To solve a system of linear equations in MATLAB, you can use the backslash operator (`\`), which provides a simple and efficient way to compute the solution of the equation \(Ax = b\), where \(A\) is the coefficient matrix and \(b\) is the constants vector.
A = [2, 1; 1, 3]; % Coefficient matrix
b = [8; 10]; % Constants vector
x = A \ b; % Solve for x
Understanding Linear Equations
Linear equations are fundamental mathematical constructs that describe relationships between variables. A linear equation can typically be expressed in the form:
\[ ax + by = c \]
Where \( a \), \( b \), and \( c \) are constants, and \( x \) and \( y \) are the variables we wish to solve for. In linear algebra, these equations can be represented in matrix form as:
\[ Ax = b \]
In this representation, A is the coefficient matrix, x is the vector of variables, and b is the constants vector on the right side of the equations.
Applications of Solving Linear Equations
Solving systems of linear equations has widespread applications across various fields such as:
- Engineering: Structural analysis, control systems, circuit analysis.
- Data Science: Linear regression models, optimization problems.
- Economics: Market equilibrium models, resource allocation.
By understanding how to represent and solve these equations, you open the door to a plethora of real-world solutions.
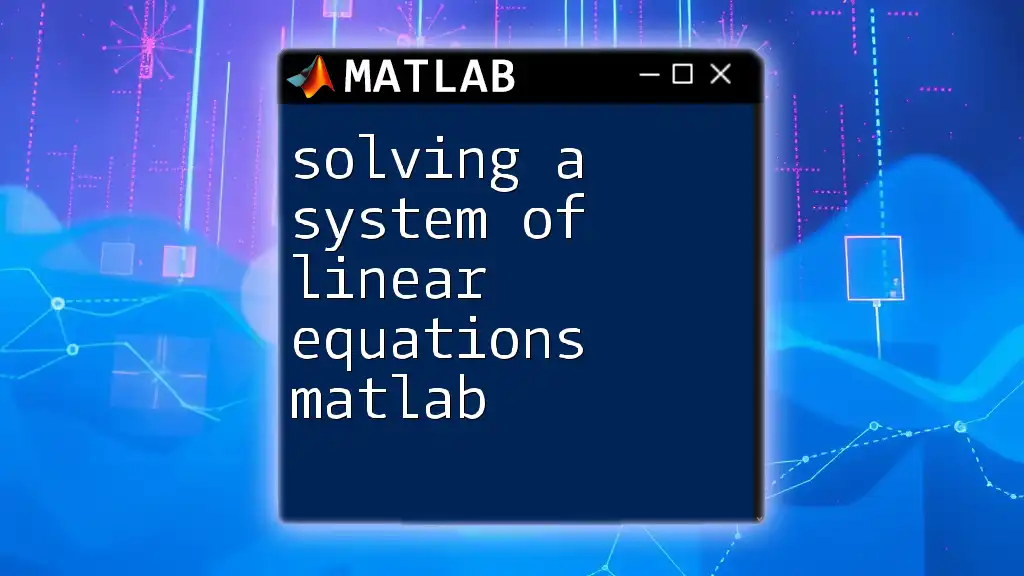
Basics of MATLAB
MATLAB, short for Matrix Laboratory, is a high-performance programming environment primarily used for numerical computation, visualization, and programming. It provides an array-oriented platform, making operations on matrices seamless and efficient.
MATLAB Environment
Familiarizing yourself with the MATLAB environment is crucial for effective coding. The key components include:
- Command Window: Where you can execute commands and view results directly.
- Workspace: Displays the variables currently in use, giving you a quick snapshot of your session.
- Editor: Allows you to write and save scripts for later use.
MATLAB Syntax
Proper syntax is fundamental in MATLAB. Every command adheres to specific formatting rules. Understanding how to execute commands, define variables, and manipulate matrices in this environment will set a strong foundation for solving linear equations effectively.
