The MATLAB `xor` function computes the logical exclusive OR of two logical arrays, returning true for each element where exactly one of the input elements is true.
% Example of using xor in MATLAB
A = [1, 0, 1, 0];
B = [0, 0, 1, 1];
result = xor(A, B); % returns [1, 0, 0, 1]
Understanding XOR in Boolean Logic
Definition of XOR
The XOR (exclusive OR) operation is a fundamental logical operation that outputs true or 1 only when the inputs differ. In contrast to standard logical operations like AND, OR, and NOT, XOR focuses on the exclusivity of conditions. If one input is true and the other is false, XOR will return true; if both inputs are the same (either both true or both false), the output will be false.
Truth Table for XOR
To grasp the behavior of XOR, it is essential to understand its truth table, which neatly summarizes all possible input pairs and their corresponding output:
Input A | Input B | Output (A XOR B) |
---|---|---|
false | false | false |
false | true | true |
true | false | true |
true | true | false |
This truth table illustrates the core functionality of XOR: it only returns true when the inputs differ.
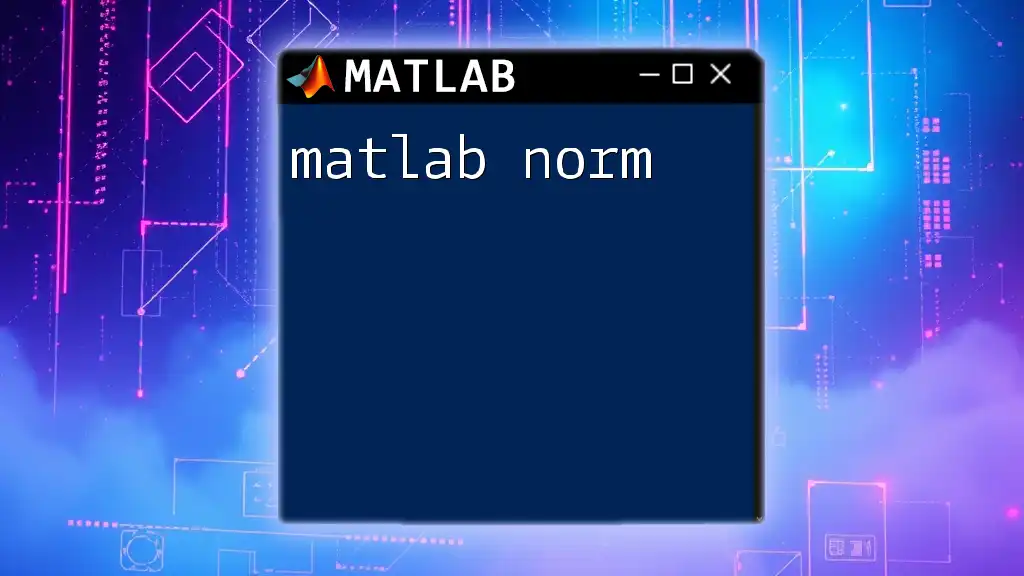
Implementing XOR in MATLAB
Using the `xor` Function
MATLAB provides a built-in function `xor` to perform the XOR operation. The syntax for using this function is straightforward:
result = xor(A, B);
Where `A` and `B` are the two logical values or arrays you want to compare.
Here’s a simple example of how to use the `xor` function with two logical inputs:
% Example 1: Basic XOR operation
A = true;
B = false;
result = xor(A, B);
disp(result); % Expected output: true
Element-wise XOR for Arrays
The `xor` function is particularly powerful when applied to arrays, executing element-wise operations. This allows for simultaneous comparisons of multiple logical conditions.
% Example 2: Element-wise XOR operation
A = [true, false, true];
B = [false, false, true];
result = xor(A, B);
disp(result); % Expected output: [true, false, false]
In this example, the function evaluates each corresponding pair of elements in the arrays `A` and `B`, returning an array of results based on the XOR logic.
