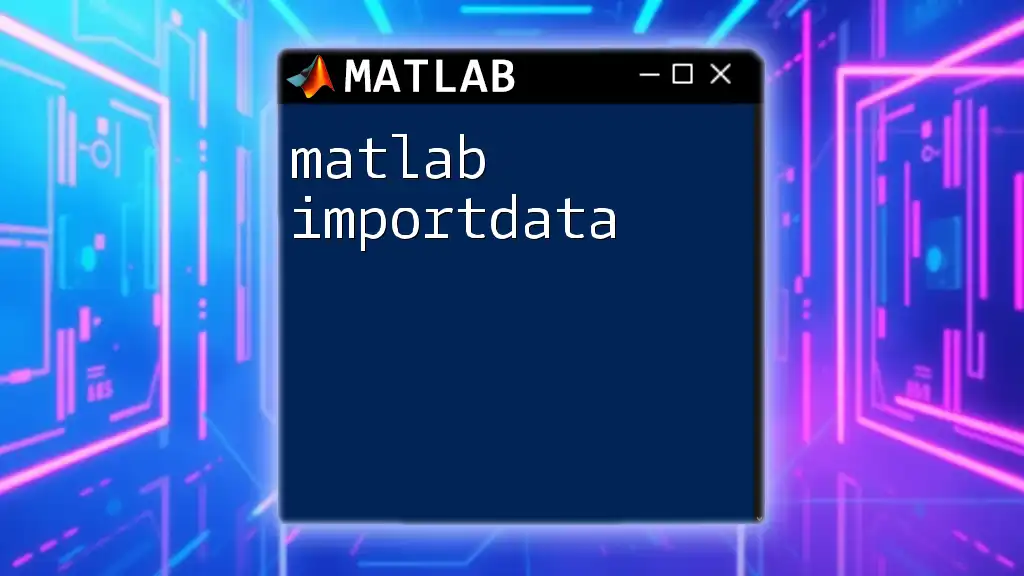
Basic Concepts of Parallel Computing in MATLAB
Introduction to Parallel Computing
Parallel computing leverages multiple processors to perform simultaneous tasks, thereby accelerating the computational process. In MATLAB, each worker acts as an independent processor, executing computations in parallel. Understanding the dynamics of these workers is essential for optimizing performance using `pollabledataqueue`.
Setting Up the Parallel Environment
Before utilizing the `pollabledataqueue`, you must initiate a parallel environment using MATLAB's `parpool` function. This command creates a pool of workers to handle computational tasks.
For example, creating a pool of four workers can be accomplished with:
parpool('local', 4); % Start a parallel pool with 4 workers
This command sets the stage for data to be exchanged using the `pollabledataqueue`.
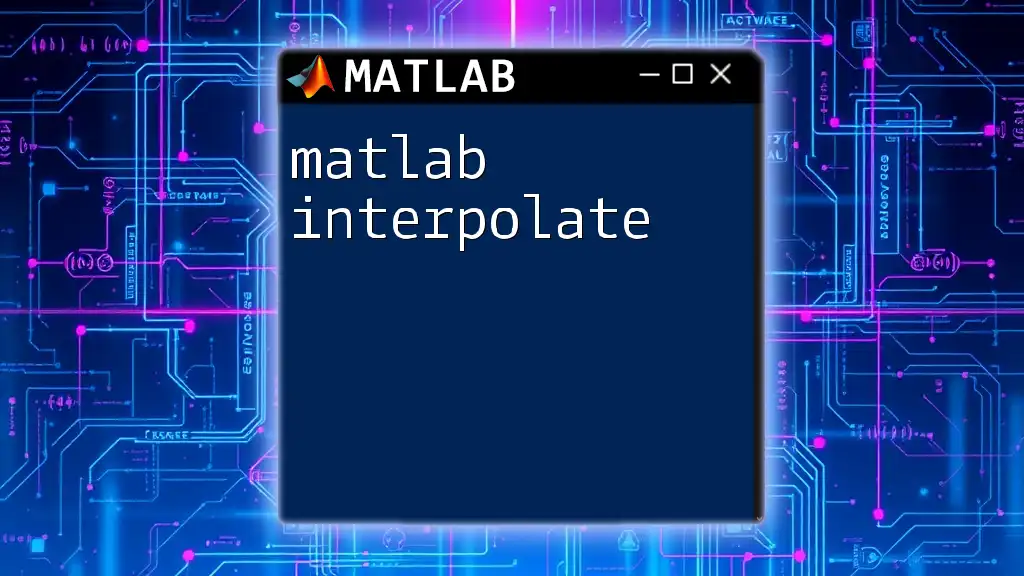
Using `pollabledataqueue` for Inter-Worker Communication
Creating a Pollable Data Queue
To begin utilizing `pollabledataqueue`, you first need to create a `pollabledataqueue` object. This can be easily done within your parallel computations by running:
pdq = parallel.pool.PollableDataQueue;
This object will serve as the channel through which data is sent and retrieved.
