You can create a plot from a matrix in MATLAB by using the `imagesc` function to visualize matrix data as an image, with color representing values.
% Example: Plotting a 2D matrix using imagesc
matrixData = rand(10); % Create a 10x10 matrix of random values
imagesc(matrixData); % Display the matrix as an image
colorbar; % Add a color bar to indicate value scale
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a two-dimensional array of numbers, which can be utilized in various mathematical operations. In MATLAB, matrices are fundamental because most numerical data is represented in matrix form. It’s essential to differentiate between vectors (one-dimensional arrays) and matrices (two-dimensional arrays) as they will dictate how you manipulate and visualize the data.
Creating Matrices
Creating matrices in MATLAB can be done using several commands:
- Zeros Matrix: Creates a matrix filled with zeros.
A = zeros(3, 3); % Creates a 3x3 matrix of zeros
- Ones Matrix: Similar to the zeros function but fills the matrix with ones.
B = ones(3, 3); % Creates a 3x3 matrix of ones
- Identity Matrix: An identity matrix that has ones on the diagonal and zeros elsewhere.
C = eye(3); % Creates a 3x3 identity matrix
- Manual Entry: You can create a matrix by explicitly defining its elements.
D = [1 2 3; 4 5 6; 7 8 9]; % A 3x3 matrix
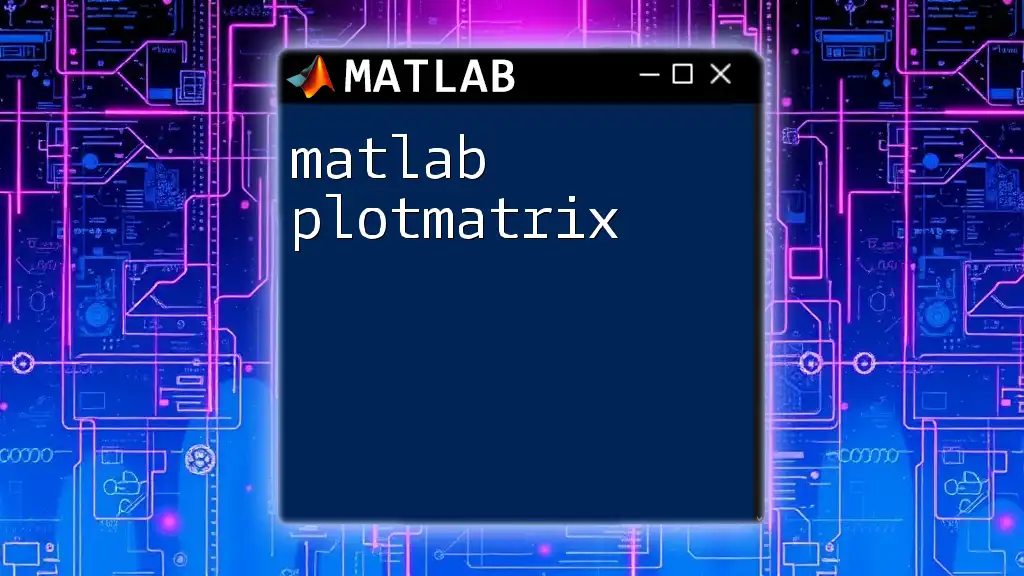
MATLAB Plotting Basics
Introduction to MATLAB Plots
Visualizing data through plotting is critical in MATLAB. Plots help you understand the underlying patterns and trends in your data, making them an indispensable tool for analysis.
Common Plotting Functions
MATLAB offers numerous plotting functions, but the most frequently used include:
- `plot`: Ideal for line graphs.
- `scatter`: Used for scatter plots.
- `surf`: Generates 3D surface plots.
- `mesh`: Creates 3D mesh plots.
