In MATLAB, you can calculate the cube root of a number using the `nthroot` function or by raising the number to the power of `1/3`.
Here’s a simple example using both methods:
% Using nthroot function
cubeRoot1 = nthroot(27, 3);
% Using power operation
cubeRoot2 = 27^(1/3);
Getting Started with MATLAB
What is MATLAB?
MATLAB is a high-performance programming language and environment primarily designed for numerical computation, visualization, and programming. It provides an array of built-in functions that facilitate mathematical computations and complex data analysis. Mastering MATLAB enables users to perform a wide range of tasks seamlessly, from simple calculations to complex simulations.
Setting Up MATLAB
To harness the power of MATLAB in your calculations, ensure you have the software properly installed on your computer. Visit the official MathWorks website for installation guides. Upon launching MATLAB, familiarize yourself with the interface, which includes elements such as the Command Window, Workspace, Command History, and Editor. These tools will significantly enhance your experience while using MATLAB for computations.
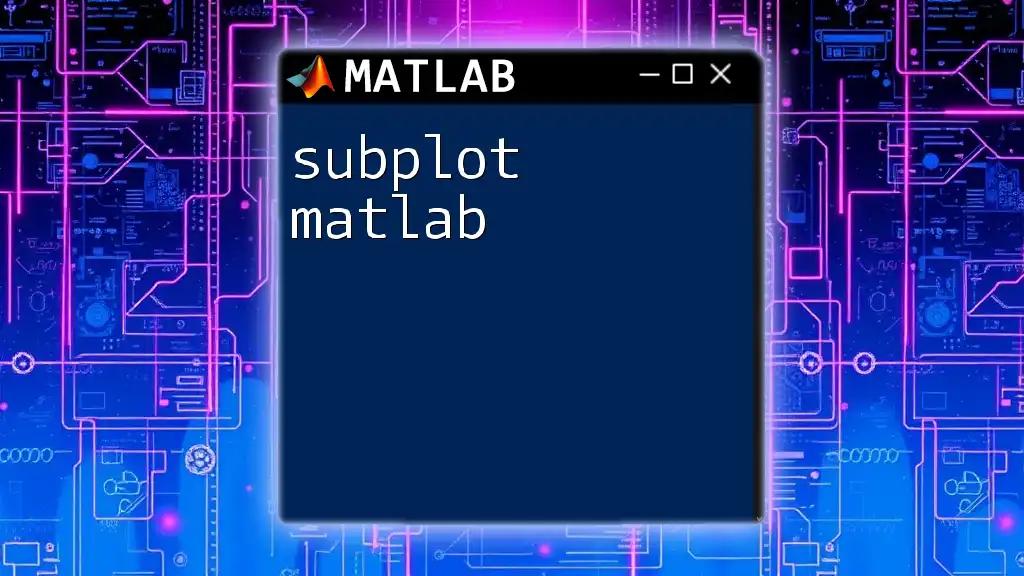
The Mathematical Background of Cube Roots
Definition of Cube Root
The cube root of a number \( x \) is a value \( y \) such that when \( y \) is multiplied by itself twice (i.e., \( y \times y \times y \)), it equals \( x \). In mathematical terms, this can be expressed as \( y = \sqrt[3]{x} \) or \( y^3 = x \). Understanding this concept is crucial for using the cube root function effectively in MATLAB.
Properties of Cube Roots
Cube roots exhibit unique characteristics that are essential for mathematical computations:
- The cube root of zero is zero, as \( 0^3 = 0 \).
- The cube root of a negative number results in a negative value. This is a differentiating factor from even roots (like square roots), which may yield complex results.
- Uniqueness: Every real number has exactly one real cube root, unlike square roots that have positive and negative roots.
