In MATLAB, you can easily write data to a CSV file using the `writematrix` function, which exports an array to a specified file in CSV format.
Here’s a simple code snippet to demonstrate this:
data = rand(5); % Generate a 5x5 matrix of random numbers
writematrix(data, 'output.csv'); % Write the matrix to a CSV file named 'output.csv'
Understanding CSV Format
What is a CSV File?
A CSV (Comma-Separated Values) file is a plain text format used to store tabular data, where each line in the file represents a row in the table, and each value is separated by a comma. This format is widely utilized for data exchange due to its simplicity and ease of use. For example, a CSV file may look like this:
ID,Name,Age
1,Alice,28
2,Bob,35
3,Charlie,22
Advantages of Using CSV
The primary advantages of using CSV files include:
- Lightweight and Readable: CSV files are simple text files that can be opened with any text editor, making them easily readable by humans.
- Compatibility: Nearly all software applications that handle data, including Excel, Google Sheets, and various databases, can import and export CSV files.
- Ease of Use: CSV files facilitate easy data importing and exporting between different programming environments, which is particularly beneficial for analysts and developers.
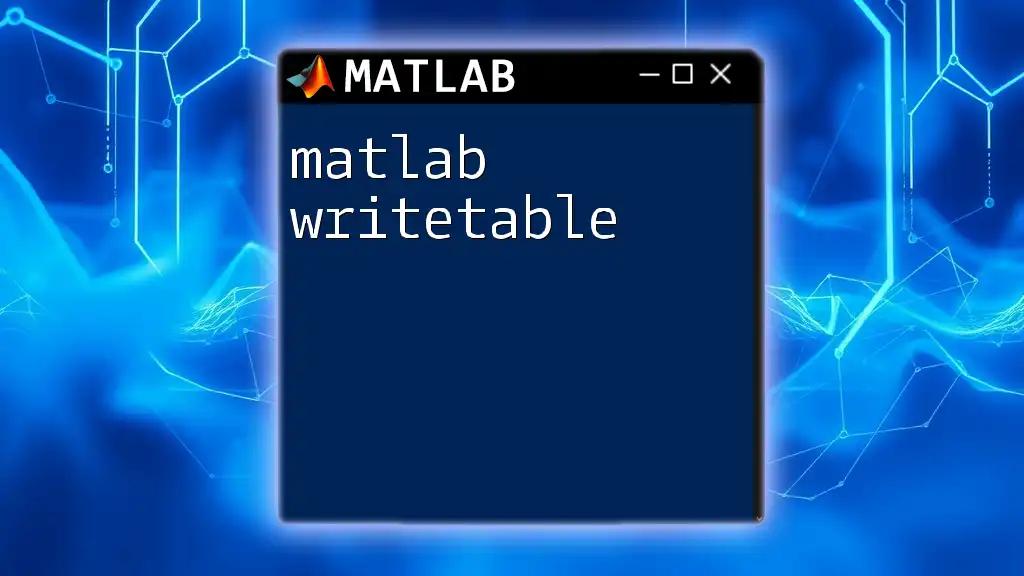
Overview of MATLAB's File I/O Functions
Introduction to MATLAB File I/O
File I/O, or Input/Output operations, are critical for reading from and writing to files. In MATLAB, this allows you to store your data and results effectively since MATLAB's extensive array manipulation capabilities often lead to large datasets that need to be preserved for future use.
Key Functions for Writing Data to CSV
MATLAB provides several functions for writing data to CSV files. The most commonly used functions include:
- `writetable`: Best for exporting data in table format, accommodating mixed data types.
- `csvwrite`: Designed specifically for writing matrices of numerical data, but limited in handling non-numeric types.
- `dlmwrite`: Offers flexibility to specify a delimiter of your choice, making it useful when you don’t want to use a comma.
