The norm of a vector in MATLAB, calculated using the `norm` function, provides a measure of its length or magnitude in Euclidean space.
v = [3, 4, 5];
vector_norm = norm(v);
Understanding Vector Norms
What is a Vector Norm?
The norm of a vector is essentially a measure of the vector's length or magnitude in a given vector space. It is a crucial concept in mathematics, particularly in linear algebra, where it serves as a tool for quantifying distances. The norm allows us to describe how "big" or "small" a vector is, which is essential for various mathematical computations and analyses.
Types of Vector Norms
Different types of norms fulfill various mathematical needs, and understanding their unique properties is vital when working in MATLAB. Here are the most common norms:
Euclidean Norm (L2 Norm)
The Euclidean norm, often referred to as the L2 norm, is the most commonly used vector norm. It represents the length of a vector in Euclidean space, computed as the square root of the sum of the squares of its elements:
\[ \text{L2 norm} = ||\mathbf{v}||2 = \sqrt{\sum{i=1}^{n} v_i^2} \]
This norm is widely applied in geometry, physics, and engineering for tasks like distance measurement.
Manhattan Norm (L1 Norm)
The Manhattan norm, or L1 norm, is calculated as the sum of the absolute values of the vector elements:
\[ \text{L1 norm} = ||\mathbf{v}||1 = \sum{i=1}^{n} |v_i| \]
This norm is particularly useful in scenarios where we want to measure distance in grid-like paths, such as urban planning or certain algorithms in data science.
Max Norm (Infinity Norm)
The Max norm, also known as the infinity norm, takes the maximum absolute value among the vector's elements:
\[ \text{L∞ norm} = ||\mathbf{v}||\infty = \max{i}|v_i| \]
This norm is critical in optimization problems where the goal is to minimize the maximum error.
Generalized Norms
Generalized norms, often referred to as p-norms, extend the idea of vector norms to any positive real number \( p \). The generalized norm is defined as follows:
\[ ||\mathbf{v}||p = \left( \sum{i=1}^{n} |v_i|^p \right)^{1/p} \]
MATLAB allows the calculation of these generalized norms, making it easy to handle various applications.
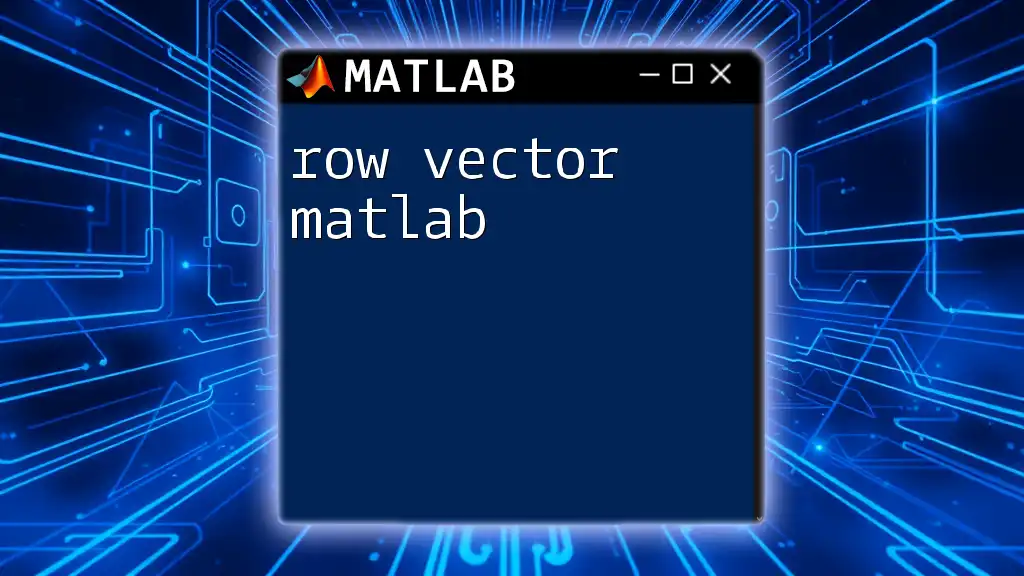
Using MATLAB to Calculate Vector Norms
Introduction to MATLAB's Built-in Functions
MATLAB simplifies the computation of vector norms through its built-in `norm` function. This function supports various types of norms, allowing users to efficiently perform calculations without needing to write extensive code.
Calculating the Euclidean Norm
To calculate the Euclidean norm in MATLAB, you can use a straightforward command:
v = [3, 4];
euclidean_norm = norm(v);
disp(euclidean_norm);
In this example, the vector `v` contains the elements 3 and 4. The `norm(v)` function computes the Euclidean norm, which evaluates to 5, as it corresponds to the length of the vector. This kind of demonstration is prevalent in physics and engineering to determine distances in space.
