The sequence centroid in MATLAB is calculated to identify the center of mass of a sequence, which can be useful in various signal processing and analysis applications.
Here’s a simple code snippet to compute the centroid of a sequence:
sequence = [1, 2, 3, 4, 5]; % Example sequence
centroid = sum((1:length(sequence)) .* sequence) / sum(sequence);
disp(centroid);
Understanding Centroids
A centroid is a central point that can represent a geometric shape or collection of points. In data analysis, it often signifies the average position of a set of data points. Understanding how to compute centroids is critical in various fields such as engineering, data science, and machine learning, where it often informs decision-making processes and pattern recognition.
Application of Sequence Centroids
Sequence centroids in MATLAB are leveraged in various real-world applications, including:
- Signal Processing: Identifying the central tendency of signal data.
- Image Segmentation: Finding the centroid of pixel values to distinguish different areas in an image.
- Data Clustering: Evaluating the center of data points in clusters to enhance classification accuracy.
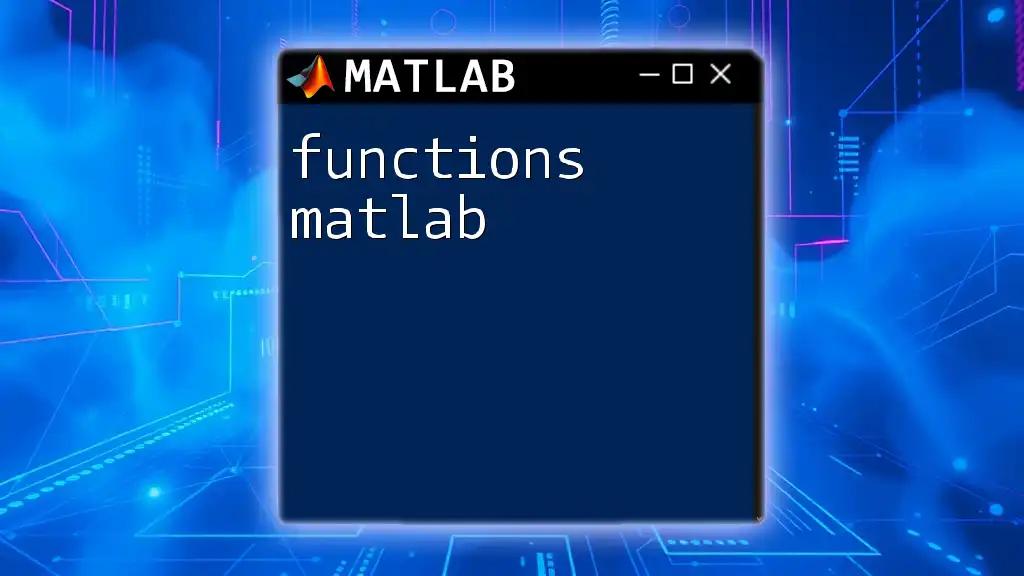
Basics of MATLAB
Introduction to MATLAB
MATLAB (Matrix Laboratory) is a programming environment designed for numerical computation, visualization, and application development. Its robust features make it an ideal tool for executing mathematical operations, making it particularly useful for engineering and scientific applications.
Getting Started with MATLAB
To begin working in MATLAB:
- Installing MATLAB: Follow the instructions provided by MathWorks for a smooth installation process tailored to your operating system.
- Basics of the MATLAB Interface: Familiarize yourself with the primary workspace, command window, script editor, and figure window to enhance your efficiency.
- Accessing the Command Window: Learn how to input commands directly for immediate feedback on calculations, which is crucial for exploring sequence centroids.

What is a Sequence?
A sequence is a list of numbers arranged in a particular order, which can be finite or infinite. In MATLAB, sequences can take various forms, including:
- Numerical Sequences: Series of numbers spaced uniformly (e.g., arithmetic sequences).
- Random Sequences: Series generated using random functions or commands.
Understanding Sequence Centroid
The sequence centroid refers to the average point of a sequence. Mathematically, it is often calculated as the arithmetic mean of all values in the sequence, providing a measure of the data's central tendency. This distinction between the mean and centroid is essential; while the mean considers all data points equally, the centroid can serve different kinds of sequences and dimensions.

Calculating the Sequence Centroid in MATLAB
MATLAB Commands for Centroid Calculation
When computing centroids, various MATLAB functions come into play. The most straightforward way is to use the `mean()` function, which computes the averages of the values in a vector or matrix.
