The error "unrecognized function or variable" in MATLAB typically occurs when you attempt to use a variable or function that has not been defined or is misspelled.
Here’s an example of how this error can occur:
% Example of unrecognized variable causing an error
result = myVariable + 10; % If myVariable is not defined, it will trigger an error
Understanding the Error: "Unrecognized Function or Variable"
The error message "unrecognized function or variable" in MATLAB indicates that the program cannot find a specified function or variable within its current workspace or scope. This error can arise from multiple common mistakes, and understanding the underlying issues can save users a lot of time and frustration.
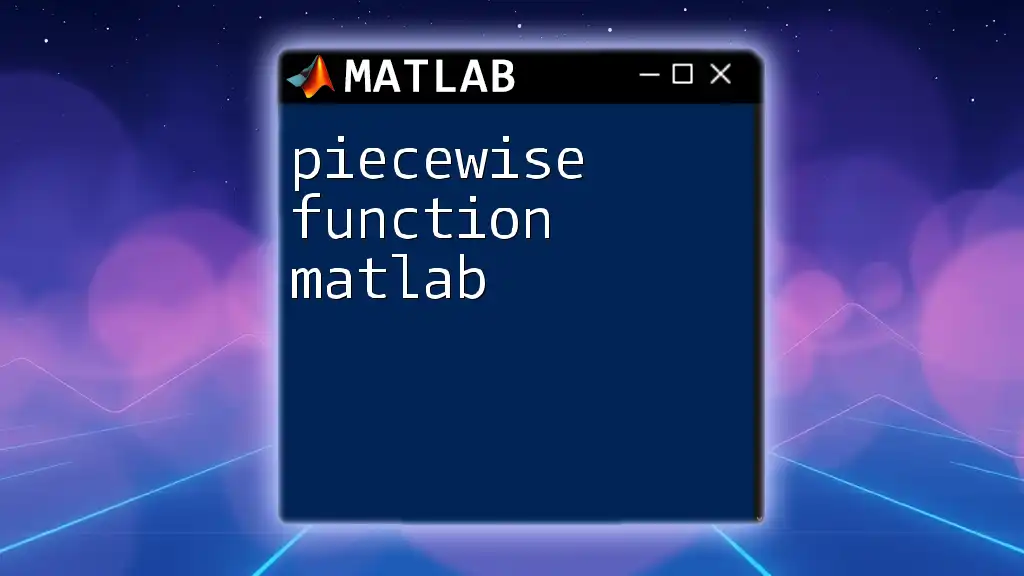
Common Causes of the Error
Typographical Errors
One of the most frequent culprits behind this error is simple typos. Even minor mistakes in spelling can lead to significant disruptions in your MATLAB script. For example:
result = summ(2, 3); % Incorrect function name
In this code snippet, the intended function `sum` is misspelled as `summ`, causing MATLAB to return the error. To avoid such issues, always check for spelling errors and pay attention to case sensitivity; MATLAB is case-sensitive.
Variable Scope Issues
Another common reason for encountering the "unrecognized function or variable" error is related to variable scope. In MATLAB, variables can have local or global visibility, which means they might not be accessible in certain contexts.
