The `sum` function in MATLAB is used to calculate the total of array elements along a specified dimension, returning the sum of the elements in the input array.
Here's a simple code snippet demonstrating its use:
% Example: Calculate the sum of elements in an array
A = [1, 2, 3, 4, 5];
total = sum(A);
disp(total); % Output will be 15
Understanding the SUM Function in MATLAB
What is the SUM Function?
The `sum` function in MATLAB is one of the most fundamental commands used for mathematical computations. It simplifies the process of aggregating data across arrays and matrices, allowing users to quickly obtain the total of specified elements. This function is particularly useful in data analysis, where summation frequently plays a critical role in deriving insights from datasets.
Syntax of the SUM Function
Understanding the syntax of the `sum` function is essential for its effective application. The basic syntax is as follows:
-
`Y = sum(A)`: This returns the sum of the elements in array A. If A is a vector, `Y` returns a scalar representing the total of the vector elements. If A is a matrix, `Y` will return a row vector containing the sum of each column.
-
`Y = sum(A, dim)`: This allows you to specify the dimension along which to operate. If dim is `1`, it sums down the columns. If dim is `2`, it sums across the rows.
-
`Y = sum(___, 'double')`: This use case is for ensuring that the output is of type double, which can help maintain precision when dealing with floating-point numbers.
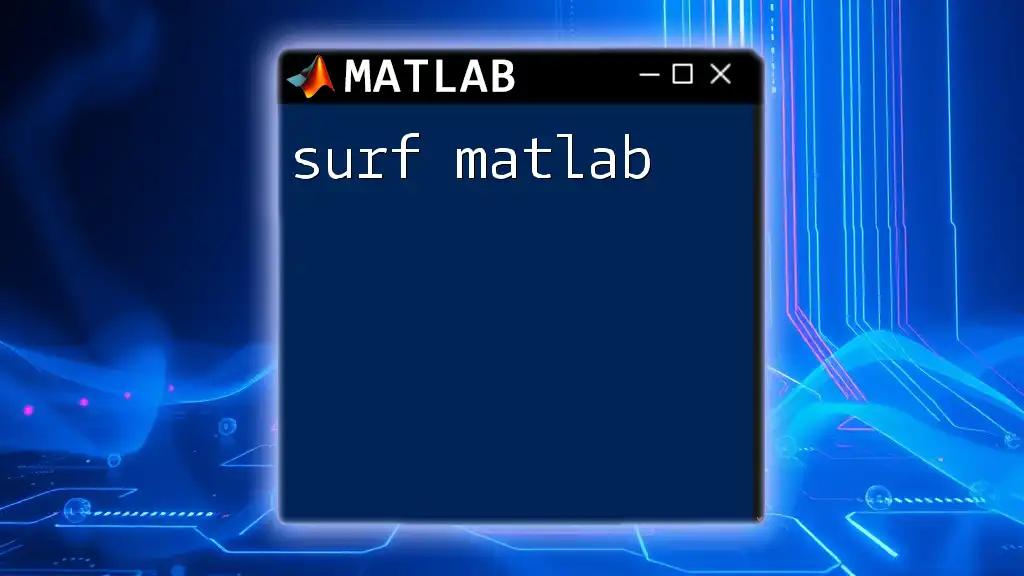
How to Use the SUM Function
Summing Elements in a Vector
Summing elements in a one-dimensional array is straightforward. For instance, if you have a vector containing numbers, you can quickly calculate the total.
vec = [1, 2, 3, 4, 5];
total = sum(vec);
disp(total);
In this example, the expected output will be 15, as it adds up all the elements: 1 + 2 + 3 + 4 + 5.
Summing Rows and Columns in a Matrix
Summing Columns
When working with matrices, you can easily sum the elements in each column. Consider the following example:
mat = [1, 2, 3; 4, 5, 6];
col_sum = sum(mat);
disp(col_sum);
Here, the output will be [5, 7, 9], as it sums each column separately:
- First column: 1 + 4 = 5
- Second column: 2 + 5 = 7
- Third column: 3 + 6 = 9
Summing Rows
Similarly, if you want to sum along the rows, you can specify this in the command:
row_sum = sum(mat, 2);
disp(row_sum);
In this case, the output will be [6; 15], corresponding to:
- First row: 1 + 2 + 3 = 6
- Second row: 4 + 5 + 6 = 15
Summing Higher-Dimensional Arrays
The `sum` function can also handle multidimensional arrays. This is useful when working with data that is organized across multiple levels of dimension. Here’s an example of summing a 3D array:
arr = rand(3, 4, 5); % Creating a 3D array
total_sum = sum(arr, [1, 2]);
disp(total_sum);
In this example, summing across the first and second dimensions will yield a 1x5 result, which consolidates the data along those dimensions into a single dimension containing the total for each slice.
