In MATLAB, a vector column is a matrix with one column and multiple rows, often used to represent a list of values or data points.
Here’s a code snippet demonstrating how to create a column vector in MATLAB:
% Create a column vector with values 1, 2, 3
column_vector = [1; 2; 3];
Understanding Vector Columns
What is a Vector?
In the context of mathematics and MATLAB, a vector is essentially a one-dimensional array that can either be arranged horizontally as a row vector or vertically as a column vector. The distinction is crucial for various operations and manipulations in MATLAB, which treats these arrangements differently.
Row and column vectors can be defined as follows:
- Row Vector: A 1 x n array, such as `[1, 2, 3]`.
- Column Vector: An n x 1 array, such as `[1; 2; 3]`.
Importance of Vectors in MATLAB
Vectors are fundamental in MATLAB because they serve as the building blocks of data analysis and manipulation. The ability to efficiently represent and manipulate data in vector form allows for:
- Streamlined mathematical operations, such as addition, subtraction, and scalar multiplication.
- Enhanced data organization for tasks like linear algebra, statistics, and signal processing.
- Simplified syntax for accessing and modifying data within complex matrices.
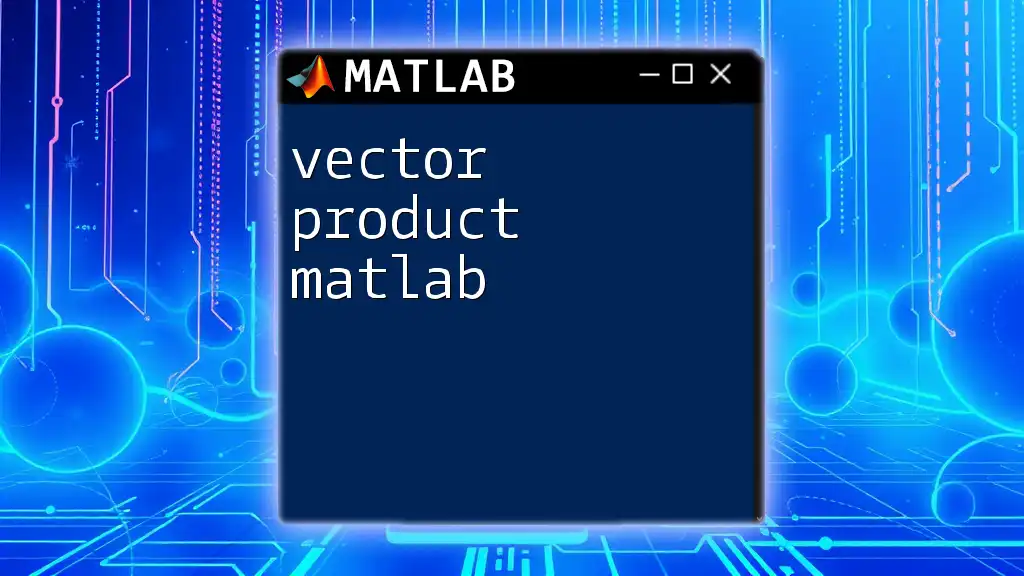
Creating Vector Columns in MATLAB
Using the `[` Operator
One of the most straightforward ways to create a column vector in MATLAB is by using square brackets and separating elements with a semicolon. Each semicolon indicates a new row, effectively setting up a vertical arrangement.
Example: Creating a simple column vector
columnVector = [1; 2; 3; 4; 5];
In this example, `columnVector` consists of five elements, arranged vertically.
Using the `reshape` Function
The `reshape` function provides a way to convert any existing matrix or vector into a column vector by specifying the new dimensions. It is especially useful when you need to transform larger datasets.
Example: Reshaping a matrix into a column vector
matrix = [1, 2; 3, 4; 5, 6];
columnVector = reshape(matrix, [], 1);
Here, the `[]` tells MATLAB to infer the number of rows needed to accommodate all elements in a single column.
Using Built-in Functions
MATLAB also provides built-in functions to simplify vector creation. Functions such as `ones`, `zeros`, and `linspace` can create column vectors quickly and efficiently.
Example: Creating a column vector of zeros
zeroVector = zeros(5, 1);
This command generates a 5 x 1 column vector filled with zeros, which can be invaluable when you need a placeholder or initial value in data computations.
