In MATLAB, errors occur when the code fails to execute as intended, often due to syntax mistakes, undefined variables, or incompatible data types.
Here’s an example of a common error when trying to use an undefined variable:
disp(myVariable)
In this case, if `myVariable` has not been defined earlier in the code, MATLAB will throw an error.
Understanding MATLAB Errors
What is a MATLAB Error?
A MATLAB error occurs when the interpreter encounters an issue that prevents the execution of the script or function. Understanding errors is essential for effective programming, as they can significantly affect performance and data integrity. By learning how to handle and fix these errors, you enhance your coding skills and increase the reliability of your MATLAB applications.
Types of Errors in MATLAB
Errors in MATLAB can be categorized into three main types:
Syntax Errors
Syntax errors arise when the code violates the language's grammatical rules. They are often the easiest types of errors to fix. Common causes include missing semicolons, incorrectly placed operators, or unmatched parentheses.
For example, consider the following incorrect code:
result = 3 + * 2; % Incorrect use of operators
This code will throw a syntax error because of the incorrect placement of the multiplication operator.
Runtime Errors
Runtime errors occur during the execution of the code, often due to logical issues or unexpected conditions. These errors can be tricky as the code may compile correctly, but fail under certain conditions.
A common runtime error example is:
a = 1;
b = 0;
result = a / b; % Division by zero
This code will result in a runtime error, specifically a division by zero, which MATLAB cannot handle because it produces an undefined result.
Logical Errors
Logical errors do not produce any error messages but lead to incorrect outcomes. Identifying these errors can be challenging, as the code runs without interruption but may yield wrong results.
For example:
x = 10;
y = 5;
result = x - y; % Intended to find the sum, but does subtraction instead
In this snippet, the intent was to sum `x` and `y`, yet the subtraction leads to an incorrect outcome.
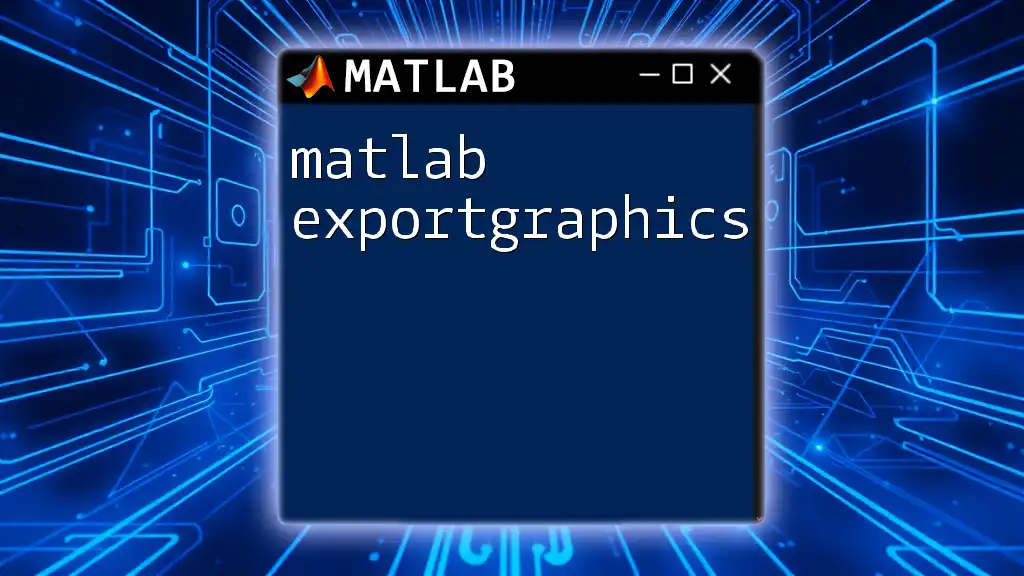
Common MATLAB Errors and Their Solutions
Syntax Error Examples
Common syntax errors include mismatches in parentheses, incorrect variable names, and unclosed strings. To resolve such errors, always review your code for any typos or grammatical inconsistencies before running it. Using MATLAB’s built-in debugging tools can also help locate syntax issues.
