In MATLAB, the error "Not enough input arguments" occurs when a function is called without the required number of arguments specified in its definition.
Here’s a code snippet that demonstrates this error:
function result = addNumbers(a, b)
result = a + b;
end
% Calling the function with only one input argument:
sum = addNumbers(5); % This will trigger the "Not enough input arguments" error.
Understanding MATLAB Input Arguments
What are Input Arguments?
Input arguments are essential components in MATLAB functions that allow users to pass information into the function for processing. Without input arguments, functions cannot perform their intended tasks or make calculations based on user input.
Types of Input Arguments
-
Required Arguments are arguments that must be provided for the function to execute. If even one required argument is missing, MATLAB will return an error message indicating there are "not enough input arguments."
-
Optional Arguments allow users to specify additional parameters. If these arguments are not supplied, the function can utilize default values, thus providing flexibility.
-
Variable-length Arguments enable a function to accept a variable number of input arguments, which is particularly useful for operations that may involve different amounts of data.
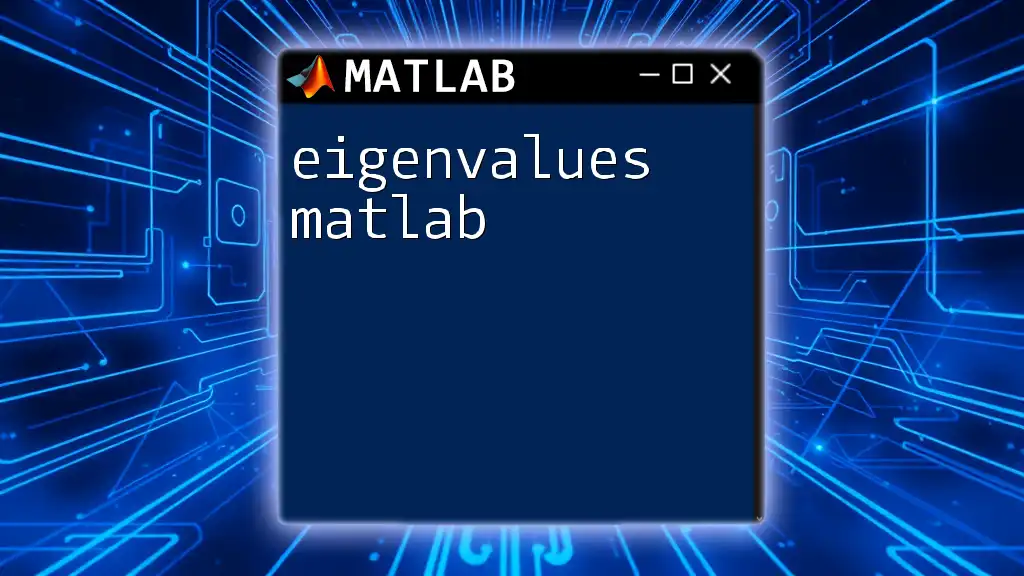
Common Causes of 'Not Enough Input Arguments' Errors
Introduction to Errors in MATLAB
Errors are a fundamental part of programming in MATLAB, serving as warnings for potential issues in your code. While some errors might be straightforward, like syntax errors, others require a more profound understanding to resolve effectively.
The 'Not Enough Input Arguments' Error
The "not enough input arguments" error occurs when a function is called without the requisite number of input parameters. Functions that rely on certain input values will not run correctly if necessary information is missing. This is particularly common in function definitions when certain arguments are required.
Understanding the Error Message
When MATLAB raises the "not enough input arguments" error, the message is clear. It typically says something like:
Error using functionName (line X)
Not enough input arguments.
This informs you which function is causing the issue and helps to direct your debugging efforts.
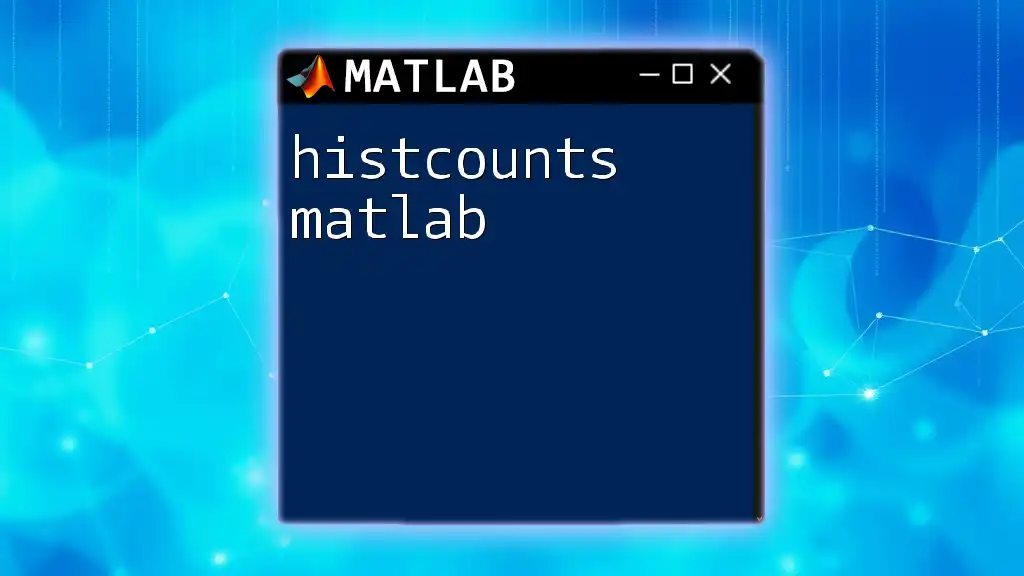
Handling Input Arguments in MATLAB Functions
Defining Functions with Required Input Arguments
To define a function in MATLAB that mandates required input arguments, you would structure it as follows:
function area = calculateArea(length, width)
area = length * width;
end
In this example, `length` and `width` are required inputs. If either is missing when the function is called, MATLAB will throw an error.