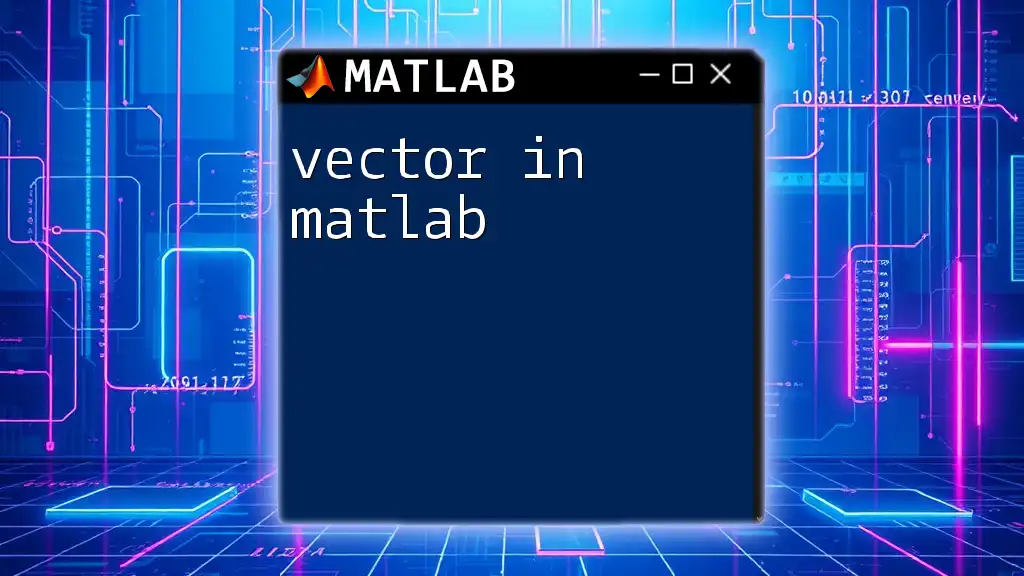
Manipulating Vector Columns
Accessing Elements
In MATLAB, accessing elements of a column vector is intuitive. You can simply use indexing to retrieve specific elements.
Example: Accessing the third element
thirdElement = columnVector(3);
This code extracts the third element from `columnVector`. Understanding MATLAB's indexing system, which starts at 1, is essential for accurate data manipulation.
Modifying Elements
You can also modify specific elements within a column vector using indexing. This allows for dynamic updates to your data.
Example: Changing the second element
columnVector(2) = 10;
In this case, the second element of `columnVector` is updated to 10. This kind of manipulation is often necessary in data analysis, where values may need to change based on user input or calculations.
Combining Column Vectors
Combining multiple column vectors into a single vector is achievable through vertical concatenation. This method can help in gathering data from different sources into one coherent structure.
Example: Concatenating two column vectors
v1 = [1; 2; 3];
v2 = [4; 5; 6];
combinedVector = [v1; v2];
This code results in a new column vector containing all elements from both `v1` and `v2` stacked vertically.
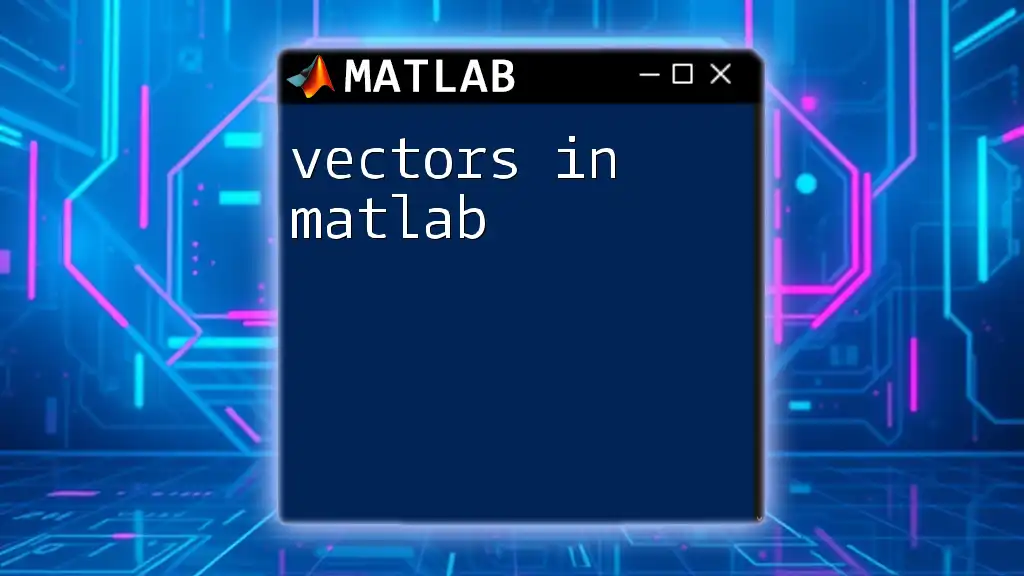
Performing Operations on Vector Columns
Arithmetic Operations
MATLAB allows for various arithmetic operations on column vectors, making it easy to perform calculations efficiently.