Step-by-Step Code Example
To demonstrate the calculation of a sequence centroid, consider a simple numerical sequence. The following MATLAB code snippet shows how to find the centroid of a sequence:
% Example: Calculating the centroid of a numeric sequence
sequence = [1, 2, 3, 4, 5];
centroid = mean(sequence);
fprintf('The centroid of the sequence is: %f\n', centroid);
In this code:
- The variable `sequence` holds the numbers.
- The `mean()` function calculates the average, which serves as the centroid, and the result is displayed using `fprintf()`.
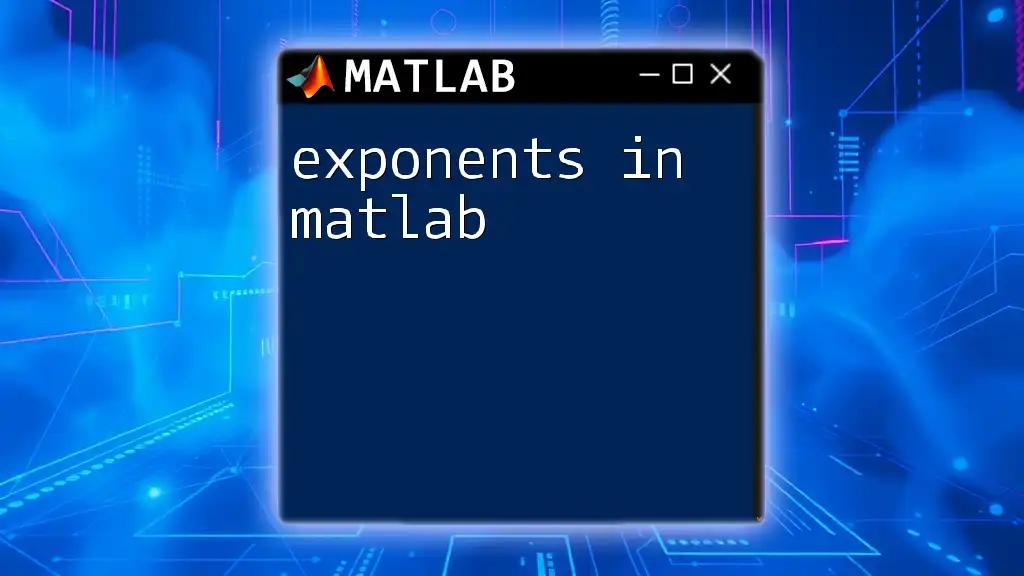
Visualizing Sequence Centroid
Importance of Visualization
Visual representation plays a crucial role in comprehending the concept of centroids. It facilitates the identification of patterns and can highlight the relationship between data points and their centroid.
Creating Plots in MATLAB
Utilizing MATLAB's plotting capabilities is essential for effective visualization. The `plot()` command can help illustrate the sequence and its centroid clearly.
Example of Sequencing Plots
To visualize both the sequence and its centroid, consider the following MATLAB code snippet:
% Visualizing a Sequence and its Centroid
sequence = [1, 2, 3, 4, 5];
centroid = mean(sequence);
plot(sequence, '-o', 'DisplayName', 'Sequence');
hold on;
xline(centroid,'r--', 'Centroid','LabelHorizontalAlignment','left');
title('Sequence and its Centroid');
xlabel('Index');
ylabel('Value');
legend;
grid on;
hold off;
In this code:
- The sequence is plotted as a line with markers (-o).
- The centroid is indicated with a vertical dashed line for easy visualization.
- Labels and legends enhance interpretation, making the plot user-friendly.