Sending Data from Workers
Once the `pollabledataqueue` is established, each worker can send data to it. The `send` method is used for this purpose, enabling workers to push data into the queue.
For example, within an `spmd` block, you can send each worker’s index to the main thread like this:
spmd % Single Program Multiple Data
pdq.send(labindex); % Sending the lab index
end
In this code, each worker sends its unique identifier, which will later be useful for tracing computations.
Receiving Data in the Primary Worker
To retrieve data sent from the workers, you will use the `poll` method. This method allows the primary worker to check the queue for incoming data without blocking other processes.
Here's how to implement it:
while true
data = pdq.poll(); % Polling for data
if isempty(data)
break; % Exit loop if no more data
end
% Process the received data
end
In this loop, the primary worker continuously checks the queue for new data. If no data is available, it exits the loop, ensuring that resources are managed efficiently.
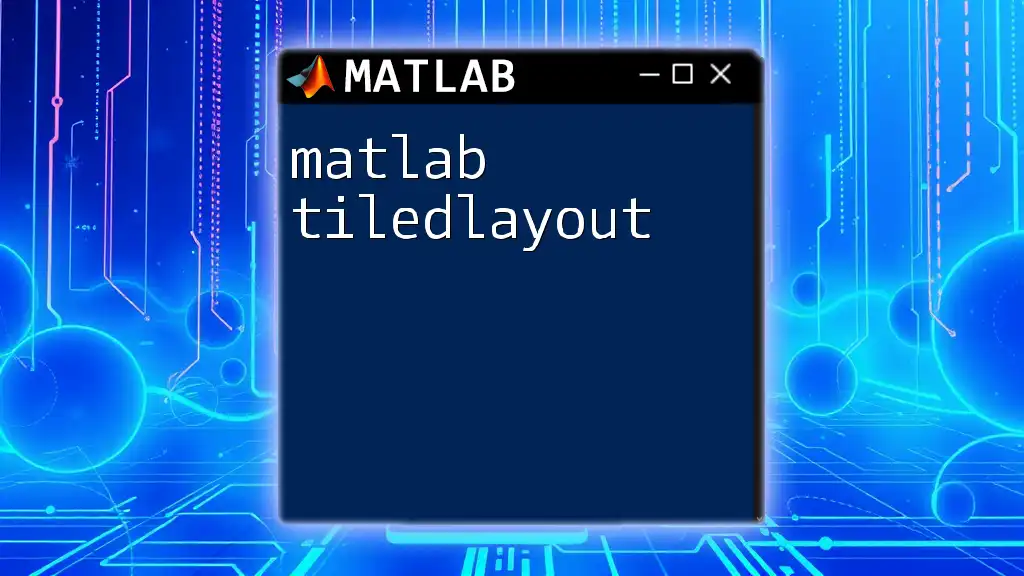
Example Application of `pollabledataqueue`
A Simple Use Case: Data Collection from Multiple Workers
Consider an example where multiple workers need to perform calculations and send their results back to the main worker for aggregation. Let’s say each worker is tasked with computing the square of its index.