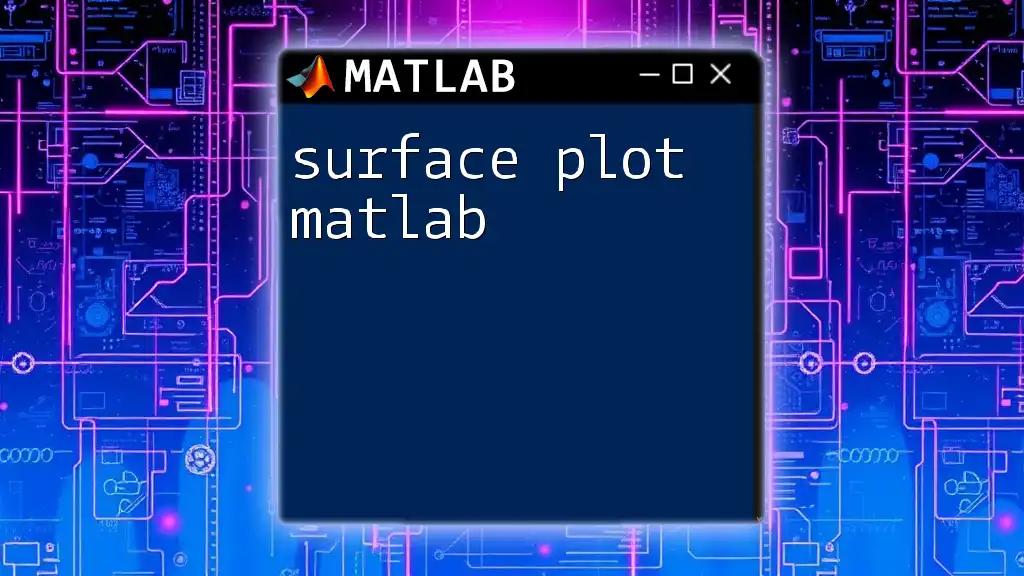
Using MATLAB to Calculate Cube Roots
Basic Command for Cube Root Calculation
To compute the cube root of a number in MATLAB, you can use the function `nthroot()`. The syntax for this function is:
y = nthroot(x, n)
Here, \( x \) is the number for which you want to find the cube root, and \( n \) is the root you wish to calculate—in this case, \( n \) is 3.
Example: Calculate the cube root of 27:
% Example: Calculate the cube root of 27
x = 27;
y = nthroot(x, 3);
fprintf('The cube root of %d is %.2f\n', x, y);
When you run this code, you will get the output showing that the cube root of 27 is 3.00.
Alternative Method: Power Operator
Another way to compute the cube root in MATLAB is by using the power operator `.^`. The syntax for finding the cube root using this method is as follows:
y = x^(1/3)
Example: Calculate the cube root of 64 using the power operator:
% Example: Calculate the cube root of 64 using power operator
x = 64;
y = x^(1/3);
fprintf('The cube root of %d is %.2f\n', x, y);
This command will output that the cube root of 64 is 4.00, demonstrating how the power operator can be an effective alternative for cube root calculations.
Handling Vectors and Matrices
MATLAB allows you to perform cube root calculations on arrays directly. The `nthroot()` function can handle vectors and matrices seamlessly.
Example: Calculate the cube roots for an array:
% Example: Cube roots of an array
x = [1, 8, 27, 64];
y = nthroot(x, 3);
disp('Cube roots of the array:');
disp(y);
The output will present the cube roots of the specified array elements, showcasing MATLAB’s capability to work with multiple numbers at once.
