Yes, MATLAB can pull files from a server using the WinSCP scripting interface by executing a system command that calls WinSCP with the appropriate parameters. Here's a code snippet illustrating how to achieve this:
system('winscp.com /command "open sftp://username:password@server.com" "get /remote/path/file.txt C:\local\path\file.txt" "exit"');
Understanding WinSCP
What is WinSCP?
WinSCP is a popular open-source SFTP and FTP client for Windows, designed to securely transfer files between local and remote systems using several protocols. Its main features include:
- Graphical User Interface: Allows for intuitive file management.
- Automation through Scripting: Supports scriptable commands for repetitive tasks, making it ideal for regular file transfers.
- File Synchronization: Provides options for synchronizing files between directories.
- Transferring Files via SSH: Ensures security while accessing remote servers.
Installation and Setup
To get started, download and install WinSCP from its official website. The installation wizard will guide you through the setup process:
- Download WinSCP: Obtain the latest version from the WinSCP website.
- Run the Installer: Follow the prompts to complete the installation.
- Configure WinSCP for Server Access: Launch WinSCP and create a new session by entering your server credentials (hostname, username, and password). This will enable you to connect to your server effortlessly.
- Creating and Saving Session Settings: Save your session settings for easier access in the future, allowing for prompt connections without re-entering your credentials each time.
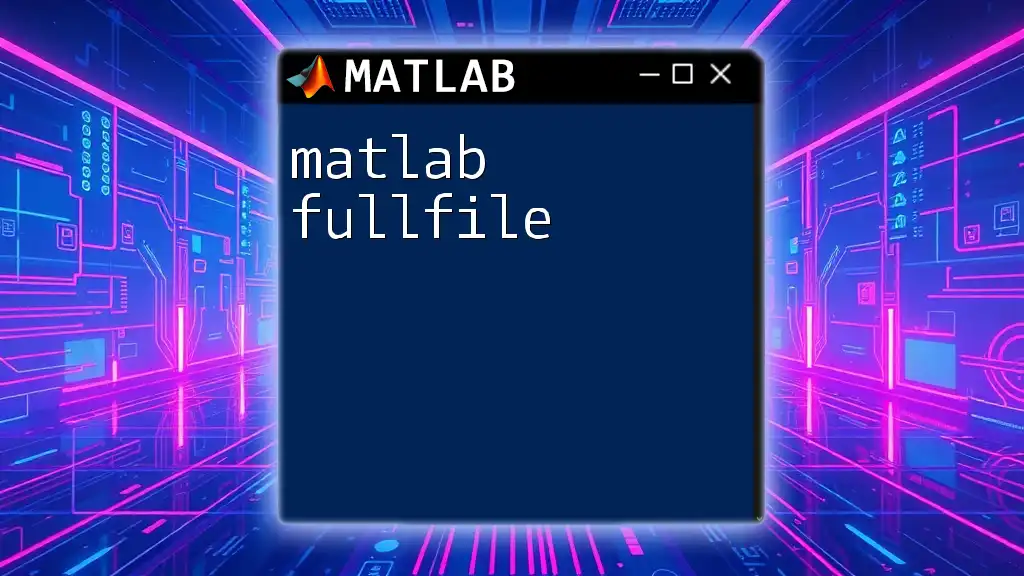
Integrating WinSCP with MATLAB
Why Use MATLAB for File Transfers?
Integrating WinSCP with MATLAB allows users to automate file transfers, enhancing workflow efficiency. For example, instead of manually transferring files each time, you can run a simple MATLAB script to handle the entire process. This can be particularly beneficial for datasets that require frequent updates or backups.
Required MATLAB Tools
To effectively use WinSCP from MATLAB, you need to utilize MATLAB's capability to run external programs. The key tool here is the `system` command, which allows you to execute operating system commands directly from your MATLAB environment.