Consider the following example:
function myFunction()
a = 5;
end
myFunction();
disp(a); % Unrecognized variable 'a'
In this case, variable `a` is defined within the local scope of `myFunction` and cannot be accessed in the command window or any other function. The solution involves understanding variable scope dynamics. To share variables across different scopes, you can declare them as global variables:
global a; % Declare 'a' as a global variable
a = 5; % Now 'a' can be accessed elsewhere
Missing Files or Dependencies
Sometimes, the error emerges from missing files that house specific functions. If MATLAB cannot locate a file, it will flag the relevant functions as unrecognized. For instance:
result = myMissingFunction(1); % Function not found because of missing file
In this scenario, if `myMissingFunction` is not defined in any accessible file, MATLAB will produce the "unrecognized function or variable" message. To solve this issue, users should ensure that all necessary files are added to the MATLAB search path. The command to add a folder is:
addpath('C:\MyFolder'); % Add your folder path
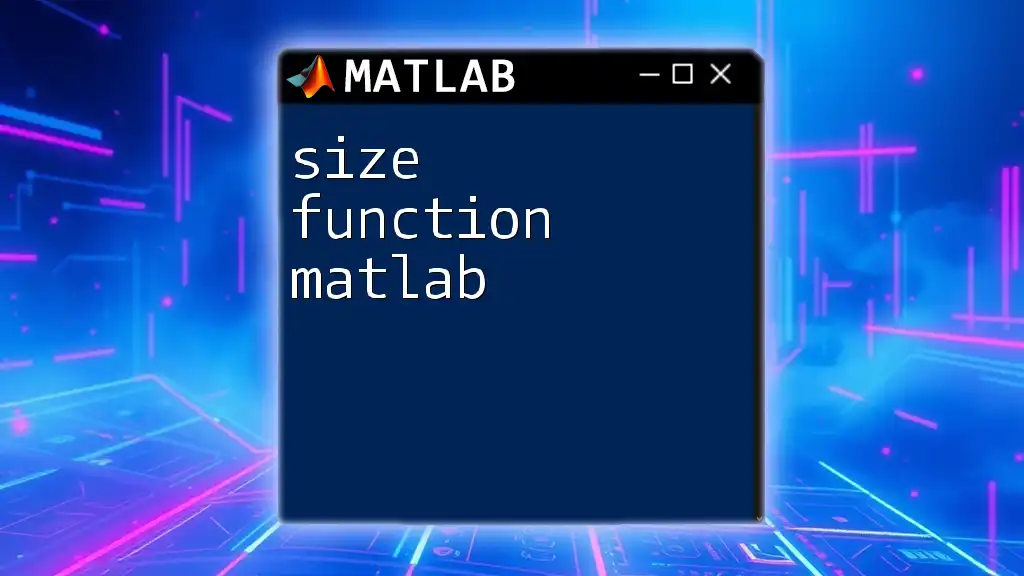
Troubleshooting the "Unrecognized Function or Variable" Error
Check for Typos
Always start troubleshooting by checking for typos. Verifying your spelling with precision can resolve many minor issues. Utilize MATLAB’s built-in function `which`, which shows the path of functions and variables:
which sum % This command will display the path of the 'sum' function
Verify Variable Initialization
A variable must be initialized before it can be used in calculations or display outputs. If you attempt to use an uninitialized variable, you'll encounter an error. For example:
% Correct initialization
b = 10; % Ensure 'b' is defined before using
disp(b);
To prevent this issue, ensure that all variables are assigned before usage. Initializing variables intuitively can help reduce the frequency of this error.
Use the MATLAB Debugger
The MATLAB debugger is a powerful tool that allows you to step through your code line-by-line, inspect variables, and identify problems. You can set breakpoints by clicking on the left margin of the editor next to the line numbers. This feature will halt execution, allowing you to check the status of variables at any point in time.
This process is invaluable when you are uncertain why a specific variable is unrecognized. It's often easier to identify scope-related problems or missing initializations using the debugger.
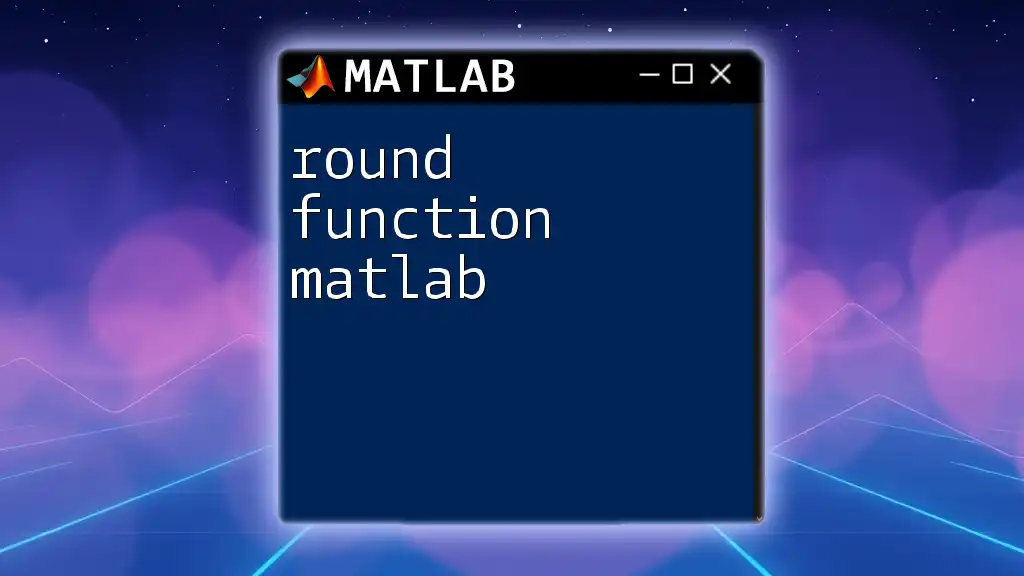
Practical Tips for Avoiding the Error
Naming Conventions
Implementing consistent naming conventions for your variables and functions can significantly reduce the chance of encountering unrecognized names. Opt for meaningful names that clearly convey the purpose of the variable or function. Additionally, be vigilant about capitalization; MATLAB treats `myVariable` and `MyVariable` as two distinct entities.