Example: Add two column vectors
sumVector = v1 + v2;
This operation adds each corresponding element in the two column vectors, yielding a new vector with the results.
Dot Product and Cross Product
Understanding dot and cross products is essential for vector analysis, particularly in physics and engineering contexts.
Example: Calculating the dot product
dotProduct = dot(v1, v2);
The `dot` function calculates the dot product of two vectors, which can provide insights into their direction and magnitude relationships.
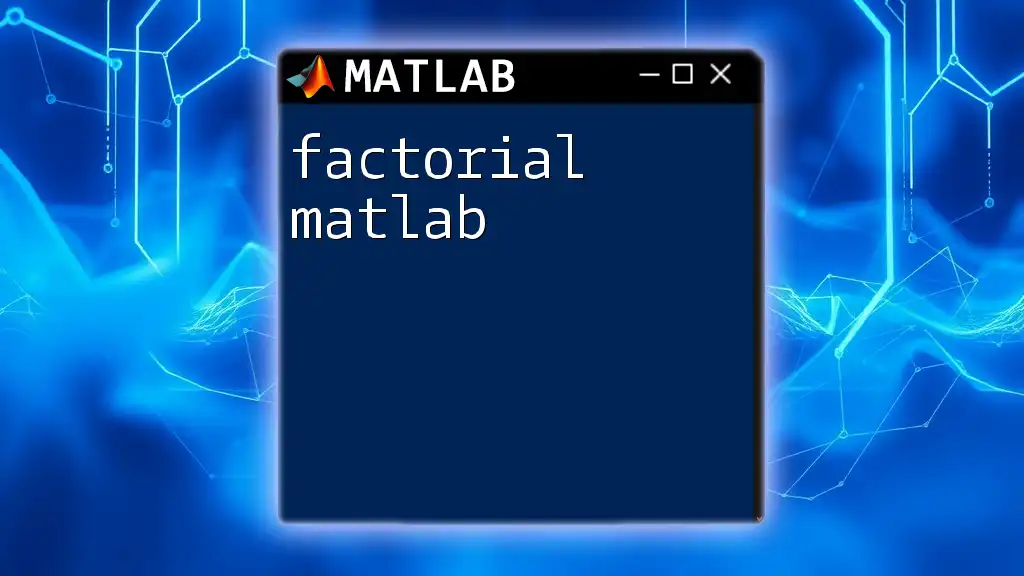
Visualizing Vector Columns
Plotting Vector Columns
Visual representation of column vectors can enhance understanding and analysis. MATLAB's plotting functions allow you to create simple graphical representations.
Example: Create a simple plot of a column vector
plot(columnVector);
xlabel('Index');
ylabel('Value');
title('Plot of Column Vector');
This code snippet generates a line plot with the column vector data. Clarity in presenting data often strengthens the interpretation and communication of results.
