In MATLAB, an array of strings can be created using a string array which allows for efficient storage and manipulation of text data, as shown in the following example:
stringArray = ["apple", "banana", "cherry"];
What is a String Array?
In MATLAB, a string is a data type used to represent text, consisting of a sequence of characters enclosed in double quotes. A string array is an array that contains multiple strings. Unlike character arrays, which are essentially arrays of characters, string arrays provide a more intuitive and flexible way to handle text data in MATLAB.
For instance, consider the following comparison between a character vector and a string array:
% Character vector
charArray = 'Hello World';
% String array
strArray = ["Hello", "World"];
In this example, `charArray` is a character vector, while `strArray` is a string array that can hold multiple strings in a more manageable format. This distinction is important when working with string data as it affects how operations are performed.
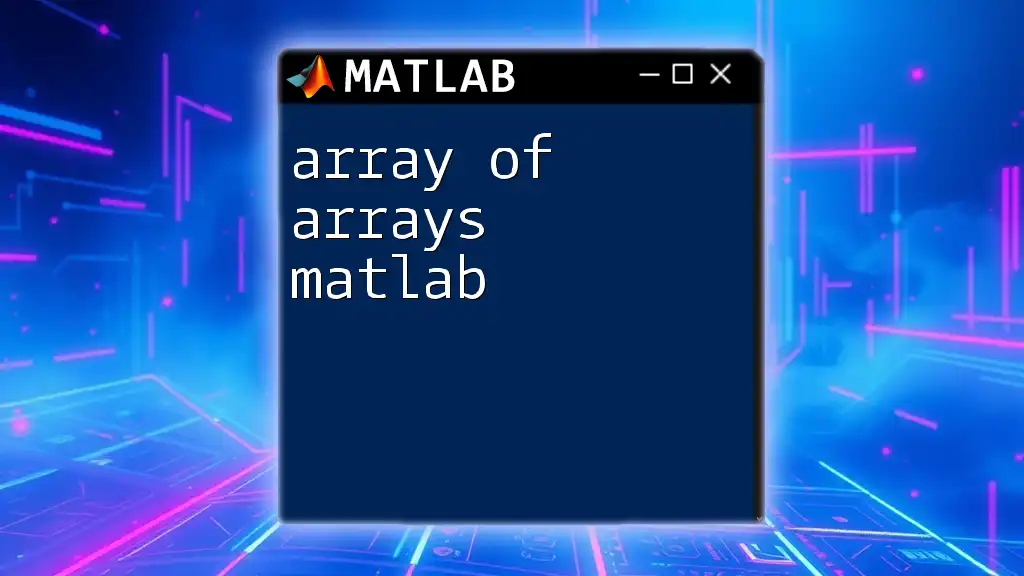
Creating String Arrays
Using Square Brackets
You can create a string array using square brackets, separating each string with a comma or space. This approach is straightforward and commonly used for initializing small string arrays.
For example, here’s how you can create a simple string array:
strArray = ["Apple", "Banana", "Cherry"];
disp(strArray);
The output will display the string array with its elements, showcasing its inherent structure.
Using the `strings` Function
Another way to create string arrays is by using the `strings` function, especially when you want to initialize an empty string array of a specific size. This function is particularly useful when you want to fill in the array later.
strArray = strings(1, 3); % Creates a 1x3 empty string array
In this case, you have an empty string array that can hold three elements, which you can populate as needed throughout your program.
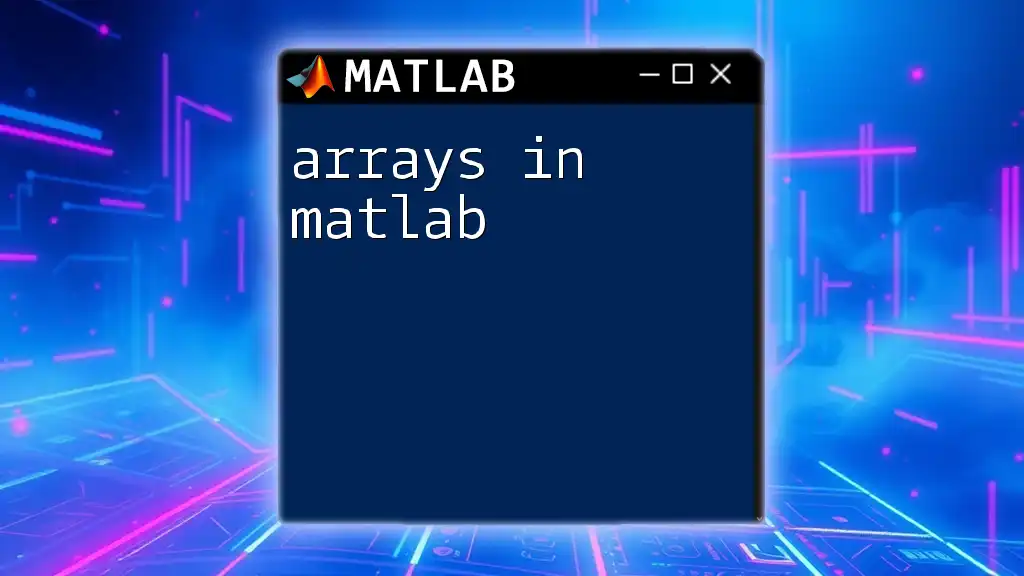
Accessing Elements of a String Array
Indexing String Arrays
Accessing elements in a string array is done through indexing, similar to how one would access elements in other array types in MATLAB. Indexing starts at 1, so the first element is accessed with an index of 1.
