In MATLAB, string concatenation is the process of combining two or more strings into a single string using square brackets or the `strcat` function.
Here's a simple example of string concatenation using both methods:
% Using square brackets
str1 = 'Hello, ';
str2 = 'world!';
result1 = [str1, str2];
% Using strcat function
result2 = strcat(str1, str2);
Understanding Strings in MATLAB
In MATLAB, strings are essential data types for representing text. Understanding how to manipulate these strings is crucial for data analysis, user interaction, and various programming tasks.
Difference Between Character Arrays and String Arrays
In MATLAB, there are two primary types for handling strings:
-
Character Arrays: These are sequences of characters enclosed in single quotes. They are treated as arrays of individual characters.
For example:
charArray = 'Hello, World!';
-
String Arrays: Introduced in newer MATLAB versions, these are enclosed in double quotes and are more flexible, allowing for easier manipulation and built-in functions.
Example:
stringArray = "Hello, World!";
Understanding the differences between these two types is vital as they have different functions and behaviors when it comes to operations like string concatenation in MATLAB.
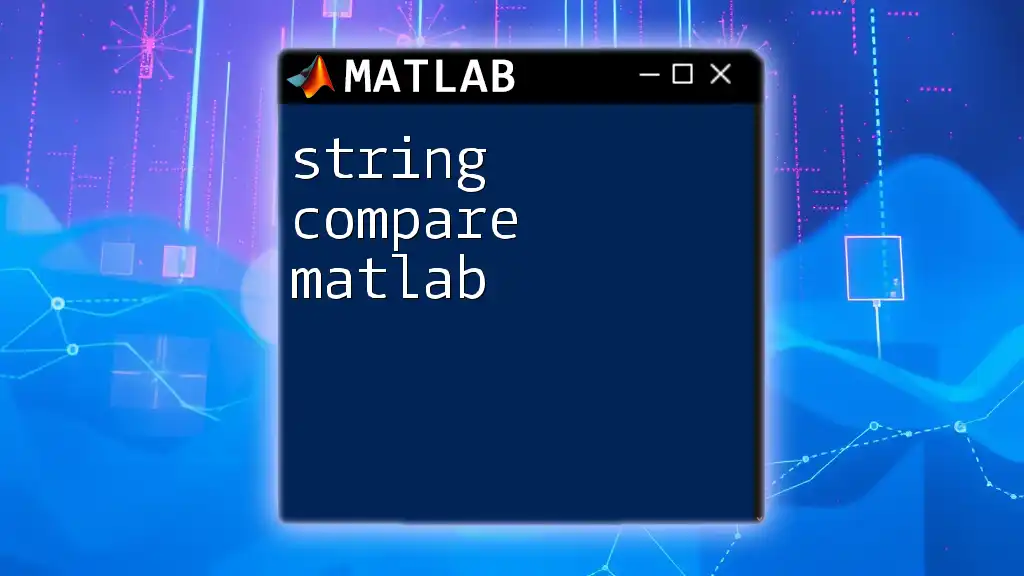
Methods for String Concatenation
Using Square Brackets
One of the simplest methods for concatenating strings in MATLAB is using square brackets. This method works seamlessly for character arrays.
str1 = 'Hello, ';
str2 = 'World!';
combinedStr = [str1, str2]; % Concatenating character arrays
disp(combinedStr); % Output: Hello, World!
When using square brackets, MATLAB will automatically concatenate the two strings without any additional syntax. This is particularly useful for quick concatenation when working with a few strings.
Using the `strcat` Function
The `strcat` function is specifically designed for string concatenation. While it can be used for both character and string arrays, it does remove trailing whitespace from the input strings, which could alter your results.
Example:
str1 = 'MATLAB ';
str2 = 'Concatenation!';
combinedStr = strcat(str1, str2);
disp(combinedStr); % Output: MATLAB Concatenation!
Using `strcat` is advantageous when you need to concatenate multiple strings at once, and it provides a cleaner syntax for complex concatenation tasks.
Using the `append` Function
The `append` function simplifies the concatenation process, especially when working with string arrays.
str1 = "Learning ";
str2 = "MATLAB!";
combinedStr = append(str1, str2);
disp(combinedStr); % Output: Learning MATLAB!
This function allows you to concatenate with a more intuitive format, making it easier for beginners to grasp the concept of string concatenation in MATLAB.