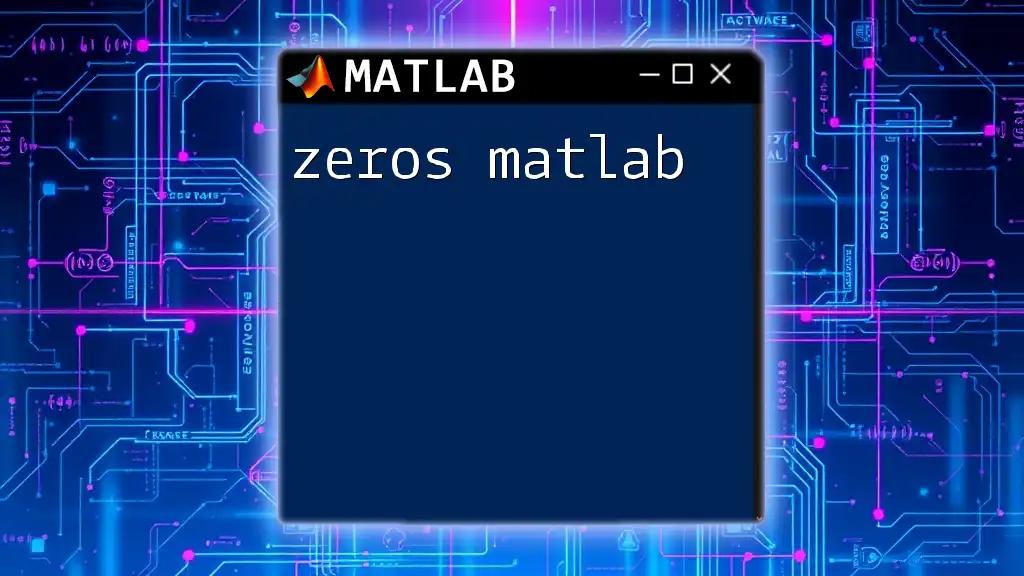
Understanding Results: Complex Numbers
Cube Roots of Negative Numbers
When dealing with negative inputs in MATLAB, it is crucial to understand how the program reacts. MATLAB can calculate cube roots for negative numbers seamlessly, resulting in a negative output, consistent with mathematical principles.
Example: Calculate the cube root of a negative number:
% Example: Cube root of a negative number
x = -27;
y = nthroot(x, 3);
fprintf('The cube root of %d is %.2f\n', x, y);
Here, the output will indicate that the cube root of -27 is -3.00.
Visualizing Cube Roots
Visual representation can greatly aid understanding. You can plot the cube root function using MATLAB for better clarity.
Example: Plotting the cube root function:
% Example: Plotting the cube root function
x = -10:0.1:10;
y = nthroot(x, 3);
plot(x, y);
title('Cube Root Function');
xlabel('x');
ylabel('Cube Root of x');
grid on;
This visualizes how the cube root function behaves across a range of values, helping you observe its properties in real-time.
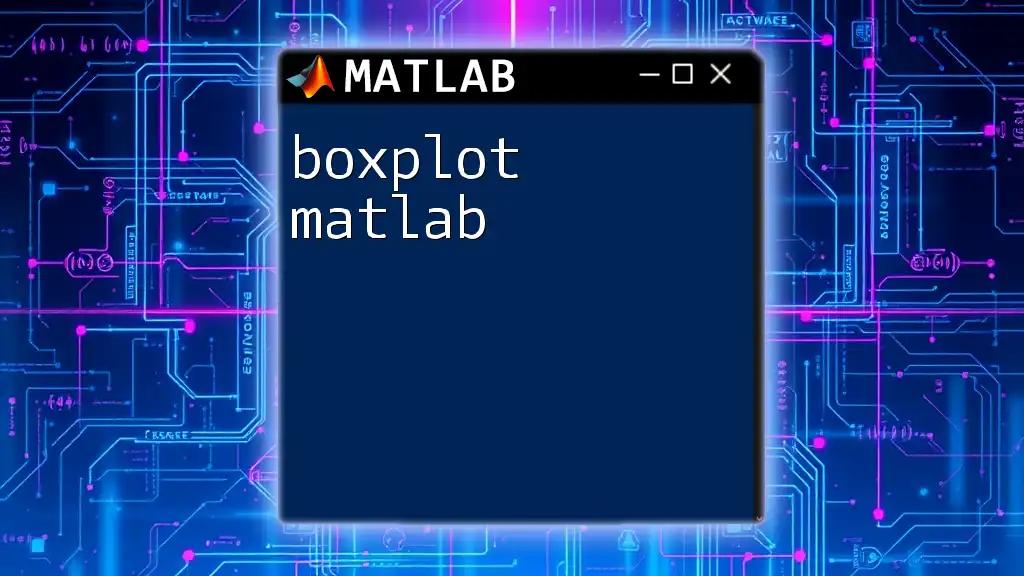
Practical Applications of Cube Roots in MATLAB
Engineering and Physics Applications
Cube roots frequently arise in engineering and physics, particularly in calculations involving volume. For instance, if you need to determine the side length of a cube given its volume, understanding and calculating the cube root is essential.