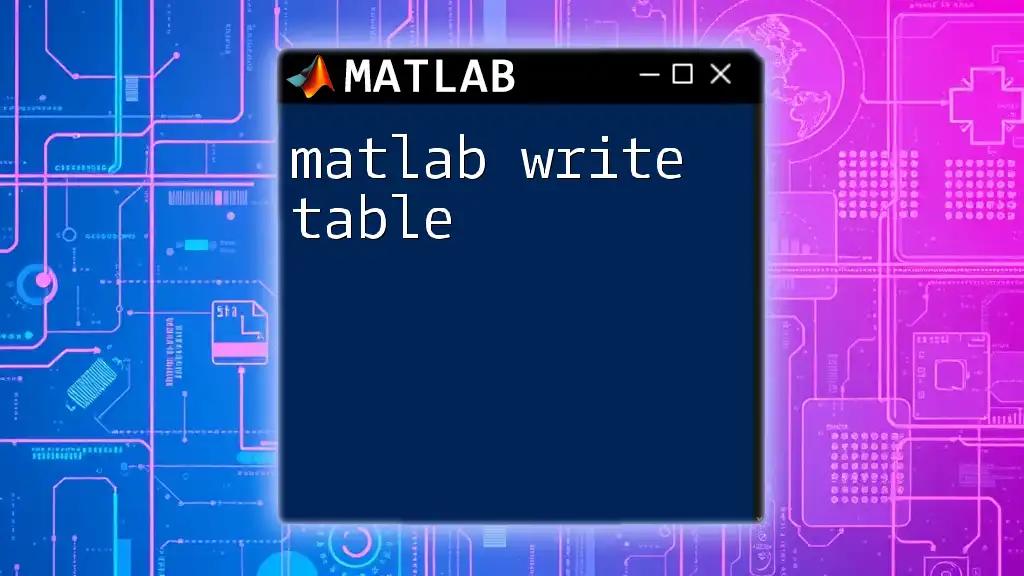
Using `writetable` to Write Data to CSV
Overview of `writetable`
The `writetable` function is a versatile tool in MATLAB for exporting tabular data. It is particularly useful when you have a table with various data types (e.g., strings, numbers, dates).
Syntax and Parameters
The basic syntax of `writetable` is:
writetable(T, filename, Name, Value)
- `T`: The table you want to write to a file.
- `filename`: The name of the CSV file.
- `Name, Value`: Additional options such as the delimiter or whether to write row names.
Step-by-Step Example
Here’s an example showing how to create a simple table and export it to a CSV file:
% Sample table creation
data = table([1; 2; 3], ["Alice"; "Bob"; "Charlie"], ...
'VariableNames', ["ID", "Name"]);
% Writing to CSV
writetable(data, 'output.csv');
In this example, a table named `data` is created, containing ID numbers and names. The `writetable` function then exports this data into a file called `output.csv`.
Error Handling
Common errors when using `writetable` include issues like incorrect filepath or permission errors. Always ensure that the specified file path is accessible and that you have permission to write files there. If you encounter errors, check the MATLAB documentation for troubleshooting guidance.
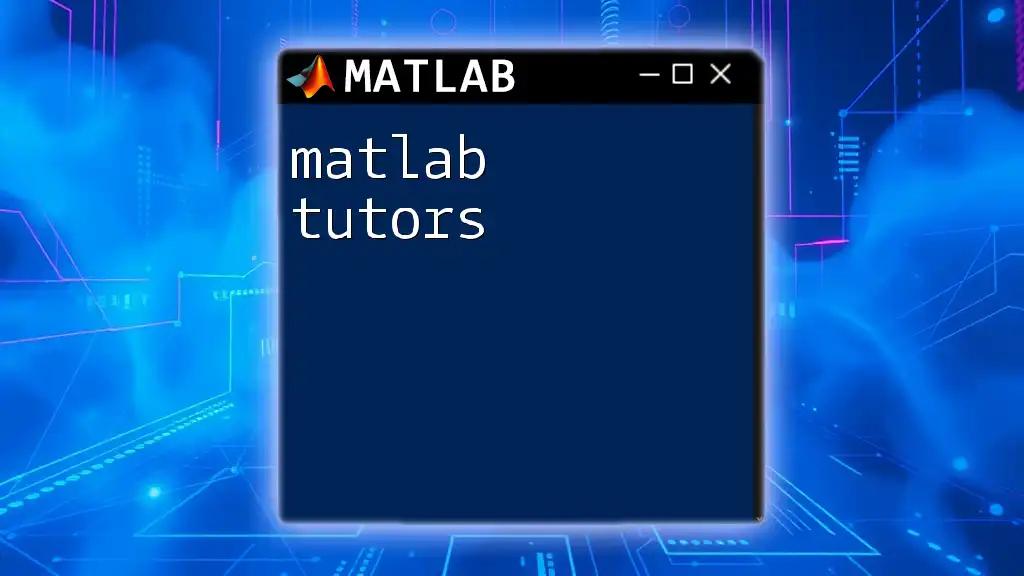
Using `csvwrite` to Write Numeric Data to CSV
Overview of `csvwrite`
`csvwrite` is straightforward and is primarily designed for outputting numerical matrices to a CSV file. This function does not handle non-numerical types, making it less flexible than `writetable`.