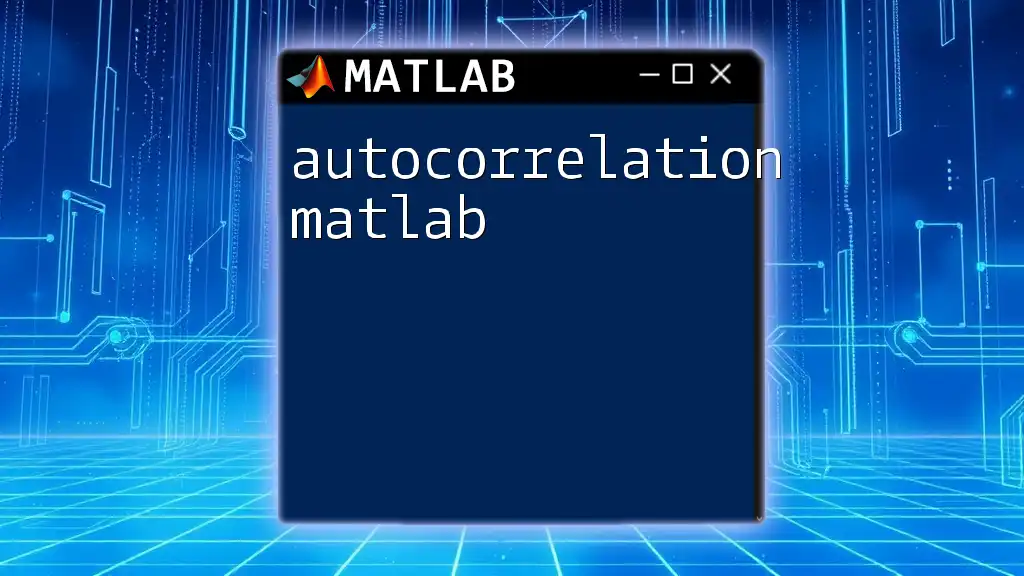
Handling Edge Cases in String Concatenation
Concatenating Empty Strings
When concatenating strings, it’s important to consider how MATLAB handles empty strings. Concatenating an empty string with text will simply result in the text.
Example:
emptyStr = '';
result = [emptyStr, 'Text'];
disp(result); % Output: Text
This shows that an empty string doesn’t affect the concatenation; the result remains unchanged.
Dealing with Whitespace and Special Characters
Spaces and special characters in string concatenation can sometimes lead to unexpected results. Always be explicit about how you handle spaces.
str1 = 'Hello,';
str2 = 'World!';
combinedStr = [str1, ' ', str2]; % Explicit space character
disp(combinedStr); % Output: Hello, World!
This example demonstrates how to include a space between concatenated strings, ensuring clear and accurate formatting, which is vital in constructing user-friendly output.
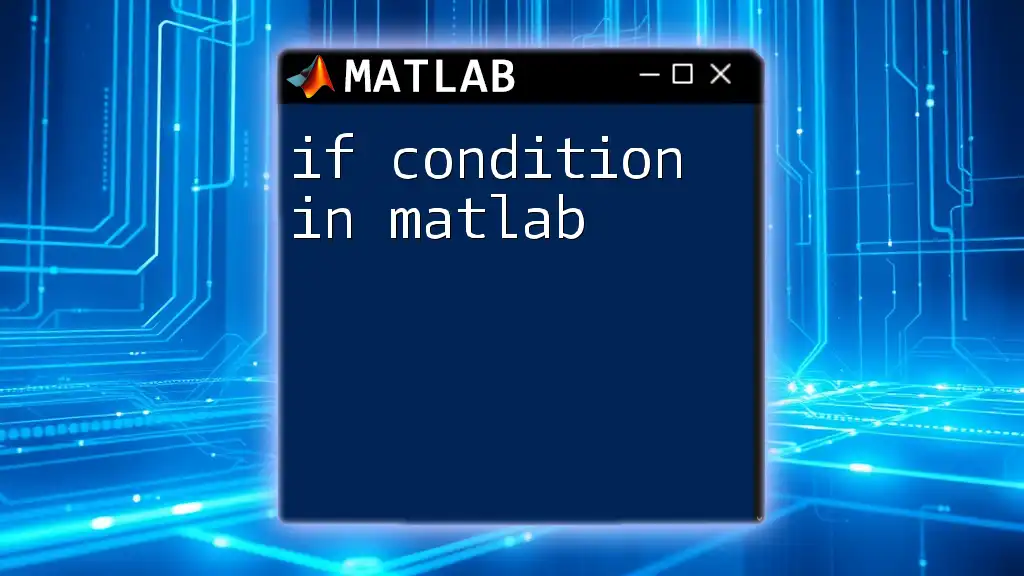
Performance Considerations
When utilizing string concatenation in loops or repeated operations, performance can become a concern due to memory management. It’s best to avoid concatenating strings directly in loops when possible, as this can lead to multiple memory allocations.
A better strategy is to preallocate space for your strings or use cell arrays for intermediate storage, improving performance and memory efficiency.
