In MATLAB, you can factorize a rank 1 matrix by decomposing it into the outer product of two vectors, which can be efficiently computed using the `outer` function.
A = a * b'; % where 'a' and 'b' are column vectors
Understanding Rank 1 Matrices
What is a Rank?
The rank of a matrix is a fundamental concept in linear algebra that indicates the dimension of the vector space generated by its rows or columns. In simpler terms, it tells us the maximum number of linearly independent vectors in a matrix. For a matrix to be considered a rank 1 matrix, it means there is exactly one linearly independent row or column.
Mathematical Representation: A matrix A has rank 1 if it can be expressed in the form:
\[ A = \mathbf{u} \mathbf{v}^T \]
where \(\mathbf{u}\) and \(\mathbf{v}\) are non-zero vectors.
Characteristics of Rank 1 Matrices
Rank 1 matrices have several distinctive properties:
- Linearity: Any matrix with a rank of 1 can be formed by the outer product of two vectors.
- Determinant: Any square matrix with a rank less than its dimension has a determinant of zero.
- Singular Values: For a rank 1 matrix, only one singular value is non-zero, while the others are zero.
Example: Consider the matrix:
\[ A = \begin{pmatrix} 1 & 2 \\ 3 & 6 \end{pmatrix} \]
Here, the second row is a scalar multiple of the first row, which confirms that the rank is 1.
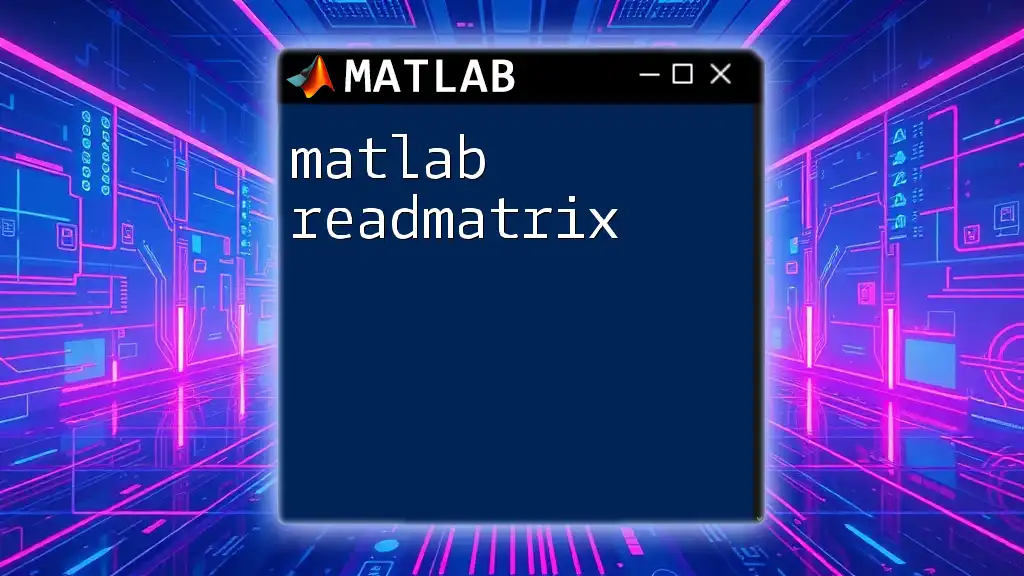
Basics of Matrix Factorization
What is Matrix Factorization?
Matrix factorization is a method to decompose a matrix into products of matrices, which can simplify various matrix computations. It is particularly crucial in applications such as dimensionality reduction, collaborative filtering, and image compression.
Applications of Matrix Factorization
Common applications include:
- Latent Semantic Analysis: For understanding relationships between documents.
- Collaborative Filtering: In recommendation systems (e.g., Netflix, Amazon).
- Image Processing: Reducing storage while keeping essential features.
