In MATLAB, the logical operators `&&` (AND) and `||` (OR) are used to perform short-circuit evaluations, where `&&` returns true if both conditions are true, and `||` returns true if at least one condition is true.
Here’s a code snippet demonstrating their usage:
a = 5;
b = 10;
if (a > 0 && b > 5)
disp('Both conditions are true.');
end
if (a < 0 || b > 5)
disp('At least one condition is true.');
end
Understanding Logical Operations in MATLAB
What Are Logical Operations?
Logical operations form the backbone of conditional programming and decision-making in software development. In MATLAB, logical operations allow us to evaluate conditions and make decisions based on true or false values. This capability is essential for algorithms, data analysis, control flow, and much more.
Overview of AND and OR Operators
Among the most fundamental logical operations are the AND (`&`) and OR (`|`) operators. These operators evaluate multiple conditions and return a single logical result (true or false) based on those evaluations. Understanding how to use these operators effectively is crucial for any MATLAB programmer.
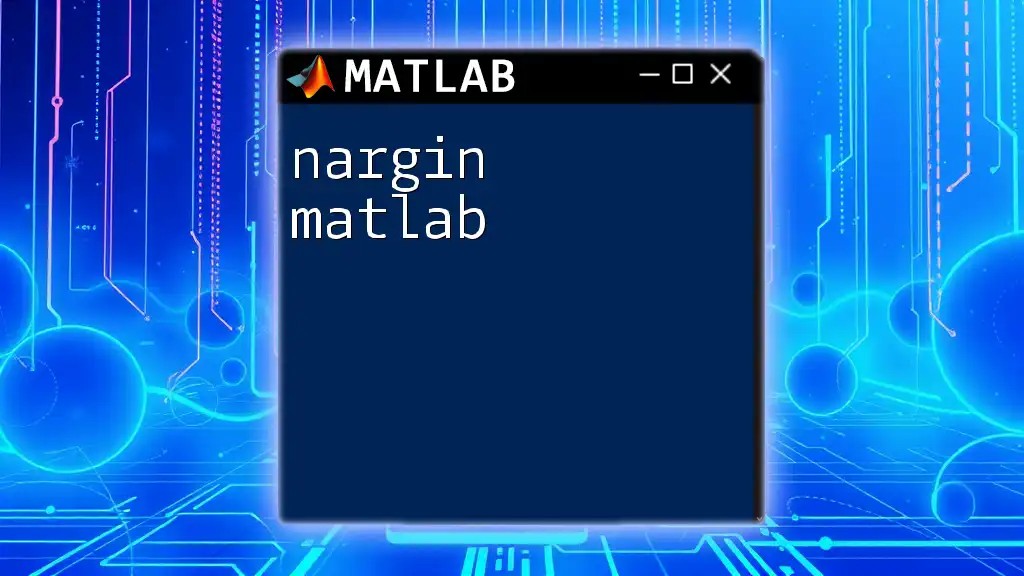
The AND Operator in MATLAB
Syntax and Basic Usage
The AND operator is used to check if two or more conditions are true simultaneously. Its basic syntax looks like this:
result = condition1 & condition2;
For example, consider the following code snippet, which checks if a number is between 10 and 20:
% Example: Check if a number is between 10 and 20
x = 15;
if x >= 10 & x <= 20
disp('x is between 10 and 20');
end
In this case, both conditions need to be satisfied for the overall expression to evaluate to true.
Short-Circuiting Behavior
Short-circuiting occurs when an operator stops evaluating as soon as the outcome is determined. In MATLAB, there are two forms of the AND operator: `&` (bitwise) and `&&` (logical). The operator `&&` is specifically designed for short-circuit evaluation.
For example:
% Using && for short-circuiting
a = false;
b = true;
if a && (b = false) % The assignment won't execute
disp('This will not execute.');
else
disp('Short-circuited, a is false.');
end
In this example, because `a` is false, the second condition is never evaluated, thus maintaining efficiency.
Applying AND with Arrays
The AND operator can also be used for element-wise comparisons in arrays. For instance, if you want to check which elements of two arrays satisfy different conditions, you can do the following:
% Example: Logical AND with arrays
A = [1, 2, 3, 4];
B = [2, 3, 4, 5];
C = (A > 1) & (B < 5); % Element-wise logical AND
disp(C); % Output will be [0, 1, 1, 0]
Here, the result is a new array where each position indicates whether both conditions were true for the corresponding elements in arrays A and B.
