In MATLAB, the `while` loop repeatedly executes a group of statements as long as a specified condition remains true, allowing for dynamic control of flow based on variable values.
% Example of a while loop in MATLAB
count = 1;
while count <= 5
disp(['Count is: ' num2str(count)]);
count = count + 1;
end
Understanding the Syntax of `while` Loops in MATLAB
Basic Structure of a `while` Loop
A `while` loop in MATLAB is used for executing a block of code repeatedly as long as a specified condition evaluates to true. The general syntax for a `while` loop is as follows:
while condition
% code to execute
end
In the syntax above:
- Condition: An expression that evaluates to either true or false. As long as this condition is true, the code within the loop will continue to execute.
- Code block: This is the set of commands that you want to execute repeatedly.
Example of a Simple `while` Loop
To illustrate how a `while` loop works, consider the following example where we count from 1 to 5:
count = 1;
while count <= 5
disp(count);
count = count + 1;
end
In this code snippet:
- The loop starts with `count` initialized to 1.
- The condition checks if `count` is less than or equal to 5.
- If the condition is true, `disp(count)` will print the current value of `count`, and then `count` is incremented by 1.
- This process continues until `count` exceeds 5, at which point the loop terminates.
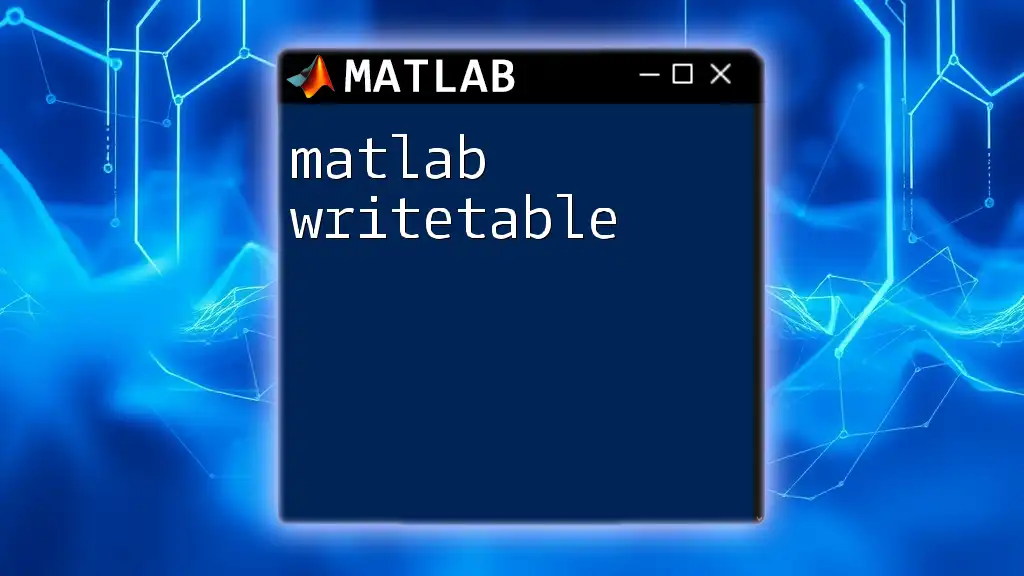
Practical Applications of `while` Loops
Use Cases Where `while` Loops Excel
`while` loops are particularly useful in scenarios where the exact number of iterations is not known at the start. This makes them ideal for:
- Data Processing: Looping through data until a specific condition is met, such as reaching the end of a file.
- User Input Validation: Continuously prompting users for correct inputs until valid input is received.
Example: User Input Validation
Consider a scenario where you want to validate user input, ensuring the user does not quit the input prompt unintentionally:
userInput = '';
while isempty(userInput)
userInput = input('Please enter a value: ', 's');
end
disp(['You entered: ', userInput]);
In this example:
- The `while` loop continues to run as long as `userInput` is empty.
- The user is prompted to enter a value. Once a valid input is entered, the loop terminates, and the input is displayed.