Runtime Error Examples
Runtime errors can often be resolved by ensuring that the inputs to functions are valid and that operations are performed under safe conditions. Always check variables before operations, and consider using assert statements to confirm valid conditions.
For instance:
assert(b ~= 0, 'Denominator must be non-zero'); % Prevents division by zero
result = a / b;
Logical Error Examples
Identifying logical errors requires careful examination of the code and possibly using print statements or breakpoints to validate the outputs at each step. Refactoring the code to ensure clarity can also help in preemptively avoiding such errors.
In the earlier example, changing the subtraction to addition would fix the logical error:
result = x + y; % Corrected to perform addition
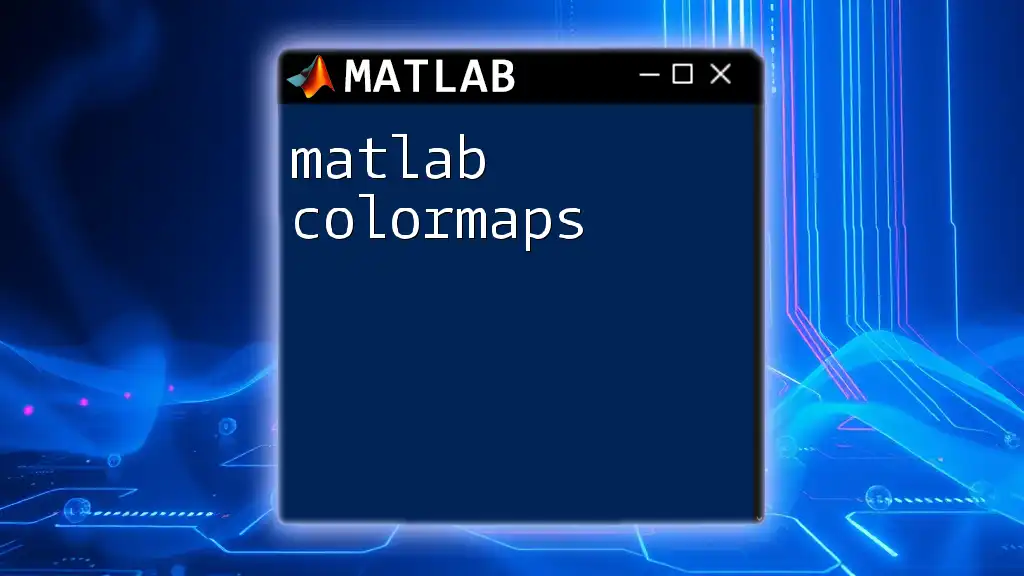
Error Messages in MATLAB
Understanding MATLAB Error Messages
MATLAB's error messages provide essential clues about what went wrong. They typically include the type of error, the location in the code where the error occurred, and a brief description of the issue.
A well-structured error message looks like this:
Error using ==> function_name
Input argument "x" must be a positive integer.
Reading error messages carefully helps you pinpoint issues effectively.
Decoding Common Error Codes
Familiarizing yourself with common error codes is crucial for fast troubleshooting. Each error code in MATLAB corresponds to specific problems, allowing you to quickly identify the root cause. For example, `Error using ==> index` might indicate issues with indexing arrays.
Resource Links for Error Codes
For a detailed understanding of MATLAB error codes, refer to its official documentation. Explore sections specifically designed for error handling and troubleshooting to enhance your knowledge.