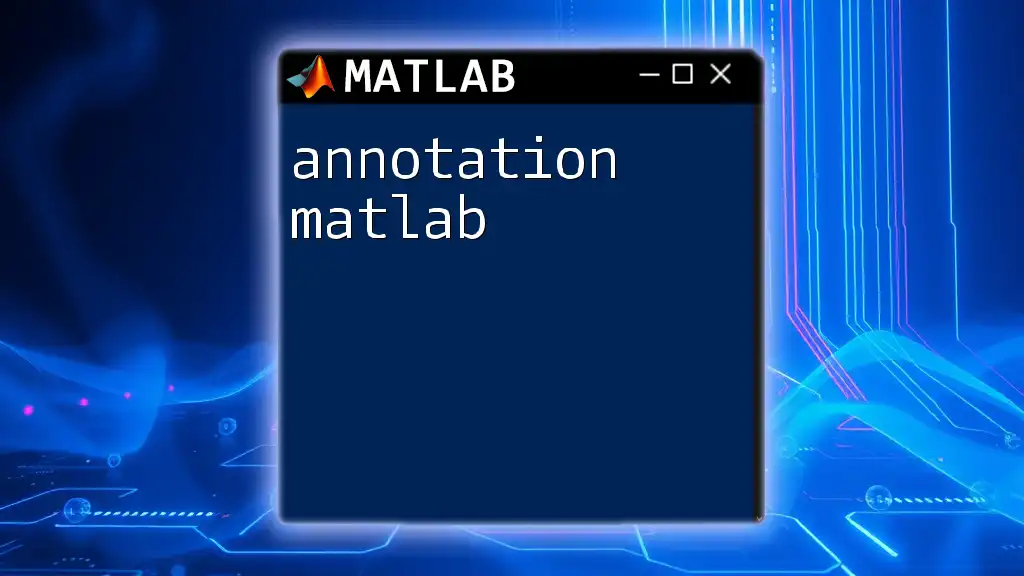
Real-World Applications of String Concatenation
Data Processing
String concatenation is often used in data manipulation tasks. For instance, when preparing data labels or concatenating parameters based on specific conditions.
names = ["Alice", "Bob", "Charlie"];
greeting = append("Hello, ", names);
disp(greeting); % Outputs greetings for multiple names
Using `append` allows you to construct dynamic messages for various datasets efficiently.
User Interaction
The ability to modularly build strings is particularly useful when greeting users or providing personalized prompts.
userName = input('Enter your name: ', 's');
personalizedGreeting = append('Welcome, ', userName, '!');
disp(personalizedGreeting);
This example dynamically incorporates user input into a greeting message, enhancing user experience in interactive applications.
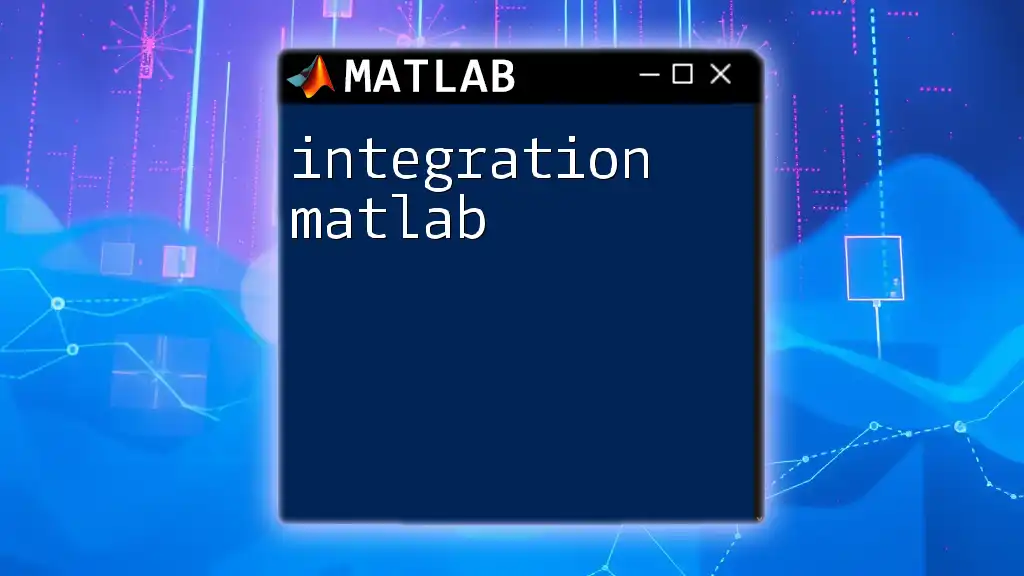
Common Errors and Troubleshooting
Errors can occur during string concatenation, especially regarding type mismatches. For example, attempting to concatenate incompatible data types can result in an error.