Each of these functions serves a specific purpose, allowing for various forms of data representation. For example, to create a simple line plot from a sine function, you can use:
x = 0:0.1:10; % Range from 0 to 10 with 0.1 intervals
y = sin(x); % Sine of x
plot(x, y); % Plotting the sine wave
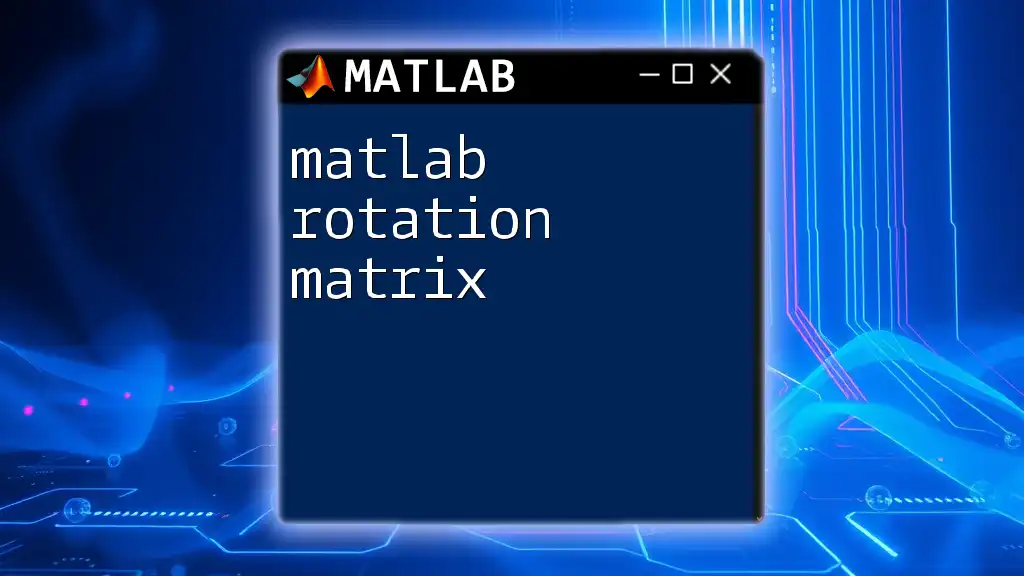
Plotting from a Matrix
2D Plotting from Matrices
Using the `plot` Function
To create a plot from a matrix, you can utilize columns or rows of the matrix directly. For instance, given a random matrix:
A = rand(5, 3); % Creates a 5x3 matrix of random values
plot(A); % Plots each column of A against their indices
title('Line Plot from Matrix');
xlabel('Index');
ylabel('Values');
legend('Column 1','Column 2','Column 3');
In this example, MATLAB automatically treats the columns as different datasets and generates a multi-line plot.
Customizing 2D Plots
Customizing your plots is crucial for clarity and impact. You can modify line colors, styles, and markers:
plot(A(:,1), 'r--', 'LineWidth', 2); % Red dashed line for the first column
hold on; % Retain the current plot
plot(A(:,2), 'b:', 'Marker', 'o', 'LineWidth', 1.5); % Blue dotted line with circles for the second column
With this technique, you can visually differentiate between the data to enhance readability.
3D Plotting from Matrices
Using `surf` and `mesh`
When you're dealing with three-dimensional data, MATLAB’s `surf` and `mesh` functions become essential tools. They allow you to visualize the relationships between three variables simultaneously.
For example, to create a surface plot from two matrices, you can prepare x and y grids and compute z values as follows:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5); % Create a grid for X and Y
Z = sin(sqrt(X.^2 + Y.^2)); % Compute Z values
surf(X, Y, Z); % Generate the surface plot
title('3D Surface Plot from Matrix');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
Understanding Axes and Color Mapping
In 3D plots, it’s equally important to control the view and the color representation of the data. You can adjust the axis limits and use colormaps to convey additional information visually.
colormap(jet); % Change colormap to 'jet' for a spectrum of colors
colorbar; % Display the color scale alongside
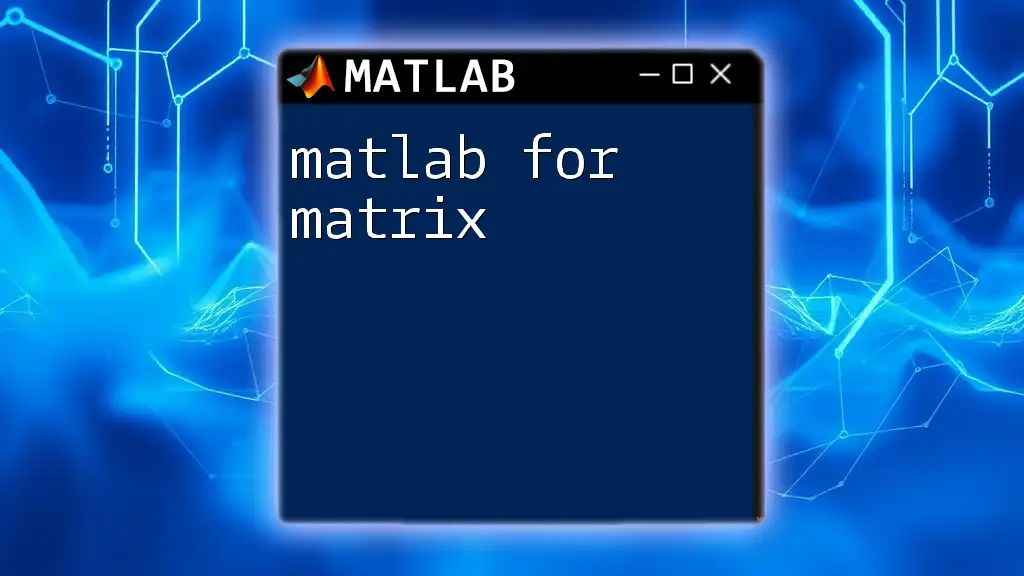
Advanced Plotting Techniques
Combining Multiple Plots
To create more complex visualizations, MATLAB enables you to arrange multiple plots in a single figure. Using `subplot`, you can customize layouts effectively.
subplot(2, 1, 1); % First subplot
plot(A(:, 1));
title('First Column');
subplot(2, 1, 2); % Second subplot
plot(A(:, 2));
title('Second Column');
This approach is particularly useful when you want to compare different datasets side by side.