For example:
fruit = strArray(2); % Access second element ("Banana")
This retrieves the second string from the array, which is particularly useful for querying or manipulating specific elements.
Slicing String Arrays
You can also slice string arrays to access multiple contiguous elements. This allows you to work with subsets of data rather than single elements, enhancing the flexibility of your code.
subArray = strArray(1:2); % Selects the first two elements
This example returns a new string array containing the first two elements, which can be useful for situations where you need to work with a group of strings.
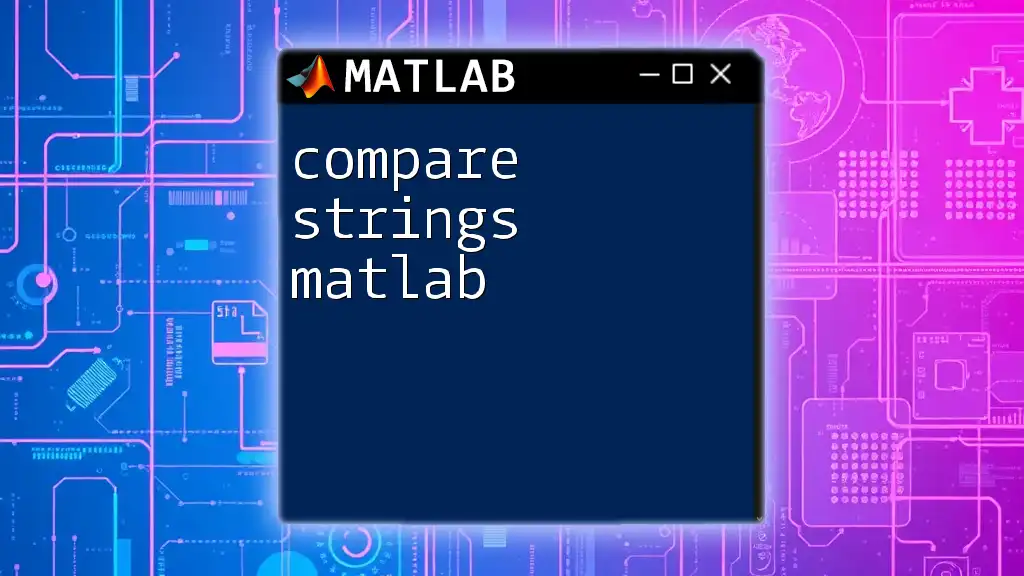
Modifying String Arrays
Updating Elements
It's seamless to modify existing strings within an array. You can update an individual string by simply assigning a new value to the desired index.
strArray(1) = "Grape"; % Updates first element
This line changes the first element from "Apple" to "Grape", demonstrating how easy it is to make changes in a string array directly.
Appending Strings
Adding more strings to an existing array can be done using concatenation. This feature is handy when you want to extend the size of your string array dynamically.
strArray = [strArray, "Orange"]; % Appending a new element
As a result, the `strArray` now includes "Orange" as an additional entry at the end, showcasing how string arrays can grow as needed.
Removing Strings
To remove strings from a string array, you can clear specific elements by assigning an empty array to the corresponding index. This operation is vital for cleaning up data or adjusting the contents of an array.
strArray(2) = []; % Removes "Banana"
With this command, the second element is removed from the array, and the remaining elements are automatically adjusted.