Syntax and Parameters
The syntax for `csvwrite` looks like this:
csvwrite(filename, M, r, c)
- `filename`: The desired name for the output CSV file.
- `M`: The matrix of numerical data you want to write.
- `r` and `c`: Optional arguments to specify the starting row and column in the file.
Example with Numerical Data
To write a simple matrix of numerical data to CSV, you could use:
% Sample numeric data
A = [1.5, 2.3, 3.6; 4.1, 5.2, 6.9];
% Writing to CSV
csvwrite('numeric_data.csv', A);
In this example, the 2x3 matrix `A` is written to a file named `numeric_data.csv`.
Limitations and Best Practices
Keep in mind that `csvwrite` cannot handle text or mixed data types. Therefore, use `writetable` for complex datasets. Additionally, try to avoid using large datasets with this function, as performance may degrade with larger matrices.
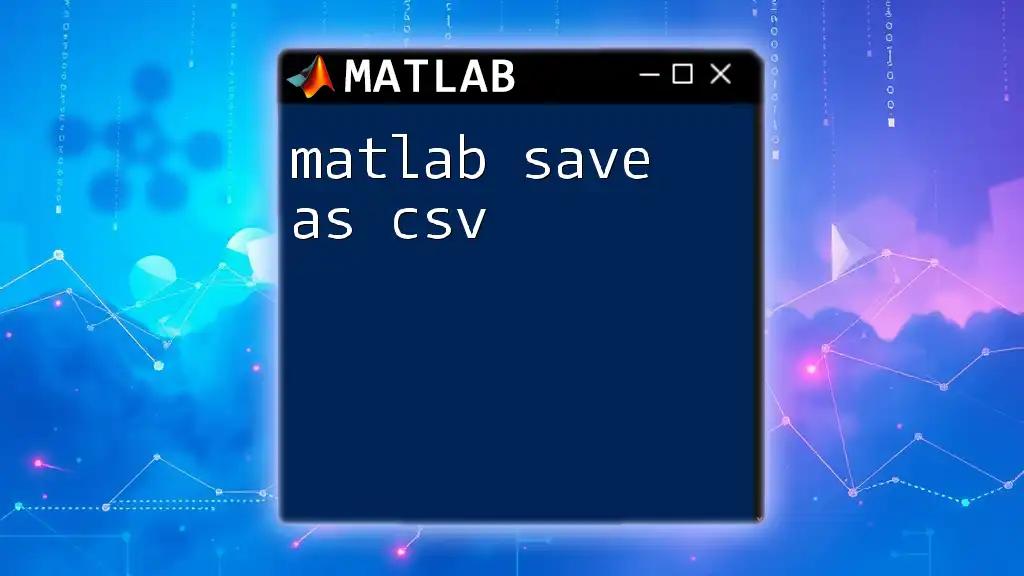
Using `dlmwrite` for Custom Delimiters
Overview of `dlmwrite`
The `dlmwrite` function is a useful option when you want to write data to a file with a custom delimiter. While it is similar to `csvwrite`, it provides the flexibility of specifying delimiters other than a comma.
Syntax and Parameters
The syntax for this function is:
dlmwrite(filename, M, delimiter, r, c)
- `delimiter`: Character to use as a delimiter (default is a comma).
- `r` and `c`: Optional initial row and column offsets where writing should begin.
Example of Custom Delimiter Usage
Here's an example of how to use a semicolon as a delimiter:
% Custom numeric data
B = [1, 2, 3; 4, 5, 6];
% Writing to CSV with a semicolon as delimiter
dlmwrite('custom_delim_data.csv', B, ';');
In this case, the matrix `B` is exported using a semicolon as the delimiter, resulting in a file structured differently than standard CSV.
Common Use Cases
Using `dlmwrite` is especially advantageous when working with data that might otherwise conflict with comma delimiters (e.g., if your data itself contains commas).