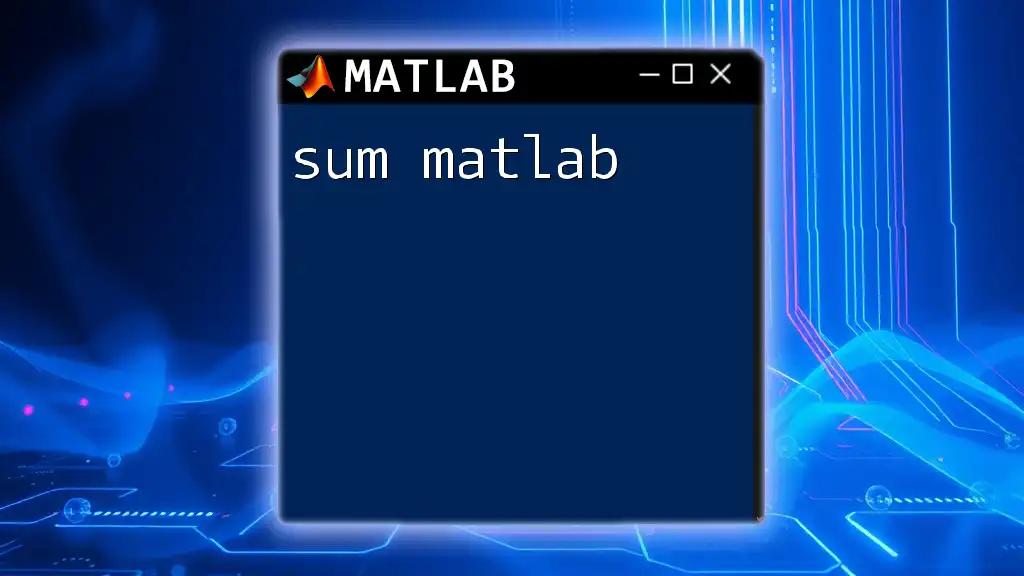
Advanced Features of the SUM Function
Using the 'omitnan' Option
Handling NaN (Not a Number) values is crucial when analyzing datasets, as they can skew results. The `omitnan` option allows the `sum` function to ignore NaN values in its calculations.
vec_with_nan = [1, NaN, 3];
total_omit_nan = sum(vec_with_nan, 'omitnan');
disp(total_omit_nan);
The output will be 4 (1 + 3), while ignoring the NaN value.
Summing with Custom Functions
MATLAB allows the use of anonymous functions with `sum`, which enables users to perform custom calculations on their data. For example, you may wish to sum the squares of the elements:
custom_sum = @(x) sum(x.^2);
result = custom_sum(vec);
disp(result);
This code will yield 55, as it calculates \(1^2 + 2^2 + 3^2 + 4^2 + 5^2\).

Practical Applications of the SUM Function
Data Analysis
In exploratory data analysis, the `sum` function plays a vital role. For instance, summing sales data over a particular period can help businesses determine their performance. Analysts use this simple command to derive meaningful insights from large datasets, leading to better-informed business decisions.
Engineering and Computational Problems
In fields such as engineering, the sum function is often used in simulations and calculations of physical systems. If you need to calculate the total force acting on an object with multiple force vectors, you can use the `sum` function to aggregate these forces efficiently.