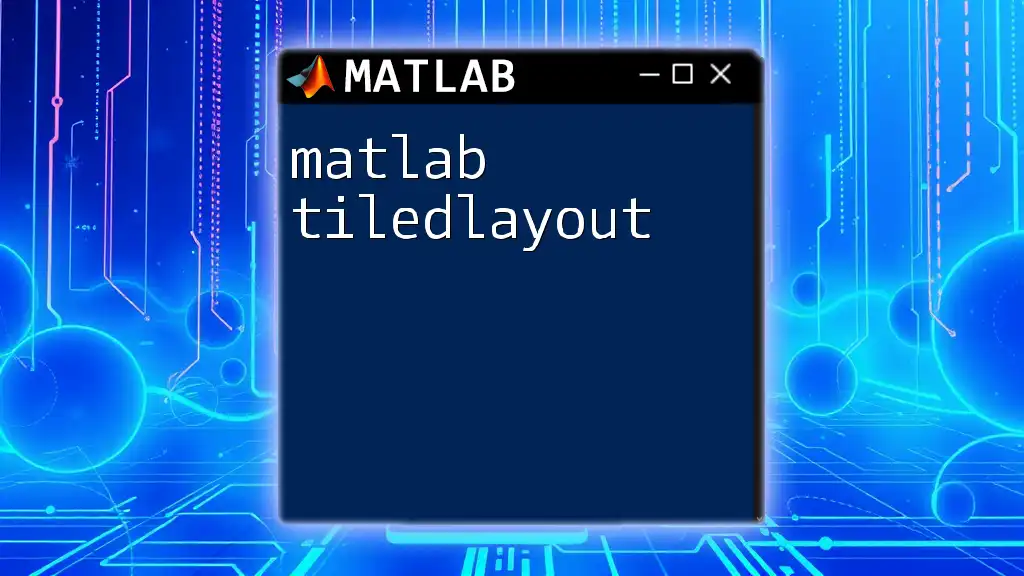
Nested `while` Loops in MATLAB
Introducing Nested Loops
Nesting `while` loops—placing one `while` loop inside another—can be an effective way to handle complex looping situations. This structure is beneficial for tasks that require multiple related iterations, such as processing multidimensional data.
Example of a Nested `while` Loop
Here is an example that generates a simple multiplication table:
row = 1;
while row <= 5
col = 1;
while col <= 5
fprintf('%d ', row * col);
col = col + 1;
end
fprintf('\n');
row = row + 1;
end
In this code:
- The outer loop handles each row of the multiplication table.
- The inner loop calculates each column within the current row.
- After each complete row is printed, a newline character is inserted to separate the rows visually.
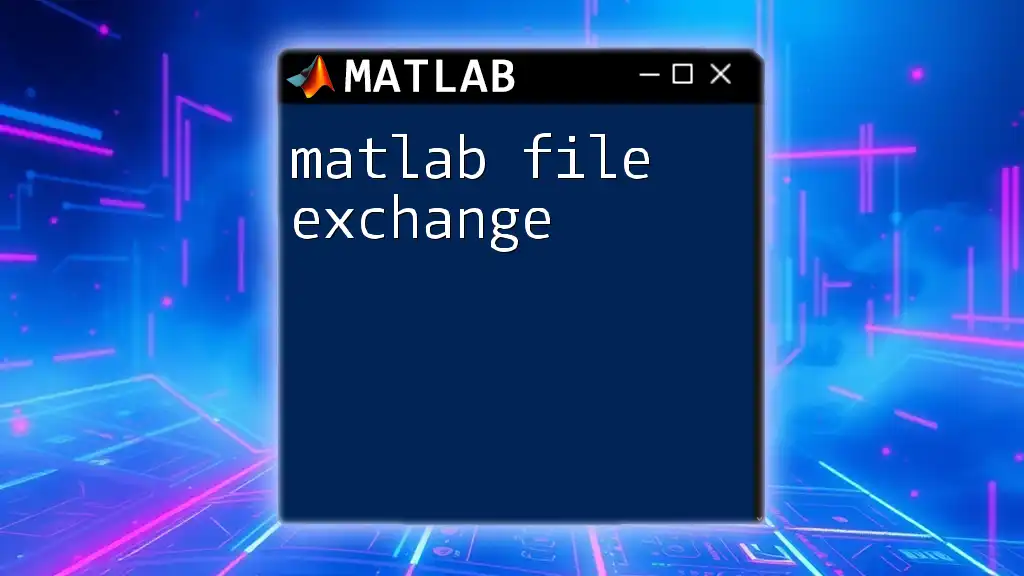
Common Pitfalls and Best Practices
Infinite Loops
A common issue faced when using `while` loops is the creation of infinite loops, where the condition never becomes false. This can happen if the loop's control variable is not updated appropriately.