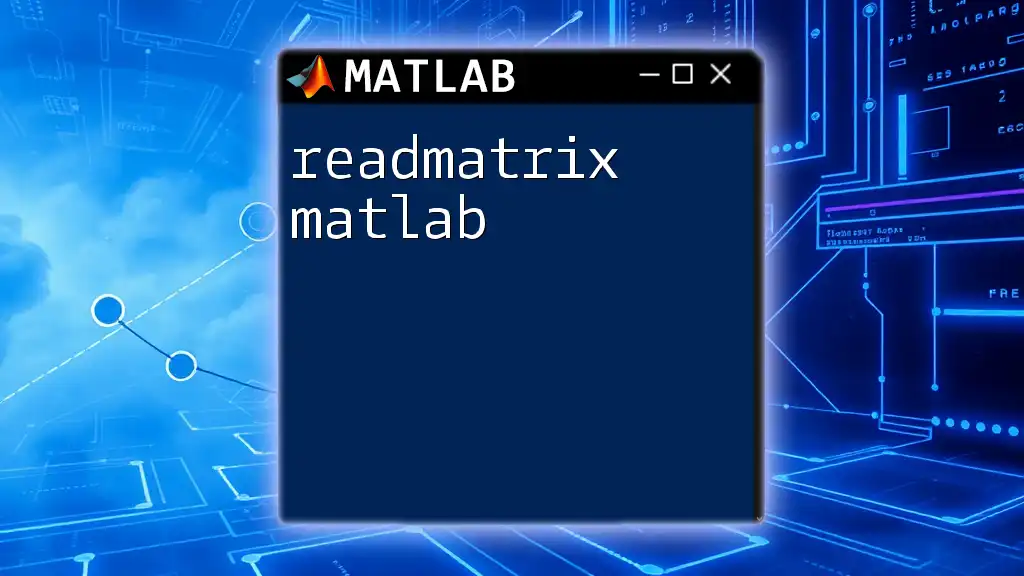
The OR Operator in MATLAB
Syntax and Basic Usage
The OR operator is used to determine if at least one of multiple conditions is true. The syntax is similar to the AND operator:
result = condition1 | condition2;
For example, you might check if a number is either less than 10 or greater than 20:
% Example: Check if a number is either less than 10 or greater than 20
x = 25;
if x < 10 | x > 20
disp('x is either less than 10 or greater than 20');
end
In this scenario, only one of the specified conditions needs to be true for the overall expression to evaluate to true.
Short-Circuiting Behavior
Like the AND operator, OR also exhibits short-circuiting behavior. The two forms available are `|` (element-wise) and `||` (logical). Using `||` allows MATLAB to stop evaluating as soon as it finds a true condition.
Consider this example:
% Using || for short-circuiting
a = true;
b = false;
if a || (b = true) % The assignment won't execute
disp('Short-circuited, a is true.');
end
Here, as soon as MATLAB evaluates `a` as true, it avoids checking the second condition, thus improving efficiency.
Applying OR with Arrays
Using the OR operator allows for flexible combinations of conditions when working with arrays. For example:
% Example: Logical OR with arrays
D = [1, 2, 3, 4];
E = [2, 3, 4, 5];
F = (D < 2) | (E > 4); % Element-wise logical OR
disp(F); % Output will be [1, 0, 0, 1]
In this example, the output array indicates which elements in either D or E meet the designated conditions.