Calculating the Manhattan Norm
For the Manhattan norm, the calculation involves a slight modification to the `norm` function by specifying the appropriate parameter:
v = [3, 4];
manhattan_norm = norm(v, 1);
disp(manhattan_norm);
In this code snippet, the `1` passed to the `norm` function specifies the Manhattan norm (L1 norm). The output will show a result of 7, highlighting the sum of the absolute values of the vector's components.
Calculating the Max Norm
Calculating the Max norm is similarly straightforward. Here’s how you can do it:
v = [3, 4];
max_norm = norm(v, Inf);
disp(max_norm);
In this scenario, the output will be 4, which is the maximum value among the components of vector `v`. This calculation is instrumental in optimization problems where controlling the maximum value is critical.
Generalized Norms in MATLAB
To compute a generalized norm, you can specify the desired value of \( p \):
v = [3, 4];
p = 2; % For Euclidean norm
generalized_norm = norm(v, p);
disp(generalized_norm);
In this example, we have calculated the Euclidean norm again but demonstrated the flexibility to choose a different \( p \) if needed. The ability to quickly switch norms makes MATLAB a powerful tool for various engineering challenges.
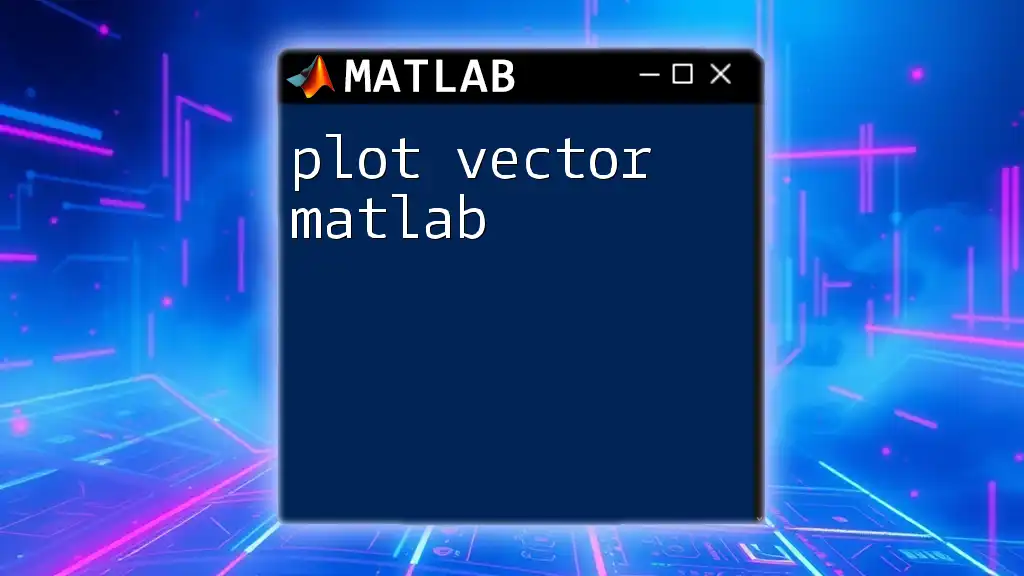
Practical Applications of Vector Norms
Usage in Data Analysis
Vector norms play a significant role in data analysis, particularly for normalizing datasets. Normalization helps ensure each feature contributes equally, often essential in preparing data for machine learning models. For instance, an L2 normalization ensures that the length of a feature vector equals 1, standardizing the data for further analysis.
Usage in Machine Learning
In machine learning, the choice of vector norm can significantly affect the performance of algorithms. For example, in k-means clustering, using the Euclidean norm allows for intuitive distance measures. In contrast, the Manhattan norm might be more appropriate for certain scenarios, such as when dealing with high-dimensional sparse data.
Usage in Physics and Engineering
Vector norms are crucial in physics for understanding forces, momentum, or any situation involving directional quantities. For example, when simulating a multi-body system, you may want to calculate the resultant forces acting on a point; vector norms help quantify these forces effectively.
