In MATLAB, you can write data to a text file using the `fprintf` function, which allows you to format the output accordingly.
data = [1.23, 4.56; 7.89, 0.12]; % Sample data
fileID = fopen('output.txt', 'w'); % Open file for writing
fprintf(fileID, '%f %f\n', data'); % Write data to file in a formatted way
fclose(fileID); % Close the file
Understanding Text Files in MATLAB
What is a Text File?
A text file is a file that contains data in a format that is easily readable by humans and machines. Characteristics of text files include their ability to store data as plain text, allowing for diverse uses across programs and systems. Common types of text files are `.txt` and `.csv`, with the latter often utilized for data interchange between different applications, particularly those involving spreadsheets.
Why Write Data to Text Files?
Writing data to text files in MATLAB is essential for several reasons. It enables data persistence, meaning you can save your results for later use to avoid repetitive calculations. Additionally, it allows for data sharing, facilitating collaboration across different platforms or software environments. Use cases vary widely—from storing experimental results in engineering to managing large datasets in finance.
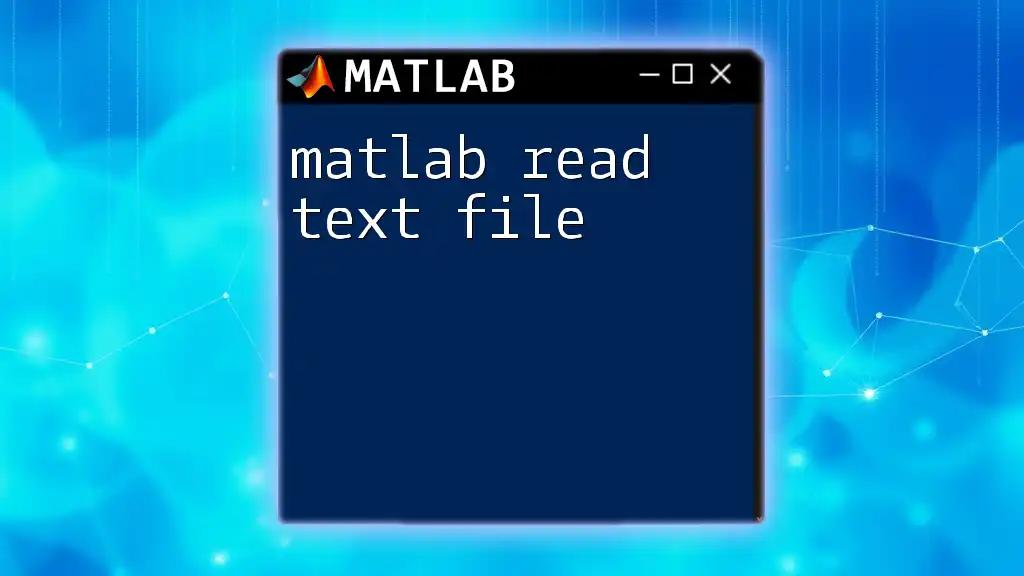
Preparing Data for Writing
Creating Sample Data
Before you can write data, you may need to create or acquire it in MATLAB. Here’s how to generate a sample dataset using MATLAB’s built-in functions. This example creates a 5x2 matrix filled with random numbers:
data = rand(5, 2); % Example of a 5x2 matrix of random numbers
Formatting Data
Choosing the Right Format
Selecting the appropriate format for your text file is critical. Options include tab-delimited and comma-separated formats. Each format has its strengths, with CSV being particularly popular for data manipulation in software like Excel. If you want to generate formatted data for CSV, you can use the following code:
formattedData = sprintf('%.2f,%.2f\n', data'); % Formatting for CSV
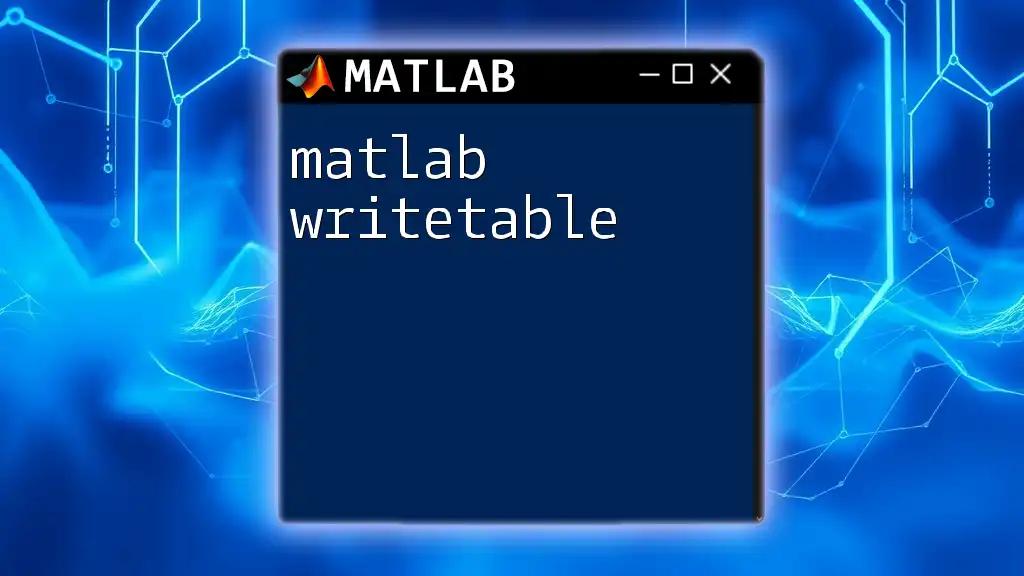
Writing Data to a Text File
Using `writematrix()` Function
The `writematrix()` function is a straightforward method to write numeric data to a text file. It provides a clean and easy interface for saving matrices as files. Here’s the syntax:
writematrix(data, 'data.txt'); % Writing random data to a text file
This command creates a file named `data.txt` in your current working directory, containing the data matrix you specified.