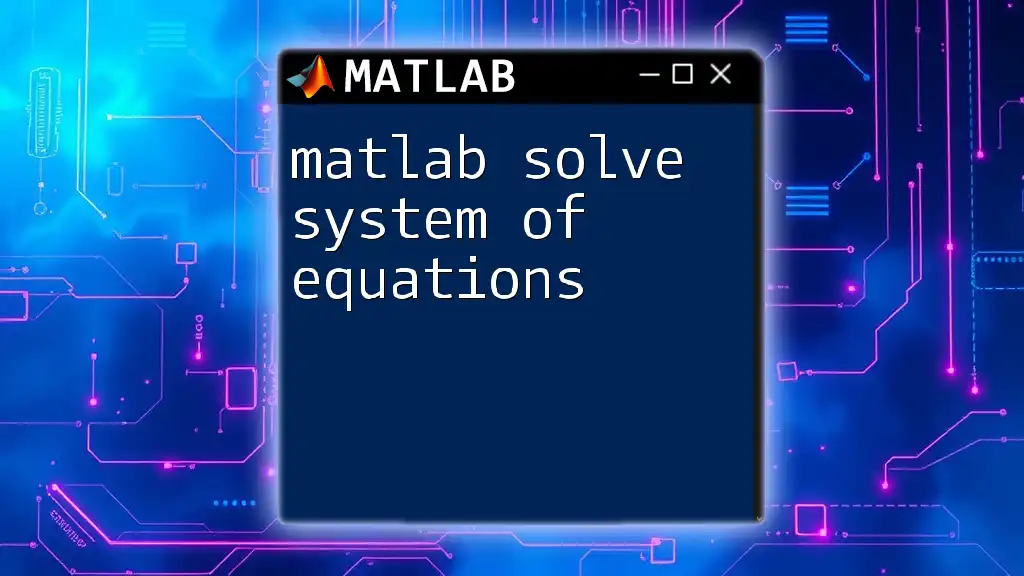
Setting Up a System of Linear Equations
To solve a system of linear equations, you first need to set it up in matrix form. Suppose you have two equations:
- \( 2x + y = 11 \)
- \( 5x + 3y = 28 \)
You can represent these equations in matrix form as follows:
Let
\[ A = \begin{bmatrix} 2 & 1 \\ 5 & 3 \end{bmatrix} \]
and
\[ b = \begin{bmatrix} 11 \\ 28 \end{bmatrix} \]
Here, Matrix A contains the coefficients of the variables, Matrix b contains the constants, and the vector x holds the unknowns \( x \) and \( y \).
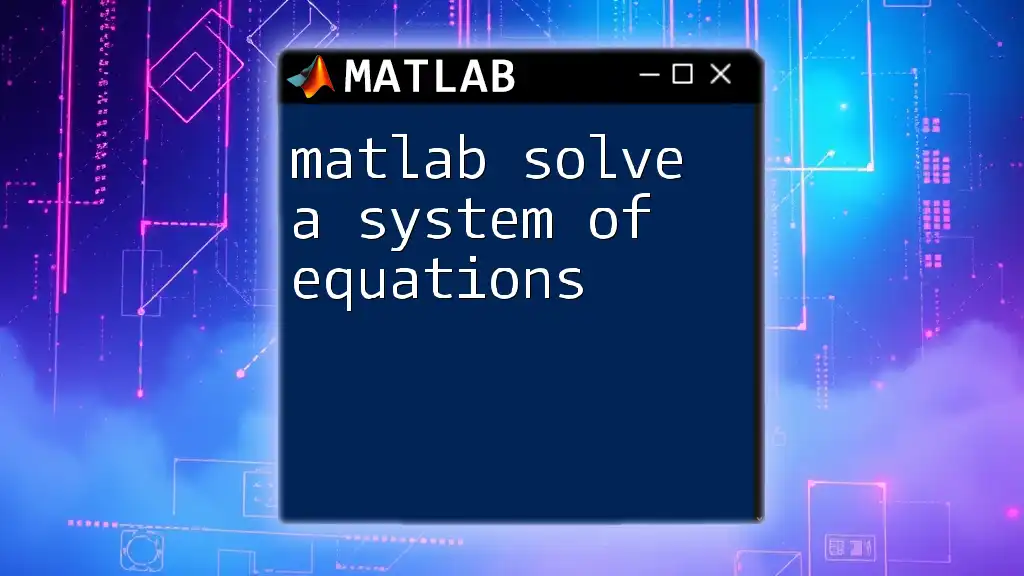
Methods to Solve Linear Equations in MATLAB
Using Backslash Operator
One of the most straightforward methods in MATLAB for solving a system of linear equations is using the backslash operator (`\`). This operator effectively finds a solution without explicitly calculating the inverse of the matrix.