Using Annotations and Text
To make your plots more informative, adding annotations and text can greatly enhance viewer understanding.
text(1, 0.5, 'Annotation Text'); % Place text at specified coordinates
Title labels, axes labels, and legends help convey the plot's message effectively and should not be overlooked.
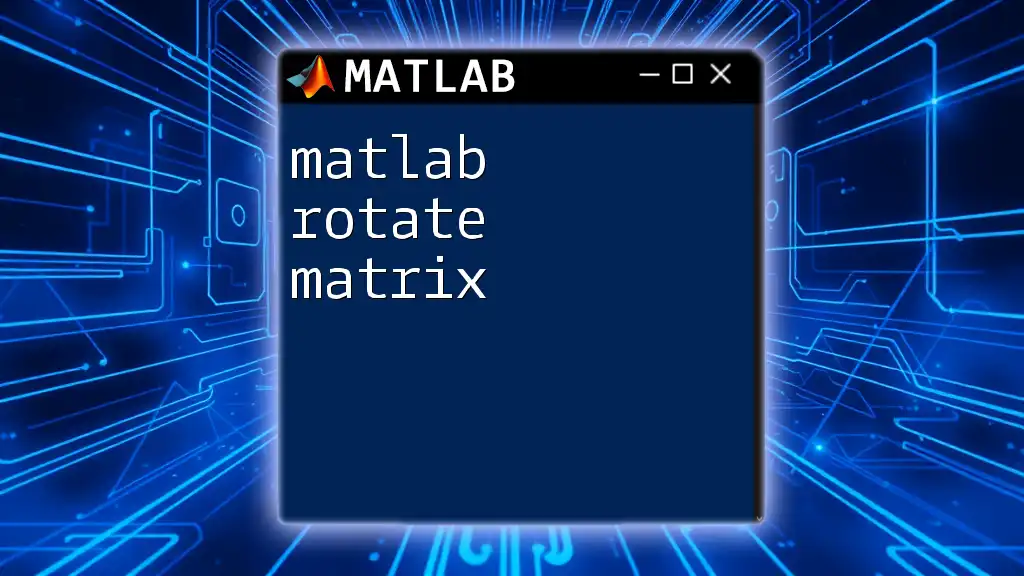
Tips for Effective Data Visualization
Clarity is Key
When crafting plots, simplicity and clarity should take precedence. Avoid cluttering your visualizations. Always strive for a balance between aesthetics and informative content.
Choose the Right Plot Type
Understanding when to use specific types of plots is essential for effective communication of data. Each plot type offers unique perspectives; for instance, line plots are excellent for trend analysis, while scatter plots are ideal for showing correlations.
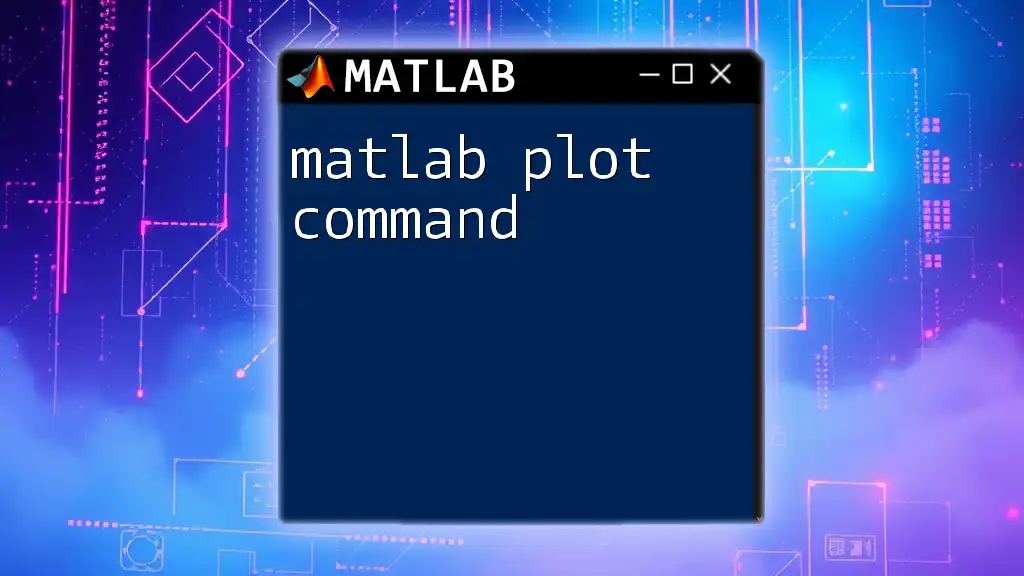
Conclusion
In summary, mastering MATLAB plots from matrices is a vital skill for anyone engaging in numerical analysis and data visualization. By experimenting with various functions and customizations, you can create compelling visual stories from your data.