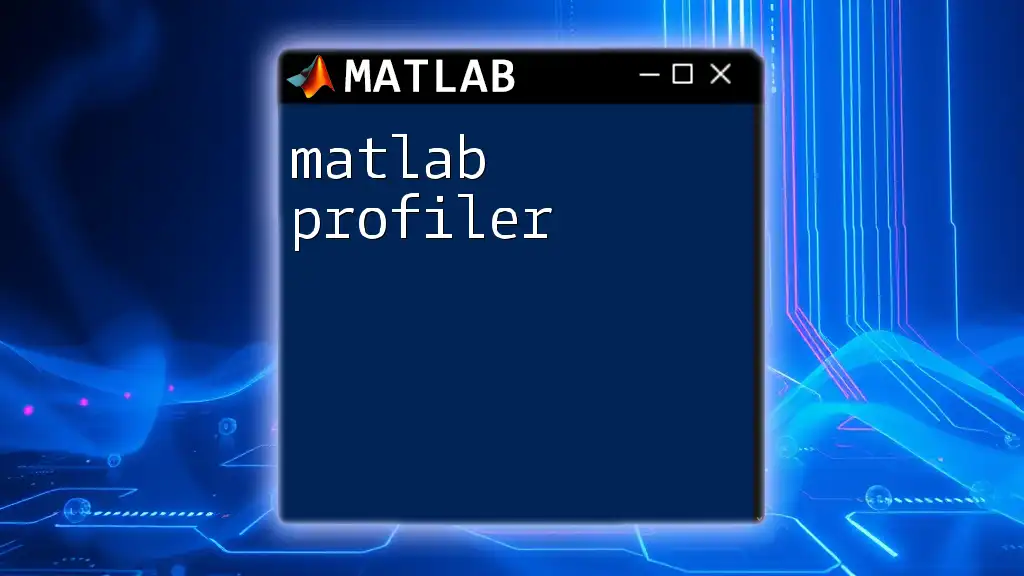
Configuring WinSCP for MATLAB Commands
Creating a WinSCP Script File
To initiate file transfers, you need to create a script for WinSCP. This script will define the operations you want to perform. Below is a simple example script that opens a connection to a server, retrieves a file, and then closes the connection:
open sftp://username:password@example.com
get /remote/path/file.txt C:\local\path\
close
exit
Each line of the script performs a specific task:
- open: Connects to the specified server using SFTP, where `username`, `password`, and `example.com` must be replaced with your actual credentials.
- get: Downloads the file from the specified remote path to your local directory.
- close: Terminates the server connection.
- exit: Closes the WinSCP application.
Saving and Executing WinSCP Scripts from MATLAB
Once you've created your script, you need to save it as a `.bat` file for execution. Here’s how you can set this up in MATLAB:
% Define paths
scriptPath = 'C:\path\to\yourscript.bat';
% Create WinSCP script and save to file
winscScript = sprintf('open sftp://username:password@example.com\nget /remote/path/file.txt C:\\local\\path\\\nclose\nexit');
fileID = fopen(scriptPath, 'w');
fwrite(fileID, winscScript);
fclose(fileID);
% Execute the script using MATLAB
status = system(sprintf('winscp.com /script=%s', scriptPath));
if status == 0
disp('File transferred successfully!');
else
disp('Error in file transfer.');
end
This MATLAB script saves your WinSCP commands and executes the command to transfer the file. It checks the `status`, indicating success or failure of the operation.