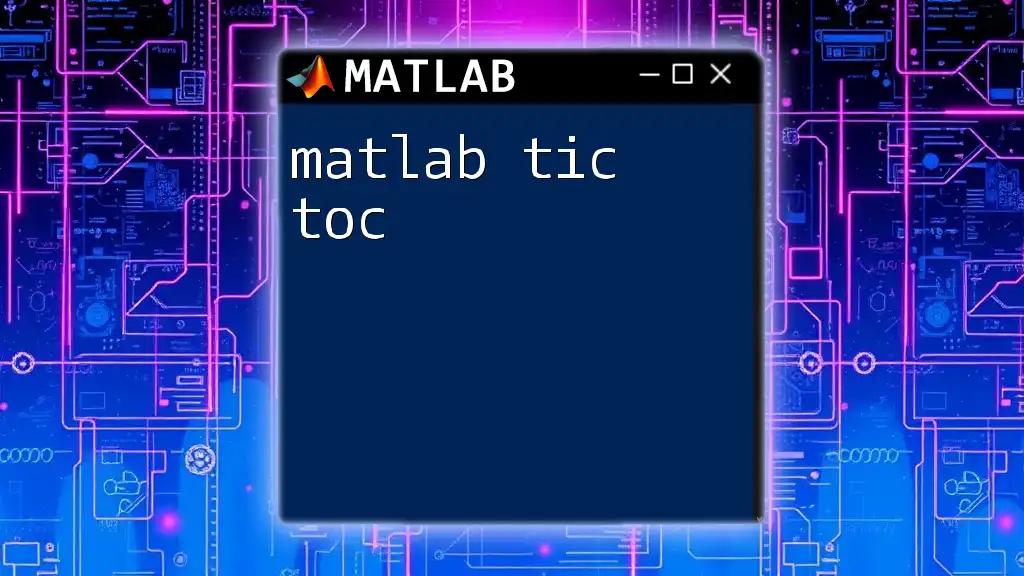
Best Practices for Writing CSV Files in MATLAB
Tips for Efficient Data Management
To optimize data management within MATLAB:
- Structure your data logically. Make use of MATLAB’s powerful data structures to keep related data together.
- Use logical indexing to filter and manipulate data before exporting it. This practice ensures that only relevant data is written to the CSV.
Recommendations for Handling Large Datasets
When dealing with large datasets:
- Consider breaking down your data into smaller segments to export them in manageable batches.
- Monitor performance and be ready to switch between the write functions based on your data types to maintain speed and efficiency.
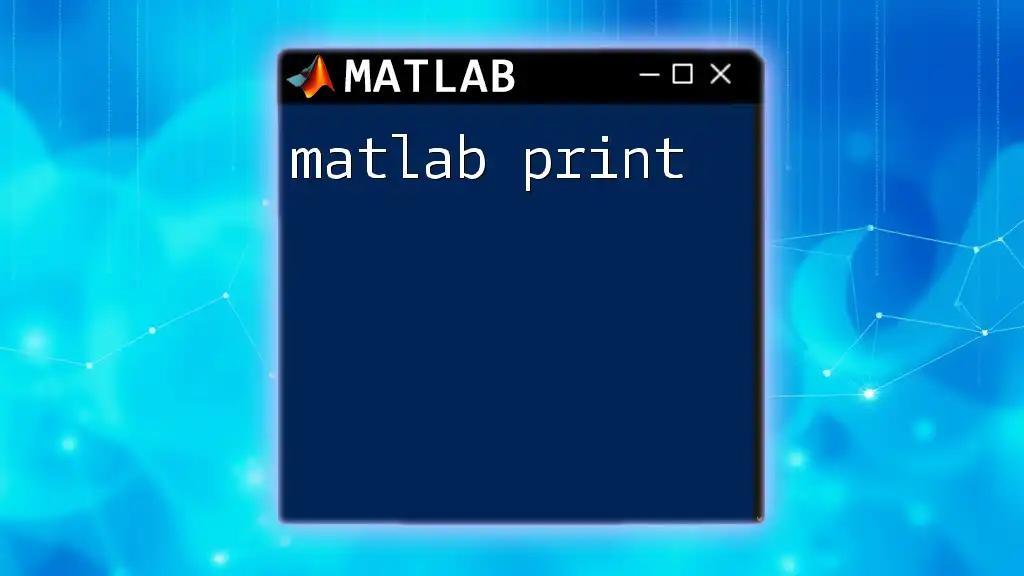
Conclusion
In summary, mastering how to matlab write to csv is essential for anyone working within the MATLAB environment. Utilizing functions like `writetable`, `csvwrite`, and `dlmwrite` accordingly will streamline your data export processes. With this guide, you should feel confident in your ability to handle various data outputs effectively.