Using Optional Input Arguments
For managing optional input arguments, you can utilize the `nargin` function to check how many arguments were provided. This allows the function to assign default values when needed. Here's how you can implement this:
function area = calculateArea(length, width)
if nargin < 2
width = 1; % default width
end
area = length * width;
end
In the code above, if the user only provides `length`, the function defaults `width` to 1, preventing the "not enough input arguments" error.
Implementing Variable-length Input Arguments
When you want to allow a function to accept a range of input sizes, you can use `varargin`. This is particularly powerful for functions that need to perform operations on an unspecified number of inputs:
function result = sumValues(varargin)
result = sum([varargin{:}]);
end
In this example, `sumValues` can accept any number of arguments, allowing for flexibility in calculations.
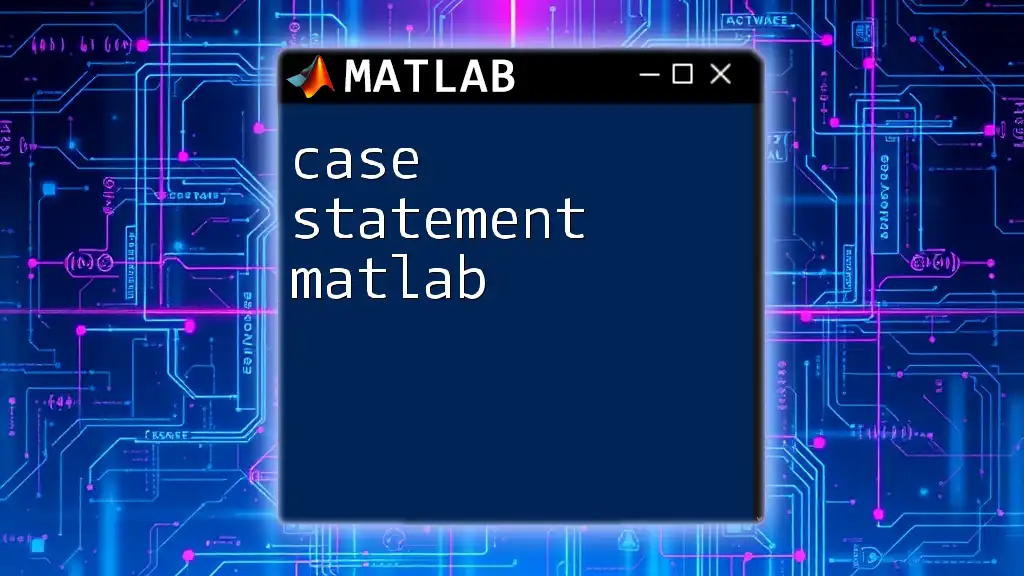
Resolving 'Not Enough Input Arguments' Issues
Debugging Techniques
Debugging in MATLAB is critical for identifying the source of the "not enough input arguments" error. You can use various techniques, such as:
- Setting Breakpoints: This allows you to pause execution at a specific line and inspect the values of variables.
- Displaying Variable Values: Using `disp` or `fprintf`, you can output the values of variables to understand what is being passed to your functions.
Modifying Function Calls
Ensuring that your function calls include the necessary arguments is crucial. When a function raises the "not enough input arguments" error, check the argument list and compare it to the function definition. For example:
% Incorrect
area1 = calculateArea(5); % Generates an error
% Correct
area2 = calculateArea(5, 4); % This works
In this case, the first call results in an error because it lacks the width parameter.
Best Practices for Avoiding the Error
To minimize the occurrence of the "not enough input arguments" error:
- Clearly define the required and optional arguments in your function documentation.
- Instruct users on how to call the function correctly.
- Validate input arguments within functions to provide meaningful error messages before the function fails.