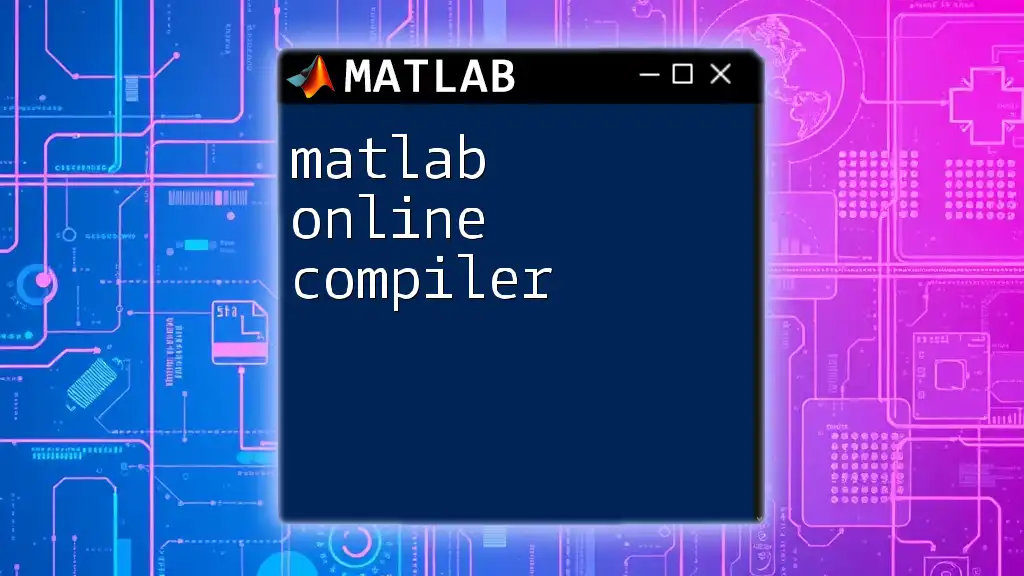
Handling File Paths in MATLAB
Understanding Local vs. Remote Paths
When setting up your file transfer commands, it’s crucial to differentiate between local and remote paths. Local paths refer to directories on your computer, while remote paths correspond to directories on the server. Being precise with these paths is vital; even a minor typographical error can lead to transfer failures.
Examples of Successful File Transfers
Here’s a more detailed example showcasing the entire process of using MATLAB to pull files from a server:
% Define local and remote paths
localPath = 'C:\local\path\';
remotePath = '/remote/path/';
% Create WinSCP script to transfer files
winscScript = sprintf('open sftp://username:password@example.com\nget %s*\nclose\nexit', remotePath);
fileID = fopen('transfer_script.txt', 'w');
fwrite(fileID, winscScript);
fclose(fileID);
% Execute the WinSCP script
status = system('winscp.com /script=C:\path\to\transfer_script.txt');
if status == 0
disp('Files transferred successfully!');
else
disp('Error in file transfer.');
end
In this example, the script uses a wildcard (`*`) to download all files from the specified `remotePath` to the `localPath`.
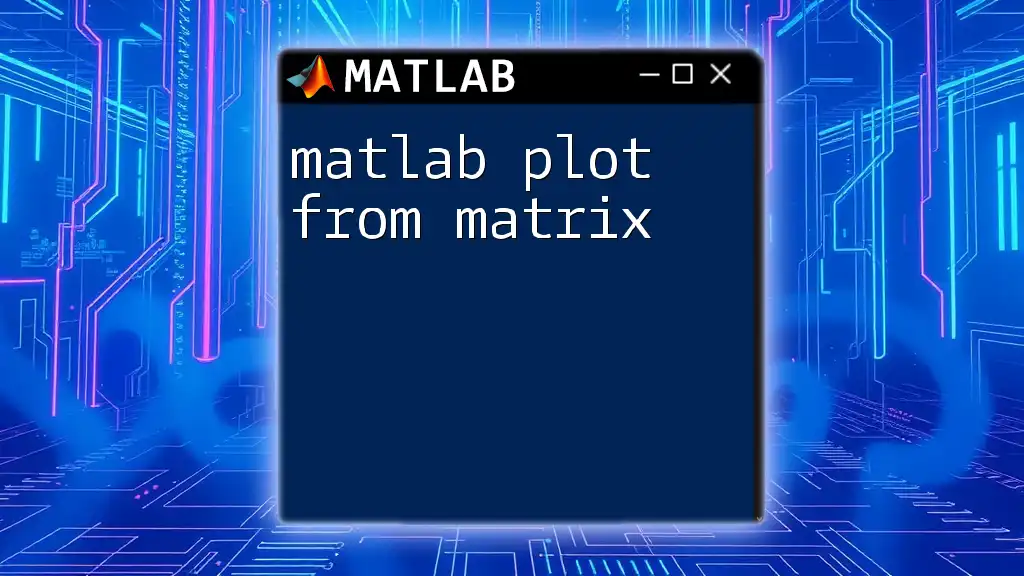
Error Handling and Troubleshooting
Common Issues When Using WinSCP with MATLAB
While integrating MATLAB with WinSCP, users may encounter several common issues:
- Authentication Errors: This usually stems from incorrect username or password. Always double-check these credentials.