Data Analysis and Statistics
In the realm of data analysis, cube roots can be utilized to transform and normalize data, particularly in scenarios where skewness influences statistical analysis. This transformation can lead to more accurate models and interpretations of data.
Example: Using cube roots in data scaling:
% Example: Normalize data using cube root transformation
data = [1, 8, 27, 64];
normalized_data = nthroot(data, 3);
disp('Normalized data:');
disp(normalized_data);
This snippet demonstrates how you can apply cube roots to adjust your data for analytical purposes.
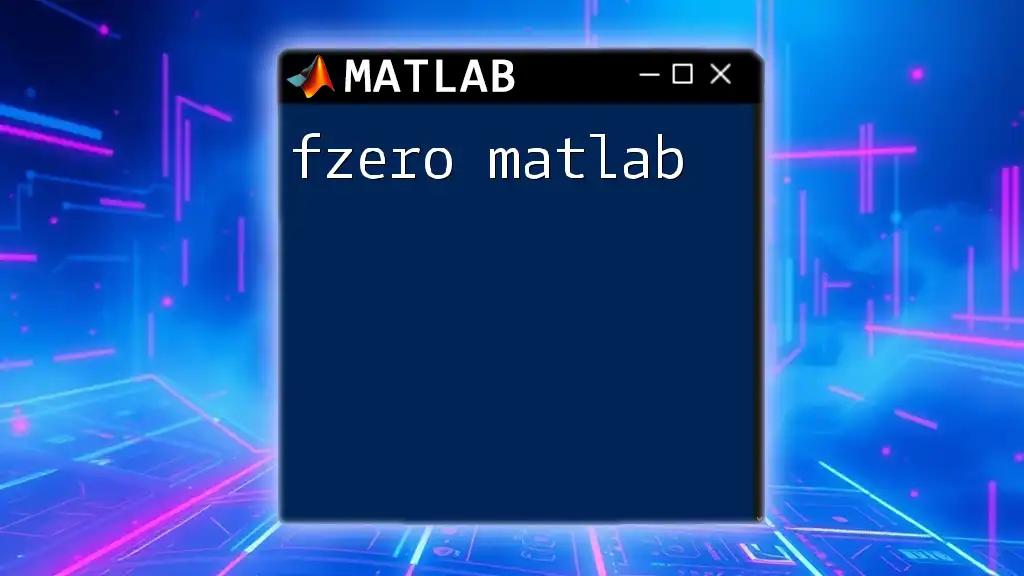
Troubleshooting Common Issues
Syntax Errors
When using MATLAB for cube root calculations, be mindful of common syntax pitfalls. Ensure that the `nthroot()` function is employed correctly, and avoid mixing types (like attempting to compute a cube root of a string).
Performance Considerations
In instances where you’re dealing with large datasets, the performance of your MATLAB code might decrease. Understanding the time complexity of cube root calculations is crucial. To optimize performance, consider using vectorization techniques and avoiding loops where possible.
