The `.fig` format in MATLAB is a file extension used to save figures, including their graphical properties and data, allowing users to easily recreate and edit visualizations later.
h = figure; % Create a new figure
plot(1:10, rand(1, 10)); % Plot random data
savefig(h, 'myFigure.fig'); % Save the figure as a .fig file
Understanding .fig Files
What is a .fig File?
A .fig file is a proprietary file format used by MATLAB to save figures created in the environment. This format allows users to store the data of a graphical figure along with its properties, making it possible to easily recreate and modify the figure later. Essentially, a .fig file encapsulates all the necessary elements of a figure, including axes, labels, legends, and even annotations.
How .fig Files Are Created
Creating a .fig file in MATLAB is a straightforward process. Users generate a figure using various plotting functions and then save it in the .fig format. The following example demonstrates how this works:
x = 0:0.1:10;
y = sin(x);
figure; % Create a new figure window
plot(x, y); % Plot the sine wave
savefig('myFigure.fig'); % Save the figure as myFigure.fig
In this snippet, a sine wave is plotted, and the resulting figure is saved as myFigure.fig. This file now contains all relevant information about the figure for future use.
The Structure of a .fig File
The .fig file format is structured to contain a variety of components from the graphical environment. These components include:
- Axes: The plotting area where data is visualized.
- Data Series: The lines, markers, or points plotted.
- Annotations: Text and graphical elements that provide context and information.
It’s noteworthy that under the hood, MATLAB stores .fig files in the HDF5 format, allowing for efficient storage of multidimensional data and enhancing interoperability with other software.
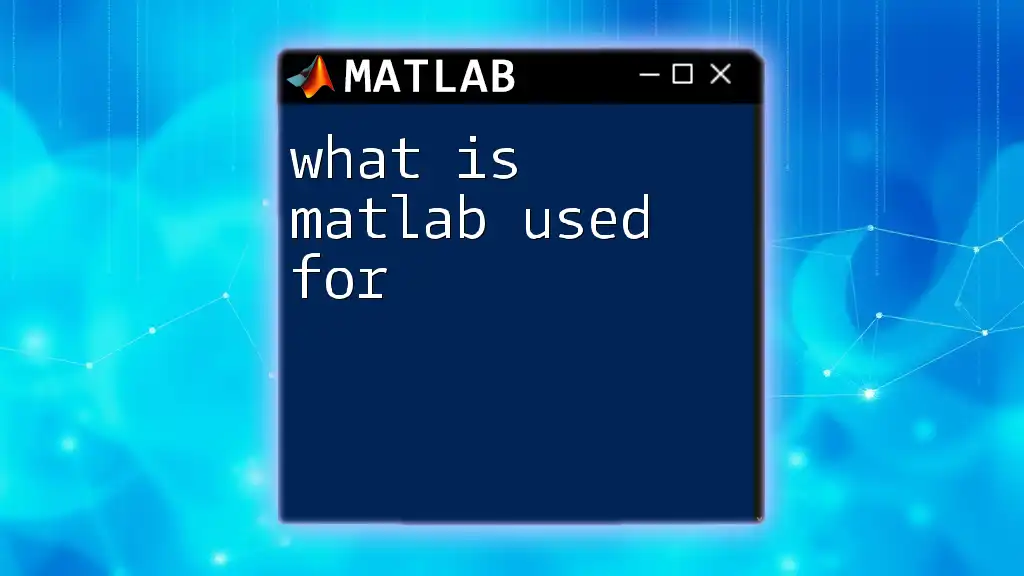
Working with .fig Files in MATLAB
Opening .fig Files
Opening a .fig file in MATLAB is simple and allows you to visualize previously saved figures. To open a .fig file, use the following command:
openfig('myFigure.fig');
This command will display the saved figure in a new window, retaining all its properties.
Modifying .fig Files
Editing Figure Properties
Once you've opened a .fig file, you can modify various properties of the figure. For instance, you can change the title of the figure as follows:
f = openfig('myFigure.fig'); % Open the figure
ax = gca; % Get current axes
ax.Title.String = 'Modified Title'; % Change the title
This snippet retrieves the current axes and modifies its title string, showcasing the capability to update visual components without reconstructing the figure.