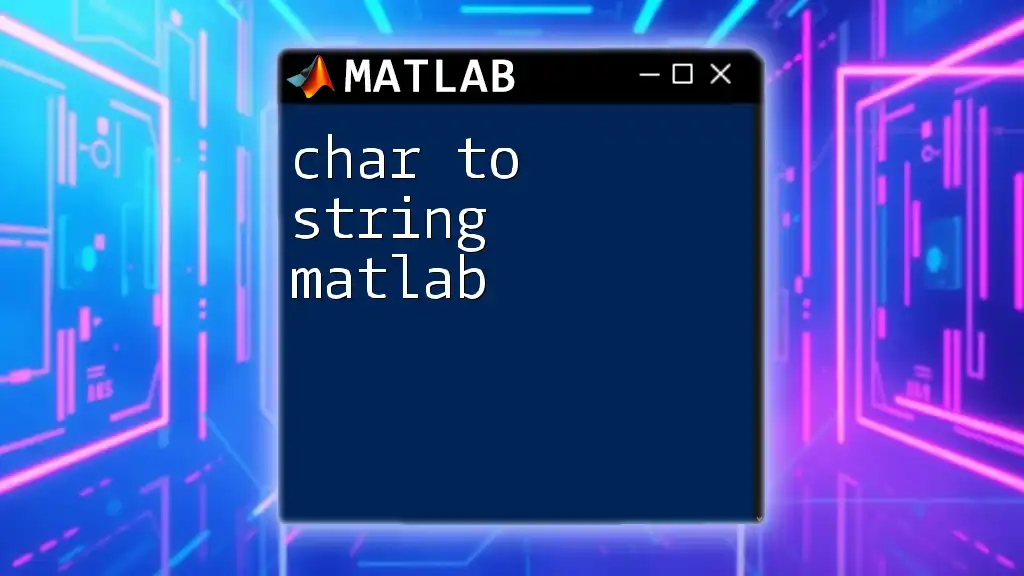
String Operations
Concatenating String Arrays
MATLAB provides the ability to concatenate multiple string arrays using square brackets as well. This function is particularly useful when you want to merge data from different sources.
combinedArray = [strArray, "Melon", "Kiwi"];
This snippet combines `strArray` with two new strings, thereby creating a new concatenated array containing all specified elements.
Searching within String Arrays
Searching for specific strings or substrings within a string array can be performed using MATLAB's built-in functions like `contains`. This functionality helps in filtering or processing string data based on given criteria.
found = contains(strArray, "Apple"); % Returns a logical array
Here, `found` will return a logical array indicating whether "Apple" is present in `strArray`, simplifying tasks that require searching for specific elements.
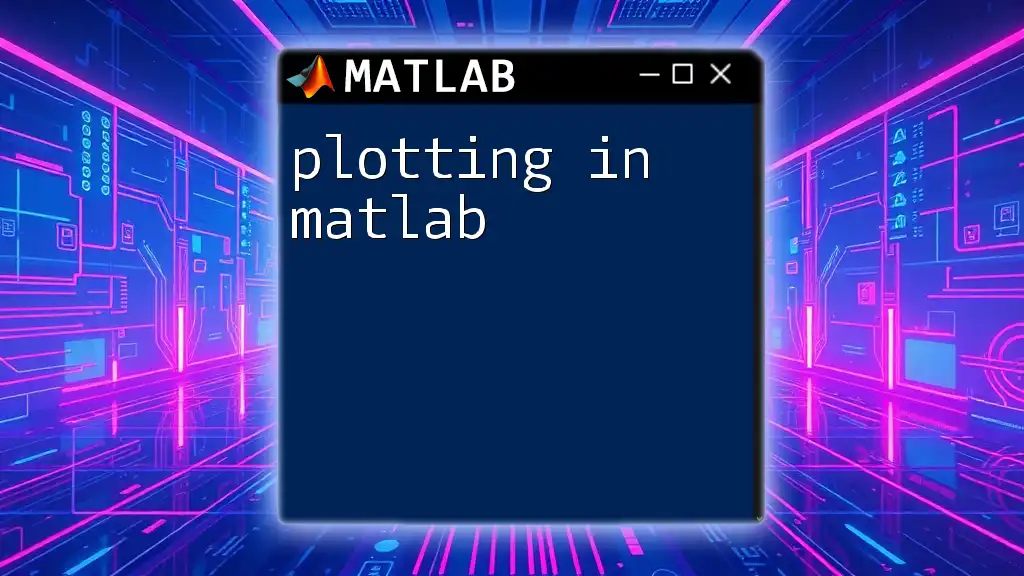
Practical Applications
Use Cases for String Arrays
Understanding and utilizing string arrays is crucial in various programming tasks, particularly in areas such as text processing and data management. They allow for better manipulation of textual data, which is often a core component in applications like:
-
Data Preprocessing for Natural Language Processing (NLP): String arrays can help in organizing and processing textual data to prepare for analysis or modeling.
-
Managing Lists of Filenames or User Inputs: By using string arrays to store file names or inputs, you can efficiently perform batch operations, validations, or modifications on multiple entities.
Example Project
Consider a simple MATLAB project that prompts users for a list of fruits and stores them in a string array. Here’s an illustrative snippet:
numFruits = input('How many fruits do you want to enter? ');
fruits = strings(1, numFruits); % Creating an empty string array
for i = 1:numFruits
fruits(i) = input('Enter a fruit: ', 's'); % 's' specifies string input
end
disp(fruits);
This example demonstrates the practicality of string arrays in a user-interactive scenario, allowing for dynamic data entry and storage.