- Incorrect File Paths: Ensure that both local and remote file paths are correct and formatted properly.
- Permissions Issues: Verify that you have adequate permissions on the server to access and download the files.
Tips for Debugging
To effectively troubleshoot issues, it’s essential to capture and review the WinSCP error logs. These logs provide detailed insights into what went wrong, allowing for quicker rectification. Additionally, check connectivity to the server using the WinSCP GUI to rule out any network-related issues.
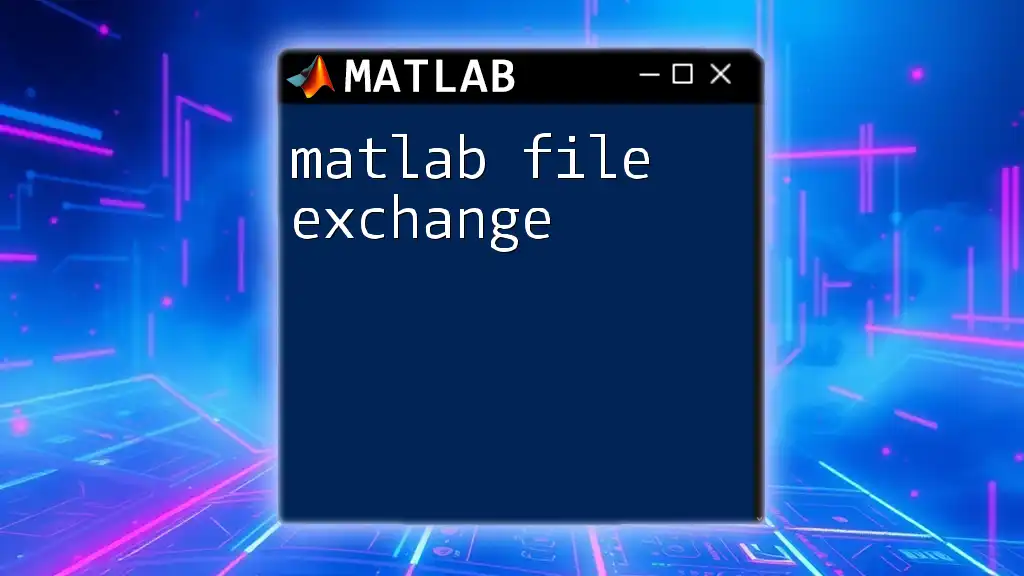
Best Practices for Script Management
Structuring Your MATLAB Script
Organizing your MATLAB scripts efficiently promotes better readability and maintainability. Using comments to describe each segment of your script will assist not only others but your future self when revisiting the code.
Regular Maintenance of WinSCP Sessions
To ensure smooth operation, regularly update your WinSCP session credentials and paths. Periodically test your scripts to confirm they function as expected and to catch any early signs of failure.