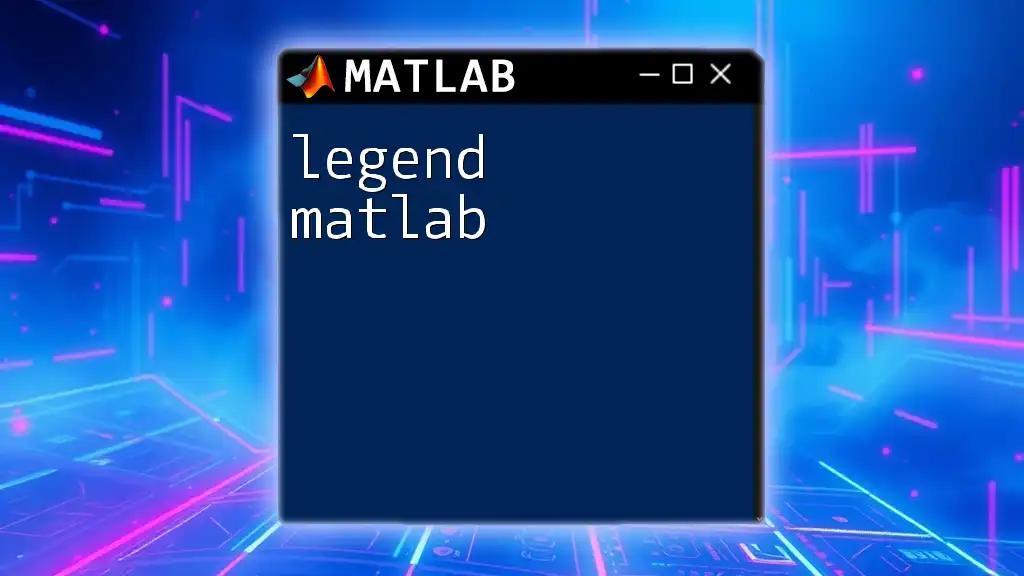
Advanced Topics in Sequence Centroids
Multi-Dimensional Sequences and Centroid Calculation
When delving into more complex data sets, you may encounter multi-dimensional sequences. These sequences can contain multiple values across different dimensions, necessitating a different approach for calculating centroids.
Code Example for Calculating Centroid in 2D
To compute the centroid of a 2D sequence, aim for the average across each dimension. Here’s an example:
% Example: Centroid of a 2D sequence
sequence2D = [1, 2; 3, 4; 5, 6];
centroid2D = mean(sequence2D);
fprintf('The centroid of the 2D sequence is: (%f, %f)\n', centroid2D(1), centroid2D(2));
In this code:
- A matrix represents the 2D sequence.
- The centroid is calculated along each dimension, offering a comprehensive view of the data's center.
Applications in Machine Learning
In machine learning, centroids are instrumental in clustering algorithms like K-means. They help define the center of a cluster, which can significantly affect the effectiveness of classification and clustering tasks.
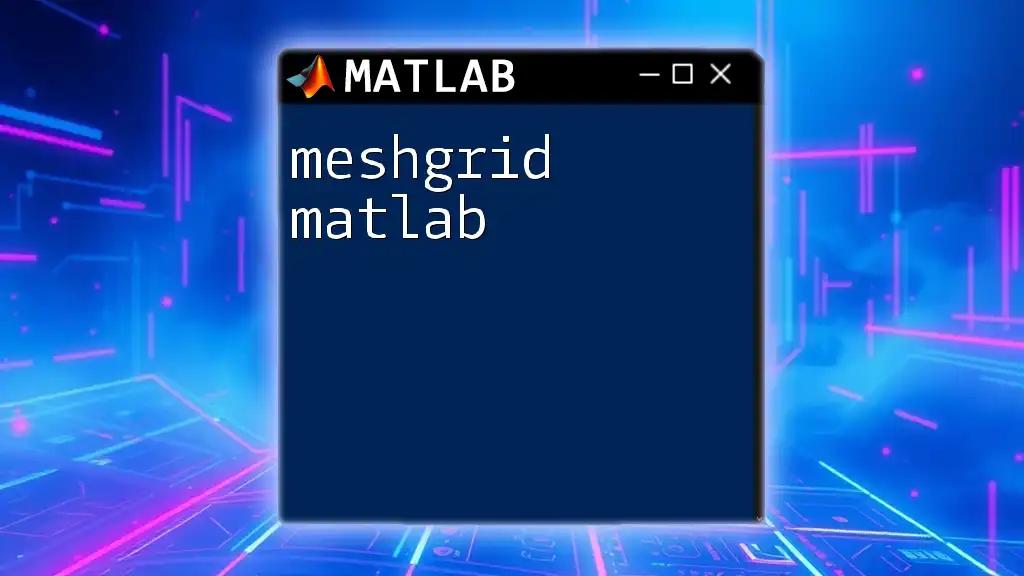
Tips and Best Practices
Code Optimization
Optimizing MATLAB code enhances performance, especially when working with large datasets. Use vectorized operations instead of loops when possible, and explore built-in functions that can handle complex calculations efficiently.
Debugging Techniques
When faced with errors during centroid calculations, employ useful MATLAB debugging commands such as `dbstop`, `disp`, and `error()`. These tools can help identify and rectify issues effectively, ensuring your centroid calculations are accurate.