Adding New Elements
In addition to modifying existing properties, you can overlay new plots or annotations on an opened .fig file. Using the `hold on` command allows you to retain the current plot while adding new elements. This is how you can incorporate additional data into your figure:
hold on; % Keep the current plot
y2 = cos(x); % Generate new data
plot(x, y2, 'r--'); % Plot the new data in red dashed line
By executing this code after opening your .fig file, you would see both the sine wave and the cosine wave appear in the same figure.
Saving Changes to .fig Files
After making modifications to a .fig file, you have options regarding saving your changes. You can choose to overwrite the original file or save it as a new one. The following shows how to save as a new file:
savefig(f, 'newFigure.fig'); % Save as a new file
This command creates newFigure.fig, ensuring that the original file remains unchanged.
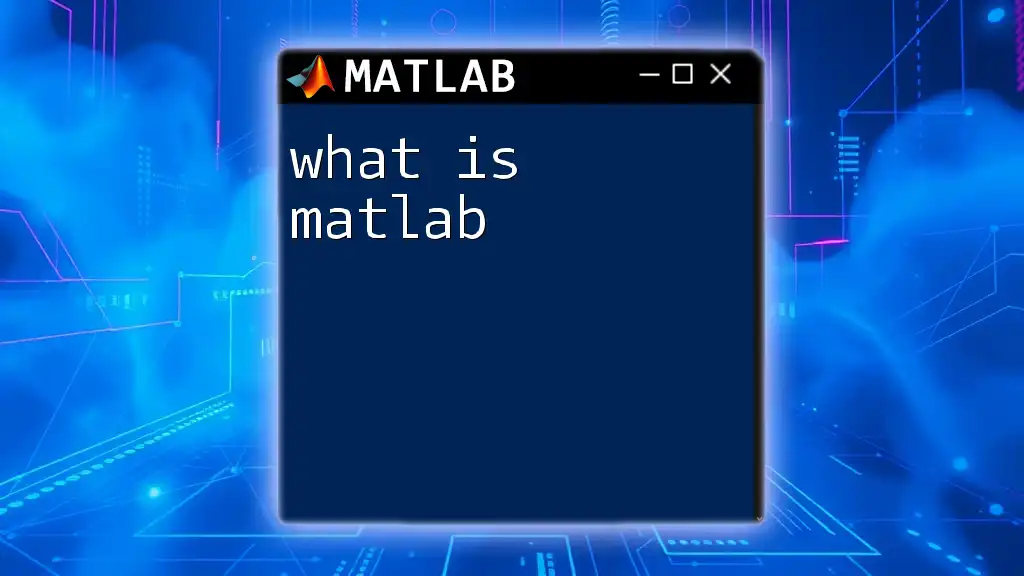
Converting .fig Files to Other Formats
Supported Formats for Conversion
MATLAB supports a range of formats for converting .fig files, including image formats like JPEG, PNG, and vector formats such as PDF. Each of these formats serves specific purposes. For example, PNG files are ideal for web use, while PDF files are better for high-quality document specifications.
Conversion Process
The conversion of .fig files to other formats can be accomplished easily using the `print` function. Here’s how you can convert a .fig file to a PNG format:
print('myFigure', '-dpng'); % Save as PNG
Executing this command will generate a PNG file named myFigure.png based on the current figure, allowing you to utilize your visual data within external contexts.