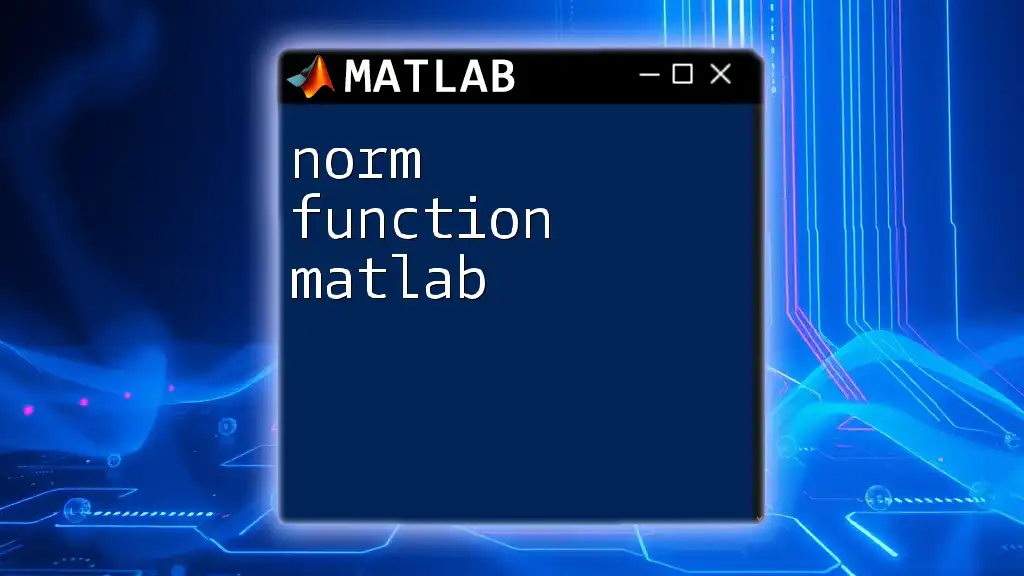
Common Mistakes and Troubleshooting
Misunderstanding Norms
One frequently encountered issue is misunderstanding the characteristics of vector norms. Many newcomers may confuse the L1 and L2 norms or misinterpret their significance. It's essential to understand that while they both measure magnitude, they provide different insights and are used in different contexts.
MATLAB Errors
When using the `norm` function, common errors include mistyping the function call or incorrectly implementing the vector inputs. MATLAB is designed to provide informative error messages, but being mindful of dimension mismatches and input types can prevent a lot of confusion.
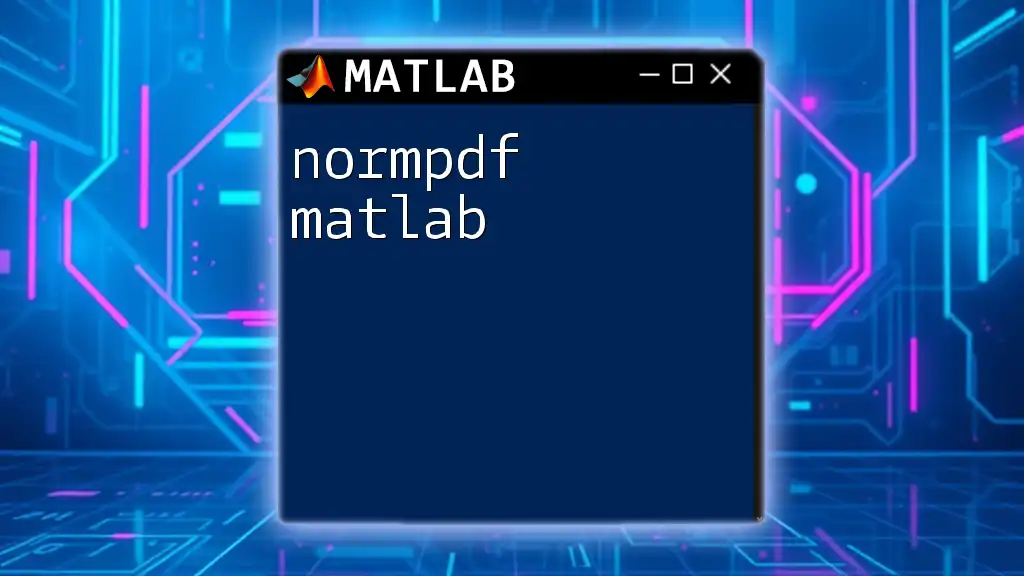
Conclusion
In conclusion, understanding the norm of vectors in MATLAB is a fundamental skill that bridges mathematical theory and practical application. Vector norms serve various purposes across multiple domains, allowing for better insights and actionable results in data analysis, machine learning, and engineering.