Using `fprintf()` for Custom Formatting
When you need more control over how your data appears in the text file, `fprintf()` is the go-to function. With `fprintf()`, you can specify formatting options for how the data is written to the file. The basic syntax is:
fprintf(fileID, formatSpec, A)
Here’s an example of using `fprintf()`:
fileID = fopen('data.txt', 'w'); % Open/create a file for writing
fprintf(fileID, 'Value1, Value2\n'); % Writing headers
fprintf(fileID, '%.2f, %.2f\n', data'); % Writing values
fclose(fileID); % Close the file
In this example, `fopen()` opens the file for writing. The `fprintf()` function is then utilized to write a header and subsequently the data from the matrix, demonstrating how to format your output neatly. Closing the file with `fclose()` is vital to ensure that all data is written and the file is properly saved.
Using `dlmwrite()` for Delimited Files
For those who prefer a simpler method of writing delimited data, `dlmwrite()` is an excellent option. It allows you to write matrix data to a text file while specifying a delimiter. The syntax is:
dlmwrite(filename, M, delimiter)
Here’s how to use it to write your data as a CSV file:
dlmwrite('data.csv', data, ','); % Writing data to a CSV file
This command will take `data` and write it into `data.csv`, using a comma as the delimiter, which facilitates easy import into spreadsheet software.
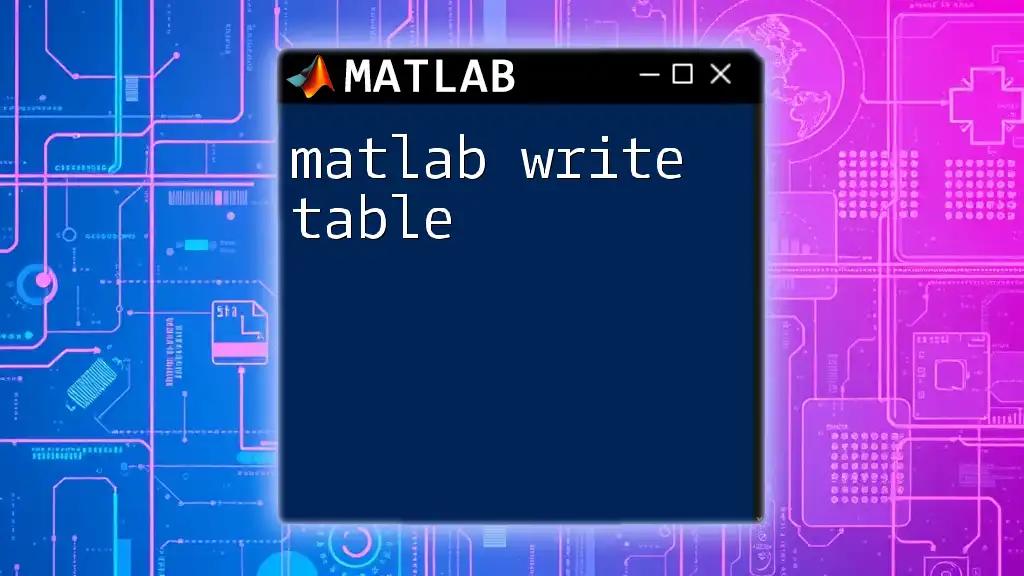
Reading Data from Text Files
Brief Insight into Data Retrieval
Once you've written data to a text file, being able to read it back into MATLAB is equally important. The `readmatrix()` function is a straightforward way to import matrix data. Here’s an example:
importedData = readmatrix('data.txt'); % Read data back into MATLAB
This command reads the data stored in `data.txt` and imports it into the variable `importedData`, allowing further manipulation or analysis.