MATLAB Path Management
Understanding the MATLAB search path is crucial in managing function accessibility. If your functions or scripts are stored in directories not included in the MATLAB path, they will be unrecognized. Always ensure that any folder containing relevant scripts is included.
Utilize the command:
addpath('C:\MyFunctionDirectory'); % Add the folder to the MATLAB path
This command adds the folder where your functions reside to the search path.
Writing Test Scripts
Incorporating test scripts into your workflow is a best practice. This allows you to isolate individual functions or variables and verify their functionality independently. Testing functions in isolation makes it easier to identify and correct unrecognized variables or missing functions effectively.
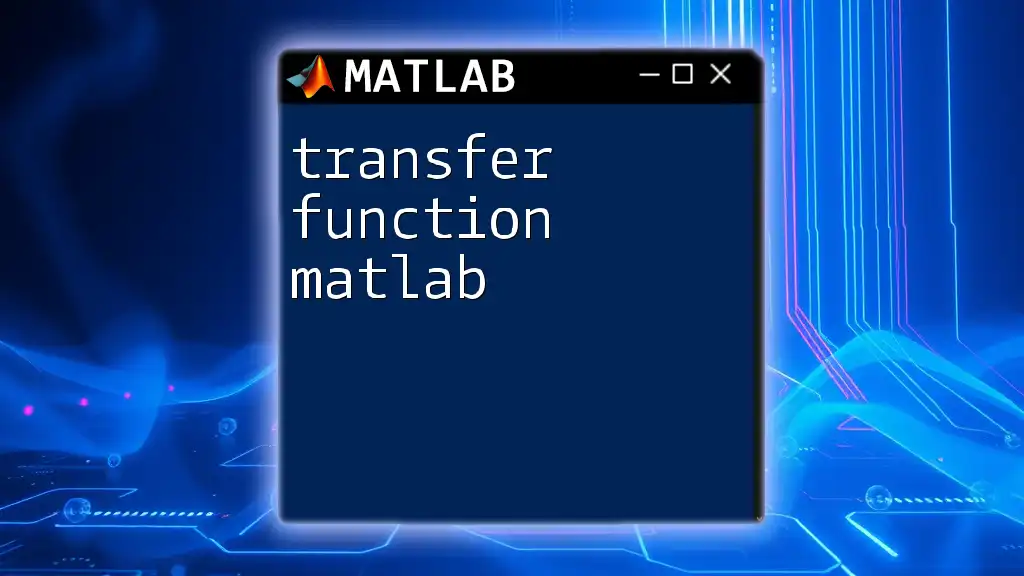
Conclusion
The "unrecognized function or variable" error in MATLAB is a common obstacle, but understanding its causes can empower users to tackle it head-on. Whether it's typographical mistakes, variable scope issues, or missing dependencies, each problem has a clear pathway to resolution. By adhering to best practices and employing debugging tools, you can minimize the occurrence of these errors and enhance your MATLAB programming efficiency.
Embrace these strategies and unlock the true potential of your MATLAB coding experience!