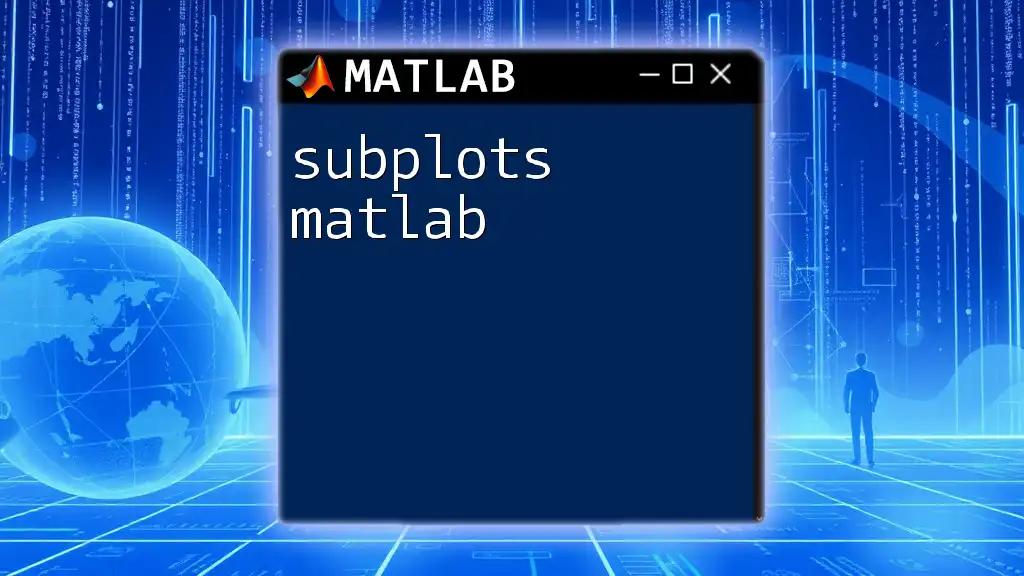
Conclusion
Understanding how to compute and utilize cube roots in MATLAB opens up a wealth of possibilities for solving a variety of mathematical problems. As you practice and explore more features of MATLAB, you will enhance your computational efficiency and accuracy. Challenge yourself with more complex cube root scenarios and take advantage of MATLAB’s powerful capabilities to solidify your grasp of this essential mathematical concept.
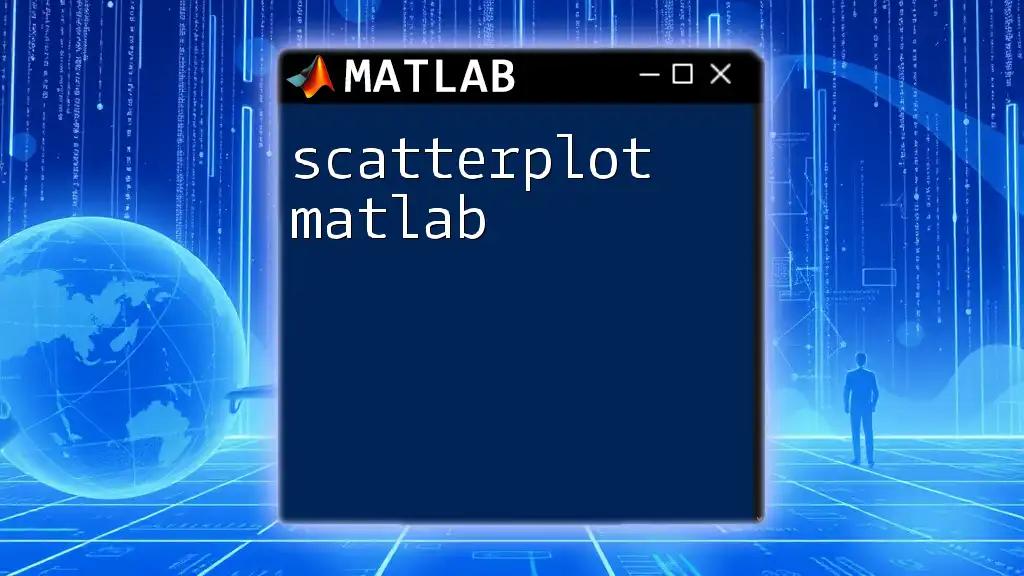
Additional Resources
For further exploration, check the official MATLAB documentation on the `nthroot()` function. Additionally, consider enrolling in online courses that cover MATLAB functionalities to deepen your understanding and application of these powerful tools.