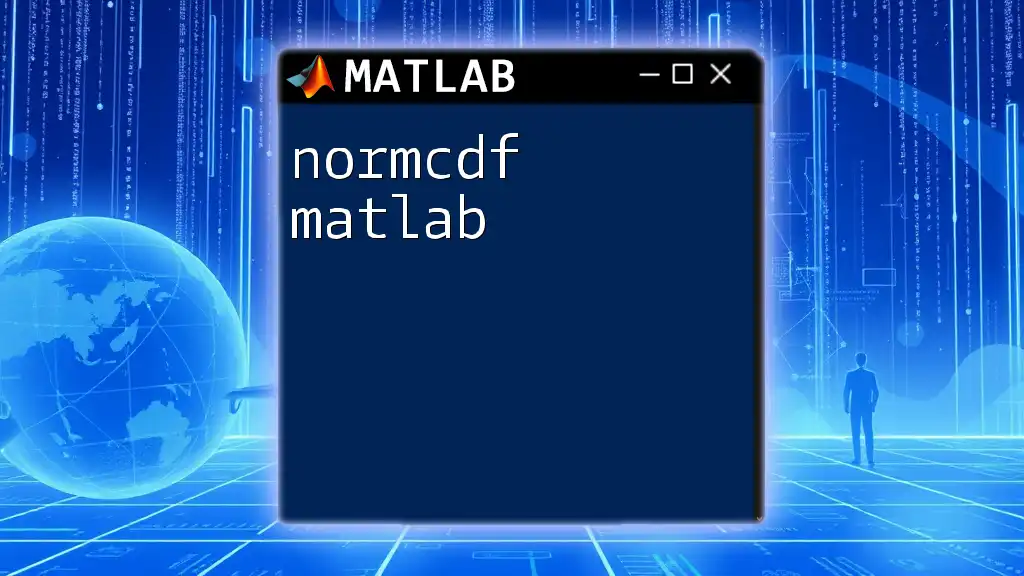
Additional Resources
For further exploration, consider referring to the MATLAB documentation on norm functions or pursuing recommended books and online courses that delve deeper into linear algebra and its applications.
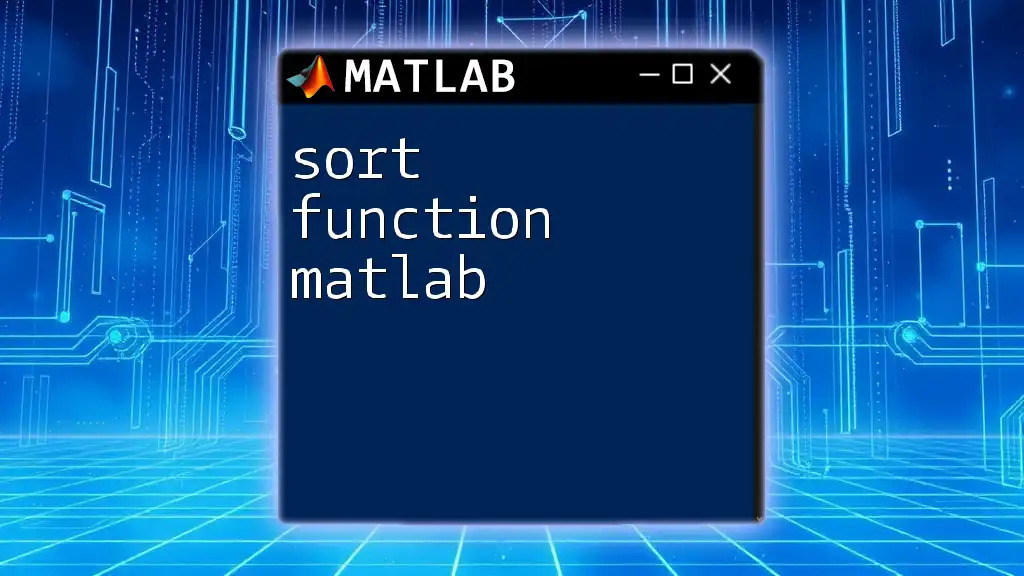
FAQs
What is the difference between L1, L2, and L∞ norms?
The L1 norm sums the absolute values of vector components, the L2 norm computes the square root of the sum of squares, and the L∞ norm finds the maximum absolute value among the components. Each norm serves different purposes based on application needs.
How do you choose which norm to use?
Choosing the right norm depends on the specific requirements of your analysis or application. For distances in continuous spaces, L2 is ideal. L1 might be more suitable when dealing with sparse data, while L∞ is useful for controlling maximum values in optimization.
Can you calculate norms for complex vectors in MATLAB?
Yes, MATLAB can handle complex vectors as well. The `norm` function calculates the magnitude of complex vectors, treating them as sums of their real and imaginary components.
This article comprehensively covers the norm of vectors in MATLAB, ensuring that readers have a solid understanding of the topic through explanations, practical examples, and guidance on common applications.