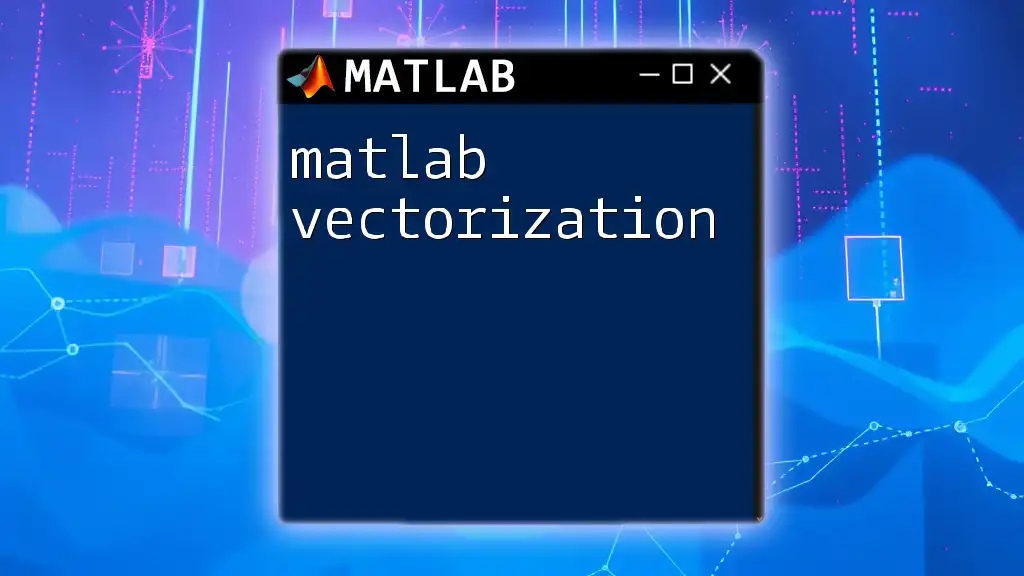
Factorizing a Rank 1 Matrix in MATLAB
Syntax and Functions
To factorize a rank 1 matrix in MATLAB, you can use several built-in functions such as:
- `rank()`: Determines the rank of a matrix.
- `svd()`: Computes the singular value decomposition.
- `eig()`: Finds the eigenvalues and eigenvectors.
Step-by-Step Guide to Factorization
Example of Creating a Rank 1 Matrix
You can create a rank 1 matrix easily in MATLAB. Here’s a simple code snippet:
% Example to create a Rank 1 matrix
A = [1; 2] * [3, 4]; % Resulting in a rank 1 matrix
This code generates the following matrix A:
\[ \begin{pmatrix} 3 & 4 \\ 6 & 8 \end{pmatrix} \]
Performing Factorization on the Rank 1 Matrix
To factorize this rank 1 matrix, you can use Singular Value Decomposition (SVD):
[U, S, V] = svd(A); % Factorization using SVD
In this context:
- U is a matrix where each column represents a left singular vector.
- S is a diagonal matrix containing singular values.
- V is a matrix composed of right singular vectors.
In the case of a rank 1 matrix, the S matrix will have only one non-zero entry, representing the only singular value, while the remaining entries will be zero.
Visual Representation of the Factorization
A rank 1 matrix can be visualized as a linear transformation that scales a single vector in space. The matrices U and V facilitate this transformation, dictating its orientation and scale, respectively.
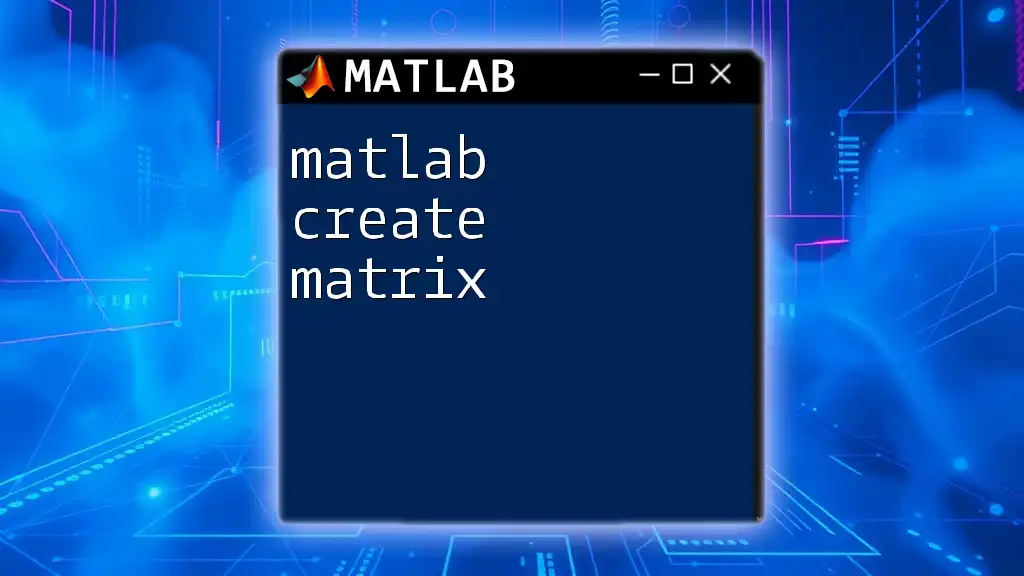
Practical Applications of Rank 1 Matrix Factorization
Case Studies
An excellent illustration of the value of rank 1 matrix factorization is in collaborative filtering. For instance, platforms like Spotify leverage matrices of user-item interactions. By factorizing these matrices into rank 1 components, they can effectively recommend new music based on users' previous preferences.