% Example of a size mismatch error
str1 = "MATLAB ";
str2 = ['R2023', 42]; % This will cause an error
To avoid such errors, ensure that all components being concatenated are either all string arrays or all character arrays. This alignment will safeguard against type mismatch issues and ensure smooth concatenation.
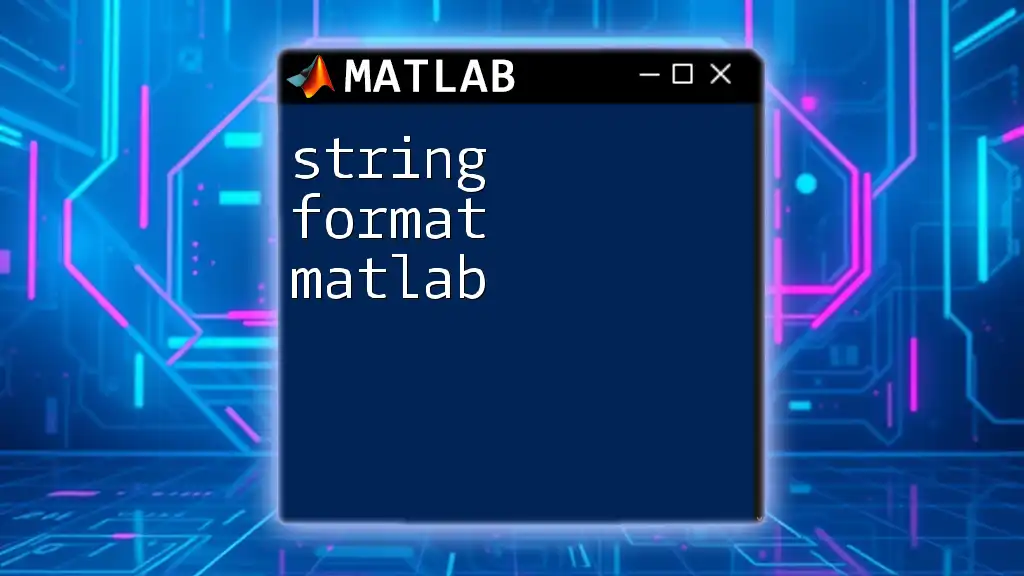
Conclusion
In summary, mastering string concatenation in MATLAB is crucial for effective string manipulation and programming. Whether using square brackets, `strcat`, or `append`, each method offers unique advantages depending on your specific needs. Practicing these techniques will enhance your programming skills and enable you to handle strings more efficiently in your projects. Exploring real-world applications and considering performance will prepare you to tackle various string manipulation challenges.
Take the time to experiment with these methods in your MATLAB environment, and don’t hesitate to reach out with questions or insights as you dive deeper into string concatenation!
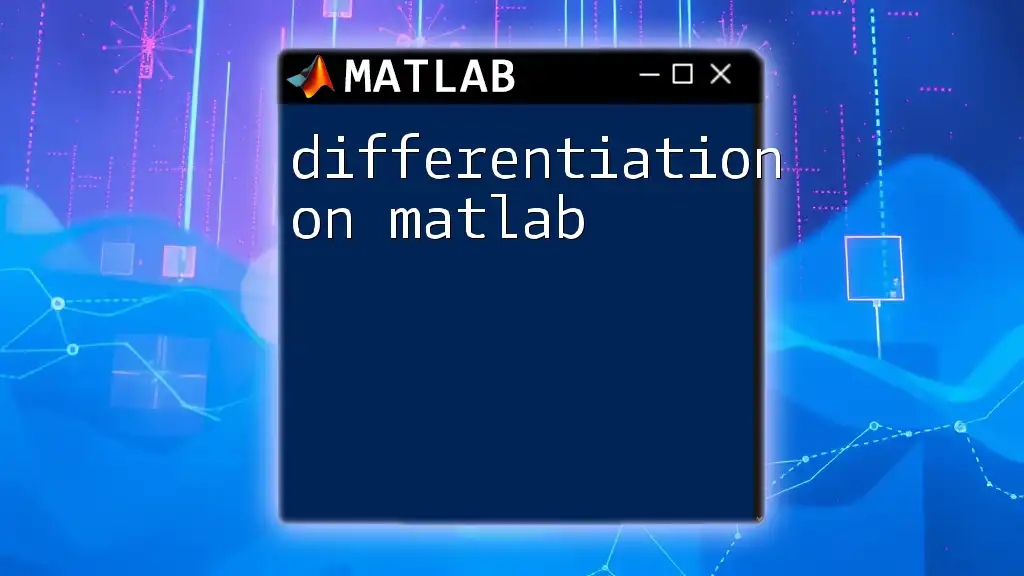
Additional Resources
For more comprehensive understanding and examples, you might consider checking out the official MATLAB documentation on strings and various supplementary resources and tutorials available online to strengthen your skills further.