Code Example:
A = [2, 1; 5, 3]; % Coefficient matrix
b = [11; 28]; % Constants matrix
x = A \ b; % Solving the system
disp(x); % Display the solution
Explanation: In this example, the backslash operator efficiently computes the values of \( x \) and \( y \) that satisfy the equations represented by matrices A and b. This method is computationally efficient, particularly for large systems.
Using the `inv()` Function
Another method is to calculate the inverse of matrix A and multiply it by vector b, though this approach is less favored due to numerical instability when A is poorly conditioned.
Code Example:
A = [2, 1; 5, 3]; % Coefficient matrix
b = [11; 28]; % Constants matrix
x = inv(A) * b; % Solving the system
disp(x); % Display the solution
Explanation: In this snippet, we first calculate the inverse of matrix A and then multiply it by vector b to find the solution. While this method works, it's essential to understand that calculating the inverse can lead to round-off errors, making the backslash operator a better choice in most cases.
Using LU Decomposition
LU decomposition is a method that breaks down matrix A into a lower triangular matrix (L) and an upper triangular matrix (U) to solve the equations efficiently.
Code Example:
A = [2, 1; 5, 3]; % Coefficient matrix
b = [11; 28]; % Constants matrix
[L, U] = lu(A); % Decomposing the matrix
y = L \ b; % Solving Ly = b
x = U \ y; % Solving Ux = y
disp(x); % Display the solution
Explanation: LU decomposition allows for two sequential substitutions — first solving for \( y \) and then solving for \( x \). This is particularly useful for larger systems as it reduces the computational complexity.
Using `linsolve()` Function
The `linsolve()` function is another powerful tool for directly solving linear equations. It is optimized for performance and provides an easy-to-use interface when compared to manual methods.
Code Example:
A = [2, 1; 5, 3]; % Coefficient matrix
b = [11; 28]; % Constants matrix
x = linsolve(A, b); % Directly solving the system
disp(x); % Display the solution
Explanation: This function offers a concise way to achieve the solution while ensuring computational efficiency. It is often recommended for users dealing with complex or large systems of equations.
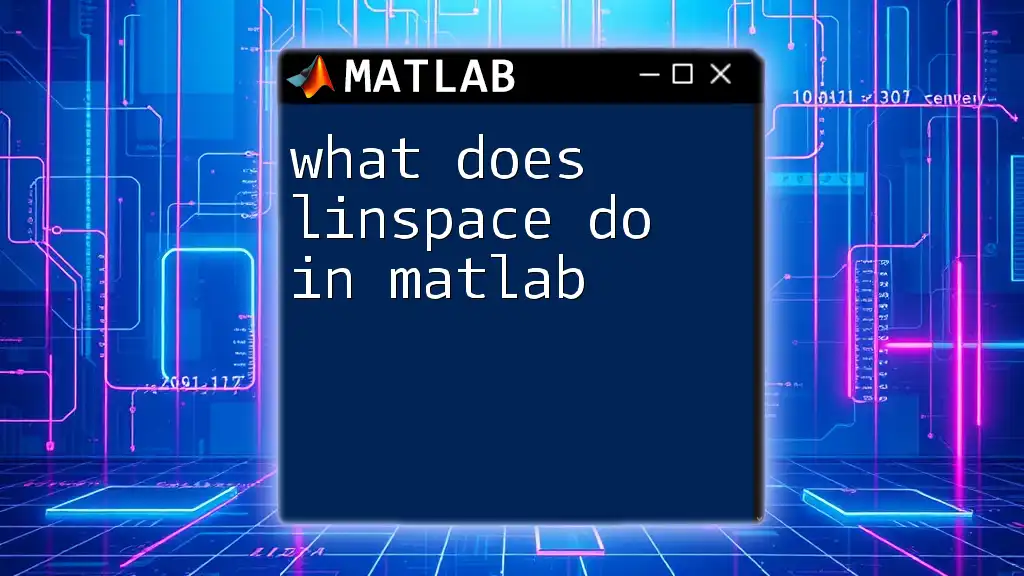
Verifying Solutions
After solving the equations, it is crucial to check the results to ensure accuracy. You can do this by substituting the computed values back into the original equations.
Code Example:
check = A * x; % Should equal b
disp(check); % Display the result
Interpreting the Results: The computed product should equal the constants matrix b. If the values align, the solution is verified; if not, re-evaluate the previous steps for potential errors.