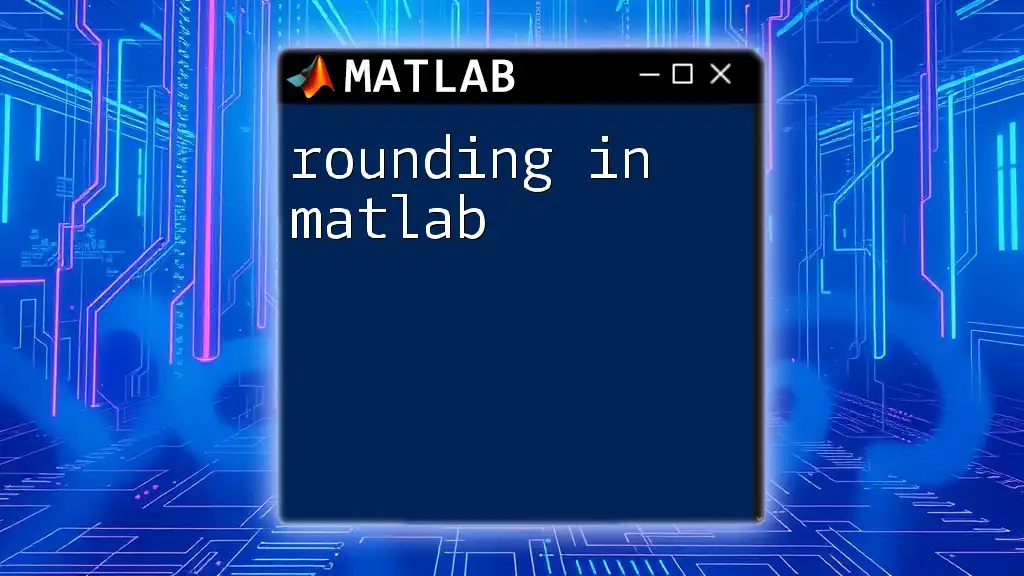
Conclusion
In summary, mastering array of strings in MATLAB is essential for effective programming and data manipulation. String arrays enable developers to handle text data holistically, from creation to modification, and through various operations. By understanding how to create, access, modify, and utilize string arrays, you equip yourself with powerful tools for a wide array of applications in MATLAB.
For further exploration, I encourage you to dive deeper into MATLAB's string functionalities and practice creating and manipulating string arrays. The more you experiment, the more proficient you will become!
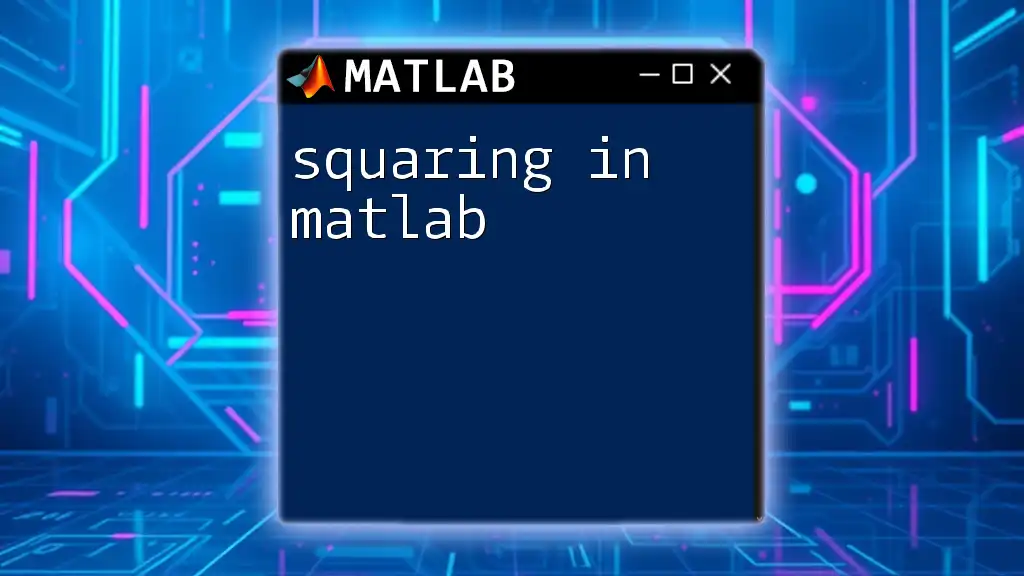
Additional Resources
To gain a more comprehensive understanding, consult MATLAB's official documentation on strings, reference books on MATLAB programming, and explore community forums where you can engage with other learners and professionals for tips and advice.