Here’s a snippet demonstrating an infinite loop:
count = 1;
while count <= 5
disp(count);
% Some mistake that leads to infinite execution
end
In this case, if the line to increment `count` is accidentally omitted, the loop will run indefinitely. To avoid this, always ensure that the condition is modified within the loop to eventually terminate it.
Best Practices for Using `while` Loops
To write efficient `while` loops:
- Keep conditions clear and concise: A straightforward condition enhances readability and reduces logical errors.
- Consider alternative loop structures where feasible: Sometimes, a `for` loop may be more suitable if the number of iterations is known.
- Commenting and documenting code for readability: This is essential for complex loops, making it easier for others (or yourself in the future) to understand the logic.
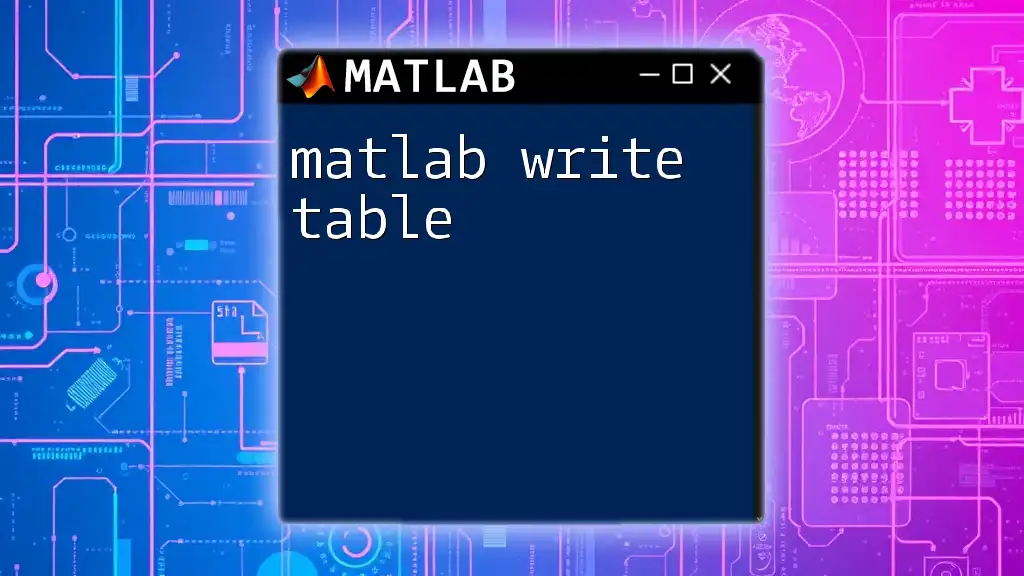
Debugging `while` Loops in MATLAB
Common Errors and Debugging Techniques
When dealing with `while` loops, you may encounter both logical errors (where the code runs without crashing but doesn't work as intended) and syntax errors (where the code fails to run due to incorrect syntax).
Example Debugging Scenario
Here's a snippet containing a logical error:
count = 0;
while count < 5
disp(count);
% Mistake: forgetting to increment count
end
In this example, the absence of an increment statement for `count` will lead to an infinite loop because the condition will always be true.
To debug effectively:
- Use breakpoints: Set breakpoints in your code to pause execution and inspect variable states.
- Check logical flow: Ensure that every loop condition has a clear path to false.