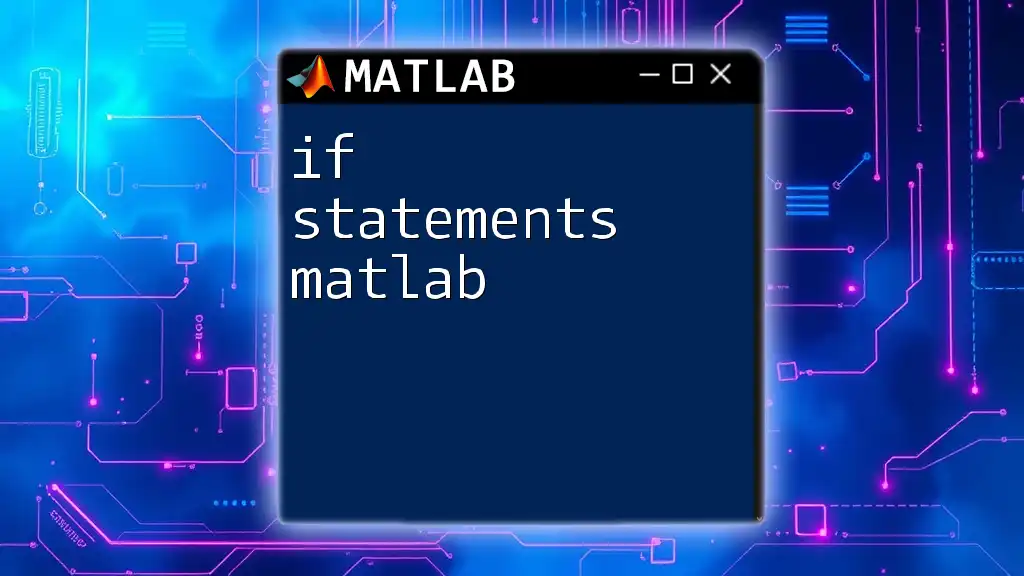
Common Patterns and Examples
Case Study: A Simple MATLAB Function
Let's consider a function that calculates the perimeter of a rectangle. Implementing robust checks can prevent errors associated with missing arguments:
function perimeter = calculatePerimeter(length, width)
if nargin < 2
error('Not enough input arguments. Please provide length and width.');
end
perimeter = 2 * (length + width);
end
Here, if a user attempts to call `calculatePerimeter(5)`, MATLAB will immediately provide a helpful error message that clarifies what is needed.
Real-World Applications
In real-world scenarios involving calculations, data analysis, and visualization tasks, users often encounter the "not enough input arguments" error, particularly in:
- Engineering applications that necessitate precise calculations.
- Data processing tasks where functions rely on input arrays of varying lengths.
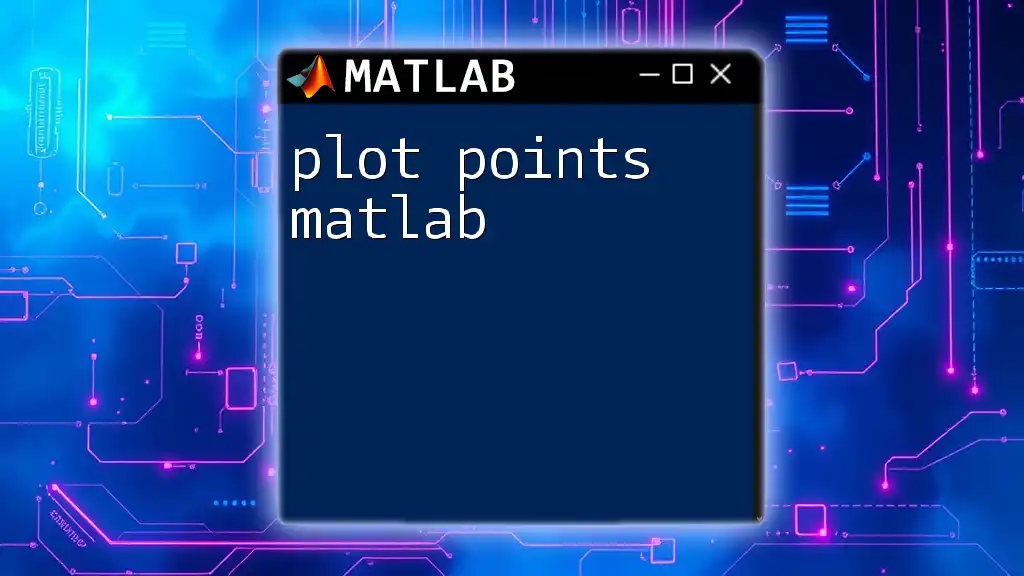
Conclusion
Recap of Key Points
Understanding how to manage input arguments in MATLAB functions is essential for effective programming. The "not enough input arguments" error can be readily avoided by structuring your functions correctly and validating inputs.
Final Thoughts
Practicing with various types of input in MATLAB will deepen your understanding of function behavior and help you become more proficient.
Additional Resources
For further learning, consult the official MATLAB documentation and explore tutorials that elaborate on using input arguments effectively.