Analyzing Data with Rank 1 Matrices
When analyzing data, factorization can reveal patterns. For instance, if a dataset has been factorized into matrices U and V, the product of these matrices can help reconstruct the approximated original matrix, highlighting relationships between items or users in a recommendatory context.
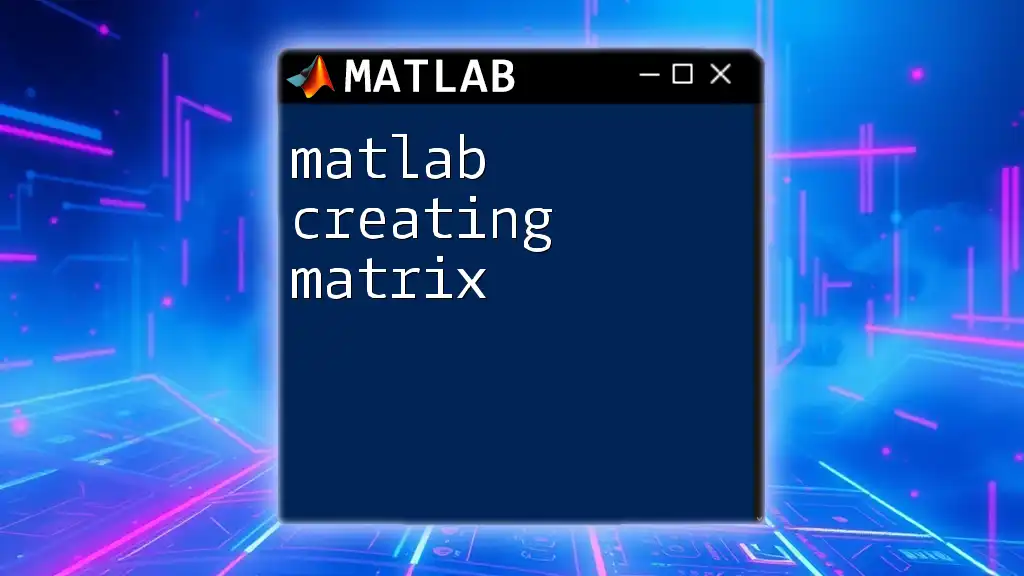
Troubleshooting Common Issues
Common Errors in MATLAB
While working with matrix factorization in MATLAB, you might encounter typical issues such as:
- Confusion regarding matrix dimensions.
- Misinterpretation of the factorization results.
Debugging Tips
When debugging problems:
- Always check the dimensions of the matrices involved using the `size()` function.
- Use `rank(A)` to ensure your original matrix is indeed a rank 1 matrix before attempting factorization.
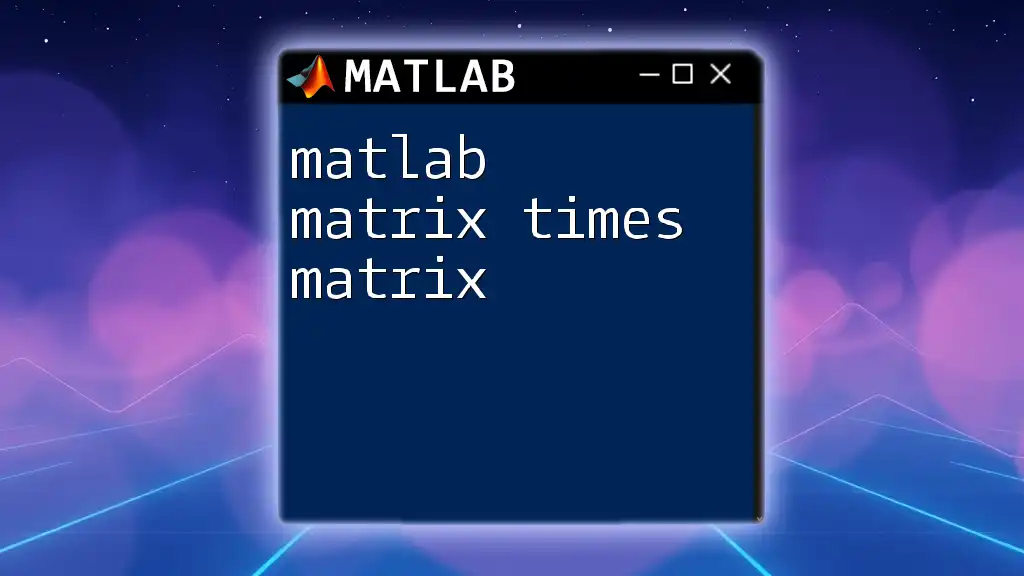
Conclusion
Understanding how to MATLAB factorize rank 1 matrix is a vital skill for anyone working in data science or engineering. By mastering both the theoretical aspects and practical implementations, you can explore vast applications from recommendation systems to advanced image processing.