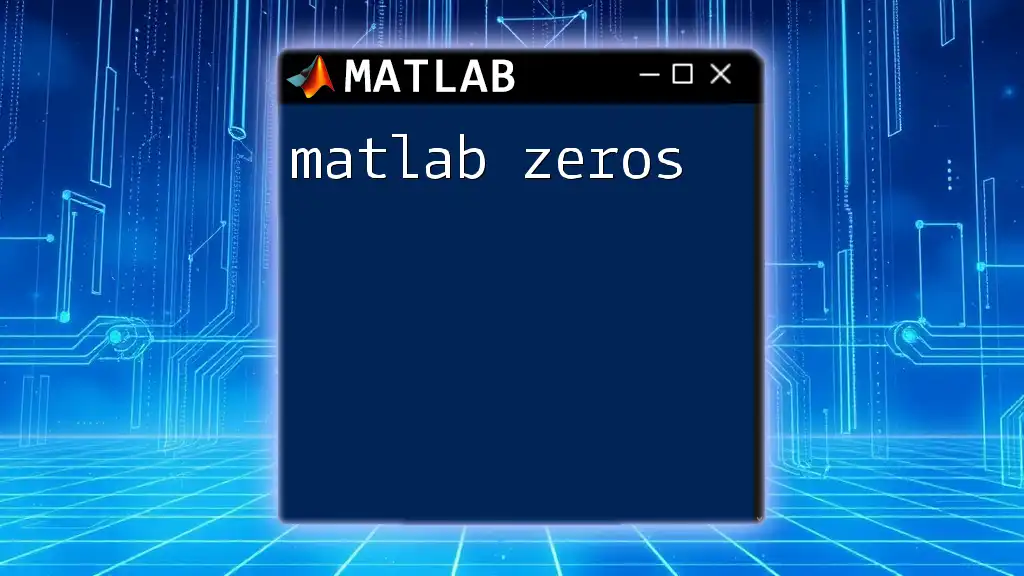
Debugging Errors in MATLAB
Using the MATLAB Debugger
The MATLAB debugger is a powerful tool for identifying and correcting errors in your code. You can set breakpoints, step through code line by line, and inspect variables at each point in execution. This process allows you to see where the code deviates from expected behavior.
Here’s a simple example of using the debugger effectively:
function result = faultyFunction(x)
result = x + 10;
pause; % Breakpoint for debugging
end
Insert breakpoints by clicking on the left margin in the editor and use the debug options to run the code interactively, observing variable states.
Tips for Debugging MATLAB Code
To streamline your debugging process, maintain a systematic approach. Break your code into smaller testable units, validate outputs at various stages, and utilize MATLAB’s toolboxes designed for error tracing. Always keep your code organized and well-commented, which aids in understanding and locating errors when they occur.
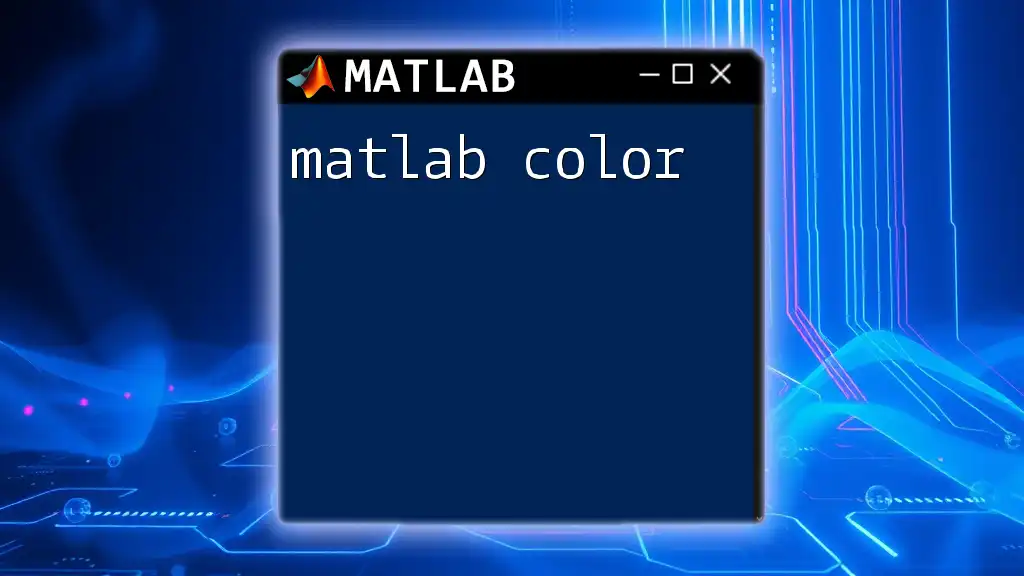
Error Handling Techniques
Using Try-Catch Blocks
Employing `try-catch` blocks in your code allows you to gracefully handle errors without halting execution. This approach can help maintain program stability and provide user-friendly messages when something goes wrong.