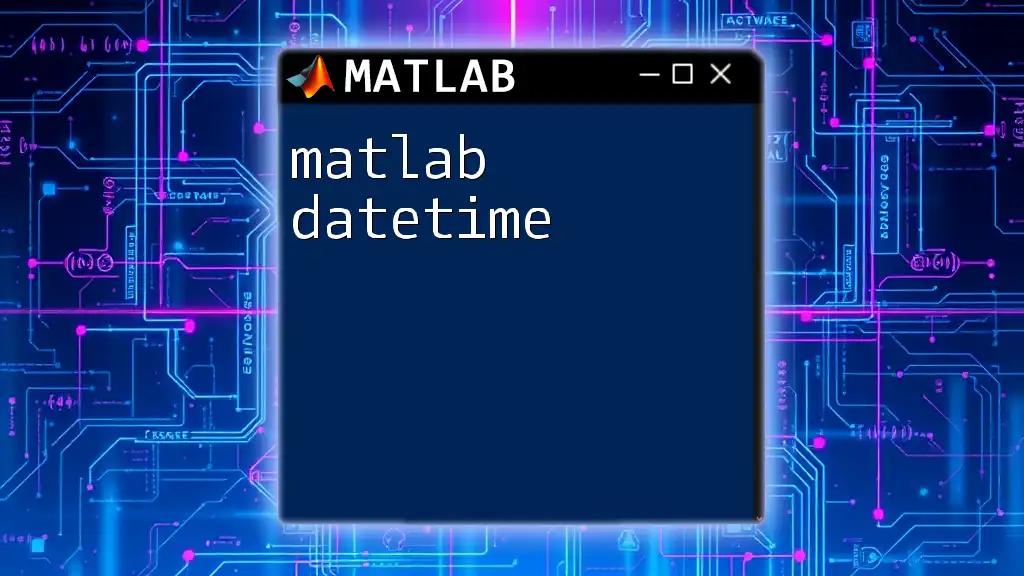
Best Practices for Writing Data to Text Files
Choosing the Right File Format
When deciding how to write your data, consider key factors such as file size, accessibility, and compatibility with other software. For instance, use CSV for interoperability, while plain text files may be sufficient for simpler applications.
Properly Closing Files
Always remember to close your files using `fclose()`. Neglecting to do so can lead to corrupted files or data loss:
fclose(fileID); % Ensure you close the file
Error Handling
Implementing error handling can safeguard against unexpected issues when dealing with file I/O. You can check if your file opened successfully using the following approach:
fileID = fopen('data.txt', 'w');
if fileID == -1
error('Failed to open file for writing.');
end
This snippet checks if the file was opened successfully and raises an error if it wasn't, ensuring you tackle potential problems early in your writing process.
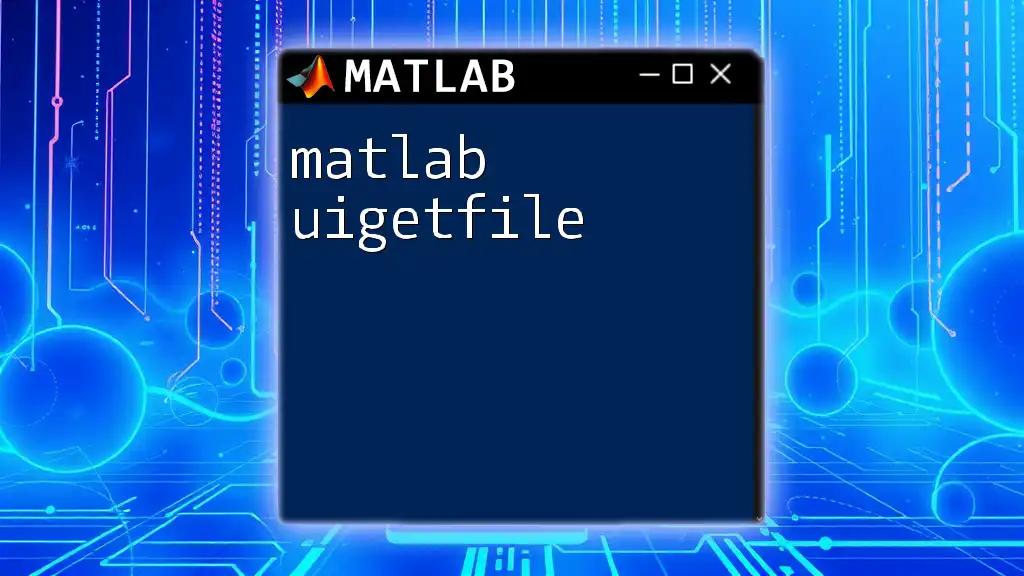
Conclusion
In summary, writing data to text files in MATLAB is crucial for data preservation, sharing, and analysis. With functions like `writematrix()`, `fprintf()`, and `dlmwrite()`, you have a variety of tools at your disposal for efficient data management. By employing best practices such as proper formatting, closing files correctly, and implementing error handling, you can optimize your MATLAB workflows for success.