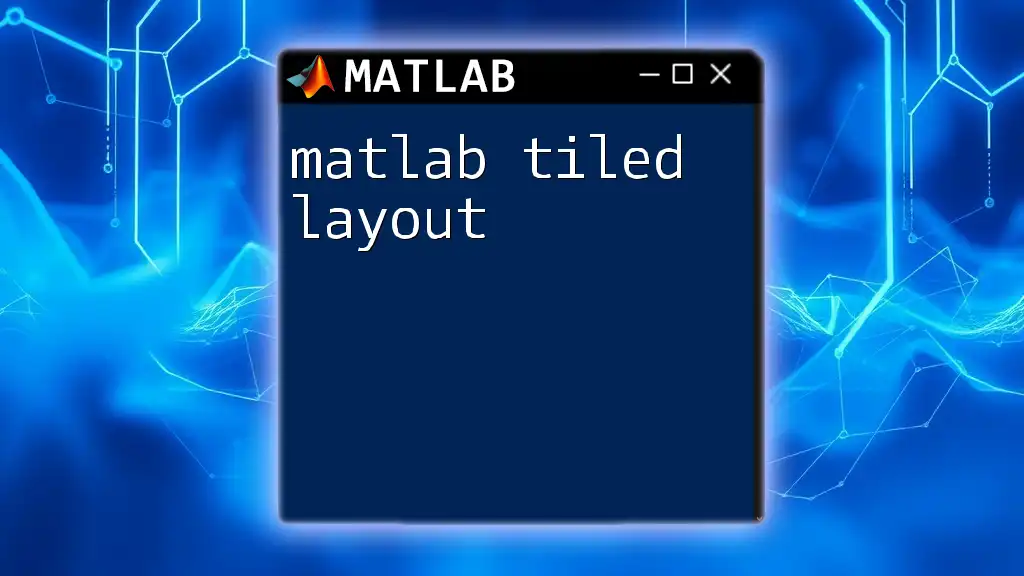
Conclusion
In conclusion, mastering `while` loops in MATLAB is crucial for effective programming. They provide a powerful means to control the flow of your programs, especially in scenarios where the number of iterations is unpredictable. By understanding the structure, applications, pitfalls, and debugging techniques related to `while` loops, you can enhance your coding skills and tackle complex problems with confidence.
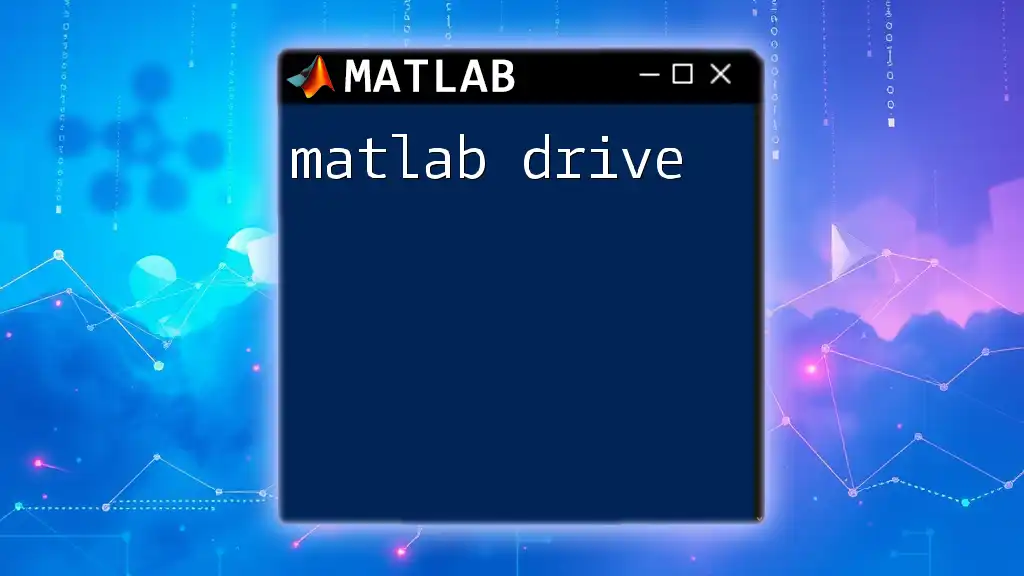
Additional Resources
For further learning, explore the official MATLAB documentation, enroll in online courses, or read books dedicated to MATLAB programming. Additionally, engaging with online forums and discussion boards can help you deepen your understanding and connect with fellow enthusiasts.