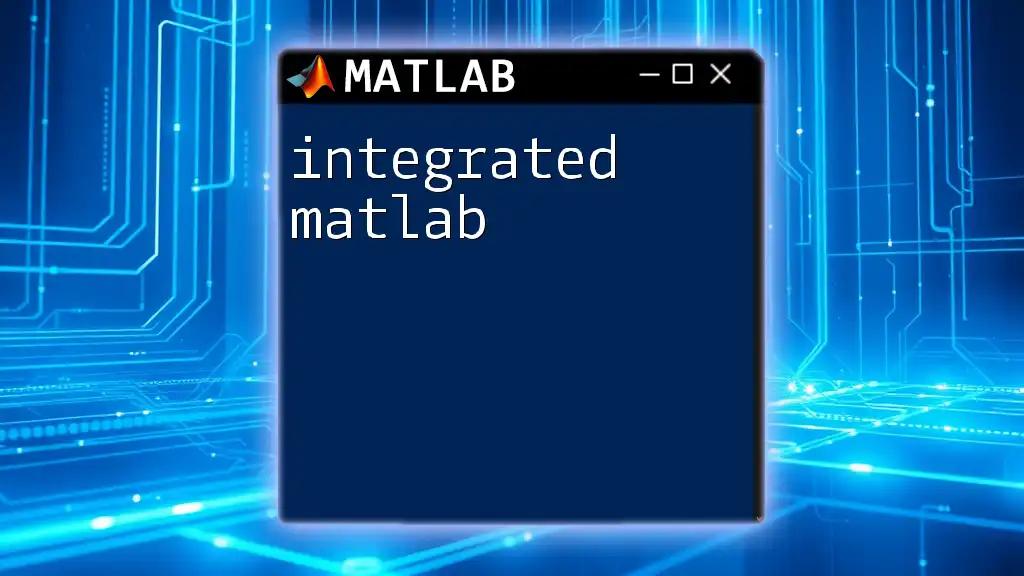
Conclusion
Throughout this article, we've explored the concept of sequence centroid in MATLAB, from its calculation to its visualization and application in advanced topics. By grasping these key points, you are well-equipped to utilize centroids in practical scenarios and deepen your understanding of MATLAB.
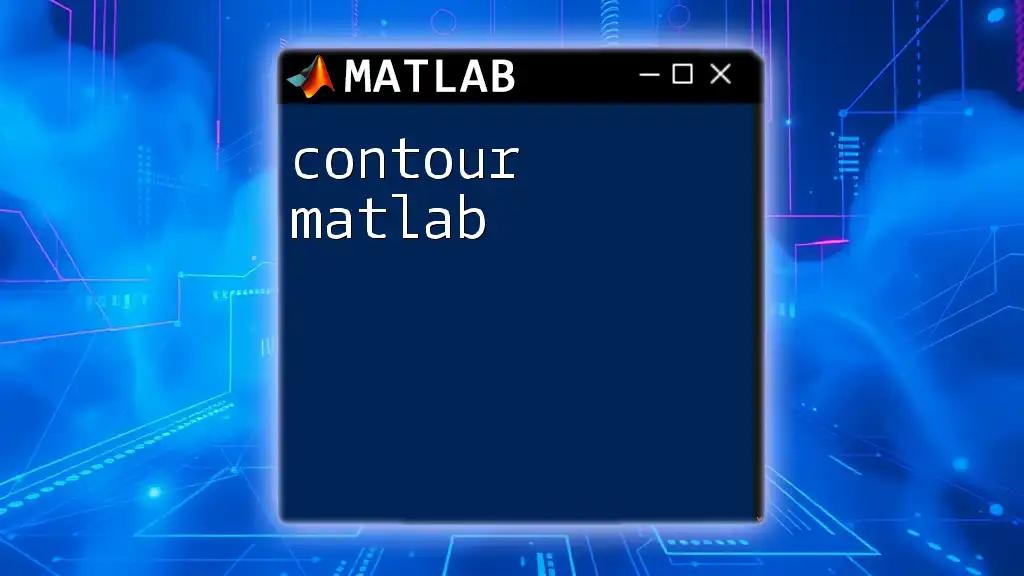
Call to Action
If you're eager to amplify your MATLAB skills further, consider joining our comprehensive training classes, designed to guide you through practical applications and advanced techniques in MATLAB. Explore firsthand testimonials from learners who have successfully boosted their proficiency with our tailored programs!