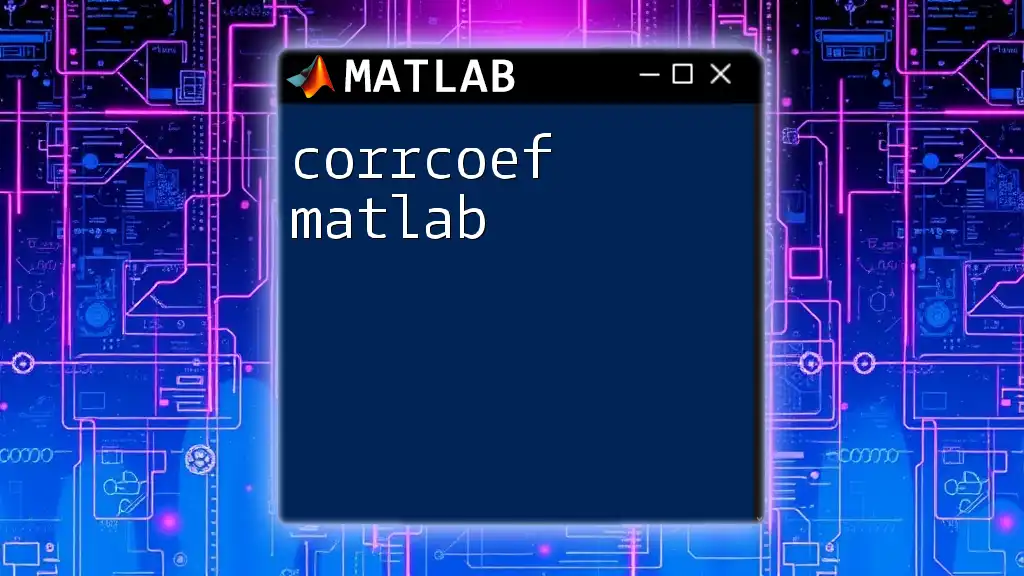
Common Errors and Debugging Tips
Common Mistakes
Even experienced users can make mistakes when dealing with vector columns. A common error arises from trying to access indices that do not exist.
Example: Attempting to access an out-of-bounds index
% This will throw an error if columnVector has less than 4 elements
errorExample = columnVector(4);
Understanding the size of your vector before accessing its elements is crucial to avoid such errors.
Debugging Techniques
When encountering errors, use debugging techniques to resolve issues. MATLAB provides features like breakpoints, which can help identify problems in your code.
Using fprintf() statements to check values at different stages of execution can also aid in diagnosing the source of errors.
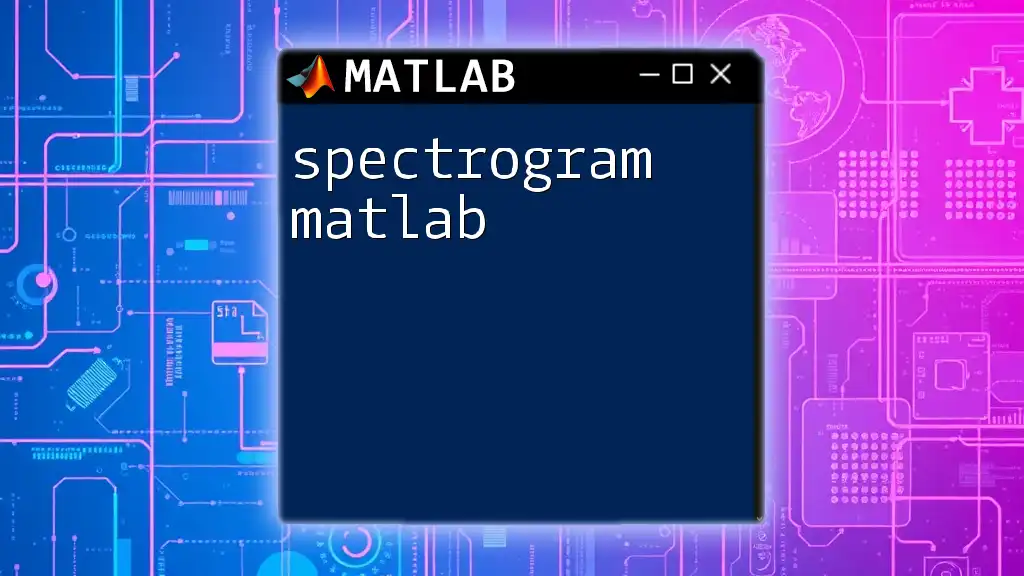
Summary
In this guide, we have explored the essential aspects of vector columns in MATLAB, covering their creation, manipulation, various operations, and visualization techniques. Understanding how to effectively handle vector columns will greatly enhance your data analysis capabilities in MATLAB, opening up new possibilities for efficient programming and computation.
With the knowledge gained here, you are encouraged to practice and explore further, reinforcing your skills and expanding your understanding of this vital aspect of MATLAB.