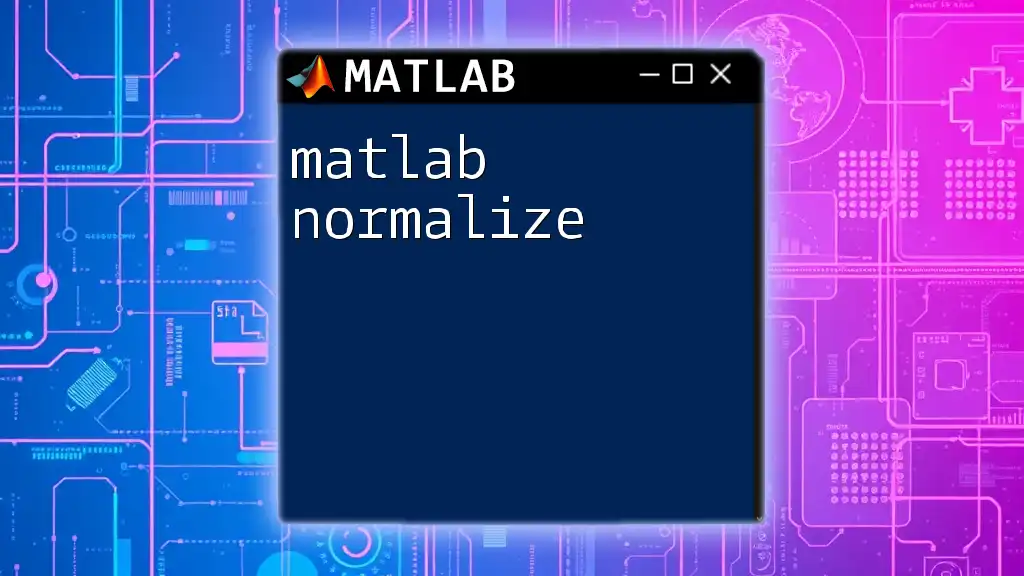
Best Practices for Using .fig Files
Organizing Your Figures
Managing your .fig files effectively is crucial for any project. Implementing well-defined naming conventions and establishing a structured folder hierarchy can significantly enhance organization. For instance, consider naming your files descriptively, such as sine_wave_analysis.fig. This makes retrieval easier in the future.
Troubleshooting Common Issues
Users may encounter various challenges when working with .fig files, particularly compatibility issues across different MATLAB versions. If you cannot open a .fig file saved in a newer version of MATLAB, consider exporting it to a more universally compatible format or upgrading your MATLAB environment.
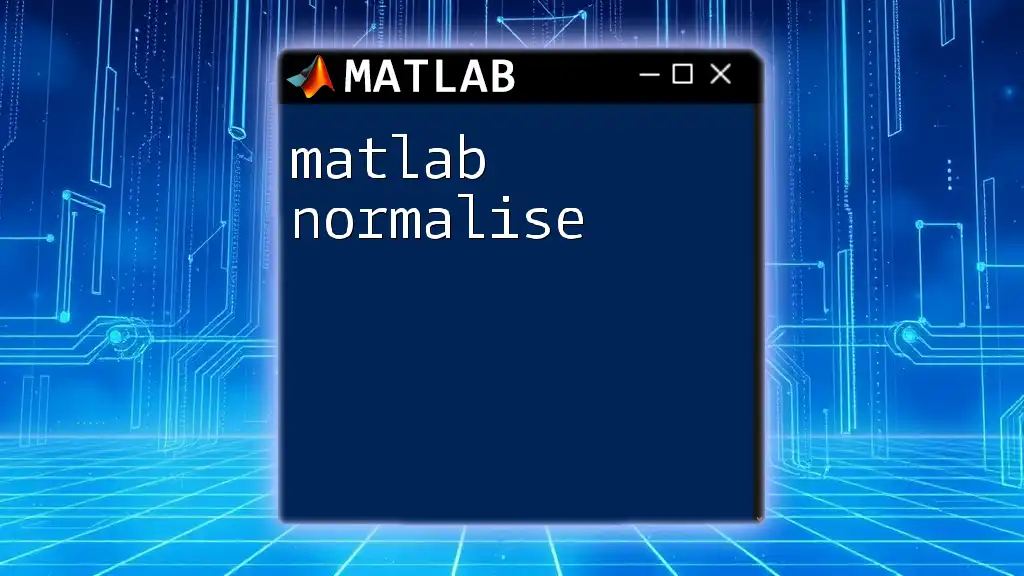
Conclusion
In summary, understanding what is MATLAB .fig format is essential for anyone looking to leverage the MATLAB environment for data visualization. The .fig format provides robust capabilities for saving, modifying, and sharing figures, thus enhancing the overall data analysis experience. Engaging with .fig files can significantly improve your data visualization skills, making it a vital aspect of mastering MATLAB.