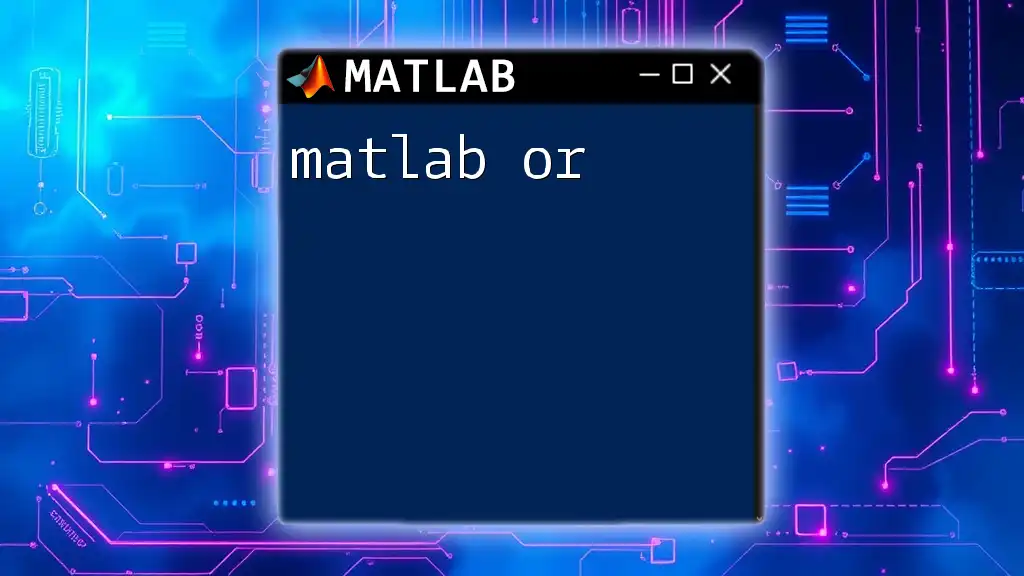
Practical Applications of XOR in MATLAB
Use Case 1: Error Detection
One practical application of XOR is for error detection in digital communications. XOR can help in verifying whether data bits have been received correctly. For instance, in a parity check system, you can determine whether the number of bits set to 1 is odd or even.
% Example 3: Parity check using XOR
data_bits = [1, 0, 1, 1];
parity_bit = mod(sum(data_bits), 2);
disp(parity_bit); % Expected output: 1 (Odd parity)
In this example, the outcome of 1 indicates that the count of 1s in the input data bits is odd, suggesting that if an even parity was expected, there may have been a transmission error.
Use Case 2: Bit Manipulation
Another fascinating application of the XOR operation is in bit manipulation. XOR can help perform tasks such as swapping two numbers without needing a temporary variable. Here’s how:
% Example 4: Swapping two variables using XOR
x = 10;
y = 20;
x = xor(x, y);
y = xor(x, y);
x = xor(x, y);
fprintf('x: %d, y: %d\n', x, y); % Expected output: x: 20, y: 10
This example showcases how XOR can achieve swaps with just a few lines of code, highlighting its efficiency in low-level operations.
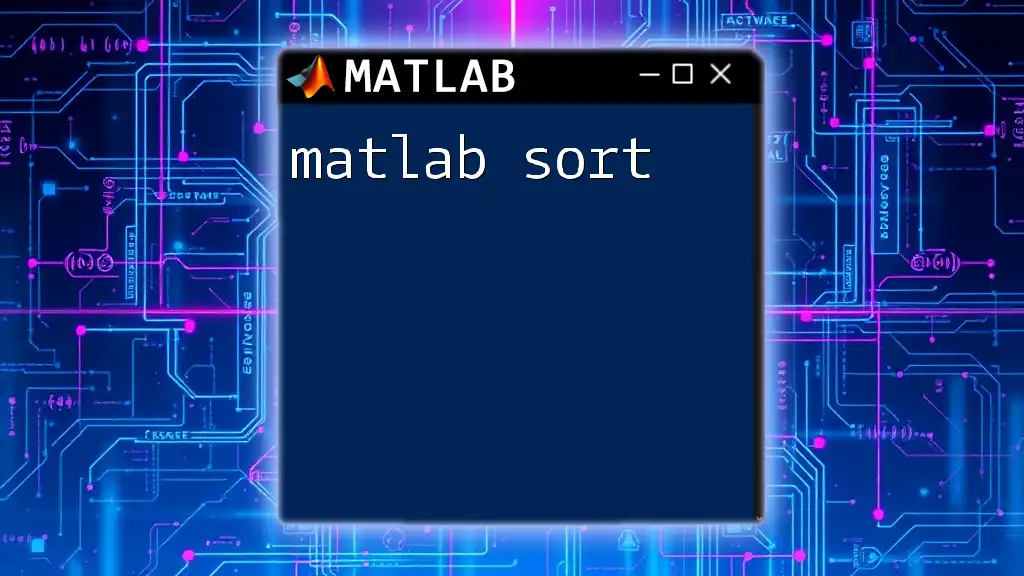
Advanced Topics in XOR Usage
XOR in Image Processing
XOR can also be beneficial in image processing, particularly for operations like masking. For instance, using XOR can help isolate particular features in an image by "masking" parts of one image with another.