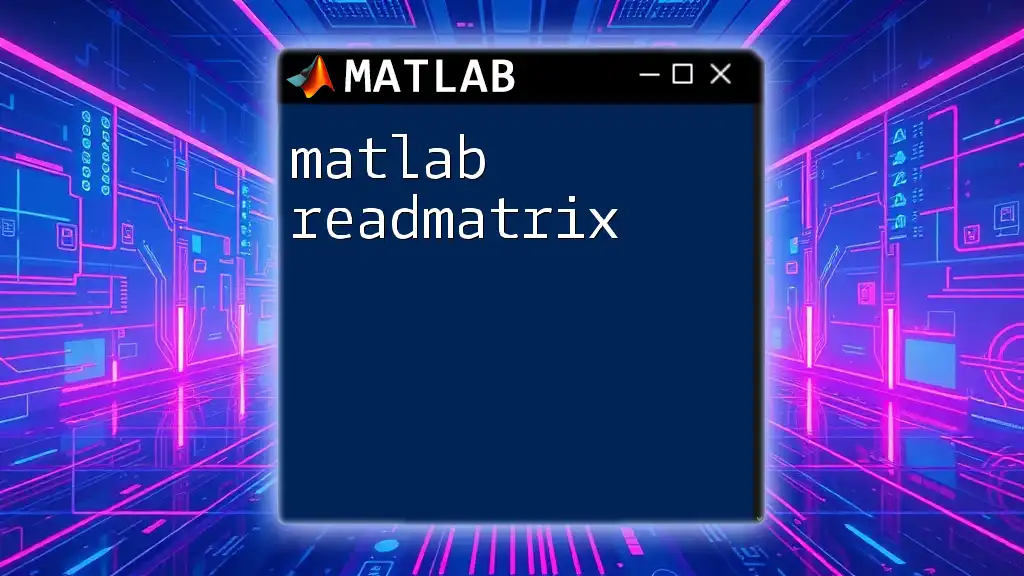
Further Reading and Resources
For those eager to deepen their knowledge, exploring the official MATLAB documentation offers invaluable insights. Additionally, engaging with online tutorials and courses can further enhance your skills.
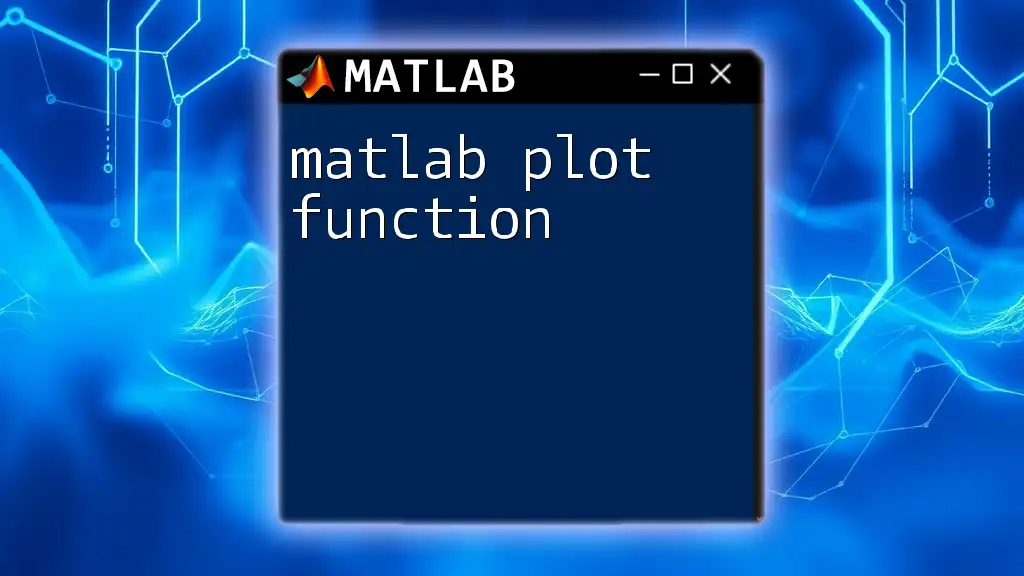
Call to Action
Stay tuned for more practical MATLab tutorials and tips by subscribing to our updates! Whether you’re a beginner or looking to refine your skills, our resources are tailored to help you master MATLAB efficiently.