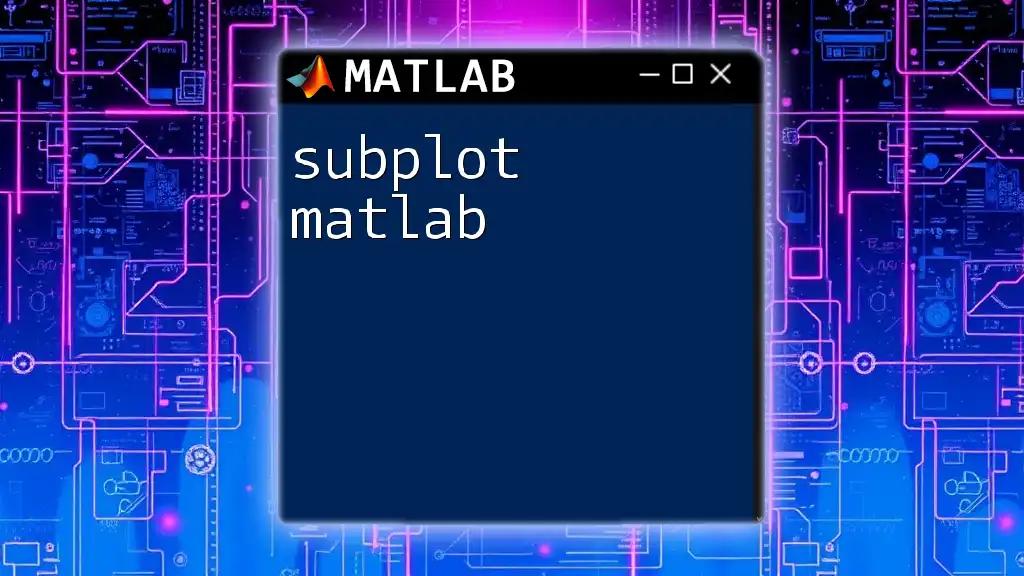
Common Pitfalls and Best Practices
Avoiding Common Errors
A common mistake when using the `sum` function arises when applying it to multidimensional arrays without specifying the dimension properly. This oversight can lead to unexpected results. For example, summing a 2D array without specifying the dimension will yield a single row vector containing the sums of each column.
Best Practices
To ensure efficient and correct use of the `sum` function:
- Always validate the dimensionality of your data before applying the sum function.
- Take advantage of vectorization, as MATLAB is optimized for working with vectors and matrices. This leads to faster computations and cleaner code.
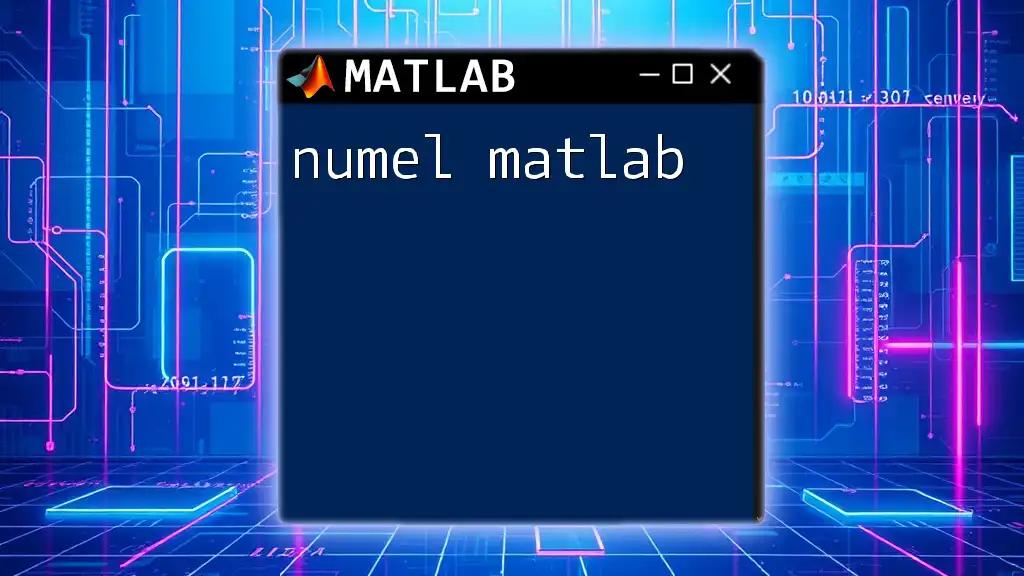
Conclusion
In this comprehensive guide, we explored the sum of MATLAB in detail, from its basic usage to advanced features and practical applications. The `sum` function is an essential tool for anyone working in data analysis, engineering, or computational fields, making it imperative to master. We encourage you to practice these commands and explore further resources to enhance your skills with MATLAB.