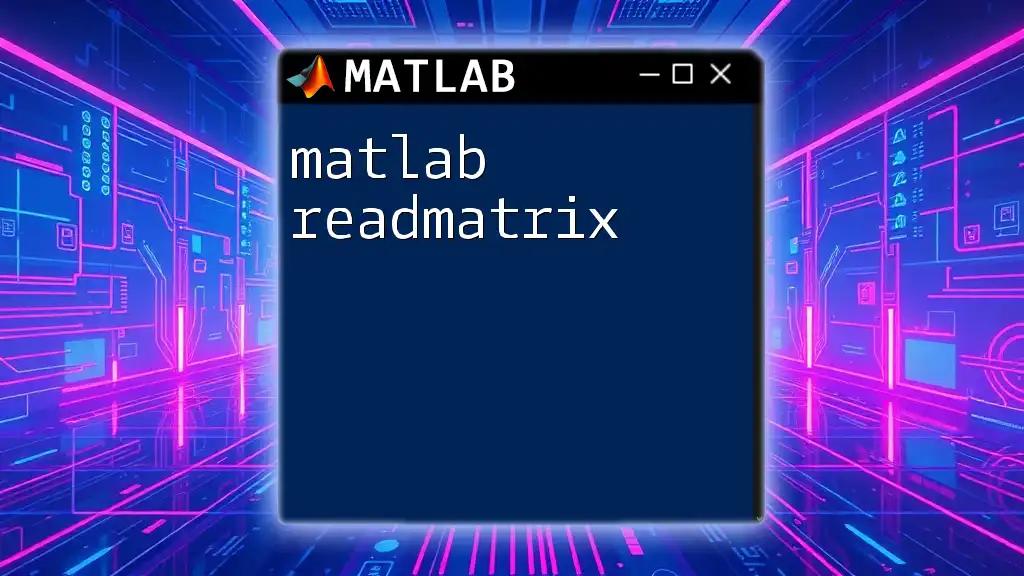
Call to Action
If you want to deepen your understanding of MATLAB capabilities, consider joining our course for in-depth tutorials and hands-on exercises. Subscribe for regular updates and tips to further enhance your MATLAB skills!
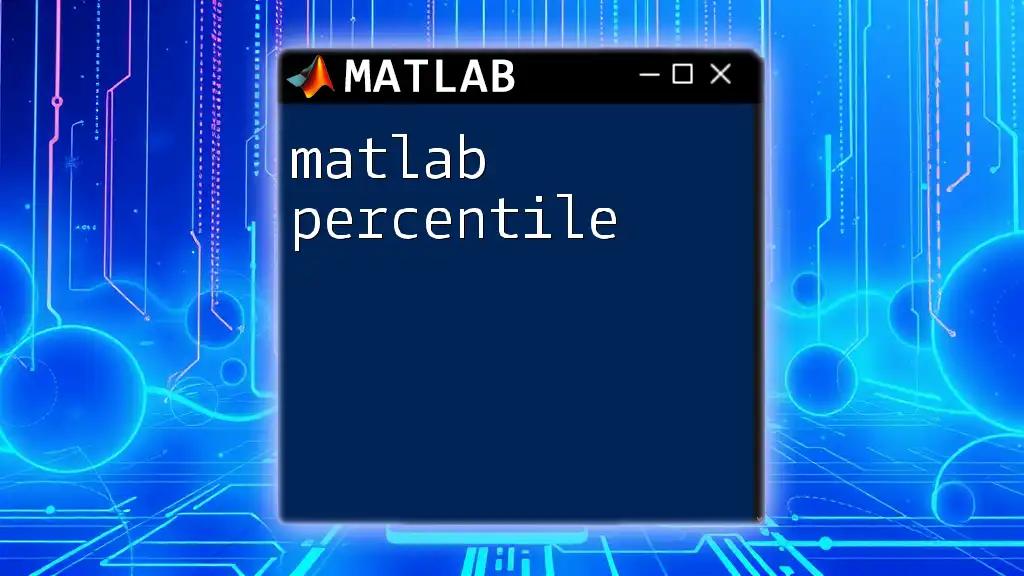
Additional Resources
For more information on file input/output, refer to the MATLAB documentation. Explore additional reading materials that can expand your knowledge in MATLAB data analysis and management.