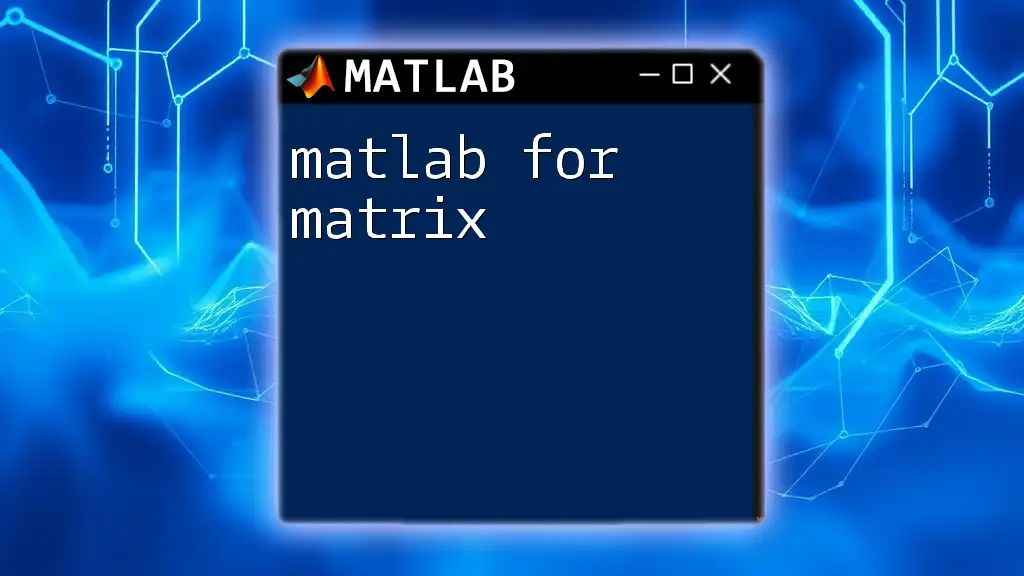
Additional Resources
For further information about .fig files and their capabilities, refer to the official MATLAB documentation. Additionally, numerous tutorials and community forums are available to help you deepen your understanding and troubleshoot any challenges you may face.
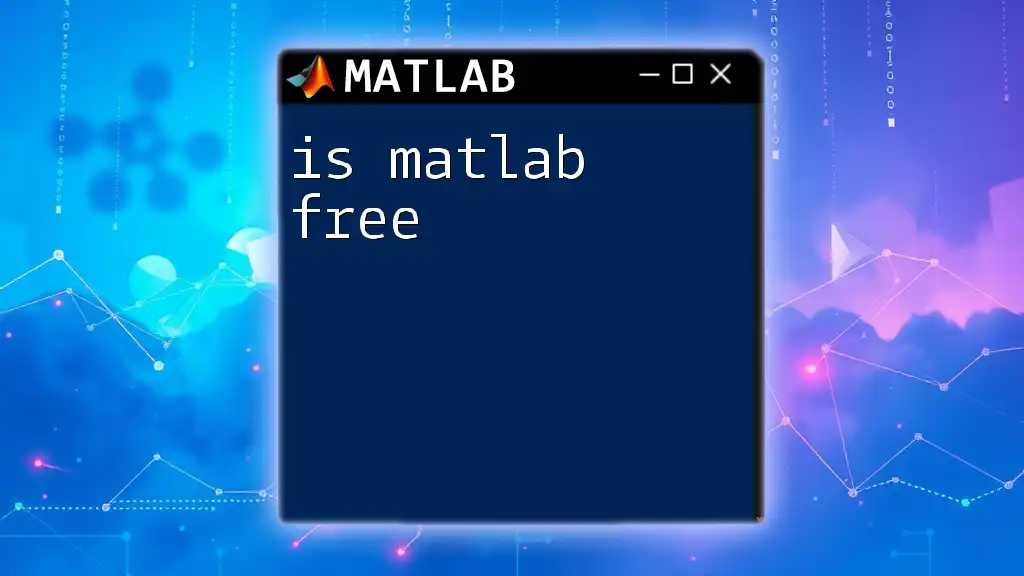
FAQs
As a common point of interest, many beginners inquire about the specific capabilities of .fig files compared to other formats. It is essential to remember that .fig files offer interactive features and full data retention that other formats do not provide, making them invaluable for MATLAB users.