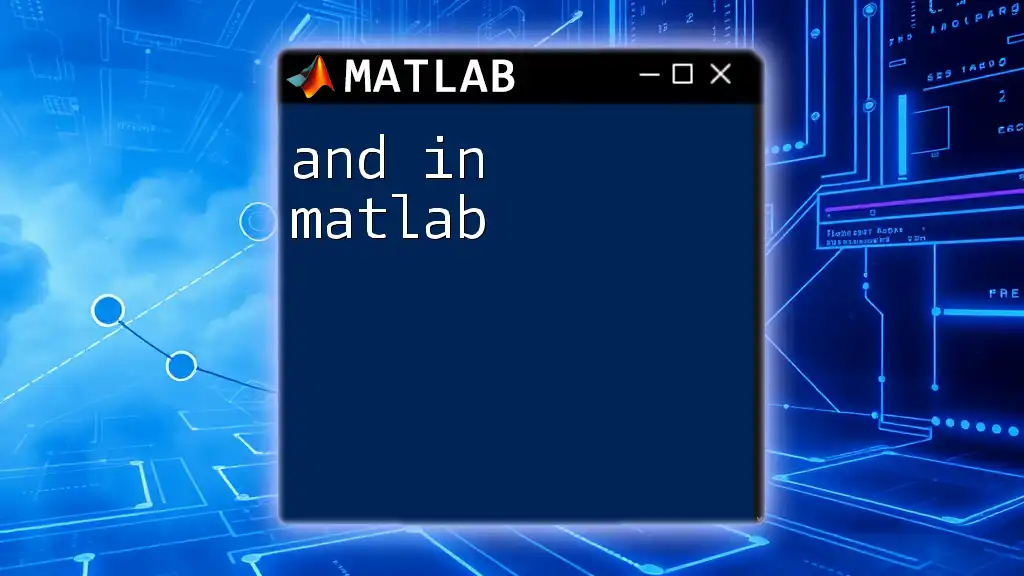
Combining AND and OR in Conditions
Using Parentheses for Clarity
When combining AND and OR operators, parentheses are vital for maintaining clarity and order of operations. Misleading combinations can lead to unexpected results. Here’s an example of how parentheses affect logic:
% Example: Combining AND and OR
x = 15;
if (x < 10 | x > 20) & (x ~= 15)
disp('x is either less than 10 or greater than 20 and not equal to 15');
else
disp('Condition not met.');
end
In this case, parentheses ensure that the conditions are evaluated in the desired order.
Practical Scenarios of Combining Operators
Combining AND and OR operators is often useful in filtering datasets or making decisions based on multiple criteria. For example, you might want to apply filters on data ranging across several variables, such as in data analysis or signal processing applications.
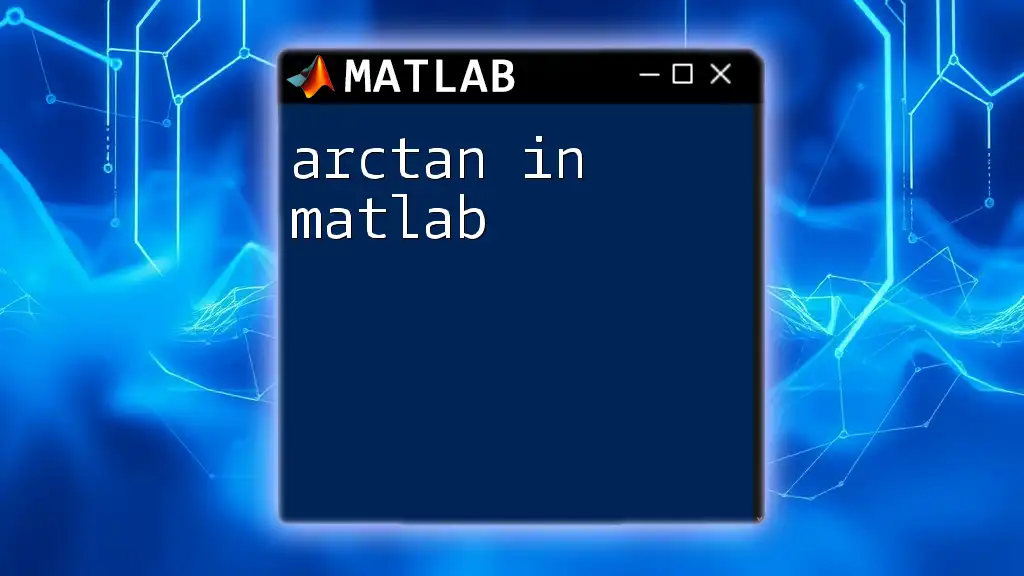
Common Mistakes and How to Avoid Them
Common Errors with Logical Operators
One common error is misusing the precedence of the operators, which can lead to unexpected results. For instance:
% Incorrect use of | leading to unexpected results
if (A > 0 | B < 5 & A < 10) % Ambiguous precedence
disp('Condition might not behave as expected.');
end
This snippet may lead to beneath-the-surface logical errors due to how the operators are interpreted without proper parentheses.
Tips for Writing Clean, Readable Conditions
When writing conditions in MATLAB, clarity is paramount. Always structure your logical conditions using parentheses to avoid confusion. Additionally, adopting a consistent style helps to enhance the readability of your code, which aids both you and others who might work with it later.