Here’s an example:
try
% Code that may cause an error
result = a / b;
catch ME
% Handle the error
disp('An error occurred: ');
disp(ME.message);
end
This structure captures any errors during the execution of the `try` block, allowing you to manage exceptions without a program crash.
Validating Input Data
Always validate input data to ensure it meets the required criteria before proceeding with computations. Input validation saves you from many runtime errors and enhances code reliability.
Basic validation might look like this:
function result = computeSquareRoot(x)
if x < 0
error('Input must be non-negative.');
end
result = sqrt(x);
end
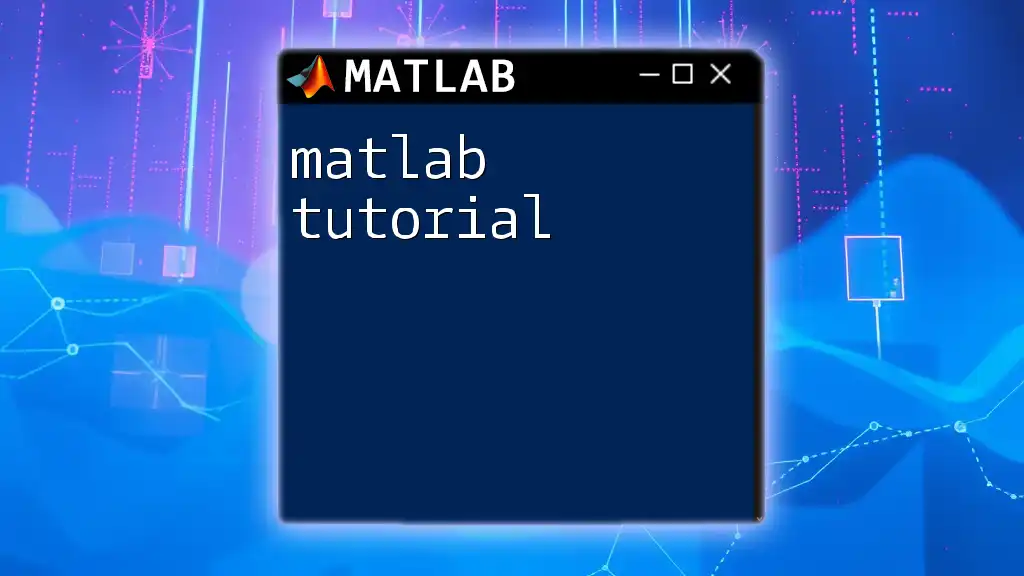
Best Practices for Minimizing Errors
Code Review Practices
Conducting regular code reviews is crucial for catching potential issues early. Utilize a checklist during these reviews to ensure adherence to coding standards and reinforce best practices.
Writing Clear and Concise Code
Clear, well-structured code is easier to read and maintain. Avoid complex logic where possible and use meaningful variable names. Keeping functions small and focused on a single purpose also decreases the likelihood of errors.
Continuous Learning and Resources
Stay updated with the latest MATLAB developments and best practices by engaging with books, online tutorials, and active forums. Resources like MATLAB’s official documentation and community forums can provide vital information for improving your coding expertise.