The overall structure of this program might look like:
results = cell(1, numlabs); % Pre-allocate results
spmd
data = labindex^2; % Each worker does some calculations
pdq.send(data); % Sending the result
end
% Collecting results in one worker
while true
new_data = pdq.poll();
if isempty(new_data)
break;
end
results{end+1} = new_data; % Store the received data
end
In this example, each worker sends its computed square back to the main thread, which collects and stores these results in a cell array.
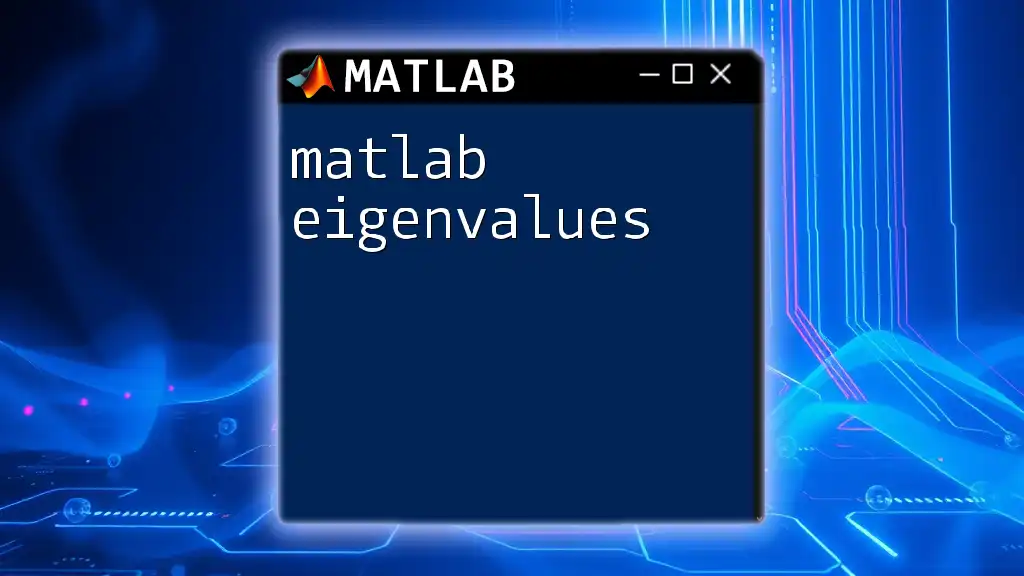
Best Practices for Using `pollabledataqueue`
Tips for Efficient Data Handling
To maximize the benefits of using `pollabledataqueue`, consider these practices:
- Limit data size: Smaller data packets are quicker to transmit. This helps maintain a fast-paced communication loop.
- Avoid frequent polling: Excessive polling can lead to increased CPU usage and reduced overall performance. Implementing a timely polling strategy can help strike the right balance.
Common Pitfalls to Avoid
While utilizing `pollabledataqueue`, it is important to be aware of potential pitfalls. Two common issues include:
- Blocking the main thread: If the main worker is busy waiting for data, it may slow down the overall process. Proper use of `poll` can mitigate this.
- Handling exceptions in worker tasks: Always ensure that error handling is implemented correctly to manage potential exceptions thrown by workers.