% Example 5: Image Masking with XOR
img1 = imread('image1.png');
img2 = imread('image2.png');
masked_image = xor(img1, img2);
imshow(masked_image);
In this example, applying XOR between two images reveals areas where the two images differ, effectively highlighting edges or features.
Combining XOR with Other Logic Operations
Combining XOR with other logical operations like AND and OR can provide powerful results in logical circuit simulations or complex condition evaluations. For example, the combination can simulate circuits by utilizing logical gates to simplify conditions.
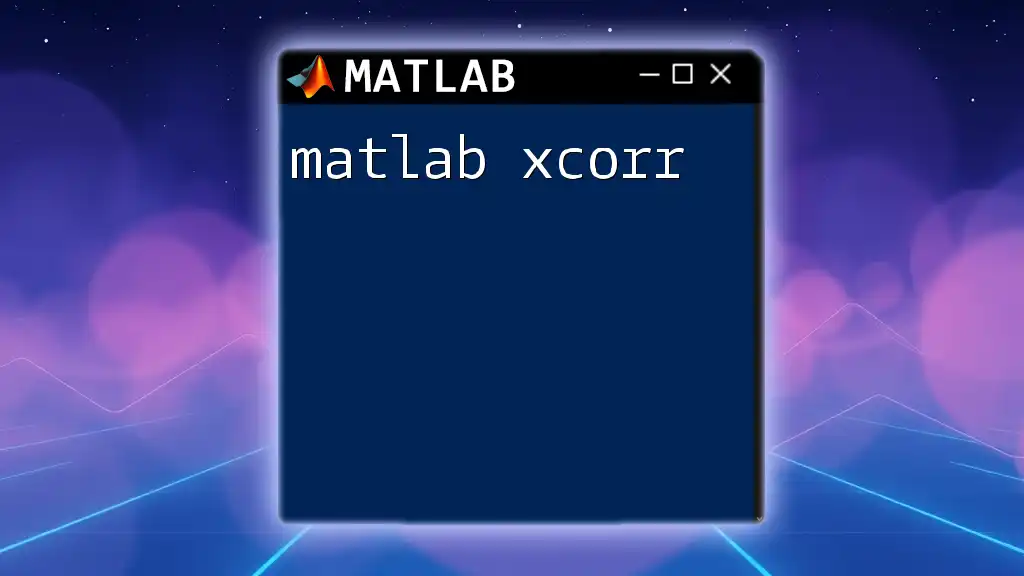
Common Mistakes when Using XOR
Users new to XOR may encounter common pitfalls such as misunderstanding its output or failing to correctly apply it with larger arrays. It's essential to remember that XOR does not simply treat inputs as zeroes and ones; it evaluates logical true and false, which may lead to confusion when integrating with numeric arrays.