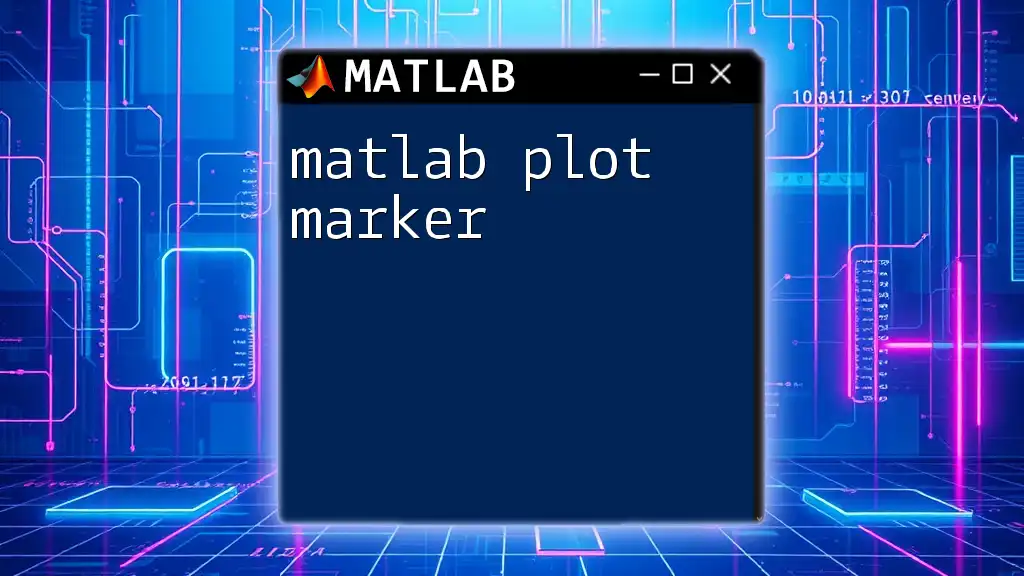
Conclusion
By leveraging the power of MATLAB in conjunction with WinSCP, users can streamline their file transfer processes. Not only does this integration save time, but it also minimizes the potential for human error in repetitive tasks.
Exploring more advanced features and automation techniques can further enhance your data management capabilities. If you're interested in deepening your understanding of MATLAB and learning about file transfer automation, consider enrolling in our courses for expert guidance.
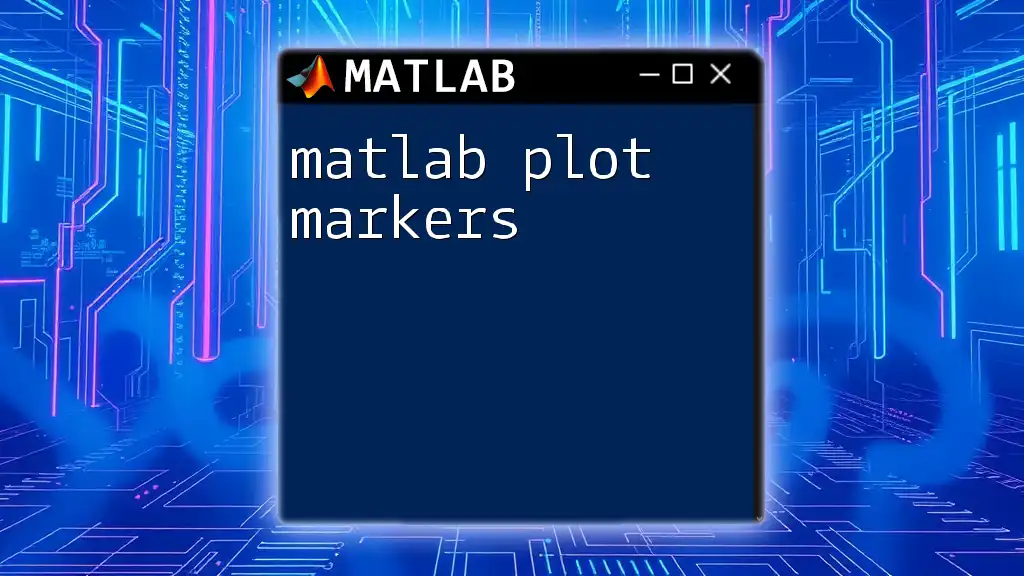
Additional Resources
Further Reading
To broaden your knowledge of MATLAB, consider exploring recommended books and articles that complement this topic. Additionally, the official WinSCP documentation will provide you with further insights into its capabilities.
Community and Support
Engaging with online forums and communities can be invaluable for troubleshooting and exchanging knowledge with other users. The dynamic nature of these platforms allows for continuous learning and support. For those eager to enhance their skills in MATLAB and automation, check out relevant online courses and resources.