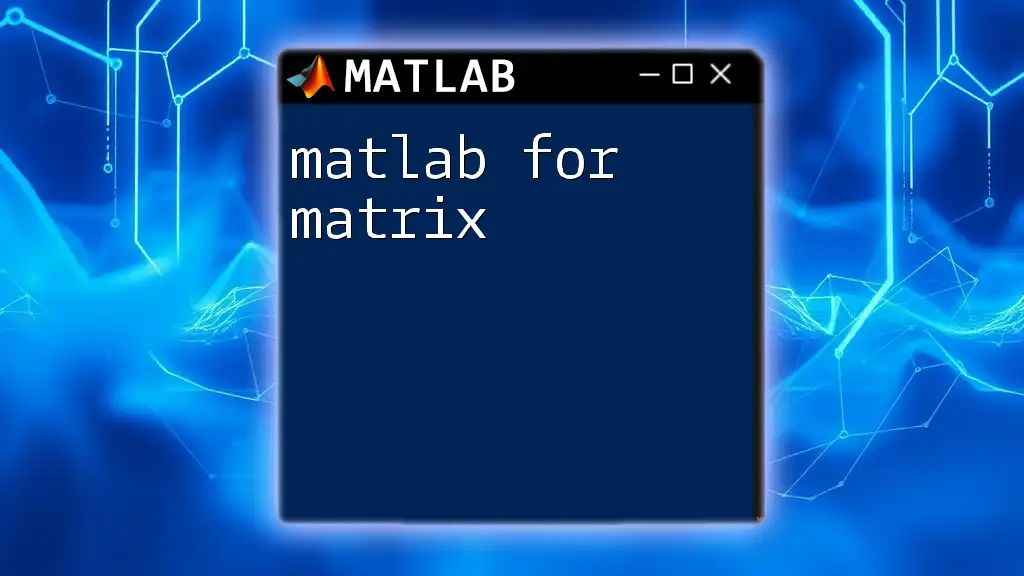
Additional Resources
References
You might want to consider some textbooks on linear algebra or machine learning to gain a deeper understanding.
MATLAB Documentation
The official MATLAB documentation offers comprehensive details on matrix operations and functions that can enhance your learning experience.
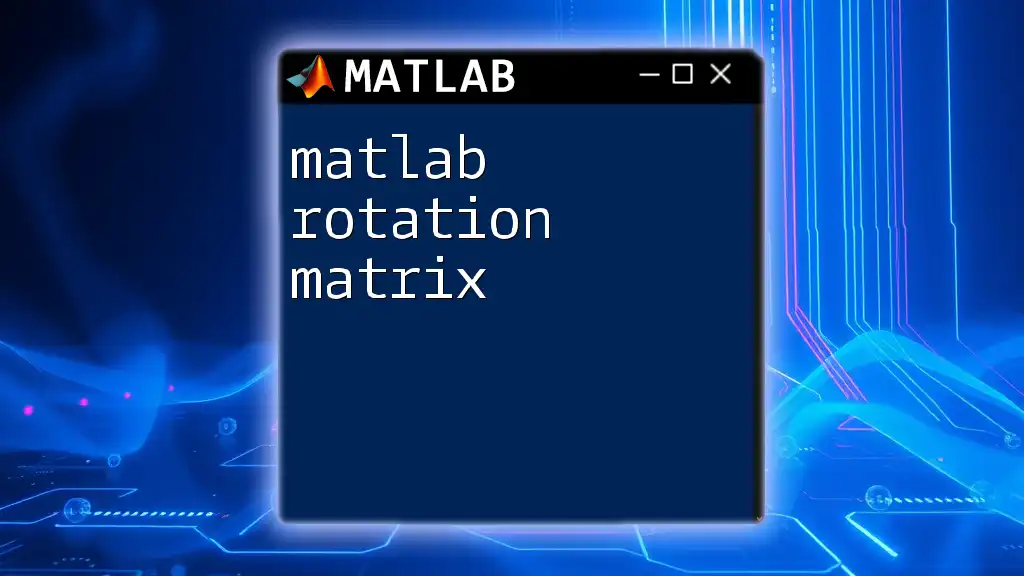
Call to Action
Now that you've acquired the fundamentals of factorizing a rank 1 matrix in MATLAB, put your knowledge to the test! Try implementing the examples provided and explore further capabilities within MATLAB. Additionally, consider signing up for advanced MATLAB courses to deepen your skills further!