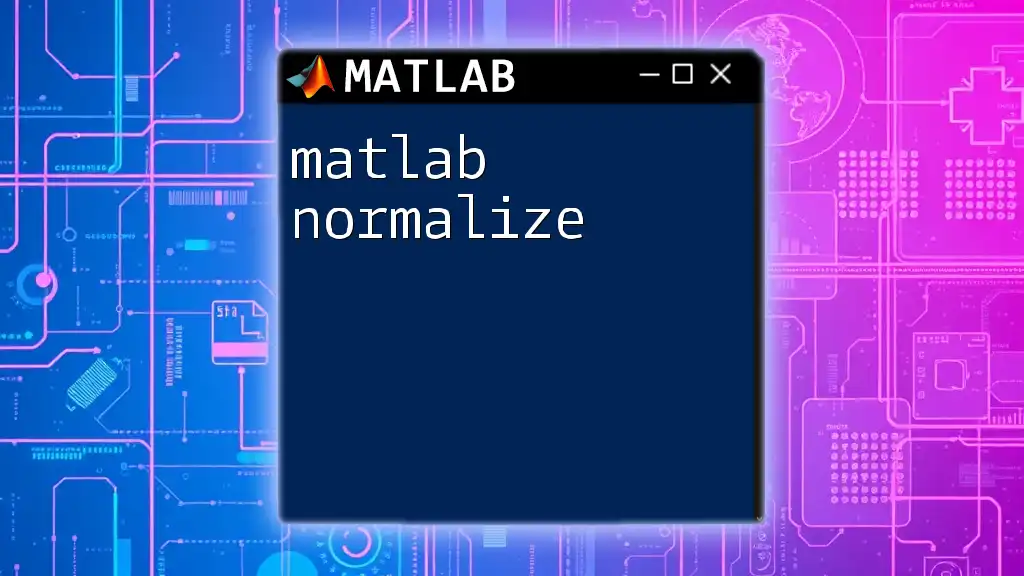
Conclusion
In summary, mastering the `matlab xor` function opens up numerous possibilities in logical operations and applications. Whether you are verifying data integrity, manipulating bits, or processing images, understanding how to use XOR effectively in MATLAB is invaluable for any programming endeavor.
By experimenting with the `xor` function and exploring its applications, you can enhance your MATLAB skills and programmer's toolkit.
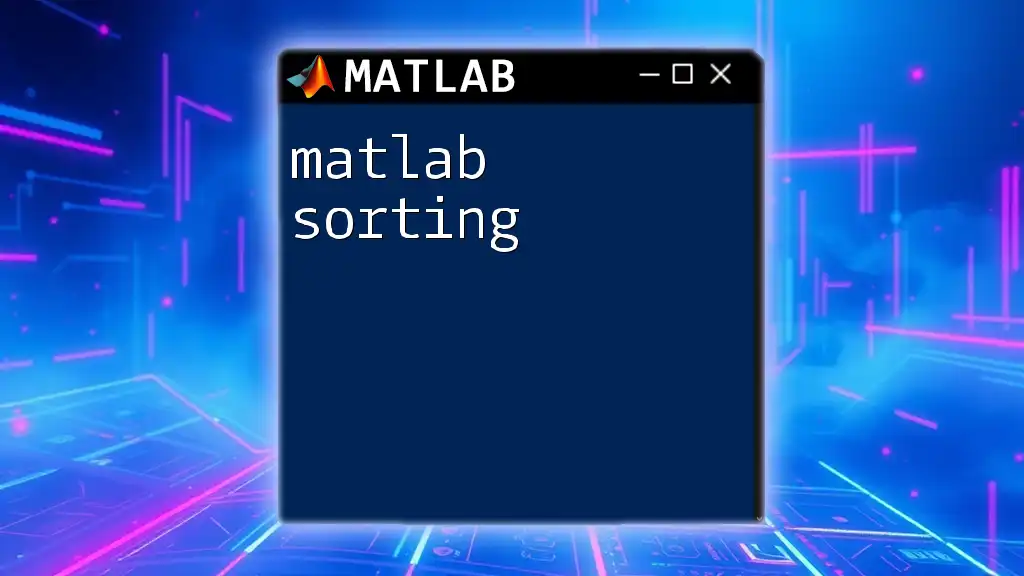
Call to Action
Dive deeper into the world of logical operations in MATLAB! Share your own experiences and examples of how you've utilized the `xor` function in your projects in the comments below. Let’s exchange knowledge and grow together!