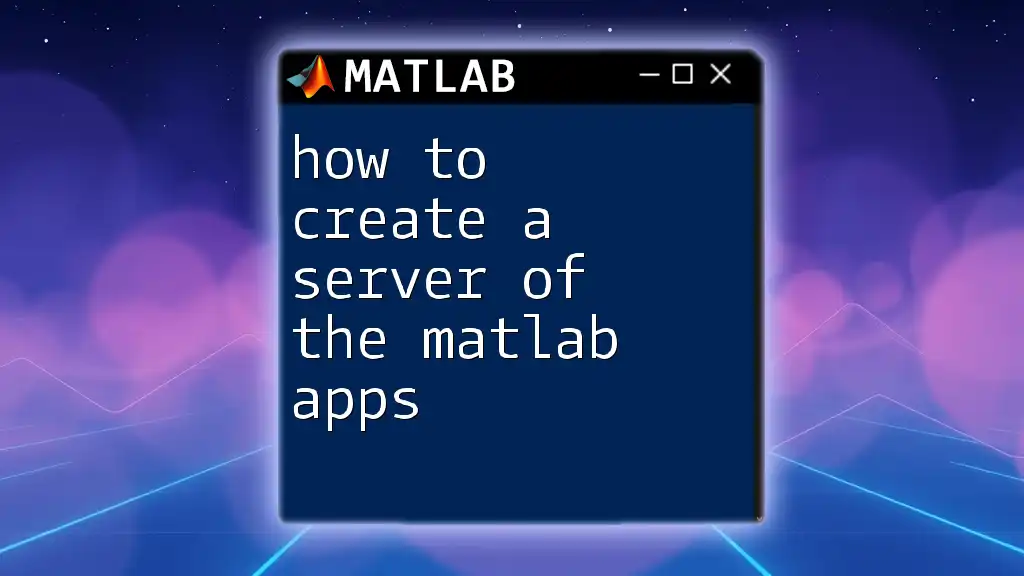
Conclusion
In summary, mastering solving a system of linear equations in MATLAB involves understanding both the theoretical aspects of linear algebra and the practical tools provided by MATLAB. From the simplicity of the backslash operator to the robustness of LU decomposition and the usability of `linsolve()`, each method offers unique advantages. As you gain experience, practice solving various systems will only enhance your proficiency in MATLAB.
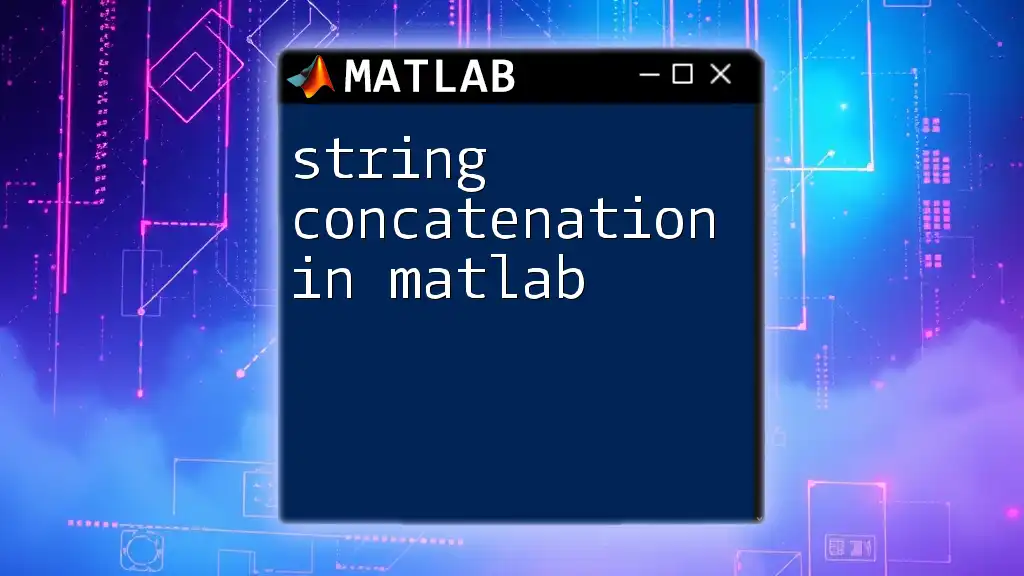
Additional Resources
To deepen your understanding, consider exploring recommended readings on linear algebra, participating in online forums, and engaging with MATLAB's extensive documentation. These resources can provide further insights and practical examples to enrich your learning experience.