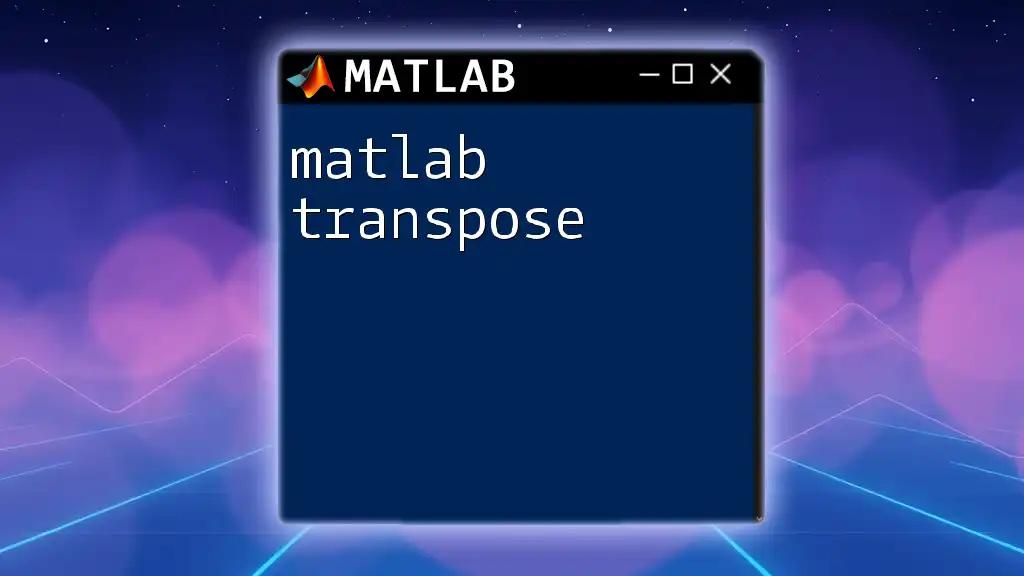
Additional Resources
Links to MATLAB Documentation
For more detailed information, consider visiting the official MATLAB documentation pages for [writetable](https://www.mathworks.com/help/matlab/ref/writetable.html), [csvwrite](https://www.mathworks.com/help/matlab/ref/csvwrite.html), and [dlmwrite](https://www.mathworks.com/help/matlab/ref/dlmwrite.html).
Recommended Books and Tutorials
Books and tutorials on MATLAB programming can further enhance your understanding, providing practical applications and examples of advanced topics in file handling.
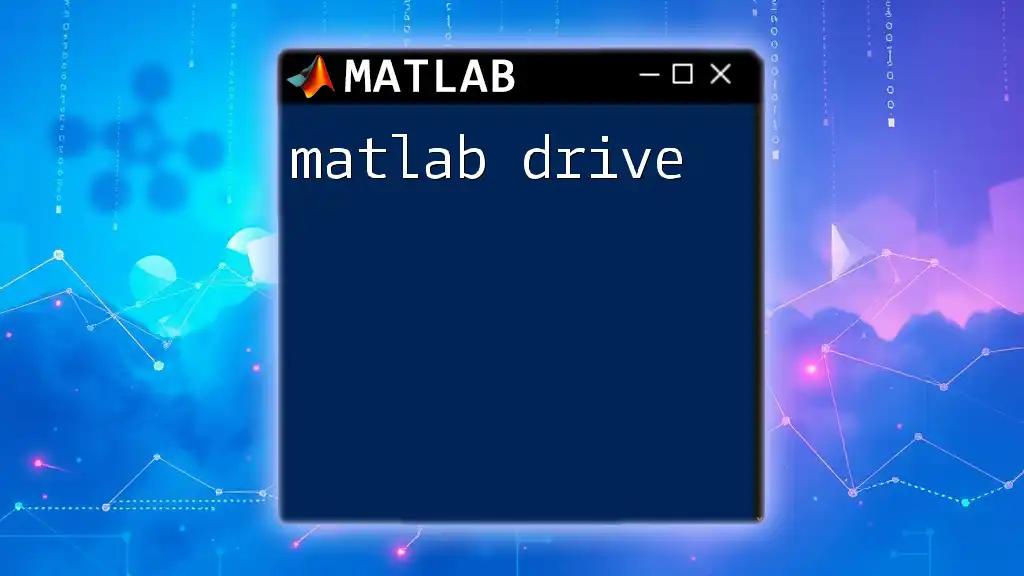
Call to Action
Feel free to share your experiences with writing to CSV in MATLAB! What challenges have you faced, and what strategies have you found effective? Engage with your fellow MATLAB learners by signing up for our courses, where we delve deeper into commands and their practical applications.