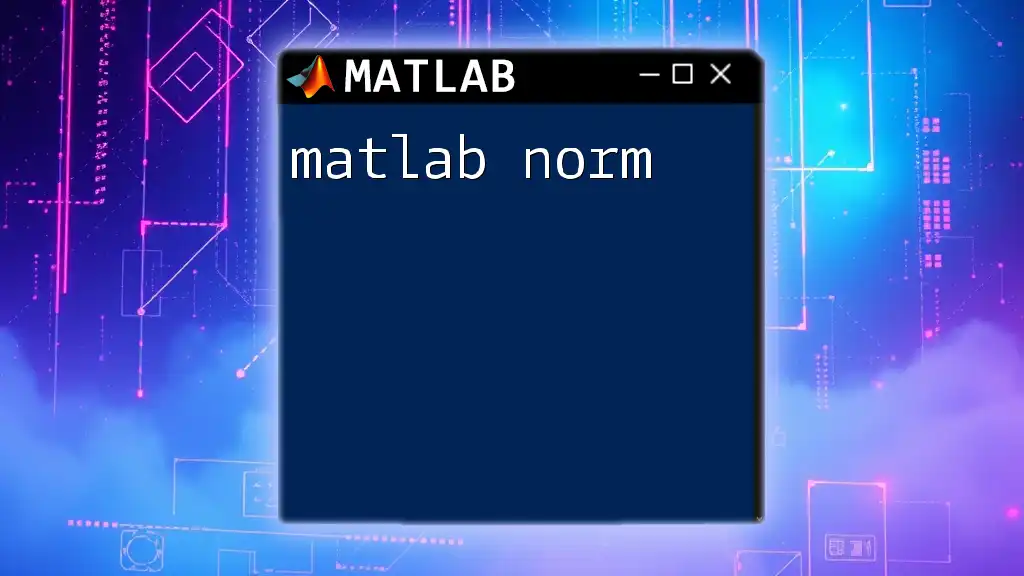
Conclusion
Understanding and handling MATLAB errors is a fundamental skill for anyone working with this powerful tool. By honing your error-handling skills, utilizing debugging techniques, and practicing best coding habits, you can develop resilient MATLAB programs. Keep learning and applying these concepts for continuous improvement and mastery in MATLAB programming.
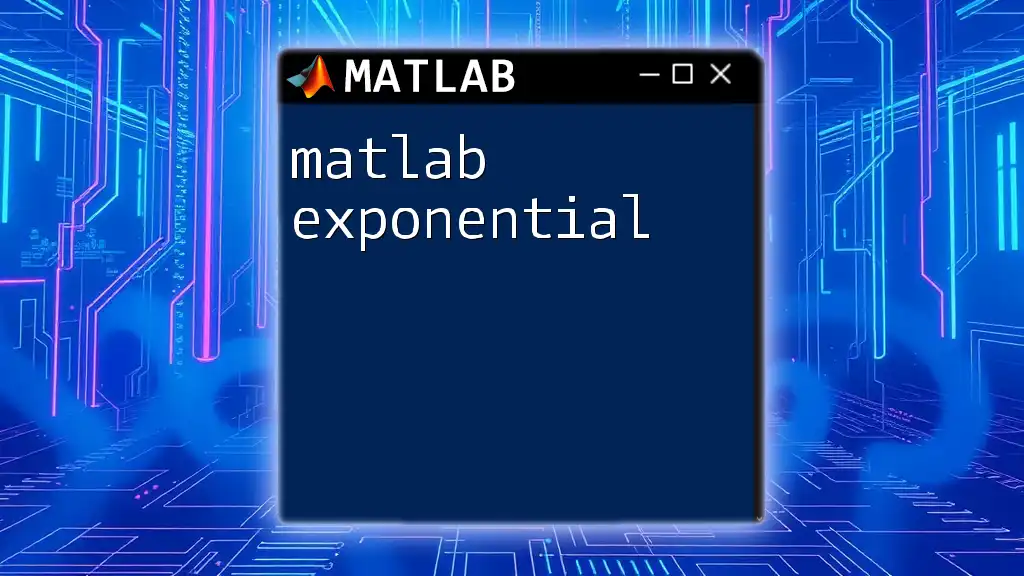
Additional Resources
For a more in-depth understanding, explore MATLAB’s official documentation and additional resources available through online tutorials, forums, and relevant literature. Engaging with the community and continuously practicing will significantly augment your MATLAB programming proficiency.