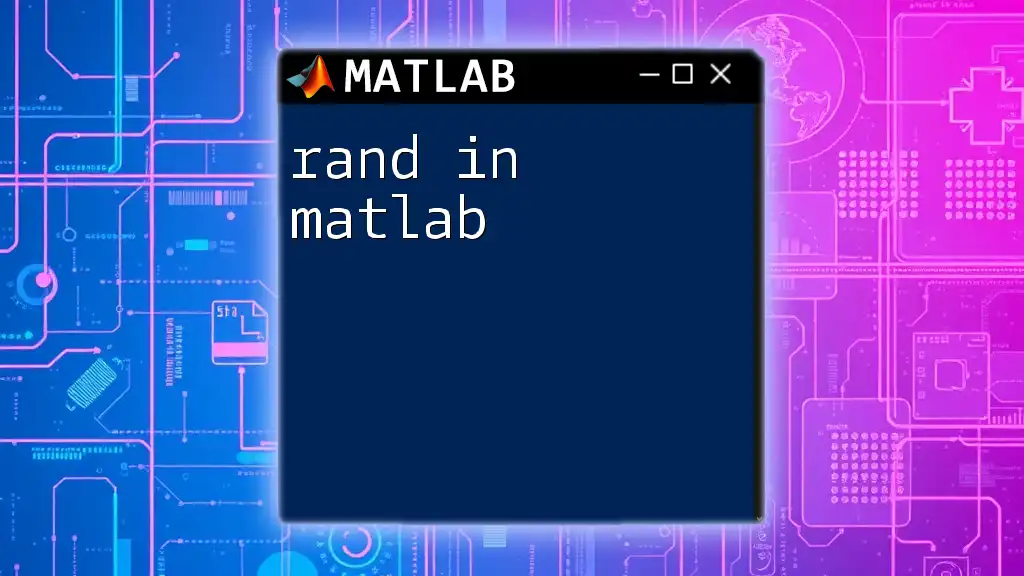
Conclusion
Summarizing Key Takeaways
In this comprehensive guide, we explored the AND and OR operators in MATLAB, understanding their syntax, behaviors, and application in various contexts. Mastery of these logical operators will immensely enhance your programming proficiency in MATLAB.
Encouraging Experimentation
Don't hesitate to practice using "and and or in MATLAB" in your coding projects. Experimenting with different combinations and scenarios will solidify your understanding and help you become a more effective MATLAB programmer.
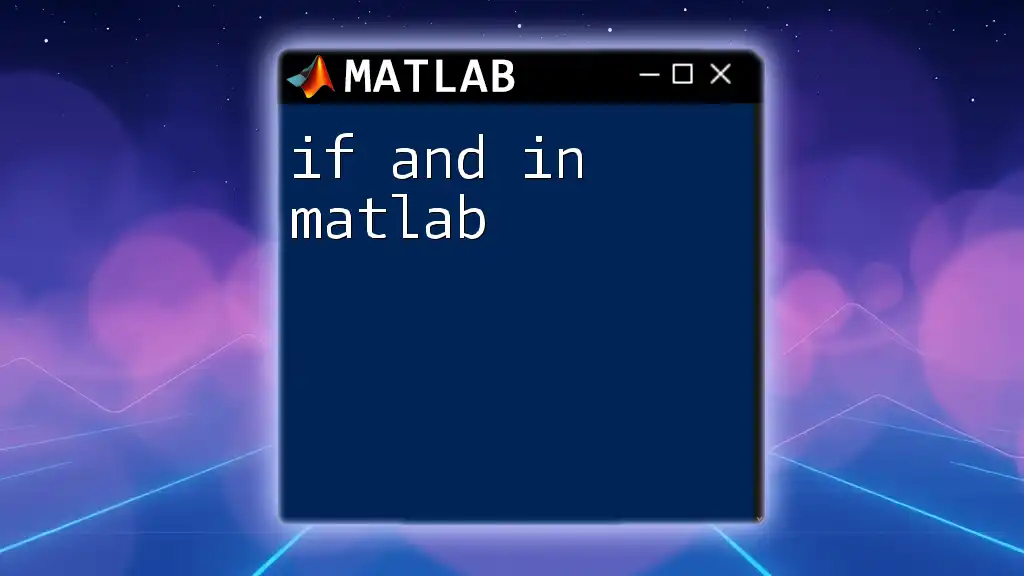
Further Reading and Resources
Recommended MATLAB Documentation
To deepen your knowledge of logical operations in MATLAB, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/logical.html).
Related Topics for Advanced Learning
For those interested in expanding their MATLAB skillset, consider exploring related topics like conditional statements, loops, and data analysis techniques. These elements often intersect with logical operations and are essential for complex problem-solving in MATLAB.