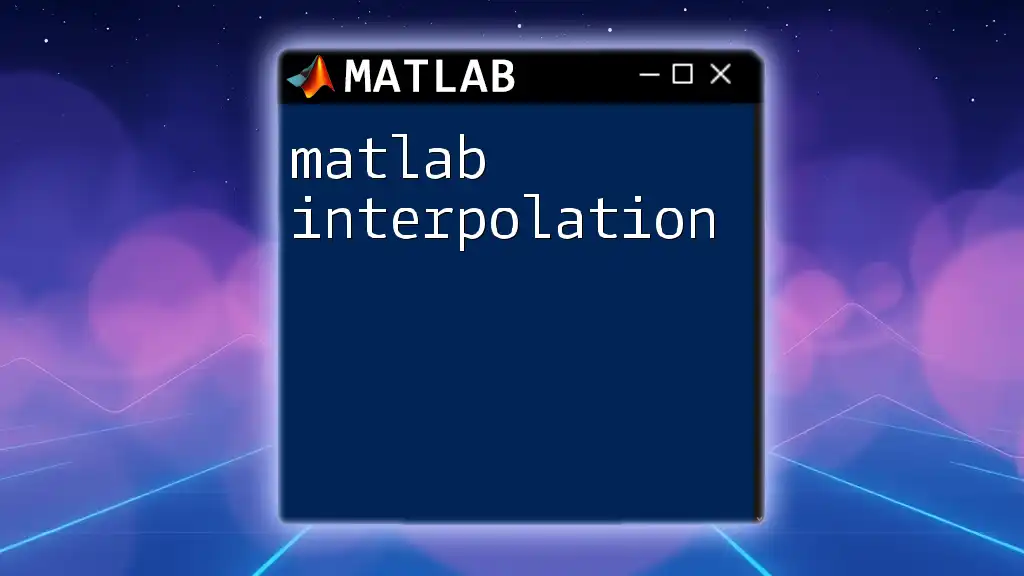
Conclusion
Utilizing MATLAB using `pollabledataqueue` to send data between workers provides a powerful approach to efficient parallel computing. By understanding how to set up and leverage this tool, you can significantly enhance the performance of your computation-heavy applications. Whether for collecting data or managing worker communications, `pollabledataqueue` is essential for anyone looking to optimize parallel computing workflows in MATLAB.
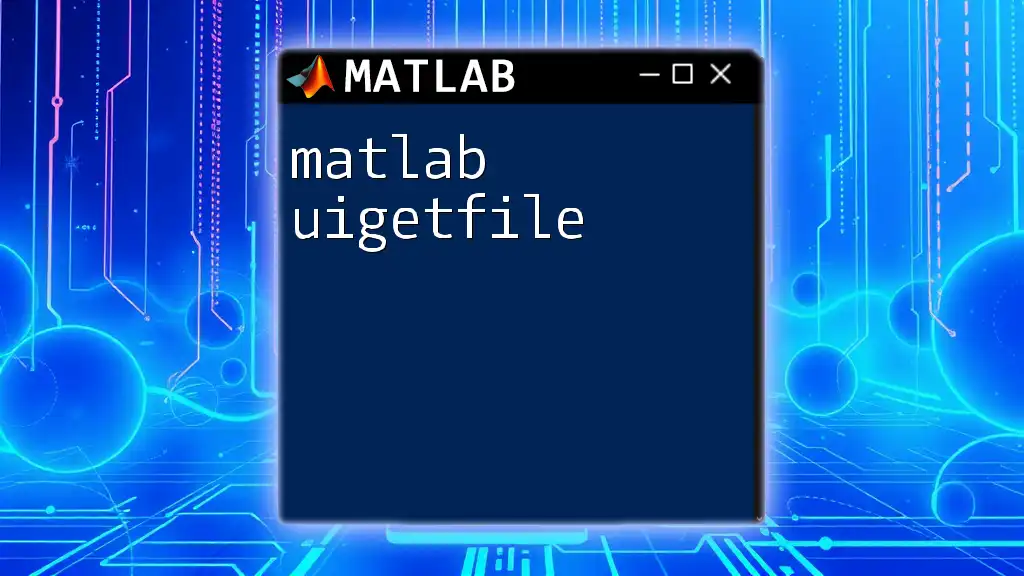
Additional Resources
To deepen your understanding of this topic, explore the official MATLAB documentation on [`pollabledataqueue`](https://www.mathworks.com/help/parallel/ref/parallel.pool.pollabledatacontainer.poll.html) and consider reviewing related tutorials on parallel programming in MATLAB for practical applications and advanced techniques.